贪吃蛇游戏代码C
c语言贪吃蛇代码的算法模块

c语言贪吃蛇代码的算法模块以下是一个简单的 C 语言贪吃蛇代码的算法模块:1. 食物生成:在游戏区域的随机位置生成食物。
```cint x = rand() % SCREEN_WIDTH;int y = rand() % SCREEN_HEIGHT;food.x = x;food.y = y;```2. 蛇的移动:根据蛇的当前方向移动蛇头。
```cswitch (snake.direction) {case UP:snake.y--;break;case DOWN:snake.y++;break;case LEFT:snake.x--;break;case RIGHT:snake.x++;break;}```3. 检查游戏结束条件:检查蛇是否吃到自己或撞到边界。
```cif (snake.x == food.x && snake.y == food.y) {// 吃到食物,蛇身增长addNode(&snake);generateFood(&food);} else {if (snake.x < 0 || snake.x >= SCREEN_WIDTH || snake.y < 0 || snake.y >= SCREEN_HEIGHT) {// 撞墙,游戏结束gameOver = true;} else {removeNode(&snake);}}```4. 控制蛇的移动速度:根据游戏难度控制蛇的移动速度。
```cif (difficulty == EASY) {// 较慢的速度sleep(100);} else if (difficulty == NORMAL) {// 正常的速度sleep(75);} else {// 较快的速度sleep(50);}```以上是一个简单的贪吃蛇游戏算法的核心部分。
你可以根据实际需求对代码进行修改和扩展,以实现更完整的贪吃蛇游戏功能。
贪吃蛇游戏源代码(C++)

贪吃蛇游戏源代码(C++)#include <windows.h>#include <stdlib.h>#include <conio.h>#include <time.h>#include <cstring>#include <cstdio>#include <iostream>#define N 22using namespace std;int gameover;int x1, y1; // 随机出米int x,y;long start;//===================================== ==//类的实现与应用initialize//===================================== ==//下面定义贪吃蛇的坐标类class snake_position{public:int x,y; //x表示行,y表示列snake_position(){};void initialize(int &);//坐标初始化};snake_position position[(N-2)*(N-2)+1]; //定义贪吃蛇坐标类数组,有(N-2)*(N-2)个坐标void snake_position::initialize(int &j){x = 1;y = j;}//下面定义贪吃蛇的棋盘图class snake_map{private:char s[N][N];//定义贪吃蛇棋盘,包括墙壁。
int grade, length;int gamespeed; //前进时间间隔char direction; // 初始情况下,向右运动int head,tail;int score;bool gameauto;public:snake_map(int h=4,int t=1,int l=4,char d=77,int s=0):length(l),direction(d),head(h),tail(t),score(s){}void initialize(); //初始化函数void show_game();int updata_game();void setpoint();void getgrade();void display();};//定义初始化函数,将贪吃蛇的棋盘图进行初始化void snake_map::initialize(){int i,j;for(i=1;i<=3;i++)s[1][i] = '*';s[1][4] = '#';for(i=1;i<=N-2;i++)for(j=1;j<=N-2;j++)s[i][j]=' '; // 初始化贪吃蛇棋盘中间空白部分for(i=0;i<=N-1;i++)s[0][i] = s[N-1][i] = '-'; //初始化贪吃蛇棋盘上下墙壁for(i=1;i<=N-2;i++)s[i][0] = s[i][N-1] = '|'; //初始化贪吃蛇棋盘左右墙壁}//===================================== =======//输出贪吃蛇棋盘信息void snake_map::show_game(){system("cls"); // 清屏int i,j;cout << endl;for(i=0;i<N;i++){cout << '\t';for(j=0;j<N;j++)cout<<s[i][j]<<' '; // 输出贪吃蛇棋盘if(i==2) cout << "\t等级:" << grade;if(i==6) cout << "\t速度:" << gamespeed;if(i==10) cout << "\t得分:" << score << "分" ;if(i==14) cout << "\t暂停:按一下空格键" ;if(i==18) cout << "\t继续:按两下空格键" ;cout<<endl;}}//输入选择等级void snake_map::getgrade(){cin>>grade;while( grade>7 || grade<1 ){cout << "请输入数字1-7选择等级,输入其他数字无效" << endl;cin >> grade;}switch(grade){case 1: gamespeed = 1000;gameauto = 0;break;case 2: gamespeed = 800;gameauto = 0;break;case 3: gamespeed = 600;gameauto = 0;break;case 4: gamespeed = 400;gameauto = 0;break;case 5: gamespeed = 200;gameauto = 0;break;case 6: gamespeed = 100;gameauto = 0;break;case 7: grade = 1;gamespeed = 1000;gameauto = 1;break; }}//输出等级,得分情况以及称号void snake_map::display(){cout << "\n\t\t\t\t等级:" << grade;cout << "\n\n\n\t\t\t\t速度:" << gamespeed;cout << "\n\n\n\t\t\t\t得分:" << score << "分" ;}//随机产生米void snake_map::setpoint(){srand(time(0));do{x1 = rand() % (N-2) + 1;y1 = rand() % (N-2) + 1;}while(s[x1][y1]!=' ');s[x1][y1]='*';}char key;int snake_map::updata_game(){gameover = 1;key = direction;start = clock();while((gameover=(clock()-start<=gamespeed))&&!kbhit()); //如果有键按下或时间超过自动前进时间间隔则终止循环if(gameover){getch();key = getch();}if(key == ' '){while(getch()!=' '){};//这里实现的是按空格键暂停,按空格键继续的功能,但不知为何原因,需要按两下空格才能继续。
贪吃蛇源程序(c语言版的)

printf("This is a game of a SNAKE.\nGOOD LUCK TO YOU !\n");
printf("Input your game speed,please.(e.g.300)\n");
scanf("%d",&gamespeed);
qipan[i][0]='|';
for(i=0;i<20;i++)
qipan[i][79]='|';
for(i=0;i<80;i++)
qipan[19][i]='_';
qipan[1][1]=qipan[1][2]=qipan[1][3]='*';//初始化蛇的位置
if(direction==80)
x=zuobiao[0][head]+1;y=zuobiao[1][head];
if(direction==75)
x=zuobiao[0][head];y=zuobiao[0][head]-1;
if(direction==77)
x=zuobiao[0][head];y=zuobiao[1][head]+1;
while(!kbhit()&&(timeover=clock()-start<=gamespeed));
if(timeover)
{
getch();
direction=getch();
}
else
direction=direction;
C语言小游戏源代码《贪吃蛇》

void init(void){/*构建图形驱动函数*/ int gd=DETECT,gm; initgraph(&gd,&gm,""); cleardevice(); }
欢迎您阅读该资料希望该资料能给您的学习和生活带来帮助如果您还了解更多的相关知识也欢迎您分享出来让我们大家能共同进步共同成长
C 语言小游戏源代码《贪吃பைடு நூலகம்》
#define N 200/*定义全局常量*/ #define m 25 #include <graphics.h> #include <math.h> #include <stdlib.h> #include <dos.h> #define LEFT 0x4b00 #define RIGHT 0x4d00 #define DOWN 0x5000 #define UP 0x4800 #define Esc 0x011b int i,j,key,k; struct Food/*构造食物结构体*/ { int x; int y; int yes; }food; struct Goods/*构造宝贝结构体*/ { int x; int y; int yes; }goods; struct Block/*构造障碍物结构体*/ { int x[m]; int y[m]; int yes; }block; struct Snake{/*构造蛇结构体*/ int x[N]; int y[N]; int node; int direction; int life; }snake; struct Game/*构建游戏级别参数体*/ { int score; int level; int speed;
贪吃蛇代码-c语言-vc6.0

#include <conio.h>
#define N 21
int apple[3];
char score[3];
char tail[3];
void gotoxy(int x, int y) //输出坐标
{
COORD pos;
pos.X = x;
pos.Y = y;
/*这是一个贪吃蛇代码,运行环境VC++6.0(亲测完美运行)*/
/*该程序在dos系统下运行,不需要graphics.h头文件*/
/*该程序由C语言小方贡献,谢谢您的支持*/
#include <windows.h>
#include <stdlib.h>
#include <time.h>
#include <stdio.h>
apple[0] = rand()%N + 1;
apple[1] = rand()%N + 1;
apple[2] = 1;
}
Sleep(200-score[3]*10);
setbuf(stdin, NULL);
if (kbhit())
{
gotoxy(0, N+2);
ch = getche();
}
snake = Move(snake, ch, &len);
}
int File_in() //取记录的分数
{
FILE *fp;
if((fp = fopen("C:\\tcs.txt","a+")) == NULL)
贪吃蛇 C语言代码

}
snake[x][y]=FOOD;
draw(snake);
/*---------------------------------------*/
/*--------控制的部分---------------------*/
while(judgeGO(snake))
}
if(x>0&&x<16&&y>0&&y<16)
{
if(a==0)
printf("█");
else printf("□");
return ;
}
}
void draw(int (*sna)[17])
draw(snake);
Sleep(100);
continue;
}
rightmove(snake);
draw(snake);
}
int randno()
{
srand(time(NULL)); //运用随机函数,取随机数,出现食物用
return rand()%15+1;
}
//判断游戏是否结束
bool judgeGO(int (*sna)[17])
{
int x,y,i=0,max=0,count=0;
while(!kbhit()&&key1!=77&&judgeGO(snake))
{
if(judgeF(snake,key))
{
draw(snake);
Sleep(100);
贪吃蛇游戏代码(C语言编写)
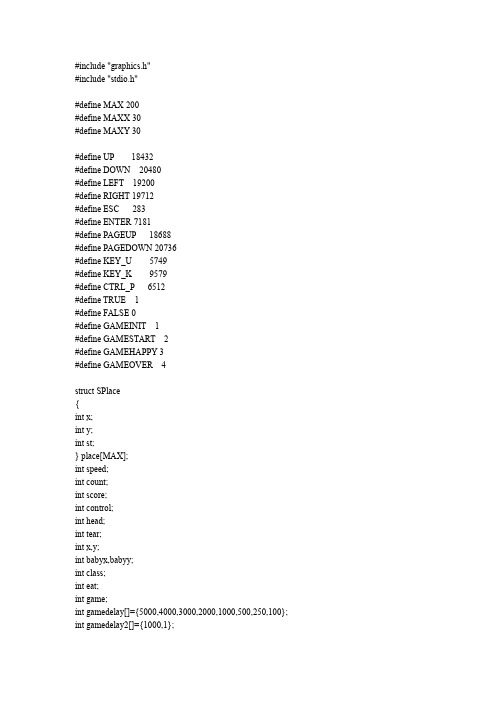
cleardevice(); if (!pause)
nextstatus(); else {
settextstyle(1,0,4); setcolor(12); outtextxy(250,100,"PAUSE"); } draw();
if(game==GAMEOVER) { settextstyle(0,0,6); setcolor(8); outtextxy(101,101,"GAME OVER"); setcolor(15); outtextxy(99,99,"GAME OVER"); setcolor(12); outtextxy(100,100,"GAME OVER"); sprintf(temp,"Last Count: %d",count); settextstyle(0,0,2); outtextxy(200,200,temp); }
place[tear].st = FALSE; tear ++; if (tear >= MAX) tear = 0; } else { eat = FALSE; exit = TRUE; while(exit) { babyx = rand()%MAXX; babyy = rand()%MAXY; exit = FALSE; for( i = 0; i< MAX; i++ ) if( (place[i].st)&&( place[i].x == babyx) && (place[i].y == babyy))
struct SPlace { int x; int y; int st; } place[MAX]; int speed; int count; int score; int control; int head; int tear; int x,y; int babyx,babyy; int class; int eat; int game; int gamedelay[]={5000,4000,3000,2000,1000,500,250,100}; int gamedelay2[]={1000,1};
贪吃蛇c语言代码

贪吃蛇c语言代码#include <graphics.h>#include <conio.h>#include <stdlib.h>#include <dos.h>#define NULL 0#define UP 18432#define DOWN 20480#define LEFT 19200#define RIGHT 19712#define ESC 283#define ENTER 7181struct snake{int centerx;int centery;int newx;int newy;struct snake *next;};struct snake *head;int grade=60; /*控制速度的*******/int a,b; /* 背静遮的位置*/void *far1,*far2,*far3,*far4; /* 蛇身指针背静遮的指针虫子*/ int size1,size2,size3,size4; /* **全局变量**/int ch=RIGHT; /**************存按键开始蛇的方向为RIGHT***********/int chy=RIGHT;int flag=0; /*********判断是否退出游戏**************/int control=4; /***********判断上次方向和下次方向不冲突***/int nextshow=1; /*******控制下次蛇身是否显示***************/int scenterx; /***************随即矩形中心坐标***************/int scentery;int sx; /*******在a b 未改变前得到他们的值保证随机矩形也不在此出现*******/int sy;/************************蛇身初始化**************************/void snakede(){struct snake *p1,*p2;head=p1=p2=(struct snake *)malloc(sizeof(struct snake));p1->centerx=80;p1->newx=80;p1->centery=58;p1->newy=58;p1=(struct snake *)malloc(sizeof(struct snake));p2->next=p1;p1->centerx=58;p1->newx=58;p1->centery=58;p1->newy=58;p1->next=NULL;}/*******************end*******************/void welcome() /*************游戏开始界面,可以选择速度**********/ {int key;int size;int x=240;int y=300;int f;void *buf;setfillstyle(SOLID_FILL,BLUE);bar(98,100,112,125);setfillstyle(SOLID_FILL,RED);bar(98,112,112,114);setfillstyle(SOLID_FILL,GREEN);bar(100,100,110,125);size=imagesize(98,100,112,125);buf=malloc(size);getimage(98,100,112,125,buf);cleardevice();setfillstyle(SOLID_FILL,BLUE);bar(240,300,390,325);outtextxy(193,310,"speed:");setfillstyle(SOLID_FILL,RED);bar(240,312,390,314);setcolor(YELLOW);outtextxy(240,330,"DOWN");outtextxy(390,330,"UP");outtextxy(240,360,"ENTER to start..." );outtextxy(270,200,"SNAKE");fei(220,220);feiyang(280,220);yang(340,220);putimage(x,y,buf,COPY_PUT);setcolor(RED);rectangle(170,190,410,410);while(1){ if(bioskey(1)) /********8选择速度部分************/ key=bioskey(0);switch(key){case ENTER:f=1;break;case DOWN:if(x>=240){ putimage(x-=2,y,buf,COPY_PUT);grade++;key=0;break;}case UP:if(x<=375){ putimage(x+=2,y,buf,COPY_PUT);grade--;key=0;break;}}if (f==1)break;} /********** end ****************/free(buf);}/*************************随即矩形*****************//***********当nextshow 为1的时候才调用此函数**********/void ran(){ int nx;int ny;int show; /**********控制是否显示***********/int jump=0;struct snake *p;p=head;if(nextshow==1) /***********是否开始随机产生***************/while(1){show=1;randomize();nx=random(14);ny=random(14);scenterx=nx*22+58;scentery=ny*22+58;while(p!=NULL){if(scenterx==p->centerx&&scentery==p->centery||scenterx==sx&&scentery==sy) {show=0;jump=1;break;}elsep=p->next;if(jump==1)break;}if(show==1){putimage(scenterx-11,scentery-11,far3,COPY_PUT); nextshow=0;break;}}}/***********过关动画**************/void donghua(){ int i;cleardevice();setbkcolor(BLACK);randomize();while(1){for(i=0;i<=5;i++){putpixel(random(640),random(80),13);putpixel(random(640),random(80)+80,2); putpixel(random(640),random(80)+160,3); putpixel(random(640),random(80)+240,4); putpixel(random(640),random(80)+320,1); putpixel(random(640),random(80)+400,14);}setcolor(YELLOW);settextstyle(0,0,4);outtextxy(130,200,"Wonderful!!");setfillstyle(SOLID_FILL,10);bar(240,398,375,420);feiyang(300,400);fei(250,400);yang(350,400);if(bioskey(1))if(bioskey(0)==ESC){flag=1;break;}}}/*************************end************************//***********************初始化图形系统*********************/ void init(){int a=DETECT,b;int i,j;initgraph(&a,&b,"");}/***************************end****************************//***画立体边框效果函数******/void tline(int x1,int y1,int x2,int y2,int white,int black){ setcolor(white);line(x1,y1,x2,y1);line(x1,y1,x1,y2);setcolor(black);line(x2,y1,x2,y2);line(x1,y2,x2,y2);}/****end*********//*************标志**********/int feiyang(int x,int y){int feiyang[18][18]={ {0,0,0,0,0,0,1,1,1,1,1,1,0,1,1,0,0,0},{0,0,0,0,0,1,1,1,0,0,1,1,1,1,1,0,0,0},{0,0,0,0,1,1,1,0,0,0,0,0,1,1,1,0,0,0},{0,0,0,1,1,1,0,0,0,0,0,0,0,1,1,0,0,0},{0,0,0,1,1,0,0,0,0,0,0,1,1,0,0,0,0,0},{0,0,1,1,0,0,0,0,0,0,1,1,1,0,0,0,0,0},{0,0,1,1,0,1,1,1,1,1,1,0,0,0,0,0,0,0},{0,0,1,1,1,1,1,0,0,1,0,0,1,1,0,0,0,0},{0,0,1,1,1,0,0,0,0,1,0,1,1,1,0,0,0,0},{0,0,1,1,0,1,1,1,0,1,1,1,0,0,0,0,0,0},{0,0,1,1,0,0,0,1,0,0,1,1,0,0,0,0,0,0},{0,0,1,1,0,0,0,1,1,0,0,1,1,0,0,1,0,0},{0,0,1,1,1,0,0,1,1,0,0,1,1,0,0,1,0,0},{0,0,1,1,1,1,0,1,1,1,1,1,1,0,1,1,0,0},{0,0,0,1,1,1,0,1,1,1,1,1,0,0,1,0,0,0},{0,0,0,0,1,1,1,0,0,0,0,0,0,1,1,0,0,0},{0,0,0,0,0,1,1,1,0,0,0,0,1,1,0,0,0,0},{0,0,0,0,0,1,1,1,1,1,1,1,1,0,0,0,0,0}};int i,j;for(i=0;i<=17;i++)for(j=0;j<=17;j++){if (feiyang[i][j]==1)putpixel(j+x,i+y,RED);}}/********"飞"字*************/int fei(int x,int y){int fei[18][18]={{1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, {0,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0},{0,1,1,1,0,0,0,0,1,1,1,0,0,0,0,0,0,0},{0,0,0,0,0,0,0,0,0,1,1,0,0,1,0,0,0,0},{0,0,0,0,0,0,0,0,0,1,1,0,0,1,1,0,0,0},{0,0,0,0,0,0,0,0,0,1,1,0,1,1,0,0,0,0},{0,0,0,0,0,0,0,0,0,1,1,1,1,0,0,0,0,0},{0,0,0,0,0,0,0,0,0,1,1,0,1,1,1,0,0,0},{0,0,0,0,0,0,0,0,0,0,1,1,0,0,1,1,0,0},{0,0,0,0,0,0,0,0,0,0,0,1,1,0,1,1,0,0},{0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0},{0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0},{0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,1},{0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,1,1},{0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1},{0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,0},{0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0}};int i,j;for(i=0;i<=17;i++)for(j=0;j<=17;j++){if (fei[i][j]==1)putpixel(j+x,i+y,BLUE);}}/*********"洋"字**************/int yang(int x,int y){int yang[18][18]={{0,0,0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0}, {1,1,0,0,0,0,1,1,1,0,0,0,1,1,0,0,0,0},{0,0,1,1,0,0,0,0,0,1,1,1,0,0,0,1,0,0}, {0,0,0,1,0,1,1,1,1,1,1,1,1,1,1,1,1,0}, {0,0,0,1,0,0,0,0,0,0,1,0,0,0,0,0,0,0}, {1,1,1,0,0,0,0,0,0,0,1,0,0,0,0,0,0,0}, {0,1,1,1,0,0,0,0,0,0,1,0,0,1,0,0,0,0}, {0,0,1,1,0,0,0,1,1,1,1,1,1,1,1,0,0,0}, {0,0,0,1,0,0,0,0,0,0,1,0,0,0,0,0,0,0}, {0,0,0,1,0,0,1,0,0,0,1,0,0,0,0,0,0,0}, {0,0,0,0,0,1,1,0,0,0,1,0,0,0,0,1,1,0}, {0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, {0,0,0,1,1,0,0,0,0,0,1,0,0,0,0,0,0,0}, {1,1,1,1,0,0,0,0,0,0,1,0,0,0,0,0,0,0}, {0,1,1,1,0,0,0,0,0,0,1,0,0,0,0,0,0,0}, {0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,0,0,0}, {0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,0,0,0}}; int i,j;for(i=0;i<=17;i++)for(j=0;j<=17;j++){if (yang[i][j]==1)putpixel(j+x,i+y,BLUE);}}/******************主场景**********************/int bort(){ int a;setfillstyle(SOLID_FILL,15);bar(49,49,71,71);setfillstyle(SOLID_FILL,BLUE);bar(50,50,70,70);size1=imagesize(49,49,71,71);far1=(void *)malloc(size1);getimage(49,49,71,71,far1);cleardevice();setfillstyle(SOLID_FILL,12);bar(49,49,71,71);size2=imagesize(49,49,71,71);far2=(void *)malloc(size2);getimage(49,49,71,71,far2);setfillstyle(SOLID_FILL,12);bar(49,49,71,71);setfillstyle(SOLID_FILL,GREEN);bar(50,50,70,70);size3=imagesize(49,49,71,71);far3=(void *)malloc(size3);getimage(49,49,71,71,far3);cleardevice(); /*取蛇身节点背景节点虫子节点end*/ setbkcolor(8);setfillstyle(SOLID_FILL,GREEN);bar(21,23,600,450);tline(21,23,600,450,15,8); /***开始游戏场景边框立体效果*******/ tline(23,25,598,448,15,8);tline(45,45,379,379,8,15);tline(43,43,381,381,8,15);tline(390,43,580,430,8,15);tline(392,45,578,428,8,15);tline(412,65,462,85,15,8);tline(410,63,464,87,15,8);tline(410,92,555,390,15,8);tline(412,94,553,388,15,8);tline(431,397,540,420,15,8);tline(429,395,542,422,15,8);tline(46,386,377,428,8,15);tline(44,384,379,430,8,15);setcolor(8);outtextxy(429,109,"press ENTER ");outtextxy(429,129,"---to start"); /*键盘控制说明*/outtextxy(429,169,"press ESC ");outtextxy(429,189,"---to quiet");outtextxy(469,249,"UP");outtextxy(429,289,"LEFT");outtextxy(465,329,"DOWN");outtextxy(509,289,"RIGHT");setcolor(15);outtextxy(425,105,"press ENTER ");outtextxy(425,125,"---to start");outtextxy(425,165,"press ESC ");outtextxy(425,185,"---to quiet");outtextxy(465,245,"UP");outtextxy(425,285,"LEFT");outtextxy(461,325,"DOWN");outtextxy(505,285,"RIGHT"); /*******end*************/setcolor(8);outtextxy(411,52,"score");outtextxy(514,52,"left");setcolor(15);outtextxy(407,48,"score");outtextxy(510,48,"left");size4=imagesize(409,62,465,88); /****分数框放到存********/far4=(void *)malloc(size4);getimage(409,62,465,88,far4);putimage(500,62,far4,COPY_PUT); /*******输出生命框***********/setfillstyle(SOLID_FILL,12);setcolor(RED);outtextxy(415,70,"0"); /***************输入分数为零**********/outtextxy(512,70,"20"); /*************显示还要吃的虫子的数目*********/bar(46,46,378,378);feiyang(475,400);fei(450,400);yang(500,400);outtextxy(58,390,"mailto:jiangzhiliang002tom."); outtextxy(58,410,"snake game");outtextxy(200,410,"made by yefeng");while(1){ if(bioskey(1))a=bioskey(0);if(a==ENTER)break;}}/******************gameover()******************/void gameover(){ char *p="GAME OVER";int cha;setcolor(YELLOW);settextstyle(0,0,6);outtextxy(100,200,p);while(1){if(bioskey(1))cha=bioskey(0);if(cha==ESC){flag=1;break;}}}/***********显示蛇身**********************/void snakepaint(){struct snake *p1;p1=head;putimage(a-11,b-11,far2,COPY_PUT);while(p1!=NULL){putimage(p1->newx-11,p1->newy-11,far1,COPY_PUT);p1=p1->next;}}/****************end**********************//*********************蛇身刷新变化游戏关键部分*******************/ void snakechange(){struct snake *p1,*p2,*p3,*p4,*p5;int i,j;static int n=0;static int score;static int left=20;char sscore[5];char sleft[1];p2=p1=head;while(p1!=NULL){ p1=p1->next;if(p1->next==NULL){a=p1->newx;b=p1->newy; /************记录最后节点的坐标************/ sx=a;sy=b;}p1->newx=p2->centerx;p1->newy=p2->centery;p2=p1;}p1=head;while(p1!=NULL){p1->centerx=p1->newx;p1->centery=p1->newy;p1=p1->next;}/********判断按键方向*******/if(bioskey(1)){ ch=bioskey(0);if(ch!=RIGHT&&ch!=LEFT&&ch!=UP&&ch!=DOWN&&ch!=ESC) /********chy为上一次的方向*********/ch=chy;}switch(ch){case LEFT: if(control!=4){head->newx=head->newx-22;head->centerx=head->newx;control=2;if(head->newx<47)gameover();}else{ head->newx=head->newx+22;head->centerx=head->newx;control=4;if(head->newx>377)gameover();}chy=ch;break;case DOWN:if(control!=1){ head->newy=head->newy+22;head->centery=head->newy; control=3;if(head->newy>377)gameover();}else{ head->newy=head->newy-22; head->centery=head->newy;control=1;if(head->newy<47)gameover();}chy=ch;break;case RIGHT: if(control!=2){ head->newx=head->newx+22;head->centerx=head->newx;control=4;if(head->newx>377)gameover();}else{ head->newx=head->newx-22;head->centerx=head->newx;control=2;if(head->newx<47)gameover();}chy=ch;break;case UP: if(control!=3){ head->newy=head->newy-22;head->centery=head->newy;control=1;if(head->newy<47)gameover();}else{ head->newy=head->newy+22;head->centery=head->newy;control=3;if(head->newy>377)gameover();}chy=ch;break;case ESC:flag=1;break;}/* if 判断是否吃蛇*/if(flag!=1){ if(head->newx==scenterx&&head->newy==scentery) { p3=head;while(p3!=NULL){ p4=p3;p3=p3->next;}p3=(struct snake *)malloc(sizeof(struct snake));p4->next=p3;p3->centerx=a;p3->newx=a;p3->centery=b;p3->newy=b;p3->next=NULL;a=500;b=500;putimage(409,62,far4,COPY_PUT); /********** 分数框挡住**************/ putimage(500,62,far4,COPY_PUT); /*********把以前的剩下虫子的框挡住********/ score=(++n)*100;left--;itoa(score,sscore,10);itoa(left,sleft,10);setcolor(RED);outtextxy(415,70,sscore);outtextxy(512,70,sleft);nextshow=1;if(left==0) /************判断是否过关**********/donghua(); /*******如果过关,播放过关动画*********************/ }p5=head; /*********************判断是否自杀***************************/ p5=p5->next;p5=p5->next;p5=p5->next;p5=p5->next; /****从第五个节点判断是否自杀************/while(p5!=NULL){if(head->newx==p5->centerx&&head->newy==p5->centery){ gameover();break;}elsep5=p5->next;}}}/************snakechange()函数结束*******************//*****************************主函数******************************************/ int main(){ int i;init(); /**********初始化图形系统**********/welcome(); /*********8欢迎界面**************/bort(); /*********主场景***************/snakede(); /**********连表初始化**********/while(1){ snakechange();if(flag==1)break;snakepaint();ran();for(i=0;i<=grade;i++)delay(3000);}free(far1); free(far2); free(far3); free(far4); closegraph(); return 0;}。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
SetPos(p->x,p->y);
cout<<"*";
p=p->next;
}
SetPos(Food_x,Food_y);
cout<<"*";
}
void Sneak::Move()
{
Body *New;
New=new(Body);//新的蛇身结点
if(Direction==1)//确定新蛇头的坐标
Speed=c;//等级1
}
void Map();//画界面函数
void Paint();//画蛇身函数
void Food();//生成食物
int Over();//判断游戏是否结束
void Gaming();//游戏进程函数
void Move();//移动
};
void Sneak::Map()//使用
temp1=new(Body);
temp1->x=3;
temp1->y=2;
temp2=new(Body);
temp2->x=2;
temp2->y=2;
head->next=temp1;
temp1->next=temp2;
temp2->next=NULL;
Direction=b;//方向为右
Count=a;//长为3
New->y=head->y+1;
New->next=head;
head=New;
}
if(Direction==4)
{
New->x=head->x+1;
New->y=head->y;
New->next=head;
head=New;
}
}
void Sneak::Gaming()
{
system("cls");//刷新屏幕
Body *next;//下一个结点
};
void SetPos(int i,int j)//设定光标位置
{
COORD pos={i-1,j-1};//坐标变量
HANDLE Out=GetStdHandle(STD_OUTPUT_HANDLE);//获取输出句柄
SetConsoleCursorPosition(Out,pos);//设定光标位置
}
class Sneak//贪吃蛇类
{
private:
Body *head;//蛇头指针
int Direction;//移动方向。1,2,3,4对应上左下右
int Count;//蛇长
int Speed;//速度(等级)
int FoodExist;//食物存在标记
int Food_x;//食物X坐标
#include<iostream>
#include<windows.h>
#include<conio.h>
#include<time.h>
#include<stdlib.h>
using namespace std;
int GameOver=0;
struct Body//蛇身(链表结构)
{
int x,y;//蛇身结点坐标
{
int i;
for(i=1;i<=50;it;<"-";
}
for(i=2;i<=25;i++)
{
SetPos(1,i);
cout<<"|";
SetPos(50,i);
cout<<"|";
}
for(i=1;i<=50;i++)
{
SetPos(i,25);
cout<<"-";
{
New->x=head->x;
New->y=head->y-1;
New->next=head;
head=New;
}
if(Direction==2)
{
New->x=head->x-1;
New->y=head->y;
New->next=head;
head=New;
}
if(Direction==3)
{
New->x=head->x;
Direction=1;
if((x=='S'||x=='s')&&Direction!=1)
Direction=3;
if((x=='A'||x=='a')&&Direction!=4)
Direction=2;
if((x=='D'||x=='d')&&Direction!=2)
}
SetPos(54,3);
cout<<"贪吃蛇";
SetPos(54,5);
cout<<"贪吃蛇长度为:"<<Count;
SetPos(54,7);
cout<<"等级:"<<Speed;
}
void Sneak::Food()
{
Body *p;
int InBody=0;//判断食物是否产生在蛇体内
srand((int)time(0));//用系统时间来做随机数种子
while(1)
{
Food_x=rand()%48+2;//随机出食物的坐标
Food_y=rand()%23+2;
p=head;
while(p!=NULL)//判断食物是否产生在蛇体内
{
if(p->x==Food_x&&p->y==Food_y)
{
InBody=1;
break;
}
p=p->next;
int Food_y;//食物Y坐标
public:
Sneak(int a=3,int b=4,int c=1,int d=0)//构造函数
{
FoodExist=d;//起始不存在食物
Body *temp1,*temp2;
head=new(Body);//申请起始蛇身3节
head->x=4;
head->y=2;
p=head->next;
while(p!=NULL)//是否撞到蛇身
{
if((head->x==p->x)&&(head->y==p->y))
return 1;
p=p->next;
}
return 0;
}
void Sneak::Paint()
{
Body *p;
p=head;
while(p!=NULL)
}
if(InBody==0)//食物不在蛇身。生成成功
break;
InBody=0;
}
}
int Sneak::Over()
{
Body *p;
p=head;
if((head->x)>=50||(head->x)<=1||(head->y)<=1||(head->y)>=25)//是否撞到边缘
return 1;
char x;
Body *p;
Map();//画界面的先
Paint();//再画蛇身
while(1)
{
if(_kbhit())//_kbhit()判断是否有键盘操作
{
x=_getch();//重缓冲区读出一个字符赋给x
if((x=='W'||x=='w')&&Direction!=3)//改变蛇的方向(不可以是反方向)