内部类详解 1、定义 一个类的定义放在另一个类的内部,这个类就叫做内部类
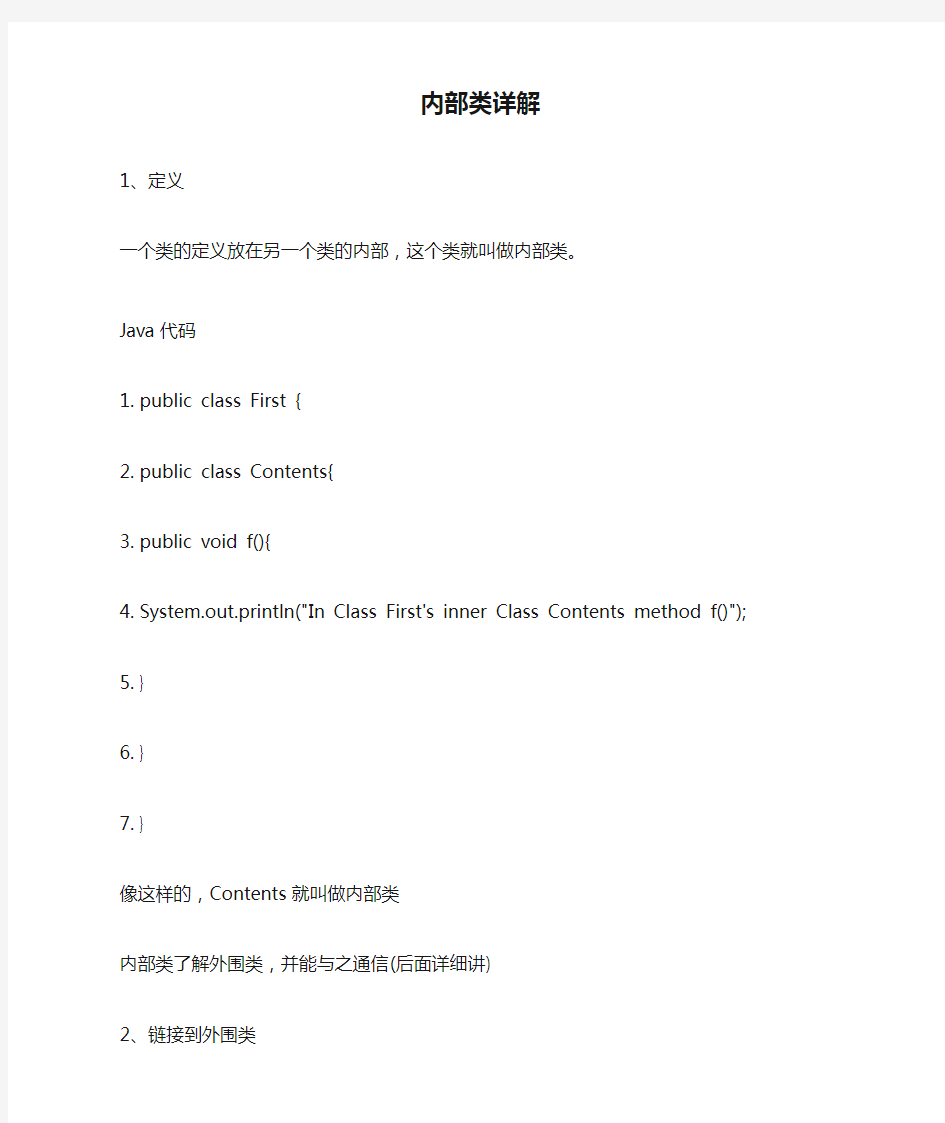

内部类详解
1、定义
一个类的定义放在另一个类的内部,这个类就叫做内部类。
Java代码
1.public class First {
2.public class Contents{
3. public void f(){
4. System.out.println("In Class First's inner Class Contents method f()")
;
5. }
6.}
7. }
像这样的,Contents就叫做内部类
内部类了解外围类,并能与之通信(后面详细讲)
2、链接到外围类
创建了内部类对象时,它会与创造它的外围对象有了某种联系,于是能访问外围类的所有成员,不需任何特殊条件。
Java代码
1. public class First {
2.public class Contents{
3. public void getStr(){
4. System.out.println("First.str="+str);
5. }
6.}
7.private String str;
8. }
9.
在内部类Contents中,可以使用外围类First的字段str。
那么,它是如何实现的呢?
是这样的,用外围类创建内部类对象时,此内部类对象会秘密的捕获一个指向外围类的引用,于是,可以通过这个引用来访问外围类的成员。
通常,这些都是编译器来处理,我们看不到,也不用关心这个。
正是因为如此,我们创建内部类对象时,必须与外围类对象相关联。
注:嵌套类(后面会讲到)除外。
3、使用关键字.this与.new
内部类中得到当前外围类对象的引用,可以使用.this关键字,注意与new的区别
Java代码
1. private int num ;
2.public Test2(){
3.
4.}
5.
6.public Test2(int num){
7. this.num = num;
8.}
9.
10.private class Inner{
11. public Test2 getTest2(){
12. return Test2.this;
13. }
14.
15. public Test2 newTest2(){
16. return new Test2();
17. }
18.}
19.
20.public static void main(String [] args){
21. Test2 test = new Test2(5);
22. Test2.Inner inner = test.new Inner();
23. Test2 test2 = inner.getTest2();
24. Test2 test3 = inner.newTest2();
25. System.out.println(test2.num);
26. System.out.println(test3.num);
27.}
28.
输出结果为5 0
使用.this后,得到时创建该内部类时使用的外围类对象的引用,new则是创建了一个新的引用。
.new关键字
如果想直接创建一个内部类对象,而不是通过外围类对象的方法来得到,可以使用.new关键字
形式是这样的:
Java代码
1.OutClass.InnerClass obj = outClassInstance.new InnerClass();
必须是外围类对象.new,而不能是外围类.new
Java代码
1. public class First {
2.public class Contents{
3. public void f(){
4. System.out.println("In Class First's inner Class Contents method f
()");
5. }
6. public void getStr(){
7. System.out.println("First.str="+str);
8. }
9.}
10.
11.public static void main(String [] args){
12. First first = new First();
13. First.Contents contents = first.new Contents();
14. contents.f();
15.}
16. }
17.
必须通过外围类First的对象first来创建一个内部类的对象
而且需要注意的是,在创建外围类对象之前,不可能创建内部类的对象(嵌套类除外)。4、内部类与向上转型
将内部类向上转型为基类型,尤其是接口时,内部类就有了用武之地。
Java代码
1. public interface Shape {
2.public void paint();
3. }
4. public class Painter {
5.
6. private class InnerShape implements Shape{
7. public void paint(){
8. System.out.println("painter paint() method");
9. }
10.}
11.
12.public Shape getShape(){
13. return new InnerShape();
14.}
15.
16. public static void main(String []args){
17. Painter painter = new Painter();
18. Shape shape = painter. getShape();
19. shape.paint();
20.}
21. }
22.
此时,内部类是private的,可以它的外围类Painter以外,没人能访问。
这样,private内部类给累的设计者提供了一种途径,通过这种方式可以完全阻止任何依赖于类型的编码,并完全隐藏实现的细节。
5、方法内的类
可以在方法内创建一个类。
Java代码
1.public void test(){
2.ass Inner{
3. public void method(){
4.ystem.out.println("在方法内创建的类");
5. }
6.
7.}
值得注意的是:方法内创建的类,不能加访问修饰符。
另外,方法内部的类也不是在调用方法时才会创建的,它们一样也被编译了(怎么知道的?后面会有讲解)。
6、匿名内部类
Java代码
1. public class Painter {
2.ublic Shape getShape(){
3.return new Shape(){
4. public void paint(){
5. System.out.println("painter paint() method");
6. }
7.};
8.
9. public static void main(String [] args){
10. Painter painter = new Painter();
11. Shape shape = painter.getShape();
12. shape.paint();
13. }
14. }
15. public interface Shape {
16.ublic void paint();
17. }
注意,匿名内部类后面的分号不可缺少!
匿名类,顾名思义,就是没有名称。
getShape()方法里,就使用了匿名内部类。
看上去很奇怪,不符合传统的写法?
第一眼看上去确实是这样的。
这样写,意思是创建了一个实现了Shape的匿名类的对象。
匿名类可以创建,接口,抽象类,与普通类的对象。创建接口时,必须实现接口中所有方法。
这是无参的,如果需要参数呢?
可以直接传。
Java代码
1. public class B {
2.public A getA(int num){
3. return new A(num){
4.
5. };
6.}
7. }
8. public class A {
9.private int num;
10.public A(int num){
11. this.num = num;
12.}
13.public A(){
14.
15.}
16. }
17.
Ok,在这个例子中,可以为A的构造方法传入一个参数。在匿名内部类中,并没有使用到这个参数。
如果使用到了这个参数,那么这个参数就必须是final的。
Java代码
1. public class B {
2.public A getA(final int num){
3. return new A(num){
4. public int getNum(){
5. return num;
6. }
7. };
8.}
9. }
10. public class A {
11.private int num;
12.public A(int num){
13. this.num = num;
14.}
15.public A(){
16.
17.}
18. }
19.
如果不是final的,编译器就会提示出错。
另外,还可以在匿名内部类里定义属性
由于类是匿名的,自然没有构造器,如果想模仿构造器,可以采用实例初始化({})
Java代码
1. public A getA(){
2.return new A(){
3. int num = 0;
4. String str;
5. {
6. str = "javaeye";
7. System.out.println("hello robbin");
8. }
9.};
10. }
11.
匿名内部类通过实例初始化,可以达到类似构造器的效果~
另外可以通过匿名内部类来改造工厂方法。
Java代码
1. public interface Service {
2.public void method1();
3. }
4. public interface ServiceFactory {
5.Service getService();
6. }
7. public class Implemention1 implements Service{
8.public void method1(){
9. System.out.println("In Implemention1 method method1()");
10.}
11.
12.public static ServiceFactory factory = new ServiceFactory(){
13. public Service getService(){
14. return new Implemention1();
15. }
16.};
17. }
18. public class Implemention2 implements Service {
19.public void method1(){
20. System.out.println("in Implemention2 method method1()");
21.}
22.
23.public static ServiceFactory factory = new ServiceFactory(){
24. public Service getService(){
25. return new Implemention2();
26. }
27.};
28.
29. }
30. public class Test {
31.public static void main(String []args){
32. service(Implemention1.factory);
33. service(Implemention2.factory);
34.
35. ServiceFactory factory1 = Implemention1.factory;
36. Service service1 = factory1.getService();
37. service1.method1();
38.
39. ServiceFactory factory2 = Implemention1.factory;
40. Service service2 = factory2.getService();
41. service2.method1();
42.}
43. }
在Implemention1和2中匿名内部类用在字段初始化地方。
7、嵌套类
static的内部类就叫做嵌套类
前面提到了很多次,嵌套类是个例外
使用嵌套类时有两点需要注意:
a、创建嵌套类对象时,不需要外围类
b、在嵌套类中,不能像普通内部类一样访问外围类的非static成员
Java代码
1. public class StaticClass {
2.private int num;
3.private static int sum = 2;
4.private static class StaticInnerClass{
5. public int getNum(){
6. //只能访问sum,不能访问num
7. return sum;
8. }
9.}
10. }
11. public class Test {
12.public static void main(String [] args){
13. //可以直接通过new来创建嵌套类对象
14. StaticClass.StaticInnerClass inner = new StaticClass.StaticInnerClass(
);
15. inner.getNum();
16.}
17. }
另外,嵌套类还有特殊之处,就是嵌套类中可以有static方法,static字段与嵌套类,而普通内部类中不能有这些。
8、内部类标识符
我们知道每个类会产生一个.class文件,文件名即为类名
同样,内部类也会产生这么一个.class文件,但是它的名称却不是内部类的类名,而是有着严格的限制:外围类的名字,加上$,再加上内部类名字。
前面说到得定义在方法内的内部类,不是在调用方法时生成,而是与外围类一同编译,就
可以通过查看.class文件的方式来证明。
9、为何要内部类?
a、内部类提供了某种进入外围类的窗户。
b、也是最吸引人的原因,每个内部类都能独立地继承一个接口,而无论外围类是否已经继承了某个接口。
因此,内部类使多重继承的解决方案变得更加完整。
在项目中,需要多重继承,如果是两个接口,那么好办,接口支持多重继承。
如果是两个类呢?这时只有使用内部类了。
Java代码
1. public interface One {
2.public void inOne();
3. }
4. public interface Two {
5.public void inTwo();
6. }
7. //两个接口,用普通类就可实现多重继承
8. public class CommonClass implements One,Two {
9.public void inOne(){
10. System.out.println("CommonClass inOne() method");
11.}
12.
13.public void inTwo(){
14. System.out.println("CommonClass inTwo() method");
15.}
16. }
17. public abstract class Three {
18.public abstract void inThree();
19. }
20. public abstract class Four {
21.public abstract void inFour();
22. }
23. //两个抽象类,使用普通类无法实现多重继承
24.
25. //使用内部类可以实现
26. public class Contents extends Three {
27.public void inThree(){
28. System.out.println("In Contents inThress() method");
29.}
30.
31.public class InnerFour extends Four{
32. public void inFour(){
33. System.out.println("In Contents");
34. }
35.
36.}
37. }
38.
另外,还有好多地方可以使用内部类。读过hibernate源代码的同学,应该可以发现,里面有好多内部类。
最常见的内部类,应该是Map.Entry了,可以看看源代码~
总结:
内部类的特性大致就是上述了,特性很直观,了解了之后,使用也很简单。
但是,何时使用我说的并不是很明确,因为本人知识有限,使用内部类也不是很多。项目中很少用,好像就是ActiveMQ那里用了一些
新版新概念英语第一册练习册附答案解析
第一节.单项填空,从A,B,C,D四个选项中选出可以填入空白处的最佳选项。(共计15小题,每小题1分,满分15分) 21.---Would you mind if I turned on the TV and watched CCTV news? ---___________. As a m atter of fact, I also like watching CCTV news. A. No, you can’t B. Yes, I do C. No, go ahead D. OK, no problem 22.She talked ______ she saw the accident. But in fact she only heard of it from others. A. so that B. as though C. even though D. once 23.The traffic in our county is very busy, for some main streets _______. A.have been rebuilt B.rebuilt C.are being rebuilt D.are rebuilding 24.Nobody knows what happened _____ her _____ the morning of May 1. A. for; in B . with; on C. to; on D. to; in 25.---I missed the first part of the film.It was really a pity. ---You ______home half an hour earlier. A.should go B.must have gone C.should leave D.should have left 26. The (H1N1) flu ________quickly in Lixin last month. A. spread B. spreads C. traveled D. travels 27. With so much homework _____, Tom has to stay at home. A. to do B. to be done C. done D. doing 28.---- Your daughter looks shy. ----After all, it is the first time that she ____ a speech to the public. A.had made B. has made C. is making D. makes 29.---- I hear Jane has gone to the Holy Island for her holiday. ---- Oh, how nice! Do you know when she ____? A. was leaving B. had left C. has left D. left
新概念英语第一册课文详解及英语语法2016
新概念第一册1-2课文详解及英语语法 课文详注Further notes on the text 1.Excuse me 对不起。 这是常用于表示道歉的客套话,相当于汉语中的“劳驾”、“对不起”。当我们要引起别人的注意、要打搅别人或打断别人的话时,通常都可使用这一表达方式。在课文中,男士为了吸引女士的注意而使用了这句客套话。它也可用在下列场合:向陌生人问路,借用他人的电话,从别人身边挤过,在宴席或会议中途要离开一会儿等等。 2.Yes?什么事? 课文中的Yes?应用升调朗读,意为:“什么事?”Yes?以升调表示某种不肯定或询问之意,也含有请对方说下去的意思。 3.Pardon?对不起,请再说一遍。 当我们没听清或没理解对方的话并希望对方能重复一遍时,就可以使用这一表达方式。较为正式的说法是: I beg your pardon. I beg your pardon? Pardon me. 它们在汉语中的意思相当于“对不起,请再说一遍”或者“对不起,请再说一遍好吗?” 4.Thank you very much.非常感谢! 这是一句表示感谢的用语,意为“非常感谢(你)”。请看下列类似的表达式,并注意其语气上的差异: Thank you. 谢谢(你)。Thanks! 谢谢! 5.数字1~10的英文写法 1—one 2—two 3—three 4—four 5—five 6—six 7—seven 8—eight 9—nine 10—ten 语法Grammar in use 一般疑问句 一般疑问句根据其结构又分为若干种。通过主谓倒装可将带有be的陈述句变为一般疑问句。即将be的适当形式移到主语之前,如:陈述句:This is your watch. 这是你的手表。 疑问句:Is this your watch? 这是你的手表吗? (可参见Lessons 15~16语法部分有关be的一般现在时形式的说明。) 词汇学习Word study 1.coat n. 上衣,外套:Is this your coat? 这是你的外套吗? coat and skirt<英>(上衣、裙子匹配的)西式女套装 2.dress n. (1)连衣裙;套裙:Is this your dress? 这是你的连衣裙吗? (2)服装;衣服:casual dress 便服;evening dress 晚礼服 新概念第一册3-4课文详解及英语语法 课文详注Further notes on the text 1.My coat and my umbrella please. 请把我的大衣和伞拿给我。 这是一个省略形式的祈使句,完整的句子应为: Give me my coat and my umbrella, please. 口语中,在语境明确的情况下通常可省略动词和间接宾语,如: (Show me your) Ticket, please. 请出示你的票。 (Show me your)Passport, please. 请出示您的护照。 2.Here's your umbrella and your coat. 这是您的伞和大衣。 Here's 是Here is的缩略形式。全句原为:Here is your umbrella and your coat.缩略形式和非缩略形式在英语的书面用语和口语中均有,但非缩略形式常用于比较正式的场合。Here's…是一种习惯用法,句中采用了倒装句式,即系动词提到了主语之前。又如Here is my ticket 这句话用正常的语序时为My ticket is here。 3.Sorry = I'm sorry。 这是口语中的缩略形式,通常在社交场合中用于表示对他人的歉意或某种程度的遗憾。 Sorry 和Excuse me 虽在汉语中都可作“对不起”讲,但sorry 常用于对自己所犯过失表示道歉,而Excuse me 则多为表示轻微歉意的客套语。 4.Sir,先生。 这是英语中对不相识的男子、年长者或上级的尊称。例如:在服务行业中,服务员对男顾客的称呼通常为sir: What can I do for you, sir? 先生,您要买什么? Thank you, sir. 谢谢您,先生。 sir 通常用于正式信函开头的称呼中: Dear sir 亲爱的先生 Dear sirs 亲爱的先生们/诸位先生们 Sir可用于有爵士称号者的名字或姓名之前(但不用于姓氏之前): Sir Winston Churchill 温斯顿·丘吉尔爵士 Sir William Brown 威廉·布朗爵士 5.数字11~15的英文写法 11—eleven 12—twelve 13—thirteen 14—fourteen 15—fifteen 语法Grammar in use 否定句 否定陈述句与肯定陈述句相反,它表示“否定”,并且含有一个如not 之类的否定词。一个内含be的否定形式的陈述句,应在其后加not,以构成否定句: 肯定句: This is my umbrella. 这是我的伞。 否定句: This is not my umbrella. 这不是我的伞。 请再看课文中的这两句话: 针对一般疑问句的否定的简略答语是No,it's not/it isn't。此处省略和非省略形式的关系为:is not =isn't;it is = it's。全句应为: No, it is not my umbrella. 不,它不是我的伞。 词汇学习Word study 1.suit n.(一套)衣服: Is this your suit? 这是你的衣服吗? a man's suit 一套男装;a woman's suit 一套女装 2.please:interjection (表示有礼貌地请求对方)请;烦劳: My coat and my umbrella please. 请把我的大衣和伞拿给我。 Please come in. 请进。
新概念英语第一册说课讲解
新概念英语第一册(1-144课)期末测试试卷 (1) 数词冠词介词动词时态变化比较级和最高级 一写出复数 1. radio 2. knife 3. glass 4. shelf 5. boss 6. dress 7. housewife 8.postman 9. leaf 10. church 11. mouth 12. family 13. tie 14. tomato 15. piano 16. baby 17. tooth 18. country 19. key 20 potato 某车间生产零件2000个,前3天生产240个零件。照这样这计算,一共需要多少天才能完成 21. match 22. box 23. hour 24. hero 二用冠词a an the 或some any 填空如果不需要则用/ 代替. 1. Alice is ____ air-hostess. Her father is ____ engineer and her mother is _____ housewife. They all play ______ tennis very well. 2. He has ____ uncle and his uncle lives in ____ United Kindom. He first saw him in ____ autumn of 1978. 7. We need _____ ink is there _____ left? 3. It is better to tell ______ truth than to tell _______ lies. 4. Will you have ______ more tea? There’s plenty in the pot. 5. There is ___university near my home. Every Saturday evening___ students hold ____ party. ___ are dancing ____ are singing. They make a lot of noise. 6. Get me ________ cigarettes please. ______ kind will do. 三用适当介词填空. 1. Can you see the words written ________ the blackboard? (in on by with)
新概念英语综合测试题
新概念英语综合测试题 班级:姓名: 听力部分 1.听音,选出你所听到的单词。10 1. ( ) A. wash B. wait C. shave D. sleep 2. ( ) A. June B. July C. September D. December 3. ( ) A. south B. north C. east D. west 4. ( ) A. season B. set C. summer D. snow 5. ( ) A. climate B. country C. chicken D. steak 6. ( ) A. mince B. meat C. sweet D. butter 7. ( ) A. bean B. pear C. pea D. peach 8. ( ) A. tomato B. potato C. pleasant D. spring 9. ( ) A. Spain B. Sweden C. Holland D. England 10( ) A. beer B. banana C. blackboard D. butter 2.为你所听到的单词选择正确的汉语意思。10 1. ( ) A. 甜的 B. 新鲜的 C. 上等的 D. 成熟的 2. ( ) A. 香蕉 B. 果酱 C. 苹果 D. 果酒 3. ( ) A. 饼干 B. 蛋糕 C. 水壶 D. 茶壶 4. ( ) A. 举起 B. 喜欢 C. 想 D. 展出 5. ( ) A. 后面 B. 找到 C. 沸腾 D. 当然 6. ( ) A. 面包 B. 咖啡 C. 肥皂 D. 烟丝 7. ( ) A. 前面 B. 后面 C. 左边 D. 右边 8. ( ) A. 花瓶 B. 茶壶 C. 盘子 D. 瓷器 9. ( ) A. 努力的 B. 工作 C. 书架 D. 锤子 10. ( ) A. 建筑物 B. 公园 C. 山谷 D. 村庄 3. 为你所听到的问题选择正确的答案。10 1. ( ) A. Yes, it is. B. Yes, she is. 2. ( ) A. I’m a keyboard operator. B. She’s my teacher. 3. ( ) A. I’m fine. B. She’s OK. 4. ( ) A. I’m blue. B. It’s blue.
新概念英语第一册课文知识讲解
新概念英语第一册课 文
$课文1 对不起! 1. Excuse me! 对不起 2. Yes? 什么事? 3. Is this your handbag? 这是您的手提包吗? 4. Pardon? 对不起,请再说一遍。 5. Is this your handbag? 这是您的手提包吗? 6. Yes, it is. 是的,是我的。 7. Thank you very much. 非常感谢! $课文3 对不起,先生。 8. My coat and my umbrella please. 请把我的大衣和伞拿给我。 9. Here is my ticket. 这是我(寄存东西)的牌子。 10. Thank you, sir. 谢谢,先生。 11. Number five. 是5号。 12. Here's your umbrella and your coat. 这是您的伞和大衣 13. This is not my umbrella. 这不是我的伞。 14. Sorry sir. 对不起,先生。 15. Is this your umbrella?
这把伞是您的吗? 16. No, it isn't. 不,不是! 17. Is this it? 这把是吗? 18. Yes, it is. 是,是这把 19. Thank you very much. 非常感谢。 $课文5 很高兴见到你。 20. Good morning. 早上好。 21. Good morning, Mr. Blake. 早上好,布莱克先生。 22. This is Miss Sophie Dupont. 这位是索菲娅.杜邦小姐。23. Sophie is a new student. 索菲娅是个新学生。 24. She is French. 她是法国人。 25. Sophie, this is Hans. 索菲娅,这位是汉斯。 26. He is German. 他是德国人。 27. Nice to meet you. 很高兴见到你。 28. And this is Naoko. 这位是直子。 29. She's Japanese. 她是日本人。 30. Nice to meet you.
新概念英语第一册95课课文
95课课文填空: 1.______ ______ _____ to ______,please. 2.______ ______ will _____ _____ _____ leave? 3.At ______ _______ ______ ______. 4.______ _______? 5.______ _______. 6.______ ______ ______. 7.______ ______ will the _____ _____ leave? 8._____ ______ ______. 9.We’ve _____ ______ ______ time. 10.It’s only ______ _______ to _______. 11.______ go and ______ ______ ______. 12.______ a _____ next ______ to the ______. 13.We ______ ______ go ______ to the _____ now, Ken. 14._______,please. 15.We _____ to ______ the ______ ______ to ______. 16.You’ve ______ _______ _____. 17.______! It’s _____ ______ ______. 18.I’m _____,sir. 19.That ______ ten ______ ______. 20.When’s the ______ ______? 21._____ five ______ ______! 95课课文填空: 1.______ ______ _____ to ______,please. 2.______ ______ will _____ _____ _____ leave? 3.At ______ _______ ______ ______. 4.______ _______? 5.______ _______. 6.______ ______ ______. 7.______ ______ will the _____ _____ leave? 8._____ ______ ______. 9.We’ve _____ ______ ______ time. 10.It’s only ______ _______ to _______. 11.______ go and ______ ______ ______. 12.______ a _____ next ______ to the ______. 13.We ______ ______ go ______ to the _____ now, Ken. 14._______,please. 15.We _____ to ______ the ______ ______ to ______. 16.You’ve ______ _______ _____. 17.______! It’s _____ ______ ______. 18.I’m _____,sir. 19.That ______ ten ______ ______. 20.When’s the ______ ______? 21._____ five ______ ______!
新概念英语第一册 解析
第一册新概念英语解析 Lesson 1 Excuse me! 对不起! 振振有“词” 1house,family和home house:房子,一般指独立的院落,更具体的指房子的建筑; family:侧重家庭的成员; home:抽象的家的概念。 说“文”解“字” 1Excuse me! 对不起。 通常在说或做可能令人不悦的事情之前或要吸引别人注意时使用。它的意思相当于中文里的“劳驾”,“对不起”,“打扰了”,“借光”,“请原谅”等等。 2Yes? 什么事? Yes后如果是问号,通常读升调,意思是“什么事?”“怎么了?”或者“干吗?”也可以表示谈话过程中期待对方说下去。 3Pardon? 原谅,请再说一遍。 口语中,当我们没有听清楚对方的话,希望对方再说一遍时就可以使用这一表达方式。较为正式的说法是:I beg your pardon? 或Pardon me? 它们在汉语中的意思相当于“对不起,请再说一遍。”或“对不起,请再说一遍好吗?”,读时一般用升调。 现身说“法” 人称代词:主格,宾格,形容词性物主代词和名词性物主代词 主格:一般作主语,放在句首; 宾格:动词或者介词之后,做宾语; 形容词性物主代词:具有形容词的修饰限定作用,不能单独使用,一般放在名词之前; 名词性物主代词:只能单独使用。 2be动词:am,is,are表示“是”,通常放在句子中做谓语,用于不同的人称。 is:用于单数名词或单数第三人称代词; am:只能跟在第一人称的单数I后面; are:搭配y ou,不管是单数还是复数。
3含有be动词的陈述句,否定句和一般疑问句 英语中句子通常分为四种类型:陈述句,疑问句,祈使句和感叹句。疑问句又被分为:一般疑问句,特殊疑问句,选择疑问句和反义疑问句。 含有be动词的任何句子,否定句就是在be动词(系动词)后面加“not”,如果变为一般疑问句就把be动词(系动词)提到句子的前面。 Lesson 3 Sorry, sir. 对不起,先生。 振振有“词” 1称呼语: Mr.:英语中对所有男性的普通称呼,一般放在姓名的前面或者只放在姓氏的前面,不能单独使用; Miss:小姐,对所有未婚女性的常用称呼,使用习惯与Mr.相同; Mrs.:夫人,对所有已婚女性的称呼,后面加上丈夫的姓氏,使用习惯与Mr.相同; Madam:女士,夫人,表示对女性的尊称,对有职位女性的称呼; sir :可以单独使用,是对上级,长着,或者陌生男子的尊称。sir后面不能加姓氏。 2sorry与excuse me的使用区别: excuse me通常用于说或者做可能令人不悦的事情之前使用;sorry是在说或者做这种事情之后使用,表示歉意。 3and:表示“和”,是连词,连接两个并列成分,位置可以互换。 说“文”解“字” 1My coat and my umbrella, please. 请把我的大衣和雨伞拿给我。 英语口语中,如果希望别人给自己“出示”什么东西时,通常是“要什么直接说什么”。 2No, it isn’t. 不,不是。 如果一般疑问句的主语是this,that,回答时主语通常改成it。 现身说“法” 1give sb sth 把某物给某人 sb.是somebody的缩写,表示“某人”;sth.是something的缩写,表示“某物”。 2否定句 陈述句通常分为两种类型,表示肯定意义的陈述句叫做肯定陈述句;表示否定意义的陈述句叫做否定陈述句。否定句与肯定句相反,它表示“否定”,并含有一个如not之类的否定词。一个含有be动词的陈述句,在其后面加not,以构成否定句。 3缩略形式常用在口语中,在正式的书面语中通常不缩写。 4代词的功能 在英语中,如果同一个名词在句子中再次出现的时候,我们通常用相应的代词将其代替,以避免同一个名词的反复使用。 5副词 副词分为:时间副词,地点副词,程度副词,频率副词和方式副词。英语中,时间副词和地点副词前不能加介词,方式
新概念英语第一册练习题完美打印打印版
新概念英语第一册练习题(一) Lesson 1 Excuse me! 请大家将你的答案写在后面吧 Lesson 1 Excuse me! 对不起! A About you Copy this dialogue. Add your own name at the end. 抄写这段对话,. 在结尾处加上你的名字。Sue: Excuse me. ______ John: Yes ______ Sue: What's your name ______ John: Pardon ______ Sue: What's your name ______ John: My name is John. ______ Sue: What's your name ______ You: My name is...... ______ B Vocabulary: Write the correct words in the questions.. 用正确的词完成以下问句。 book car coat dress house√. pen pencil shirt wathc 1 Is this your h______ 6 Is this your c______ 2 Is this your w______ 7 Is this your c______ 3 Is this your sh ______ 8 Is this your d______ 4 Is this your b______ 9 Is this your p______ 5 Is this your p______ 10 Is this your s ______ C Numbers: Write the numbers in figures.. 用阿拉伯数字表示以下数词。 three 3 ten______ one______ four______ six______ five______ eight_________ seven______ two______ nine______ 新概念英语第一册练习题(二) Lesson 2 Is this your.... 这是你的....吗? A Structure Write questions with the words.用所给的词写出问句。 Handbag Is this your handbag? 1 book ______ 2 car ______ 3 coat ______ 4 dress ______ 5 house ______ 6 pen ______ 7 pencil ______ 8 shirt ______ 9 skirt ______ 10 watch ______
新概念英语第一册课文详解及英语语法21-22
新概念英语第一册课文详解及英语语法21-22 课文详注 Further notes on the text 1.Give me a book please, Jane.请拿本书给我,简。 这是一个祈使句。祈使句表示请求或命令。(请参见Lessons 13~14语法部分的说明。)表示客气的请求时,通常加please。 2.Which book? 哪一本? 是Which book do you want?的省略形式。下文中的This one? 是Do you want this one?的省略形式。No, not that one是No, I do not want that one的省略形式。口语中常用这样的省略句。 3.This one?是这本吗? 相当于:Do you want this one? one是不定代词,代替 a book,以避免重复。one的复数形式是ones。one和ones前面都可用定冠词,也可有自己的定语。 4.数字1,010,1,011,1,016的英文写法 1,010----a thousand and ten; 1,011----a thousand and eleven; 1,016----a thousand and sixteen 语法 Grammar in use 1.人称代词 代词,顾名思义,就是用来代替名词或名词短语的词,在已经知 道所指的是谁或什么的情况下使用,以免行文重复。人称代词有主格 和宾格之分。在陈述句中,主格代词差不多总是位于动词之前。宾格 代词可代替处于宾语位置上的名词,它们能够作直接宾语和间接宾语。 Give me/him/her/us/them a book. 给我/他/她/我们/他(她) 们一本书。(宾格代词)
学霸英语新概念第三册语法详解和总结(带习题和答案)
学霸英语新概念第三册语法详解和总结(带习题和答案) 第一章英语从句 Subordination 英语从句主要有定语从句,状语从句和名词性从句(主语从句,宾语从句,表语从句,同位语从句) 一.定语从句 定语从句:由关系代词who, whom, whose, that, which; 关系副词when, where, why 引导。 (下面十个句子请读5遍并脱口译出!) 1. The death notices tell us about people who have died during the week. 2. The man (whom) you spoke to just now is my friend. 3. The building whose lights are on is beautiful. 4. Please find a place which we can have a private talk in. 5. The knee is the joint where the thighbone meets the large bone of the lower leg. 6. He still remembers the day when he went to school. 7. It is no need telling us the reason why you didn't finish it in time. 8. He has three sons, two of whom died in the war. 9. Mr. Smith, whose wife is a clerk, teaches us English. 10. In the Sunday paper there are comics, which children enjoy. 只能用that和who引导的定语从句 A.all, nothing, anything, a few, one做先行词指物时 B.先行词前有形容词最高级修饰时,后面常跟that而不是which. C.先行词前有the only, the first, the last, the next, the very等词修饰时,引导词只能用that。 D.当先行词是anyone, anybody, everyone, everybody, someone, somebody时,后面要用who或whom; ●All that glitters is not gold. 闪光的并非都是金子。 as 引导定语从句 as 引导的定语从句有两种形式: A.引导限制性语从句。 在此类定语从句中,as常与主语中作为其先行词的such, the same或as联用构成,“such... as”,“the same... as”和“as ...as”句型,可代替先行词。 例如:We hope to get such a tool as he is using. 我们希望得到他正在用的那种工具。 B.as 引导非限制性定语从句时,作用与which相同,as作为关系代词代替整个主句。 (这是语法考试的一个考点。) [注意区别]: as 引导的从句用于句首、句中或句后,而which引导的定语从句不能放在句首。 例如:As is reported, a foreign delegation will visit the city. 据报道,一个外国代表团将访问这个城市。 二.状语从句 超级作文联接词及词组,全部拿下! ①原因:because, since, now that(既然)as, for, this reason.... ②结果:so that, so, therefore, consequently, so as to, as a result .... ③时间:after, before, when, while, as, until, as soon as, since, by the time, once, lately, presently, shortly after, currently, at present, nowadays ... ④条件:if, only if., once, unless, in the event (that), in case (that), provided that, on the condition that, etc.
新概念英语第一册课文详解及英语语法1-2
新概念英语第一册课文详解及英语语法1-2课文详注 Further notes on the text 1.Excuse me 对不起。 这是常用于表示道歉的客套话,相当于汉语中的“劳驾”、“对不起”。当我们要引起别人的注意、要打搅别人或打断别人的话时,通常都可使用这个表达方式。在课文中,男士为了吸引女士的注意而使用了这句客套话。它也可用在下列场合:向陌生人问路,借用他人的电话,从别人身边挤过,在宴席或会议中途要离开一会儿等等。 2.Yes?什么事? 课文中的 Yes?应用升调朗读,意为:“什么事?”Yes?以升调表示某种不肯定或询问之意,也含有请对方说下去的意思。 3.Pardon?对不起,请再说一遍。 当我们没听清或没理解对方的话并希望对方能重复一遍时,就能够使用这个表达方式。较为正式的说法是: I beg your pardon. I beg your pardon? Pardon me. 它们在汉语中的意思相当于“对不起,请再说一遍”或者“对不起,请再说一遍好吗?” 4.Thank you very much.非常感谢! 这是一句表示感谢的用语,意为“非常感谢(你)”。请看下列类似的表达式,并注意其语气上的差异: Thank you. 谢谢(你)。 Thanks! 谢谢! 5.数字1~10的英文写法 1—one 2—two 3—three 4—four 5—five
6—six 7—seven 8—eight 9—nine 10—ten 语法 Grammar in use 一般疑问句 一般疑问句根据其结构又分为若干种。通过主谓倒装可将带有be 的陈述句变为一般疑问句。即将be的适当形式移到主语之前,如:陈述句:This is your watch. 这是你的手表。 疑问句:Is this your watch? 这是你的手表吗? (可参见 Lessons 15~16语法部分相关 be的一般现在时形式的 说明。) 词汇学习 Word study 1.coat n. 上衣,外套: Is this your coat? 这是你的外套吗? coat and skirt(上衣、裙子匹配的)西式女套装 2.dress n. (1)连衣裙;套裙: Is this your dress? 这是你的连衣裙吗? (2)服装;衣服: casual dress 便服; evening dress 晚礼 服
新概念英语第二册:第1课课文详解及语法解析
新概念英语第二册:第1课课文详解及语法解析 课文详注 Further notes on the text https://www.360docs.net/doc/886718691.html,st week I went to the theatre. 上星期我去看戏。 (1)句首的“Last week”点明叙述的事情发生的时间是上星期。 所以整篇课文的时态基本上应是过去时(包括过去实行时),直接引语 部分的时态除外。 (2)动词go的原义是离开一个地方去另一个地方,与介词to连 用后,常加上主语所要去的目的地来代表主语的动作目的。 课文中go to the theatre = go to the theatre to see a play,即去剧场看戏。类似的还有go to the cinema = go to the cinema to see a film(去电影院看电影)。这种表达方式简明扼要。 请注意在以下的短语中名词前通常不加冠词: go to school 上学 go to bed 上床睡觉 go to church 上教堂,去做礼拜 (cf.第1册第68课at school, at church;第1册第85课have been to school/church) 2.had a very good seat 座位很好 seat一般指戏院、汽车等配置的固定座位,也能够抽象地表示“座位”或“位子”的概念: the front seat of a car 汽车的前座 Take a seat, please. 请坐。 3.The play was very interesting. 戏很有意思。
interesting属于现在分词形式的形容词,意思是“使人感兴趣”。 它通常与非人称主语连用或修饰某个事物: This is an interesting book/idea. 这是一本有趣的书/一个令人感兴趣的主意。 4.…were sitting behind me. They were talking loudly. ……坐在我的身后,大声地说着话。 这两句的时态为过去实行时。(cf.第7课语法) 5.I got very angry. 我变得非常生气。 get在这里有“逐渐变得”的含义,接近become,是个表示过程的动词,表示状态的变化。而I was very angry则仅表示当时的状态是生气,并不暗示过程。 6.in the end 最后,终于 表示一段较长的时间之后或某种努力之后: She tried hard to finish her homework by herself. In the end, she had to ask her brother for help. 她试图自已完成家庭作业,但最后她不得不请她兄弟帮忙。 7.none of your business 不关你的事 (1) sb. 's business 某人(所关心的或份内)的事 It is my business to look after your health. 我必须照顾你的身体健康。 This is none of his business.
新概念英语第1册第95-96课重点语法
新概念英语第1册第95-96课重点语法 第95-96课的内容: 一、重要句型或语法 1、一般将来时 本课侧重的是表将来的时间状语:in+一段时间+'s+time;其中, 如果一段时间是以s结尾的复数名词,则要省略's中的s。如: When's the next train? In five hours' time. 2、准确时刻的表达 本课侧重的是past和to用来表示时刻的用法。 提问:What's the exact time? 回答:It's twenty minutes past four. / It's five minutes to nine. 二、课文主要语言点 Two return tickets to London, please. 1)..., please.,用 在购物时,省略号部分就是所要购买的东西。 2)return ticket,往 返票。也就是说,如果要从上海到北京,提前把从北京回到上海的票 一起买了。 What time will the next train leave? At nineteen minutes past eight. 1)what time用来提问几点钟,when用来提问什么时候。 2)时刻前要用介词at,几点过几分要用“分钟+past+小时”的结构。 Which platform? Platform Two. Over the bridge. 1)platform,站台。 2)当表示几号站台时,platform要放前面,且首字母要大写。 3)over the bridge,意思是指要走过天桥才能达到二 号站台。
What time will the next train leave? At eight nineteen. 几点几分也能够直接表达为:小时+分钟。 We've got plenty of time. 1)have got,相当于have,表示“有”。 2)plenty of,很多,一般后接不可数名词。 It's only three minutes to eight. 几点差几分的表达为:所 差分钟+to+小时。 Let's go and have a drink. 1)Let's do sth.,表示“让我们 干什么事情”。注意Let's后面要用动词原形。 2)have a drink,喝 点东西。 There's a bar next door to the station. next door to,靠近、邻门。 We had better go back to the station now, Ken. 1)had better,属于情态动词范畴,后面要紧跟动词原形;否定形式直接在better后面加not;口语中had经常省略。 2)go back to,回到。 We want to catch the eight nineteen to London 1)catch, 赶上。 2)句中的the eight nineteen用来代指表示八点十九分的火车。 You've just missed it! miss,错过。 What! It's only eight fifteen. What!,常用来表示惊讶或感叹。 I'm sorry, sir. That clock's ten minutes slow. 时间+slow,表示钟慢了多长时间。 When's the next train? In five hours' time! 注意将来时间 状语的表达:in+一段时间+'s+time。 三、双课补充内容
新概念英语第二册第二十课课后习题答案详解 Lesson 20
新概念英语第二册第二十课课后习题答案详解 Lesson 20 1. b根据课文第4行 I never catch anything---not even old boots, 只有 b. nevercatches anything 与课文实际内容相同,而其他3个选择都与课文内容不符。 2.c根据课文最后一句I am only interested in sitting in a boat and doing nothingat all! 只有c. doing nothing 是正确答案,而其他3个选择都不符合课文内容。 3.b 本句是对主语提问的,回答是Fishing.a. who (谁)是对主语提问的;c. whom(谁)是对宾语提问的;d. whose(谁的)确是对定语提问的;只有b. which 是对做主语的事物提问的,所以选b. 4.b本句是带never的否定句,只有b. anything 可以用在否定句中。而a. nothing若用在否定句中,就会使原句变成肯定意义的句子,不符合题目意思;c. something 只能用于肯定句中;d. everything也不能用在否定句中。 5.c前面句子 I am even less lucky 中的 less(不及)是表示否定意义的比较级,只有c. not so是表示否定意义的,而其他3个选择都没有否定的意思. 6.b只有b. an empty bag 最符合语法。而其他3个选择都有语法错误。 a. a empty bag 中empty是以元音开头的,前面不能用a 而应该用an; c. empty bag 中 bag 是可数名词,需要用an 来修饰; d. oneempty bag 不符合习惯用法,单数可数名词前通常用不