Codec C90 and PrecisionHD 1080p June 2008
高质量DXT压缩使用CUDA技术(2009年)说明书

March 2009High Quality DXT Compression using OpenCL for CUDAIgnacio Castaño*******************Document Change HistoryVersion Date Responsible Reason for Change0.1 02/01/2007 Ignacio Castaño First draft0.2 19/03/2009 Timo Stich OpenCL versionAbstractDXT is a fixed ratio compression format designed for real-time hardware decompression of textures. While it’s also possible to encode DXT textures in real-time, the quality of the resulting images is far from the optimal. In this white paper we will overview a more expensivecompression algorithm that produces high quality results and we will see how to implement it using CUDA to obtain much higher performance than the equivalent CPU implementation.MotivationWith the increasing number of assets and texture size in recent games, the time required to process those assets is growing dramatically. DXT texture compression takes a large portion of this time. High quality DXT compression algorithms are very expensive and while there are faster alternatives [1][9], the resulting quality of those simplified methods is not very high. The brute force nature of these compression algorithms makes them suitable to be parallelized and adapted to the GPU. Cheaper compression algorithms have already been implemented [2] on the GPU using traditional GPGPU approaches. However, with the traditional GPGPU programming model it’s not possible to implement more complex algorithms where threads need to share data and synchronize.How Does It Work?In this paper we will see how to use CUDA to implement a high quality DXT1 texturecompression algorithm in parallel. The algorithm that we have chosen is the cluster fit algorithm as described by Simon Brown [3]. We will first provide a brief overview of the algorithm and then we will describe how did we parallelize and implement it in CUDA.DXT1 FormatDXT1 is a fixed ratio compression scheme that partitions the image into 4x4 blocks. Each block is encoded with two 16 bit colors in RGB 5-6-5 format and a 4x4 bitmap with 2 bits per pixel. Figure 1 shows the layout of the block.Figure 1. DXT1 block layoutThe block colors are reconstructed by interpolating one or two additional colors between the given ones and indexing these and the original colors with the bitmap bits. The number of interpolated colors is chosen depending on whether the value of ‘Color 0’ is lower or greater than ‘Color 1’.BitmapColorsThe total size of the block is 64 bits. That means that this scheme achieves a 6:1 compression ratio. For more details on the DXT1 format see the specification of the OpenGL S3TCextension [4].Cluster FitIn general, finding the best two points that minimize the error of a DXT encoded block is a highly discontinuous optimization problem. However, if we assume that the indices of the block are known the problem becomes a linear optimization problem instead: minimize the distance from each color of the block to the corresponding color of the palette.Unfortunately, the indices are not known in advance. We would have to test them all to find the best solution. Simon Brown [3] suggested pruning the search space by considering only the indices that preserve the order of the points along the least squares line.Doing that allows us to reduce the number of indices for which we have to optimize theendpoints. Simon Brown provided a library [5] that implements this algorithm. We use thislibrary as a reference to compare the correctness and performance of our CUDAimplementation.The next section goes over the implementation details.OpenCL ImplementationPartitioning the ProblemWe have chosen to use a single work group to compress each 4x4 color block. Work items that process a single block need to cooperate with each other, but DXT blocks are independent and do not need synchronization or communication. For this reason the number of workgroups is equal to the number of blocks in the image.We also parameterize the problem so that we can change the number of work items per block to determine what configuration provides better performance. For now, we will just say that the number of work items is N and later we will discuss what the best configuration is.During the first part of the algorithm, only 16 work items out of N are active. These work items start reading the input colors and loading them to local memory.Finding the best fit lineTo find a line that best approximates a set of points is a well known regression problem. The colors of the block form a cloud of points in 3D space. This can be solved by computing the largest eigenvector of the covariance matrix. This vector gives us the direction of the line.Each element of the covariance matrix is just the sum of the products of different colorcomponents. We implement these sums using parallel reductions.Once we have the covariance matrix we just need to compute its first eigenvector. We haven’t found an efficient way of doing this step in parallel. Instead, we use a very cheap sequential method that doesn’t add much to the overall execution time of the group.Since we only need the dominant eigenvector, we can compute it directly using the Power Method [6]. This method is an iterative method that returns the largest eigenvector and only requires a single matrix vector product per iteration. Our tests indicate that in most cases 8iterations are more than enough to obtain an accurate result.Once we have the direction of the best fit line we project the colors onto it and sort them along the line using brute force parallel sort. This is achieved by comparing all the elements against each other as follows:cmp[tid] = (values[0] < values[tid]);cmp[tid] += (values[1] < values[tid]);cmp[tid] += (values[2] < values[tid]);cmp[tid] += (values[3] < values[tid]);cmp[tid] += (values[4] < values[tid]);cmp[tid] += (values[5] < values[tid]);cmp[tid] += (values[6] < values[tid]);cmp[tid] += (values[7] < values[tid]);cmp[tid] += (values[8] < values[tid]);cmp[tid] += (values[9] < values[tid]);cmp[tid] += (values[10] < values[tid]);cmp[tid] += (values[11] < values[tid]);cmp[tid] += (values[12] < values[tid]);cmp[tid] += (values[13] < values[tid]);cmp[tid] += (values[14] < values[tid]);cmp[tid] += (values[15] < values[tid]);The result of this search is an index array that references the sorted values. However, this algorithm has a flaw, if two colors are equal or are projected to the same location of the line, the indices of these two colors will end up with the same value. We solve this problem comparing all the indices against each other and incrementing one of them if they are equal:if (tid > 0 && cmp[tid] == cmp[0]) ++cmp[tid];if (tid > 1 && cmp[tid] == cmp[1]) ++cmp[tid];if (tid > 2 && cmp[tid] == cmp[2]) ++cmp[tid];if (tid > 3 && cmp[tid] == cmp[3]) ++cmp[tid];if (tid > 4 && cmp[tid] == cmp[4]) ++cmp[tid];if (tid > 5 && cmp[tid] == cmp[5]) ++cmp[tid];if (tid > 6 && cmp[tid] == cmp[6]) ++cmp[tid];if (tid > 7 && cmp[tid] == cmp[7]) ++cmp[tid];if (tid > 8 && cmp[tid] == cmp[8]) ++cmp[tid];if (tid > 9 && cmp[tid] == cmp[9]) ++cmp[tid];if (tid > 10 && cmp[tid] == cmp[10]) ++cmp[tid];if (tid > 11 && cmp[tid] == cmp[11]) ++cmp[tid];if (tid > 12 && cmp[tid] == cmp[12]) ++cmp[tid];if (tid > 13 && cmp[tid] == cmp[13]) ++cmp[tid];if (tid > 14 && cmp[tid] == cmp[14]) ++cmp[tid];During all these steps only 16 work items are being used. For this reason, it’s not necessary to synchronize them. All computations are done in parallel and at the same time step, because 16 is less than the warp size on NVIDIA GPUs.Index evaluationAll the possible ways in which colors can be clustered while preserving the order on the line are known in advance and for each clustering there’s a corresponding index. For 4 clusters there are 975 indices that need to be tested, while for 3 clusters there are only 151. We pre-compute these indices and store them in global memory.We have to test all these indices and determine which one produces the lowest error. In general there are indices than work items. So, we partition the total number of indices by the number of work items and each work item loops over the set of indices assigned to it. It’s tempting to store the indices in constant memory, but since indices are used only once for each work group, and since each work item accesses a different element, coalesced global memory loads perform better than constant loads.Solving the Least Squares ProblemFor each index we have to solve an optimization problem. We have to find the two end points that produce the lowest error. For each input color we know what index it’s assigned to it, so we have 16 equations like this:i i i x b a =+βαWhere {}i i βα, are {}0,1, {}32,31, {}21,21, {}31,32 or {}1,0 depending on the index and the interpolation mode. We look for the colors a and b that minimize the least square error of these equations. The solution of that least squares problem is the following:∑∑⋅∑∑∑∑= −i i i i i ii i i i x x b a βαββαβαα122 Note: The matrix inverse is constant for each index set, but it’s cheaper to compute it everytime on the kernel than to load it from global memory. That’s not the case of the CPU implementation.Computing the ErrorOnce we have a potential solution we have to compute its error. However, colors aren’t stored with full precision in the DXT block, so we have to quantize them to 5-6-5 to estimate the error accurately. In addition to that, we also have to take in mind that the hardware expands thequantized color components to 8 bits replicating the highest bits on the lower part of the byte as follows:R = (R << 3) | (R >> 2); G = (G << 2) | (G >> 4); B = (B << 3) | (B >> 2);Converting the floating point colors to integers, clamping, bit expanding and converting them back to float can be time consuming. Instead of that, we clamp the color components, round the floats to integers and approximate the bit expansion using a multiplication. We found the factors that produce the lowest error using an offline optimization that minimized the average error.r = round(clamp(r,0.0f,1.0f) * 31.0f); g = round(clamp(g,0.0f,1.0f) * 63.0f); b = round(clamp(b,0.0f,1.0f) * 31.0f); r *= 0.03227752766457f; g *= 0.01583151765563f; b *= 0.03227752766457f;Our experiment show that in most cases the approximation produces the same solution as the accurate solution.Selecting the Best SolutionFinally, each work item has evaluated the error of a few indices and has a candidate solution.To determine which work item has the solution that produces the lowest error, we store the errors in local memory and use a parallel reduction to find the minimum. The winning work item writes the endpoints and indices of the DXT block back to global memory.Implementation DetailsThe source code is divided into the following files:•DXTCompression.cl: This file contains OpenCL implementation of the algorithm described here.•permutations.h: This file contains the code used to precompute the indices.dds.h: This file contains the DDS file header definition. PerformanceWe have measured the performance of the algorithm on different GPUs and CPUscompressing the standard Lena. The design of the algorithm makes it insensitive to the actual content of the image. So, the performance depends only on the size of the image.Figure 2. Standard picture used for our tests.As shown in Table 1, the GPU compressor is at least 10x faster than our best CPUimplementation. The version of the compressor that runs on the CPU uses a SSE2 optimized implementation of the cluster fit algorithm. This implementation pre-computes the factors that are necessary to solve the least squares problem, while the GPU implementation computes them on the fly. Without this CPU optimization the difference between the CPU and GPU version is even larger.Table 1. Performance ResultsImage TeslaC1060 Geforce8800 GTXIntel Core 2X6800AMD Athlon64 DualCore 4400Lena512x51283.35 ms 208.69 ms 563.0 ms 1,251.0 msWe also experimented with different number of work-items, and as indicated in Table 2 we found out that it performed better with the minimum number.Table 2. Number of Work Items64 128 25654.66 ms 86.39 ms 96.13 msThe reason why the algorithm runs faster with a low number of work items is because during the first and last sections of the code only a small subset of work items is active.A future improvement would be to reorganize the code to eliminate or minimize these stagesof the algorithm. This could be achieved by loading multiple color blocks and processing them in parallel inside of the same work group.ConclusionWe have shown how it is possible to use OpenCL to implement an existing CPU algorithm in parallel to run on the GPU, and obtain an order of magnitude performance improvement. We hope this will encourage developers to attempt to accelerate other computationally-intensive offline processing using the GPU.High Quality DXT Compression using CUDAMarch 2009 9References[1] “Real-Time DXT Compression”, J.M.P. van Waveren. /cd/ids/developer/asmo-na/eng/324337.htm[2] “Compressing Dynamically Generated Textures on the GPU”, Oskar Alexandersson, Christoffer Gurell, Tomas Akenine-Möller. http://graphics.cs.lth.se/research/papers/gputc2006/[3] “DXT Compression Techniques”, Simon Brown. /?article=dxt[4] “OpenGL S3TC extension spec”, Pat Brown. /registry/specs/EXT/texture_compression_s3tc.txt[5] “Squish – DXT Compression Library”, Simon Brown. /?code=squish[6] “Eigenvalues and Eigenvectors”, Dr. E. Garcia. /information-retrieval-tutorial/matrix-tutorial-3-eigenvalues-eigenvectors.html[7] “An Experimental Analysis of Parallel Sorting Algorithms”, Guy E. Blelloch, C. Greg Plaxton, Charles E. Leiserson, Stephen J. Smith /blelloch98experimental.html[8] “NVIDIA CUDA Compute Unified Device Architecture Programming Guide”.[9] NVIDIA OpenGL SDK 10 “Compress DXT” sample /SDK/10/opengl/samples.html#compress_DXTNVIDIA Corporation2701 San Tomas ExpresswaySanta Clara, CA 95050 NoticeALL NVIDIA DESIGN SPECIFICATIONS, REFERENCE BOARDS, FILES, DRAWINGS, DIAGNOSTICS, LISTS, AND OTHER DOCUMENTS (TOGETHER AND SEPARATELY, “MATERIALS”) ARE BEING PROVIDED “AS IS.” NVIDIA MAKES NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT,MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE.Information furnished is believed to be accurate and reliable. However, NVIDIA Corporation assumes no responsibility for the consequences of use of such information or for any infringement of patents or otherrights of third parties that may result from its use. No license is granted by implication or otherwise under any patent or patent rights of NVIDIA Corporation. Specifications mentioned in this publication are subject to change without notice. This publication supersedes and replaces all information previously supplied. NVIDIA Corporation products are not authorized for use as critical components in life support devices or systems without express written approval of NVIDIA Corporation.TrademarksNVIDIA, the NVIDIA logo, GeForce, and NVIDIA Quadro are trademarks or registeredtrademarks of NVIDIA Corporation in the United States and other countries. Other company and product names may be trademarks of the respective companies with which they are associated. Copyright© 2009 NVIDIA Corporation. All rights reserved.。
DMK 33UX264 相机技术手册说明书

技术细节1.要件速览 42.尺寸图 6 2.1DMK 33UX264 带脚架适配器的C型接口 (6)2.2DMK 33UX264 不带脚架适配器的C型接口 (7)2.3DMK 33UX264 带脚架适配器的CS型接口 (8)2.4DMK 33UX264 不带脚架适配器的CS型接口 (9)3.I/O 连接器 10 3.112-pin I/O 连接器 (10)3.1.1TRIGGER_IN (11)3.1.2STROBE_OUT (11)4.光谱特征 124.1光谱灵敏度 - IMX264LLR-C (12)5.相机控制 13 5.1传感器读出控制 (13)5.1.1像素格式 (13)5.1.1.18-Bit Monochrome (13)16-Bit Monochrome (13)5.1.1.25.1.2分辨率 (14)5.1.3读出模式 (14)5.1.4帧速率 (15)5.1.5局部扫描偏移 (16)5.1.6图像翻转 (17)5.2图像传感器控制 (17)5.2.1曝光时间 (17)5.2.2增益 (18)5.2.3黑电平 (18)5.3自动曝光及增益控制 (18)5.3.1自动曝光 (19)5.3.2自动增益 (19)5.3.3自动参考值 (19)5.3.4强光缩减 (20)5.3.5自动曝光限制 (20)5.3.6自动增益限制 (21)5.4触发 (22)5.4.1触发模式 (22)5.4.2触发极性 (22)5.4.3软件触发 (22)5.4.4触发曝光模式 (23)5.4.5触发脉冲计数 (23)5.4.6触发源 (23)5.4.7触发重叠 (24)5.4.8IM X低延迟模式 (24)5.5触发定时参数 (24)5.5.1触发延迟 (24)5.5.2触发去抖时间 (25)5.5.3触发遮罩时间 (25)5.5.4触发噪声抑制时间 (25)5.6数字I/O (26)5.6.1通用输入 (26)5.6.2通用输出 (26)5.7频闪 (27)5.7.1频闪启用 (27)5.7.2频闪极性 (27)5.7.3频闪操作 (28)5.7.4频闪时间 (28)5.7.5頻閃延遲 (28)5.8图像处理 (28)伽玛 (29)5.8.15.8.2查找表 (29)5.9自动功能感兴趣的区域 (30)5.9.1自动功能ROI启用 (30)5.9.2自动功能ROI预设 (30)5.9.3自动功能ROI自定义矩形 (31)5.10用户设置 (32)5.10.1用户设置选择器 (32)5.10.2加载用户设置 (32)5.10.3保存用户设置 (33)5.10.4默认用户配置 (33)5.11多帧输出模式启用 (33)5.11.1多帧输出模式启用 (33)5.11.2多帧输出模式帧计数 (34)5.11.3多帧输出模式曝光时间 (34)5.11.4多帧输出模式自定义增益 (34)5.11.5多帧输出模式增益 (34)6.R ev i s i o n H i story 361要件速览2尺寸图2.1DMK 33UX264 带脚架适配器的C型接口2.4DMK 33UX264 不带脚架适配器的CS型接口3I/O 连接器3.112-pin I/O 连接器相机后视图1开极闸M O S FET最大限制0.2A(I D)!2启动电流最低条件3.5 m A!3G:地O:输出 I:输入3.1.1T RI GGE R_I NTRIGGER_IN线可用于将曝光时间的开始与外部事件同步。
Intensifier T Series HD-TVI 1080p 2MP 传统摄像头说明书

DIMENSIONSFEATURESHTINTT5T – Grey housingSPECIFICATIONSImage Sensor.................. 1/3” Progressive Scan CMOS, 2MP Minimum Illumination....... 0.001 lux (Sens-up X32) Effective Pixels................ 1984 (H) x 1105 (V) Total Pixels...................... 2000 (H) x 1121 (V) Scanning System............ Progressive S/N Ratio......................... More than 50dB Output Resolution............ 1920x1080 @ 30fps Video Output.................... 1.0Vp-p / 75 OhmsElectronic Shutter............ Auto / Manual (1/30 – 1/30,000 sec.) Day / Night....................... Auto / Color / BW / External Communication............... UTC (up the coax) Power............................. 12VDC / 24VACPower Consumption........ 150mA (DC), 2W (AC) Operating Temperature....14° F – 122° FUnit Dimensions.............. 2.5”(W) x 2.2”(H) x 4”(L) Unit Weight...................... 1.5Ibs.Certifications.................... FCC, RoHS Signal Distance............... Up to 1600 feet(depending on coax quality)*• Full HD resolution over coax (HD-TVI) • Supports up to Full HD 1080p @ 30fps • True WDR operation• Superior low-light performance• Amplify existing light with no distance limitation• PRESET (Indoor/Outdoor/Lowlight/Hallway/Lobby/Elevator) • Full OSD operation thru on-board control and UTC (up the coax) • Additional 960H analog output • Signal distance up to 1600 feet* • 12VDC/24VAC dual voltage operation • 5 year warrantyOSD M ENU T REEEXPOSURE• B /W• E XTERN• L OW• M IDDLE• H IGH• S HARPNESS • G AMMA • M IRROR • F LIPDAY / N IGHTWHITE B AL.SPECO D NRIMAGEEXIT• B RIGHTNESS• S HUTTERBACKLIGHT• H LC• B LC• W DR• A UTO• A WB• C OLOR G AIN• A CE• D EFOGSYSTEM• C OM.• I MAGE R ANGE • C OLOR S PACE• F RAME R ATE• I NTENSIFY• A GC• C OLOR• P RIVACYLENS MOTION • O UTPUT • L ANGUAGE• C OLOR B AR• R ESET• C AMERA T ITLE• F REQLENS : S elect t he l ens t ype (ALC/ELC)EXPOSURE : A djusts e xposure s eEngs.B RIGHTNESS : A djusts i mage b rightness. (0~ 20) S HUTTER : A uto / M anual S huTer c an b e s electable -‐ A UTO : S huTer c an b e s electable a utomaVcally.-‐ F LICKER(Flicker L ess) : S elects t his w hen p icture flickering.-‐ M anual : C ontrols t he s huTer s peed m anually f rom 1/30 ~1/30000 I NTENSIFY : S ets t he d esired m ulVple o f t he d igital e xposure l ength. (OFF/x2 ~ x 32)A GC(Auto G ain C ontrol): A djusts t he A GC l evel. (0~10)OSD M ENU D etailsPRESET MODE• INDOOR • OUTDOOR • LOW LIGHT • LOBBY • HALLWAY • ELEVATORMAIN SETUP Side ViewFront ViewBack panel ConfigurationUnit : inchTVI OutCVBS Out (Analogue)Power In (12VDC/24VAC)OSD joystick4” L2.5” W2.2” HSpeco T echnologies i s c onstantly d eveloping p roduct i mprovements. We r eserve t he r ight t o m odify p roduct d esign a nd s pecificaRons w ithout noRce a nd w ithout i ncurring a ny o bligaRon. R ev. 160809OSD M ENU D etailsDAY / N IGHT : A djust D ay / N ight o pRons . C OLOR : A lways C olor m ode. B /W : A lways B /W m ode.-‐ A NTI-‐SAT. : A djusts t he A nV S aturaVon l evel (0~20) E XT : p lease d o n ot u se t his m ode f or t his i temA UTO : D ay / N ight i s s witching a utomaVcally b y A GC l evel. -‐ A NTI-‐SAT. : A djusts t he A nV S aturaVon l evel (0~20) -‐ A GC T HRES : A djusts A GC T HRES (0~20) -‐ A GC M ARGIN : A djusts A GC m argin (0~20)-‐ D ELAY : A djusts t he c hanging d elay V me (LOW/MIDDLE/HIGH)WHITE B AL. : A djusts w hite b alancing o pRons.A WB : i t g oes t o o pVmized c olor l evel a utomaVcally.C OLOR G AIN : S ets t he d esired C olor G ain v alue (0~20)S PECO D NR : U ses t o r educe t he b ackground n oise i n a l ow l uminance e nvironment w ith 2D + 3D filtering s ystem.BACKLIGHT : A djusts b acklight o pRons.W DR : W DR i lluminates d arker a reas o f a n i mage w hile r etaining t hes ame l ight l evel f or b righter a reas t o e ven o ut t he o verall b rightness o f i mages w ith h igh c ontrast b etween b right a nd d ark a rea -‐ A djusts t he W DR W eight (LOW/MIDDLE/HIGH) * C VBS o ut c annot a djust t his f uncVon.B LC : P roduces a c learer i mage o f a n o bject d arkened b y s trong b acklighVng. -‐ H -‐POS : A djusts t he h orizontal p osiVon(0~20) -‐ V -‐POS : A djusts t he V erVcal p osiVon(0~20) -‐ H -‐SIZE : A djusts t he h orizontal b lock s ize (0~20) -‐ V -‐SIZE : A djusts t he v erVcal b lock s ize (0~20)H LC : U ses t o c ontain e xtremely b right a reas s uch a s f rom c ar h eadlight, t he l ight c an b e m asked o ut m uch o f t he o n-‐screen d etails. IMAGE : A djusts v arious i mage o pRons.S HARPNESS: A djusts s harpness l evel. I ncreasing t his v alue, t he p icture o utline b ecomes s tronger a nd c lear. (0~10) G AMMA : S ets t he d esired G amma v alue. (0.45 ~ 0.75) M IRROR : C hange t he v ideo d irecVon h orizontally. F LIP: C hange t he v ideo d irecVon p erpendicularly.A CE (D-‐WDR) : U ses a d igital w ide d ynamic r ange t o b alance d ark a nd o ver s aturated a reas w ithin t he i mage.D EFOG : A cVvated t his m ode w hen t he v ideo o r t he w eather i s f oggy. P RIVACY : U sed t o h ide r egions o f t he i mage. -‐ Z ONE N UM : S elects t he z one n umber u p 15. -‐ Z ONE D ISP : S elects d esired z one w ith O N o r O FF -‐ H -‐POS : A djusts t he h orizontal p osiVon(0~60) -‐ V -‐POS : A djusts t he v erVcal p osiVon(0~40) -‐ H -‐SIZE : A djusts t he h orizontal s ize(0~40) -‐ V -‐SIZE : A djusts t he v erVcal s ize(0~40)-‐ Y -‐LEVEL : A djusts t he y ellow c olor l evel (0~20) -‐ C B L EVEL : A djusts t he b lue c olor l evel (0~20) -‐ C R L EVEL : A djusts t he r ed c olor l evel (0~20)SYSTEM : A djusts v arious c amera s ystem o pRons. O UTPUTF RAME R ATE: S elect t he f rame r ate 30fps/60fps a ccording t o t he v ideooutput m ode. F REQ C OM.-‐ C AM I D : S ets t he c amera I D f or t he R S-‐485 (0~255)-‐ B AUD R ATE : S ets t he b aud r ate f or t he R S-‐485 (2400~115200). I MAGE R ANGE C OLOR S PACEC OLOR B AR : M anufacturer’s o pVonL ANGUAGE : S ets t he d esired O SD l anguage C AMERA T ITLER ESET: P ress w ith l ong t o r eset a ll s elngs t o f actory d efaults.MOTION : A djust m oRon d etecRon s eEngs.-‐ S ENSITIVITY : S ets t he d esired o f “MoVon” (0~20)-‐ W INDOW T ONE : A djusts t he w indow t one v alue (0~60) -‐ W INDOW U SE : A djusts t he w indow s elng s ize (0~3) -‐ W INDOW Z ONE : a cVvate o r d eacVvate m oVon w indow -‐ D ET H -‐POS :Adjusts t he h orizontal p osiVon(0~60) -‐ D ET V -‐POS : A djusts t he v erVcal p osiVon(0~40) -‐ D ET H -‐SIZE : A djusts t he h orizontal s ize(0~60) -‐ D ET V -‐SIZE : A djusts t he v erVcal s ize(0~40) -‐ A LARM : S elect A larm b etween O N o f O FFHTINTT5T – Grey housing。
Hikvision DS-7200HQHI-K1 商品说明书
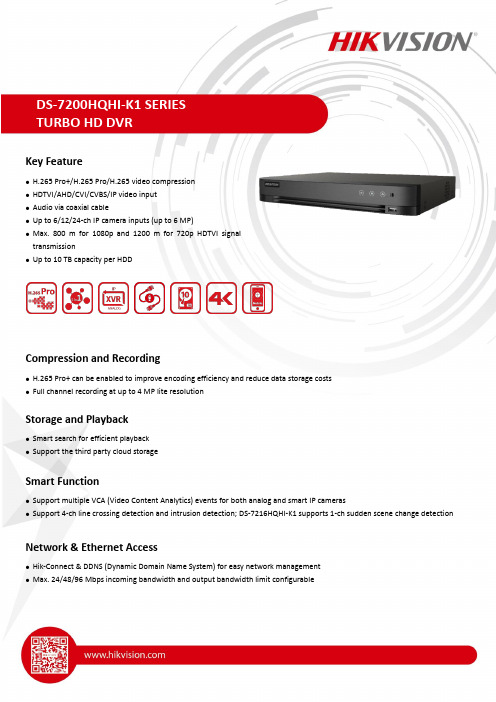
Key Feature● H.265 Pro+/H.265 Pro/H.265 video compression ● HDTVI/AHD/CVI/CVBS/IP video input ● Audio via coaxial cable● Up to 6/12/24-ch IP camera inputs (up to 6 MP) ● Max.800 m for 1080p and 1200 m for 720p HDTVI signaltransmission● Up to 10 TB capacity per HDDCompression and Recording● H.265 Pro+ can be enabled to improve encoding efficiency and reduce data storage costs ● Full channel recording at up to 4 MP lite resolutionStorage and Playback● Smart search for efficient playback ● Support the third party cloud storageSmart Function● Support multiple VCA (Video Content Analytics) events for both analog and smart IP cameras● Support 4-ch line crossing detection and intrusion detection; DS-7216HQHI-K1 supports 1-ch sudden scene change detectionNetwork & Ethernet Access● Hik-Connect & DDNS (Dynamic Domain Name System) for easy network management ● Max. 24/48/96 Mbps incoming bandwidth and output bandwidth limit configurableSpecificationModel DS-7204HQHI-K1DS-7208HQHI-K1DS-7216HQHI-K1 Perimeter Protection¹Line Crossing Detection/ Intrusion Detection 2-chEnhanced VCA mode on: 4-ch2-chEnhanced VCA mode on: 4-ch2-chEnhanced VCA mode on: 4-chRecordingVideo compression H.265 Pro+/H.265 Pro/H.265/H.264+/H.264Encoding resolution When 1080p lite mode is not enabled:4 MP lite/3 MP/1080p/720p/VGA/WD1/4CIF/CIF When 1080p Lite mode is enabled:4 MP lite/3 MP/1080p lite/720p lite/VGA/WD1/4CIF/CIFFrame rate Main stream:When 1080p Lite mode is not enabled:For 4 MP stream access:4 MP lite@15fps; 1080p lite/720p/WD1/4CIF/VGA/CIF@25fps (P)/30fps (N)For 3 MP stream access: 3 MP/1080p/720p/VGA/WD1/4CIF/CIF@15fpsFor 1080p stream access:1080p/720p@15fps; VGA/WD1/4CIF/CIF@25fps (P)/30fps (N)For 720p stream access: 720p/VGA/WD1/4CIF/CIF@25fps (P)/30fps (N)When 1080p Lite mode is enabled:4 MP lite/3 MP@15fps; 1080p lite/720p lite/VGA/WD1/4CIF/CIF@25fps (P)/30fps (N) Sub-stream:WD1/4CIF@12fps; CIF@25fps (P)/30fps (N)Video bit rate32 Kbps to 6 Mbps Dual stream SupportStream type Video, Video & Audio Audio compression G.711uAudio bit rate64 KbpsVideo and AudioIP video input21-ch (up to 5-ch)Enhanced IP mode on:2-ch (up to 6-ch), each upto 4 Mbps2-ch (up to 10-ch)Enhanced IP mode on:4-ch (up to 12-ch), each upto 4 Mbps2-ch (up to 18-ch)Enhanced IP mode on:8-ch (up to 24-ch), each upto 4 MbpsUp to 6 MP resolutionSupport H.265+/H.265/H.264+/H.264 IP camerasAnalog video input 4-ch8-ch16-ch BNC interface (1.0 Vp-p, 75 Ω), supporting coaxitron connectionHDTVI input 4 MP, 3 MP, 1080p30, 1080p25, 720p60, 720p50, 720p30, 720p25*: The 3 MP signal input is only available for channel 1 of DS-7204HQHI-K1, for channel 1/2 of DS-7208HQHI-K1, and for channel 1/2/3/4 of DS-7216HQHI-K1.AHD input 4 MP, 1080p25, 1080p30, 720p25, 720p30 HDCVI input 4 MP, 1080p25, 1080p30, 720p25, 720p30CVBS input PAL/NTSCCVBS output1-ch, BNC (1.0 Vp-p, 75 Ω), resolution: PAL: 704 × 576, NTSC: 704 × 480VGA output1-ch, 1920 × 1080/60Hz, 1280 × 1024/60Hz, 1280 × 720/60Hz, 1024 × 768/60HzHDMI output 1-ch, 1920 × 1080/60Hz, 1280 × 1024/60Hz, 1280 ×720/60Hz, 1024 × 768/60Hz1-ch, 4K (3840 ×2160)/30Hz, 2K (2560 ×1440)/60Hz, 1920 ×1080/60Hz, 1280 ×1024/60Hz, 1280 ×720/60Hz, 1024 ×768/60HzAudio input 1-ch, RCA (2.0 Vp-p, 1 KΩ)4-ch via coaxial cable8-ch via coaxial cable16-ch via coaxial cableAudio output1-ch, RCA (Linear, 1 KΩ)Two-way audio1-ch, RCA (2.0 Vp-p, 1 KΩ) (using the first audio input) Synchronous playback4-ch8-ch16-ch NetworkTotal bandwidth72 Mbps96 Mbps128 Mbps Incoming bandwidth(Enhanced IP mode)24 Mbps48 Mbps96 Mbps Remote connection3264128Network protocol TCP/IP, PPPoE, DHCP, Hik-Connect, DNS, DDNS, NTP, SADP, NFS, iSCSI, UPnP™, HTTPS, ONVIFNetwork interface1, RJ45 10M/100M self-adaptive Ethernet interface 1, RJ4510M/100M/1000M self-adaptive Ethernet interfaceAuxiliary interfaceSATA 1 SATA interfaceCapacity Up to 10 TB capacity for each disk Serial interface RS-485 (half-duplex)USB interface 2 × USB 2.0Front panel: 1 × USB 2.0 Rear panel: 1 × USB 3.0Alarm in/out N/AGeneralPower supply12 VDC, 1.5 A12 VDC, 1.5 A12 VDC, 3.33 A Consumption(without HDD)≤ 6 W≤ 7.5 W≤ 25 WWorking temperature-10 °C to +55 °C (+14 °F to +131 °F)Working humidity10% to 90%Dimension (W × D × H)315 × 242 × 45 mm (12.4 × 9.5 × 1.8 inch)Weight (without HDD)≤ 1.16 kg (2.6 lb)≤ 1.78 kg (3.9 lb)≤ 2 kg (4.4 lb)Note:1: Enabling enhanced VCA mode will maximize the connectable channel number for perimeter protection (line crossing detection and intrusion detection), but disable 2K/4K HDMI output resolution and 4 MP signal input.2: Enhanced IP mode might be conflicted with smart events (perimeter protection) or other functions, please refer to the user manual for details.Physical Interface*: The rear panel of DS-7204/7208HQHI-K1 provides 4/8 video input interfaces.No.Description No.Description1Video and Coaxial Audio In7LAN Network Interface2AUDIO IN, RCA Connector8RS-485 Serial Interface3AUDIO OUT, RCA Connector9Power Input4VGA Interface10Power Switch5HDMI Interface11GND6USB Interface12VIDEO OUTAvailable ModelDS-7204HQHI-K1, DS-7208HQHI-K1, DS-7216HQHI-K104250002010224。
(完整版)视频会议厂家介绍
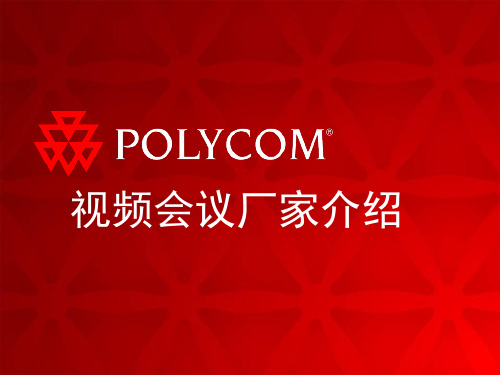
2024/2/21 | Polycom Confidential
SD卡接口 CVBS / RCA 3.5mm 耳机输出接口 PSTN FXO接口
2024/2/21 | Polycom Confidential
8
Page 8
LifeSize(丽视)
LifeSize公司介绍
公司成立于2003年,是全球最早推出高清视频通讯 产品的厂商。 LifeSize公司目前在全球共有3个办事 处,在中国没有常驻办事机构,所有产品在中国区的 总代理为慷泰丽视。
H.264 1080p、720p全高清/多画面/适配/AAC-LD宽频语音 支持H.320/H.323/SIP协议(与IMS无缝融合) 全备份电信级平台架构,高清/标清电视墙输出,多通道级联 IP/E1/4E1线路接入,支持1024路2M IP用户或256路E1用户 支持多点智真,支持智真会场、高清、标清的混合会议
2M 不支持 不支持
1M 选配4倍光学或数 字变焦 选配lifeSize Phone或MicPod 双显
Express
2M 不支持 不支持
1M 选配4倍光学或数 字变焦 标配lifeSize MicPod 单显
2024/2/21 | Polycom Confidential
19
TandBerg(腾博)
2024/2/21 | Polycom Confidential
7
桌面视讯终端产品
VP 8053 MC 850/851
FCC声明说明书

Federal Communications Commission (FCC) Statement (011898)This equipment has been tested and found to comply with the limits for a Class B digital device, pursuant to Part 15 of FCC Rules. These limits are designed to provide reasonable protection against harmful interference in a residential installation. This equipment generates, uses and can radiate radio frequency energy and, if not installed and used in accordance with instructions contained in this manual, may cause harmful interference to radio and television communications. However, there is no guarantee that interference will not occur in a particular installation.If this equipment does cause harmful interference to radio or television reception, which can be determined by turning the equipment off and on, the user is encouraged to try to correct the interference by one or more of the following measures:-REORIENT OR RELOCATE THE RECEIVING ANTENNA-INCREASE THE SEPARATION BETWEEN THE EQUIPMENT AND THE RECEIVER -CONNECT THE EQUIPMENT INTO AN OUTLET ON A CIRCUIT DIFFERENT FROM THAT OF THE RECEIVER-CONSULT THE DEALER OR AN EXPERIENCED AUDIO/TELEVISION TECHNICIANNOTE:Connecting this device to peripheral devices that do not comply with Class B requirements, or using an unshielded peripheral data cable, could also result in harmful interference to radio or television reception.The user is cautioned that any changes or modifications not expressly approved by the party responsible for compliance could void the user’s authority to operate this equipment.To ensure that the use of this product does not contribute to interference, it isnecessary to use shielded I/O cables.CopyrightThis manual is copyrighted with all rights reserved. No portion of this manual may be copied or reproduced by any means.While every precaution has been taken in the preparation of this manual, no responsibility for errors or omissions is assumed. Neither is any liability assumed for damages resulting from the use of the information contained herein.TrademarksAll brand names, logos and registered trademarks mentioned are property of their respective owners.Table of ContentsHARDWARE CONFIGURATION (3)Key Features (3)Motherboard Layout (5)Jumper Settings (6)CPU Speed Selection (6)SW1, SW2 - CPU Frequency Ratio (6)SW3 - VID[0:4] Code to Voltage Definition (7)J7 - CMOS Clear (8)J2 - On Board AC97 Codec Sound (8)Pin Assignment (9)HARDWARE SETUP (10)T o Install DIMMs (10)Installing a New Processor (11)To Install a Processor to ZIF Socket (11)Connect the processor Fan Connector (11)BIOS SETUP (12)Starting Setup (12)Main Menu (13)Standard CMOS Features (14)Advanced BIOS Features (16)Advanced Chipset Features (16)Integrated Peripherals (16)Power Management Setup (16)PnP/PCI Configurations (16)PC Health Status (16)Frequency/Voltage Control (16)Set Supervisor/User Password (16)Flash Update Procedure (18)APPENDIX (19)Select the Heatsink (19)Select the Power Supply (20)HARDWARE CONFIGURATIONKey Features:Chipset•VIA® KT133/KT133A Chipset.Processor•Full support for the AMD Duron TM and Athlon TM processors using Socket A.•Supports 100MHz & 133MHz (optional) bus speed including all processors using Socket A.VRM 9.0 (Voltage Regulator Modules) on Board•Flexible motherboard design with on board VRM 9.0.System Memory• A total of three 168-pin DIMM sockets (3.3V Synchronous DRAM).•Supports Synchronous DRAM PC100/PC133.•Memory size up to 1.5G bytes.•Supports SDRAM memory types.•Supports single-density DIMMs of 1MB, 2MB, 4MB, 8MB and 16MB depth (x64).•Supports double-density DIMMs of 2MB, 4MB, 8MB, 16MB and 32MB depth (x64).•Supports single & double sided DIMMs.•Banks of different DRAM types depths can be mixed.System BIOS•2MB flash BIOS supporting PnP, APM, ATAPI and Windows® 95.•Auto detects and supports LBA hard disks with capacities up to 8.4GB.•Full support of ACPI & DMI.•Easy to upgrade BIOS by end-user.Dual BIOS•As our dual BIOS use the awdflash ver.7.83 to flash the dual BIOS, so our dual BIOS support the flash type of the awdflash ver.7.83, such as Syncmos,ATMEL etc..•The normal boot sequence is from main BIOS. If one BIOS is fail to boot, you can press the ‘Reset’ button to boot from the other BIOS (Note: no every time issuccess, you can try it several times or touch button time is enough long, forexample 2~3 second).•If the BIOS error that contained in compressed part of BIOS bin file, our dual BIOS can know to automatic flash, but the boot block error can not know to flash, that is: If the BIOS bin file is damage, it will auto flash BIOS, and if the bin file is OKeven different with the board bin file, computer will boot normal without enter the flash screen). If the user want to flash the BIOS unconditionally, you can press the ‘Alt + F2’ key to flash another BIOS.On-board I/O•On board two PCI fast IDE ports supporting up to 4 ATA and Ultra DMA33/66/ 100 (optional) IDE devices.•Supports bus master IDE, PIO mode 4 (up to 16.6M bytes/sec) and Ultra DMA33/66/100 (optional) (up to 33/66/100M (optional) bytes/sec) transfer.•One ECP/EPP parallel port.•Two 16550-compatible UART serial ports.•One floppy port, supports two FDDs of 360KB, 720KB, 1.2MB, 1.44MB and 2.88MB capacity.•Four USB ports.•PS/2 mouse port.•PS/2 keyboard port.•Infrared (IrDA) is supported.•One Line/Speaker out, one Mic in, one Line in and MIDI/Game port (optional).Plug-and-Play•Supports plug-and-play specification 1.1.•Plug-and-play for DOS, Windows® 3.X, Windows® 95 as well as Windows® 98.•Fully steerable PCI interrupts.On-board AC97 Sound•Integrated AC97 controller with standard AC97 codec.•Direct Sound and Sound Blaster compatible.•Full-Duplex 16-bit record and playback.•PnP and APM 1.2 support.On-board Creative Sound (optional)•Creative ES1373 Audio controller.•128 voice wave table synthesizer.•DOS game compatibility.•PCI bus master for fast DMA.•Fully compliant with PC97 power management specification.(Note: If Creative PCI Sound is on board, only Primary AMR Card can be used. If Creative PCI Sound is enabled, Bus Master Device on one PCI cannot be used.)Power Management•Supports SMM, APM and ACPI.•Break switch for instant suspend/resume on system operations.•Energy star “Green PC” compliant .•WOL (Wake-On-Lan) Header support.•External Modem Ring-in Wake-up support.•Support auto setting or manual setting for CPU voltage.•Supports suspend-to-RAM (STR) (optional).Expansion Slots• 5 PCI bus master slots (Rev. 2.1 compliant).• 1 Audio Modem Riser (AMR) (optional).• 1 ISA slot (1 ISA slot share with 1 PCI slot).• 1 universal AGP slot (AGP 2.0 compliant - 4X mode support).CAUTIONStatic electricity can harm delicate components of the motherboard. To prevent damage caused by static electricity, discharge the static electricity from yourbody before you touch any of the computers electronic components.Motherboard LayoutThe following diagrams show the relative positions of the jumpers, connectors, major components and memory banks on the motherboard.# The AMR slot is optional.NOTE1)Be sure to check the cable orientation in order to match the colored strip tothe pin 1 end of the connector.2)When you start up the system, please wait for 5 seconds after you poweron AC.Jumper SettingsThis chapter explains how to configure the motherboard’s hardware. Before using your computer, make sure all jumpers and DRAM modules are set correctly. Refer to this chapter whenever in doubt.CPU Speed SelectionIn this motherboard, you can set the CPU speed by manual or auto way, but over clock isn’t recommended.SW1, SW2 - CPU Frequency RatioSW2[1:5]SW1[1:4] SW2[1:5] CPU FREQ1 2 3 4 5SW3 - VID[0:4] Code to Voltage DefinitionSW1(6) Off, Auto Setting SW1(6) On, Manual Setting。
索尼BDP-S5000ES蓝光播放器产品说明书

• I solated audio circuit board andhigh quality parts• R igid beam chassis and dual shieldconstruction• R-Core transformer• S upports Bonus View andBD-Live ™contentBDP-S5000ESBlu-ray Disc ™ PlayerProduct FeaturesExperience ultra-smooth , ultra-detailed picture qualitywith the Sony ® BDP-S5000ES Blu-ray Disc™ Player. Super BitMapping and the 14-bit HD video processor deliver anincredible Blu-ray cinema experience, while upscaling DVDsto full HD 1080p. Ideal for custom installs with a rigid framefor reduced vibration, an IR input and Control4 certification.Audio and Video• F ull HD 1080p/24p video output• 14-bit HD video processor• 192kHz/24-bit audio• DVD upscaling to 1080p over HDMI• A djustments for contrast, brightness,color, gamma and many morevideo options• Super Bit Mapping• D olby ® TrueHD ® and dts ®-HDdecoding/bitstream output with7.1 channels Inputs and Outputs • H DMI, component, composite, S-video outputs • 7.1 channnel analog outputs • RS232 and IR input • E thernet for firmware updates and BD-Live Control Features • I ntegrates with control systems via RS232 or IR • C ontrol4 certified/AMX Device Discovery Beacon/CrestronIntegration PartnerDimensions• 17" x 4.92" x 14.37"1. 1080p playback requires HDMI connector (sold separately) and compatible HDTV with 1080p display capability. 24p True Cinema requires 24p-capable HDTV.2. Broadband Internet connection and home network required. Fees may apply.3. Certain circumstances may limit/prevent Blu-ray Disc™ media playback. DVD Media/formats are not universally compatible.4. 1GB memory size. Storage capacity may varry.5. BRAVIA® Sync for Theatre works with select Sony televisions and home theater products, excluding camcorders. Please check your owner’s manual for compatibility.6. Only when displaying video from x.v.Color™ enabled video sources such as select Sony camcorders. ©2010 Sony Electronics Inc. All Rights Reserved. Reproduction in whole or in part without written permission is prohibited. Sony, like.no.other, BRAVIA, 24p True Cinema, Precision Drive, Super Bit Mapping and xross media bar are trademarks of Sony. Blu-ray Disc is a trademark. Bonus View is a trademark of Blu-ray Disc Association. Dolby, Dolby Digital, and ProLogic are registered trademarks of Dolby Laboratories. dts is a trademark of DTS, Inc. All other trademarks are properties of their respective owners. Features and specifications are subject to change without notice. Non-metric weights and measures are approximate.Specs。
NVIDIA Quadro P400 专业图形卡商品说明书
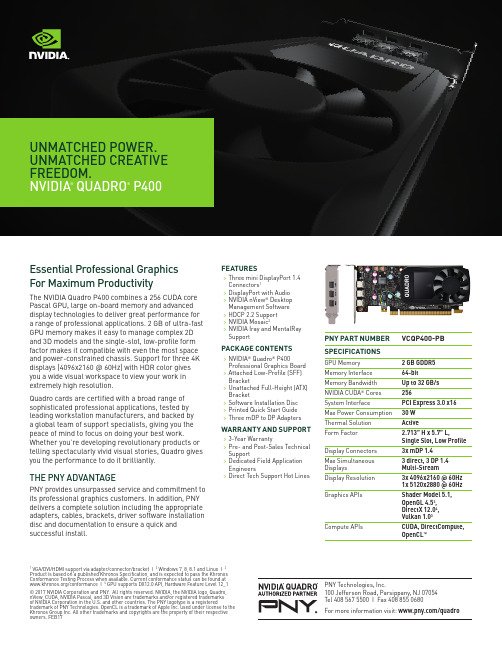
Essential Professional Graphics For Maximum ProductivityThe NVIDIA Quadro P400 combines a 256 CUDA core Pascal GPU, large on-board memory and advanced display technologies to deliver great performance for a range of professional applications. 2 GB of ultra-fast GPU memory makes it easy to manage complex 2D and 3D models and the single-slot, low-profile form factor makes it compatible with even the most space and power-constrained chassis. Support for three 4K displays (4096x2160 @ 60Hz) with HDR color gives you a wide visual workspace to view your work in extremely high resolution.Quadro cards are certified with a broad range of sophisticated professional applications, tested by leading workstation manufacturers, and backed by a global team of support specialists, giving you the peace of mind to focus on doing your best work. Whether you’re developing revolutionary products or telling spectacularly vivid visual stories, Quadro gives you the performance to do it brilliantly. THE PNY ADVANTAGE PNY provides unsurpassed service and commitment to its professional graphics customers. In addition, PNY delivers a complete solution including the appropriate adapters, cables, brackets, driver software installation disc and documentation to ensure a quick and successful install.FEATURES >Three mini DisplayPort 1.4 Connectors 1 >DisplayPort with Audio >NVIDIA nView ® Desktop Management Software >HDCP 2.2 Support >NVIDIA Mosaic 2 >NVIDIA Iray and MentalRay Support PACKAGE CONTENTS >NVIDIA ® Quadro ® P400 Professional Graphics Board >Attached Low-Profile (SFF) Bracket >Unattached Full-Height (ATX) Bracket >Software Installation Disc >Printed Quick Start Guide >Three mDP to DP Adapters WARRANTY AND SUPPORT >3-Year Warranty >Pre- and Post-Sales Technical Support >Dedicated Field Application Engineers >Direct Tech Support Hot Lines PNY PART NUMBER VCQP400-PB SPECIFICATIONS GPU Memory 2 GB GDDR5 Memory Interface 64-bit Memory Bandwidth Up to 32 GB/s NVIDIA CUDA ® Cores 256 System Interface PCI Express 3.0 x16 Max Power Consumption 30 W Thermal Solution Active Form Factor 2.713” H x 5.7” L, Single Slot, Low Profile Display Connectors3x mDP 1.4 Max Simultaneous Displays3 direct, 3 DP 1.4 Multi-Stream Display Resolution3x 4096x2160 @ 60Hz 1x 5120x2880 @ 60Hz Graphics APIs Shader Model 5.1, OpenGL 4.53, DirectX 12.04, Vulkan 1.03Compute APIsCUDA, DirectCompute, OpenCL ™ UNMATCHED POWER.UNMATCHED CREATIVE FREEDOM. NVIDIA ® QUADRO ®P4001 VGA/DVI/HDMI support via adapter/connector/bracket | 2 Windows 7, 8, 8.1 and Linux | 3 Product is based on a published Khronos Specification, and is expected to pass the Khronos Conformance Testing Process when available. Current conformance status can be found at /conformance | 4 GPU supports DX12.0 API, Hardware Feature Level 12_1© 2017 NVIDIA Corporation and PNY. All rights reserved. NVIDIA, the NVIDIA logo, Quadro, nView, CUDA, NVIDIA Pascal, and 3D Vision are trademarks and/or registered trademarks of NVIDIA Corporation in the U.S. and other countries. The PNY logotype is a registered trademark of PNY Technologies. OpenCL is a trademark of Apple Inc. used under license to the Khronos Group Inc. All other trademarks and copyrights are the property of their respective owners. FEB17PNY Technologies, Inc. 100 Jefferson Road, Parsippany, NJ 07054 Tel 408 567 5500 | Fax 408 855 0680For more information visit: /quadro。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
INSPIRING
Compatible with standards-based video without losing features Take advantage of the TANDBERG Total Solution Management Expanded HD Multipoint Recording & Streaming Ensuring the call can go through firewalls
POWERFUL
1080p* High Definition Full Duplex Audio with High Quality Stereo Sound High Definition MultiSiteTM 720p30 with individual transcoding HD Collaboration with UXGA* ready
Ultimate Performance
12X optical zoom with smooth, quiet handling Improved accuracy for pan & tilt HDMI and HD-SDI outputs with ability to output both simultaneously
HD-SDI allows camera to be placed up to 100m from the codec
Mount upside down—camera orientation automatically detected and picture flipped
pan speed: 100o/s, range: -90o to +90o, precision 0.3o | tilt speed 30o/s, range: -25o to +15o, precision: 0,15o
* ready, future version of software
flexible by design
More Ins and Outs
Full High Definition Video with up to 12 HD Sources Highest Quality Audio with flexibility to add up to 8 microphones directly from the codec Collaborate on anything with 5 simultaneous video inputs (image compositing) Professional Grade Connectors Full APIs
* ready, future version of software
TANDBERG PrecisionHD 1080p
The Ultimate Camera for Telepresence
Exquisite Picture
1080p60 True High Definition BestView (preview feature) auto adjust pan/tilt/zoom based on facial recognition technology Improved auto focus with multi-focus points Improved white balance with ambient light sensor
Video Outputs
Flexible for many high definition or analog devices…monitors, projectors, TVs, whatever you need. 2 HDMI 2 DVI-I 1 Composite
Audio
Expansive options with the highest quality audio and professional connectors. Audio Inputs 8 Balanced XLR 4 RCA 4 HDMI Audio Output 2 Balanced XLR 4 RCA 2 HDMI
flexible
More ins-and-outs then one box should really ever have
inspiring
The possibilities are endless…integrate into anything
powerful by intent
Ultimate Video and Audio
FLEXIBLE
Full High Definition Video with up to 12 HD sources Highest Quality Audio with flexibility to add up to 8 microphones on the interface Collaborate on anything with 5 simultaneous video inputs Professional grade connectors Full APIs
– Codec C90 – PrecisionHD 1080p camera – 2 X tabletop microphones
Availability:
First Order: October 2008 First Ship: Mid-Q4 2008
Codec C90 Product Offerings
TANDBERG Codec C90 and PrecisionHD 1080p
Date: June 2008
TANDBERG C90
The Telepresence Engine
Codec C90
Power the video revolution
powerful
The ultimate video and audio quality
Codec C90 Product Offering
What is the product offering? Codec C90 including rails for easy rack mounting, remote control, cables, documentation PrecisionHD 1080p Camera including power supply, lens hood, cables Integrator Package:
Codec C90
Includes: C90, Remote Control, Cables
Codec C90 Integration Package
Includes: C90, PrecisionHD 1080p Camera, Remote Control, 2 x mics, Cables
PrecisionHD 1080p Camera
Other
Options are practically limitless and use high quality professional connections Network 2 X Gigabit Ethernet Other 1 USB Host 1 USB Device 2 X RS-232 1 GPIO
inspiring for every application
Applications
Telepresence Studios Collaboration Studios Boardroom Auditorium / Education Telemedicine
Key Features & Benefits
Thank you
Power
12V DC 2.5 mm
Visca
Daisy Chain
Visca
Camera Control & Power
HDMI
Video Out
HD-SDI
Video Out
Ethernet
10/100Mb
PrecisionHDTM Camera Comparison
PrecisionHD 720p Resolution, Framerate Zoom Zoom speed Outputs Pan/Tilt Accuracy Wide view angle Tilt range 1280x720p30 7x 6 sec HDMI, Proprietary 1.35deg / 0.4deg 70deg 30deg (15/15) PrecisionHD 1080p 1920x1080p60 12x <3 sec HDMI, HD-SDI 0.4deg / 0.3deg 72deg 40deg (25/15)
Clearest, smoothest video up to 1080p Full Duplex Audio with High Quality Stereo Sound High Definition Collaboration with UXGA* ready High Definition MultiSiteTM 720p30 with individual transcoding
Codec C90
The Telepresence Engine
Video It video input possibilities on the market. Up to five different video inputs can be active simultaneously. 4 HDMI 2 DVI-I 4 HD-SDI 2 Analog Component 1 S-Video/Composite