基于java的ATM模拟程序
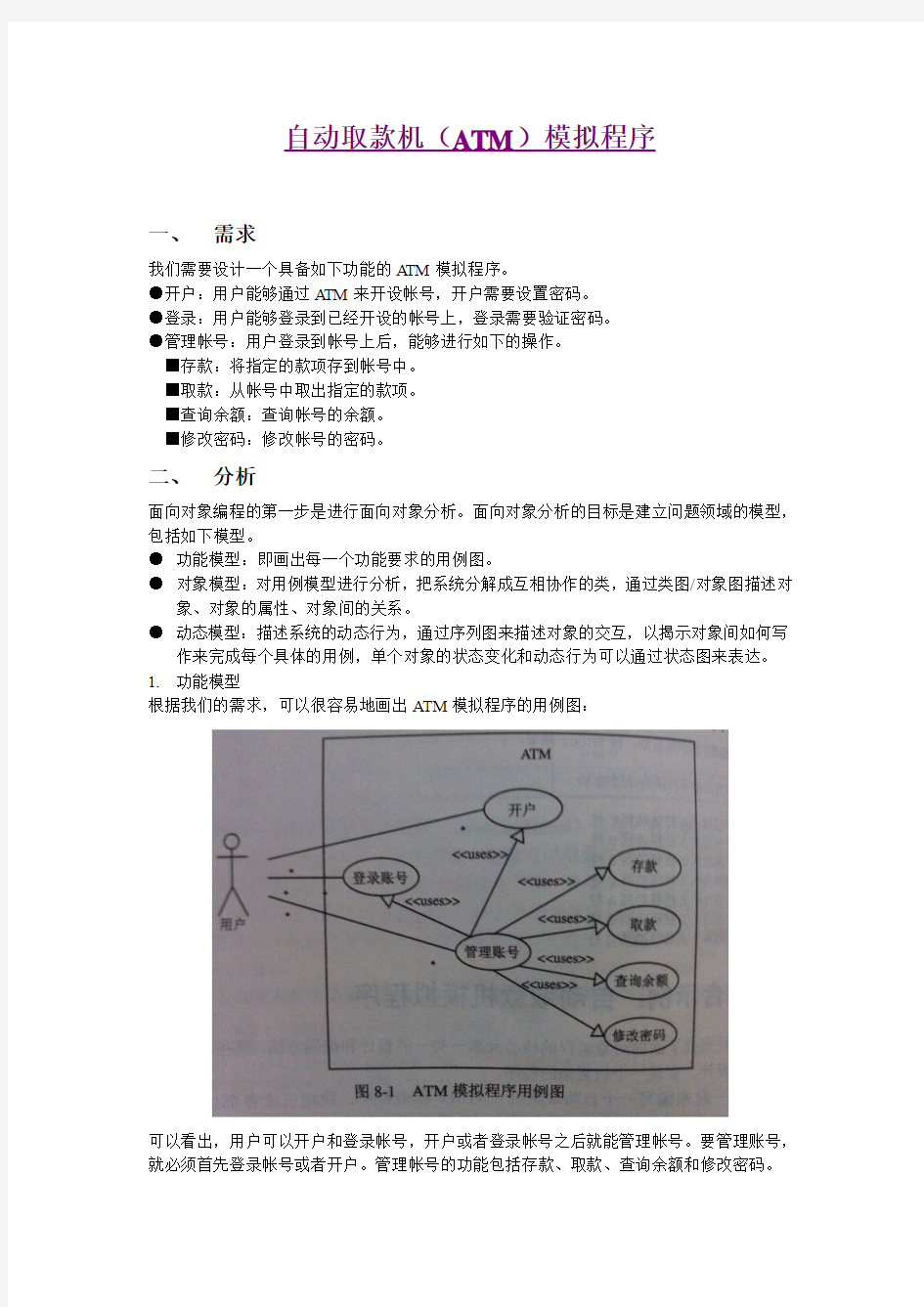

自动取款机(ATM)模拟程序
一、需求
我们需要设计一个具备如下功能的ATM模拟程序。
●开户:用户能够通过ATM来开设帐号,开户需要设置密码。
●登录:用户能够登录到已经开设的帐号上,登录需要验证密码。
●管理帐号:用户登录到帐号上后,能够进行如下的操作。
■存款:将指定的款项存到帐号中。
■取款:从帐号中取出指定的款项。
■查询余额:查询帐号的余额。
■修改密码:修改帐号的密码。
二、分析
面向对象编程的第一步是进行面向对象分析。面向对象分析的目标是建立问题领域的模型,包括如下模型。
●功能模型:即画出每一个功能要求的用例图。
●对象模型:对用例模型进行分析,把系统分解成互相协作的类,通过类图/对象图描述对
象、对象的属性、对象间的关系。
●动态模型:描述系统的动态行为,通过序列图来描述对象的交互,以揭示对象间如何写
作来完成每个具体的用例,单个对象的状态变化和动态行为可以通过状态图来表达。1.功能模型
根据我们的需求,可以很容易地画出A TM模拟程序的用例图:
可以看出,用户可以开户和登录帐号,开户或者登录帐号之后就能管理帐号。要管理账号,就必须首先登录帐号或者开户。管理帐号的功能包括存款、取款、查询余额和修改密码。
2.对象模型
ATM是银行相连的设备,因此银行是A TM模拟程序的相关对象,所有的操作都是针对帐号的,因此帐号是其中的核心对象。此外,需要一个程序来启动ATM。因此,在ATM模拟程序中,我们需要处理四个类,或者说四类对象:启动程序、A TM、银行及帐号。
1)帐号类Account
帐号类Account包含所有的帐号信息负责所有的帐号操作。基本的帐号信息包括:
●帐号名name
●帐号密码password
●帐号余额balance
主要的帐号操作包括:
●登录Login
●存款Deposit
●取款Withdraw
●查询余额Get_Balance
●修改密码ChangePassword
2)银行类Bank
银行类Bank的本质就是一组帐号的组合,并负责管理帐号。基本的银行信息包括:
●银行名name
●已经开户的帐号数usedAccountNum
●可以容纳的最大账户数MaxAccountNum
●帐号集accounts
主要的银行操作包括:
●开户OpenAccount
●登录帐号LoginAccount
●撤销帐号,但是由于A TM不能撤销帐号,因此我们不关心这个操作
3)ATM类
ATM类与银行类之间在一对一的关联关系,ATM提供用户界面,并将用户的请求提交给银行、将银行的反馈提交给用户。基本的ATM信息包括:
●银行Bank
主要的ATM操作包括:
●启动Start
●开户OpenAccount
●登录帐号LoginAccount
●管理帐号ManageAccount
●一些显示不同信息的辅助操作(PrintLogo、Print、Pause等)。
4)启动程序类Program
启动程序类Program的唯一功能就是创建银行类和ATM类的实例,并将它们关联起来,然后启动ATM(执行A TM对象的Start方法)。因此,这个类仅仅包含程序的入口点Main方法。
5)ATM模拟程序的类图
根据上述各类的分析,可以画出A TM模拟程序的类图:
注意:Bank类和Account类的数据成员都使用protected访问模式,主要是为了使它们的之类都能集成它们的这些数据成员。
3.动态模型
ATM模拟程序的基本工作流程:
三、设计
经过分析,我们已经了解了A TM模拟程序中的四个类的基本结构。在设计阶段需要进一步细化个各类的结构。
对Account类,我们将进行如下的调整:
●用只读属性来实现Get_Balance方法
●增加赌气name的只读属性。
●增加构造函数。
●重载Deposit方法,并提供三个重载方法。
●重载Withdraw方法,并提供三个重载方法。
对Bank类,主要调整包括:
●提供构造函数,传入银行的名称。
●增加读取name的只读属性。
●用数组来实现帐号集。
●用常量来表示MaxAccountNum
对ATM类,主要增加几个与用户交互的函数(打印信息和输入信息),以及一个退出系统的密码。
在设计类的时候,为了提供代码的重用性,一个重要的原则是,不要将功能与用户界面紧密耦合在一起。在这个程序中,Account类和Bank类是功能类,A TM类是用户界面类。因此,在设计Account类和Bank类时候,不要涉及任何的用户界面操作。所有的用户界面都放在ATM类中。这样做的好处是:功能类可以适用于任何的用户界面,比如,Bank类和Account
可以用于网上银行,用户界面类可以适用于其他功能,比如,ATM类可以用于信用卡机构。
四、实现
1)在ATM项目中增加Account类
using System;
using System.Collections.Generic;
using System.Text;
//Account.cs
//ATM项目中的Account类源文件
namespace ATM
{
class Account
{
protected string name;
protected string password;
protected decimal balance;
public decimal Banlance
{
get
{
return balance;
}
}
public string Name
{
get
{
return name;
}
}
public Account(string name,string password) {
this.balance = 0;
https://www.360docs.net/doc/a617358677.html, = name;
this.password = password;
}
public bool Deposit(decimal amount)
{
if(amount<=0)
{
return false;
}
balance += amount;
return true;
}
public bool Deposit(double amount)
{
return Deposit((decimal)amount);
}
public bool Deposit(int amount)
{
return Deposit((decimal)amount);
}
public bool Deposit(decimal amount,out decimal balance)
{
bool succeed = Deposit(amount);
balance = this.balance;
return succeed;
}
public bool Withdraw(decimal amount)
{
if (amount > balance || amount <= 0)
{
return false;
}
balance -= amount;
return true;
}
public bool Withdraw(double amount)
{
return Withdraw((decimal)amount);
}
public bool Withdraw(int amount)
{
return Withdraw((decimal)amount);
}
public bool Withdraw(decimal amount, out decimal balance)
{
bool succeed = Withdraw(amount);
balance = this.balance;
return succeed;
}
public bool ChangePassword(string oldPassword, string newPassword) {
if(oldPassword!=password)
{
return false;
}
password = newPassword;
return true;
}
public bool Login(string name,string password)
{
return (https://www.360docs.net/doc/a617358677.html,==name&&this.password==password);
}
}
}
2)在ATM项目中增加Bank类
using System;
using System.Collections.Generic;
using System.Text;
//Bank.cs
//ATM项目中的Bank类源文件
namespace ATM
{
class Bank
{
protected string name;
protected const int MaxAccountNum = 2048;
protected int usedAccountNum;
protected Account[] accounts;
public string Name
{
get
{
return name;
}
}
public Bank(string name)
{
https://www.360docs.net/doc/a617358677.html, = name;
https://www.360docs.net/doc/a617358677.html,edAccountNum = 0;
accounts=new Account[MaxAccountNum];
}
public bool LoginAccount(string name,string password,out Account account) {
account = null;
for (int i = 0; i < usedAccountNum;++i )
{
if(accounts[i].Login(name,password))
{
account=accounts[i];
return true;
}
}
return false;
}
public bool OpenAccount(string name, string password, out Account account) {
account = null;
for (int i = 0; i < usedAccountNum;++i )
{
if(accounts[i].Name==name)
{
return false;
}
}
account = new Account(name, password);
accounts[usedAccountNum++] = account;
return true;
}
}
}
3)在ATM项目中增加ATM类
using System;
using System.Collections.Generic;
using System.Text;
//ATM.cs
//ATM项目中的ATM类源文件
namespace ATM
{
class ATM
{
private const string quitcode = "20130814";
private Bank bank;
public ATM(Bank bank)
{
this.bank = bank;
}
public void Start()
{
while (true)
{
//主界面
PrintLogo();
Console.WriteLine("---------------1.开户---------------");
Console.WriteLine("---------------2.登录---------------");
Console.WriteLine("---------------3.退出---------------");
Console.WriteLine("------------------------------------");
Console.WriteLine("");
Console.Write("请输入您的选择(回车结束):");
string code = Console.ReadLine();
//quit system
if (code == quitcode)
{
return;
}
if (code == "1")
{
OpenAccount();
}
else if(code=="2")
{
LoginAccount();
}
else if (code == "3")
{
Console.WriteLine("按一下任意键直接退出...");
Console.ReadKey();
return;
}
}
}
private void LoginAccount()
{
PrintLogo();
Console.WriteLine("---------------请输入您的帐号的用户名和密码:
---------------");
Console.WriteLine("------------------------------------------------------------");
Console.WriteLine("");
string name = Input("用户名(回车结束):");
string password = Input("密码(回车结束):");
//登录帐号
Account account;
if (!bank.LoginAccount(name, password, out account))
{
Console.WriteLine("---登录错误,请检查用户名和密码是否正确。按Enter键继续...---");
Console.Read();
}
else
{
ManageAccount(ref account);
}
}
private void OpenAccount()
{
PrintLogo();
Console.WriteLine("---------------请输入您的帐号的用户名和密码:
---------------");
Console.WriteLine("------------------------------------------------------------");
Console.WriteLine("");
string name = Input("用户名(回车结束):");
string password = Input("密码(回车结束):");
//开户
Account account;
if (!bank.OpenAccount(name, password, out account))
{
Console.WriteLine("---开户错误,用户名和密码已经存在。按Enter键继续...---");
Console.Read();
}
else
{
Print("开户",0,account);
Pause();
ManageAccount(ref account);
}
}
private void ManageAccount(ref Account account)
{
while (true)
{
//管理帐号界面
PrintLogo();
Console.WriteLine("---------------1.存款---------------");
Console.WriteLine("---------------2.取款---------------");
Console.WriteLine("---------------3.查询余额---------------");
Console.WriteLine("---------------4.修改密码---------------");
Console.WriteLine("---------------5.退出---------------");
Console.WriteLine("------------------------------------");
Console.WriteLine("");
Console.Write("您的选择是(回车结束):");
string code = Console.ReadLine();
string s;
decimal amount;
bool succeed;
switch(code)
{
case"1":
amount = InputNumber("\n请输入存款数目:");
succeed = account.Deposit(amount);
if (succeed)
{
Print("存入", amount, account);
}
else
{
Console.WriteLine("存款失败!");
}
Pause();
break;
case"2":
amount = InputNumber("\n请输入取款数目:");
succeed = account.Withdraw(amount);
if (succeed)
{
Print("取出", amount, account);
}
else
{
Console.WriteLine("取款失败!");
}
Pause();
break;
case"3":
Print(account); ;
Pause();
break;
case"4":
string oldPassword = Input("当前密码(回车结束):");
string newPassword = Input("新密码(回车结束):");
succeed = account.ChangePassword(oldPassword,newPassword);
if (succeed)
{
Console.WriteLine("密码修改成功!");
}
else
{
Console.WriteLine("密码修改失败!");
}
Pause();
break;
case"5":
return;
//break;
default:
break;
}
}
}
private void PrintLogo()
{
Console.WriteLine("\n------------------------------------------");
Console.WriteLine("{0}自动取款机用专业的心做专业的事",https://www.360docs.net/doc/a617358677.html,);
Console.WriteLine("------------------------------------------");
}
private string Input(string prompt)
{
Console.Write(prompt);
string str = Console.ReadLine();
while(str=="")
{
Console.Write("不能为空,{0}",prompt);
str = Console.ReadLine();
}
return str;
}
private decimal InputNumber(string prompt)
{
Console.Write(prompt);
string s = Console.ReadLine();
decimal amount = Decimal.Parse(s);
return amount;
}
private void Pause()
{
Console.Write("按Enter继续...");
Console.Read();
}
private void Print(string operation,decimal amount,Account account)
{
Console.WriteLine("---------------------------------------------");
Console.WriteLine("姓名:{0}",https://www.360docs.net/doc/a617358677.html,);
Console.WriteLine("{0}:{1}",operation,amount);
Console.WriteLine("余额:{0}",account.Banlance);
Console.WriteLine("---------------------------------------------");
Console.WriteLine("{0}成功!", operation);
}
private void Print(Account account)
{
Console.WriteLine("---------------------------------------------");
Console.WriteLine("姓名:{0}",https://www.360docs.net/doc/a617358677.html,);
Console.WriteLine("余额:{0}",account.Banlance);
Console.WriteLine("---------------------------------------------"); }
}
}
4)修改ATM项目中自动创建的启动程序类Program
using System;
using System.Collections.Generic;
using System.Text;
namespace ATM
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("欢迎来到\"老徐银行ATM机!\"");
Bank bank = new Bank("老徐银行");
ATM atm = new ATM(bank);
atm.Start();
}
}
}