手机游戏设计与实现英文译文
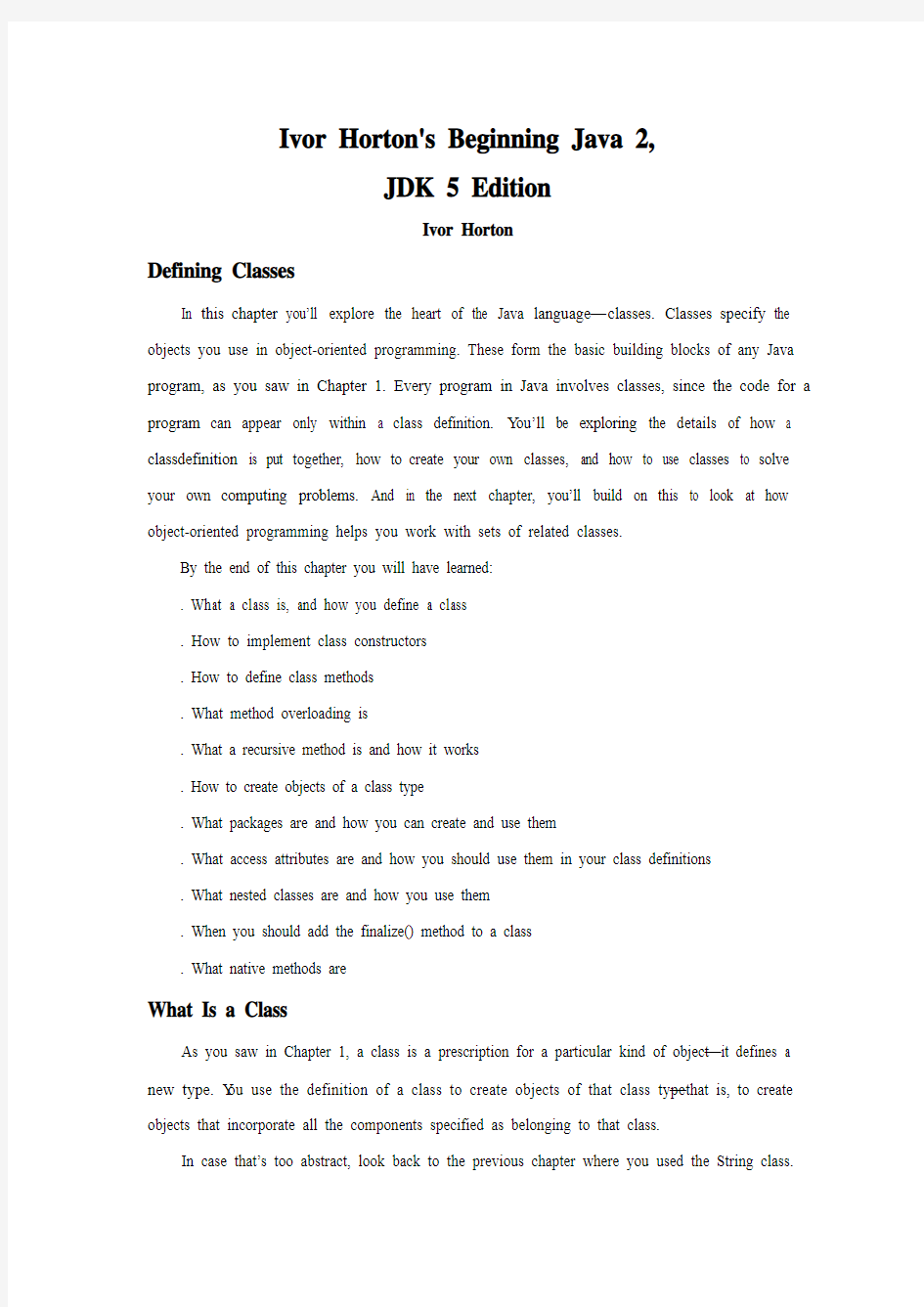
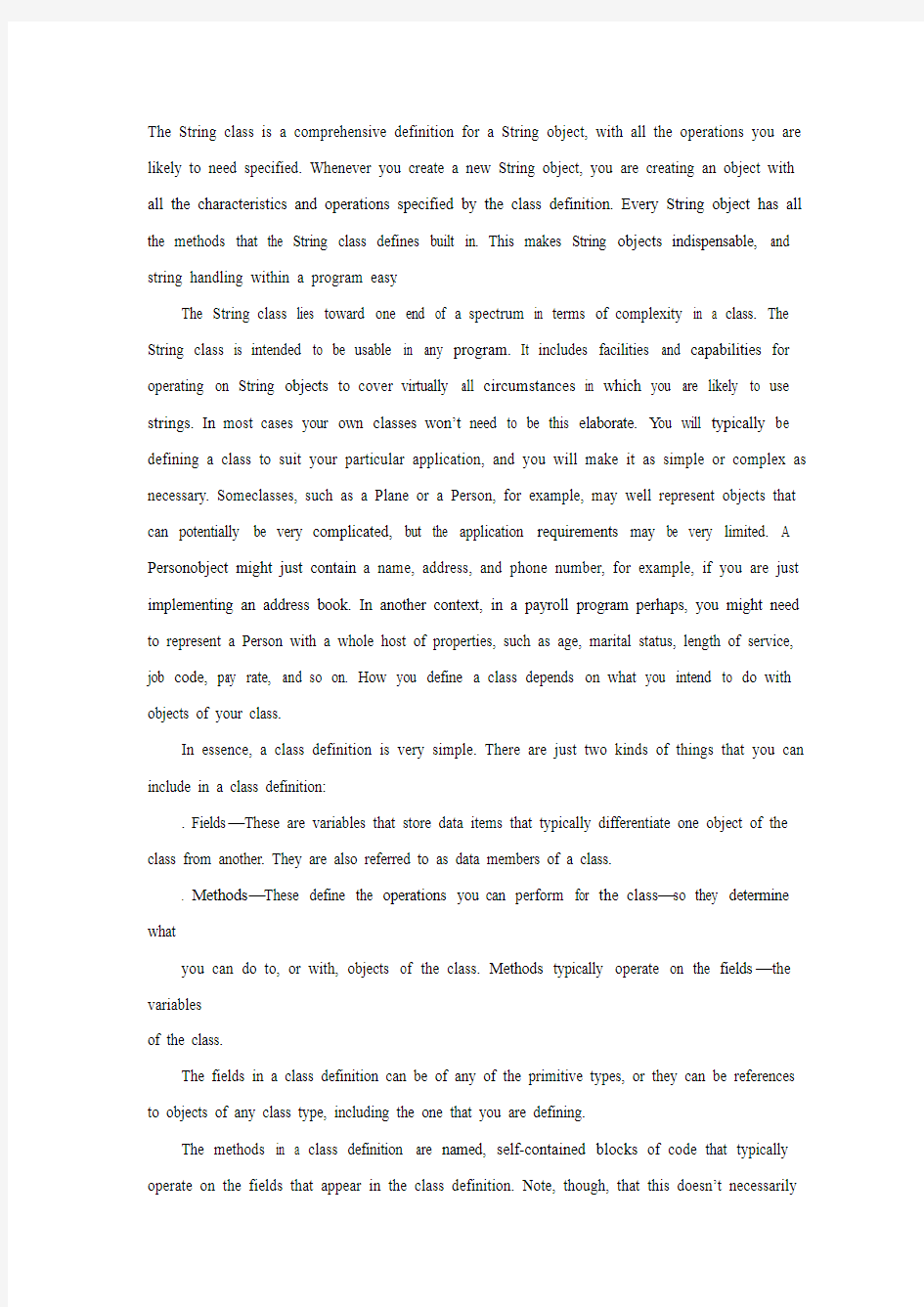
Ivor Horton's Beginning Java 2,
JDK 5 Edition
Ivor Horton
Defining Classes
In this chapter you’ll explore the heart of the Java language—classes. Classes specify the objects you use in object-oriented programming. These form the basic building blocks of any Java program, as you saw in Chapter 1. Every program in Java involves classes, since the code for a program can appear only within a class definition. Y ou’ll be exploring the details of how a classdefinition is put together, how to create your own classes, and how to use classes to solve your own computing problems. And in the next chapter, you’ll build on this to look at how object-oriented programming helps you work with sets of related classes.
By the end of this chapter you will have learned:
. What a class is, and how you define a class
. How to implement class constructors
. How to define class methods
. What method overloading is
. What a recursive method is and how it works
. How to create objects of a class type
. What packages are and how you can create and use them
. What access attributes are and how you should use them in your class definitions
. What nested classes are and how you use them
. When you should add the finalize() method to a class
. What native methods are
What Is a Class
As you saw in Chapter 1, a class is a prescription for a particular kind of object—it defines a new type. Y ou use the definition of a class to create objects of that class type—that is, to create objects that incorporate all the components specified as belonging to that class.
In case that’s too abstract, look back to the previous chapter where you used the String class.
The String class is a comprehensive definition for a String object, with all the operations you are likely to need specified. Whenever you create a new String object, you are creating an object with all the characteristics and operations specified by the class definition. Every String object has all the methods that the String class defines built in. This makes String objects indispensable, and string handling within a program easy.
The String class lies toward one end of a spectrum in terms of complexity in a class. The String class is intended to be usable in any program. It includes facilities and capabilities for operating on String objects to cover virtually all circumstances in which you are likely to use strings. In most cases your own classes won’t need to be this elaborate. Y ou will typically be defining a class to suit your particular application, and you will make it as simple or complex as necessary. Someclasses, such as a Plane or a Person, for example, may well represent objects that can potentially be very complicated, but the application requirements may be very limited. A Personobject might just contain a name, address, and phone number, for example, if you are just implementing an address book. In another context, in a payroll program perhaps, you might need to represent a Person with a whole host of properties, such as age, marital status, length of service, job code, pay rate, and so on. How you define a class depends on what you intend to do with objects of your class.
In essence, a class definition is very simple. There are just two kinds of things that you can include in a class definition:
. Fields—These are variables that store data items that typically differentiate one object of the class from another. They are also referred to as data members of a class.
. Methods—These define the operations you can perform for the class—so they determine what
you can do to, or with, objects of the class. Methods typically operate on the fields—the variables
of the class.
The fields in a class definition can be of any of the primitive types, or they can be references to objects of any class type, including the one that you are defining.
The methods in a class definition are named, self-contained blocks of code that typically operate on the fields that appear in the class definition. Note, though, that this doesn’t necessarily
have to be the case, as you might have guessed from the main() methods you have written in all the examples up to now.
Fields in a Class Definition
An object of a class is also referred to as an instance of that class. When you create an object, the object will contain all the fields that were included in the class definition. However, the fields in a class definition are not all the same—there are two kinds.
One kind of field is associated with the class, and is shared by all objects of the class. There is only one copy of each of these kinds of fields no matter how many class objects are created, and they exist even if no objects of the class have been created. This kind of variable is referred to as a class variable because the field belongs to the class and not to any particular object, although as I’ve said, all objects of the class will share it. These fields are also referred to as static fields because you use the static keyword when you declare them.
The other kind of field in a class is associated with each object uniquely—each instance of the class will have its own copy of each of these fields, each with its own value assigned. These fields differentiate one object from another, giving an object its individuality—the particular name, address, and telephone number in a given Person object, for example. These are referred to as non-static fields or instance variables because you specify them without using the static keyword, and each instance of a class type will have its own independent set.
Because this is extremely important to understand, let’s summarize the two kinds of fields that you can include in your classes:
. Non-static fields, also called instance variables—Each object of the class will have its own copy of each of the non-static fields or instance variables that appear in the class definition. Each object will have its own values for each instance variable. The name instance variable originates from the fact that an object is an instance or an occurrence of a class, and the values stored in the instance variables for the object differentiate the object from others of the same class type. An instance variable is declared within the class definition in the usual way, with a type name and a variable name, and can have an initial value specified.
. Static fields, also called class variables—A given class will have only one copy of each of its static fields or class variables, and these will be shared between and among all the objects of the
class. Each class variable exists even if no objects of the class have been created. Class variables belong to the class, and they can be referenced by any object or class method, not just methods belonging to instances of that class. If the value of a static field is changed, the new value is available equally in all the objects of the class. This is quite different from non-static fields, where changing a value for one object does not affect the values in other objects. A static field must be declared using the keyword static preceding the type name.
Why would you need two kinds of variables in a class definition? The instance variables are clearly necessary since they store the values that distinguish one particular object from another. The radius and the coordinates of the center of the sphere are fundamental to determining how big a particular Sphere object is, and where it is in space. However, although the variable PI is a fundamental parameter for every sphere—to calculate the volume, for example—it would be wasteful to store a value for PI in every Sphere object, since it is always the same. As you know, it is also available from the standard class Math so it is somewhat superfluous in this case, but you get the general idea. So one use for class variables is to hold constant values such as ( that are common to all objects of the class.
Another use for class variables is to track data values that are common to all objects of a class and that need to be available even when no objects have been defined. For example, if you wanted to keep a count of how many objects of a class have been created in your program, you could define a variable to store the count of the number of objects as a class variable. It would be essential to use a class variable, because you would still want to be able to use your count variable even when no objects have been declared.
Methods in a Class Definition
The methods that you define for a class provide the actions that can be carried out using the variables specified in the class definition. Analogous to the variables in a class definition are two varieties of methods—instance methods and class methods. Y ou can execute class methods even when no objects of a class exist, whereas instance methods can be executed only in relation to a particular object, so if no objects exist, you have no way to execute any of the instance methods defined in the class. Again, like class variables, class methods are declared using the keyword static, so they are sometimes referred to as static methods. Y ou saw in the previous chapter that the
valueOf() method is a static member of the String class.
Since static methods can be executed when there are no objects in existence, they cannot refer to instance variables. This is quite sensible if you think about it—trying to operate with variables that might not exist would be bound to cause trouble. In fact the Java compiler won’t let you try. If you reference an instance variable in the code for a static method, it won’t compile—you’ll just get an error message.The main() method, where execution of a Java application starts, must always be declared as static, as you have seen. The reason for this should be apparent by now. Before an application starts execution, no objects exist, so to start execution, you need a method that is executable even though there are no objects around—a static method therefore.
The Sphere class might well have an instance method volume() to calculate the volume of a particular object. It might also have a class method objectCount() to return the current count of how many objects of type Sphere have been created. If no objects exist, you could still call this method and get the count 0.
Note that although instance methods are specific to objects of a class, there is only ever one copy of each instance method in memory that is shared by all objects of the class, as it would be extremely expensive to replicate all the instance methods for each object. A special mechanism ensures that each time you call a method the code executes in a manner that is specific to an object, but I’ll defer explaining how this is possible until a little later in this chapter.
Apart from the main() method, perhaps the most common use for static methods is when you use a class just as a container for a bunch of utility methods, rather than as a specification for a set of objects. All executable code in Java has to be within a class, but lots of general-purpose functions you need don’t necessarily have an object association—calculating a square root, for example, or generating a random number. The mathematical functions that are implemented as class methods in the standard Math class are good examples. These methods don’t relate to class objects at all—they operate on values of the primitive types. Y ou d on’t need objects of type Math; you just want to use the methods from time to time, and you can do this as you saw in Chapter 2. The Math class also contains some class variables containing useful mathematical constants such as e and (.
Accessing V ariables and Methods
Y ou’ll normally want to access variables and methods that are defined within a class from outside it. Y ou will see later that it is possible to declare class members with restrictions on accessing them from outside, but let’s cover the pr inciples that apply where the members are accessible. I’ll consider accessing static members—that is, static fields and methods—and instance members separately.
Y ou can access a static member of a class using the class name, followed by a period, followed by the member name. With a class method you also need to supply the parentheses enclosing any arguments to the method after the method name. The period here is called the dot operator. So, if you wanted to calculate the square root of (, you could access the class method sqrt() and the class variable PI that are defined in the Math class as follows:
This shows how you call a static method—you just prefix it with the class name and put the dot operator between them. Y ou also reference the static data member, PI, in the same way—as Math.PI. If you have a reference to an object of a class type available, then you can also use that to access a static member of the class because every object always has access to the static members of its class. Y ou just use the variable name, followed by the dot operator, followed by the member name.
Of course, as you’ve seen in previous chapters, you can import the names of the static members of the class by using an import statement. Y ou can then refer to the names of the static members you have imported into your source file without qualifying their names at all.
Instance variables and methods can be called only using an object reference, because by definition they relate to a particular object. The syntax is exactly the same as I have outlined for static members. Y ou put the name of the variable referencing the object followed by a period, followed by the member name. To use a method volume() that has been declared as an instance method in the Sphere class, you might write:
double ballV olume = ball.volume();
Here the variable ball is of type Sphere and it contains a reference to an object of this type. Y ou call its volume() method, which calculates the volume of the ball object, and the result that is
returned is stored in the variable ballV olume.
Defining Classes
To define a class you use the keyword class followed by the name of the class, followed by a pair of braces enclosing the details of the definition. Let’s consider a concrete example to see ho w this works in practice. The definition of the Sphere class that I mentioned earlier could be: class Sphere {
static final double PI = 3.14; // Class variable that has a fixed value
static int count = 0; // Class variable to count objects
// Instance variables
double radius; // Radius of a sphere
double xCenter; // 3D coordinates
double yCenter; // of the center
double zCenter; // of a sphere
// Plus the rest of the class definition...
}
Y ou name a class using an identifier of the same sort y ou’ve been using for variables. By convention, though, class names in Java begin with a capital letter, so the class name is Sphere with a capital S. If you adopt this approach, you will be consistent with most of the code you come across. Y ou could enter this source code and save it as the file Sphere.java. Y ou’ll be adding to this class definition and using it in a working example a little later in this chapter.
Y ou may have noticed that in the examples in previous chapters the keyword public in this context preceded the keyword class in the first line of the class definition. The effect of the keyword public is bound up with the notion of a package containing classes, but I’ll defer discussing this until a little later in this chapter when you have a better idea of what makes up a class definition.
The keyword static in the first line of the Sphere class definition specifies the variable PI as a class variable rather than an instance variable. The variable PI is also initialized with the value 3.14. The keyword final tells the compiler that you do not want the value of this variable to be changed, so the compiler will check that this variable is not modified anywhere in your program.
Obviously, this is a very poor value for (. Y ou would normally use Math.PI—which is defined to 20 decimal places, close enough for most purposes.
Whenever you want to fix the initial value that you specify for a variable—that is, make it a constant—you just need to declare the variable with the keyword final. By convention, constants have names in capital letters.
Y ou have also declared the next variable, count, using the keyword static. All objects of the Sphere class will have access to and share the one copy of count and the one copy of PI that exist. Y ou have initialized the variable count to 0, but since you have not declared it using the keyword final, you can change its value.
The next four variables in the class definition are instance variables, as they don’t have the keyword static applied to them. Each object of the class will have its own separate set of these variables, storing the radius and the coordinates of the center of the sphere. Although you haven’t put initial values for these variables here, you could do so if you wanted. If you don’t specify an initial value, a default value will be assigned automatically when the object is created. Fields of numeric types will be initialized with zero, fields of type char will be initialized with ‘\u000’, and fields that store class references or references to arrays will be initialized with null.
产品设计中英文文献
中文译文 产品设计,语义和情绪反应 摘要 本文探讨了人体工程学理论与语义和情感容的设计问题。其目的是要找到以下问题的答案:如何设计产品引发人心中的幸福;怎样的产品属性能够帮助你们沟通积极的情绪,最后,如何通过产品唤起某种情绪。换言之,这是对“意义”——可以成为一个产品,旨在与用户在情感层面上进行“沟通”的调查。 1、介绍 当代生活是促进社会和技术变革的代名词。同样,产品设计通过材料技术,生产技术,信息处理技术等工序的发展而正在迅速转变。在技术方面正在发生变化的速度和规模超出任何期望。数字革命的对象是逐步转向与我们互动成更小,更聪明的黑盒子,使我们很难理解这一机制或工作方法(博尔茨2000年)。 因此,在设计时比以前不同的框架,参照社会变革,资源和能源节约,新出现的环境问题,以及客户导向的趋势(大平1995年,琼斯1997年)。因此,无论是通过广告和营销推动战略,或潮流,时尚和社会活动,从消费产品的用户的期望也已改变。功能性,吸引力,易于被使用中,可负担性,可回收性和安全性,预计所有已经存在于一个产品属性。用户希望有更多的日常用品。最近设计的趋势表明了用户对激励对象的倾向,提高他们的生活,帮助触发情绪,甚至唤起梦想(詹森1999年,阿莱西2000年)。詹森预计,梦会快到了,下面的数据为基础的社会,所谓的信息社会(1999年)。他还说,作为信息和智力正成为电脑和高科技,社会领域将放在一个人的能力还没有被自动然而新的价值:情绪。功能是越来越多的产品中理所当然的,同时用户也可以实现在寻找一个完全不同的欣赏水平。想象,神话和仪式(即情感的语言)会对我们的行为产生影响,从我们的购买决定,我们与他人(詹森1999年)的沟通。此外,哈立德(2001:196)指出这是决定购买,可瞬间的,因此客户的需求可以被创建,速度非常快,而其他需要长期建立了'。 因此,情感和'影响'一般,都收到了最后一个(Velásquez1998)几年越来越多的关注。'影响'是指消费者的心理反应产品的符号学的容。情绪和影响途径,可以研究在许多不同的层次,都提供不同的见解。正如Velásquez指出,一些模型已经被提出来的领域和多种环境。一些例子,他给包括情绪来创建逼真的品质和性格(贝茨1994年,克莱恩和布隆伯格1999年,埃利奥特1992年,赖利1996)系统合成剂,大约在叙事情感(艾略特等人在Velásquez1998年)。原因,在情绪处理系统,依靠调解社会互动(Breazeal 在Velásquez1998年),该模型和体系结构,行为和学习情绪的影响(Ca?mero1997年,
室内设计空间-中英文对照1
室内设计空间-中英文对照 General: 一般 Plan - 平面图 Elevation -立面图 Section - 剖面图 Detail Drawing- 大样图 Ceiling Plan - 天花图 Plan: 平面 lighting layout Plan - 灯光设计图 Electrical Layout Plan - 电图 (一般指带有电制-socket 的图) Fire Service Layout Plan - 消防系统 MVAC Layout Plan - 空调系统 Detail Drawing: 详图 Floor Pattern Detail - 地板图 Stone Pattern Detail - 石图 Schedule: ( 附助图表 ) Lighting Schedule - 灯具表 1\ Florescent Light 2\ Spot Light (directional /non-directional) 3\ Light trough Socket Schedule - 电气表 Window and Door Schedule - 门窗表 Hardware Schedule - 五金器具表 Sanitary fixture Schedule - 洁具(卫生设备)表 家居篇: Living Room - 客厅 Dining Room - 饭厅 foyer - 玄关 Kitchen – Bath - 厕所、浴室 Study - 书房 Store - 贮物室 Master Bed Room - 主人房 Guest Bed Room - 客房 Suite - 套房 Balcony - 露台
包装设计外文翻译文献
包装设计外文翻译文献(文档含中英文对照即英文原文和中文翻译)
包装对食品发展的影响 消费者对某个产品的第一印象来说包装是至关重要的,包括沟通的可取性,可接受性,健康饮食形象等。食品能够提供广泛的产品和包装组合,传达自己加工的形象感知给消费者,例如新鲜包装/准备,冷藏,冷冻,超高温无菌,消毒(灭菌),烘干产品。 食物的最重要的质量属性之一,是它的味道,其影响人类的感官知觉,即味觉和嗅觉。味道可以很大程度作退化的处理和/或扩展存储。其他质量属性,也可能受到影响,包括颜色,质地和营养成分。食品质量不仅取决于原材料,添加剂,加工和包装的方法,而且其预期的货架寿命(保质期)过程中遇到的运输和储存条件的质量。越来越多的竞争当中,食品生产商,零售商和供应商;和质量审核供应商有着显著的提高食品质量以及急剧增加包装食品的选择。这些改进也得益于严格的冷藏链中的温度控制和越来越挑剔的消费者。 保质期的一个定义是:在规定的贮存温度条件下产品保持其质量和安全性的时间。在保质期内,产品的生产企业对该产品质量符合有关标准或明示担保的质量条件负责,销售者可以放心销售这些产品,消费者可以安全使用。 保质期不是识别食物等产品是否变质的唯一标准,可能由于存放方式,环境等变化物质的过早变质。所以食物等尽量在保质期未到期就及时食用。包装产品的质量和保质期的主题是在第3章中详细讨论。
包装为消费者提供有关产品的重要信息,在许多情况下,使用的包装和/或产品,包括事实信息如重量,体积,配料,制造商的细节,营养价值,烹饪和开放的指示,除了法律准则的最小尺寸的文字和数字,有定义的各类产品。消费者寻求更详细的产品信息,同时,许多标签已经成为多语种。标签的可读性会是视觉发现的一个问题,这很可能成为一个对越来越多的老年人口越来越重要的问题。 食物的选择和包装创新的一个主要驱动力是为了方便消费者的需求。这里有许多方便的现代包装所提供的属性,这些措施包括易于接入和开放,处置和处理,产品的知名度,再密封性能,微波加热性,延长保质期等。在英国和其他发达经济体显示出生率下降和快速增长的一个相对富裕的老人人口趋势,伴随着更加苛刻的年轻消费者,他们将要求和期望改进包装的功能,如方便包开启(百货配送研究所,IGD)。 对零售商而言存在有一个高的成本,供应和服务的货架体系。没有储备足够的产品品种或及时补充库存,特别是副食品,如鲜牛奶,可能导致客户不满和流失到竞争对手的商店,这正需要保证产品供应。现代化的配送和包装系统,允许消费者在购买食品时,他们希望在他们想任何时间地点都能享用。近几年消费者的选择已在急剧扩大。例如在英国,20世纪60年代和90年代之间在一般超市的产品线的数量从2000年左右上升到超过18000人(INCPEN)。 自20世纪70年代以来,食品卫生和安全问题已成为日益重要的关注和选择食物的驱动力。媒体所关注的一系列问题,如使用化学添
步进电机及单片机英文文献及翻译
外文文献: Knowledge of the stepper motor What is a stepper motor: Stepper motor is a kind of electrical pulses into angular displacement of the implementing agency. Popular little lesson: When the driver receives a step pulse signal, it will drive a stepper motor to set the direction of rotation at a fixed angle (and the step angle). You can control the number of pulses to control the angular displacement, so as to achieve accurate positioning purposes; the same time you can control the pulse frequency to control the motor rotation speed and acceleration, to achieve speed control purposes. What kinds of stepper motor sub-: In three stepper motors: permanent magnet (PM), reactive (VR) and hybrid (HB) permanent magnet stepper usually two-phase, torque, and smaller, step angle of 7.5 degrees or the general 15 degrees; reaction step is generally three-phase, can achieve high torque output, step angle of 1.5 degrees is generally, but the noise and vibration are large. 80 countries in Europe and America have been eliminated; hybrid stepper is a mix of permanent magnet and reactive advantages. It consists of two phases and the five-phase: two-phase step angle of 1.8 degrees while the general five-phase step angle of 0.72 degrees generally. The most widely used Stepper Motor. What is to keep the torque (HOLDING TORQUE) How much precision stepper motor? Whether the cumulative: The general accuracy of the stepper motor step angle of 3-5%, and not cumulative.
机械毕业设计英文外文翻译71车床夹具设计分析
附录A Lathe fixture design and analysis Ma Feiyue (School of Mechanical Engineering, Hefei, Anhui Hefei 230022, China) Abstract: From the start the main types of lathe fixture, fixture on the flower disc and angle iron clamp lathe was introduced, and on the basis of analysis of a lathe fixture design points. Keywords: lathe fixture; design; points Lathe for machining parts on the rotating surface, such as the outer cylinder, inner cylinder and so on. Parts in the processing, the fixture can be installed in the lathe with rotary machine with main primary uranium movement. However, in order to expand the use of lathe, the work piece can also be installed in the lathe of the pallet, tool mounted on the spindle. THE MAIN TYPES OF LATHE FIXTURE Installed on the lathe spindle on the lathe fixture
室内设计中英文对照
常用室内设计词汇-中英文对照 室内设计-interior design 室内设计师- interior designer 建筑-architecture 建筑师-architect 景观-landscape 家具-furniture 灯光-lighting 照明-illumination 家居设计-residential design 商业设计-commercial design 软装-FF&E(furniture fixture and equipment) 人体工程学-ergonomics 空间-space 精品酒店-boutique hotel 草图-draft or sketch 规格-specification 汇报或讲解-presentation 渲染或着色-rendering 透视-perspective 规范-code 对称-symmetry 不对称-asymmetry 轴线-axis 空间篇 住宅类 客厅,起居室—living room 餐厅-dinning room 玄关-foyer 卧室-bedroom 主卧-master bedroom 次卧-second bedroom 客卧-guest bedroom 厨房-Kitchen 厨岛kitchen island 书房-study room 衣帽间-cloakroom 卫生间-bathroom , toilet , bath 储藏室-storage 楼梯-stair 阳台-balcony 花园-garden 露台-patio 商业类 1办公类 接待处- reception 候客区-waiting Area or lounge 会议室(小型的)-meeting room or seminar 会议室(大型的)-conference room 办公室- office 经理办公室manager office 开放式的工作区-work area 多功能室-multi-function room 2酒店类 入口-entrance 出口-exit 大堂-lobby 前厅-vestibule 过道-corridor 休闲区,等候区-lounge 宴会厅-ballroom 客房-guestroom 套间-suite 行政套房-executive suite 总统套房-presidential suite 健身中心—fitness center or gym 瑜伽-yoga 泳池-swimming pool Spa 咖啡厅-cafe 酒吧-bar 餐厅-restaurant 备餐-pantry 电梯-elevation or lift 卫生间-restroom 男-men's 女women's 影院-cinema 商务中心-business center 行李间-luggage store 盥洗室-lavatory 3其他类 天花-ceiling 长廊-pavilion 零售店-retail store 大厅(堂)-hall 展览-gallery
产品设计外文文献及翻译
英文原文 Modern product design ---Foreign language translation original text With the growing of economices and the developing of technologies, the essential definition of Industral Design has been deepening while its extension meaning enlarging,which resulted in the transformation and renovation of some original design theories and concepts. In the new IT epoch, the contents, methodologies, concepts etc. of design have taken a great change from what they were before.However,the method of comparison and analysis is always playing a plvotal role, during the whole process of maintaining the traditional quintessence and innovating novel conceptions. 1.1 Traditional Design Traditional industrial design and product development mainly involved to three fields,vis.Art, Engineering and Marketing. The designers, who worked in the art field, always had outstanding basic art skills and visual sketching expression capacity as well as plentiful knowledge on ergonomics and aesthetics . So they could easily solve the problems of products about art . Works in the area of the project engineer with strong technical background, they used the method of logical analysis, you can design a detailed, in line with the requirements of the drawings of a total production, manufacture use. They can you good solution to the technical aspects of products. However, they often overlook the aesthetics of products that do not pay attention to fashion and cost-effective products in the market. In the field of commercial marketing staff proficient in the knowledge economy, will use marketing theory to predict customer behavior, they focus on products in the market development trends, but do not understand aesthetic and technical aspects of the problem. In a traditional industrial product design process, the three areas of general staff in their respective areas of independent work. Product engineers solve the technical problems so that products with the necessary functional and capable of producing manufactured, the product is "useful." Designers are using aesthetics,
at89c52单片机中英文资料对照外文翻译文献综述
at89c52单片机简介 中英文资料对照外文翻译文献综述 A T89C52 Single-chip microprocessor introduction Selection of Single-chip microprocessor 1. Development of Single-chip microprocessor The main component part of Single-chip microprocessor as a result of by such centralize to be living to obtain on the chip,In immediate future middle processor CPU。Storage RAM immediately﹑memoy read ROM﹑Interrupt system、Timer /'s counter along with I/O's rim electric circuit awaits the main microcomputer section,The lumping is living on the chip。Although the Single-chip microprocessor r is only a chip,Yet through makes up and the meritorous service be able to on sees,It had haveed the calculating machine system property,calling it for this reason act as Single-chip microprocessor r minisize calculating machine SCMS and abbreviate the Single-chip microprocessor。 1976Year the Inter corporation put out 8 MCS-48Set Single-chip microprocessor computer,After being living more than 20 years time in development that obtain continuously and wide-ranging application。1980Year that corporation put out high performance MCS -51Set Single-chip microprocessor。This type of Single-chip microprocessor meritorous service capacity、The addressing range wholly than early phase lift somewhat,Use also comparatively far more at the moment。1982Year that corporation put out the taller 16 Single-chip microprocessor MCS of performance once
室内设计 外文翻译 外文文献 英文文献 自然 简约—对室内设计现象分析
室内设计外文翻译外文文献英文文献自然简约—对 室内设计现象分析 附件2:外文原文 Natural simplicity - on interior design Analysis Abstract: The natural, simple interior design show is a way of life, it allows us closer to nature, more emphasis on functionality, more concerned about life itself. create a poetic space. Keywords: minimalism; space; grade; interior design; feeling Ancient times, Chinese wooden framework architecture of ancient India, the Orient, Europe, building caves in ancient Greece, ancient Rome and so on decorative stone building closely integrated with the components, with the main building, however. dissolved into Europe in the early seventeenth century Baroque times and the mid-eighteenth century the Rococo era, began with the interior decoration of the main building separated from the main building external and internal fitting-out period in the use of the mismatch, thus leading to the main building and interior decoration of the separation, in the construction of the French court architecture and aristocratic mansion, the new occupation "decorative artisan" was born, the building's internal frequency continuous modification, fixed the main building, the replacement building, "clothing" the time has come. Baroque-style architecture of
机械设计外文翻译(中英文)
机械设计理论 机械设计是一门通过设计新产品或者改进老产品来满足人类需求的应用技术科学。它涉及工程技术的各个领域,主要研究产品的尺寸、形状和详细结构的基本构思,还要研究产品在制造、销售和使用等方面的问题。 进行各种机械设计工作的人员通常被称为设计人员或者机械设计工程师。机械设计是一项创造性的工作。设计工程师不仅在工作上要有创造性,还必须在机械制图、运动学、工程材料、材料力学和机械制造工艺学等方面具有深厚的基础知识。如前所诉,机械设计的目的是生产能够满足人类需求的产品。发明、发现和科技知识本身并不一定能给人类带来好处,只有当它们被应用在产品上才能产生效益。因而,应该认识到在一个特定的产品进行设计之前,必须先确定人们是否需要这种产品。 应当把机械设计看成是机械设计人员运用创造性的才能进行产品设计、系统分析和制定产品的制造工艺学的一个良机。掌握工程基础知识要比熟记一些数据和公式更为重要。仅仅使用数据和公式是不足以在一个好的设计中做出所需的全部决定的。另一方面,应该认真精确的进行所有运算。例如,即使将一个小数点的位置放错,也会使正确的设计变成错误的。 一个好的设计人员应该勇于提出新的想法,而且愿意承担一定的风险,当新的方法不适用时,就使用原来的方法。因此,设计人员必须要有耐心,因为所花费的时间和努力并不能保证带来成功。一个全新的设计,要求屏弃许多陈旧的,为人们所熟知的方法。由于许多人墨守成规,这样做并不是一件容易的事。一位机械设计师应该不断地探索改进现有的产品的方法,在此过程中应该认真选择原有的、经过验证的设计原理,将其与未经过验证的新观念结合起来。 新设计本身会有许多缺陷和未能预料的问题发生,只有当这些缺陷和问题被解决之后,才能体现出新产品的优越性。因此,一个性能优越的产品诞生的同时,也伴随着较高的风险。应该强调的是,如果设计本身不要求采用全新的方法,就没有必要仅仅为了变革的目的而采用新方法。 在设计的初始阶段,应该允许设计人员充分发挥创造性,不受各种约束。即使产生了许多不切实际的想法,也会在设计的早期,即绘制图纸之前被改正掉。只有这样,才不致于堵塞创新的思路。通常,要提出几套设计方案,然后加以比较。很有可能在最后选定的方案中,采用了某些未被接受的方案中的一些想法。
MCS_51系列单片机中英文资料对照外文翻译文献综述
MCS-51系列单片机 中英文资料对照外文翻译文献综述 Structure and function of the MCS-51 series Structure and function of the MCS-51 series one-chip computer MCS-51 is a name of a piece of one-chip computer series which Intel Company produces. This company introduced 8 top-grade one-chip computers of MCS-51 series in 1980 after introducing 8 one-chip computers of MCS-48 series in 1976. It belong to a lot of kinds this line of one-chip computer the chips have, such as 8051, 8031, 8751, 80C51BH, 80C31BH,etc., their basic composition, basic performance and instruction system are all the same.8051 daily representatives-51 serial one-chip computers. A one-chip computer system is made up of several following parts: (1) One microprocessor of 8 (CPU). ( 2) At slice data memory RAM (128B/256B),it use not depositing not can reading /data that write, such as result not middle of operation, final result and data wanted to show, etc. (3) Procedure memory ROM/EPROM (4KB/8K B ), is used to preserve the
基于solidworks机床夹具设计外文翻译详解
2604130359 CNC Cutting Technology Review Numerical control high speed cutting technology (High Speed Machining, HSM, or High Speed Cutting, HSC), is one of the advanced manufacturing technology to improve the machining efficiency and quality, the study of related technology has become an important research direction of advanced manufacturing technology at home and abroad. China is a big manufacturing country, in the world of industry transfer to accept the front instead of back-end of the transfer, to master the core technology of advanced manufacturing, or in a new round of international industrial structure adjustment, our country manufacturing industry will further behind. Imminent research on the theory and application of advanced technology. 1, high-speed CNC machining meaning High speed cutting theory put forward by the German physicist Carl.J.Salomon in the last century and early thirty's. He concluded by a lot of experiments: in the normal range of cutting speed, cutting speed if the increase, will cause the cutting temperature rise, exacerbating the wear of cutting tool; however, when the cutting speed is increased to a certain value, as long as more than the inflection point, with the increase of the cutting speed, cutting temperature can not rise, but will decline, so as long as the cutting speed is high enough, it can be solved very well in high cutting temperature caused by tool wear is not conducive to the cutting problem, obtained good processing efficiency. With the development of manufacturing industry, this theory is gradually paid more attention to, and attracted a lot of attention, on the basis of this theory has gradually formed the field of high-speed cutting technology of NC, relatively early research on NC High-speed Machining Technology in developed countries, through the theoretical basis of the research, basic research and applied research and development application, at present applications have entered the substantive stage in some areas. The high-speed cutting processing category, generally have the following several kinds of classification methods, one is to see that cutting speed, cutting speed over conventional cutting speed is 5-10 times of high speed cutting. Also has the scholar to spindle speed as the definition of high-speed processing standards, that the spindle speed is higher than that of 8000r\/min for high speed machining. And from the machine tool spindle design point of view, with the product of DN diameter of spindle and spindle speed, if the value of DN to (5~2000) * 105mm.r\/min, is considered to be of high speed machining. In practice, different processing methods, different materials, high speed cutting speed corresponding to different. Is generally believed that the turning speed of (700~7000) m\/min, milling speed reaches m\/min (300~6000), that is in the high-speed cutting. In addition, from the practical considerations, high-speed machining concept not only contains the high speed cutting process, integration and optimization also contains the process of cutting, is a
室内设计中英文翻译
毕业设计英文资料翻译 Translation of the English Documents for Graduation Design Interior Design Susan Yelavich Interior design embraces not only the decoration and furnishing of space, but also considerations of space planning, lighting, and programmatic issues pertaining to user behaviors, ranging from specific issues of accessibility to the nature of the activities to be conducted in the space. The hallmark of interior design today is a new elasticity in typologies, seen most dramatically in the domestication of commercial and public spaces. Interior design encompasses both the programmatic planning and physical treatment of interior space: the projection of its use and the nature of its furnishings and surfaces, that is, walls, floors, and ceilings. Interior design is distinguished from interior decoration in the scope of its purview. Decorators are primarily concerned with the selection of furnishings, while
设计类外文翻译
贯穿绿色工程的原则 近年来,许多报纸、书籍、和会议的主题围绕出资行星上的负面影响,人类在其能力来维系生命。常,这些讨论,具体目标也应运而生,如,最大限度地减少浪费,接近可持续发展。目标语句可以提供非常有用的一个视觉所需要的实现,而且多数的这些的重要组成部分,有助于视力。然而,目标是当他们成为才生效。方法正在研制达到这些目标跨学门、工业、和部门。很明显,然而,这些方法目前既不系统也不全面。 绿色工程()着重论述如何通过科学和技术能否实现可持续发展( )。原则的绿色工程(参见页),提供了一个框架下对科学家和从事在设计新材料、产品、过程和系统,是“良性”健康和环境。一个设计基于原则行走基线工程质量和安全规格以、经济、社会因素的影响。宽的适用性的原则是重要的。当处理设计建筑,不管它是化合物分子结构要求、产品体系结构,以创造一个汽车、城市新建筑的建造一个相同的绿色工程原则必须适用、有效和适当的。否则,这些不会原则,而是一个列表的技术,已经成功地特定条件下。在这篇文章中,我们说明这些原则可以应用在一系列的鳞片。也适用于查看原则在一个复杂的、完整的体系。就像每一个参数优化系统不能在任何一个时间,特别是当他们在,也是同样的道理这些原则。有一些案例表明,协同的成功应用的一个最重要的原则提出了一个或更多的其他人。在其他情况下,一个平衡的原则将再保险——优化系统整体解决方案。然而,也有一些两个基本概念应该努力整合在每:生命周期的考虑和第一的原则的绿色工程。 生命周期和 材料和能量的生命周期阶段进入每一个每一件产品和过程有他们自己的生活周期。如果一个产品是有益于环保但是由危险废物或不可再生物质,其基本上已被转移到另一边的整体生活周期。例如,如果一个产品或过程具有高效节能甚至能源发电(例如),但生产过程中影响程度抵消任何能量的收获,没有樊笼可持续发展优势。因此应该考虑的整个生命周期,包括物料及能源的投入。生命周期的物料及能源开始采集(例如,挖掘、钻孔、收获),然后移动整个制造,分销,使用,和结束的生命。这是必须考虑所有的申请时,是需要绿色工程的原则。这一策略的选择进行了补充,更能减低我们固有的良性输入在生命周期阶段的环境影响。使产品、过程和系统更有益于环保一般情况下遵循的两个基本方法:变化的内在属性的系统或改变的情况下条件时才能被感觉到的制度。虽然可能固有的毒性,降低;一个有条件的一种化学物质,包括控制变化的,以及是否接触,有毒化学物质。者优先,原因也是多方面的,最重要的是,以避免“失败”。依靠控制系统环境,如空气净化器或废水的处理,有一个潜在的失败,这可能导致重大危险到人健康和自然系统。然而,随着更多的良性设计,无论在条件或情况下,变化的本质系统不能失败。 在这些情况下,该系统的固有属性是预先定义的,因此,它经常是很必要的,通过提高系统的变化情况和条件。虽然技术和经济因素的影响,往往可以排除通过系统设计具有更多的固有的良性、渐进性的变革在这种情况下,可以有很重大的影响,整个系统。选择一个之间的个人设计在最有益于环保和可持续的方式与设计了一个汽油发动的运动型多用途车是最能符合可持续发展。原则提供了一个绿色工程结构制造出和评估这些设计元素相关的最大限度的可持续发展。工程师可以使用这些原则为指导,以帮助确保设计的产品、过程或系统有基本的元件,条件,和被需要更稳定。 原则 虽然带来的负面影响的固有的有害物质(无论是毒理、乃至全球)可能会降到最低,这是