河南理工大学C语言课后习题答案精解第六章
c语言第六章 答案(全)

6.1用筛选法求100以内的素数。
参考答案如下:# include “math.h”main(){ int i,j,a[101];for(i=1;i<=100;i++) a[i]=i; /*初始化数组*/a[1]=0; //1不是素数,所有将a[1]赋值为0for(i=2;i<=sqrt(100);i++) //循环2,3,4-----10if(a[i]!=0) //只要a[i]不是0,那么i就是素数,再把100以内该素数的倍数赋值为0 for(j=i+1;j<=100;j++) //因为给素数的倍数肯定不是素数if (a[j]!=0&&a[j]%i==0) a[j]=0;for(i=1;i<=100;i++) //输出所有素数,只要不是素数的值通过上面for语句赋值为0了if (a[i]!=0) printf(“%d ”,a[i]);//即只要a[i]不是0,那么i就是素数}算法思想:首先,将1,2,3---100存在数组a的a[1],a[2],a[3]----a[100]中,即计算时数组元素下标就表示对应的自然数。
然后从2,3到10中的素数,逐一将10以内的素数的倍数的元素置为0(对应的自然数就是合数)。
最后,将所有素数输出(对应的元素值不为0就是素数)。
6.2用选择法对10个数进行排序(从大到小)。
参考答案如下(降序排列):用逐个比较的方法进行。
*/#include <stdio.h>main(){ int a[10],i,j,k,x;printf("Input 10 numbers:\n");for(i=0;i<10;i++)scanf("%d",&a[i]);printf("\n");for(i=0;i<9;i++) //10个数要排9趟,每趟确定a[i],即从a[i]到a[n-1]找到最大的数// 用k记录其下标,然后a[k]和a[i]交换{ k=i; //假设当前无序序列第一个数为最大数for(j=i+1;j<10;j++) //从下一个数开始到最后去找//是否有更大的数if(a[j]<a[k]) k=j; //若有,则把下标记录在k变量中if(i!=k) //将找到的最大数k下标元素和当前的第一个元素i下标元素交换{ t=a[i]; a[i]=a[k]; a[k]=t; }}printf("The sorted numbers:\n");for(i=0;i<10;i++)printf("%d ",a[i]);}6.3 求一个3*3的整型矩阵对角线元素之和参考答案如下:(思考如果求N*N的整型矩阵对角线元素之和怎么修改程序)main(){ int a[3][3],i,j,sum;for(i=0;i<3;i++) //输入3行3列的矩阵{printf("\nInput three integar for %d line",i+1);for(j=0;j<3;j++)scanf("%d",&a[i][j]);}printf("\nThe array is:\n");for(i=0;i<3;i++) //输出3行3列的矩阵{ for(j=0;j<3;j++)printf("%5d",a[i][j]);printf("\n");}sum=0; //sum累加器,初值为0for(i=0;i<3;i++) //将对角线的元素值累加到sum中sum=sum+a[i][i];for(i=2;i>=0;i--) //将反对角线的元素值累加到sum中sum=sum+a[i][2-i];sum=sum-a[1][1]; //a[1][1]加了2次,所以减去一次printf("sum=%d",sum);}思考如果求N*N的整型矩阵对角线元素之和怎么修改程序?参考答案如下:#define N 5main(){ int a[N][N],i,j,sum;for(i=0;i<N;i++){printf("\nInput %d integar for %d line",N,i+1);for(j=0;j<N;j++)scanf("%d",&a[i][j]);}printf("\nThe array is:\n");for(i=0;i<N;i++){ for(j=0;j<N;j++)printf("%5d",a[i][j]);printf("\n");}sum=0;for(i=0;i<N;i++)sum=sum+a[i][i];for(i=N-1;i>=0;i--)sum=sum+a[i][N-1-i];if((N-1)%2==0) sum=sum-a[(N-1)/2][(N-1)/2]; //当N为偶数时,//没有叠加两次的中间对角线元素printf("sum=%d",sum);}6.4已有一个排好序的数组,要求输入一个数后,按原来的排序规律将它插入数组中。
C语言第6章习题解答

第6章函数和模块设计【习题6-1】更正下面函数中的错误。
(1)返回求x和y平方和的函数。
(2)返回求x和y为直角边的斜边的函数。
sum_of_sq(x,y) hypot(double x,double y){ {double x,y; h=sqrt(x*x+y*y);return(x*x+y*y); return(h);} }程序如下:/*c6_1(1).c*/ /*c6_1(2).c*/(1) (2)double sum_of_sq(double x,double y) double hypot(double x,double y) { {return(x*x+y*y); double h;} h=sqrt(x*x+y*y);return(h);}【习题6-2】说明下面函数的功能。
(1)itoa(int n,char s[ ])(2)int htod(char hex [ ]){ { int i,dec=0;static int i=0,j=0; for(i=0;hex[i]!='\0';i++)int c; { if(hex[i]>='0'&&hex[i]<='9') if(n!=0) dec=dec*16+hex[i]-'0';{ if(hex[i]>='A'&&hex[i]<='F') j++; dec=dec*16+hex[i]-'A'+10;c=n%10+'0'; if(hex[i]>='a'&&hex[i]<='f') itoa(n/10,s); dec=dec*16+hex[i]-'a'+10;s[i++]=c; }} return(dec);else }{ (3)void stod(int n)if(j==0) s[j++]='0'; { int i;s[j]='\0'; if(n<0){ putchar('-');n=-n;} i=j=0; if((i=n/10)!=0) stod(i);} putchar(n%10+'0');} }功能:(1)(略)(2)(略)【习题6-3】编写已知三角形三边求面积的函数,对于给定的3个量(正值),按两边之和大于第三边的规定,判别其能否构成三角形,若能构成三角形,输出对应的三角形面积。
c语言第六七章习题答案

scanf("%d",&a[i]);
for(i=0;i<N;i++)
{
s=1;
for(j=0;j<N-1-i;j++)
if(a[j]>a[j+1])
{
s=0;
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
if(s==1) break;/*无反序则跳出*/
}
for(i=0;i<N;i++)
printf("%d ",a[i]);
printf("\n");
}
6.3
#include <stdio.h>
#define N 10
gets(ch);
l=strlen(ch);
for(i=0;i<l;i++)
{
n=(long)(ch[i]-48);/*将字符形式的整数转换成整型*/
j=l-i-1;
while(j>0)/*整数每位乘以其位权*/
{
n*=10;
j--;
}
num+=n;
{
t=a[i];
a[i]=a[j];
a[j]=t;
}
i++;
j--;
}
for(k=0;k<N;k++)
printf("%d ",a[k]);
C语言第六章课后题详解
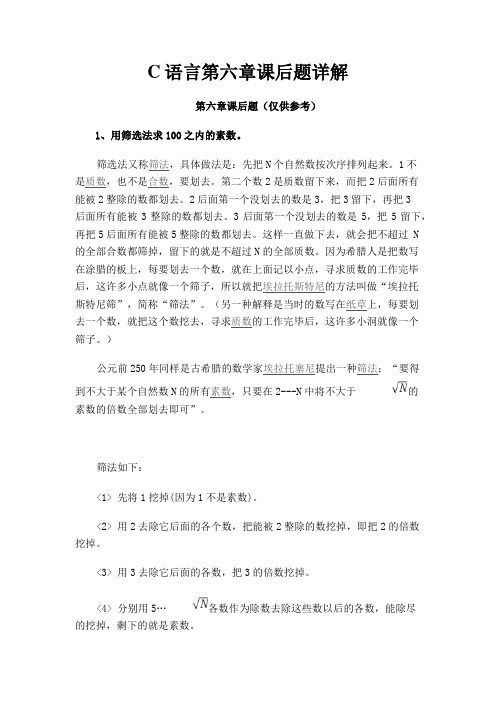
C语言第六章课后题详解第六章课后题(仅供参考)1、用筛选法求100之内的素数。
筛选法又称筛法,具体做法是:先把N个自然数按次序排列起来。
1不是质数,也不是合数,要划去。
第二个数2是质数留下来,而把2后面所有能被2整除的数都划去。
2后面第一个没划去的数是3,把3留下,再把3后面所有能被3整除的数都划去。
3后面第一个没划去的数是5,把5留下,再把5后面所有能被5整除的数都划去。
这样一直做下去,就会把不超过 N的全部合数都筛掉,留下的就是不超过N的全部质数。
因为希腊人是把数写在涂腊的板上,每要划去一个数,就在上面记以小点,寻求质数的工作完毕后,这许多小点就像一个筛子,所以就把埃拉托斯特尼的方法叫做“埃拉托斯特尼筛”,简称“筛法”。
(另一种解释是当时的数写在纸草上,每要划去一个数,就把这个数挖去,寻求质数的工作完毕后,这许多小洞就像一个筛子。
)公元前250年同样是古希腊的数学家埃拉托塞尼提出一种筛法:“要得到不大于某个自然数N的所有素数,只要在2---N中将不大于的素数的倍数全部划去即可”。
筛法如下:<1> 先将1挖掉(因为1不是素数)。
<2> 用2去除它后面的各个数,把能被2整除的数挖掉,即把2的倍数挖掉。
<3> 用3去除它后面的各数,把3的倍数挖掉。
<4> 分别用5…各数作为除数去除这些数以后的各数,能除尽的挖掉,剩下的就是素数。
算法如下:<1> 用数组a[1]……a[100]分别存放1~100各数,先将a[1]挖掉(因为1不是素数),我们可以把挖的数组元素统一置为0,既a[1]=0。
<2> 设第一个除数i=2,<3> 令被除数j为i+1<4> 若a[j]不等于0,用i去除a[j],若能整除则令a[j]=0。
即把a[i]的倍数挖掉。
<4> 下一个被除数,既j=j+1,若j≤100,回到第<4>步。
C语言第6章习题答案
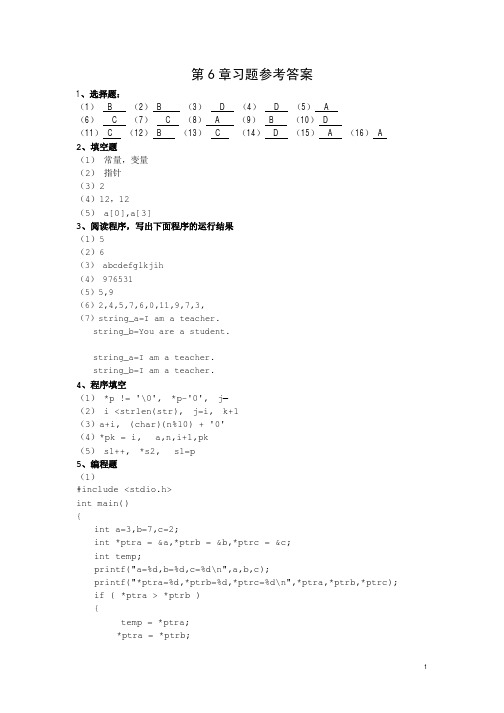
int a[10],n=10; inputdata(a,n); outputdata(a,n); process(a,n); outputdata(a,n); system("Pause"); return 0; } void inputdata(int a[],int n) //0~99 之间的数 {
string_b=You are a student.
(5) A (10) D (15) A
(16) A
string_a=I am a teacher. string_b=I am a teacher. 4、程序填空 (1) *p != '\0', *p-'0', j— (2) i <strlen(str), j=i, k+1 (3)a+i, (char)(n%10) + '0' (4)*pk = i, a,n,i+1,pk (5) s1++, *s2, s1=p 5、编程题 (1) #include <stdio.h> int main() { int a=3,b=7,c=2; int *ptra = &a,*ptrb = &b,*ptrc = &c; int temp; printf("a=%d,b=%d,c=%d\n",a,b,c); printf("*ptra=%d,*ptrb=%d,*ptrc=%d\n",*ptra,*ptrb,*ptrc); if ( *ptra > *ptrb ) {
C语言第六章习题答案
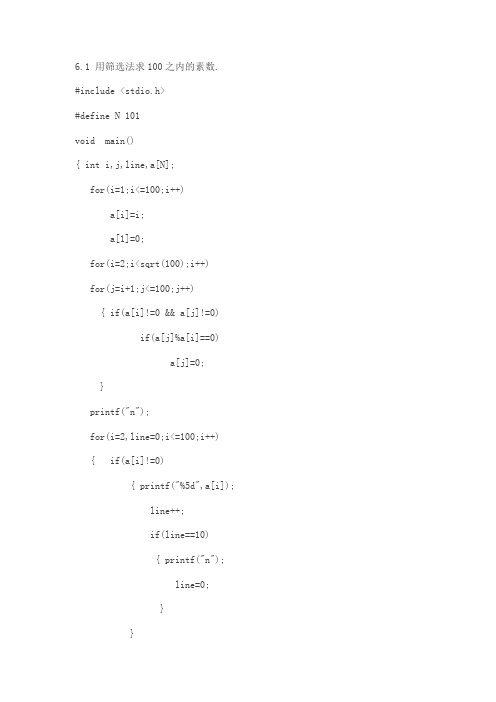
6.1 用筛选法求100之内的素数. #include <stdio.h>#define N 101void main(){ int i,j,line,a[N];for(i=1;i<=100;i++)a[i]=i;a[1]=0;for(i=2;i<sqrt(100);i++)for(j=i+1;j<=100;j++){ if(a[i]!=0 && a[j]!=0) if(a[j]%a[i]==0)a[j]=0;}printf("n");for(i=2,line=0;i<=100;i++) { if(a[i]!=0){ printf("%5d",a[i]); line++;if(line==10){ printf("n");line=0;}}}}6.2 用选择法对10个数排序.#define N 10main(){int i,j,min,temp,a[N];printf("请输入十个数:n"); for (i=0;i<=10;i++){ printf("a[%d]=",i);scanf("%d",&a[i]);}printf("n");for(i=1;i<=10;i++)printf("%5d",a[i]);printf("n");for (i=1;i<=9;i++){ min=i;for(j=i+1;j<=10;j++)if(a[min]>a[j]) min=j; temp=a[i];a[i]=a[min];a[min]=temp;}printf("n排序结果如下:n");for(i=0;i<N;i++)printf("%5d",a[i]);}6.3 对角线和:main(){float a[3][3],sum=0;int i,j;printf("请输入矩阵元素:n");for(i=0;i<3;i++)for(j=0;j<3;j++)scanf("%f",&a[i][j]);for(i=0;i<3;i++)sum=sum+a[i][i];printf("对角元素之和= 6.2f",sum); }6.4 插入数据到数组{ int a[11]={1,4,6,9,13,16,19,28,40,100}; int temp1,temp2,number,end,i,j;printf("初始数组如下:");for (i=0;i<10;i++)printf("%5d",a[i]);printf("n");printf("输入插入数据:");scanf("%d",&number);end=a[9];if(number>end)a[10]=number;else{ for(i=0;i<10;i++){ if(a[i]>number){ temp1=a[i];a[i]=number;}for(j=i+1;j<11;j++){ temp2=a[j];a[j]=temp1;temp1=temp2;}break;}}for(i=0;j<11;i++)printf("a%6d",a[i]);}6.5 将一个数组逆序存放。
c语言教学资料—第6章课后答案.docx
第6章练习与思考1.指针变量有哪几种运算类型?除了一般变量能做的它都能做以外,还有取地址和取内容运算。
2.有一个4x4的矩阵,试用指针变量按照4行4列格式,输岀矩阵中各元素的值。
#include "stdio.h"void main(){int 询4][4]={{1,1丄1},{1丄1,1},{1丄1,1},{1,1,1,1}};int *p=a;for(i=0;i<4*4;i++){ printf(n%3d",*(p+i));if((i+l)%4=0)printf(H\n H);}}3•输出一个字符串,将这个字符串屮大写字母改为小写字母,第一个字母变大写。
#include "stdio.h"void main(){char a[100],*p 二NULL;int i;printf(n Please input a string:\n H);gets(a);P=a;if(*p>=,a,&&*p<=,z,)*p=*p・32;for(i=l;a[i]!='\O f;i++){if(*(p+i)>='A '&&*(p+i)v=Z) *(p+i)=*(p+i)+32;}puts(a);}4.指向函数的指针有哪些特点?(1)可以通过调用指向函数的指针来调用函数。
(2)对于已定义的函数指针可以指向返回值类型相同的不同函数。
(3)函数指针可做函数的参数。
5.编写程序,在己知字符串str中截取一子串。
要求该子串是从血的第m个字符开始, 由n个字符组成。
#include "stdio.h"void main()char str[100],s[100];char *pl=str,*p2=s;int i,m,n;printf(n please input string:\n");scanf(n%s n,str);printf(”please input m,n:\n n);scanf("%d,%d",&m,&n);for(i=0;i<n;i++)*(p2+i)=*(pl+m+i);for(i=0;*(p2+i)U\0:i++) prin(f(”%c”,*(p2+i));}6.编写程序,利用指针将二维数组屮各元素输出。
大学C语言课本课后习题相应答案及详细解答
5-1#include"stdio.h" void main(){char c1,c2;printf("c1:");c1=getchar();if(c1>='a'&&c1<='z') c2=c1-32;else if(c1>='A'&&c1<='Z') c2=c1+32;else c2=c1;printf("=>%c\n",c2);}5-2#include"stdio.h"void main(){char c;printf("c:");c=getchar();if(c>='A'&&c<='Z')if(c=='A') printf("没有前面的字母!");else if(c=='Z') printf("没有后面的字母!!");else printf("前面的字母=%c,后面的字母=%c",c-1,c+1);printf("\n");} 5-3#include"stdio.h"void main(){int s;char g;printf("s:");scanf("%d",&s);if(s>=0&&s<=100){switch(s/10){case 9:case 10: g='A';break;case 8: g='B';break;case 7: g='C';break;case 6: g='D';break;default: g='E';}printf("g=%c\n",g);}else printf("成绩不在百分制范围内!\n"); }5-4#include"stdio.h"void main(){int y,m,d,dok;int yy,mm,dd;printf("y,m,d:");scanf("%d,%d,%d",&y,&m,&d);switch(m){case 1: case 3: case 5: case 7: case 8: case 10: case 12:if(d>0&&d<=31) dok=4;else dok=0;break;case 4: case 6: case 9: case 11:if(d>0&&d<=30) dok=3;else dok=0;break;case 2: if(y%4==0&&y%100!=0||y%400==0)if(d>0&&d<=29)dok=2;else dok=0;elseif(d>0&&d<=28)dok=1;else dok=0;break;default: dok=0;}if(dok==0) printf("月份或日期不对!\n");else{switch(dok){case 1: if(d==28){yy=y;dd=1;mm=m+1;}else{yy=y;dd=d+1;mm=m;}break;case2:if(d==29){yy=y;dd=1;mm=m+1;}else{yy=y;dd=d+1;mm=m;}break;case3:if(d==30){yy=y;dd=1;mm=m+1;}else{yy=y;dd=d+1;mm=m;}break;case 4:if(d==31)if(m==12){yy=y+1;dd=1;mm=1;}else{yy=y;dd=1;mm=m+1;}else{yy=y;dd=d+1;mm=m;}break;}printf("Tomorrow:%d年%d月%d日\n",yy,mm,dd);}}5-5#include"stdio.h"void main(){int a,b,c,t;printf("a,b,c:");scanf("%d,%d,%d",&a,&b,&c);if(a+b>c&&b+c>a&&a+c>b){if(a>b){t=a;a=b;b=t;}if(a>c){t=a;a=c;c=t;}if(b>c){t=b;b=c;c=t;}if(a==b&&b==c) printf("等边三角形.");else if(a==b||b==c||a==c) printf("等腰角形.");else if(c*c==a*a+b*b) printf("直角三角形.");else printf("任意三角形.");printf("\n");}else printf("不能构成三角形!\n");}6-1#include"stdio.h"void main(){int a,b,m,n,k;printf("m,n:");scanf("%d,%d",&m,&n);a=m;b=n;k=a%b;while(k){a=b;b=k;k=a%b;}printf("%d\n",(m*n/b));}6-2#include"stdio.h"void main(){int n;int i,j,s;printf("n=");scanf("%d",&n);for(i=1;i<=n;i++){s=0;for(j=1;j<i;j++)if(i%j==0) s=s+j;if(s==i) {printf("%d:",i);for(j=1;j<i;j++)if(i%j==0) printf("%d ",j);printf("\n");}}} 6-3#include"stdio.h"#include"math.h"void main(){double x,y,zd,zx,x1,x2;zd=zx=50;for(x=0;x<=2;x=x+0.2){y=2*pow(x,3)-3*pow(x,4)+6*pow(x,5)-4*x+50;if(y>zd){ zd=y;x1=x;}if(y<zx){ zx=y;x2=x;}}printf("x=%f,max=%f\n",x1,zd);printf("x=%f,min=%f\n",x2,zx);}6-4#include"stdio.h"void main(){double x,sum=0,a,b,c;int i;printf("x=");scanf("%lf",&x);a=x;b=1.0;c=1.0;for(i=1;i<=10;i++){sum=sum+a/(b*c);a=a*x*x;b=b+2;c=c*i;}printf("y(%.2lf)=%lf\n",x,sum);}7-1/*选择排序*/#include"stdio.h"void main(){int i,j,n,k,temp;int a[10];printf("n(<10):");scanf("%d",&n);printf("Original:");for(i=0;i<n;i++) scanf("%d",&a[i]);for(i=0;i<n-1;i++){ /*趟*/k=i;for(j=i+1;j<n;j++) /*比较次数*/if(a[j]<a[k]) k=j;/*<升序,>?*/if(k!=i){temp=a[i];a[i]=a[k];a[k]=temp;}}printf("Ordered:");for(i=0;i<n;i++) printf("%d ",a[i]);printf("\n");}7-2#include"stdio.h"void main(){int a[3][3];int i,j,s=1;printf("Input:\n");for(i=0;i<3;i++)for(j=0;j<3;j++){scanf("%d",&a[i][j]);if(i==j) s=s*a[i][j];}printf("s=%d\n",s);} 7-3/*杨辉三角*/#include"stdio.h"void main(){int x[7][7];int i,j;for(i=0;i<7;i++) {x[i][0]=1;x[i][i]=1;}for(i=2;i<7;i++)for(j=1;j<i;j++)x[i][j]=x[i-1][j]+x[i-1][j-1];for(i=0;i<7;i++){for(j=0;j<=i;j++)printf("%3d",x[i][j]);printf("\n");}}7-4#include<stdio.h>#include<string.h>void main(){char str[21];int i,j;printf("str:");gets(str);for(i=0,j=strlen(str)-1;i<=j;i++,j--)if(str[i]!=str[j]) break;if(i>j) printf("%s是回文\n",str);else printf("%s不是回文\n",str);}7-5/*输入一维数组的10个元素,并将最小值与第1个数交换,最大值与最后一个数交换,然后输出交换后的结果*/#include<stdio.h>void main(){int a[10],i,zx,zd;printf("Input:\n");zx=zd=0;for(i=0;i<10;i++){scanf("%d",&a[i]);if(a[i]<a[zx]) zx=i;if(a[i]>a[zd]) zd=i;}if(zx!=0){int t;t=a[0];a[0]=a[zx];a[zx]=t;}if(zd!=9){int t;t=a[9];a[9]=a[zd];a[zd]=t;}for(i=0;i<10;i++)printf("%d ",a[i]);printf("\n");} 8-2#include"stdio.h"double xexp(double x,int n){double c;if(n==0) c=1.0;else c=x*xexp(x,n-1);return c;}void main(){int n;double x;printf("x:");scanf("%lf",&x);printf("n:");scanf("%d",&n);printf("Result=%g\n",xexp(x,n));}8-3#include"stdio.h"int find(int x[],int n,int y){int i,pos=-1;for(i=0;i<n;i++)if(x[i]==y) pos=i;return pos;}void main(){int a[10]={11,1,13,15,18,7,19,27,3,8};int i,y,pos;for(i=0;i<10;i++) printf("%d ",a[i]);printf("\ny:");scanf("%d",&y);pos=find(a,10,y);if(pos==-1) printf("Not found!\n");else printf("Position=%d\n",pos);}8-1#include"stdio.h"#include"conio.h" //getch()#include"stdlib.h" //srand(),rand(),system("cls") #include"time.h" //time()void main(){void init(int a[][5],int m,int n);void input(int a[][5],int m,int n);void output(int a[][5],int m,int n);int min(int b[][5],int m,int n);int a[5][5],ch='0';while(1){system("cls"); //清屏printf("1.初始化 2.键盘输入0.结束程序:");printf("\n");if(ch=='0') break;else if(ch=='1'){init(a,5,5);output(a,5,5);}else if(ch=='2'){input(a,5,5);output(a,5,5);}else printf("Error!\n");printf("Min element:%d\n",min(a,5,5));printf("按任意键继续!\n");getch();}}void init(int a[][5],int m,int n){int i,j;srand(time(0)); //time(0)表示以当前的时间做种子,增加每次运行的随机性for(i=0;i<5;i++)for(j=0;j<5;j++)a[i][j]=rand()%100; //随机数范围:0~32767,将它控制在0~99的范围}void input(int a[][5],int m,int n){int i,j;printf("Input Array 5X5:\n");for(i=0;i<m;i++)for(j=0;j<n;j++)scanf("%d",&a[i][j]);}void output(int a[][5],int m,int n){int i,j;printf("Output Array 5X5:\n");for(i=0;i<5;i++){for(j=0;j<5;j++)printf("%2d ",a[i][j]);printf("\n");}}int min(int b[][5],int m,int n){int i,j,zx;zx=b[0][0];for(i=0;i<m;i++)for(j=0;j<n;j++)if(i==j&&b[i][j]<zx) zx=b[i][j];return zx;}8-4#include"stdio.h"float pave,nave;void saver(float a[],int n){int i,z,f;float psum,nsum;psum=nsum=0.0;z=f=0;for(i=0;i<n;i++)if(a[i]<0) {nsum=nsum+a[i];z++;}else if(a[i]>0) {psum=psum+a[i];f++;}else continue;pave=(z!=0?psum/z:0.0);nave=(f!=0?nsum/f:0.0);}void main(){float a[10]={1.0,11.0,3.0,-1.5,-5.5,-2};saver(a,10);printf("pave=%.1f,nave=%.2f\n",pave,nave); } 8-5#include"stdio.h"#include"math.h"void p1(int a,int b){printf("has two equal roots:%\n",-b/(2*a));}void p2(int a,int b, int disc){float x1,x2;x1=(-b+sqrt(disc))/(2*a);x2=(-b-sqrt(disc))/(2*a);printf("Has distant real roots:% and %\n",x1,x2);}void p3(int a,int b, int disc){float real,image;real=-b/(2*a);image=sqrt(-disc)/(2*a);printf("Has complex roots:\n");printf("%+%8.4fi\n",real,image);printf("%-%8.4fi\n",real,image);}void main(){ int a,b,c,disc;printf("a,b,c:");scanf("%d,%d,%d",&a,&b,&c);disc=b*b-4*a*c;if(fabs(disc)<=1e-6) p1(a,b);else if(disc>1e-6) p2(a,b,disc);else p3(a,b,disc);}8-6#include"stdio.h"#include"stdlib.h"#include"conio.h"#include"time.h"void main(){void printaverage(int score[][5],int m,int n);void printname(int score[][5],int m,int n);int score[10][5];int i,j;srand(time(0));for(i=0;i<10;i++)for(j=0;j<5;j++)score[i][j]=50+rand()%50+1;printf("Output Students' score:\n");printf("Course 1 2 3 4 5\n");printf("-------------------------\n");for(i=0;i<10;i++){printf("No.%2d:",i+1);for(j=0;j<5;j++)printf("%3d ",score[i][j]);printf("\n");}printaverage(score,10,5);printname(score,10,5);} void printaverage(int score[][5],int m,int n){int i,j,sum;printf("\nAverage score:\n");for(i=0;i<m;i++){sum=0;for(j=0;j<n;j++)sum=sum+score[i][j];printf("No.%d:%.1f\n",i+1,sum/5.0);}}void printname(int score[][5],int m,int n){int i,j,max0,row0;for(j=0;j<5;j++){max0=score[0][j];for(i=0;i<10;i++)if(score[i][j]>max0){max0=score[i][j];row0=i;}printf("Course %d,max score=%d,student:No.%d\n",j+1,max0,row0+1);}}大学英语I词汇练习题Choose the best one to complete each sentence.1. She felt like ____C___ frustration, but she was determined not to lose her self-control.A. to cry out ofB. to cry forC. crying out ofD. crying for2. The method he used turned out to be ___A____ in improving the students' English.A. effectiveB. abilityC. responseD. explicit3. Measures had to be taken in face of the housing problem that ____C____ in the city.A. foundedB. raisedC. aroseD. produced4. Without electronic computers, much of today's advanced technology ___D____.A. haven't been achievedB. wouldn't be achievedC. hadn't been achievedD. wouldn't have been achieved5. Jane said to her husband, "Don't worry. There is no cause for __B______ about our daughter'sability to manage herself."A. careB. concernC. attentionD. love6. The tap won't ____A____, and there is water all over the floor.A. turn offB. turn downC. turn onD. turn up7. Wearing the right shoes and clothes ____B____ being fit can make all the difference.A. in additionB. as well asC. alsoD. too8. She didn't try to do anything for her daughter, and ____B____ it's too late now.A. in caseB. in any caseC. at this caseD. in case of9. The girl said she hated _____C___ he smiled at her.A. that wayB. this wayC. the wayD. all the way10. I'll call the hotel. I'll tell them we'll ____A____ tomorrow morning and stay there for two nights.A. check inB. check outC. check offD. check over11. My father didn't go to New York; the doctor suggested that he ___C____ there.A. not to goB. won'tC. not goD. not to go to12. She _____C____ him to find answers to her problems.A. learned fromB. came intoC. leaned onD. subjected to13. The large wings of that bird ______B_____ it to fly high and fast.A. makeB. enableC. forceD. realize14. If he ____D___ the policeman honestly, he would not have been arrested.A. would answerB. answerC. should answerD. had answered15. I intended ___A____ you last Sunday, but I had no time.A. to have called onB. calling onC. to be calling onD. to be called on16. If you try to learn too many things at a time, you may get ____D___.A. alarmedB. scaredC. surprisedD. confused17. I haven't read this book, and my brother hasn't ____A____.A. eitherB. neitherC. alsoD. too18. No sooner ____B_____ than the truck started off.A. his luggage was loadedB. had his luggage been loadedC. loaded his luggageD. his luggage was being loaded19. At the end of the game, the whole crowd ____C____ their feet and cheered wildly.A. emerged fromB. rose fromC. got toD. stood up20. Darren has decided to give ___D_____ football at the end of this season.A. inB. forC. offD. up21. Neither his friends nor his mother ____B____ his marriage to that girl.A. acceptB. acceptsC. agreeD. agrees22. Most people believe that he is quite _____A___ of lying to get out of trouble.A. capableB. enabledC. ableD. skilled23. We were told that most of our luggage would be _____B___ by sea.A. approachedB. transportedC. handledD. communicated24. The Broadcasting Museum also offers Saturday workshops to _____B___ children with the world of radio.A. contriveB. acquaintC. acquireD. admit25. Without a passport, leaving the country is ____D___.A. out of questionB. without questionC. in the questionD. out of the question26. She states her views very ___A______.A. definitelyB. definiteC. infiniteD. infinitely27. You need to ____D____ the employers that you can do the job.A. convictB. confuseC. confirmD. convince28.The little boy was so lucky to have ___B_____ the earthquake.A. surviveB. survived C .surviving D. survival29. It is ___B___ that later in life you will see each other again.A. likeB. likelyC. likedD. seemed30.Women’s social ____B____ has changed much over the years.A. stateB. statusC. statesD. statement31.The novel _____D____ the imagination of thousands of readers.A. caterB. cateredC. captureD. captured32.There are good chances of ______D______ working in this firm.A. prospectB. portionC. positionD. promotion33.If you are ______A_____ a night owl, having the first class start in the afternoon might be thebest thing that could ever happen to you.A. more ofB. more thanC. much moreD. more34. The conference discussed the fair ______A_____of income and wealth.A. distributionB. distributeC. contributionD. contribute35. I was so ___C__ in today's history lesson that I didn't understand anything.A. amazedB. confusingC. confusedD. amazing36. ___B___ in England, Anne Bradstreet both admired and imitated several English poets.A. Having born and educatedB. Born and educatedC. Since born and educatedD. To be born and educated37. You will see this product ___B___ wherever you go.A. to be advertisedB. advertisedC. advertiseD. advertising38. I never forget my __B__ with you for the first time.A. to meetB. meetingC. to have metD. having to be meeting39.She was so angry that she left like __B__ something at him.A. to throwB. throwingC. to have thrownD. having thrown40. We are ___C__ in different kinds of extracurricular activities on campus.A. having involvedB. to involveC. involvedD. involving41.Let me assure you that it was not done ______D_____.A. intensiveB. intensivelyC. intentionalD. intentionally42.This medicine can ____A____ the pain in no time.A. lessenB. lessC. looseD. loosen43.Advertising is often the most ____A____ method of product promotion.A. effectiveB. effectedC. emotionalD. efficient44.It is at 5 a.m. _C___ the train from Beijing to Guangzhou will arrive.A. forB. withC. thatD. when45.Things might have been much worse if the mother _B___ on her right to keep the baby.A. has been insistingB. had insistedC. would insistD. insisted46.Had he worked harder, he __B__ the exams.A. must have got throughB. would have got throughC. permitted are freshmenD. are permitted freshmen47.God knows, how can I ____A____ six exams a week?.A. endureB. tolerateC. bear D .suffer48.All their attempts ______C______ the child from the burning building were in vain.A. rescuedB. to have rescueC. to rescueD. in rescuing49,Could you find someone ______C_______ for me to play tennis with?A. to fitB. fitC. fittingD. suit50. The smooth space flight marks a big ______D______ for Chinese scientists.A. breakB. breakingC. breakupD. breakthrough51 Economic crises destroy the capitalist system, and they grow in size and ____A____.A. durationB. duringC. endureD. enduringpanies will have to do more than this if they are to ___B_____ the earthquake.A. survivedB. surviveC. survivesD. surviving53. The baby felt ____C____ by her parents.A. hateB. loveC. ignoredD. ignore54. There are good chances of ______D______ in this firm.A. moveB. motiveC. motionD. promotion55. She tried to ______D______ our friendship.A. underminedB. keptC. maintainedD. undermine56. The new airport will ______C___ tourism.A. helpedB. useC. facilitateD. benefited57. They were full of ____B____ when they saw my new car.A. jealousB. envyC. pitifulD. loves58. This medicine can ____A____ the pain in no time.A. lessenB. lessC. leastD. little59.He _______A_____ her on her last physics paper.A. complimentedB. complimentC. completeD. completed60. Mrs. Hill is _______B____ on Tom’s marrying Susan.A. awareB. keenC. kindD. keep61. Let me assure you that it was not done ______C_____.A. internationalB. purposeC. intentionallyD. intentional62.The novel _____D_____ the imagination of thousands of readers.A. seizeB. catchC. captureD. captured63. If you yell ___A____ someone, you should apologize.A. atB. outC. ofD. under64. He came over and ____B____ a piece of paper into my hand.A. shoveB. shovedC. shovesD. shoving65. The girl student whose father is a senior engineer used to ___A____ abroad.A. studyB. studyingC. studiesD. studied66.The doctor tried to help ____D____ the function of his limbs.A. storeB. storesC. restoresD. restore67. Women’s social ____B____ has changed much over the years.A. placeB. statusC. landD. work68. It was he ___A___ knew he didn’t need to be afraid to make mistakes at that moment.A. whoB. whomC. whichD. whose69. A dropped cigarette is being ____C____ for the fire.A. firedB. fireC. blamedD. blame70. Advertising is often the most _____D___ method of promotion.A. effectB. affectC. affectedD. effective71.In time you’ll _____A_____ the beauty of this language.A. appreciateB. appreciatesC. appreciatedD. appreciating72. ______B___ is his favorite toy is hard for the kid to decide.A. whoB. whichC. howD. where73. After dinner, I took a ____A____ around the park.A. strollB. divingC. runD. walking74. The boy really enjoyed the useful and ____B____ work in science.A. creationB. creativeC. createdD. creator75. Some professions are _____A_______ with people who have not grown up.A. stuffedB. stuffC. stiffD. stiffed76. Economic crises destroy the capitalist system, and they grow in size and ____A____.A. durationB. duringC. endureD. enduring77. The new airport will ____C_____ tourism.A. helpedB. useC. facilitateD. benifited78. Let me assure you that it was not done _____C______.A. internationalB. purposeC. intentionallyD. intentional79. The girl student whose father is a senior engineer used to ___A____ abroad.A. studyB. studyingC. studiesD. studied80. Advertising is often the most ____D____ method of promotion.A. effectB. affectC. affectedD. effective81. It was he ___A___ knew he didn’t need to be afraid to make mistakes at that moment.A. whoB. whomC. whichD. whosepanies will have to do more than this if they are to _____B___ the earthquake.A. survivedB. surviveC. survivesD. surviving83. The baby felt ____C____ by her parents.A. hateB. loveC. ignoredD. ignore84. If you yell ___A____ someone, you should apologize.A. atB. outC. ofD. under85. Mrs. Hill is ______B_____ on Tom’s marrying Susan.A. awareB. keenC. kindD. keep86. _____A____ college takes hard work!A. SurvivingB. SurviveC. SurvivesD. To surviving87. It is absolutely ____A___ for the human beings to do something to save the earth.A. necessaryB. necessarilyC. unnecessaryD. unnecessarily88. An employer is more ____D____ to choose the candidate with high grades.A. likeB. probablyC. possiblyD. likely89. I don’t like this young woman. She seems a social ___D____.A. flowerB. workerC. negotiatorD. butterfly90. How should this ___B____ girl see the coldness of the letter?A. blindB. innocentC. warmD. pretty91. Be careful not to ___B____ yourself in their local conflicts.A. attendB. involveC. take part inD. participate92. Home prices are holding ____C___ in most regions, and people live with steady income.A. flexibleB. fierceC. stableD. shortage93. He was ______C______ by a newspaper reporter at that time.A. being interviewB. interviewC. being interviewedD. to interview94. They were full of ____C____ when they saw my new car.A. enviousB. envilyC. envyD. envies95. ________C_________, it is a very stable pattern producing results through effort .A. ContraryB. ConverseC. On the contraryD. Diverse96. The teacher doesn’t permit _____B____ in classA. smokeB. smokingC. to smokeD. to have a smoke97. The man in the corner confessed to ___A____ a lie to the manager of the company.A. have toldB. be toldC. being toldD. having told98. I never forget __B____ you for the first time.A. to meetB. meetingC. to have metD. having to be meeting.99. _______A___ a man has, ___________ he wants.A. The more, the moreB. More, moreC. The more, moreD. More, the more100. I see no _____B____ of his success.A. possibleB. possibilityC. possibilitiesD. probable101. He could be ____B____ about everything else in the world, but not about Manet, his loving child.A. visualB. criticalC. favoriteD. essential102. Some see themselves as the provider of ideas, ____C____ others view their role as essentially managerial.A. whenB. thereforeC. whileD. otherwise103. The idea ____A____ to him so much that he took it without hesitation.A. appealedB. interestedC. drewD. attracted104. The teacher evaluated the performance of each of his students who ____B____ evaluated his performance.A. by turnB. in turnC. at turnD. on turn105. Disease _____A____ during the journey and many passengers had to be rushed to hospital for treatment.A. broke outB. broke downC. started offD. started out106. The products have been _____A_____ to strict tests before leaving the factory.A. subjectedB. adjustedC. objectedD. constricted107. Our television set is out of order. Could you come and _____C_____ it for us?A. see throughB. see outC. see toD. see over108. _____C_____ they have taken matters into their hands, the pace of events has quickened.A. As thatB. So thatC. Now thatD. For that109. Our neighbor said if we made more noise he would _____B____ us to the police.A. inform ofB. complain aboutC. report toD. care for110. People working in the government should not _____A______ business affairs that might affect their political decisions.A. engage inB. hope forC. choose betweenD. pick on111. It's not easy to ______B______ to the top in show business.A. make outB. make itC. make upD. make them112. Carter is in charge of the office while I'm ______C______.A. leavingB. on leavingC. on leaveD. on relief113. We must ____B____ our attention on the question of reducing our cost.A. payB. focusC. absorbD. promote 114. Snap judgments, if ___B_____, have usually been considered signs of immaturity or lack of common sense.A. taking seriouslyB. taken seriouslyC. take seriouslyD. to be taken seriously115. Being with his family for a few days, I gained one or two insights ____A____ the reason he behaves the way he does.A. intoB. ofC. onD. off116. ___D___ your age or knowledge of the language, you'll be 100% involved in your studies from the first lesson to the last.A. As a result ofB. In spiteC. Despite ofD. Regardless of117. He said he had caught a bad cold and told me to ____D____ him.A. stay atB. stay upC. stay inD. stay away from118. Good managing of a company ___A_____ great efforts.A. calls forB. calls outC. calls inD. calls at119. I've looked ___C_____ all my papers but I still can't find the contract.A. uponB. outC. throughD. in120. When I'm going out in the evening I use the bike if I can, ___B_____ the car.A. regardless ofB. more thanC. other thanD. rather than121. We can't speak ___A____ our teacher.A. too highly ofB. too high ofC. highly of tooD. to highly of122. No one in our class ____D___ in sports than he.A. are more interestedB. are much interestedC. is much interestedD. is more interested123. As a teacher you have to ____B____ your methods to suit the needs of slower children.A. enlargeB. adjustC. affectD. afford124. ________A___ the hotel before soldiers armed with pistols and clubs charged into the crowd.A. Scarcely had they leftB. Scarcely they had leftC. Scarcely they leftD. Scarcely left they125. In the film, the peaceful life of a monk _____D_____ the violent life of a murderer.A. is compared withB. is compared toC. is contrasted toD. is contrasted with126 I didn't realize the food problem was so _____B___ in this city; with winter coming, many people would starve to death without more help.A. essentialB. criticalC. explicitD. effective127. Female socialization places importance on getting along with others, _____B___ male socialization places importance on becoming independent.。
c语言第六章函数习题答案
c语言第六章函数习题答案C语言第六章函数习题答案在C语言学习的过程中,函数是一个非常重要的概念。
函数不仅可以提高代码的可读性和可维护性,还可以使程序模块化,提高代码的复用性。
在C语言的第六章中,我们学习了关于函数的一些基础知识,并进行了一些习题的练习。
下面,我将为大家提供一些常见的函数习题答案,希望能够对大家的学习有所帮助。
1. 编写一个函数,实现两个整数的交换。
```c#include <stdio.h>void swap(int *a, int *b) {int temp = *a;*a = *b;*b = temp;}int main() {int num1 = 10;int num2 = 20;printf("交换前:num1 = %d, num2 = %d\n", num1, num2);swap(&num1, &num2);printf("交换后:num1 = %d, num2 = %d\n", num1, num2);return 0;}```2. 编写一个函数,实现判断一个数是否为素数。
```c#include <stdio.h>int isPrime(int num) {if (num <= 1) {return 0;}for (int i = 2; i < num; i++) {if (num % i == 0) {return 0;}}return 1;}int main() {int num;printf("请输入一个整数:");scanf("%d", &num);if (isPrime(num)) {printf("%d是素数。
\n", num);} else {printf("%d不是素数。
\n", num);}return 0;}```3. 编写一个函数,实现计算一个数的阶乘。
C语言编程第六章作业答案
1. 输入两个正整数m和n,求其最大公约数和最小公倍数。
辗除法——辗转相除法,又名欧几里德算法(Euclidean algorithm)乃求两个正整数之最大公因子的算法。
它是已知最古老的算法,其可追溯至3000年前。
它首次出现于欧几里德的《几何原本》(第VII卷,命题i和ii)中,而在中国则可以追溯至东汉出现的《九章算术》。
它并不需要把二数作质因子分解。
证明:设两数为a、b(a>b),求它们最大公约数(a、b)的步骤如下:用a除以b,余数为r 1。
若r1=0,则(a,b)=b;若r1≠0,则再用b除以r1,得r2 .若r2=0,则(a,b)=r1,若r2≠0,则继续用r1除以r2,……如此下去,直到能整除为止。
其最后一个非零余数即为(a,b)。
例如,和7890 的最大公因子是6, 这可由下列步骤看出:a b a mod b7890 51067890 5106 27845106 2784 23222784 2322 4622322 462 12462 12 612 6 0#include<stdio.h>#include<conio.h>main(){int a,b,num1,num2,temp;/*temp主要用来转换大小*/printf("please input two numbers:\n");scanf("%d,%d",num1,&num2);if(num1<num2) /*如果num2比num1大,则执行下面循环体,为调整num1要比num2大*/{temp=num1;num1=num2;num2=temp;}a=num1;b=num2;/*a比b大*/while(b!=0) /*只要b不等于0,就一直执行下面的循环体,直至整除完为止。
*/{temp=a%b;a=b;b=temp;/*以上3句调整了a,b大小,使其整除为止*/}printf("公约数:%d\n",a);printf("公倍数:%d\n",num1*num2/a); /*由公式知*/getch();}评析:关键是判断两数的大小,先排好位置,再大除小,一直除尽即可。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
a[1][1] a[1][2] a[1][3] 1 0 -1
a[2][1] a[2][2] a[2][3] 2 1 0
a[3][1] a[3][2] a[3][3]
0 1 2
0 1
0
第六章 数组
2. 写出程序的运行结果
(4) 程序四:
#include <stdio.h> main( ) { int i, s; i的ASCII码为105 char s1[100], s2[100]; printf(“input string1:\n”); n的ASCII码为110 gets(s1); printf(“input string2:\n”); gets(s2); s=-5 i=0; while((s1[i]==s2[i]) && (s1[i]!=„\0‟)) i++; if((s1[i] ==„\0‟) && (s2[i]==„\0‟)) s=0; 输入数据: aid else s=s1[i]-s2[i]; printf(“%d\n”,s); and }
第六章 数组
2. 写出程序的运行结果 (1) 程序一: 1 0 3 1 5 2 main( ) a[0][0] a[0][1] a[0][2] { int a[3][3]={1,3,5,7,9,11,13,15,17}; 7 1 9 2 11 3
int sum=0,i,j; a[1][0] a[1][1] a[1][2] for(i=0;i<3;i++) 13 2 15 3 17 4 for(j=0;j<3;j++) a[2][0] a[2][1] a[2][2] { a[ i ][ j ]=i+j; if(i==j) sum=sum+a[ i ][ j ]; } sum=6 printf(“sum=%d”,sum);
第六章 数组
3. 程序填空 (1) 用“两路合并法”把 两个已按升序排列的数 组合并成一个升序数 组. a 15 34 48 98
main( ) { int a[4]={15,34,48,98} int b[5]={12,32,55,67,78}; int c[10]; int i, j, k;
i=0,j=0,k=0;
while(i<4 && j<5) if(a[i]<b[j]) { c[k]=a[i]; k++; i++;} else {c[k]=b[j]; k++; j++;} while( i<4 ) { c[k]=a[i]; i++; k++;} while( j<5 ) { c[k]=b[j]; k++; j++;} for(i=0; i<k; i++) printf(“%5d”,c[i]); printf(“\n”); }
b
c
12
12 55
32
55
67
78
48
15 32 34 67 78 98
第六章 数组
3. 程序填空 (2)求能整除k且是偶数 #include <coino.h> #include <stdio.h>
的数,把这些数保
存在数组中,并按 从大到小 输出。
main() { int i,j=0,k,a[100]; scanf ("%d",&k); for( i=2 ;i<=k;i++) if(k%i==0 && i%2==0) a[j++]=i; printf("\n\n "); for(i= j-1 ;i>=0;i--) printf("%d",a[i]); }
#include <string.h> 第六章 数组 #include <stdio.h> 4.#define 改错题: M 80 (3) 计算一个字符串中包含指定子字符串的数目 . void main() { char s1[M],s2[M]; int num,i,j=0; gets(s1); /*输入字符串*/ gets(s2); /*输入子字符串*/ num=0; /******found******/ for(i=0;s1[i]<>'\0';i++) <> → != { if(s2[j]==s1[i]) { j++; if(s2[j]=='\0') { num++; /******found******/ j=i; j=0; } } } printf("\nThe result is : m=%d\n",num); }
第六章 数组
1. 选择题 (1) C语言中一维数组的定义方式为:类型说明符 数组名 A. [整型常量] B. [整型表达式] C
C. [整型常量]或[整型常量表达式] D. [变量表达式] (2) C语言中引用数组元素时,下标表达式的类型为 A.单精度型 B.双精度型 C.整型 D.指针型
C
。
(3) 若有说明 “int a[3][4];”,则对a数组元素的非法引用是 D A. a[0][3*1] B. a[2][3] C. a[1+1][0] D. a[0][4]
第六章 数组 #include <stdio.h> 4. void 改错题: main() (4){将大于整数m且紧靠m的k个素数存入数组xx。例如,若输入17(m), /******found******/ 5(k),则应输出 19,23,29,31,37. int m,k,xx[100],cnt,i; cnt → cnt=0; scanf("%d%d",&m,&k); while(cnt<k) { m++; /******found******/ for(i=2;i<m;i++) if!(m%i)break; if(i==m) xx[cnt++]=m; if(!(m%i))break; } /******found******/ for(i=0;i<=cnt;i++) i<=cnt → i<cnt; printf("%d ",xx[i]); }
printf(“The longest string is: \n%s\n”,str);
}
#include <ctype.h> 第六章 数组 #include <string.h> <stdio.h>/****found****/下面的语句中都有一处错误, 4.#include 改错题:每个 main() { 请将错误的地方改正。注意:不得增行或删行,也不得更 char str[81]; 改程序的结构。 int i=0,j,n; gets(str); (1) 读入一个英文文本行,将其中每个单词的第一个字母改成 /******found******/ n=stringlen(str); n=strlen(str) 大写,然后输出此文本行(这里的“单词”是指由空格隔 for(j=0;j<n;j++) 开的字符串。 if(i) { /******found******/ if(s[i]==' ') i=0; if(str[j]==' ') i=0; } else { /******found******/ if(str[j]!= " "); if(str[j]!=' ') { i=1; str[j]=toupper(str[j]); } } printf("\nAfter changing:\n %s\n",str); }
B.a[a[4]]
C. a[a[3]]
D. a[a[5]]
第六章 数组
1. 选择题 (6) 要求定义包含8个int类型元素的一维数组,以下错误的定义 语句是 A A. int N=8; B. #define N 3 int a[N]; int a[2*N+2]; C. int a[ ]={0,1,2,3,4,5,6,7} D. int a[1+7]={0}
字符串,并倒序保
存在字符数组s中。 例如:当n=123 时,s=“321”。
第六章 数组
3. 程序填空 (4)找出三个字符串中的最大者 #include <string.h> main( ) { char str[20],s[3][20]; int i; for(i=0; i<3; i++) gets(s[i]); if(strcmp( s[0],s[1] )>0) strcpy(str,s[0]); else strcpy( str,s[1] ); if(strcmp( s[2],str )>0) strcpy(str,s[2]);
abcdef”;
第六章 数组
1. 选择题 (9) 下面程序段的运行结果是 B char c[ ]=“\t\v\\\0will\n”; printf(“%d”,strlen(c)); A. 14 B. 3 C.9 D. 字符串中有非法字符,输出值不确定 (10) 判断字符串s1是否等于字符串s2,应当使用 D A. if(s1==s2) B. if(s1=s2) C. if(strcpy(s1,s2)) D. if(strcmp(s1,s2))==0)
}
第六章 数组
2. 写出程序的运行结果 (2) 程序二: main( ) { int i,j,row,col,max; int a[3][4]={{1,2,3,4},{9,8,7,6},{-1,-2,0,5}}; max=a[0][0]; row=0; col=0; for(i=0; i<3; i++) max=9 for(j=0; j<4; j++) if(a[i][j]>max) row=1 { max=a[i][j]; col=0 row=i; col=j; } printf(“max=%d, row=%d, col=%d\n”,max,row,col); }