实验一 Java常用工具类编程
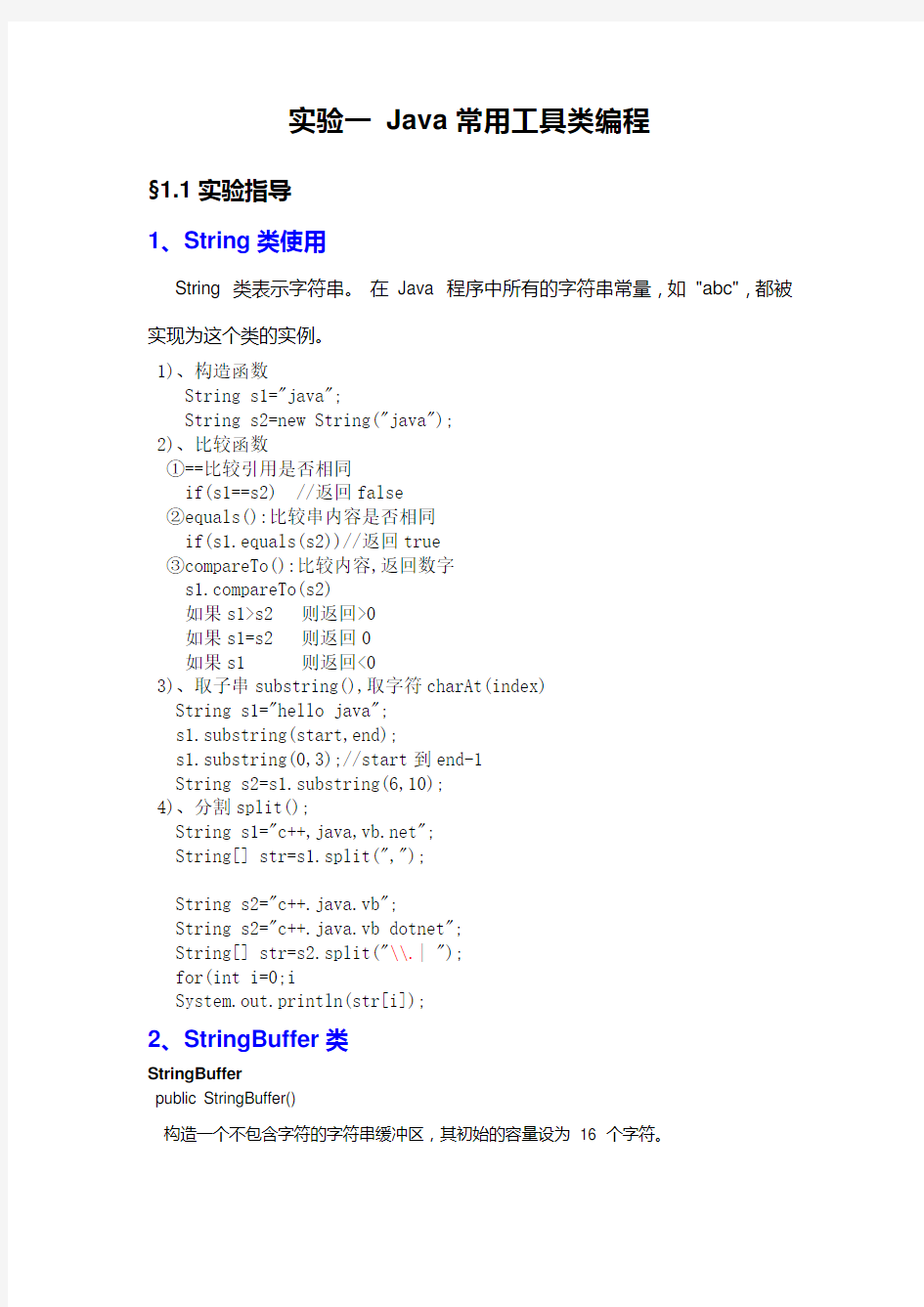
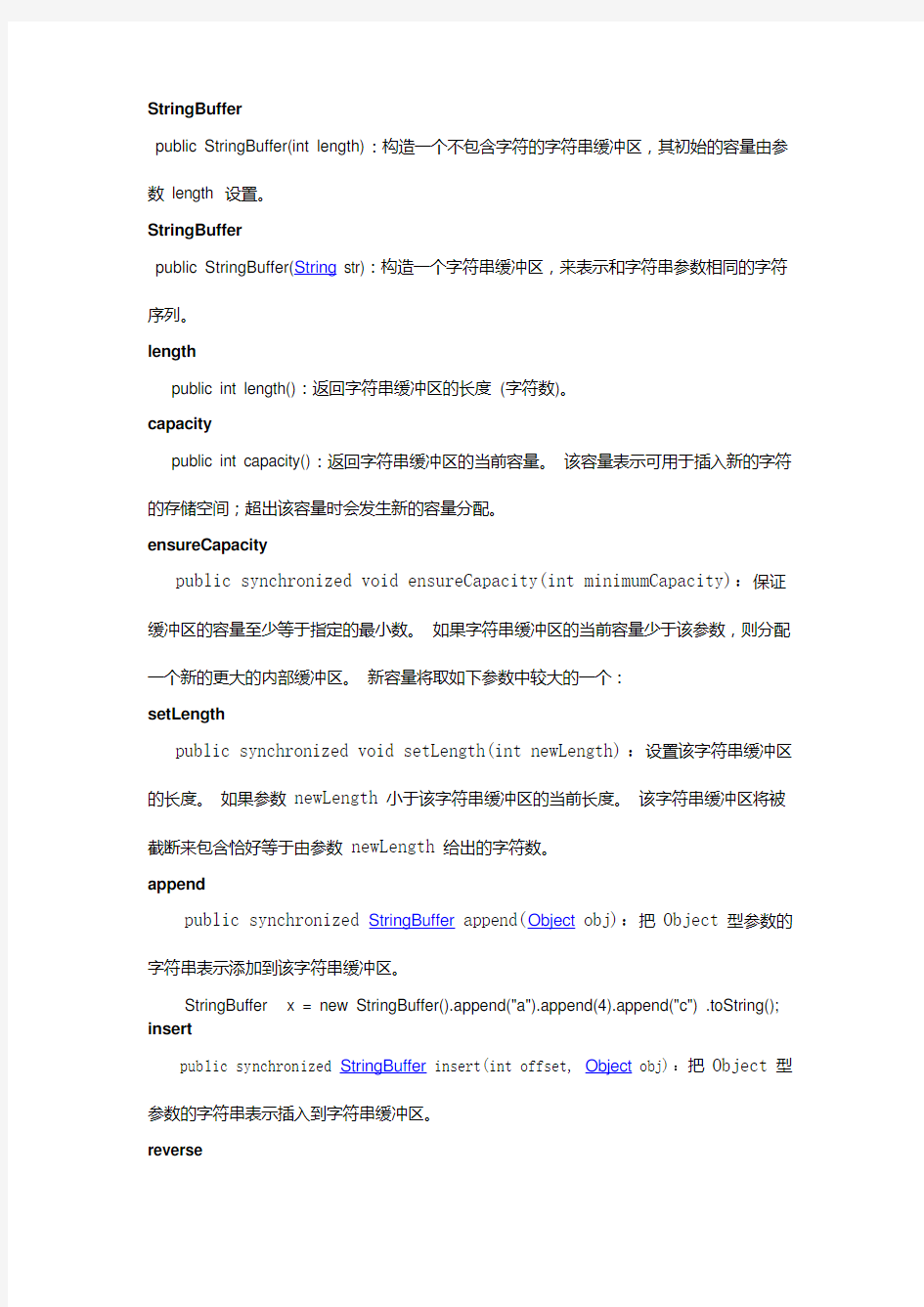
实验一Java常用工具类编程
§1.1实验指导
1、String类使用
String 类表示字符串。在Java 程序中所有的字符串常量,如"abc",都被实现为这个类的实例。
1)、构造函数
String s1="java";
String s2=new String("java");
2)、比较函数
①==比较引用是否相同
if(s1==s2) //返回false
②equals():比较串内容是否相同
if(s1.equals(s2))//返回true
③compareTo():比较内容,返回数字
https://www.360docs.net/doc/fc4630968.html,pareTo(s2)
如果s1>s2 则返回>0
如果s1=s2 则返回0
如果s1 3)、取子串substring(),取字符charAt(index) String s1="hello java"; s1.substring(start,end); s1.substring(0,3);//start到end-1 String s2=s1.substring(6,10); 4)、分割split(); String s1="c++,java,https://www.360docs.net/doc/fc4630968.html,"; String[] str=s1.split(","); String s2="c++.java.vb"; String s2="c++.java.vb dotnet"; String[] str=s2.split("\\.| "); for(int i=0;i System.out.println(str[i]); 2、StringBuffer类 StringBuffer public StringBuffer() 构造一个不包含字符的字符串缓冲区,其初始的容量设为16 个字符。 StringBuffer public StringBuffer(int length):构造一个不包含字符的字符串缓冲区,其初始的容量由参数length 设置。 StringBuffer public StringBuffer(String str):构造一个字符串缓冲区,来表示和字符串参数相同的字符序列。 length public int length():返回字符串缓冲区的长度(字符数)。 capacity public int capacity():返回字符串缓冲区的当前容量。该容量表示可用于插入新的字符的存储空间;超出该容量时会发生新的容量分配。 ensureCapacity public synchronized void ensureCapacity(int minimumCapacity):保证缓冲区的容量至少等于指定的最小数。如果字符串缓冲区的当前容量少于该参数,则分配一个新的更大的内部缓冲区。新容量将取如下参数中较大的一个: setLength public synchronized void setLength(int newLength):设置该字符串缓冲区的长度。如果参数newLength小于该字符串缓冲区的当前长度。该字符串缓冲区将被截断来包含恰好等于由参数newLength给出的字符数。 append public synchronized StringBuffer append(Object obj):把Object型参数的字符串表示添加到该字符串缓冲区。 StringBuffer x = new StringBuffer().append("a").append(4).append("c") .toString(); insert public synchronized StringBuffer insert(int offset, Object obj):把Object型参数的字符串表示插入到字符串缓冲区。 reverse public synchronized StringBuffer reverse():该字符串缓冲区的字符序列被其反向字符序列所替换。 toString public String toString():转换为一个表示该字符串缓冲区数据的字符串。分配一个新的String对象,并且用字符串缓冲区所表示的字符序列进行初始化。于是此String 被返回。随后缓冲区发生的变化不再影响该String 的内容。 3、日期类示例 1)获取服务器端当前日期: import java.util.Date; Date myDate = new Date(); 2) 获取当前年、月、日: Date myDate = new Date(); int thisYear = myDate.getYear() + 1900;//thisYear = 2009 int thisMonth = myDate.getMonth() + 1;//thisMonth = 10 int thisDate = myDate.getDate();//thisDate = 30 3)按本地时区输出当前日期 Date myDate = new Date(); out.println(myDate.toLocaleString()); 输出结果为: 2003-5-30 4)按照指定格式打印日期 import "java.util.Date"; import "java.text.DateFormat"; Date dNow = new Date(); SimpleDateFormat formatter = new SimpleDateFormat("E yyyy.MM.dd 'at' hh:mm:ss a zzz"); System.out.println("It is " + formatter.format(dNow)); 输出的结果为: It is 星期五2003.05.30 at 11:30:46 上午CST 5)将字符串转换为日期 import="java.util.Date" import="java.text.DateFormat" String input = "1222-11-11"; SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); Date t = null; try{ t = formatter.parse(input); System.out.println(t); }catch(ParseException e){ System.out.println("unparseable using" + formatter); } 输出结果为: Fri Nov 11 00:00:00 CST 1222 6) 计算日期之间的间隔getTime()函数返回日期与1900-01-01 00:00:00相差的毫秒数 import="java.util.Date" import="java.text.DateFormat" String input = "2003-05-01"; SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); Date d1 = null; Date d2 = new Date();long diff = d2.getTime() - d1.getTime(); out.println("Difference is " + (diff/(1000*60*60*24)) + " days."); 输出结果为: Difference is 29 days. 7) 日期的加减运算 方法:用Calendar类的add()方法 Calendar now = Calendar.getInstance(); SimpleDateFormat formatter = new SimpleDateFormat("E yyyy.MM.dd 'at' hh:mm:ss a zzz"); out.println("It is now " + formatter.format(now.getTime())); now.add(Calendar.DAY_OF_YEAR,-(365*2)); out.println(" out.println("Two years ago was " + formatter.format(now.getTime())); 输出结果为: It is now 星期五2003.05.30 at 01:45:32 下午CST Two years ago was 星期三2001.05.30 at 01:45:32 下午CST 8) 比较日期 方法:用equals()、before()、after()方法 import="java.util.*" import="java.text.*" DateFormat df = new SimpleDateFormat("yyy-MM-dd"); Date d1 = df.parse("2000-01-01"); Date d2 = df.parse("1999-12-31"); String relation = null; if(d1.equals(d2)) relation = "the same date as"; else if(d1.before(d2)) relation = "before"; else relation = "after"; System.out.println(d1 +" is " + relation + ' ' + d2); 输出结果为: Sat Jan 01 00:00:00 CST 2000 is after Fri Dec 31 00:00:00 CST 1999 §1.2实验题目 1、使用类String类的分割split 将字符串“Solutions to selected exercises can be found in the electronic document The Thinking in Java Annotated Solution Guide, available for a small fee from BruceEckel”单词提取输出。单词以空格或,分割。 package job1; public class Split { public static void main(String[] args){ String s1="Solutions to selected exercises " + "can be found in the electronic document " + "The Thinking in Java Annotated Solution Guide, " + "available for a small fee from BruceEckel"; String s2[]=s1.split(" |,"); for(int i=0;i System.out.println(s2[i]); } } 2、设计一个类Student,类的属性有:姓名,学号,出生日期,性别,所在系等。并生成学 生类对象数组。按照学生的姓名将学生排序输出。使用String类的compareT o方法。package job1; public class Student { private String name; private int num; private String birth; private String sex; private String sdept; public Student(){ } public Student(String name,int num,String birth,String sex,String sdepet){ https://www.360docs.net/doc/fc4630968.html,=name; this.num=num; this.birth=birth; this.sex=sex; this.sdept=sdept; } public String getName(){ return name; } public void setName(String name){ https://www.360docs.net/doc/fc4630968.html,=name; } public int getNum(){ return num; } public void setNum(int num){ this.num=num; } public String getBirth(){ return birth; } public void setBirth(String birth){ this.birth=birth; } public String getSex(){ return sex; } public void setSex(String sex){ this.sex=sex; } public String getSdept(){ return sdept; } public void setSdept(String sdept){ this.sdept=sdept; } } package job1; import https://www.360docs.net/doc/fc4630968.html,parator; import java.text.CollationKey; import java.text.Collator; public class MyCmp implements Comparator{ public int compare(Object obj1,Object obj2){ Collator collator=Collator.getInstance(); CollationKey key1=collator.getCollationKey(obj1.toString()); CollationKey key2=collator.getCollationKey(obj2.toString()); return https://www.360docs.net/doc/fc4630968.html,pareTo(key2); } } package job1; import java.util.*; public class TreeText { public static void main(String[] args){ TreeMap map=new TreeMap(new MyCmp()); map.put("蒋高登","20081851 1990-08-03 男 CS"); map.put("蒋稀文","20081852 1989-01-11 男 CS"); map.put("董骏","20081850 1990-04-05 男 CS"); Set keys=map.entrySet(); Iterator it=keys.iterator(); while(it.hasNext()){ Map.Entry e=(Map.Entry) it.next(); System.out.println(e.getKey()+" "+e.getValue()); } } } 3、设计一个程序计算2011-05-01日与系统当前日期相差的天数。 package job1; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; public class Days { public static void main(String[] args) throws ParseException{ Date d1=new Date(); SimpleDateFormat format=new SimpleDateFormat("yyyy-MM-dd"); String s="2011-05-01"; Date d2=format.parse(s); int days=(int)((d2.getTime()-d1.getTime())/(1000*60*60*24)); System.out.print("2011-05-01日与系统当前时间相差"+days+"天"); } } 4、使用日历类等相关方法按截图做出一个日历参照书本示例,研究其中代码回顾与复习利用Java Swing编程。 参考:以下函数根据输入的年和月计算相应的数字 public void showCalendar(int year,int month){ Calendar cal=Calendar.getInstance(); cal.set(Calendar.YEAR, year); cal.set(Calendar.MONTH, month-1); //计算当前月一共有多少天 int days=cal.getActualMaximum(Calendar.DAY_OF_MONTH); //计算当前月的1号为星期几 cal.set(Calendar.DAY_OF_MONTH, 1);//设置为1号 int firstweek=cal.get(Calendar.DAY_OF_WEEK); 。。。。。 } package job1; import java.awt.BorderLayout; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.Calendar; import javax.swing.*; import javax.swing.table.DefaultT ableModel; public class CalendarTable extends JFrame implements ActionListener{ private JLabel label_year=new JLabel("年"); private JLabel label_month=new JLabel("月"); private JComboBox box_year=new JComboBox(); private JComboBox box_month=new JComboBox(); private JButton btn=new JButton("确定"); private JTable table; private String week[]={"日","一","二","三","四","五","六"}; private String rows[][]=new String[6][7]; public CalendarTable(){ JPanel jp_north=new JPanel(new GridLayout(1,5)); for(int i=1;i<13;i++) box_month.addItem(i); jp_north.add(box_year); jp_north.add(label_year); jp_north.add(box_month); jp_north.add(label_month); jp_north.add(btn); table=new JTable(rows,week); JScrollPane scroll=new JScrollPane(table); this.add(jp_north,BorderLayout.NORTH); this.add(scroll); calendarinit(); btn.addActionListener(this); box_year.addActionListener(this); box_month.addActionListener(this); } public void actionPerformed(ActionEvent e){ Calendar cal=Calendar.getInstance(); int year=cal.get(Calendar.YEAR);//box_year.getSelectedIndex(); int month=box_month.getSelectedIndex(); showCalendar(year,month); table.setModel(new DefaultTableModel(rows,week)); } private void calendarinit(){ Calendar cal=Calendar.getInstance(); box_year.addItem(new Integer(cal.get(Calendar.YEAR))); cal.set(Calendar.DAY_OF_MONTH, 1); box_month.setSelectedIndex(cal.get(Calendar.MONTH)); showCalendar(Calendar.YEAR,Calendar.MONTH); } private void showCalendar(int year,int month){ Calendar cal=Calendar.getInstance(); cal.set(Calendar.YEAR, year); cal.set(Calendar.MONTH, month); int days=cal.getActualMaximum(Calendar.DAY_OF_MONTH); cal.set(Calendar.DAY_OF_MONTH, 1); int firstweek=cal.get(Calendar.DAY_OF_WEEK); for(int i=0;i for(int j=0;j rows[i][j]=""; } } int line=0; for(int i=1;i if(i%7==0){ line++; } if(firstweek==1){ rows[0][0]="1"; } if(i>=firstweek-1){ rows[line][i%7]=(i-firstweek+2)+""; } } } public static void main(String[] args){ CalendarTable cal=new CalendarTable(); cal.setBounds(40, 40, 400, 200); cal.setVisible(true); cal.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } 5日期类的构造 定义一个日期类,属性有年、月、 日;以及对应这些属性的构造函数 以及set-get访问器方法。其它功能 包括: 1)、按照中国日期输出格式,编写toString()函数。即形如2010年12月10日. public String toString (){ … } 2)、定义日期相加的函数,可以加年,月,日。 public void addDate(int part,int nums){ … } 其中part表示加哪个部分,年,月还是日,nums表示加多少? 如:d1.addDate(1,10);表示加上10年,d1.addDate(2,10);表示加上10月, d1.addDate(3,10);表示加上10天。 参考:可以定义一个私有函数,将日期加一天后的日期 3)、定义日期差函数可以相差多少年,月,日。 public int diffDate(int part,Date date){ … } 如:d1. diffDate (1,d2);表示d1与d2相差多少年。d1. diffDate (2,d2);表示d1与d2相差多少月。d1. diffDate (3,d2);表示d1与d2相差多少天。 4)、计算输出当前日期实例对应的是星期几函数 public int calcuWeekDay(){ …. } 注意:考虑到1900-1-1日这一天是星期一的现实. package job1; import java.text.SimpleDateFormat; import java.util.Date; public class MyDate { private int year; private int month; private int day; public MyDate(){} public MyDate(int year,int month,int day){ this.year=year; this.month=month; this.day=day; } public int getYear(){ return this.year; } public void setYear(int year){ this.year=year; } public int getMonth(){ return this.month; } public void setMonth(int month){ this.month=month; } public int getDay(){ return this.day; } public void setDay(int day){ this.day=day; } private void addOneMonth(){ this.month++; if(this.month>12){ this.year++; this.month=1; } } private void addOneDay(){ this.day++; if(this.day>maxMonthDays()){ this.month++; this.day=1; if(this.month>12){ this.year++; this.month=1; } } } public int maxMonthDays(){ int monthDays=31; if(this.month==4||this.month==6||this.month==9||this.month==11){ monthDays=30; } else if(this.month==2&&isLeapYear()){ monthDays=29; } else if(this.month==2&&!isLeapYear()){ monthDays=28; } return monthDays; } private boolean isLeapYear() { if(this.year%4==0&&this.year%100!=0||this.year%400==0) return true; return false; } public String toString() { return this.year + "年" + this.month + "月" + this.day + "日"; } public void addDate(int part,int nums){ if(part==1){ this.year=this.year+nums; } else if(part==2){ for(int i=0;i addOneMonth(); } } else if(part==3){ for(int i=0;i addOneDay(); } } } public boolean isEquals(MyDate date){ boolean f = false; if (this.year == date.year && this.month == date.month && this.day == date.day) f = true; return f; } public boolean isBefore(MyDate date){ boolean f=false; if(this.year f=true; else if(this.year==date.year){ if(this.month f=true; } else if(this.year==date.year&&this.month==date.month){ if(this.day f=true; } return f; } public int diffDate(int part,MyDate date){ int diffDays=0; if(part==1){ if(this.isBefore(date)) diffDays=date.year-this.year; else if(this.isEquals(date)) diffDays=0; else diffDays=this.year-date.year; } else if(part==2){ if(this.isBefore(date)) diffDays=date.year*12+date.month-this.year*12-this.month; else if(this.isEquals(date)) diffDays=0; else diffDays=this.year*12+this.month-date.year*12-date.month; } else if(part==3){ if(this.isBefore(date)) diffDays=date.year*12*date.maxMonthDays()+date.month*date.maxMonthDay s() +date.day-this.year*12*this.maxMonthDays()-this.month*this.maxMonthDa ys() -this.day; else if(this.isEquals(date)) diffDays=0; else diffDays=this.year*12*this.maxMonthDays()+this.month*this.maxMonthDay s() +this.day-date.year*12*date.maxMonthDays()-date.month*date.maxMonthDa ys() -date.day; } return diffDays; } public int calcuWeekDay(MyDate date){ int weekday=((date.year-1900)*365+(date.month)*date.maxMonthDays()+date.d ay-1)%7; return weekday; } public static void main(String[] args){ MyDate date1=new MyDate(2010,3,3); MyDate date2=new MyDate(2012,3,17); System.out.println(date1.toString()); System.out.println(date2.toString()); date1.addDate(1, 2); System.out.println(date1.toString()); int diffDays=0; diffDays=date1.diffDate(3, date2); System.out.println("2012年3月3日与2012年3月17日相差"+diffDays+"天"); int weekday1=0,weekday2=0; weekday1=date1.calcuWeekDay(date1); weekday2=date2.calcuWeekDay(date2); System.out.println("2012年3月3日是星期"+weekday1); System.out.println("2012年3月17日是星期"+weekday2); } }
");