JSON数据读写
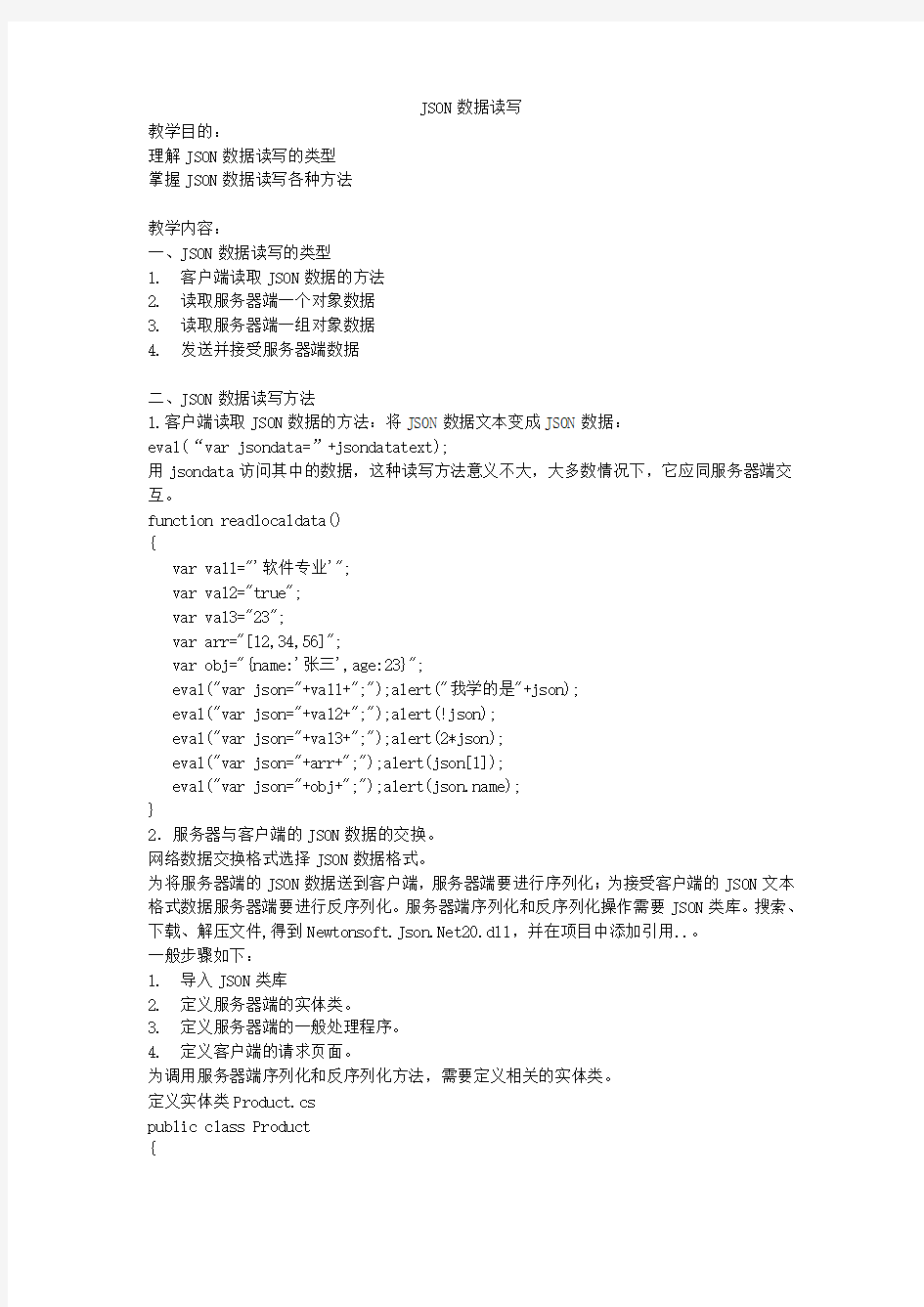

JSON数据读写
教学目的:
理解JSON数据读写的类型
掌握JSON数据读写各种方法
教学内容:
一、JSON数据读写的类型
1.客户端读取JSON数据的方法
2.读取服务器端一个对象数据
3.读取服务器端一组对象数据
4.发送并接受服务器端数据
二、JSON数据读写方法
1.客户端读取JSON数据的方法:将JSON数据文本变成JSON数据:
eval(“var jsondata=”+jsondatatext);
用jsondata访问其中的数据,这种读写方法意义不大,大多数情况下,它应同服务器端交互。
function readlocaldata()
{
var val1="'软件专业'";
var val2="true";
var val3="23";
var arr="[12,34,56]";
var obj="{name:'张三',age:23}";
eval("var json="+val1+";");alert("我学的是"+json);
eval("var json="+val2+";");alert(!json);
eval("var json="+val3+";");alert(2*json);
eval("var json="+arr+";");alert(json[1]);
eval("var json="+obj+";");alert(https://www.360docs.net/doc/e14563188.html,);
}
2.服务器与客户端的JSON数据的交换。
网络数据交换格式选择JSON数据格式。
为将服务器端的JSON数据送到客户端,服务器端要进行序列化;为接受客户端的JSON文本格式数据服务器端要进行反序列化。服务器端序列化和反序列化操作需要JSON类库。搜索、下载、解压文件,得到https://www.360docs.net/doc/e14563188.html,20.dll,并在项目中添加引用..。
一般步骤如下:
1.导入JSON类库
2.定义服务器端的实体类。
3.定义服务器端的一般处理程序。
4.定义客户端的请求页面。
为调用服务器端序列化和反序列化方法,需要定义相关的实体类。
定义实体类Product.cs
public class Product
{
public string Name { get; set; }
public DateTime Expiry { get; set; }
public float Price { get; set; }
public string[] Sizes { get; set; }
}
定义实体类Person.cs
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public Person(string name, int age)
{
Name = name;
Age = age;
}
}
用一般处理程序ashx接受并响应客户端。
定义返回一个对象数据的服务器端处理程序GetProduct.ashx
<%@ WebHandler Language="C#" Class="GetProduct" %>
using System;
using System.Web;
using Newtonsoft.Json;
using Newtonsoft.Json.Serialization;
public class GetProduct : IHttpHandler {
public void ProcessRequest (HttpContext context) {
context.Response.ContentType = "text/plain";
Product product = new Product();
https://www.360docs.net/doc/e14563188.html, = "Apple";
product.Expiry = new DateTime(2008, 12, 28);
product.Price = 3.99F;
product.Sizes = new string[] { "Small", "Medium", "Large" }; string output = JsonConvert.SerializeObject(product);
context.Response.Write(output);
}
public bool IsReusable {
get {
return false;
}
}
}
定义返回一组对象数据的服务器端处理程序
GetPersons.ashx
<%@ WebHandler Language="C#" Class="GetPersons" %>
using System;
using System.Web;
using Newtonsoft.Json;
using Newtonsoft.Json.Serialization;
public class GetPersons : IHttpHandler {
public void ProcessRequest (HttpContext context) {
context.Response.ContentType = "text/plain";
Person[] persons = new Person[]{
new Person("aa",32),
new Person("bb",33),
new Person("cc",34),
new Person("dd",35)};
//序列化
string output = JsonConvert.SerializeObject(persons);
context.Response.Write(output);
}
}
发送客户端请求数据并接受服务器端响应数据GetClientJSON.ashx
<%@ WebHandler Language="C#" Class="GetClientJSON" %>
using System;
using System.Web;
using Newtonsoft.Json;
using Newtonsoft.Json.Serialization;
public class GetClientJSON : IHttpHandler
{
public void ProcessRequest(HttpContext context)
{
context.Response.ContentType = "text/plain";
//string jsonText = "{name:'aa',age:23}";
string jsonText = context.Request["person"];
//反序列化
Person person = (Person)JsonConvert.DeserializeObject(jsonText, typeof(Person));
context.Response.Write(https://www.360docs.net/doc/e14563188.html,+"
"+person.Age);
}
定义客户端页面,用AJAX方式请求并更新页面
读取服务器端数据GetServerData.htm
function readdata()
{
$.getJSON("getproduct.ashx", function(data){
$("div#product").append(https://www.360docs.net/doc/e14563188.html,+"
");
$("div#product").append(data.Expiry+"="+ChangeDateFormat(data.Expiry)+"
");
$("div#product").append(data.Price+"
");
for(i=0;i //或:for(i in data.Sizes) $("div#product").append(data.Sizes[i]+" "); $("div#product").append(" }); } function readdataarray() { $.getJSON("getpersons.ashx", function(data){ for(i in data) { $("div#persons").append(data[i].Name+" "+data[i].Age+" } $.getJSON("getpersons.ashx", function(data){ var s= " { s+=" } s+="
");
");
" "; for(i in data)Name Age ";"+data[i].Name+" "+data[i].Age+"
$("div#persons").append(s);
$("table tr:odd").css('background','#fee');
$("table tr:even").css('background','#efe');
$("table tr td").bind('mouseenter mouseleave',function()
{
$(this).parent().children().toggleClass('mouse');
}
);
}
function senddata()
{
$.post("GetClientJSON.ashx",{person:"{ age:23,name:'aa'}"},function(data) {
$("div#person").append(data);
});
}
function ChangeDateFormat(cellval)//日期格式的转换
{
var date = new Date(parseInt(cellval.replace("/Date(", "").replace(")/", ""), 10));
var month = date.getMonth() + 1 < 10 ? "0" + (date.getMonth() + 1) : date.getMonth() + 1;
var currentDate = date.getDate() < 10 ? "0" + date.getDate() : date.getDate(); return date.getFullYear() + "-" + month + "-" + currentDate;
}
作业:
举例说明JSON数据客户端解析方法
举例说明用JSON数据格式获取登录信息的过程。
从数据集中读取JSON数据
<%@ WebHandler Language="C#" Class="GetData" %>
using System;
using System.Web;
using System.Data;
using System.Data.SqlClient;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
public class GetData : IHttpHandler
{
public void ProcessRequest (HttpContext context) {
context.Response.ContentType = "text/plain";
SqlConnection cnn = new SqlConnection("Data Source=.;Initial Catalog=Northwind;User ID=sa;Password=123");
SqlDataAdapter adp = new SqlDataAdapter("SELECT CategoryID,CategoryName FROM Categories", cnn);
DataSet ds = new DataSet();
adp.Fill(ds);
DataRowCollection rows = ds.Tables[0].Rows;
//直接序列化ds.Tables[0].Rows会产生许多的冗余信息。
Category[] Categories=new Category[rows.Count];
for (int i = 0; i < rows.Count; i++)
Categories[i] = new Category((int)rows[i]["CategoryID"], rows[i]["CategoryName"].ToString());
context.Response.Write(JsonConvert.SerializeObject(Categories));
}
public bool IsReusable {
get {
return false;
}
}
//定义内部实体类
public class Category
{
int categoryID;
public int CategoryID
{
get { return categoryID; }
set { categoryID = value; }
}
string categoryName;
public string CategoryName
{
get { return categoryName; }
set { categoryName = value; }
}
public Category(int categoryID,string categoryName) {
this.categoryID = categoryID;
this.categoryName = categoryName;
}
}
}
显示数据如下:
[
{"CategoryID":1,"CategoryName":"Beverages"}, {"CategoryID":2,"CategoryName":"Condiments"}, {"CategoryID":3,"CategoryName":"Confections"}, {"CategoryID":4,"CategoryName":"Dairy Products"}, {"CategoryID":5,"CategoryName":"Grains/Cereals"}, {"CategoryID":6,"CategoryName":"Meat/Poultry"}, {"CategoryID":7,"CategoryName":"Produce"}, {"CategoryID":8,"CategoryName":"Seafood"}
]
后台转换JSON数据类型,前台解析JSON数据等等例子总结
后台转换JSON数据类型,前台解析JSON数据等等例子总结 JSON对象: JSONObject obj = new JSONObject(); obj.put("result", 0); obj.put("message", message); return obj.toJSONString(); 前台解析: Success:function(data){ var result = data.result; var message = data.message; } json对象中有json对象的写法: Objstr为一个JSONObject obj的String转换 private String getsuccess(String objstr,int number){ JSONObject obj = new JSONObject(); obj.put("result", 1); obj.put("obj", objstr); obj.put("number", number); return obj.toJSONString(); }
前台解析: Picjson为success返回的data var result = picjson.result; if (result==1) { var objdata = picjson.obj; var data = eval('(' + objdata + ')'); var num = picjson.number; picurl = null; showpiclist(data, num); } else{ alert(picjson.message); picurl = null; } list转成json对象 需要的包: https://www.360docs.net/doc/e14563188.html,mons-lang.jar https://www.360docs.net/doc/e14563188.html,mons-beanutils.jar https://www.360docs.net/doc/e14563188.html,mons-collections.jar https://www.360docs.net/doc/e14563188.html,mons-logging.jar
Java获取http和https协议返回的json数据
Java获取http和https协议返回的json数据 现在很多公司都是将数据返回一个json,而且很多第三方接口都是返回json数据,而且还需要使用到http协议,http协议是属于为加密的协议,而https协议需要SSL证书,https是将用户返回的信息加密处理,然而我们要获取这些数据,就需要引入SSL证书。现在我提供两个方法,帮助各位如何获取http和https返回的数据。 获取http协议的数据的方法,如下: public static JSONObject httpRequest(String requestUrl, String requestMethod) { JSONObject jsonObject = null; StringBuffer buffer = new StringBuffer(); try { URL url = new URL(requestUrl); // http协议传输 HttpURLConnection httpUrlConn = (HttpURLConnection) url.openConnection(); httpUrlConn.setDoOutput(true); httpUrlConn.setDoInput(true); httpUrlConn.setUseCaches(false); // 设置请求方式(GET/POST)
httpUrlConn.setRequestMethod(requestMethod); if ("GET".equalsIgnoreCase(requestMethod)) httpUrlConn.connect(); // 将返回的输入流转换成字符串 InputStream inputStream = httpUrlConn.getInputStream(); InputStreamReader inputStreamReader = new InputStreamReader(inputStream, "utf-8"); BufferedReader bufferedReader = new BufferedReader(inputStreamReader); String str = null; while ((str = bufferedReader.readLine()) != null) { buffer.append(str); } bufferedReader.close(); inputStreamReader.close(); // 释放资源 inputStream.close(); inputStream = null; httpUrlConn.disconnect(); jsonObject =
jQuery+AJAX+JSON
jQuery 1. 什么是jQuery?? jQuery是一个优秀的JavaScript框架,一个轻量级的JavaScript类库。 jQuery的核心理念是Write less,Do more。 使用jQuery可以兼容各种浏览器,方便的处理HTML、Events、动画效果等,并且方便的为网站提供AJAX交互。 2.jQuery的特点: 利用选择器来查找要操作的节点,然后将这些节点封装成一个jQuery对象,通过调用jQuery对象的方法或者属性来实现对底层被封装的节点的操作。 好处:a、兼容性更好;b、代码更简洁 3.编程步骤: step1、使用选择器查找节点 step2、调用jQuery的属性和方法 4.jQuery对象与DOM对象之间的转换 1. 什么是jQuery对象?? jQuery对象是jQuery对底层对象的一个封装,只有创建了这个对象,才能使用类库中提供的方法。 2. DOM对象 ----> jQuery对象 DOM对象向jQuery对象的转变很容易,外面追加$和圆括号即可。 function f( ){ var obj = document.getElementById(‘d1’); //DOM -> jQuery对象
var $obj = $(obj); $obj.html(‘hello jQuery’); } 3. jQuery对象 ----> DOM对象 jQuery对象向DOM对象转化,通过调用get方法加参数值0即可$obj.get(0)。 function f( ){ var $obj = $(‘#d1’); //jQuery对象 -> DOM var obj = $(obj).get (0); obj.innerHTML = ‘hello jQuery’; } 5. jQuery选择器 1. 什么是jQuery选择器?? jQuery选择器是一种类似CSS选择器的特殊说明符号,能够帮助jQuery 定位到要操作的元素上,使用了选择器可以帮助HTML实现内容与行为的分离。只需要在元素上加上Id属性。 2. 选择器的种类 a、基本选择器 #id根据指定的ID匹配一个元素 .class根据指定的类匹配一个元素 element根据的指定的元素名匹配所有的元素
Jquery Ajax 异步处理Json数据
啥叫异步,啥叫Ajax.咱不谈啥XMLHTTPRequest.通俗讲异步就是前台页面javascript能调用后台方法.这样就达到了无刷新.所谓的Ajax.这里我们讲二种方法 方法一:(微软有自带Ajax框架) 在https://www.360docs.net/doc/e14563188.html,里微软有自己的Ajax框架.就是在页面后台.cs文件里引入 using System.Web.Services 空间然后定义静态方法(方法前加上 [WebMethod]) [WebMethod] public static string ABC(string ABC) { return ABC; } 好了,现在我们谈谈前台Js怎么处理后台返回的数据吧,可利用Jquery处理返回的纯html,json,Xml等数据.这里我们演示返回返回的数据有string、集合(List<>)、类. 但都返回Json格式(Json轻量级比XML处理起来简单).看看前台是怎么解析这些数据的. 代码如下: 前台页面: <%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>