java习题及答案第9章 习题参考答案
《Java语言程序设计(基础篇)》(第10版 梁勇 著)第九章练习题答案
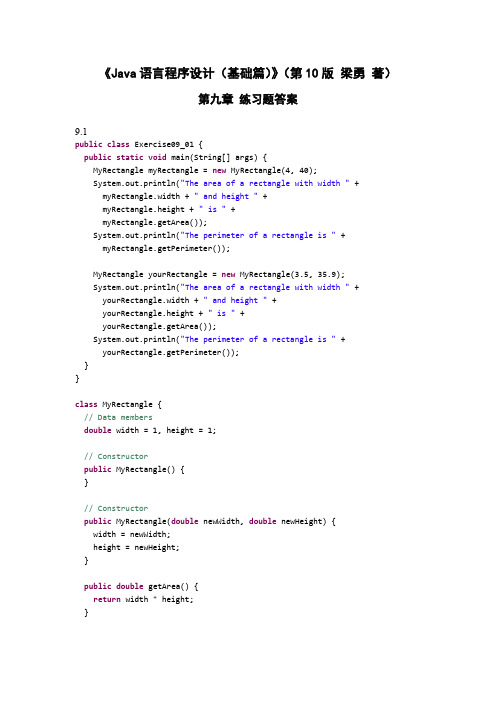
《Java语言程序设计(基础篇)》(第10版梁勇著)第九章练习题答案9.1public class Exercise09_01 {public static void main(String[] args) {MyRectangle myRectangle = new MyRectangle(4, 40);System.out.println("The area of a rectangle with width " +myRectangle.width + " and height " +myRectangle.height + " is " +myRectangle.getArea());System.out.println("The perimeter of a rectangle is " +myRectangle.getPerimeter());MyRectangle yourRectangle = new MyRectangle(3.5, 35.9);System.out.println("The area of a rectangle with width " +yourRectangle.width + " and height " +yourRectangle.height + " is " +yourRectangle.getArea());System.out.println("The perimeter of a rectangle is " +yourRectangle.getPerimeter());}}class MyRectangle {// Data membersdouble width = 1, height = 1;// Constructorpublic MyRectangle() {}// Constructorpublic MyRectangle(double newWidth, double newHeight) {width = newWidth;height = newHeight;}public double getArea() {return width * height;}public double getPerimeter() {return 2 * (width + height);}}9.2public class Exercise09_02 {public static void main(String[] args) {Stock stock = new Stock("SUNW", "Sun MicroSystems Inc."); stock.setPreviousClosingPrice(100);// Set current pricestock.setCurrentPrice(90);// Display stock infoSystem.out.println("Previous Closing Price: " +stock.getPreviousClosingPrice());System.out.println("Current Price: " +stock.getCurrentPrice());System.out.println("Price Change: " +stock.getChangePercent() * 100 + "%");}}class Stock {String symbol;String name;double previousClosingPrice;double currentPrice;public Stock() {}public Stock(String newSymbol, String newName) {symbol = newSymbol;name = newName;}public double getChangePercent() {return (currentPrice - previousClosingPrice) /previousClosingPrice;}public double getPreviousClosingPrice() {return previousClosingPrice;}public double getCurrentPrice() {return currentPrice;}public void setCurrentPrice(double newCurrentPrice) {currentPrice = newCurrentPrice;}public void setPreviousClosingPrice(double newPreviousClosingPrice) { previousClosingPrice = newPreviousClosingPrice;}}9.3public class Exercise09_03 {public static void main(String[] args) {Date date = new Date();int count = 1;long time = 10000;while (count <= 8) {date.setTime(time);System.out.println(date.toString());count++;time *= 10;}}}9.4public class Exercise09_04 {public static void main(String[] args) {Random random = new Random(1000);for (int i = 0; i < 50; i++)System.out.print(random.nextInt(100) + " ");}9.5public class Exercise09_05 {public static void main(String[] args) {GregorianCalendar calendar = new GregorianCalendar();System.out.println("Year is " + calendar.get(GregorianCalendar.YEAR)); System.out.println("Month is " + calendar.get(GregorianCalendar.MONTH)); System.out.println("Date is " + calendar.get(GregorianCalendar.DATE));calendar.setTimeInMillis(1234567898765L);System.out.println("Year is " + calendar.get(GregorianCalendar.YEAR)); System.out.println("Month is " + calendar.get(GregorianCalendar.MONTH)); System.out.println("Date is " + calendar.get(GregorianCalendar.DATE)); }}9.6public class Exercise09_06 {static String output = "";/** Main method */public static void main(String[] args) {Scanner input = new Scanner(System.in);// Prompt the user to enter yearSystem.out.print("Enter full year (i.e. 2001): ");int year = input.nextInt();// Prompt the user to enter monthSystem.out.print("Enter month in number between 1 and 12: ");int month = input.nextInt();// Print calendar for the month of the yearprintMonth(year, month);System.out.println(output);}/** Print the calendar for a month in a year */static void printMonth(int year, int month) {// Get start day of the week for the first date in the monthint startDay = getStartDay(year, month);// Get number of days in the monthint numOfDaysInMonth = getNumOfDaysInMonth(year, month);// Print headingsprintMonthTitle(year, month);// Print bodyprintMonthBody(startDay, numOfDaysInMonth);}/** Get the start day of the first day in a month */static int getStartDay(int year, int month) {// Get total number of days since 1/1/1800int startDay1800 = 3;long totalNumOfDays = getTotalNumOfDays(year, month);// Return the start dayreturn (int)((totalNumOfDays + startDay1800) % 7);}/** Get the total number of days since Jan 1, 1800 */static long getTotalNumOfDays(int year, int month) {long total = 0;// Get the total days from 1800 to year -1for (int i = 1800; i < year; i++)if (isLeapYear(i))total = total + 366;elsetotal = total + 365;// Add days from Jan to the month prior to the calendar month for (int i = 1; i < month; i++)total = total + getNumOfDaysInMonth(year, i);return total;}/** Get the number of days in a month */static int getNumOfDaysInMonth(int year, int month) {if (month == 1 || month==3 || month == 5 || month == 7 ||month == 8 || month == 10 || month == 12)return 31;if (month == 4 || month == 6 || month == 9 || month == 11)return 30;if (month == 2)if (isLeapYear(year))return 29;elsereturn 28;return 0; // If month is incorrect.}/** Determine if it is a leap year */static boolean isLeapYear(int year) {if ((year % 400 == 0) || ((year % 4 == 0) && (year % 100 != 0))) return true;return false;}/** Print month body */static void printMonthBody(int startDay, int numOfDaysInMonth) { // Pad space before the first day of the monthint i = 0;for (i = 0; i < startDay; i++)output += " ";for (i = 1; i <= numOfDaysInMonth; i++) {if (i < 10)output += " " + i;elseoutput += " " + i;if ((i + startDay) % 7 == 0)output += "\n";}output += "\n";}/** Print the month title, i.e. May, 1999 */static void printMonthTitle(int year, int month) {output += " " + getMonthName(month)+ ", " + year + "\n";output += "-----------------------------\n";output += " Sun Mon Tue Wed Thu Fri Sat\n";}/** Get the English name for the month */static String getMonthName(int month) {String monthName = null;switch (month) {case 1: monthName = "January"; break;case 2: monthName = "February"; break;case 3: monthName = "March"; break;case 4: monthName = "April"; break;case 5: monthName = "May"; break;case 6: monthName = "June"; break;case 7: monthName = "July"; break;case 8: monthName = "August"; break;case 9: monthName = "September"; break;case 10: monthName = "October"; break;case 11: monthName = "November"; break;case 12: monthName = "December";}return monthName;}}9.7public class Exercise09_07 {public static void main (String[] args) {Account account = new Account(1122, 20000);Account.setAnnualInterestRate(4.5);account.withdraw(2500);account.deposit(3000);System.out.println("Balance is " + account.getBalance()); System.out.println("Monthly interest is " +account.getMonthlyInterest());System.out.println("This account was created at " +account.getDateCreated());}}class Account {private int id;private double balance;private static double annualInterestRate;private java.util.Date dateCreated;public Account() {dateCreated = new java.util.Date();}public Account(int newId, double newBalance) {id = newId;balance = newBalance;dateCreated = new java.util.Date();}public int getId() {return this.id;}public double getBalance() {return balance;}public static double getAnnualInterestRate() {return annualInterestRate;}public void setId(int newId) {id = newId;}public void setBalance(double newBalance) {balance = newBalance;}public static void setAnnualInterestRate(double newAnnualInterestRate) { annualInterestRate = newAnnualInterestRate;}public double getMonthlyInterest() {return balance * (annualInterestRate / 1200);}public java.util.Date getDateCreated() { return dateCreated;}public void withdraw(double amount) {balance -= amount;}public void deposit(double amount) {balance += amount;}}9.8public class Exercise09_08 {public static void main(String[] args) { Fan1 fan1 = new Fan1();fan1.setSpeed(Fan1.FAST);fan1.setRadius(10);fan1.setColor("yellow");fan1.setOn(true);System.out.println(fan1.toString());Fan1 fan2 = new Fan1();fan2.setSpeed(Fan1.MEDIUM);fan2.setRadius(5);fan2.setColor("blue");fan2.setOn(false);System.out.println(fan2.toString()); }}class Fan1 {public static int SLOW = 1;public static int MEDIUM = 2;public static int FAST = 3;private int speed = SLOW;private boolean on = false;private double radius = 5;private String color = "white";public Fan1() {}public int getSpeed() {return speed;}public void setSpeed(int newSpeed) {speed = newSpeed;}public boolean isOn() {return on;}public void setOn(boolean trueOrFalse) {this.on = trueOrFalse;}public double getRadius() {return radius;}public void setRadius(double newRadius) { radius = newRadius;}public String getColor() {return color;}public void setColor(String newColor) {color = newColor;}@Overridepublic String toString() {return"speed " + speed + "\n"+ "color " + color + "\n"+ "radius " + radius + "\n"+ ((on) ? "fan is on" : " fan is off"); }}public class Exercise09_09 {public static void main(String[] args) {RegularPolygon polygon1 = new RegularPolygon();RegularPolygon polygon2 = new RegularPolygon(6, 4);RegularPolygon polygon3 = new RegularPolygon(10, 4, 5.6, 7.8);System.out.println("Polygon 1 perimeter: " +polygon1.getPerimeter());System.out.println("Polygon 1 area: " + polygon1.getArea());System.out.println("Polygon 2 perimeter: " +polygon2.getPerimeter());System.out.println("Polygon 2 area: " + polygon2.getArea());System.out.println("Polygon 3 perimeter: " +polygon3.getPerimeter());System.out.println("Polygon 3 area: " + polygon3.getArea());}}class RegularPolygon {private int n = 3;private double side = 1;private double x;private double y;public RegularPolygon() {}public RegularPolygon(int number, double newSide) {n = number;side = newSide;}public RegularPolygon(int number, double newSide, double newX, double newY) {n = number;side = newSide;x = newX;y = newY;}public int getN() {return n;}public void setN(int number) {n = number;}public double getSide() {return side;}public void setSide(double newSide) {side = newSide;}public double getX() {return x;}public void setX(double newX) {x = newX;}public double getY() {return y;}public void setY(double newY) {y = newY;}public double getPerimeter() {return n * side;}public double getArea() {return n * side * side / (Math.tan(Math.PI / n) * 4); }}9.10public class Exercise09_10 {public static void main(String[] args) {Scanner input = new Scanner(System.in);System.out.print("Enter a, b, c: ");double a = input.nextDouble();double b = input.nextDouble();double c = input.nextDouble();QuadraticEquation equation = new QuadraticEquation(a, b, c);double discriminant = equation.getDiscriminant();if (discriminant < 0) {System.out.println("The equation has no roots");}else if (discriminant == 0){System.out.println("The root is " + equation.getRoot1());}else// (discriminant >= 0){System.out.println("The roots are " + equation.getRoot1()+ " and " + equation.getRoot2());}}}class QuadraticEquation {private double a;private double b;private double c;public QuadraticEquation(double newA, double newB, double newC) {a = newA;b = newB;c = newC;}double getA() {return a;}double getB() {return b;}double getC() {return c;}double getDiscriminant() {return b * b - 4 * a * c;}double getRoot1() {if (getDiscriminant() < 0)return 0;else {return (-b + getDiscriminant()) / (2 * a);}}double getRoot2() {if (getDiscriminant() < 0)return 0;else {return (-b - getDiscriminant()) / (2 * a);}}}9.11public class Exercise09_11 {public static void main(String[] args) {Scanner input = new Scanner(System.in);System.out.print("Enter a, b, c, d, e, f: ");double a = input.nextDouble();double b = input.nextDouble();double c = input.nextDouble();double d = input.nextDouble();double e = input.nextDouble();double f = input.nextDouble();LinearEquation equation = new LinearEquation(a, b, c, d, e, f);if (equation.isSolvable()) {System.out.println("x is " +equation.getX() + " and y is " + equation.getY());}else {System.out.println("The equation has no solution");}}}class LinearEquation {private double a;private double b;private double c;private double d;private double e;private double f;public LinearEquation(double newA, double newB, double newC, double newD, double newE, double newF) {a = newA;b = newB;c = newC;d = newD;e = newE;f = newF;}double getA() {return a;}double getB() {return b;}double getC() {return c;}double getD() {return d;}double getE() {return e;}double getF() {return f;}boolean isSolvable() {return a * d - b * c != 0;}double getX() {double x = (e * d - b * f) / (a * d - b * c);return x;}double getY() {double y = (a * f - e * c) / (a * d - b * c);return y;}}9.12public class Exercise09_12 {public static void main(String[] args) {Scanner input = new Scanner(System.in);System.out.print("Enter the endpoints of the first line segment: ");double x1 = input.nextDouble();double y1 = input.nextDouble();double x2 = input.nextDouble();double y2 = input.nextDouble();System.out.print("Enter the endpoints of the second line segment: ");double x3 = input.nextDouble();double y3 = input.nextDouble();double x4 = input.nextDouble();double y4 = input.nextDouble();// Build a 2 by 2 linear equationdouble a = (y1 - y2);double b = (-x1 + x2);double c = (y3 - y4);double d = (-x3 + x4);double e = -y1 * (x1 - x2) + (y1 - y2) * x1;double f = -y3 * (x3 - x4) + (y3 - y4) * x3;LinearEquation equation = new LinearEquation(a, b, c, d, e, f);if (equation.isSolvable()) {System.out.println("The intersecting point is: (" +equation.getX() + ", " + equation.getY() + ")");}else {System.out.println("The two lines do not cross ");}}}9.13public class Exercise09_13 {public static void main(String[] args) {Scanner input = new Scanner(System.in);System.out.print("Enter the number of rows and columns of the array: ");int numberOfRows = input.nextInt();int numberOfColumns = input.nextInt();double[][] a = new double[numberOfRows][numberOfColumns];System.out.println("Enter the array: ");for (int i = 0; i < a.length; i++)for (int j = 0; j < a[i].length; j++)a[i][j] = input.nextDouble();Location location = locateLargest(a);System.out.println("The location of the largest element is " + location.maxValue + " at ("+ location.row + ", " + location.column + ")");}public static Location locateLargest(double[][] a) {Location location = new Location();location.maxValue = a[0][0];for (int i = 0; i < a.length; i++)for (int j = 0; j < a[i].length; j++) {if (location.maxValue < a[i][j]) {location.maxValue = a[i][j];location.row = i;location.column = j;}}return location;}}class Location {int row, column;double maxValue;}9.14public class Exercise09_14 {public static void main(String[] args) {int size = 100000;double[] list = new double[size];for (int i = 0; i < list.length; i++) {list[i] = Math.random() * list.length;}StopWatch stopWatch = new StopWatch();selectionSort(list);stopWatch.stop();System.out.println("The sort time is " + stopWatch.getElapsedTime()); }/** The method for sorting the numbers */public static void selectionSort(double[] list) {for (int i = 0; i < list.length - 1; i++) {// Find the minimum in the list[i..list.length-1]double currentMin = list[i];int currentMinIndex = i;for (int j = i + 1; j < list.length; j++) {if (currentMin > list[j]) {currentMin = list[j];currentMinIndex = j;}}// Swap list[i] with list[currentMinIndex] if necessary;if (currentMinIndex != i) {list[currentMinIndex] = list[i];list[i] = currentMin;}}}}class StopWatch {private long startTime = System.currentTimeMillis(); private long endTime = startTime;public StopWatch() {}public void start() {startTime = System.currentTimeMillis();}public void stop() {endTime = System.currentTimeMillis();}public long getElapsedTime() {return endTime - startTime;}}。
《Java基础入门》_课后习题答案大一计算机专业9
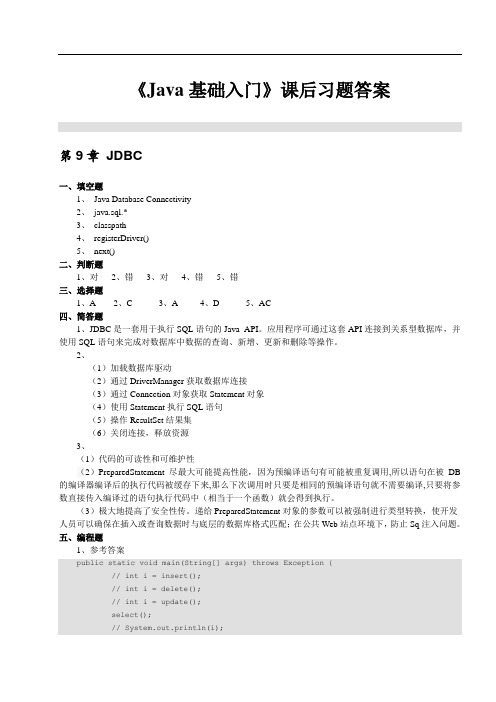
《Java基础入门》课后习题答案第9章JDBC一、填空题1、Java Database Connectivity2、java.sql.*3、classpath4、registerDriver()5、next()二、判断题1、对2、错3、对4、错5、错三、选择题1、A2、C3、A4、D5、AC四、简答题1、JDBC是一套用于执行SQL语句的Java API。
应用程序可通过这套API连接到关系型数据库,并使用SQL语句来完成对数据库中数据的查询、新增、更新和删除等操作。
2、(1)加载数据库驱动(2)通过DriverManager获取数据库连接(3)通过Connection对象获取Statement对象(4)使用Statement执行SQL语句(5)操作ResultSet结果集(6)关闭连接,释放资源3、(1)代码的可读性和可维护性(2)PreparedStatement尽最大可能提高性能,因为预编译语句有可能被重复调用,所以语句在被DB 的编译器编译后的执行代码被缓存下来,那么下次调用时只要是相同的预编译语句就不需要编译,只要将参数直接传入编译过的语句执行代码中(相当于一个函数)就会得到执行。
(3)极大地提高了安全性传。
递给PreparedStatement对象的参数可以被强制进行类型转换,使开发人员可以确保在插入或查询数据时与底层的数据库格式匹配;在公共Web站点环境下,防止Sq注入问题。
五、编程题1、参考答案public static void main(String[] args) throws Exception {// int i = insert();// int i = delete();// int i = update();select();// System.out.println(i);}// 获取连接对象private static Connection getConn() {String driver = "com.mysql.jdbc.Driver";String url = "jdbc:mysql://localhost:3306/javatest";String username = "root";String password = "1234";Connection conn = null;try {Class.forName(driver); // classLoader,加载对应驱动conn = DriverManager.getConnection(url, username, password);} catch (ClassNotFoundException e) {e.printStackTrace();} catch (SQLException e) {e.printStackTrace();}return conn;}// 插入操作private static int insert() {Connection conn = getConn();int i = 0;String sql = "insert into tb_user(name,sex,email,birthday) values(?,?,?,?)";PreparedStatement pstmt;try {pstmt = conn.prepareStatement(sql);pstmt.setString(1, "itcast");pstmt.setString(2, "男");pstmt.setString(3, "itcast@");pstmt.setString(4, "2000-01-01");i = pstmt.executeUpdate();pstmt.close();conn.close();} catch (SQLException e) {e.printStackTrace();}return i;}// 删除操作private static int delete() {Connection conn = getConn();int i = 0;String sql = "delete from tb_user where name=?";PreparedStatement pstmt;try {pstmt = conn.prepareStatement(sql);pstmt.setString(1, "itcast");i = pstmt.executeUpdate();} catch (Exception e) {e.printStackTrace();}return i;}// 更新操作private static int update() {Connection conn = getConn();int i = 0;String sql = "update tb_user set name=? where name =?";PreparedStatement pstmt;try {pstmt = conn.prepareStatement(sql);pstmt.setString(1, "itcast");pstmt.setString(2, "abc");i = pstmt.executeUpdate();System.out.println("resutl: " + i);pstmt.close();conn.close();} catch (SQLException e) {e.printStackTrace();}return i;}// 查询操作private static void select() {Connection conn = getConn();String sql = "select * from tb_user";PreparedStatement pstmt;try {pstmt = conn.prepareStatement(sql);ResultSet rs = pstmt.executeQuery();while (rs.next()) {int id = rs.getInt("id"); // 通过列名获取指定字段的值String name = rs.getString("name");String sex = rs.getString("sex");String email = rs.getString("email");Date birthday = rs.getDate("birthday");System.out.println(id + " | " + name + " | " + sex + " | " + email + " | " + birthday);}pstmt.close();conn.close();} catch (Exception e) {e.printStackTrace();}}2、参考答案import java.sql.*;public class Test {public static void main(String[] args) throws Exception {transferAccounts(2, 1, 100);}public static void transferAccounts(int fromid,int toid,int transferMoney) throwsException{ Class.forName("com.mysql.jdbc.Driver");String url = "jdbc:mysql://localhost:3306/jdbc";Connection con = DriverManager.getConnection(url,"root", "root");int fromMoney = getMoney(fromid);// 转出账户String sql = "update tb_count set money=? where id =?";PreparedStatement ps = con.prepareStatement(sql);ps.setInt(1, fromMoney-transferMoney);ps.setInt(2, fromid);// 转入账户int toMoney = getMoney(toid);String sql2 = "update tb_count set money=? where id =?";PreparedStatement ps2 = con.prepareStatement(sql2);ps2.setInt(1, toMoney+transferMoney);ps2.setInt(2, toid);ps.executeUpdate();ps2.executeUpdate();ps.close();ps2.close();con.close();}// 获取当前账户id的账户余额public static int getMoney(int id) throws Exception{Class.forName("com.mysql.jdbc.Driver");String url = "jdbc:mysql://localhost:3306/jdbc";Connection con = DriverManager.getConnection(url,"root", "root");String sql = "select money from tb_count where id =?";PreparedStatement ps = con.prepareStatement(sql);ps.setInt(1, id);ResultSet resultSet = ps.executeQuery();int money = 0;while(resultSet.next()){money = resultSet.getInt(1);}ps.close();con.close();return money;}}。
Java语言程序设计(郑莉)第九章课后习题答案

1.编写一个程序,该程序绘制一个//NetWork 类Java语言程序设计第九章课后习题答案5X 9的网络,使用drawLineimport java.awt.Graphics;import javax.swing.JFrame;public class NetWork extends JFrame{public NetWork(){// 设置窗体大小this .setSize(130, 130);// 设置窗体大小不可改变this .setResizable( false );// 设置默认关闭方式,关闭窗体的同时结束程序this .setDefaultCloseOperation(JFrame. EXIT// 将窗体显示出来this .setVisible( true );}// 横纵格之间都间隔10 像素,起点在( 20,40 ) public voidpaint(Graphics g){// 绘制横向线for ( int i=0;i<=5;i++){g.drawLine(20, 40+i*10, 110, 40+i*10);}// 绘制纵向线for ( int i=0;i<=9;i++){g.drawLine(20+i*10, 40, 20+i*10, 90);}}}//test9_1 类public class test9_1 {public static void main(String[] args){new NetWork();}}运行结果:方法。
ON_CLOSE );2•编写一个程序,该程序以不同的颜色随机产生三角形, //Triangle 类import java.awt.Color;import java.awt.Graphics;import java.util.Ra ndom;import javax.swi ng.JFrame;public class Tria ngle exte nds JFrame{Ran dom rnd = new Ran dom();//这里定义4个三角形int [][] x = new int [4][3];int [][] y = new int [4][3];int [][] color =new int [4][3];public Tria ngle(){//窗体标题this .setTitle( "随机三角形“);//窗体大小this .setSize(500,500);//窗体大小不可变this .setResizable( false );//关闭窗体的同时结束程序this .setDefaultCloseOperati on( JFrame.//显示窗体每个三角形用不同的颜色进行填充。
Java语言程序设计(基础篇)第10版习题答案Chapter9-1
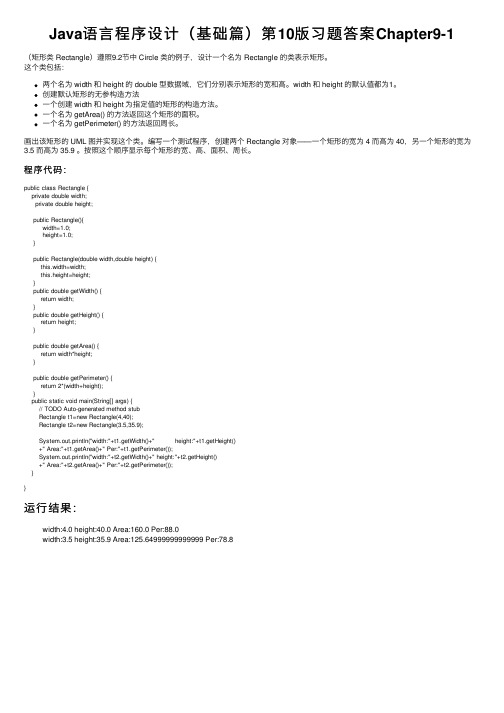
Java语⾔程序设计(基础篇)第10版习题答案Chapter9-1(矩形类 Rectangle)遵照9.2节中 Circle 类的例⼦,设计⼀个名为 Rectangle 的类表⽰矩形。
这个类包括:两个名为 width 和 height 的 double 型数据域,它们分别表⽰矩形的宽和⾼。
width 和 height 的默认值都为1。
创建默认矩形的⽆参构造⽅法⼀个创建 width 和 height 为指定值的矩形的构造⽅法。
⼀个名为 getArea() 的⽅法返回这个矩形的⾯积。
⼀个名为 getPerimeter() 的⽅法返回周长。
画出该矩形的 UML 图并实现这个类。
编写⼀个测试程序,创建两个 Rectangle 对象——⼀个矩形的宽为 4 ⽽⾼为 40,另⼀个矩形的宽为3.5 ⽽⾼为 35.9 。
按照这个顺序显⽰每个矩形的宽、⾼、⾯积、周长。
程序代码:public class Rectangle {private double width;private double height;public Rectangle(){width=1.0;height=1.0;}public Rectangle(double width,double height) {this.width=width;this.height=height;}public double getWidth() {return width;}public double getHeight() {return height;}public double getArea() {return width*height;}public double getPerimeter() {return 2*(width+height);}public static void main(String[] args) {// TODO Auto-generated method stubRectangle t1=new Rectangle(4,40);Rectangle t2=new Rectangle(3.5,35.9);System.out.println("width:"+t1.getWidth()+" height:"+t1.getHeight()+" Area:"+t1.getArea()+" Per:"+t1.getPerimeter());System.out.println("width:"+t2.getWidth()+" height:"+t2.getHeight()+" Area:"+t2.getArea()+" Per:"+t2.getPerimeter());}}运⾏结果:width:4.0 height:40.0 Area:160.0 Per:88.0width:3.5 height:35.9 Area:125.64999999999999 Per:78.8。
《Java基础入门》课后题答案

《Java基础入门》习题答案第1章Java开发入门一、填空题1、Java EE、Java SE、Java ME2、JRE3、javac4、bin5、path、classp ath二、选择题1、ABCD2、C3、D4、B5、B三、简答题1、面向对象、跨平台性、健壮性、安全性、可移植性、多线程性、动态性等。
2、JRE(Java Runtim e Enviro nment,Java运行时环境),它相当于操作系统部分,提供了Jav a程序运行时所需要的基本条件和许多Jav a基础类,例如,IO类、GUI控件类、网络类等。
JRE是提供给普通用户使用的,如果你只想运行别人开发好的Jav a程序,那么,你的计算机上必须且只需安装JRE。
JDK(Java Develo pment Kit,Java开发工具包),它包含编译工具、解释工具、文档制作工具、打包工具多种与开发相关的工具,是提供给Ja va开发人员使用的。
初学者学习和使用Jav a语言时,首先必须下载和安装JD K。
JDK中已经包含了JR E部分,初学者安装J DK后不必再去下载和安装JRE了。
四、编程题public classHelloW orld{public static void main(String[] args) {System.out.println("这是第一个J ava程序!");}}第2章Java编程基础一、填空题1、class2、true和false3、单行注释、多行注释、文档注释4、基本数据类型、引用数据类型5、1、2、4、86、& && | ||7、08、59、3410、56二、判断题1、错2、对3、错4、对5、错三、选择题1、AD2、AD3、C4、ABCD5、C 6 、A 7、AC 8、A 9、B 10、A四、程序分析题1、编译不通过。
《java2实用教程》(第四版)课后答案 第九章-第十三章

Java作业4(第九章---第十三章)第九章3.1.1程序代码import java.awt.*;import javax.swing.event.*;import javax.swing.*;import java.awt.event.*;public class kehou31 {public static void main(String args[]) {Computer fr=new Computer();}}class Computer extends JFrame implements DocumentListener { JTextArea text1,text2;int count=1;double sum=0,aver=0;Computer() {setLayout(new FlowLayout());text1=new JTextArea(6,20);text2=new JTextArea(6,20);add(new JScrollPane(text1));add(new JScrollPane(text2));text2.setEditable(false);(text1.getDocument()).addDocumentListener(this);setSize(300,320);setVisible(true);validate();setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);}public void changedUpdate(DocumentEvent e) {String s=text1.getText();String []a =s.split("[^0123456789.]+");sum=0;aver=0;for(int i=0;i<a.length;i++) {try { sum=sum+Double.parseDouble(a[i]);}catch(Exception ee) {}}count=a.length;aver=sum/count;text2.setText(null);text2.append("\n和:"+sum);text2.append("\n平均值:"+aver);}public void removeUpdate(DocumentEvent e){changedUpdate(e); }public void insertUpdate(DocumentEvent e){changedUpdate(e);}}3.1.2 运行界面3.2.1程序代码import java.awt.*;import javax.swing.event.*;import javax.swing.*;import java.awt.event.*;public class kehou932 {public static void main(String args[]) {ComputerFrame fr=new ComputerFrame();fr.setBounds(100,100,650,120);fr.setTitle("多功能计算器");fr.setBackground(Color.blue);}}class ComputerFrame extends JFrame implements ActionListener {JTextField text1,text2,text3;JButton buttonAdd,buttonSub,buttonMul,buttonDiv;JLabel label;public ComputerFrame() {setLayout(new FlowLayout());text1=new JTextField(10);text2=new JTextField(10);text3=new JTextField(10);label=new JLabel(" ",JLabel.CENTER);label.setBackground(Color.green);add(text1);add(label);add(text2);add(text3);buttonAdd=new JButton("加");buttonSub=new JButton("减");buttonMul=new JButton("乘");buttonDiv=new JButton("除");add(buttonAdd);add(buttonSub);add(buttonMul);add(buttonDiv);buttonAdd.addActionListener(this);buttonSub.addActionListener(this);buttonMul.addActionListener(this);buttonDiv.addActionListener(this);setSize(300,320);setVisible(true);validate();setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); }public void actionPerformed(ActionEvent e) {double n;if(e.getSource()==buttonAdd) {double n1,n2;try{ n1=Double.parseDouble(text1.getText()); n2=Double.parseDouble(text2.getText()); n=n1+n2;text3.setText(String.valueOf(n));label.setText("+");}catch(NumberFormatException ee){ text3.setText("请输入数字字符");}}else if(e.getSource()==buttonSub) {double n1,n2;try{ n1=Double.parseDouble(text1.getText()); n2=Double.parseDouble(text2.getText()); n=n1-n2;text3.setText(String.valueOf(n));label.setText("-");}catch(NumberFormatException ee){ text3.setText("请输入数字字符");}}else if(e.getSource()==buttonMul){double n1,n2;try{ n1=Double.parseDouble(text1.getText()); n2=Double.parseDouble(text2.getText()); n=n1*n2;text3.setText(String.valueOf(n));label.setText("*");}catch(NumberFormatException ee){ text3.setText("请输入数字字符");}}else if(e.getSource()==buttonDiv){double n1,n2;try{ n1=Double.parseDouble(text1.getText()); n2=Double.parseDouble(text2.getText()); n=n1/n2;text3.setText(String.valueOf(n));label.setText("/");}catch(NumberFormatException ee){ text3.setText("请输入数字字符");}}validate();}}3.2.2运行界面3.3.1 程序代码import java.awt.*;import java.awt.event.*;import javax.swing.*;public class kehou933 {public static void main(String args[]){Window win = new Window();win.setTitle("使用MVC结构");win.setBounds(100,100,420,260);}}class Window extends JFrame implements ActionListener { Lader lader; //模型JTextField textAbove,textBottom,textHeight; //视图 JTextArea showArea; //视图JButton controlButton; //控制器Window() {init();setVisible(true);setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);}void init() {lader = new Lader();textAbove = new JTextField(5);textBottom = new JTextField(5);textHeight = new JTextField(5);showArea = new JTextArea();controlButton=new JButton("计算面积");JPanel pNorth=new JPanel();pNorth.add(new JLabel("上底:"));pNorth.add(textAbove);pNorth.add(new JLabel("下底:"));pNorth.add(textBottom);pNorth.add(new JLabel("高:"));pNorth.add(textHeight);pNorth.add(controlButton);controlButton.addActionListener(this);add(pNorth,BorderLayout.NORTH);add(new JScrollPane(showArea),BorderLayout.CENTER);}public void actionPerformed(ActionEvent e) {try{double above = Double.parseDouble(textAbove.getText().trim());double bottom = Double.parseDouble(textBottom.getText().trim());double height = Double.parseDouble(textHeight.getText().trim());lader.setAbove(above) ;lader.setBottom(bottom);lader.setHeight(height);double area = lader.getArea();showArea.append(" 梯形的面积:"+area+"\n");}catch(Exception ex) {showArea.append("\n"+ex+"\n");}}}class Lader {double above,bottom,height;public double getArea() {double area = (above+bottom)*height/2.0;return area;}public void setAbove(double a) {above = a;}public void setBottom(double b) {bottom = b;}public void setHeight(double c) {height = c; }}3.3.2运行界面第十章4.1.1程序代码import java.io.*;public class E {public static void main(String args[]) {File f=new File("E.java");;try{ RandomAccessFile random=new RandomAccessFile(f,"rw");random.seek(0);long m=random.length();while(m>=0) {m=m-1;random.seek(m);int c=random.readByte();if(c<=255&&c>=0)System.out.print((char)c);else {m=m-1;random.seek(m);byte cc[]=new byte[2];random.readFully(cc);System.out.print(new String(cc));} }}catch(Exception exp){} }}4.1.2运行界面4.2.1程序代码import java.io.*;public class E {public static void main(String args[ ]) {File file=new File("E.java");File tempFile=new File("temp.txt");try{ FileReader inOne=new FileReader(file);BufferedReader inTwo= new BufferedReader(inOne);FileWriter tofile=new FileWriter(tempFile);BufferedWriter out= new BufferedWriter(tofile);String s=null;int i=0;s=inTwo.readLine();while(s!=null) {i++;out.write(i+" "+s);out.newLine();s=inTwo.readLine();}inOne.close();inTwo.close();out.flush();out.close();tofile.close();}catch(IOException e){}}}4.3.1程序代码import java.io.*;import java.util.*;public class kehou1043 {public static void main(String args[]) {File file = new File("a.txt");Scanner sc = null;double sum=0;int count = 0;try { sc = new Scanner(file);eDelimiter("[^0123456789.]+");while(sc.hasNext()){try{ double price = sc.nextDouble(); count++;sum = sum+price;System.out.println(price);}catch(InputMismatchException exp){String t = sc.next();}}System.out.println("平均价格:"+sum/count); }catch(Exception exp){System.out.println(exp);}}}4.3.2运行界面第十一章2.1.1程序代码import java.sql.*;import java.util.*;public class kehou1121 {public static void main(String args[]) {Query query=new Query();String dataSource="myData";String tableName="goods";Scanner read=new Scanner(System.in);System.out.print("输入数据源名:");dataSource = read.nextLine();System.out.print("输入表名:");tableName = read.nextLine();query.setDatasourceName(dataSource);query.setTableName(tableName);query.setSQL("SELECT * FROM "+tableName);query.inputQueryResult();}}class Query {String datasourceName=""; //数据源名String tableName=""; //表名String SQL; //SQL语句public Query() {try{ Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); }catch(ClassNotFoundException e) {System.out.print(e);}}public void setDatasourceName(String s) {datasourceName = s.trim();}public void setTableName(String s) {tableName = s.trim();}public void setSQL(String SQL) {this.SQL = SQL.trim();}public void inputQueryResult() {Connection con;Statement sql;ResultSet rs;try {String uri = "jdbc:odbc:"+datasourceName;String id = "";String password = "";con = DriverManager.getConnection(uri,id,password);DatabaseMetaData metadata = con.getMetaData();ResultSet rs1 = metadata.getColumns(null,null,tableName,null);int字段个数 = 0;while(rs1.next()) {字段个数++;}sql = con.createStatement();//创建SQL语句rs = sql.executeQuery(SQL);//执行SQL语句while(rs.next()) {for(int k=1;k<=字段个数;k++) {System.out.print(" "+rs.getString(k)+" ");}System.out.println("");}con.close();}catch(SQLException e) {System.out.println("请输入正确的表名"+e);}}}2.1.2运行界面2.2.1程序代码import java.sql.*;import java.util.*;public class kehou1122 {public static void main(String args[]) {Q query = new Q();String dataSource = "myData";String tableName = "goods";query.setDatasourceName(dataSource);query.setTableName(tableName);String name = "";Scanner read=new Scanner(System.in);System.out.print("商品名:");name = read.nextLine();String str="'%["+name+"]%'";String SQL = "SELECT * FROM "+tableName+" WHERE name LIKE "+str; query.setSQL(SQL);System.out.println(tableName+"表中商品名是"+name+"的记录");query.inputQueryResult();}}class Q {String datasourceName=""; //数据源名String tableName=""; //表名String SQL; //SQL语句public Q() {try{ Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");}catch(ClassNotFoundException e) {System.out.print(e);}}public void setDatasourceName(String s) {datasourceName = s.trim();}public void setTableName(String s) {tableName = s.trim();}public void setSQL(String SQL) {this.SQL = SQL.trim();}public void inputQueryResult() {Connection con;Statement sql;ResultSet rs;try {String uri = "jdbc:odbc:"+datasourceName;String id = "";String password = "";con = DriverManager.getConnection(uri,id,password);DatabaseMetaData metadata = con.getMetaData();ResultSet rs1 = metadata.getColumns(null,null,tableName,null);int字段个数 = 0;while(rs1.next()) {字段个数++;}sql = con.createStatement();rs = sql.executeQuery(SQL);while(rs.next()) {for(int k=1;k<=字段个数;k++) {System.out.print(" "+rs.getString(k)+" ");}System.out.println("");}con.close();}catch(SQLException e) {System.out.println("请输入正确的表名"+e);}}}2.2.2运行界面2.3.1程序代码import java.sql.*;import java.util.*;public class kehou1123 {public static void main(String args[]) {Q1 query = new Q1();String dataSource = "myData";String tableName = "goods";query.setDatasourceName(dataSource);query.setTableName(tableName);String SQL = "SELECT * FROM "+tableName+" ORDER BY madeTime";query.setSQL(SQL);System.out.println(tableName+"表记录按商品生产日期前后排序是: "); query.inputQueryResult();}}class Q1 {String datasourceName=""; //数据源名String tableName=""; //表名String SQL; //SQL语句public Q1() {try{ Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");}catch(ClassNotFoundException e) {System.out.print(e);}}public void setDatasourceName(String s) {datasourceName = s.trim();}public void setTableName(String s) {tableName = s.trim();}public void setSQL(String SQL) {this.SQL = SQL.trim();}public void inputQueryResult() {Connection con;Statement sql;ResultSet rs;try {String uri = "jdbc:odbc:"+datasourceName;String id = "";String password = "";con = DriverManager.getConnection(uri,id,password);DatabaseMetaData metadata = con.getMetaData();ResultSet rs1 = metadata.getColumns(null,null,tableName,null);int字段个数 = 0;while(rs1.next()) {字段个数++;}sql = con.createStatement();rs = sql.executeQuery(SQL);while(rs.next()) {for(int k=1;k<=字段个数;k++) {System.out.print(" "+rs.getString(k)+" ");}System.out.println("");}con.close();}catch(SQLException e) {System.out.println("请输入正确的表名"+e);}}}2.3.2运行界面第十二章4.1.1程序代码public class kehou1241{public static void main(String args[]) {Cinema a=new Cinema();a.zhang.start();a.sun.start();a.zhao.start();}}class TicketSeller //负责卖票的类。
Java语言程序设计(一)课后习题第九章(附答案)
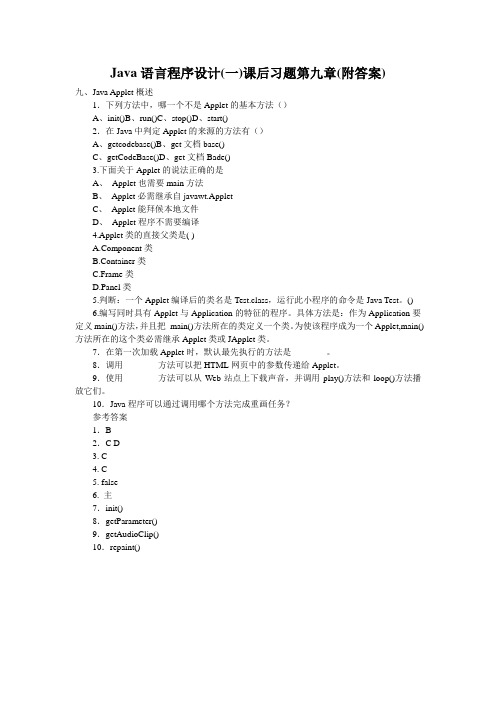
Java语言程序设计(一)课后习题第九章(附答案)九、Java Applet概述1.下列方法中,哪一个不是Applet的基本方法()A、init()B、run()C、stop()D、start()2.在Java中判定Applet的来源的方法有()A、getcodebase()B、get文档base()C、getCodeBase()D、get文档Bade()3.下面关于Applet的说法正确的是A、Applet也需要main方法B、Applet必需继承自javawt.AppletC、Applet能拜候本地文件D、Applet程序不需要编译4.Applet类的直接父类是( )ponent类B.Container类C.Frame类D.Panel类5.判断:一个Applet编译后的类名是Test.class,运行此小程序的命令是Java Test。
()6.编写同时具有Applet与Application的特征的程序。
具体方法是:作为Application要定义main()方法,并且把main()方法所在的类定义一个类。
为使该程序成为一个Applet,main()方法所在的这个类必需继承Applet类或JApplet类。
7.在第一次加载Applet时,默认最先执行的方法是________。
8.调用________方法可以把HTML网页中的参数传递给Applet。
9.使用________方法可以从Web站点上下载声音,并调用play()方法和loop()方法播放它们。
10.Java程序可以通过调用哪个方法完成重画任务?参考答案1.B2.C D3. C4. C5. false6. 主7.init()8.getParameter()9.getAudioClip()10.repaint()。
AnjoyoJava9章节考试题带答案

第九章考试题一、选择题:(每题3分,共60题)1.下列关于Java线程的说法那些是正确的(D)。
A、每一个Java线程可以看成由代码、一个真实的CPU以及数据三部份组成。
虚拟CPUB、创建线程的两种方法中,从Thread类中继承的创建方式可以防止出现多父类问题。
C、Thread类属于java.util程序包。
Lang包D、以上说法无一正确。
2.运行下列程序,会产生什么结果?public class X extends Thread implements Runable{public void run(){System.out.println("this is run()");}public static void main(String args[]){Thread t=new Thread(new X());t.start();}}(D)。
A、第一行会产生编译错误B、第六行会产生编译错误C、第六行会产生运行错误D、程序会运行和启动3.下面哪个方法不可以在任何时候被任何线程调用?(D)A、wait()B、sleep()C、yield()D、synchronized(this)4.下列关于线程优先级的说法中,正确的是(D)。
A、线程的优先级是不能改变的B、线程的优先级是在创建线程时设置的C、在创建线程后的任何时候都可以设置D、B和C5.线程生命周期中正确的状态是(C)。
A、新建状态、运行状态和终止状态B、新建状态、运行状态、阻塞状态和终止状态C、新建状态、可运行状态、运行状态、阻塞状态和终止状态D、新建状态、可运行状态、运行状态、恢复状态和终止状态6.Thread类中能运行线程体的方法是()。
A、start()B、resume()C、init()D、run()7.在线程同步中,为了唤醒另一个等待的线程,使用下列方法(C)。
A、sleep()B、wait()C、notify()notifyall()D、join()8.为了得到当前正在运行的线程,可使用下列哪个方法?(B)。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
第9章习题解答
1.与输入/输出有关的流类有哪些?
答:与输入/输出有关的流类主要有InputStream、OutputStream和Reader、Writer类及其子类。
除此以外,与流有关的类还有File类、FileDescriptor类、StreamTokenizer类和RandomAccessFile类。
2.字节流与字符流之间有哪些区别?
答:字节流是面向字节的流,流中的数据以8位字节为单位进行读写,是抽象类InputStream和OutputStream的子类,通常用于读写二进制数据,如图像和声音。
字符流是面向字符的流,流中的数据以16位字符(Unicode字符)为单位进行读写,是抽象类Reader和Writer的子类,通常用于字符数据的处理。
3.什么是节点流?什么是处理流或过滤流?分别在什么场合使用?
答:一个流有两个端点。
一个端点是程序;另一个端点可以是特定的外部设备(如键盘、显示器、已连接的网络等)和磁盘文件,甚至是一块内存区域(统称为节点),也可以是一个已存在流的目的端。
流的一端是程序,另一端是节点的流,称为节点流。
节点流是一种最基本的流。
以其它已经存在的流作为一个端点的流,称为处理流。
处理流又称过滤流,是对已存在的节点流或其它处理流的进一步处理。
对节点流中的数据只能按字节或字符读写。
当读写的数据不是单个字节或字符,而是一个数据块或字符串等时,就要使用处理流或过滤流。
4.标准流对象有哪些?它们是哪个类的对象?
答:标准流对象有3个,它们是:System.in、System.out和System.err。
System.in 是InputStream类对象,System.out和System.err是PrintStream类对象。
5.顺序读写与随机读写的特点分别是什么?
答:所谓顺序读写是指在读写流中的数据时只能按顺序进行。
换言之,在读取流中的第n个字节或字符时,必须已经读取了流中的前n-1个字节或字符;同样,在写入了流中n-1个字节或字符后,才能写入第n个字节或字符。
顺序读写的缺点是文件中数据的修改比较麻烦。
所谓随机读写就是直接从文件的某个指定位置(文件位置指针所确定)开始读或写,而不必读写文件位置指针之前的数据。
6.使用对象流读/写对象数据时,要求对象所属的类必须实现哪个接口?
答:使用对象流读/写对象数据时,要求对象所属的类必须实现java.io.Serializable 接口以启用其序列化功能。
7.如何判断一个文件是否已经存在?如何删除一个文件?如何重命名一个文件?可以使用File类来获取一个文件包含的字节数吗?
答:用File类的方法exists()判断一个文件是否已经存在;用File类的方法delete()删除一个文件;用File类的方法renameTo(File dest)重命名一个文件;用File类的方法length()获取一个文件包含的字节数。
8.阅读程序,写出程序的输出结果。
//管道流PipedInputStream和PipedOutputStream应用。
import java.io.*;
public class Xt8_8_PipeDemo {
public static void main(String args[])throws Exception{
PipedInputStream pis;
PipedOutputStream pos;
byte b;
pis=new PipedInputStream();
pos=new PipedOutputStream(pis);
pos.write('a');
pos.write('b');
b=(byte)pis.read();
System.out.println(b);
b=(byte)pis.read();
System.out.println(b);
}
}
答:程序的输出结果是:
97
98
9.编写一个程序,求2~200之间的素数,并将结果保存在文件prime.dat中。
再从该文件中读取内容并在屏幕上显示山来。
答:程序设计如下:
import java.io.*;
public class Xt9_9_PrimeInputOutputStream {
public static void main(String[] args) throws IOException{ int i=2,j;
DataOutputStream output=
new DataOutputStream(new FileOutputStream(".\\prime.dat"));
output.writeInt(i);
for(i=3;i<200;i+=2){
for(j=2;j<=i/2;j++)
if(i%j==0) break;
if(j>i/2)
output.writeInt(i);
}
output.close();
DataInputStream input=
new DataInputStream(new FileInputStream(".\\prime.dat"));
while(input.available()>0){
i=input.readInt();
System.out.print(i+" ");
}
input.close();
}
}
10.编写一个程序,比较两个文件的内容是否相同。
答:程序设计如下:
import java.io.*;
import java.util.Scanner;
public class Xt9_10_FileCompare {
public static void main(String[] args)throws IOException { String str1="",str2="";
Scanner scan=new Scanner(System.in);
System.out.print("输入要比较两个文件的文件名:");
str1=scan.next();
str2=scan.next();
if(FileCompare(str1,str2))
System.out.println("要比较的两个文件内容相同!");
else
System.out.println("要比较的两个文件内容不同!");
}
static boolean FileCompare(String filename1,String filename2)throws IOException{
boolean identical;
FileInputStream fis1,fis2;
fis1=new FileInputStream(filename1); //打开文件输入流1
fis2=new FileInputStream(filename2); //打开文件输入流2
while(fis1.available()>0&&fis2.available()>0)
if(fis1.read()!=fis2.read())
break;
if(fis1.available()>0||fis2.available()>0)
identical=false;
else
identical=true;
fis1.close(); //关闭文件输入流,即关闭文件
fis2.close(); //关闭文件输入流,即关闭文件
return identical;
}
}
11.编写程序从一个文件读前10行并在屏幕上显示出来。
如果文件少于10行,就显示所有行。
文件名由用户从键盘输入。
答:程序设计如下:
import java.io.*;
import java.util.Scanner;
public class Xt9_11_BuffereStream {
public static void main(String[] args) throws IOException{ String filename="",str;
Scanner scan=new Scanner(System.in);
System.out.print("输入文件名:");
filename=scan.next();
FileReader fin=new FileReader(filename);//打开文本文件读
BufferedReader bin=new BufferedReader(fin);//字符流转换为缓冲流
int count=0;
while((str=bin.readLine())!=null&&count<10){//从文件读一行字符System.out.println(str); //显示
count++;
}
bin.close();
}
}。