acm编程大赛之输入输出
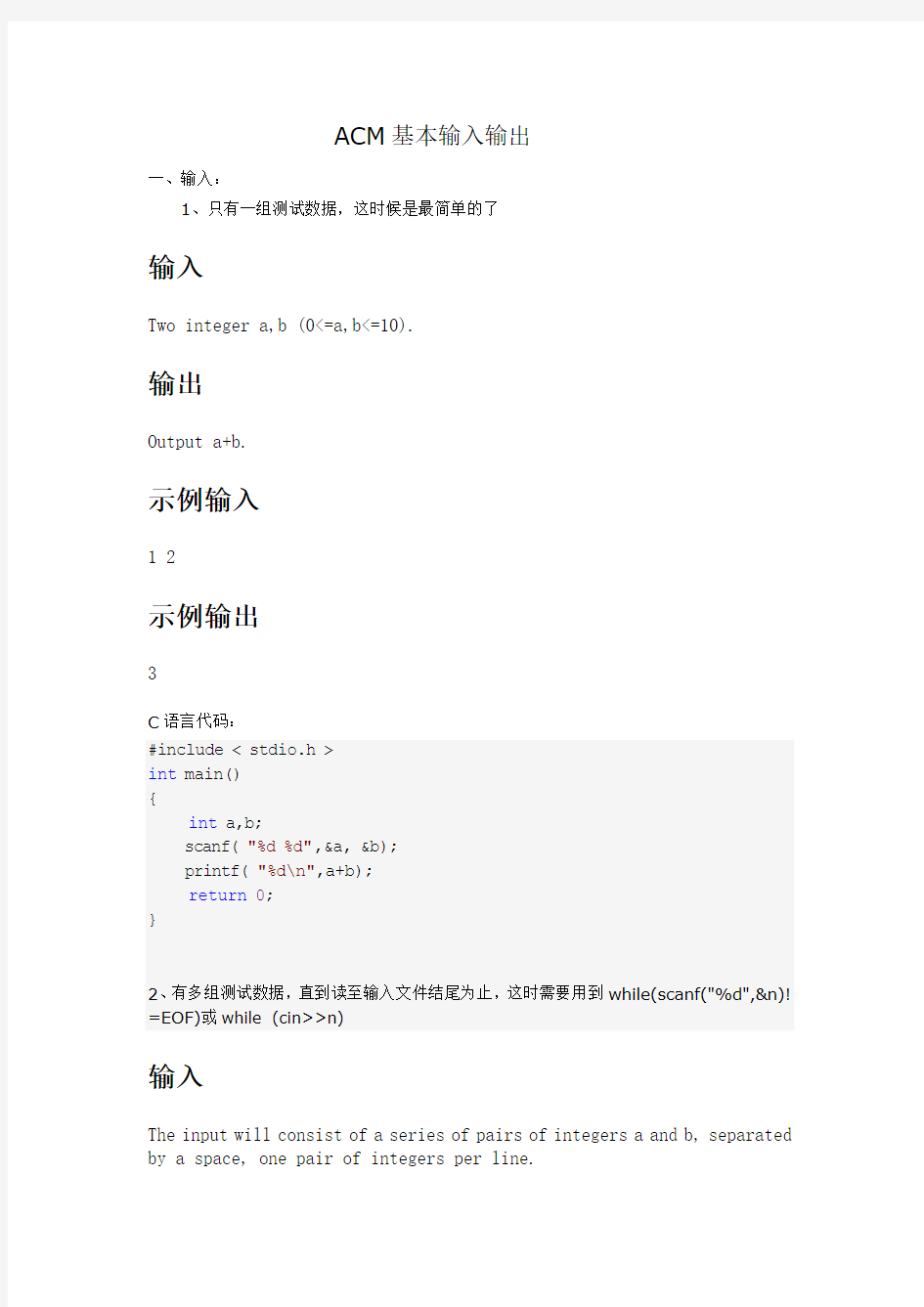

ACM基本输入输出
一、输入:
1、只有一组测试数据,这时候是最简单的了
输入
Two integer a,b (0<=a,b<=10).
输出
Output a+b.
示例输入
1 2
示例输出
3
C语言代码:
#include < stdio.h >
int main()
{
int a,b;
scanf("%d %d",&a, &b);
printf("%d\n",a+b);
return0;
}
2、有多组测试数据,直到读至输入文件结尾为止,这时需要用到while(scanf("%d",&n)! =EOF)或while (cin>>n)
输入
The input will consist of a series of pairs of integers a and b, separated by a space, one pair of integers per line.
输出
For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.
示例输入
1 5
10 20
示例输出
6
30
C语言代码:
#include < stdio.h >
int main()
{
int a,b;
while(scanf("%d %d",&a, &b) != EOF)
printf("%d\n",a+b);
return0;
}
3、在开始的时候输入一个N,接下来是N组数据
输入
Your task is to Calculate a + b.
输出
For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input.
示例输入
2
1 5
10 20
示例输出
6
30
C语言代码:
#include
int main()
{
int n,i;
int a,b;
scanf("%d",&n);
for(i=0;i { scanf("%d%d",&a,&b); printf("%d\n",a+b); } return0; } 4、输入不说明有多少组数据,但以某个特殊输入为结束标志 输入 Input contains multiple test cases. Each test case contains a pair of integers a and b, one pair of integers per line. A test case containing 0 0 terminates the input and this test case is not to be processed. 输出 For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input. 示例输入 1 5 10 20 0 0 示例输出 6 30 C语言代码: #include int main() { int a,b; while(scanf("%d %d",&a, &b) &&(a||b)) printf("%d\n",a+b); } 5、还有一种是前几种的组合 输入 Input contains multiple test cases. Each test case contains a integer N, and then N integers follow in the same line. A test case starting with 0 terminates the input and this test case is not to be processed. 输出 For each group of input integers you should output their sum in one line, and with one line of output for each line in input. 示例输入 4 1 2 3 4 5 1 2 3 4 5 示例输出 10 15 C语言代码: #include int main() { int n,sum,a; while(scanf("%d",&n) && n) { sum=0; while(n--) { scanf("%d",&a); sum+=a; } printf("%d\n",sum); } return0; } 6、输入字符串。 输入是一整行的字符串的,C语法: char buf[20]; gets(buf); C++语法: 如果用string buf;来保存: getline( cin , buf ); 如果用char buf[ 255 ]; 来保存: cin.getline( buf, 255 ); scanf(“ %s%s”,str1,str2),在多个字符串之间用一个或多个空格分隔; 若使用gets函数,应为gets(str1); gets(str2); 字符串之间用回车符作分隔。 通常情况下,接受短字符用scanf函数,接受长字符用gets函数。 而getchar函数每次只接受一个字符,经常c=getchar()这样来使用。 getline 是一个函数,它可以接受用户的输入的字符,直到已达指定个数,或者用户输入了特定的字符。它的函数声明形式(函数原型)如下: istream& getline(char line[], int size, char endchar = '\n'); 不用管它的返回类型,来关心它的三个参数: char line[]:就是一个字符数组,用户输入的内容将存入在该数组内。 int size : 最多接受几个字符?用户超过size的输入都将不被接受。 char endchar :当用户输入endchar指定的字符时,自动结束。默认是回车符。 结合后两个参数,getline可以方便地实现:用户最多输入指定个数的字符,如果超过,则仅指定个数的前面字符有效,如果没有超过,则用户可以通过回车来结束输入。 char name[4];cin.getline(name,4,'\n'); 由于 endchar 默认已经是 '\n',所以后面那行也可以写成: cin.getline(name,4); 最后需要说明的是,C++的输入输出流用起来比较方便,但速度比C要慢得多。在输入输出量巨大时,用C++很可能超时,应采用C的输入输出。 二、输出: 输出有不同的格式要求,不注意的话经常会出现“Presentation Error”,而且PC2很多时候还判断不出来输出格式错误,就简单的判为"Wrong Answer",所以输出格式一定要注意。 1、一组输出接着一组输出,中间没有空行,这也是最简单的 输入 The input will consist of a series of pairs of integers a and b, separated by a space, one pair of integers per line. 输出 For each pair of input integers a and b you should output the sum of a and b in one line, and with one line of output for each line in input. 示例输入 1 5 10 20 示例输出 6 30 C语言代码: #include int main() { int a, b; while (scanf("%d%d", &a, &b) != EOF) printf("%d\n\n", a + b); return0; } 每输出一组结果后输出两个换行就可以了。 3、一组接着一组,每两组之间有一个空行,注意与前一种区分开 输入 Input contains an integer N in the first line, and then N lines follow. Each line starts with a integer M, and then M integers follow in the same line 输出 For each group of input integers you should output their sum in one line, and you must note that there is a blank line between outputs. 示例输入 3 4 1 2 3 4 5 1 2 3 4 5 3 1 2 3 示例输出 10 15 6 #include int main() { int n,m,sum,a; int i; scanf("%d",&n); for (i = 0; i < n;i++) { scanf("%d",&m); sum = 0; while (m--){ scanf("%d",&a); sum +=a; } printf("%d\n",sum); if (i != n-1) printf("\n"); } return0; } 判断是否到达最后一组测试数据了,如果不是最后一组测试数据就多输出一个换行