mongodb-java-driver基本用法
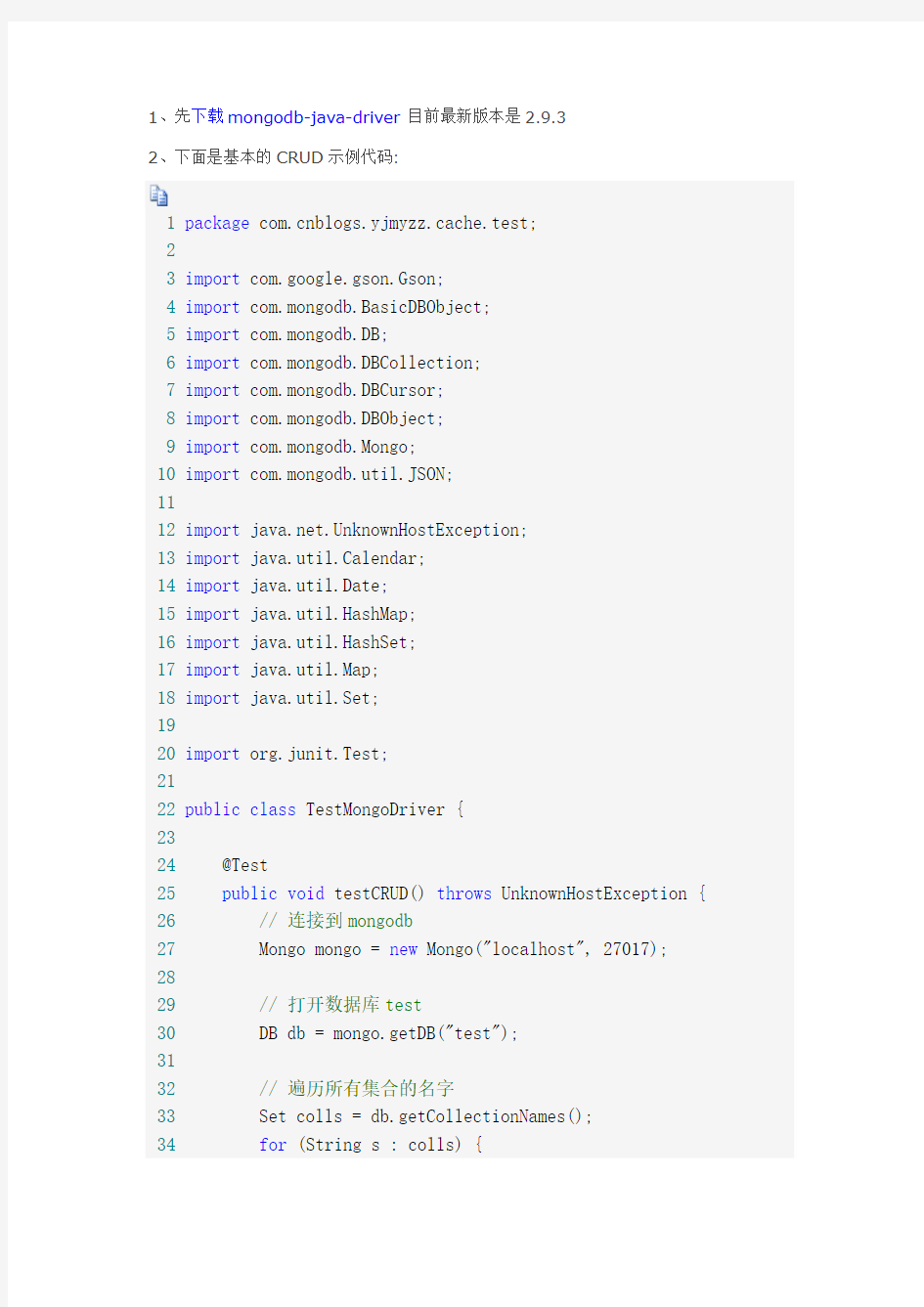
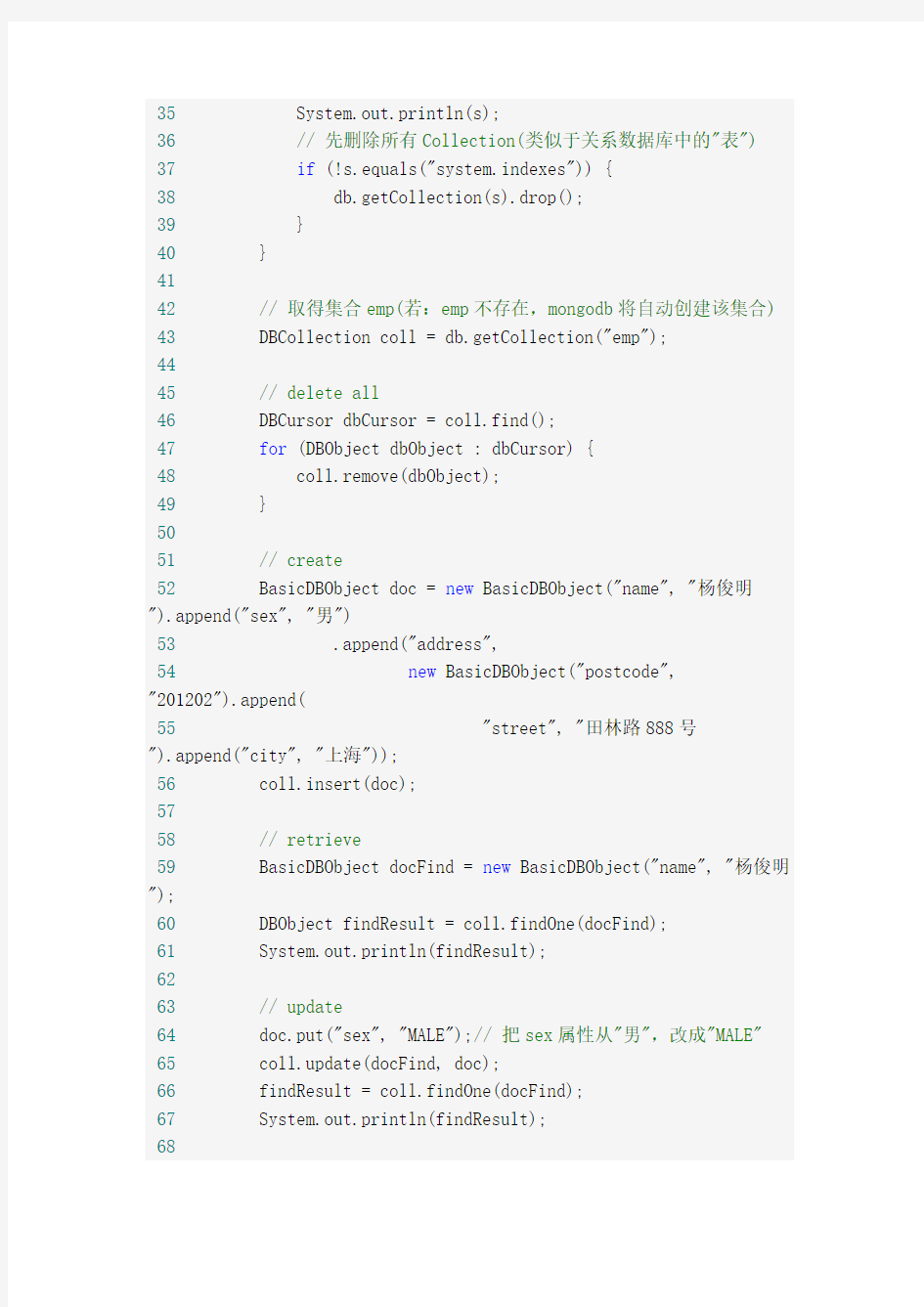
1、先下载mongodb-java-driver目前最新版本是2.9.3
2、下面是基本的CRUD示例代码:
1package https://www.360docs.net/doc/d13298335.html,blogs.yjmyzz.cache.test;
2
3import com.google.gson.Gson;
4import com.mongodb.BasicDBObject;
5import com.mongodb.DB;
6import com.mongodb.DBCollection;
7import com.mongodb.DBCursor;
8import com.mongodb.DBObject;
9import com.mongodb.Mongo;
10import com.mongodb.util.JSON;
11
12import https://www.360docs.net/doc/d13298335.html,.UnknownHostException;
13import java.util.Calendar;
14import java.util.Date;
15import java.util.HashMap;
16import java.util.HashSet;
17import java.util.Map;
18import java.util.Set;
19
20import org.junit.Test;
21
22public class TestMongoDriver {
23
24 @Test
25public void testCRUD() throws UnknownHostException { 26// 连接到mongodb
27 Mongo mongo = new Mongo("localhost", 27017);
28
29// 打开数据库test
30 DB db = mongo.getDB("test");
31
32// 遍历所有集合的名字
33 Set
34for (String s : colls) {
35 System.out.println(s);
36// 先删除所有Collection(类似于关系数据库中的"表")
37if (!s.equals("system.indexes")) {
38 db.getCollection(s).drop();
39 }
40 }
41
42// 取得集合emp(若:emp不存在,mongodb将自动创建该集合) 43 DBCollection coll = db.getCollection("emp");
44
45// delete all
46 DBCursor dbCursor = coll.find();
47for (DBObject dbObject : dbCursor) {
48 coll.remove(dbObject);
49 }
50
51// create
52 BasicDBObject doc = new BasicDBObject("name", "杨俊明").append("sex", "男")
53 .append("address",
54new BasicDBObject("postcode", "201202").append(
55 "street", "田林路888号
").append("city", "上海"));
56 coll.insert(doc);
57
58// retrieve
59 BasicDBObject docFind = new BasicDBObject("name", "杨俊明");
60 DBObject findResult = coll.findOne(docFind);
61 System.out.println(findResult);
62
63// update
64 doc.put("sex", "MALE");// 把sex属性从"男",改成"MALE"
65 coll.update(docFind, doc);
66 findResult = coll.findOne(docFind);
67 System.out.println(findResult);
68
69 coll.dropIndexes();// 先删除所有索引
70// create index
71 coll.createIndex(new BasicDBObject("name", 1)); // 1代表升序
72
73// 复杂对象
74 UserData userData = new UserData("jimmy", "123456");
75 Set
76 pets.add("cat");
77 pets.add("dog");
78 Map
79 favoriteMovies.put("dragons", "Dragons II");
80 favoriteMovies.put("avator", "Avator I");
81 userData.setFavoriteMovies(favoriteMovies);
82 userData.setPets(pets);
83 userData.setBirthday(getDate(1990, 5, 1));
84 BasicDBObject objUser = new BasicDBObject("key", "jimmy").append(
85 "value", toDBObject(userData));
86 coll.insert(objUser);
87 System.out.println(coll.findOne(objUser));
88 }
89
90/**
91 * 将普通Object对象转换成mongodb的DBObject对象
92 *
93 * @param obj
94 * @return
95*/
96private DBObject toDBObject(Object obj) {
97 Gson gson = new Gson();
98 String json = gson.toJson(obj);
99return (DBObject) JSON.parse(json);
100 }
101
102/**
103 * 获取指定日期
104 *
105 * @param year
106 * @param month
107 * @param day
108 * @return
109*/
110private Date getDate(int year, int month, int day) { 111 Calendar calendar = Calendar.getInstance();
112 calendar.clear();
113 calendar.set(year, month - 1, day);
114return calendar.getTime();
115
116 }
117
118 }
其中,为了演示复杂对象的持久化,类UserData定义如下:
package https://www.360docs.net/doc/d13298335.html,blogs.yjmyzz.cache.test;
import java.io.Serializable;
import java.util.Date;
import java.util.Map;
import java.util.Set;
public class UserData implements Serializable {
private static final long serialVersionUID = -4770493237851400594L;
private String userName;
private String password;
private Set
private Map
private Date birthday;
public UserData() {
public UserData(String userName, String passWord) {
https://www.360docs.net/doc/d13298335.html,erName = userName;
this.password = passWord;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
https://www.360docs.net/doc/d13298335.html,erName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Set
return pets;
}
public void setPets(Set
this.pets = pets;
}
public Map
return favoriteMovies;
}
public void setFavoriteMovies(Map
this.favoriteMovies = favoriteMovies;
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
}
运行效果如下:
emp
system.indexes
{ "_id" : { "$oid" : "53d34795744ec171e7f6980a"} , "name" : "杨俊明" , "sex" : "男" , "address" : { "postcode" : "201202" , "street" : "田林路888号" , "city" : "上海"}}
{ "_id" : { "$oid" : "53d34795744ec171e7f6980a"} , "name" : "杨俊明" , "sex" : "MALE" , "address" : { "postcode" : "201202" , "street" : "田林路888号" , "city" : "上海"}}
{ "_id" : { "$oid" : "53d34796744ec171e7f6980b"} , "key" : "jimmy" , "value" : { "userName" : "jimmy" , "password" : "123456" , "pets" : [ "cat" , "dog"] , "favoriteMovies" : { "dragons" : "Dragons II" , "avator" : "Avator I"} , "birthday" : "May 1, 1990 12:00:00 AM"}}
延伸阅读:
mondodb-java-driver 官方在线文档
8天学通mongodb系列
MongoDB基本用法
现在进行时的基本用法及练习题
{ 现在进行时的基本用法 一.定义:(1)现在进行时表示此时此刻正在发生的动作。 如:we are having English now. 我们正在上英语课。 (2)现在进行时表示现阶段正在发生的动作。 如:we are studying for a test these days. 这些天来我们一直在复习备考。 (3)现在进行时与always,forever(永远),constantly(不断地)continually(频繁地)等连用,表示赞赏或厌恶等感情色彩。 如: I’m always losing my books.我总丢书。(生气)He is always helping others. We all love him.他总是乐于助人,我们大家都喜欢他。(赞赏) ( He is constantly talking to me.他不停地跟我讲话。(厌烦)二.谓语构成及变化规则(观察例子进行总结) 如:I am running with my parents now. Look! Bob is swimming in the river. It’s 9:00. They are having
(1).结合以上例子分析:现在进行时谓语部分为:be(am/is/are)+现在分词(动词-ing) (2)动词有原形变为现在分词的基本规则: A.一般情况,在动词原形后直接加-ing. 如:watch---- watching, $ go--- going, walk--- walking, do--- doing, fly--- flying. B.以不发音的字母e结尾的动词,去掉e,再加-ing.如:take--- taking. make--- making,drive--- driving, leave---leaving C.以“辅音字母+元音字母+辅音字母”的结构且中间的元音字母发的不是字母表上的音时即重读闭音节结构,双写最后一个辅音字母,再加-ing。如:run---running, plan---planning,set---setting,put--- putting, cut---cutting,shop---shopping,swim---swimming,stop---stoppi ng, sit---siting. 例外情况:visit---visiting, follow---following,slow(延缓)---
人教版英语英语现在完成时的用法大全含解析
人教版英语英语现在完成时的用法大全含解析 一、初中英语现在完成时 1.—They say there is a new restaurant near here. —Yes, and it ______ for more than a week. A. has been open B. open C. is opening D. opens 【答案】 A 【解析】【分析】句意:——他们说在这附近有一个新的餐馆。——是的,它已经开了一个多星期了。根据 for more than a week ,可知用现在完成时,have/has been done,故选A。 【点评】考查现在完成时,注意识记其标志词。 2.Mike used to be a top student, but he behind since he lost himself in computer games. A. fell B. has fallen C. was D. has been 【答案】 D 【解析】【分析】句意为“Mike过去是尖子生,但自从迷上电子游戏以来成绩落后了”。由since可知but后的主句用现在完成时,瞬间动词fall不能和since引导的时间状语从句连用,故用延续性动词be。故选D。 【点评】本题考查现在完成时中非延续性动词和延续性动词的转换。 3.—Hi, Tom! you ever the Bird's Nest? —Yes, I have. It's fantastic. A. Have, been to B. Have, gone to C. Did, go to 【答案】 A 【解析】【分析】句意:——你好,Tom!你曾经去过鸟巢吗?——是的,我去过。它是极好的。根据答语—Yes, I have.可知是以have开头的现在完成时的一般疑问句,排除C。have been to+地点名词,去过某地,去了并且回来了;have gone to+地点名词,去了某地,去了还没有回来,在去或者回来的路上。根据It's fantastic.可知去了并且回来了,故选A。 【点评】考查短语辨析,注意平时识记其区别,理解句意。 4.——Where is Mr. Wang? ——He together with his students ________ Zhuyuwan Park. A. has gone to B. have gone to C. has been to D. have been to 【答案】 A
现在进行时的用法
现在进行时用法 —、定义:1、表示说话时正在进行的动作及行为2、表示现阶段正在进行的动作。 二、结构:现在进行时常有三种句型: (1)肯定式:主语+ be+ v-i ng +其它。如:He is men di ng his bike .他正在修自行车。 (2)否定式:主语+ be+ not + v-i ng +其它。如: He is not (isn't ) men di ng his bike .他没在修自行车。 (3)疑问式:主要分一般疑问句和特殊疑问句两种句式。 一般疑问句:Be+主语+ v-ing +其它?如: —Is he men di ng his bike ?他正在修自行车吗?一Yes, he is . ( No, he isn't .) 特殊疑问句:疑问词+ be +主语+ v-i ng +其它?如: —What is he doi ng ?他正在干什么? 三、何时用现在进行时? (1)以Look !或Listen !开头的句子提示我们说话时动作正在进行,应用现在进行时。女口 : Look! The children are playing games over there . Listen ! Who's singing in the classroom ? (2)当句子中有now (现在)时,常表示说话时动作正在进行,这时也常用现在进行时。 女口:We are reading English now (3 )描述图片中的人物的动作时常用现在进行时,以示生动。如: Look at the picture . The girl is swimming . ( 4)表示当前一段时间内的活动或现
一般将来时和过去将来时的用法
一般将来时和过去将来时的用法 一、一般将来时 表示在现在看来即将要发生的动作或存在的状态。 1、“will/shall+动词原形”表示客观上势必将发生的事情或临时做出的打算。Shall 用于第一人称,will用于第二人称。 Later I shall tell you about some of the work I have done. Next month we will have our school open day. 记一记:常与一般将来时连用的时间状语有:next time,tomorrow,before long,in the future,later on,the day after tomorrow等。 2、be going to+动词原形,表示将来。表示主观计划、打算做某事或根据某种迹象表明某事即将要发生。 (1)表示主语的意图,即将做某事。 What are you going to do tomorrow? (2)表示按计划、安排要发生的事。 The play is going to be produced next month.这部戏下月开播。 (3)表示根据某种迹象表明要发生的事。 Look at the dark clouds. It is going to rain. 3、be to do 表示按计划、约定或按职责、义务必须去做的事情或即将发生的动作。 We are to discuss the report next Saturday. 注意:be to do 和be going to的区别: be to do表示客观安排或受人指示而做某事,be going to 表示主观的打算或计划。
最新现在进行时 的两种用法
现在进行时的两种用法 1.(1)当句子表示动作正在进行,这时要用现在进行时。如: They are playing basketball now.现在他们正在打篮球。 (2)以look, listen开头的句子,提示我们动作正进行,这时要用现在进行时。如: Listen!She is singing an English song.听,她正在唱英语歌。 (3)表示当前一段时间或现阶段正在进行的动作,且此时有this week, these days等时间状语,这时常用现在进行时。如: We are making model planes these days.这些天我们在做飞机模型。 (4)描述图片中的人物的动作,也为了表达更生动。此时也常用现在进行时。如: Look at the picture. The children are flying kites in the park.看这幅图,那些孩子正在公园放风筝。 2.现在进行时表达按计划安排近期内即将发生的动作[进行时表将来] 有这种用法的动词或短语有:come, go, start, leave, take off, fly, see off, meet, get(to), arrive, stay, return, take 等。 例1 Mr. Ma is coming. 马老师要来了。 例2 I am leaving Washington for New York. 我马上要离开华盛顿到纽约去了。 你仔细体会一下,这些动词都是表示“来,去”之类的瞬时动词。 所以不能说I'm being a teacher 现在进行时的基本用法: a. 表示现在(指说话人说话时)正在发生的事情。例如: We are waiting for you. 我们正在等你。 b. 习惯进行:表示长期的或重复性的动作,说话时动作未必正在进行。例如: Mr. Green is writing another novel. 他在写另一部小说。(说话时并未在写,只处于写作的状态。) c. 表示渐变,这样的动词有:get, grow, become, turn, run, go, begin 等。例如:
现在完成时的基本用法
现在完成时讲解与练习 (一)现在完成时的基本用法 (1)强调动作是从过去持续到现在,并有可能继续持续下去。 (2)强调对现在的影响或结果,此用法容易和一般过去时混淆。两者的区别是:一般过去时有动作发生的时间点,即过去某一时间发生某一动作;现在完成时则没有,即不强调是哪个时间点发生的动作,而强调过去的动作对现在造成的影响和结果。 (3)在过去不确定的时间里发生的动作,但是结果对现在有影响。一、现在完成时的构成 (一)肯定式 主语+助动词have /has +过去分词+其它 说明:这里的have /has是助动词,没有什么具体意义。当主语是第三人称单数时助动词用has,其余人称一律用have。has,have的缩略式分别为's或've。规则动词过去分词的构成与过去式的构成方式一样,不规则动词可参看不规则动词表。实例: 1)I've just copied all the new words .我刚抄写了所有的生词。(表示不要再抄了) 2)She has lost her books .她丢失了她的书。(表示到目前为止还没有找到) (如果用过去时:She lost her books . 则强调书是过去丢的这一动作,而不知现在有没有找到) 3)We've just cleaned the classroom .我们刚好打扫了教室。(表明现在教室是干净的) (二)否定式 主语+助动词have /has+not+过去分词+其它 说明:现在完成时构成否定句时,只需在助动词have /has后面加not就行。have not,has not的缩略式分别为haven't ,hasn't。另外,肯定句中有some,already时,改为否定时要分别改成any,yet。实例: 1)I haven't finished my homework yet.我还没有完成我的作业。 2)She hasn't travelled on a train .她没有坐火车旅行过。 3)We have never spoken to a foreigner.我们从来没有和外国人说过话。注:有时not可以用never代替,表示“从来没有”的意思。又如:
现在进行时用法归纳
现在进行时用法归纳 基本用法: 1. 表示说话时正在进行的动作,强调“此时此刻”,常和now, look, listen连用。 Look! A train is coming. 看,火车来了。 Listen! He is playing the piano. 听,他在弹琴。 2. 表示现阶段正在进行的事情,不一定说话时正在进行。常和at present ,this week ,these days…等时间状语连用。 What lesson are you studying this week? 你们本周学哪一课了?(说话时并不在学) 3. 现在进行时有时可用来表示一个最近按计划或安排要进行的动作,即用现在进行时代替一般将来时, 动词一般多为表示位置移动的动词。如:go, come, leave等。 Where are you going? 你去哪? I am coming. 我来了。 Are you going to Tianjin tomorrow? 你明天去天津吗? How many of you are coming to the party next week? 你们有多少人下周要来参加晚会? 4. wear用现在进行时表示一种状态。如: He is wearing a blue coat. 他穿着一件蓝外套。 5. 有些动词意思只是表示一种状态,不能用于现在进行时。如:have(有),like等。 Now each of us has a dictionary. 现在我们每一个人都有一本词典。 6. 现在进行时与always, often, forever等连用表示赞扬、厌烦等语气。该知识点仅作了解之用, 不作为中考考查内容。如: You’re always interrupting me! 你老打断我的话!(抱怨) My father is always losing his car keys. 我爸老丢车钥匙。(不满) She’s always helping people. 她老是帮助别人。(赞扬)
英语一般将来时用法总结(完整)
英语一般将来时用法总结(完整) 一、单项选择一般将来时 1.Peace is necessary to all. After all, it is the United States and China, as the two largest economies in the world, that ________ most from a peaceful and stable Asia-Pacific. A.are benefited B.will benefit C.will be benefited D.had benefited 【答案】B 【解析】 试题分析:根据语境“美国和中国将受益于一个和平稳定的亚太地区”可知该句要用一般将来时,故选B。 考点:考查时态 2.--- I’d like a mountain bike which ____ well? --- Will this one _____? A.rides; work B.rides; do C.is ridden; do D.is ridden; work 【答案】B 【解析】 试题分析:考查主动形式表示被动含义用法。一些不及物动词与副词连用,表示主语的特征。如wash well,write well等;用主动形式表示被动的含义。本句中的ride well指自行车好骑;第二空的do表示行。句意:—我想买一辆很好骑的山地车。—这个行吗?根据句意说明B正确。 考点:考查主动形式表示被动含义的用法。 点评:。一些不及物动词与副词连用,表示主语的特征。如wash well,write well等;用主动形式表示被动的含义。 3.It every day so far this month. I can't tell you if it tomorrow. A.rained; rains B.is raining; shall rain C.has been raining; rains D.has rained; will rain 【答案】D 【解析】 试题分析:本题第一空应该使用现在完成式,关键词是后面的时间状语so far(到目前为止),so far通常都是和现在完成时连用。第二空是一个if引导的宾语从句,并非if引导的条件句,在这个宾语从句中,时间状语是tomorrow,这是一个将来时的时间状语,故该宾语从句使用将来时。句义:这个月到现在为止天天都在下雨,所以我无法告诉你明天是否还要要下雨。故D正确。 考点:考察时态 4.What you learn today ______ of practical use when you hunt for a job. A.is proved B.proves C.will be proved D.will prove
现在完成时的基本用法
现在完成时的基本用法 1、现在完成时表示影响 Hehasleftthecity.他已离开这个城市。(结果:他不在这个城市。) Someonehasbrokenthewindow.有人把窗户打破了。(结果:窗户 仍破着。) Ihavelostmypen.我把钢笔丢了。(结果:我现在无钢笔用。) Hehasfinishedhiswork.他把工作做完了。(结果:他现在可以做其他的事了。) 2、现在完成时表示持续 该用法的现在完成时表示一个过去发生的动作或开始的状语在过去并未完成或结束,而是一直持续到现在,并且有可能继续下去(也 可能到此结束),如汉语说“他在我们学校教书已有30年了”,显 然“他在我们学校教书”是从30年前开始,并且一直教到现在,已 经持续了30年;又如汉语说“自上个星期以来他一直很忙”,显然“忙”是从上个星期开始的,并且这一“忙”就一直忙到现在。如: Hehastaughtinourschoolfor30years.他在我们学校教书已有30 年了。 Hehasbeenbusysincelastweek.自上个星期以来他一直很忙。 Hehasworkedforuseversinceheleftschool.他离开学校以后就一直为我们工作。 3、现在完成时表示重复
即表示从过去某个时间直到现在的这个时间范围内不断重复发生的动作或情况,并且这个不断重复的动作有可能继续下去,也有可能到现在就结束。如: Howoftenhaveyouseenher?你隔多少见她一次? Myfatherhasalwaysgonetoworkbybike.我父亲一向骑车上班。 4、现在完成时表示将来 同一般现在时可以表示将来一样,现在完成时也可以在时间状语从句里表示将来。如: I’llwaituntilhehaswrittenhisletter.我愿等到他把信写完。 Whenyouhaverested,I’llshowyouthegarden.等你休息好之后,我领你看我们的花园。 二、现在完成时的基本定义和句型构成 基本定义 现在完成时有两种用法 1.过去发生的某一动作对现在造成的影响或结果。到现在为止已经发生或完成的动作。 2.表示过去发生的、持续到现在的动作或状态。 句型构成 基本结构:主语+have/has+动词的过去分词(p.p) 现在完成时用法 现在完成时用法 ①肯定句:主语+have/has+动词的过去分词(p.p)(V-ed)+宾语(或者其他). ②否定句:主语+havenot/hasnot+动词的过去分词(p.p)(V-ed)+宾语.
英语现在进行时用法详解
英语现在进行时用法详解 一、单项选择现在进行时 1.As the job-hunting season is on the way,many graduates from anxiety. A.will be suffered B.are suffering C.were suffering D.are being suffered 【答案】B 【解析】 B。大学毕业生在找工作前就已经开始为工作焦虑了,故用现在进行时。 2.Hi, Mike. I’ve to borrow your car this afternoon mine _______ in the garage. A.is repairing B.is being repaired C.has repaired D.has been repaired 【答案】B 【解析】 试题分析:考察时态语态。句义:今天下午我不得不借用你的汽车,因为我的汽车正在修理厂被修理。根据句义可知我的汽车正在被修理是正在发生的事情。根据句义说明B项使用现在进行时的被动语态正确。 考点:考察时态语态 3.—I some courses at university,so I can’t work full time at the moment. A.take B.am taking C.took D.have taken 【答案】B 【解析】 试题分析:考查动词的时态。句意:因为我正在上学,所以不能在这时候做全职的工作。根据下方提到so I can’t work full time at the moment 可知我正在上学,故选B 项。 考点 : 考查动词的时态 4.The young parents _________ too much a pet of their son, which is bound to destroy him in the end. A.have made B.are making C.made D.will be making 【答案】B 【解析】 试题分析:句意:这对年轻的父母太宠爱他们的儿子,这一定会最后毁了他。词组:make a pet of sb 宠爱某人宠爱某人,因为指现阶段一直的行为,用现在进行时,所以选B。 考点:考查动词时态 5.The water supply has been cut off temporarily because the workers the main pipe.A.repair B.repaired C.have repaired D.are repairing
(完整版)八年级上册一般将来时用法讲解及练习
一般将来时: 一、由“will+动词原形”构成。一般将来时表示将来某个时间要发 生的动作或存在的状态,也表示将来经常或反复发生的动作,常与表将来的时间状语连用,如: tomorrow(明天),the day after tomorrow(后天), next…(下个……), soon(不久), one day(某天), from now(从今后), in…(time)(在……时间之后),in the future(在将来)等。 最基本的结构:will / shall + 动词原形 “主谓(宾)句型”的一般将来时: 肯定句:主语+ will +动词原形+(宾语)+其他成份People will have robots in their homes. 否定句:在will 的后面加not即可。will not = won’t People will not (won’t) have robots in their homes. 一般疑问句:把will 提到句子主语之前,结尾变问号。Will people have robots in their homes? 特殊疑问句:特殊疑问词+will +主语+动词原形+其他成份?When will people have robots in their homes? 二、“There be”句型的一般将来时 肯定句:There will be +名词+其他成份=There is /are going to be [注意]:无论后面加单数名词或复数形式,be都必须用原形。There will be only one country. 否定句:在will后面加not. There won’t be only one country. 一般疑问句:把will提到there之前。 Will there be only one country? Yes, there will. / No, there won’t. 三、be going to+动词原形 a.表示打算、准备做的事。例如: We are going to put up a building here.我们打算在这里盖一座楼。 How are you going to spend your holidays?假期你准备怎样过? b.表示即将发生或肯定要发生的事。例如: I think it is going to snow. 我看要下雪了。 There’s going to be a lot of trouble about this. 这事肯定会有很多麻烦。 c.“will”句型与“be going to”句型,前者表示纯粹将来,后者表示打算、计划、准备做的事情,更强调主语的主观意愿。例如: Tomorrow will be Saturday. 明天是周六了。 We are going to visit Paris this summer.今年夏天我们打算游览巴黎。 四、用be doing表示将来:主要意义是表示按计划、安排即将发生的动作,常用于位置转移的动词。 如:go,come,leave,arrive等,也可用于其他动作动词。 We are having fish for dinner. We are moving to a different hotel the day after tomorrow. 这种用法通常带有表示将来的时间状语,如果不带时间状语,则根据上下文可表示最近即将发生的动作。 A: Where are you going? B: I am going for a walk. Are you coming with me? A: Yes,I am just coming. Wait for me. 课堂检测 ( ) 1. There __________ a meeting tomorrow afternoon. A. will be going to B. will going to be C. is going to be D. will go to be ( ) 2. Charlie ________ here next month. A. isn’t working B. doesn’t working C. isn’t going to working D. won’t work ( ) 3. He ________ very busy this week, he ________ free next week. A. will be; is B. is; is C. will be; will be D. is; will be ( ) 4. There ________ a dolphin show in the zoo tomorrow evening. A. was B. is going to have C. will have D. is going to be ( ) 5. –________ you ________ free tomorrow? – No. I ________ free the day after tomorrow. A. Are; going to; will B. Are; going to be; will C. Are; going to; will be D. Are; going to be; will be ( ) 6. Mother ________ me a nice present on my next birthday. A. will gives B. will give C. gives D. give ( ) 7. – Shall I buy a cup of tea for you? –________. (不,不要。) A. No, you won’t. B. No, you aren’t. C. No, please don’t. D. No, please. ( ) 8. – Where is the morning paper? – I ________ if for you at once. A. get B. am getting C. to get D. will get ( ) 9. ________ a concert next Saturday? A. There will be B. Will there be C. There can be D. There are ( ) 10. If they come, we ________ a meeting. A. have B. will have C. had D. would have ( ) 11. He ______ her a beautiful hat on her next birthday. A. gives B. gave C. will giving D. is going to giving ( ) 12. He ________ to us as soon as he gets there. A. writes B. has written C. will write D. wrote ( ) 13. He ________ in three days. A. coming back B. came back C. will come back D. is going to coming back ( ) 14. If it _____ tomorrow, we’ll go roller-skating. A. isn’t rain B. won’t rain C. doesn’t rain D. doesn’t fine ( ) 15. – Will his parents go to see the Terra Cotta Warriors tomorrow?–No, ________ (不去). A. they will n’t. B. they won’t. C. they aren’t. D. they don’t. ( ) 16. Who ________ we ________ swimming with tomorrow afternoon? A. will; go B. do; go C. will; going D. shall; go ( ) 17. We ________ the work this way next time. A. do B. will do C. going to do D. will doing
英语现在进行时用法总结(完整)
英语现在进行时用法总结(完整) 一、单项选择现在进行时 1.Why are you here? You are supposed to ______ the experiment in the lab. A.perform B.be performing C.have performed D.be performed 【答案】B 【解析】 试题分析:考查被动语态。句意:你为什么在这里?你应该在实验室里做实验。(是别人让他在实验室做实验)故选B项。 考点:考查被动语态 【名师点睛】 被动语态由“助动词be+及物动词的过去分词”构成。人称、数和时态的变化是通过be的变化表现出来的。把主动语态的宾语变为被动语态的主语。把谓语变成被动结构(be+过去分词),根据被动语态句子里的主语的人称和数,以及原来主动语态句子中动词的时态来决定be的形式。把主动语态中的主语放在介词by之后作宾语,将主格改为宾格。 2.—I some courses at university,so I can’t work full time at the moment. A.take B.am taking C.took D.have taken 【答案】B 【解析】 试题分析:考查动词的时态。句意:因为我正在上学,所以不能在这时候做全职的工作。根据下方提到so I can’t work full time at the moment 可知我正在上学,故选B 项。 考点 : 考查动词的时态 3.The young parents _________ too much a pet of their son, which is bound to destroy him in the end. A.have made B.are making C.made D.will be making 【答案】B 【解析】 试题分析:句意:这对年轻的父母太宠爱他们的儿子,这一定会最后毁了他。词组:make a pet of sb 宠爱某人宠爱某人,因为指现阶段一直的行为,用现在进行时,所以选B。 考点:考查动词时态 4.—Have you seen recently? —No, but I _______ dinner with him on Friday. A.had B.have had C.am having D.was having 【答案】C 【解析】 试题分析:句意:---你最近见过Sean吗? ---不,但是我周五要和他一起吃饭。A.had一般过去时;B.have had现在完成时;C.am having是现在进行时表将来;D.was having
现在完成时延续性与暂短性动词用法
现在完成时(短暂性动词与延续性动词)的用法 基本结构:主语+have/has+过去分词(done) ①肯定句:主语+have/has+过去分词+其他 ②否定句:主语+have/has+not+过去分词+其他 ③一般疑问句:Have/Has+主语+过去分词+其他 ④特殊疑问句:特殊疑问词+一般疑问句(have/has+主语+过去分词+其他)(1)现在完成时用来表示现在之前已发生过或完成的动作或状态,但其结果却和现在有联系,也就是说,动作或状态发生在过去但它的影响现在还存在. I have spent all of my money.(含义是:现在我没有钱花了.) Jane has laid the table.(含义是:现在桌子已经摆好了.) Michael has been ill.(含义是:现在仍然很虚弱) He has returned from abroad. (含义是:现在已在此地) (2)现在完成时可以用来表示发生在过去某一时刻的,持续到现在的动作(用行为动词表示)或状态(be动词表示)常与for(+时间段),since(+时间点或过去时的句子)连用. Mary has been ill for three days. I have lived here since 1998. 注(超重要):瞬间动词(buy,die,join,lose……)不能直接与for since 连用。要改变动词 come-be go out-be out finish-be over open-be open die-be dead 1.have代替buy My brother has had(不能用has bought) this bike for almost four years. 2、用keep或have代替borrow I have kept(不能用have borrowed) the book for quite a few days. 3、用be替代become How long has your sister been a teacher? 4、用have a cold代替catch a cold Tom has had a cold since the day before yesterday. 5、用wear代替put on b)用“be+形容词”代终止性动词 1、be+married代marry 2、be+ill代fall (get) ill 3、be+dead代die 4、be+asleep代fall (get) asleep 5、be+awake代wake/wake up 6、be+gone代lose,die,sell,leave 7、be+open代open 8、be closed代close/shut 9、be+missing(gone,lost)代lose c)用“be+副词”代终止性动词 1“be+on”代start,begin 2“be+up”代get up 3“be+back(to)”代return to,come back to,go back to 4“be here (there)”代come(arrive,reach,get) here或go (arrive,reach,get) there等等
一般将来时用法小结
一般将来时用法小结: 一般将来时表示在将来某个时间要发生的动作或存在的状态。 一 . 一般将来时的构成: 1. 由助动词“ shall/ will +动词原形”构成, shall 用于第一人称, will 用于第二、第三人称, 2. 一般将来时的否定和疑问形式: 一般将来时的否定形式是 will not ,缩写为 won't; shall not ,缩写为 shan't 。 一般将来时的疑问形式是把 will/ shall 提到主语前。 如: He won’t go to the park this Sunday. 本周日他不去公园。 Will you go swimming with me? 和我一起去游泳好吗? 二 . 一般将来时的基本用法: 1.表示“纯粹的将来”:①表示将要发生的动作或情况,常带有表示将来的时间状语,如 tomorrow, next week, in two days, from now on 等。如: It will be fine tomorrow.明天天气晴朗。②表示预料将要发生的动作或情况。如:You will feel better after having this medicine. 吃了这药,你就会感觉好些的。③表示由于习惯倾向而会经常发生的动作,本用法中的 will 要重读。如: Boys will be boys. [谚语]男孩毕竟是男孩。 2. 表示“带有情态意义的将来”,用来表示意图,用 will 来表示。 如: I will be more careful next time. 下次我要更加小心。 I won't go shopping this afternoon, but she will. 今天下午我不想去购物,但她想去。 will 在疑问句中,用来表示有礼貌地征询对方的意见。如:Will you have some more tea? 要不要再喝点茶?What shall we do this weekend? 本周末我们要干什么? 三 . 一般将来时的其它几种表示法: 1. 用 be going to 表示be going to 相当于一个助动词,与其后的动词原形一起构成句子的谓语,表
英语中现在完成时的基本用法讲解
英语中现在完成时的基本用法讲解 (含义:我对他有所了解了,现在能够谈谈他的情况了。 )Have you been to Paris?你去过巴黎吗?(含义:如果你去过,你可以谈谈巴黎的情况。 如果你没有去过,我建议你去看看,或者我现在给你介绍一下巴黎的情况。 )We have never heard of such a man.我们从来没有听说过这样的人。 (含义:因此我们对“他一无所知,你问我们也是白问。 )模仿造句:1.因此,我们以前参观莫斯科(Moscow)。 2.你弟弟去去印度(India)吗? 3.这些孩子从未用过电脑。 2.现在完成时,表示过去某种行为的结果对现在有直接的影响Tom has lost his pen.汤姆的钢笔已经丢了。 (含义:结果是现在没笔用,必须借一支笔,或者买一支笔。 )Dad, I have finished my homework.爸爸,我已经做完家庭作业了。 (含义:现在应该可以让我出去玩会或者看下电视了。 )He has gone to Hong Kong.他已经去香港了。 (含义:结果是他不在这里,你在这儿见不到他了。 )模仿造句:1.杰克已把铅笔弄断了。 2.妈,我已经吃过中饭了。 3.玛丽已经去广州了。 3.现在完成时,表示某经历的时间长度(一般用for引导的时间状
语)Mr. Smith has been in China for 10 years.史密斯先生在中国待过10年了。 (含义:他待的时间够长的了,他对中国的情况很熟了,或者他的中文自然讲得很棒了。 )Jane has stayed in Shanghai for 3 days.珍妮在上海待了两天。 (含义:珍妮待在上海的时间短,对这里的情况还很不熟悉,或者她不应该马上离开,应该多玩几天。 )I have taught English for 10 years.我已经教英语10年了。 (含义:我教英语的时间已经非常长了,有丰富的教学经验了。 )模仿造句:1.简(Jane)已经在杭州住了8年了。 2.我妈已经在这家公司工作20年了。 3.今天我已经练英语口语2个小时了。 本文作者:丹丹英语(公众号:英语语法学习)本文已获转载授权,版权归作者所有,如需转载,请联系原微信公众号“英语语法学习。
现在进行时的用法六年级英语
课题:现在进行时用法课型:复习课学案号:22 科目:六年级英语主备人:王玉丽审核人:蒋连伟使用日期:2015.11.5 现在进行时专项练习: 现在进行时,用来表示正在进行或发生的动作。"结构是:be (am, is, are)+动词现在分词形式。 一、陈述句(肯定句) 主语+be(am, is, are)+现在分词,如: I am reading English.我正在读英语。He is writing.他正在写字。You are running.你正在跑步。 二、一般疑问句 Be(Am, Is, Are)+主语+现在分词,如: 1. -Are you singing?你正在唱歌吗? -Yes, I am.是的,我在唱歌。(No, I'm not.不,我不在唱歌。) 2. -Is he (she) listening to music?他(她)在听音乐吗? -Yes, he (she) is.是的,他(她)在听音乐。[No, he (she) isn't.不,他(她)不在听音乐。 三、特殊疑问句 疑问词+be (am, is, are)+主语+现在分词,如: 1. -What are you doing? 你正在干什么? -I am doing my homework.我正在做作业。 2. -What is he (she) doing?他(她)正在干什么? -He (She) is riding a bike.他(她)正在骑自行车。 四、现在进行时表示现在正在进行或发生的动作,也可表示当前一段时间内的活动或现阶段正在进行的动作。 五、动词加ing的变化规则 1.一般情况下,直接加ing,如:cook-cooking 以不发音的e结尾,去e加ing,如:make-making, taste-tasting 如果末尾是一个元音字母和一个辅音字母,双写末尾的辅音字母,再加ing,如:run-running, stop-stopping 六、需要掌握的进行时变化形式:sleeping, climbing, fighting, swinging, drinking water。 drawing pictures, doing the dishes, cooking dinner, reading a book, answering the phone,listening to music, washing clothes, cleaning the room, writing a letter, writing an e-mail,flying, jumping, walking, running, swimming,sleeping, climbing, fighting, swinging, drinking water. 其次,"我"向你们介绍"我"的三位好伙伴:look(看)、listen(听)、now(现在),它们和"我"经常出现在句子中。请看: Look! Jack is swimming.看!杰克正在游泳。 Listen! She is singing.听!她正在唱歌。 I am cleaning my room now.现在我正在打扫房间。 听了"我"的自述后,小朋友们,你们一定更加了解"我"了吧。 一、写出下列动词的现在分词: play_____________run______________swim_____________make______________ go______________ like____________ write_____________ _ski_____________ read_____________ have____________ sing _____________ dance___________ put______________ see____________ buy _____________ jog____________ live___________ take______________ come _____________ get_____________ stop____________ sit _____________ begin____________ shop___________ 二、用所给的动词的正确形式填空: 1.The boy __________________ ( draw)a picture now. 2. Listen .Some girls _______________ ( sing)in the classroom . 3. My mother _________________ ( cook )some nice food now. 4. What _____ you ______ ( do ) now? 5. Look . They _______________( have) an English lesson . 6.They ____________(not ,water) the flowers now. 7.Look! the girls ________________(dance )in the classroom . 8.What is our granddaughter doing? She _________(listen ) to music. 9. It's 5 o'clock now. We _____________(have)supper now 【学(教)后反思】