实验四 多态性
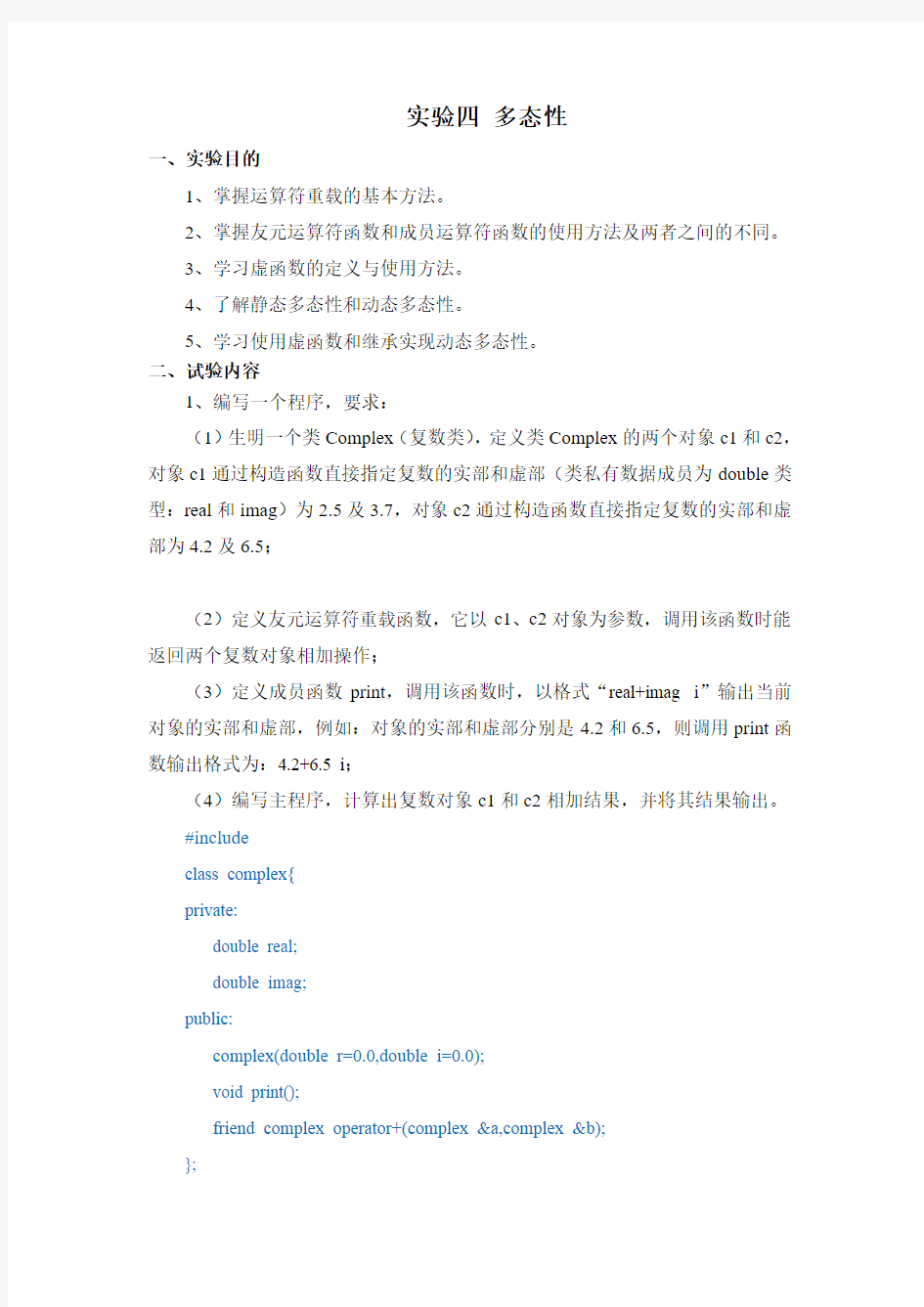
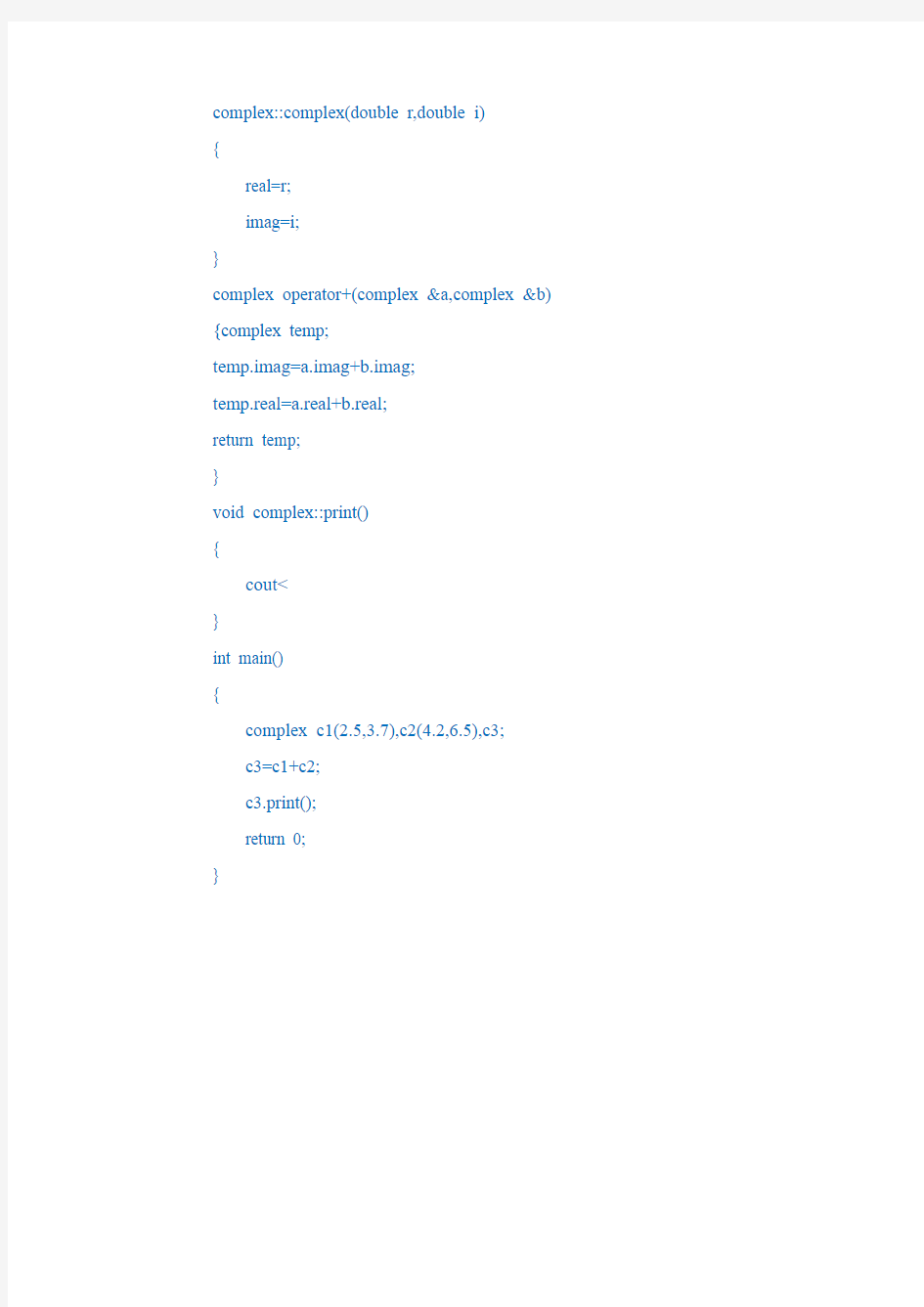
实验四多态性
一、实验目的
1、掌握运算符重载的基本方法。
2、掌握友元运算符函数和成员运算符函数的使用方法及两者之间的不同。
3、学习虚函数的定义与使用方法。
4、了解静态多态性和动态多态性。
5、学习使用虚函数和继承实现动态多态性。
二、试验内容
1、编写一个程序,要求:
(1)生明一个类Complex(复数类),定义类Complex的两个对象c1和c2,对象c1通过构造函数直接指定复数的实部和虚部(类私有数据成员为double类型:real和imag)为2.5及3.7,对象c2通过构造函数直接指定复数的实部和虚部为4.2及6.5;
(2)定义友元运算符重载函数,它以c1、c2对象为参数,调用该函数时能返回两个复数对象相加操作;
(3)定义成员函数print,调用该函数时,以格式“real+imag i”输出当前对象的实部和虚部,例如:对象的实部和虚部分别是4.2和6.5,则调用print函数输出格式为:4.2+6.5 i;
(4)编写主程序,计算出复数对象c1和c2相加结果,并将其结果输出。
#include
class complex{
private:
double real;
double imag;
public:
complex(double r=0.0,double i=0.0);
void print();
friend complex operator+(complex &a,complex &b);
};
complex::complex(double r,double i)
{
real=r;
imag=i;
}
complex operator+(complex &a,complex &b) {complex temp;
temp.imag=a.imag+b.imag;
temp.real=a.real+b.real;
return temp;
}
void complex::print()
{
cout< } int main() { complex c1(2.5,3.7),c2(4.2,6.5),c3; c3=c1+c2; c3.print(); return 0; } 2、编写一个程序,其中设计一个时间类Time,用来保存时、分、秒等私有数据成员,通过重载操作符“+”实现两个时间的相加。要求将小时范围限制在大于等于0,分钟范围限制在0~59分,秒钟范围限制在0~59秒。 提示:时间类Time的参考框架如下: class Time { public: Time(int h=0,int m=0,int s=0);//构造函数 Time operator+(Time &);//运算符重载函数,实现两个时间的相加 Time operator+();//运算符重载函数,实现两个时间的相加 void disptime();//显示时间函数 private: int hours,minutes,seconds; }; #include #include class Time { public: Time(int h=0,int m=0,int s=0);//构造函数 Time operator+(Time &);//运算符重载函数,实现两个时间的相加 Time operator+();//运算符重载函数,实现两个时间的相加void disptime();//显示时间函数 private: int hours,minutes,seconds; }; Time::Time(int h,int m,int s) { hours=h; minutes=m; seconds=s; } Time Time::operator +(Time &t1) { Time p; p.seconds=seconds+t1.seconds; int t=p.seconds; p.seconds=t%60; p.minutes=minutes+t1.minutes+t/60; int m=p.minutes; p.minutes=m%60; p.hours=hours+t1.hours+m/60; return p; } void Time::disptime() { cout< } int main() { Time t1(12,20,33),t2(5,17,58),t3; t3=t1+t2; cout<<"时间t1"< t1.disptime(); cout<<"时间t2"< t2.disptime(); cout<<"相加后的时间:"< t3.disptime(); return 0; } 3、写一个程序,定义抽象类Container: class Container { protected: double radius; public: Container(double r);//抽象类Container的构造函数 virtual double surface_area()=0;//纯虚函数surface_area virtual double volume()=0;//纯虚函数volume }; 【要求】 建立3个继承Container的派生类:Sphere(球体)、Cylinder(圆柱体)、Cube (正方体),让每一个派生类都包含虚函数surface_area()和volume(),分别用来球体、圆柱体和正方体的表面积和体积。要求写出主程序,应用C++的多态性,分别计算边长为6.0的正方体、半径为5.0的球体,以及半径为5.0和高为6.0的圆柱体的表面积和体积。 #include using namespace std; class Container{ protected: double radius; public: Container(double r);//抽象类Container 的构造函数 virtual double surface_area()=0;//纯虚函数surface_area virtual double volume()=0;//纯虚函数volume }; Container::Container(double r=0) { radius=r; } class Sphere:public Container { public: Sphere(double x):Container(x) { } double surface_area() { return 4*radius*radius*3.14; } double volume() { return 0.75*3.14*radius*radius*radius; } }; class Cylinder:public Container{ public: Cylinder(double h,double r):Container(r) { hight=h; } double surface_area() { return 2*3.14*radius*radius+2*3.14*radius*hight; } double volume() { return 3.14*radius*radius*hight; } protected: double hight; }; class Cube:public Container { public: Cube(double r):Container(r){ } double surface_area() { return 6*radius*radius; } double volume() { return radius*radius*radius;} }; int main() { Container *p; Cube a(6.0); p=&a; cout<<"正方体表面积:"< cout<<"正方体体积:"< Sphere b(5.0); p=&b; cout<<"球表面积:"< cout<<"球体积:"< Cylinder c(6.0,5.0); p=&c; cout<<"圆柱表面积:"< cout<<"圆柱体体积:"< return 0; } 4、设计一个点类Point,其结构如下: (1)Point类表示二维平面点的集合,数据成员由点的坐标值表示,类型为int;(2)三个重载构造函数: a)一个是无参数的构造函数; b)一个是带坐标值参数的构造函数,实现对数据成员的初始化; c)一个是copy构造函数,实现用一个对象初始化本对象; (3)两个重载成员函数: a)v oid offert(int , int );实现点的偏移,参数是偏移量; b)v oid offert(Point &);实现点的偏移,参数Point类对象是偏移量;(4)6个运算符重载函数: a)b ool operator = = (Point &);判断两个点对象是否相等; b)v oid operator + =(Point &);将两个点对象相加; c)v oid operator ++();将当前对象自增1(前缀); d)v oid operator ++(int );将当前对象自增1(后缀); e)f riend Point& operator + (Point &, Point &);将两个点对象相加; f)friend Point &operator ? (Point &, Point &);将两个点对象相减; (5)两个成员函数提供实例对象对私有数据的访问: a)i nt GetX(); b)i nt GetY(); (6)公有成员函数void Display();输出对象的数据成员;#include class Point{ private: int x; int y; public: Point() { x=0; y=0; } Point(int a,int b) { x=a; y=b; } Point(const Point &p) {x=p.x; y=p.y; } void offert(int,int); void offert(Point &); bool operator==(Point &); void operator+=(Point &); void operator++(); void operator++(int); friend Point& operator+(Point &, Point &); friend Point& operator-(Point &, Point &); void display(); int GetX() {return x;} int Gety() {return y;} }; void Point::offert(int a,int b) { x+=a; y+=b; } void Point::offert(Point &p) { x+=p.x; y+=p.y; } bool Point::operator==(Point &p) { if(x==p.x&&y==p.y) cout<<"=="< else cout<<"!="< return 0; } void Point::operator+=(Point &p) { x+=p.x; y+=y+p.y; } void Point::operator++() { x++; } void Point::operator++(int) { y++; } Point&operator+(Point &a,Point &b) { a.x=a.x+ b.x; a.y=a.y+ b.y; return a; } Point&operator-(Point &a,Point &b) { a.x=a.x- b.x; a.y=a.y- b.y; return a; } void Point::display() { cout<<'('< } int main() { Point a(2,2),b(4,5),c(3,1),d,e,f,g(5,3),h; cout<<"a坐标"; a.display(); cout<<"b坐标"; b.display(); cout<<"c坐标"; c.display(); cout<<"g坐标"; g.display(); cout<<"判断a b两点坐标是否相等\n"; if(a==b) cout<<"a=b"< else cout<<"a!=b"< cout<<"a b两个点对象相加:"< d=a+b; d.display(); cout<<"b c两个点对象相减:"< e=b-c; e.display(); c++; cout<<"c坐标后缀加1"< c.display(); ++g; cout<<"g坐标前缀加1"< g.display(); return 0; }