C++ 回调函数总结及回调函数的封装
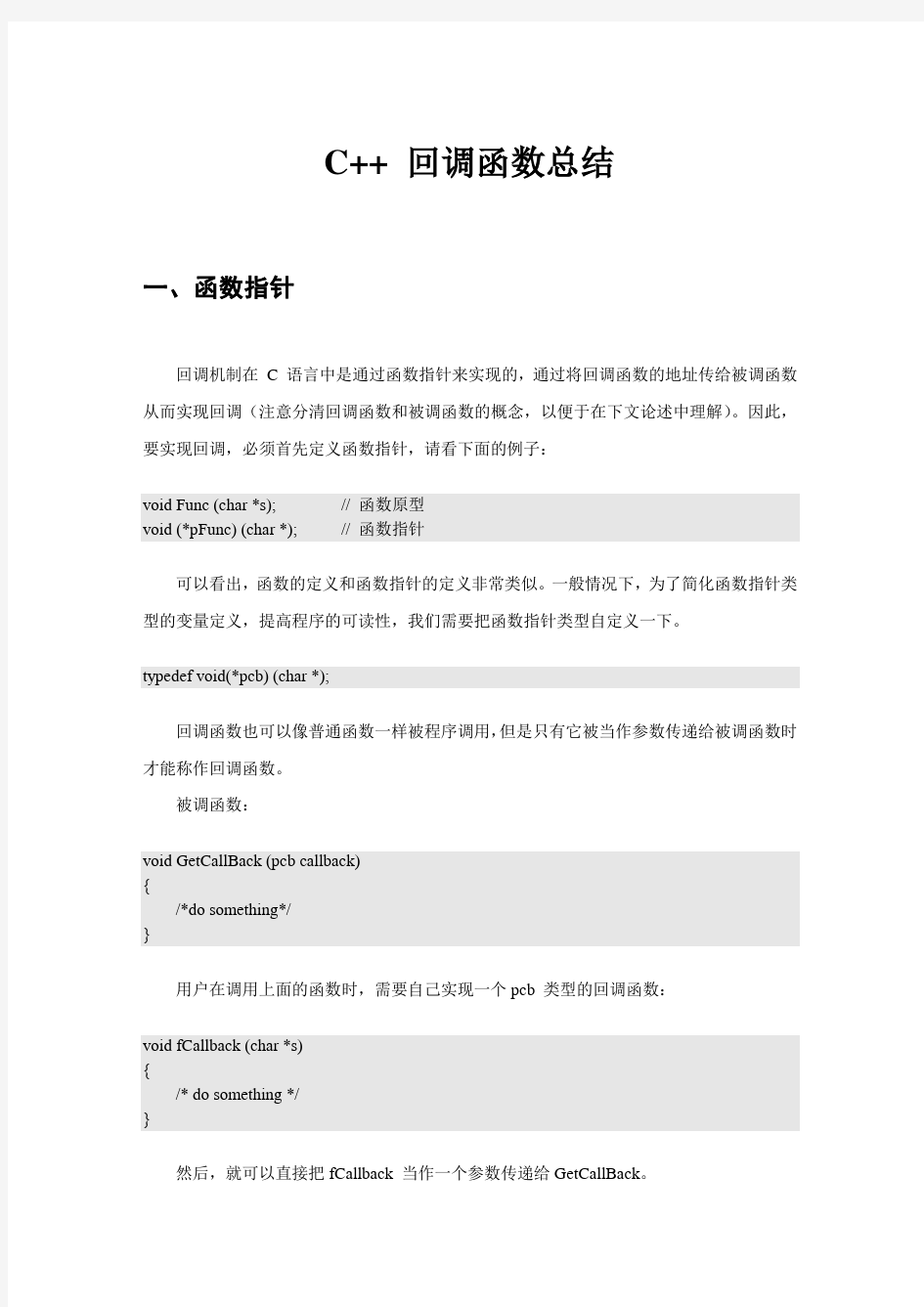
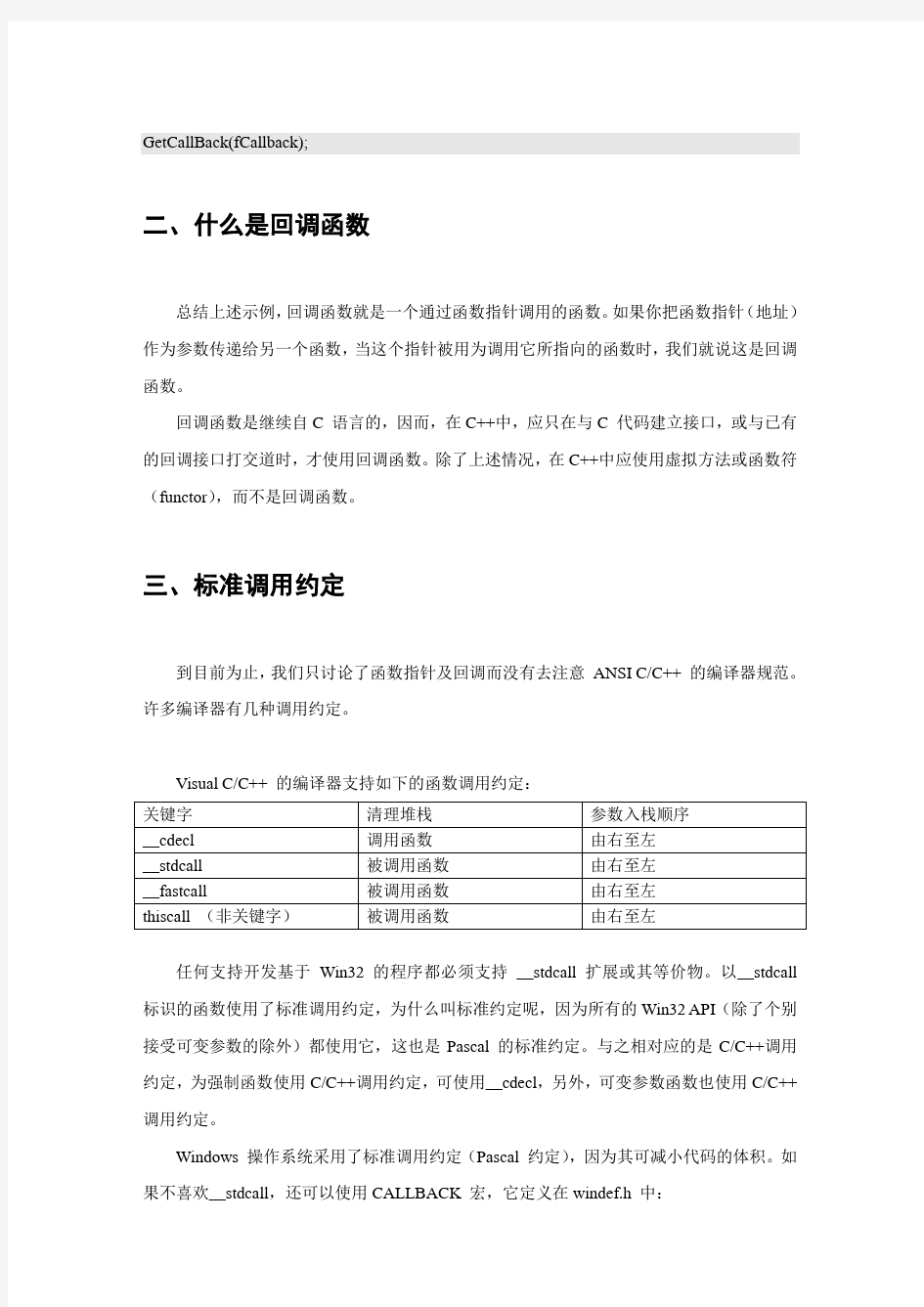
C++ 回调函数总结
一、函数指针
回调机制在C 语言中是通过函数指针来实现的,通过将回调函数的地址传给被调函数从而实现回调(注意分清回调函数和被调函数的概念,以便于在下文论述中理解)。因此,要实现回调,必须首先定义函数指针,请看下面的例子:
void Func (char *s); // 函数原型
void (*pFunc) (char *); // 函数指针
可以看出,函数的定义和函数指针的定义非常类似。一般情况下,为了简化函数指针类型的变量定义,提高程序的可读性,我们需要把函数指针类型自定义一下。
typedef void(*pcb) (char *);
回调函数也可以像普通函数一样被程序调用,但是只有它被当作参数传递给被调函数时才能称作回调函数。
被调函数:
void GetCallBack (pcb callback)
{
/*do something*/
}
用户在调用上面的函数时,需要自己实现一个pcb 类型的回调函数:
void fCallback (char *s)
{
/* do something */
}
然后,就可以直接把fCallback 当作一个参数传递给GetCallBack。
GetCallBack(fCallback);
二、什么是回调函数
总结上述示例,回调函数就是一个通过函数指针调用的函数。如果你把函数指针(地址)作为参数传递给另一个函数,当这个指针被用为调用它所指向的函数时,我们就说这是回调函数。
回调函数是继续自C 语言的,因而,在C++中,应只在与C 代码建立接口,或与已有的回调接口打交道时,才使用回调函数。除了上述情况,在C++中应使用虚拟方法或函数符(functor),而不是回调函数。
三、标准调用约定
到目前为止,我们只讨论了函数指针及回调而没有去注意ANSI C/C++ 的编译器规范。许多编译器有几种调用约定。
Visual C/C++ 的编译器支持如下的函数调用约定:
任何支持开发基于Win32 的程序都必须支持__stdcall 扩展或其等价物。以__stdcall 标识的函数使用了标准调用约定,为什么叫标准约定呢,因为所有的Win32 API(除了个别接受可变参数的除外)都使用它,这也是Pascal 的标准约定。与之相对应的是C/C++调用约定,为强制函数使用C/C++调用约定,可使用__cdecl,另外,可变参数函数也使用C/C++调用约定。
Windows 操作系统采用了标准调用约定(Pascal 约定),因为其可减小代码的体积。如果不喜欢__stdcall,还可以使用CALLBACK 宏,它定义在windef.h 中:
#define CALLBACK __stdcallor
#define CALLBACK PASCAL // 而PASCAL 在此被#defined 成__stdcall 四、简单示例
int __stdcall CompareInts(const byte* velem1, const byte* velem2)
{
int elem1 = *(int*)velem1;
int elem2 = *(int*)velem2;
if(elem1 < elem2)
return -1;
if(elem1 > elem2)
return 1;
return 0;
}
int __stdcall CompareStrings(const byte* velem1, const byte* velem2)
{
const char* elem1 = (char*)velem1;
const char* elem2 = (char*)velem2;
return strcmp(elem1, elem2);
}
int main(int argc, char* argv[])
{
int i;
int array[] = {5432, 4321, 3210, 2109, 1098};
cout << "Before sorting ints with Bubblesort\n";
for(i=0; i < 5; i++)
cout << array[i] << '\n';
Bubblesort((byte*)array, 5, sizeof(array[0]), &CompareInts);
cout << "After the sorting\n";
for(i=0; i < 5; i++)
cout << array[i] << '\n';
const char str[5][10] = {"estella","danielle","crissy","bo","angie"};
cout << "Before sorting strings with Quicksort\n";
for(i=0; i < 5; i++)
cout << str[i] << '\n';
Quicksort((byte*)str, 5, 10, &CompareStrings);
cout << "After the sorting\n";
for(i=0; i < 5; i++)
cout << str[i] << '\n';
return 0;
}
五、回调函数的C++封装
使用到C++编写代码,一般会把回调函数写成类中的一个方法,但先来看看以下的代码:
class CCallbackTester
{
public:
int CALLBACK CompareInts(const byte* velem1, const byte* velem2);
};
Bubblesort((byte*)array, 5, sizeof(array[0]), &CCallbackTester::CompareInts);
如果使用微软的编译器,将会得到下面这个编译错误:
error C2664: 'Bubblesort' : cannot convert parameter 4 from 'int (__stdcall CCallbackTester::*)(const unsigned char *,const unsigned char *)' to 'int (__stdcall *)(const unsigned char *,const unsigned char *)' There is no context in which this conversion is possible 这是因为非静态成员函数有一个额外的参数:this 指针,这将迫使你在成员函数前面加
上static 关键字。
class CCallbackTester
{
public:
static int CALLBACK CompareInts(const byte* velem1, const byte* velem2);
};
静态成员函数虽然很好的解决了this 指针的问题,但是由于静态成员函数只可以调用静态成员函数,这样似乎失去了C++ 类的大部分优点。那么怎样在静态成员函数中使用类的非静态成员呢?可以给静态成员函数传入参数,而且一般设计较好的回调函数都提供一个
数据块指针作为传入参数,这时候可以采用如下的结构来解决此问题。
class CCallbackTester
{
public:
static int CALLBACK CompareInts(void * pData, const byte* velem1, const byte* velem2) {
CCallbackTester * pThisObject = (CCallbackTester * ) pData;
pThisObject -> CompareInts2(velem1, velem2);
}
void CompareInts2 (const byte* velem1, const byte* velem2)
{
// do something
}
};
回调函数
对于很多初学者来说,往往觉得回调函数很神秘,很想知道回调函数的工作原理。本文将要解释什么是回调函数、它们有什么好处、为什么要使用它们等等问题,在开始之前,假设你已经熟知了函数指针。 什么是回调函数? 简而言之,回调函数就是一个通过函数指针调用的函数。如果你把函数的指针(地址)作为参数传递给另一个函数,当这个指针被用为调用它所指向的函数时,我们就说这是回调函数。 为什么要使用回调函数? 因为可以把调用者与被调用者分开。调用者不关心谁是被调用者,所有它需知道的,只是存在一个具有某种特定原型、某些限制条件(如返回值为int)的被调用函数。 如果想知道回调函数在实际中有什么作用,先假设有这样一种情况,我们要编写一个库,它提供了某些排序算法的实现,如冒泡排序、快速排序、shell排序、shake排序等等,但为使库更加通用,不想在函数中嵌入排序逻辑,而让使用者来实现相应的逻辑;或者,想让库可用于多种数据类型(int、float、string),此时,该怎么办呢?可以使用函数指针,并进行回调。 回调可用于通知机制,例如,有时要在程序中设置一个计时器,每到一定时间,程序会得到相应的通知,但通知机制的实现者对我们的程序一无所知。而此时,就需有一个特定原型的函数指针,用这个指针来进行回调,来通知我们的程序事件已经发生。实际上,SetTimer() API使用了一个回调函数来通知计时器,而且,万一没有提供回调函数,它还会把一个消息发往程序的消息队列。 另一个使用回调机制的API函数是EnumWindow(),它枚举屏幕上所有的顶层窗口,为每个窗口调用一个程序提供的函数,并传递窗口的处理程序。如果被调用者返回一个值,就继续进行迭代,否则,退出。EnumWindow()并不关心被调用者在何处,也不关心被调用者用它传递的处理程序做了什么,它只关心返回值,因为基于返回值,它将继续执行或退出。 不管怎么说,回调函数是继续自C语言的,因而,在C++中,应只在与C代码建立接口,或与已有的回调接口打交道时,才使用回调函数。除了上述情况,在C++中应使用虚拟方法或函数符(functor),而不是回调函数。 一个简单的回调函数实现 下面创建了一个sort.dll的动态链接库,它导出了一个名为CompareFunction的类型--typedef int (__stdcall *CompareFunction)(const byte*, const byte*),它就是回调函数的类型。另外,它也导出了两个方法:Bubblesort()和Quicksort(),这两个方法原型相同,但实现了不同的排序算法。
中考英语口语辅导:consider的用法
中考英语口语辅导:consider的用法 表示“考虑”,其后可接动名词,但不能接不定式。如He is considering changing his job. 他在考虑调换工作。I’ve never really considered getting married. 我从未考虑过结婚的事。注:consider 之后虽然不能直接跟不定式,但可跟“疑问词+不定式”结构。如Have you considered how to get there / how you could get there. 你是否考虑过何到那儿去?2. 表示“认为”、“把……看作”,下面三个句型值得注意(有时三者可互换) (1) consider +that从句(2) consider+宾语+(as +)名词或形容词(3) consider+宾语+(to be +)名词或形容词I consider him (as) honest (或an honest man). I consider him (to be) honest (或an honest man). I consider that he is honest (或an honest man). 注:(1) 以上备句意思大致相同,对于consider 之后能否接as 的问题,尚有不同看法(即有人认为不能接as ,有人认为可以拉as,但实际上接as 的用法已很普遍)。(2) 在“consider+宾语”之后除可接to be 外,有时也可to do 型动词(但多为完成形式)。如We all considered him to have acted disgracefully. 我们都认为他的行为很不光彩。
疑问代词用法总结及练习
疑问代词用法总结及练习 句子是英语学习的核心。从句子使用的目的来分,它可分为四类 1、陈述句(肯定句和否定句) 2、疑问句(一般疑问句、特殊疑问句和选择疑问句) 3、祈使句(肯定句和否定句) 4、感叹句。 四大句子类型的相互转换,对于学生来讲是个难点,为此,可通过说顺口溜的形式来帮助学生解决这一难题。 如:将陈述句变成一般疑问句,可以变成这样的顺口留:疑问疑问调个头,把be(系动词“is are am”)放在最前头。 如:将陈述句的肯定句变成否定句,我们就可以这样说:否定,否定加“not”,加在何处,加在系动词的后面。 在句子相互转换的题型中,最难的要算“就下列划线部分提问”或是“看答句,写问句”这种题型了,其实,我们只要熟练掌握疑问词(what,what time, what colour, where, when, who, whose, which, how, how old ,how tall, how long, how big, how heavy , how much, how many等等)具体用法。
习题 一、选择正确的单词填空 (who, where, when) is that pretty girl She is my sister. are Jack and Tom They are behind you. do you go to school I go to school from Monday to Friday. has a beautiful flower John has a beautiful flower.
are they They are my parents. is my mother She is in the living room. are you going We are going to the bakery(面包坊). Jim and Wendy play ball They play ball in the afternoon. does he jog He jogs in the park. are you from I'm from Changchun city. 11. _______ is your birthday –On May 2nd. 12、_______ are you --- I`m in the office. 13. are you ---- I`m Alice. 二.用(what time, what color, what day, what)填空。 1. A: ______ _______ is it B: It is nine o’clock. 2. A: ______ _______ does your mother get up B: My mother gets up at 6:30. 3. A: ______ _______ do you go to bed B: I go to bed at 10:00. 4. A: ______ _______ do Diana and Fiona have supper B: Diana and Fiona have supper at 18:00. 5. A: ______ _______is it B: It is purple. 6. A: ______ _______ is the sky B: The sky is blue. 7. A: ______ _______ is your coat B: My coat is black. 8. A: ______ _______ is the dog B: The dog is white. 9. A: ______ _______ is today B: Today is Monday. 10. A: ______ _______ is tomorrow B: Tomorrow is Tuesday. 11. A: ______ _______ was yesterday B: Yesterday was Sunday. 12. A: ______ _______ do you like B: I like red. 13. A: ______ is this This is a computer. 14. A: ______ are you doing B: We are playing basketball.
回调函数与回调机制
回调函数与回调机制 1. 什么是回调函数 回调函数(callback Function),顾名思义,用于回调的函数。回调函数只是一个功能片段,由用户按照回调函数调用约定来实现的一个函数。回调函数是一个工作流的一部分,由工作流来决定函数的调用(回调)时机。回调函数包含下面几个特性: ?属于工作流的一个部分; ?必须按照工作流指定的调用约定来申明(定义); ?他的调用时机由工作流决定,回调函数的实现者不能直接调用回调函数来实现工作流的功能; 2. 回调机制 回调机制是一种常见的设计模型,他把工作流内的某个功能,按照约定的接口暴露给外部使用者,为外部使用者提供数据,或要求外部使用者提供数据。 如上图所示,工作流提供了两个对外接口(获取参数、显示结果),以回调函数的形式实现。 ?“获取参数”回调函数,需要工作流使用者设定工作流计算需要的参数。 ?“显示结果”回调函数,提供计算结果给工作流使用者。
再以Windows的枚举顶级窗体为例。函数EnumWindows用于枚举当前系统中的所有顶级窗口,其函数原型为: BOOL EnumWindows( WNDENUMPROC lpEnumFunc, // callback function LPARAM lParam // application-defined value ); 其中lpEnumFunc是一个回调函数,他用于返回枚举过程中的获得的窗口的句柄。其定义约定为: BOOL CALLBACK EnumWindowsProc( HWND hwnd, // handle to parent window LPARAM lParam // application-defined value ); 在这个例子中,EnumWindows 是一个工作流,这个工作流用于遍历windows的所有窗口并获得其句柄。用户使用EnumWindows工作流的目的是想通过工作流来来获取窗口的句柄以便针对特定的一个或多个窗口进行相关处理。于是EnumWindows就扩展出接口lpEnumFunc,用于返回遍历的窗口句柄。 EnumWindows工作流的结束有两个方式:1,用户在回调函数中返回FALSE;2,再也找不到顶级窗口。我们可以推测EnumWindows的实现机制如下: 注:下列代码中的FindFirstTopWindows(), FindNextTopWindow()为假设的,Windows API 没有此函数,只是为了表明Enumwindows的内部流程。 BOOL EnumWindows( WNDENUMPROC lpEnumFunc, // callback function LPARAM lParam // application-defined value ) { BOOL bRet = TRUE; HWND hWnd = ::FindFirstTopWindows(); // 此函数是假设的,查找第一个顶级窗口 // 当hWnd为0时表示再也找不到顶级窗口 while( hWnd ) { bRet = (*lpEnumFunc)( hWnd, value ); if( !bRet) break; // 终止EnumWindows工作流; hWnd = ::FindNextWindow(); // 此函数是假设的,查找下一个顶级窗口 } } 在EnumWindows(...)函数中,实现了窗口枚举的工作流,他通过回调机制把用户关心(顶级窗口句柄)的和枚举工作流分开,用户不需要知道EnumWindows的具体实现,用户只要知道,设定了lpEnumFunc函数,然后把函数指针传给EnumWindwos就可以获得想要的窗口句柄。
consider的基本用法及与regardthinkofabout
consider的基本用法及与regard,think ofabout和look(up)on as的区别 consider的基本用法及与regard,think of/about和look(up)on as的区别 consider一词在历年高考中是一个常考的要点,其用法应分为两部分来讲。第一、作“考虑、思考”时的搭配如下: 1.consider+n./pron.,例如: Have you considered the suggestion? That’s what we have to consider now. 2.consider+v-ing,但不能接不定式的一般式,例如: We considered going to see the doctor the next day. Have you considered moving to shanghai recently? You must consider to tell him something about it.(错误) 3.consider+疑问词+不定式,例如: He is considering how to improve his English. We must consider what to do next.
4.consider+从句,例如: We didn’t consider whether he should go or not. Have you considered when we should go there? 第二、作“认为、把……当作/看作”等意思时的搭配如下:1.consider+sb/sth+(to be/as)+n./adj.,例如: I consider him to(be/as)my best friend. Everyone considers him(to be)clever. He considered it much improved. 2.consider+sb./sth.+不定式短语(作宾语补足语),不能接不定式的一般式,例如: We consider them to be working very hard. We consider them to have finished the work. We consider him to be the clever in our class. We must consider him to go there at once.(错误) 3.consider+it+adj./n.+不定式短语,其中it为形式宾语,不定式短语为真正的宾语,例如: Jiao Yulu considered it his duty to serve the people heart and soul. They consider it necessary to set the slaves free.
hook的使用实例
在网上找了好久都没有找到消息hook的实例,下面是我的例子给大家分享一下 下面是dll中的代码: //////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// //我的经验,编译的时候会提示DllMain,已在DllMain.cpp中定义,把DllMain.cpp从源文件里删掉就好了 #include "stdafx.h" #include
英语一般疑问句用法总结
英语一般疑问句用法总结 1. 基本用法及结构 一般疑问句用于对某一情况提出疑问,通常可用yes和no来回答,读时用升调。其基本结构是“be / have / 助动词+主语+谓语(表语)”: Is he interested in going? 他有兴趣去吗? Have you ever been to Japan? 你到过日本吗? Does she often have colds? 她常常感冒吗? Did you ask her which to buy? 你问没问她该买哪一个? 2. 陈述句变一般疑问句的方法 (1) 动词be的否定式。动词be根据不同的时态和人称可以有am, is, are, was, were等不同形式,可用作连系动词(表示“是”、“在”等)和助动词(用于构成进行时态和被动语态等),但不管何种情况,构成疑问式时,一律将动词be的适当形式置于句首: Am I right? 我是对的吗? Are you feeling better today? 你今天感到好些了吗? Was he late for school? 他上学迟到了吗? (2) 动词have的疑问式。动词have根据不同的时态和人称可以有have, has, had等形式,可以用作实意动词和助动词,分以下情况讨论:
①用作实意动词表示状态,如表示拥有、患病或用于have to 表示“必须”等,在构成构成式时可以直接将have, has, had置于句首,也可根据情况在句首使用do, does, did: Does he have [Has he] anything to say? 他有什么话要说吗? Do you have [Have you] to leave so soon? 你必须这么早走吗? Did you have [Had you] any friends then? 他当时有朋友吗? ②用作实意动词表示动作,如表示“吃(=eat)”、“喝(=drin k)”、“拿(=take)”、“收到(=receive)”、“度过(=spend)”等,构成疑问式时不能将have提前至句首,而应在句首使用do, does, did: Does he have breakfast at home? 他在家吃早餐吗? Did you have a good time at the party? 你在晚会上玩得高兴吗? ③用作助动词构成完成时态,其疑问式总是将have等置于句首: Have you finished your work? 你的工作做完了吗? Has he left when you arrived? 你到达时他已离开了吗? (3) 情态动词的疑问式。情态动词的疑问式通常是将情态动词置于句首: Can you speak English? 你会说英语吗?
Consider的用法
Consider的用法: -Have you considered_____ the job ss a teacher? -Yes.I like it because a teacher is often considered _______ a gardener. A.to take,to be B.to take,being C.taking,being D.taking,to be 答案:d译文:你考虑过做老师的工作吗? 是的,我非常喜欢,因为老师通常被认为是园丁 一、consider作“考虑”解,常用于以下句型: 1.consider+名词/代词/动名词。 You'd better consider my suggestion. 你最好考虑我的建议。 I’m considering going abroad some day.我一直考虑有一天出国。 2.consider+从句或“疑问词+不定式”。 Have you considered what he suggested? 你们考虑他的建议了吗? We must consider what to do next. 我们必须考虑下一步要做什么。 二.consider作“认为”解时,常用于以下句型: 1.consider sb./sth+.(as)+形容词/名词。其中,as可以省略。 We consider him honest. 我们认为他很诚实。 At first they considered me as a doctor.起初他们认为我是医生。 2.consider+sb./sth.+不定式。其中,不定式通常是to be(可以省略)或其他动词的完成式。We consider this matter to be very important. 我们认为这件事很重要。 We all consider him to have stolen the bike.我们都认为他偷了自行车。 3.consider+it+形容词/名词+不定式短语。 We consider it hard to study English well.我们认为学好英语很难。 I consider it my duty to help you with your studies.我认为帮助你学习英语是我的职责。 4.consider+宾语从句。 We consider that the music is well worth listening to.我们这首音乐很值得一听。 在该题中,前一个句子中的consider作“考虑”解,后接动名词作宾语,但不可接不定式,由此可以排除A, B; 后一个句子中的consider作“认为”解,用到句型consider+sb./sth.+不定式,此处使用的是被动语态结构。故答案为D项。 请看下面两道考题,均考查“with+宾语+非谓语动词”结构: 1.—Come on, please give me some ideas about the project. —Sorry. With so much work _________my mind, I almost break down. A.filled B.filling C.to fill D.being filled 2.John received an invitation to dinner, and with his work _________, he gladly acc epted it. A.finished B.finishing C.having finished D.was finished 以上两题答案分别为B和A,均考查“with+宾语+非谓语动词”结构。该结果中的“非谓语动词”可以是不定式、现在分词、过去分词,它们在用法上的区别如下:
一般疑问句、选择疑问句的详细用法备课讲稿
一般疑问句、选择疑问句的详细用法
一般疑问句、 (一)一般疑问句 1、一般疑问句概述 一般疑问句(general questions),也可称为“yes/no” questions(是否型问句),因为它一般是由yes或no回答的,如: —Can you swim to the other side?你能游到对岸吗? —Yes, I can.是的,我能。 —No,I can’t.不,我不能。 —Have you locked the door?你锁门了吗? —Yes,I have.是的,锁了。 —No,I haven’t. 不,没有锁。 2一般疑问句的结构 (1)基本的结构为:be/助动词/情态动词+主语+谓语/表语+(其他),句子要读升调,如: Are they your friends?他们是你的朋友吗? Does he go to school on foot?他是步行去上学吗? Will you be free tonight?你今晚有空吗? Can you play basketball?你会打篮球吗? (2)陈述句亦可用作一般疑问句,多用在非正式文体中,句末有问号,用升调,如: Somebody is with you?有人和你一起吗? He didn’t finish the work?他没有做完活吗? You are fresh from America,I suppose?我猜,你刚从美国回来吧? 3、一般疑问句的答语 (1)一般疑问句一般由yes或no来回答,如: —Are you tired?你累了吗? —Yes,I am.是的,累了。 —No, I’m not.不,不累。 —Does she do the cleaning?她扫除了吗?
关于回调函数的几个例子(c)
以下是一个简单的例子。实现了一个repeat_three_times函数,可以把调用者传来的任何回调函数连续执行三次。 例 1. 回调函数 /* para_callback.h */ #ifndef PARA_CALLBACK_H #define PARA_CALLBACK_H typedef void (*callback_t)(void *); extern void repeat_three_times(callback_t, void *); #endif /* para_callback.c */ #include "para_callback.h" void repeat_three_times(callback_t f, void *para) { f(para); f(para); f(para); } /* main.c */ #include
} int main(void) { repeat_three_times(say_hello, "Guys"); repeat_three_times(count_numbers, (void *)4); return 0; } 回顾一下前面几节的例子,参数类型都是由实现者规定的。而本例中回调函数的参数按什么类型解释由调用者规定,对于实现者来说就是一个void *指针,实现者只负责将这个指针转交给回调函数,而不关心它到底指向什么数据类型。调用者知道自己传的参数是char *型的,那么在自己提供的回调函数中就应该知道参数要转换成char *型来解释。 回调函数的一个典型应用就是实现类似C++的泛型算法(Generics Algorithm)。下面实现的max函数可以在任意一组对象中找出最大值,可以是一组int、一组char或者一组结构体,但是实现者并不知道怎样去比较两个对象的大小,调用者需要提供一个做比较操作的回调函数。 例 2. 泛型算法 /* generics.h */ #ifndef GENERICS_H #define GENERICS_H typedef int (*cmp_t)(void *, void *); extern void *max(void *data[], int num, cmp_t cmp); #endif /* generics.c */ #include "generics.h" void *max(void *data[], int num, cmp_t cmp) { int i; void *temp = data[0];
consider的用法归纳有哪些
consider的用法归纳有哪些 consider的用法:作名词 consideration作名词,意为careful thought and attention斟酌,考虑 Several considerations have influenced my decision.好几个因素影响了我的决定。 1.Consideration for顾及,体贴 He has never shown much consideration for his wife’s needs.他从来不顾及他妻 子的需要。 2.Under consideration在讨论/考虑中 Several projects are under consideration.好几个项目在讨论中。 There are some proposals under consideration. 有几个建议在审议中。 3.Take sth. into consideration考虑到某事,体谅 Your teachers will take your recent illness into consideration when marking your exams. 你的几位老师在给你的考试评分时,会考虑你最近生病这一情况的。 4.Leave sth. out of consideration 忽略/不重视某事 It was wrong to leave my feelings out of consideration.不顾及我的情感是不对的。 5.Show consideration for体谅,顾及 Jeff never shows any consideration for his mother’s feelings.杰夫从来不体谅他 母亲的感受。 6.of. No / little consideration无关紧要的,不重要的 Whether he would go with us or not was of no consideration. 他是否跟我们一起 去是无关紧要的。 7.In consideration of sth.作为对……的汇报,考虑到 It’s a small payment in consideration of your services.这是答谢您服务的微薄酬金。 consider的用法:作动词 1.Consider作动词,意为think about sth.考虑,斟酌 常用搭配:consider sth. / doing sth. / where(how, why)+to do /that clause; all things considered通盘考虑,考虑到问题的各个方面。如:
OpenGL一个简单的例子
先编译运行一个简单的例子,这样我们可以有一个直观的印象。从这个例子我们可以看到OpenGL可以做什么,当然这个例子只做了很简单的一件事--绘制一个彩色的三角形。除此以外,我们还可以看到典型的OpenGL程序结构及openGL的运行顺序。 例1:本例在黑色的背景下绘制一个彩色的三角形,如图一所示。
图一:一个彩色的三角形首先创建工程,其步骤如下:
1)创建一个Win32 Console Application。 2)链接OpenGL libraries。在Visual C++中先单击Project,再单击Settings,再找到Link单击,最后在Object/library modules 的最前面加上OpenGL32.lib GLu32.lib GLaux.lib 3)单击Project Settings中的C/C++标签,将Preprocessor definitions 中的_CONSOLE改为__WINDOWS。最后单击OK。 现在你可以把下面的例子拷贝到工程中去,编译运行。你可以看到一个彩色的三角形。 我们先看看main函数。函数中以glut开头的函数都包含在glut.h中。GLUT库的函数主要执行如处理多窗口绘制、处理回调驱动事件、生成层叠式弹出菜单、绘制位图字体和笔画字体,以及各种窗口管理等任务。 ·glutInit用来初始化GLUT库并同窗口系统对话协商。 ·glutInitDisplayMode用来确定所创建窗口的显示模式。本例中的参数GLUT_SINGLE 指定单缓存窗口,这也是缺省模式,对应的模式为GLUT_DOUBLE 双缓存窗口。参数GLUT_RGB指定颜色RGBA模式,这也是缺省模式,对应的模式为GLUT_INDEX 颜色索引模式窗口。 ·glutInitWindowSize初始化窗口的大小,第一个参数为窗口的宽度,第二个参数为窗口的高度,以像素为单位。 ·glutInitWindowPosition设置初始窗口的位置,第一个参数为窗口左上角x的坐标,第二个参数为窗口左上角y的坐标,以像素为单位。屏幕的左上角的坐标为(0,0),横坐标向右逐渐增加,纵坐标向下逐渐增加。 ·glutCreateWindow创建顶层窗口,窗口的名字为扩号中的参数。 ·background() 这是自己写的函数,设置背景。其实这个函数中的语句可以写在display 函数中,但为了使功能块更加清晰,所以把背景这一部分单独提出来。 ·glutReshapeFunc注册当前窗口的形状变化回调函数。当改变窗口大小时,该窗口的形状改变回调函数将被调用。在此例中就是myReshape指定形状变化函数。 ·glutDisplayFunc注册当前窗口的显示回调函数。当一个窗口的图像层需要重新绘制时,GLUT将调用该窗口的的显示回调函数。在此例中的mydisplay就是显示回调函数,显示回调函数不带任何参数,它负责整个图像层的绘制。我们的大部分工作将集中在这个函数中。 ·glutMainLoop进入GLUT事件处理循环。glutMainLoop函数在GLUT程序中最多只能调用一次,它一旦被调用就不再返回,并且调用注册过的回调函数。所以这个函数必须放在注册回调函数的后面,此例中为glutReshapeFunc,glutDisplayFunc。
Matlab的GUI回调函数
Kinds of Callbacks The following table lists the callback properties that are available, their triggering events, and the components to which they apply. Note:User interface controls include push buttons, sliders, radio buttons, check boxes, editable text boxes, static text boxes, list boxes, and toggle buttons. They are sometimes referred to as uicontrols.
GUIDE Callback Arguments All callbacks in a GUIDE-generated GUI code file have the following standard input arguments: hObject —Handle of the object, e.g., the GUI component, for which the callback was triggered. For a button group SelectionChangeFcn callback, hObject is the handle of the selected radio button or toggle button. eventdata — Sequences of events triggered by user actions such as table selections emitted by a component in the form of a MATLAB struct (or an empty matrix for components that do not generate eventdata) handles — A MATLAB struct that contains the handles of all the objects in the GUI, and may also contain application-defined data. See handles Structure for information about this structure. Object Handle The first argument is the handle of the component issuing the callback. Use it to obtain relevant properties that the callback code uses and change them as necessary. For example, theText = get(hObject,'String'); places the String property (which might be the contents of static text or name of a button) into the local variable theText. You can change the property by setting it, for example set(hObject,'String',date) This particular code changes the text of the object to display the current date. Event Data Event data is a stream of data describing user gestures, such as key presses, scroll wheel movements, and mouse drags. The auto-generated callbacks of GUIDE GUIs can access event data for Handle Graphics? and uicontrol and uitable object callbacks. The following ones receive event data when triggered: CellEditCallback in a uitable CellSelectionCallback in a uitable KeyPressFcn in uicontrols and figures KeyReleaseFcn in a figure SelectionChangeFcn in a uibuttongroup WindowKeyPressFcn in a figure or any of its child objects WindowKeyReleaseFcn in a figure or any of its child objects WindowScrollWheelFcn in a figure Event data is passed to GUIDE-generated callbacks as the second of three standard arguments. For components that issue no event data the argument is empty. For those that provide event data, the argument contains a structure, which varies in composition according to the component that generates it and the type of event. For example, the event data for a key-press provides information on the key(s) currently being pressed. Here is a GUIDE-generated KeyPressFcn callback template: % --- Executes on key press with focus on checkbox1 and none of its controls. function checkbox1_KeyPressFcn(hObject, eventdata, handles)
特殊疑问句和一般疑问句的用法
(一)由be(am,is,are)引导的一般疑问句 1、Am I a student? 我是学生吗? Yes,you are./ No,you aren’t. 2、Is this /that/it a chair?这/那/它/是一把椅子吗? Yes,it is. /No,it isn’t. 3、Is she/Amy your sister?她/艾米是你的妹妹吗?Yes,she is ./ No,she isn’t. 4、Is he/Mike your brother?他/迈克是你的哥哥吗?Yes,he is./No,he isn’t. 5、Is your brother helpful at home? 你哥哥在家有用吗?Yes, he is./ No, he isn’t. 6、Is there a forest in the park? 在公园有一个森林吗? Yes,there is./No,there isn’t. 是的,有。/不,没有。 7、Are there any panda s in the mountains?在山上有熊猫吗? Yes,there are./No,there aren’t.是的,有。/不,没有。 8、Are they dusk s? 它们是鸭子吗?(问物) Yes, they are. /No,they aren’t.是的,它们是。/不,它们不是。 9、Are they famers? 他们是农民吗?(问人) Yes, they are. /No,they aren’t. 是的,他们是。/不,他们不是。 10、Are you a teacher?你是一个老师吗?(问you 用I回答) Yes, I am./No,I’m not.是的,我是。/不,我不是。 11、Are you teacher s?你们是老师吗?
Yes,we are./No,we aren’t.是的,我们是。/不,我们不是。 (二)、由do引导的一般疑问句