Java_循环链表
javafor循环结构

javafor循环结构Java中,for循环是一种重要的循环结构,它主要用于重复执行某个任务。
for循环通常包括三个部分:初始化表达式、循环条件和更新表达式,它通过控制循环变量的取值来控制循环的次数。
具体地讲,for循环的语法如下:```for (初始化表达式; 循环条件; 更新表达式) {// 循环体代码}```其中,初始化表达式用于初始化循环变量;循环条件是一个布尔表达式,用于判断循环变量是否满足条件;更新表达式用于更新循环变量的值。
在每次循环迭代开始时,都会先执行初始化表达式,然后判断循环条件是否满足,如果满足则进入循环体执行任务,否则跳出循环。
下面是一个简单的例子,演示了如何使用for循环输出1到10之间的所有整数:```javafor (int i = 1; i <= 10; i++) {System.out.print(i + " ");}```执行结果为:1 2 3 4 5 6 7 8 9 10在实际开发中,for循环常常和其他语句结合使用,以实现更为复杂的逻辑。
例如,我们可以使用for循环遍历数组中的元素,计算数组中所有元素的和,并输出结果:```javaint[] arr = {1, 2, 3, 4, 5};int sum = 0;for (int i = 0; i < arr.length; i++) {sum += arr[i];}System.out.println("数组元素的和为:" + sum);```执行结果为:数组元素的和为:15除了简单的计数循环和数组遍历,for循环还可以嵌套,以实现更加复杂的逻辑。
例如,我们可以使用for循环嵌套输出九九乘法表:```javafor (int i = 1; i <= 9; i++) {for (int j = 1; j <= i; j++) {System.out.print(j + "×" + i + "=" + i * j + "\t");}System.out.println();}```执行结果为:1×1=11×2=22×2=41×3=32×3=63×3=91×4=42×4=83×4=124×4=161×5=52×5=103×5=154×5=205×5=251×6=62×6=123×6=184×6=245×6=306×6=36 1×7=72×7=143×7=214×7=285×7=356×7=42 7×7=491×8=82×8=163×8=244×8=325×8=406×8=487×8=568×8=641×9=92×9=183×9=274×9=365×9=456×9=54 7×9=638×9=729×9=81总之,for循环是Java编程中常用的循环结构,它可以帮助我们简化复杂的逻辑,提高代码的复用性和可读性。
java中while循环的用法示例

java中while循环的用法示例while循环是一种在满足条件时重复执行一段代码的结构。
它会在每次循环开始之前检查一个条件,如果条件为真,则执行循环体内的代码。
只有在条件为假时,循环才会停止执行。
下面是一些使用while循环的常见示例:1.循环指定的次数```javaint count = 0;while (count < 5)System.out.println("循环次数:" + count);count++;```这个例子使用while循环打印出循环次数,直到count的值达到5为止。
2.读取用户输入直到满足条件```javaimport java.util.Scanner;Scanner scanner = new Scanner(System.in);int input = 0;while (input != 5)System.out.println("请输入一个数字(输入5以停止):");input = scanner.nextInt(;```这个例子使用while循环读取用户输入的数字,直到输入的数字为5为止。
3.迭代数组元素```javaint[] numbers = {1, 2, 3, 4, 5};int index = 0;while (index < numbers.length)System.out.println(numbers[index]);index++;```这个例子使用while循环迭代数组中的元素,并打印每个元素的值。
4.循环处理链表节点```javaclass Nodeint value;Node next;Node(int value)this.value = value;}Node head = new Node(1);head.next = new Node(2);head.next.next = new Node(3);Node current = head;while (current != null)System.out.println(current.value);current = current.next;```这个例子使用while循环遍历链表中的节点,并打印每个节点的值。
java linkedlist 方法

Java LinkedList 类提供了许多方法,用于操作链表。
以下是一些常用的LinkedList 方法:1. add(E e):在链表末尾添加元素e。
2. add(int index, E element):在指定位置index 插入元素element。
3. addFirst(E e):在链表头部添加元素e。
4. addLast(E e):在链表尾部添加元素e。
5. clear():移除链表中的所有元素。
6. contains(Object o):判断链表中是否包含元素o。
7. containsAll(Collection<?> c):判断链表中是否包含集合c 中的所有元素。
8. get(int index):获取链表中指定位置index 的元素。
9. getFirst():获取链表头部的元素。
10. getLast():获取链表尾部的元素。
11. remove(Object o):移除链表中第一个出现的指定元素o。
12. remove(int index):移除链表中指定位置index 的元素。
13. removeFirst():移除链表头部的元素。
14. removeLast():移除链表尾部的元素。
15. size():返回链表中元素的个数。
16. isEmpty():判断链表是否为空。
17. isSingleton():判断链表是否只有一个元素。
18. poll():移除并返回链表头部的元素,如果链表为空则返回null。
19. pop():移除并返回链表尾部的元素,如果链表为空则抛出NoSuchElementException 异常。
20. peek():返回链表头部的元素,但不移除,如果链表为空则返回null。
21. push(E e):将元素e 添加到链表头部。
22. offer(E e):将元素e 添加到链表尾部,如果成功则返回true,否则返回false。
23. removeFirstOccurrence(Object o):移除链表中第一个出现的指定元素o。
Java基础之代码死循环详解

Java基础之代码死循环详解⽬录⼀、前⾔⼆、死循环的危害三、哪些场景会产⽣死循环?3.1 ⼀般循环遍历3.1.1 条件恒等3.1.2 不正确的continue3.1.3 flag线程间不可见3.2 Iterator遍历3.3 类中使⽤⾃⼰的对象3.4 ⽆限递归3.5 hashmap3.5.1 jdk1.7的HashMap3.5.2 jdk1.8的HashMap3.5.3 ConcurrentHashMap3.6 动态代理3.7 我们⾃⼰写的死循环3.7.1 定时任务3.7.2 ⽣产者消费者四、⾃⼰写的死循环要注意什么?⼀、前⾔代码死循环这个话题,个⼈觉得还是挺有趣的。
因为只要是开发⼈员,必定会踩过这个坑。
如果真的没踩过,只能说明你代码写少了,或者是真正的⼤神。
尽管很多时候,我们在极⼒避免这类问题的发⽣,但很多时候,死循环却悄咪咪的来了,坑你于⽆形之中。
我敢保证,如果你读完这篇⽂章,⼀定会对代码死循环有⼀些新的认识,学到⼀些⾮常实⽤的经验,少⾛⼀些弯路。
⼆、死循环的危害我们先来⼀起了解⼀下,代码死循环到底有哪些危害?程序进⼊假死状态,当某个请求导致的死循环,该请求将会在很⼤的⼀段时间内,都⽆法获取接⼝的返回,程序好像进⼊假死状态⼀样。
cpu使⽤率飙升,代码出现死循环后,由于没有休眠,⼀直不断抢占cpu资源,导致cpu长时间处于繁忙状态,必定会使cpu使⽤率飙升。
内存使⽤率飙升,如果代码出现死循环时,循环体内有⼤量创建对象的逻辑,垃圾回收器⽆法及时回收,会导致内存使⽤率飙升。
同时,如果垃圾回收器频繁回收对象,也会造成cpu使⽤率飙升。
StackOverflowError,在⼀些递归调⽤的场景,如果出现死循环,多次循环后,最终会报StackOverflowError栈溢出,程序直接挂掉。
三、哪些场景会产⽣死循环?3.1 ⼀般循环遍历这⾥说的⼀般循环遍历主要是指:for语句foreach语句while语句这三种循环语句可能是我们平常使⽤最多的循环语句了,但是如果没有⽤好,也是最容易出现死循环的问题的地⽅。
java 循环语句用法

java 循环语句用法Java是一种面向对象的编程语言,它提供了多种循环语句来帮助程序员实现重复执行某些代码的功能。
循环语句是Java编程中最常用的语句之一,它可以让程序重复执行某些代码,直到满足某个条件为止。
在本文中,我们将介绍Java中常用的循环语句及其用法。
1. for循环for循环是Java中最常用的循环语句之一,它可以让程序重复执行某些代码,直到满足某个条件为止。
for循环的语法如下:for (初始化; 条件; 更新) {// 循环体}其中,初始化语句用于初始化循环变量,条件语句用于判断循环是否继续执行,更新语句用于更新循环变量的值。
for循环的执行过程如下:1. 执行初始化语句;2. 判断条件语句的值,如果为true,则执行循环体,然后执行更新语句;3. 再次判断条件语句的值,如果为true,则重复执行步骤2,否则退出循环。
2. while循环while循环是Java中另一种常用的循环语句,它可以让程序重复执行某些代码,直到满足某个条件为止。
while循环的语法如下:while (条件) {// 循环体}其中,条件语句用于判断循环是否继续执行。
while循环的执行过程如下:1. 判断条件语句的值,如果为true,则执行循环体;2. 再次判断条件语句的值,如果为true,则重复执行步骤1,否则退出循环。
3. do-while循环do-while循环是Java中另一种常用的循环语句,它可以让程序重复执行某些代码,直到满足某个条件为止。
do-while循环的语法如下:do {// 循环体} while (条件);其中,条件语句用于判断循环是否继续执行。
do-while循环的执行过程如下:1. 执行循环体;2. 判断条件语句的值,如果为true,则重复执行步骤1,否则退出循环。
4. 增强for循环增强for循环是Java中一种简化版的for循环,它可以遍历数组或集合中的元素。
增强for循环的语法如下:for (元素类型元素变量 : 数组或集合) {// 循环体}其中,元素类型用于指定数组或集合中元素的类型,元素变量用于指定当前循环中的元素。
循环链表

2、循环链表import java.io.*;class student {public String name;public int score;public student next;}class CircularList{static student ptr,head,current,prev;static DataInputStream infile;static DataOutputStream outfile;CircularList(){head =new student();head.next=head;}public static void anykey_f(){char tChar;System.out.println(" Press any key to continue...");try{tChar=(char)System.in.read();}catch(IOException e){}}public static void load_f(){boolean done=false;System.out.print("File loading...");try{infile =new DataInputStream(new FileInputStream("circularlist.dat"));}catch(IOException e){System.err.println("failed!");System.err.println("circularlist.dat not found!");return;}while(done==false){ptr=new student();try{=infile.readUTF();ptr.score=infile.readInt();}catch(EOFException eof){done =true;}catch(IOException e){}if(done==true)ptr=null;}System.out.println("OK!");try{infile.close();}catch(IOException e){}}public static void save_f(){student node;System.out.print("File saving...");try{outfile =new DataOutputStream(new FileOutputStream("circularlist.dat"));}catch(IOException e){System.out.println("failed!");return;}node=head.next;do{try{outfile.writeUTF();outfile.writeInt(node.score);}catch(IOException e){}node=node.next;}while(node!=head);System.out.println("OK!");try{outfile.close();}catch(IOException e){}}public static void insert_f() {DataInputStream in =new DataInputStream(System.in);String st=" ";ptr=new student();System.out.println("\n\n************Insert Node************\n");System.out.print(" Please enter student name:");System .out.flush();try{=in.readLine();} catch (IOException e){}System.out.print("Please enter student score:");System.out.flush();try{st=in.readLine();}catch(IOException e) {}try{ptr.score=Integer.valueOf(st).intValue();} catch(NumberFormatException e){}System.out.println("\n*************************************\n\n");prev=head;current=head.next;if(current!=null){while((current!=head)&&(current.score>=ptr.score)){prev=current;current=current.next;}}ptr.next=current;prev.next=ptr;}public static void delete_f() {DataInputStream in =new DataInputStream(System.in);String del_name="";int count=0;if(head.next==null)System.out.println("\n\n No student record!!\n");else{System.out.println("\n\n************Delete Node************\n");System.out.print(" Delete student name:");System.out.flush();try{del_name=in.readLine();}catch(IOException e) {}prev=head;current=head.next;while((current!=head)&&(!(del_name.equals()))){prev=current;current=current.next;}if(current !=null){prev.next=current.next;current=null;System.out.print("Student"+del_name+"record deleted\n");}elseSystem.out.print("Student"+del_name+"not found\n");}anykey_f();System.out.println("\n*************************************\n\n");}public static void modify_f() {DataInputStream in =new DataInputStream(System.in);int count=0;String n_temp="",st="";if(head.next==null)System.out.println("\n\n No student record!!\n");else{System.out.println("\n\n************Modify Node************\n");System.out.print(" Modify student name:");System.out.flush();try{n_temp=in.readLine();}catch(IOException e) {}current=head.next;do{if(n_temp.equals()){System.out.println("\n*************************************\n\n");System.out.print("Student name:"++"\n");System.out.print("Student score:"+current.score+"\n");System.out.println("\n*************************************\n\n");System.out.print(" Please enter new score:");System.out.flush();try {st =in.readLine();}catch (IOException e){}try {current.score=Integer.valueOf(st).intValue();}catch (NumberFormatException e){}count++;}current=current.next;}while (current !=head);if (count>0)System.out.println("\n "+count +"student record(s) modified !!\n");elseSystem.out.println ("\n Student "+n_temp +"not found !!\n");}anykey_f();System.out.println("\n******************************\n\n");}public static void dispiay_f(){int count=0;if(head.next==null)System.out.println ("\n\n NO student record !!\n");else {System.out.println("\n\n*************Dispiay Node ************\n");System.out.println(" NAME SCORE");System.out.println("-------------------------------");current=head.next;do{System.out.print(" "+current .name+" ");System.out.println(current .score);count++;current=current.next;if(count % 20 ==0)anykey_f();}while (current!=head);System.out.println("-------------------\n");System.out.println(" Total "+count +"record(s) found!!\n");}anykey_f();System.out.println("***************************\n\n");}public static void main (String args[]){DataInputStream in =new DataInputStream(System.in);String op="";int option=0;CircularList obj =new CircularList();load_f();do{System.out.println("****** CircularList Program *****");System.out.println(" ");System.out.println(" <1> Insert Node ");System.out.println(" <2> Delete Node ");System.out.println(" <3> Modify Node ");System.out.println(" <4> List Node ");System.out.println(" <5> Exit ");System.out.println(" ");System.out.println("***********************************");System.out.println("\n Choice :");System.out.flush();op="";try{op=in.readLine();}catch (IOException e){}option=0;try{option=Integer.valueOf(op).intValue();}catch (NumberFormatException e){System.out.println("\n Please input (1,2,3,4,5) ...");}switch(option){case 1:obj.insert_f();break;case 2:obj.delete_f();break;case 3:obj.modify_f();break;case 4:obj.dispiay_f();break;case 5:obj.save_f();System.exit(0);}}while (true);}}3、双向链表import java.io.*;class student {public String name ="";public int score=0;public student llink;public student rlink;}class DoubleList{static student prev,ptr,head,current;static DataInputStream infile;static DataOutputStream outfile;DoubleList (){ptr = new student (); = "0";ptr.llink = ptr;ptr.rlink = ptr;head = ptr ;}public static void anykey_f(){char tChar;System.out.println(" press any key to continue ... ");try {tChar = (char) System.in.read () ;} catch (IOException e) {}}public static void load_f(){boolean done =false;System.out.print("File loading...");try{infile=new DataInputStream(new FileInputStream("dblink.dat"));} catch(IOException e) {System.err.println("failed!");System.err.println("dblink.dat not found!");return;}while(done==false){ptr=new student();try{=infile.readUTF();ptr.score=infile.readInt();}catch(EOFException eof){done=true;}catch(IOException e){}if(done==true)ptr=null;}System.out.println("OK!");try{infile.close();}catch(IOException e){}}public static void save_f(){student node;System.out.print("File saveing...");try{outfile=new DataOutputStream(new FileOutputStream("dblink.dat"));}catch(IOException e){System.out.println("failed!");return;}node=head;do{try{outfile.writeUTF();outfile.writeInt(node.score);}catch(IOException e){}node=node.rlink;}while(node!=head);System.out.println("OK!");try{outfile.close();}catch(IOException e) {}}public static void insert_f(){DataInputStream in = new DataInputStream(System.in);String name_t="" , st="";ptr = new student();System.out.print("\n please enter student name : ");System.out.flush();try { = in.readLine();} catch (IOException e) {}System.out.print(" please enter student score: ");System.out.flush();try {st = in.readLine();} catch (IOException e) {}try {ptr.score = Integer.valueOf (st).intValue();} catch (NumberFormatException e) {}System.out.println("");prev = head;current = head.rlink;while ((current != head) && (current.score >= ptr.score)) {prev = current;current = current.rlink;}ptr.rlink = current;ptr.llink = prev;prev.rlink = ptr;current.llink = ptr;}public static void delete_f(){DataInputStream in=new DataInputStream(System.in);String del_name="";int count =0;student clear;if(head.rlink==head)System.out.println("\n No student record !!");else{System.out.print("\n Delete student name:");System.out.flush();try{del_name=in.readLine();}catch (IOException e){}prev=head;current=head.rlink;while((current.rlink!=head)&&(! del_name.equals())){ prev=current;current=current.rlink;}prev.rlink=current.rlink ;current.rlink.llink=prev;current=null;System.out.println("Student "+del_name +"record(s) deleted!\n");}if (current==head){System.out.println("Student "+del_name +"not found!\n");}anykey_f();}public static void modify_f(){DataInputStream in=new DataInputStream(System.in);int count=0;String n_temp="",s_temp="";if(head.rlink==head)System.out.println("\n No student record !!");else{System.out.print("\n Modify student name: ");System.out .flush();try{n_temp=in.readLine();}catch(IOException e){}current=head.rlink;while(current!=head){if(n_temp.equals()) {System.out.println("\n ***************************");System.out.println(" Student name:"+);System.out.println(" Student score:"+current.score);System.out.println(" ***************************\n");System.out.print("Please enter new score:");System.out.flush();try{s_temp=in.readLine();}catch (IOException e) {System.out.println(e);}try{current.score=Integer.valueOf(s_temp).intValue();} catch(NumberFormatException e){System.out.println(e);}count++;}current=current.rlink;}if(count>0)System.out.println(" "+count+"student record(s) modified!!\n"); elseSystem.out.println(" Student"+n_temp+"not found!!\n");}anykey_f();}public static void display_f(){int count=0;if(head.rlink==head)System.out.println("\n No student record!!");else{System.out.println("\n NAME SCORE");System.out.println(" --------------------------");current=head.rlink;while(current!=head){System.out.println(" "++" "+current.score);count++;current=current.rlink;if(count%20==0)anykey_f();}System.out.println("--------------");System.out.println(" Total"+count+"record(s)found!!\n");}anykey_f();}public static void main (String args[]){DataInputStream in=new DataInputStream(System.in);String op="";int option=0;DoubleList DBLink =new DoubleList();load_f();do{System.out.println("******* Double Link Program ********");System.out.println(" ");System.out.println(" <1>Insert Node ");System.out.println(" <2>Delete Node ");System.out.println(" <3>Modify Node ");System.out.println(" <4>List Node ");System.out.println(" <5>Exit ");System.out.println(" ");System.out.println("**************************************");System.out.print(" \n choice: ");System.out.flush();op="";try {op=in.readLine();} catch (IOException e) {System .out.println(e);}option=0;try {option=Integer.valueOf(op).intValue();}catch (NumberFormatException e){System.out.println("\n Please input (1,2,3,4,5)...");System.out.println ("\n\n\n");}switch (option){case 1:insert_f();break;case 2:delete_f();break;case 3:modify_f();break;case 4:display_f();break;case 5:save_f();System.exit(0);}}while(true);}}4、多项式相加import java.io.*;class Poly{public int coef;public int exp;Poly next;};class Poly_add{public Poly ptr,eq_h1,eq_h2,ans_h;public static void main(String args[]){Poly_add obj=new Poly_add();System.out.print("************************************\n");System.out.print("--Polynomial add using format ax^b--\n");System.out.print("************************************\n");System.out.println("\n<< Please enter the first equation(enter 0 to exit input) >>\n");obj.eq_h1=obj.input();System.out.println("\n<< Please enter the second equation(enter 0 to exit input) >>\n");obj.eq_h2=obj.input();obj.poly_add();System.out.println("");obj.show_poly(obj.eq_h1,"The first equation:");obj.show_poly(obj.eq_h2,"The second equation:");obj.show_poly(obj.ans_h,"The answer equation:");}public Poly input(){Poly eq_h =null,prev=null;String temp;DataInputStream in=new DataInputStream(System.in);while(true){ptr=new Poly();ptr.next=null;System.out.print("Enter coefficient...");try{ptr.coef=Integer.valueOf(in.readLine()).intValue();}catch(IOException e){}if(ptr.coef==0)return eq_h;System.out.print("Enter exponent...");try{ptr.exp=Integer.valueOf(in.readLine()).intValue();}catch(IOException e){}if(eq_h==null)eq_h=ptr;elseptr.next=ptr;prev=ptr;}}public void poly_add(){Poly this1,this2,prev;this1=eq_h1;this2=eq_h2;prev=null;while(this1 !=null|| this2!=null){&& ptr=new Poly();ptr.next=null;if((this1 !=null && this2!==null)||this1 != null&& this.exp >this2.exp){ptr.coef=this1.coef;ptr.exp=this1.exp;this1=this1.next;}elseif(this1 !=null || this1.exp<this2.exp){ptr.coef=this2.coef;ptr.exp=this2.exp;this2=this2.next;}else{ptr.coef=this1coef+this2.coef;ptr.exp=this1.exp;if(this1 !=null)this1=this1.next;if(this2 !=null)this2=this2.next;}if(ptr.coef !=0){if (ans_h==null)ans_h=ptr;elseprev.next=ptr;pre=ptr;}elseptr=null;}}public oid show_poly(Poly head,String text){Poly node;node=head;System.out.print(text);while(node!=null){System.out.print(node.coef+"x^"+node.exp);if(node.next!=null && node.next.coef>=0)System.out.print("+");node=node.next;}System.out.print("\n");}}。
java中的4种循环方法示例详情
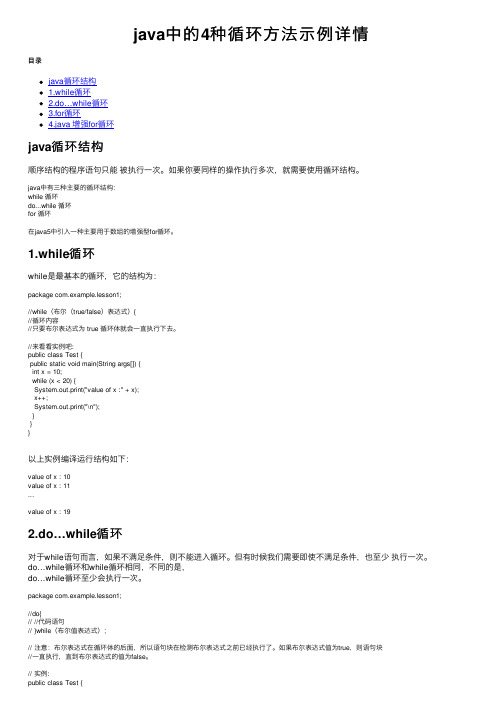
java中的4种循环⽅法⽰例详情⽬录java循环结构1.while循环2.do…while循环3.for循环4.java 增强for循环java循环结构顺序结构的程序语句只能被执⾏⼀次。
如果你要同样的操作执⾏多次,就需要使⽤循环结构。
java中有三种主要的循环结构:while 循环do...while 循环for 循环在java5中引⼊⼀种主要⽤于数组的增强型for循环。
1.while循环while是最基本的循环,它的结构为:package com.example.lesson1;//while(布尔(true/false)表达式){//循环内容//只要布尔表达式为 true 循环体就会⼀直执⾏下去。
//来看看实例吧:public class Test {public static void main(String args[]) {int x = 10;while (x < 20) {System.out.print("value of x :" + x);x++;System.out.print("\n");}}}以上实例编译运⾏结构如下:value of x : 10value of x : 11...value of x : 192.do…while循环对于while语句⽽⾔,如果不满⾜条件,则不能进⼊循环。
但有时候我们需要即使不满⾜条件,也⾄少执⾏⼀次。
do…while循环和while循环相同,不同的是,do…while循环⾄少会执⾏⼀次。
package com.example.lesson1;//do{// //代码语句// }while(布尔值表达式);// 注意:布尔表达式在循环体的后⾯,所以语句块在检测布尔表达式之前已经执⾏了。
如果布尔表达式值为true,则语句块//⼀直执⾏,直到布尔表达式的值为false。
// 实例:public class Test {public static void main(Staing args[]) {int x = 10;do {System.out.print("value of x :" + x);x++;System.out.print("\n");} while (x < 20);}}以上实例编译运⾏结果如下:value of x : 10...value of x :193.for循环虽然所有循环结构都可以⽤while或者do…while表⽰,但java提供了另⼀种语句(for循环),使⼀些循环结构变得更简单。
java中loop的用法
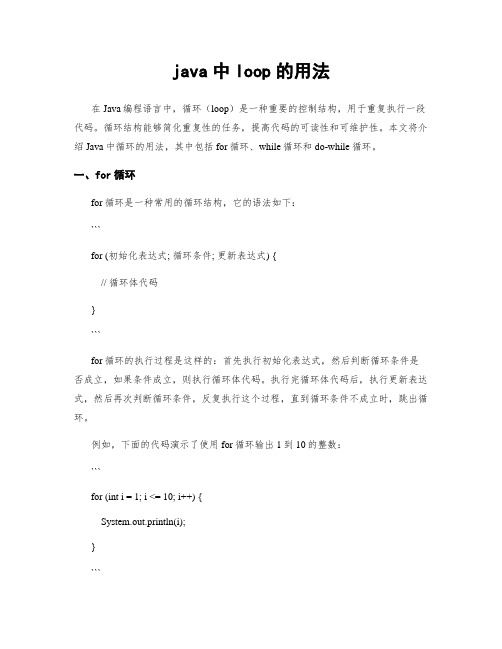
java中loop的用法在Java编程语言中,循环(loop)是一种重要的控制结构,用于重复执行一段代码。
循环结构能够简化重复性的任务,提高代码的可读性和可维护性。
本文将介绍Java中循环的用法,其中包括for循环、while循环和do-while循环。
一、for循环for循环是一种常用的循环结构,它的语法如下:```for (初始化表达式; 循环条件; 更新表达式) {// 循环体代码}```for循环的执行过程是这样的:首先执行初始化表达式,然后判断循环条件是否成立,如果条件成立,则执行循环体代码。
执行完循环体代码后,执行更新表达式,然后再次判断循环条件,反复执行这个过程,直到循环条件不成立时,跳出循环。
例如,下面的代码演示了使用for循环输出1到10的整数:```for (int i = 1; i <= 10; i++) {System.out.println(i);}```在这个例子中,初始化表达式为`int i = 1;`,循环条件为`i <= 10;`,更新表达式为`i++`。
循环体代码`System.out.println(i);`会在每次循环时输出当前的循环变量i 的值。
二、while循环while循环是另一种常用的循环结构,它的语法如下:```while (循环条件) {// 循环体代码}```while循环的执行过程是这样的:首先判断循环条件是否成立,如果成立则执行循环体代码,执行完循环体代码后再次判断循环条件,如此循环,直到循环条件不成立时,跳出循环。
例如,下面的代码演示了使用while循环输出1到10的整数:```int i = 1;while (i <= 10) {System.out.println(i);i++;}```在这个例子中,定义了一个循环变量i,并初始化为1。
在每次循环中,先判断循环条件`i <= 10`是否成立,如果成立,则输出i的值,并将i加1。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
学习数据结构已经三天,刚开始自然遇到线性表。
其中线性表内含顺序表和链表。
顺序表较为简单,仅仅需要一个数组就可以完成,当然最好也要添加一个表示长度的数据成员,如size。
而链表,显然比较多变看,小可不才,用了将近三天的时间才能明白,不能不说见笑于大方之家;皆因链表之中还有循环链表,双向链表,双向循环链表。
好了,言归正传:循环链表的程序奉上:
链表,不过增(insert)删(delete)改(update)查(select)而已。
在于Java程序中,还要加上构造(Java有垃圾回收机制,故没有析构,但可以手动回收)。
先看代码如下:
1、关于构造函数,小生理解到:需要什么样的初始化,就写出什么样的构造函数,当然,没有时也可以构造一个空的构造函数;本人就节点有一个构造函数
2、在方法中,注意index的具体代表就行。
其中,在找上一节点时,很多重复了,可以另外写入一个函数中。
3、最后只是一个测试形式的,可以自己设置
4、自认为一个比较简单的程序了
package link;
class Node {
public int num;
public Node next;
public Node(int num, Node next) {
this.num = num;
this.next = next;
}
}
public class CycleList {
public Node head;
public int size;
public void insertHead(int element){ //在头结点的地方插入if(size == 0){
head = new Node(element, null);
head. next = head;
}else {
Node no = head;
head = new Node(element, no);
}
size ++;
}
public void insert(int index, int element) { //插入元素if (size == 0) {
head = new Node(element, head);
} else {
if (index < 0) {
index = 0;
}
if (index > size) {
index = size;
}
Node no1 = head;
for (int i = 0; i < index - 1; i++) {
no1 = no1.next;
}
Node no2 = new Node(element, no1.next);
no1.next = no2;
}
size++;
}
public void delete(int index) { // 删除函数if (index < 0) {
index = 0;
}
if (index > size) {
index = size;
}
Node no3 = head;
for (int i = 0; i < index - 1; i++) {
no3 = no3.next;
}
no3.next = no3.next.next;
size--;
}
public void select() { // 查询所有元素
int sizelong = size;
Node no4 = head;
for (int i = 0; i < sizelong; i++) {
System.out.print(no4.num);
no4 = no4.next;
}
}
public void update(int index, int element){ //更换index位置的内容Node no7 = head;
for(int i=0; i<index-1; i++){
no7 = no7.next;
}
no7.num = element;
}
public void sel(int index){ //查询index位置的内容Node no8 = head;
for(int i=0; i<index-1; i++){
no8 = no8.next;
}
System.out.println(no8.num);
}
public static void main(String[] args) {
CycleList cl = new CycleList();
cl.insert(0, 4); // index 代表第几个后面,当然,第0个后面,即为插头节点
cl.insert(2, 2); // 无论插入还是删除
cl.insert(3, 5); //更改很准确
cl.insert(4, 6); // 查询单个也是可以的
cl.insert(5, 9);
cl.select();
System.out.print(" ----");
cl.insert(0, 8);
cl.select();
System.out.print(" ----");
cl.insertHead(3);
cl.select();
System.out.println("------");
cl.delete(3);
cl.select();
System.out.println("---------");
cl.update(1, 1);
cl.select();
System.out.print("----");
cl.sel(0);
}
}。