数据结构上机实验源代码
数据结构上机实验源代码

数据结构上机实验源代码栈的应用十进制数转换为八进制数,逆序输出所输入的数实验代码://stack.h,头文件class stack{public:stack();bool empty()const;bool full()const;error_code gettop(elementtype &x)const;error_code push(const elementtype x);error_code pop();private:int count;elementtype data[maxlen];};stack::stack(){count=0;}bool stack::empty()const{return count==0;}bool stack::full()const{return count==maxlen;}error_code stack::gettop(elementtype &x)const{if(empty())return underflow;else{x=data[count-1];return success;}}error_code stack::push(const elementtype x){if(full())return overflow;data[count]=x;count++;return success;}error_code stack::pop(){if(empty())return underflow;count--;return success;}//主程序#include<iostream.h>enum error_code{overflow,underflow,success};typedef int elementtype;const int maxlen=20;#include"stack.h"void read_write() //逆序输出所输入的数{stack s;int i;int n,x;cout<<"please input num int n:";cin>>n;for(i=1;i<=n;i++){cout<<"please input a num:";cin>>x;s.push(x);}while(!s.empty()){s.gettop(x);cout<<x<<" ";s.pop();}cout<<endl;}void Dec_to_Ocx(int n) //十进制转换为八进制{stack s1;int mod,x;while(n!=0){mod=n%8;s1.push(mod);n=n/8;}cout<<"the ocx of the dec is:";while(!s1.empty()){s1.gettop(x);cout<<x;s1.pop();}cout<<endl;}void main(){int n;// read_write();cout<<"please input a dec:";cin>>n;Dec_to_Ocx(n);}队列的应用打印n行杨辉三角实验代码://queue.hclass queue{public:queue(){count=0;front=rear=0;}bool empty(){return count==0;}bool full(){return count==maxlen-1;}error_code get_front(elementtype &x){if(empty())return underflow;x=data[(front+1)%maxlen];return success;}error_code append(const elementtype x){if(full())return overflow;rear=(rear+1)%maxlen;data[rear]=x;count++;return success;}error_code serve(){if(empty())return underflow;front=(front+1)%maxlen;count--;return success;}private:int count;int front;int rear;int data[maxlen];};//主程序#include<iostream.h>enum error_code{overflow,underflow,success};typedef int elementtype;const int maxlen=20;#include"queue.h"void out_number(int n) //打印前n行的杨辉三角{int s1,s2;int i;int j;int k;queue q;for(i=1;i<=(n-1)*2;i++)cout<<" ";cout<<"1 "<<endl;q.append(1);for(i=2;i<=n;i++){s1=0;for(k=1;k<=(n-i)*2;k++)cout<<" ";for(j=1;j<=i-1;j++){q.get_front(s2);q.serve();cout<<s1+s2<<" ";q.append(s1+s2);s1=s2;}cout<<"1 "<<endl;q.append(1);}}void main(){int n;cout<<"please input n:";cin>>n;out_number(n);}单链表实验实验目的:实验目的(1)理解线性表的链式存储结构。
数据结构报告C语言上机操作

}
ht[l].parent=i; /*下标为i的新结点成为权值最小的两个结点的双亲*/
ht[r].parent=i;
ht[i].weight=ht[l].weight+ht[r].weight; /*新结点的权值为两个结点的权值之和*/
ht[i].lchild=l; /*权值最小的结点是新结点的左孩子*/
i=2*n-2; /*重新回到根结点,准备下一次搜索*/
}
bit++; /*取编码中的下一个代码*/
}
}
void main()
{
int i,j,k,n,t,x,cnt[27];
char st[50],sr[27],bm[200];
HTNode ht[2*N-1]; /*用于存放树中的所有结点*/
HCode hcd[N]; /*用于存放字符的哈夫曼编码*/
ht[i].rchild=r; /*权值次小的结点是新结点的右孩子*/
}
}
typedef struct
{
char bits[N]; /*存放哈夫曼编码的字符数组*/
int start; /*记录编码的起始位置,因为每种字符的编码长度不同*/
}HCode;
void HufmCode(HTNode ht[],HCode hcd[],int n) /*利用哈夫曼树求出各字符的哈夫曼编码*/
printList(head);
break;
case 0:
printf("再见!\n");
getch();
return;
}
}
}
int menu_select()
{
int sn;
数据结构试验完整代码
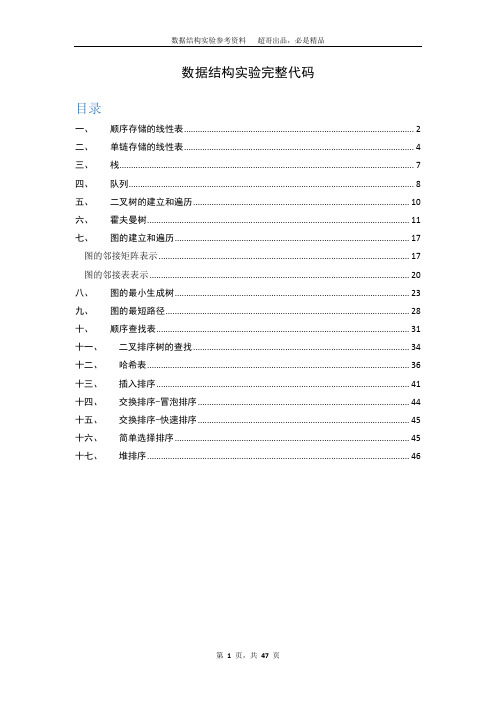
数据结构实验完整代码目录一、顺序存储的线性表 (2)二、单链存储的线性表 (4)三、栈 (7)四、队列 (8)五、二叉树的建立和遍历 (10)六、霍夫曼树 (11)七、图的建立和遍历 (17)图的邻接矩阵表示 (17)图的邻接表表示 (20)八、图的最小生成树 (23)九、图的最短路径 (28)十、顺序查找表 (31)十一、二叉排序树的查找 (34)十二、哈希表 (36)十三、插入排序 (41)十四、交换排序-冒泡排序 (44)十五、交换排序-快速排序 (45)十六、简单选择排序 (45)十七、堆排序 (46)一、顺序存储的线性表typedef struct{char name[10];char no[10];double grade;}Student;typedef struct{Student *elem;int length;int listsize;}SqList;void Display(SqList *L){int i;for (i=0;i<L->length ;i++){cout<<i+1<<":姓名"<<L->elem[i].name<<",学号:"<<L->elem[i].no<<",成绩:"<<L->elem[i].grade <<endl;}cout<<"请选择菜单项:";}SqList *CreateList(){SqList *L;L=(SqList*)malloc(sizeof(SqList));if(!L) cout<<"建立线性表失败!";else cout<<"建立线性表成功!";return(L);}int InitList(SqList *L){int i;char name[10],no[10];double grade;L->elem=(Student *)malloc(ListInitSize * sizeof(Student));if (!(L->elem)) cout<<"初始化表失败!";L->length = 0;L->listsize = ListInitSize;cout<<"请输入要录入信息的学生个数:"<<endl;cin>>i;if (i>(L->listsize)){L->elem =(Student *)realloc(L->elem ,i*sizeof(Student));}for (int j=0;j<i;j++){cout<<"请输入第"<<j+1<<"个学生的信息:"<<endl;cin>>name>>no>>grade;strcpy((L->elem+L->length)->name,name);strcpy((L->elem+L->length)->no,no);(L->elem+L->length)->grade =grade;L->length ++;}cout<<"信息录入完成!";return 0;}int Insert(SqList *l){Student e;int i,j;Student *newbase;cout<<"请输入要插入的位置:";cin>>j;j--;cout<<"请输入学生信息:";cin>>>>e.no>>e.grade;if(l->length==l->listsize){newbase=(Student*)realloc(l->elem,(l->listsize+ListIncreasement)*sizeof(Studen t));if(!newbase){cout<<"出错!";return 0;}l->elem=newbase;l->listsize+=ListIncreasement;}for(i=l->length;i>=j;i--){l->elem[i+1] = l->elem[i];}l->elem[j]=e;l->length++;cout<<"插入成功!";return 0;}int Delect(SqList *L){int i,j;cout<<"输入删除信息的位置:";cin>>j;j--;cout<<"删除的信息为:姓名:"<<L->elem[j].name<<",学号:"<<L->elem[j].no<<"成绩:"<<L->elem[j].grade<<endl;for(i=j+1;i<=L->length;i++){L->elem[i-1]=L->elem[i];}L->length--;cout<<"请按回车继续"<<endl;getchar();getchar();cout<<"删除成功!";return 0;}二、单链存储的线性表typedef struct Student{char name[10];char no[10];double grade;}Student;typedef struct LNode{Student data;LNode *next;}LNode,*LinkList;void CreateList(LinkList &l){l=(LinkList)malloc(sizeof(LNode));if (!l) cout<<"建立失败。
数据结构上机实验程序

链栈#include<iostream.h>#include<stdlib.h>typedef int ElemType;struct SNode{ElemType data;SNode* next;};void InitStack(SNode*& HS){HS=NULL;//将栈置空}void Push(SNode*& HS,const ElemType& item) {//为插入元素获取动态结点SNode* newptr=new SNode;//给新分配的结点赋值newptr->data=item;//向栈顶插入新结点newptr->next=HS;HS=newptr;}ElemType Pop(SNode*& HS){if(HS==NULL){cerr<<"Linked stack is empty!"<<endl;exit(1);}SNode*p=HS;HS=HS->next;ElemType temp=p->data;delete p;return temp;}ElemType Peek(SNode* HS){if(HS==NULL){cerr<<"Linked stack is empty!"<<endl;exit(1);}return HS->data;//返回栈顶结点的值}bool EmptyStack(SNode* HS){return HS==NULL;}void ClearStack(SNode*& HS){SNode *cp,*np;cp=HS;//给cp指针赋初值,使之指向栈顶结点while(cp!=NULL){//从栈顶到栈底依次删除每个结点np=cp->next;delete cp;cp=np;}HS=NULL;}void main(){SNode* a;InitStack(a);int x;cin>>x;while(x!=-1){Push(a,x);cin>>x;}while(!EmptyStack(a))//栈不为空时依次退栈打印出来cout<<Pop(a)<<" ";cout<<endl;ClearStack(a);}顺序栈#include<iostream.h>#include<stdlib.h>typedef int ElemType;struct Stack{ElemType *stack;int top;int MaxSize;};void InitStack(Stack& S){//初始设置栈空间大小为10个元素位置S.MaxSize=10;//动态空间分配,若分配失败侧退出运行S.stack=new ElemType[S.MaxSize];if(!S.stack){cerr<<"动态存储分配失败!"<<endl;exit(1);}S.top=-1;}void Push(Stack& S,ElemType item){if(S.top==S.MaxSize-1){int k=sizeof(ElemType);S.stack=(ElemType*)realloc(S.stack,2*S.MaxSize*k);S.MaxSize=2*S.MaxSize;}//栈顶指针后移一个位置S.top++;//新元素插入栈顶S.stack[S.top]=item;}ElemType Pop(Stack& S){//若栈空则退出运行if(S.top==-1){cerr<<"Stack is empty!"<<endl;exit(1);}//栈顶指针减1表示退栈S.top--;//返回原栈顶元素的值return S.stack[S.top+1];}ElemType Peek(Stack& S){if(S.top==-1){cerr<<"Stack is empty!"<<endl;exit(1);}return S.stack[S.top];}bool EmptyStack(Stack& S){return S.top==-1;}void ClearStack(Stack& S){if(S.stack){delete []S.stack;S.stack=0;}S.top=-1;S.MaxSize=0;}void main(){Stack s;InitStack(s);int a[8]={4,5,6,20,42,30,58,65};int i;for(i=0;i<8;i++) Push(s,a[i]);cout<<Pop(s);cout<<' '<<Pop(s)<<endl;Push(s,60);cout<<Peek(s);cout<<' '<<Pop(s)<<endl;while(!EmptyStack(s)) cout<<Pop(s)<<' ';cout<<endl;ClearStack(s);}栈的应用----运算#include<iostream.h>#include<stdlib.h>typedef char ElemType;struct Stack{ElemType *stack;int top;int MaxSize;};void InitStack(Stack& S){//初始设置栈空间大小为10个元素位置S.MaxSize=10;//动态存储空间分配,若分配失败则退出运行S.stack=new ElemType[S.MaxSize];if(!S.stack){cerr<<"动态存储分配失败!"<<endl;exit(1);}S.top=-1;}void Push(Stack& S,ElemType item){//若栈空间用完则自动扩大2倍空间,原有栈内容不变if(S.top==S.MaxSize-1){int k=sizeof(ElemType);S.stack=(ElemType*)realloc(S.stack,2*S.MaxSize*k);S.MaxSize=2*S.MaxSize;}S.top++;S.stack[S.top]=item;}ElemType Pop(Stack& S){if(S.top==-1){cerr<<"Stack is empty!"<<endl;exit(1);}S.top--;return S.stack[S.top+1];}ElemType Peek(Stack& S){if(S.top==-1)cerr<<"Stack is empty!"<<endl;exit(1);}return S.stack[S.top];}bool EmptyStack(Stack& S){return S.top==-1;}void ClearStack(Stack& S){if(S.stack){delete[]S.stack;S.stack=0;}S.top=-1;S.MaxSize=0;}int Precedence(char op){switch(op){case '+':case '-':return 1;case '*':case '/':return 2;case '(':case '@':default:return 0;}}void Change(char*S1,char*S2){Stack R;InitStack(R);Push(R,'@');int i=0,j=0;char ch=S1[i];while(ch!='\0')if(ch==' ') ch=S1[++i];else if(ch=='('){Push(R,ch); ch=S1[++i];}else if(ch==')'){while(Peek(R)!='(') S2[j++]=Pop(R);Pop(R);ch=S1[++i];}else if(ch=='+'||ch=='-'||ch=='*'||ch=='/'){char w=Peek(R);while(Precedence(w)>=Precedence(ch)){S2[j++]=w;Pop(R);w=Peek(R);}Push(R,ch);ch=S1[++i];}else{if((ch<'0'||ch>'9')&& ch!='.'){cout<<"中缀表达式错误!"<<endl;exit(1);}while((ch>='0'&&ch<='9')||ch=='.'){S2[j++]=ch;ch=S1[++i];}S2[j++]=' ';}}ch=Pop(R);while(ch!='@'){if(ch=='('){cerr<<"expression error!"<<endl;exit(1);}else{S2[j++]=ch;ch=Pop(R);}}S2[j++]='\0';}void main(){char a[30];char b[30];cout<<"请输入一个中缀算术表达式:"<<endl;cin.getline(a,sizeof(a));Change(a,b);cout<<"对应的后缀算术表达式: "<<endl;cout<<b<<endl;}。
数据结构实验报告代码

《数据结构》实验报告实验序号:5 实验项目名称:链式栈五、分析与讨论对上机实践结果进行分析,上机的心得体会。
六、教师评语成绩签名:日期:附源程序清单:实验要求编程实现如下功能:(1)按照输入的栈中元素个数n和各元素值成立一个顺序栈,并输出栈中各元素值。
(2)将数据元素e入栈,并输出入栈后的顺序栈中各元素值。
(3)将顺序栈中的栈顶元素出栈,并输出出栈元素的值和出栈后顺序栈中各元素值。
2. 实验相关原理:栈是一种插入和删除操作都限制在表的一端进行的特殊线性表,它的操作具有“先进后出”的特性。
采用顺序存储结构的栈称为顺序栈。
栈的存储结构描述如下:#define MAXSIZE 100; /*顺序栈的最大长度*/typedef struct{ Selemtype base[MAXSIZE]; /*存储栈中数据元素的数组*/int top; /*top为栈顶指针,它指示栈顶元素的存储空间的下一个存储单元*/}Sqstack;【核心算法提示】1.顺序栈入栈操作的大体步骤:第一判断顺序栈是不是为满,若是满,则函数返回ERROR,不然将待入栈的数据元素寄存在top所指示的存储单元中,再使top后移一个存储单元位置,即将top值加1,最后函数返回OK。
2.顺序栈出栈操作的大体步骤:第一判断顺序栈是不是为空,若是空,则函数返回ERROR,不然将栈顶指针前移一个存储单元位置,即将top值减1,再将top所指示的栈顶元素用e返回其值,并使函数返回OK。
【核心算法描述】status Push(Sqstack &S,Selemtype e)/*将数据元素e压入到顺序栈S中,使其成为新的栈项元素*/{ if >=MAXSIZE) /*若是栈满,则函数返回ERROR*/return ERROR;[++]=e;/*将新元素e寄存在top所指示的存储单元中,并使top值加1*/return OK;}status Pop(Sqstack &S,Selemtype &e)/*将顺序栈S中的栈顶元素从栈中删除,并用e返回其值*/{ if ==0) /*若是栈空,则函数返回ERROR*/Return ERROR;e=[];/*将top值减1,并用e保留top所指示的栈顶元素值*/return OK;}3.源程序代码参考#define MAXSIZE 100typedef struct{ int base[MAXSIZE];int top; /*top指示存储栈顶元素的下一存储单元*/}Sqstack; /*顺序栈的类型概念*/Sqstack Push(Sqstack S,int e) /*顺序栈的入栈操作函数*/{ if >=MAXSIZE)printf("Stack is Overflow\n");else[++]=e;return S;}Sqstack Pop(Sqstack S,int *e) /*顺序栈的出栈操作函数*/{ if ==0)printf("Stack is Empty\n");else*e=[];return S;}void Stack_display(Sqstack S) /*顺序栈的输出函数*/{ int i;for(i=0; i<;i++) /*依次输出栈中各元素的值,栈顶元素在表的尾部*/printf("%4d", [i]);printf("\n");}main(){ Sqstack S;int i,j,n,x,e;printf("please input the length:");/*请求输入顺序栈中元素个数*/scanf("%d",&n);printf("please input the Value:\n ");/*请求输入顺序栈中各个元素值*/for(i=0;i<n;i++)scanf("%d",&[i]);=n;printf("the stack is:\n");Stack_display(S);printf("please input the insert node:");/*请求输入需要入栈的新元素*/scanf("%d",&x);S=Push(S,x);printf("the stack after push is:\n");/*提示输出入栈后栈中各个元素值*/Stack_display(S); /*挪用顺序栈的输出函数*/S=Pop(S,&e);printf("the pop value is:%d\n",e); /*输出出栈元素的值*/printf("the stack after pop is:\n");/*提示输出出栈后栈中各个元素值*/Stack_display(S); /*挪用顺序栈的输出函数*/}(1)按照输入的栈中元素个数和各元素值成立一个链栈,并输出链栈中各元素值, 观察输入的内容与输出的内容是不是一致,特别注意栈顶元素的位置。
数据结构实验系统源代码(期末作业)
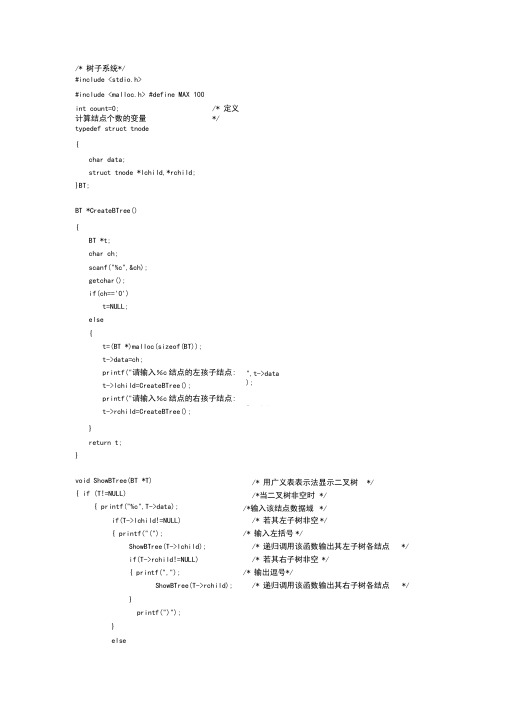
/* 树子系统*/#include <stdio.h>#include <malloc.h> #define MAX 100int count=0; /* 定义计算结点个数的变量*/ typedef struct tnode{char data;struct tnode *lchild,*rchild;}BT;BT *CreateBTree(){BT *t;char ch;scanf("%c",&ch);getchar();if(ch=='0')t=NULL;else{t=(BT *)malloc(sizeof(BT));t->data=ch;printf("请输入%c结点的左孩子结点:t->lchild=CreateBTree();printf("请输入%c结点的右孩子结点:t->rchild=CreateBTree();}return t;}void ShowBTree(BT *T){ if (T!=NULL){ printf("%c",T->data);if(T->lchild!=NULL){ printf("(");ShowBTree(T->lchild);if(T->rchild!=NULL){ printf(",");ShowBTree(T->rchild);}printf(")");}else",t->data);",t->data/* 用广义表表示法显示二叉树*//*当二叉树非空时*//*输入该结点数据域*//* 若其左子树非空*//* 输入左括号*//* 递归调用该函数输出其左子树各结点*/ /* 若其右子树非空*//* 输出逗号*//* 递归调用该函数输出其右子树各结点*//* 二叉树左子树为空,右子树不为空时*/ if(T->rchild!=NULL){printf("(");ShowBTree(T->lchild);if(T->rchild!=NULL){ printf(",");ShowBTree(T->rchild);} printf(")");}}}void PreOrder(BT *T){ if(T==NULL) return;else{ printf("%c",T->data);PreOrder(T->lchild);PreOrder(T->rchild);}}void InOrder(BT *T){ if(T==NULL) return;else{ InOrder(T->lchild);printf("%c",T->data);InOrder(T->rchild);}}void PostOrder(BT *T){ if (T==NULL) return;else{ PostOrder(T->lchild);PostOrder(T->rchild);printf("%c",T->data);}}void LevelOrder(BT *T){ int f,r;BT *p,*q[MAX];p=T;/* 输入左括号*//* 递归调用该函数输出其左子树各结点*/ /* 若其右子树非空*//* 输出逗号*//*递归调用该函数输出其右子树各结点*//* 先序遍历二叉树T*//*递归调用的结束条件*//* 输出结点的数据域*//*先序递归遍历左子树*//*先序递归遍历右子树*//* 中序遍历二叉树T*//* 递归调用的结束条件*//* 中序递归遍历左子树*//* 输出结点的数据域*//* 中序递归遍历右子树*//* 后序遍历二叉树T*//* 递归调用的结束条件*//* 后序递归遍历左子树*//* 后序递归遍历右子树*//* 输出结点的数据域*//* 按层次遍历二叉树T*//* 定义队头队尾指针*//* 定义循环队列,存放结点指针*if(p!=NULL){ f=1; q[f]=p; r=2; } while(f!=r) { p=q[f];printf("%c",p->data); if(p->lchild!=NULL) { q[r]=p->lchild; r=(r+1)%MAX; }if(p->rchild!=NULL) { q[r]=p->rchild; r=(r+1)%MAX; }f=(f+1)%MAX; }}/* 若二叉树非空,则根结点地址入队*//* 队列不空时 *//* 访问队首结点的数据域 */ /* 将队首结点的左孩子入队 *//* 将队首结点的右孩子入队 */void Leafnum(BT *T) /* 求二叉树叶子结点数 */ { if(T)/* 若树不为空 */{ if(T->lchild==NULL && T->rchild==NULL)count++; Leafnum(T->lchild); Leafnum(T->rchild);} }/* 全局变量 count 为计数值, 其初值为 0*/ /* 递归统计 T 的左子树叶子结点数 */ /* 递归统计 T 的右子树叶子结点数 */return rdep+1;void Nodenum(BT *T) { if(T) { count++;Nodenum(T->lchild); Nodenum(T->rchild);}} int TreeDepth(BT *T) { int ldep=0,rdep=0; 的深度 */ if(T==NULL) return 0; else { ldep=TreeDepth(T->lchild); rdep=TreeDepth(T->rchild); if(ldep>rdep)return ldep+1; else /* 若树不为空 *//* 全局变量 c ount 为计数值, 其初值为0*/ /* 递归统计 T 的左子树结点数 */ /* 递归统计 T 的右子树结点数 *//* 求二叉树深度 *//* 定义两个整型变量, 用以存放左、右子树/* 递归统计 T 的左子树深度 */ /* 递归统计 T 的右子树深度 */二叉树子系统");printf ("\n ================================================="); printf ("\n| 1——建立一个新二叉树 |"); printf ("\n| 2 --- 广义表表示法显示 |"); printf ("\n| 3 --- 先序遍历 |"); printf ("\n| 4 --- 中序遍历 |"); printf ("\n| 5 --- 后序遍历 |"); printf ("\n| 6 --- 层次遍历 |"); printf ("\n| 7 --- 求叶子结点数目 |"); printf ("\n| 8 --- 求二叉树总结点数目 |"); printf ("\n| 9——求树深度 |"); printf ("\n|0 --- 返回|");printf ("\n ================================================"); printf ("\n 请输入菜单号(0-9 ):");btree () (BT *T=NULL; char ch1,ch2,a; ch1='y';while (ch1=='y'||ch1=='Y') { MenuTree ();scanf ("%c",&ch2); getchar (); switch (ch2) {case '1':printf ("请按先序序列输入二叉树的结点: \n");printf ("说明:输入结点后按回车(’0'表示后继结点为空):\n"); printf ("请输入根结点:"); T=CreateBTree ();printf ("二叉树成功建立! ");break; case '2':printf ("二叉树广义表表示法如下: "); ShowBTree (T );break; case '3':printf ("二叉树的先序遍历序列为: "); PreOrder (T );break; case '4':printf(" 二叉树的中序遍历序列为: ");void (MenuTree ()/*显示菜单子函数*/printf ("\nInOrder(T);break;case '5':printf(" 二叉树的后序遍历序列为:");PostOrder(T);break;case '6':printf(" 二叉树的层次遍历序列为:");LevelOrder(T);break;case '7':count=0;Leafnum(T);printf("该二叉树有%~个叶子。
数据结构与算法实验源代码
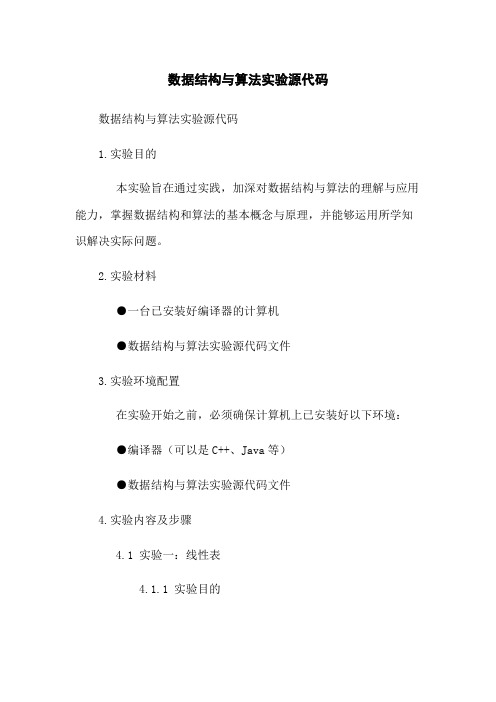
数据结构与算法实验源代码数据结构与算法实验源代码1.实验目的本实验旨在通过实践,加深对数据结构与算法的理解与应用能力,掌握数据结构和算法的基本概念与原理,并能够运用所学知识解决实际问题。
2.实验材料●一台已安装好编译器的计算机●数据结构与算法实验源代码文件3.实验环境配置在实验开始之前,必须确保计算机上已安装好以下环境:●编译器(可以是C++、Java等)●数据结构与算法实验源代码文件4.实验内容及步骤4.1 实验一:线性表4.1.1 实验目的通过实现线性表的相关操作,加深对线性表及其操作的理解,并能够灵活应用。
4.1.2 实验步骤1.实现线性表的初始化函数2.实现线性表的插入操作3.实现线性表的删除操作4.实现线性表的查找操作5.实现线性表的排序操作6.实现线性表的输出操作7.编写测试代码,对线性表进行测试4.1.3 实验结果与分析进行若干测试用例,验证线性表的正确性,并分析算法的时间复杂度与空间复杂度。
4.2 实验二:栈与队列4.2.1 实验目的通过实现栈与队列的相关操作,加深对栈与队列的理解,并掌握栈与队列的应用场景。
4.2.2 实验步骤1.实现栈的初始化函数2.实现栈的入栈操作3.实现栈的出栈操作4.实现栈的查看栈顶元素操作5.实现队列的初始化函数6.实现队列的入队操作7.实现队列的出队操作8.实现队列的查看队首元素操作4.2.3 实验结果与分析进行若干测试用例,验证栈与队列的正确性,并分析算法的时间复杂度与空间复杂度。
(继续添加实验内容及步骤,具体根据实验项目和教学要求进行详细分析)5.实验附件本文档所涉及的实验源代码文件作为附件随文档提供。
6.法律名词及注释6.1 版权:著作权法所规定的权利,保护作品的完整性和原创性。
6.2 开源:指软件可以被任何人免费使用、分发和修改的一种软件授权模式。
(继续添加法律名词及注释)。
数据结构上机实验

数据结构1.创建一个链表并将他输出#include <stdio.h>#include <stdlib.h>typedef struct node{ int data;struct *link;}*linklist,Lnode;linklist Creat(int n){linklist p,list=NULL,r; int i,d;for(i=1;i<=n;i++){p=(linklist)malloc(sizeof(Lnode));printf("Enter a int data:"); scanf("%d",&d);p->data=d; p->link=NULL;if(list==NULL)list=p;elser->link=p;r=p; }return list;}void print(linklist list){linklist p; p=list;while(p!=NULL){printf("%d\n",p->data); p=p->link ; }}void main(){int n;linklist L;printf("Enter the number of data"); scanf("%d",&n); L=Creat(n); print(L);}创建线性链表在表头插一行#include <stdio.h>#include <stdlib.h>typedef struct node{ int data;struct node * link ;}*linklist,lnode;linklist creat(int n){ linklist p,list=NULL,r;int i ,d;for (i=1;i<=n; i++){ p=(linklist)malloc(sizeof(lnode));printf("enter a int data");scanf ("%d",&d);p->data=d;p->link=NULL;if(list==NULL)list=p;elser->link=p;r=p;}return list;}void print(linklist list){ linklist p;p=list;while (p!=NULL){ printf("%d\n",p->data);p=p->link;}}linklist insertf(linklist list,int item) { linklist p;p=(linklist)malloc(sizeof(lnode));p->data=item;p->link=list;list=p;return list;}void main(){int n,newdata;linklist l;printf("Enter the number of element:"); scanf("%d",&n);l=creat(n);print(l);printf("Enter a newdata:");scanf("%d",&newdata);l=insertf(l,newdata);print(l);}2.检查字符串是否匹配#include<stdio.h>int main(){char stack[20],s[256],w; int top=-1,i=0;printf("Enter a string:"); scanf("%s",s);while(s[i]!='\0'){ if(s[i]=='('||s[i]=='{'||s[i]=='['){stack[++top]=s[i];printf( "push %c %d\n",s[i],i);}elseif(s[i]==')'||s[i]=='}'||s[i]==']'){ w=stack[top--];if((s[i]==')'&&w!='(')||(s[i]==']'&&w!='[')||(s[i]=='}'&&w!='{')) {printf("Error\n");return 0;}}i++;}if(top==-1)printf("OK\n");elseprintf("ERROR\n");return 1;}3、顺序存储方式判断两字符串是否相等#include<stdio.h>int comstr(char A[],char B[]){int i=0;while(A[i]!='\0'&&B[i]!='\0'){if(A[i]!=B[i])return 0;i++; }if(A[i]==B[i])return 1;return 0;}void main(){char s1[256],s2[256];printf("Enter the first string:");gets(s1);printf("Enter the second string:");gets(s2);if(comstr(s1,s2))printf("YES\n");elseprintf("NO\n");}4、创建排序二叉树,采用中序遍历输出#include<stdio.h> #include<stdlib.h>typedef struct node{int data;struct node *L,*R;} *Tlink,Tnode;Tlink insert(Tlink T,int a) {Tlink q=T,p;p=(Tlink)malloc(sizeof(Tnode));p->data=a;p->L=p->R=NULL;if(T==NULL)T=p;elsewhile(1)if(a<q->data)if(q->L==NULL){q->L=p;break;}elseq=q->L;elseif(q->R==NULL){q->R=p;break;}elseq=q->R;return T;}void print(Tlink T){if(T!=NULL){ print(T->L);printf("%d,",T->data);print(T->R);}}void main(){ int a;Tlink T=NULL;while(1){ printf("Enter a int data:");scanf("%d",&a);if(a==-999)break;T=insert(T,a);}print(T);}5. 建立该图的邻接表存储做深度优先遍历。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
}
cout<<"the ocx of the dec is:";
while(!s1.empty())
{
s1.gettop(x);
cout<<x;
s1.pop();
}
cout<<endl;
}
void main()
{int n;
//read_write();
cout<<"please input a dec:";
}
while(!s.empty())
{
s.gettop(x);
cout<<x<<" ";
s.pop();
}
cout<<endl;
}
void Dec_to_Ocx(int n) //十进制转换为八进制
{
stack s1;
int mod,x;
while(n!=0)
{
mod=n%8;
s1.push(mod);
<1>求链表中第i个结点的指针(函数),若不存在,则返回NULL。
实验测试数据基本要求:
第一组数据:链表长度n≥10,i分别为5,n,0,n+1,n+2
第二组数据:链表长度n=0,i分别为0,2
<2>在第i个结点前插入值为x的结点。
实验测试数据基本要求:
第一组数据:链表长度n≥10,x=100, i分别为5,n,n+1,0,1,n+2
s->next=p->next;
p->next=s;
count++;
cout<<"插入成功!"<<endl;
return success;
}
/***************删除第i个节点*****************************/
error_code delete_element(const int i)
实验任务
说明1:本次实验中的链表结构均为带头结点的单链表。
说明2:为使实验程序简洁直观,下面的部分实验程序中将所需要的函数以调用库函数的形式给出,并假设将库函数放在程序文件“linklist.h”中,同时假设该库函数文件中定义了链表结构中的指针类型为link,结点类型为node,并定义了部分常用运算。
q.append(1);
for(i=2;i<=n;i++)
{
s1=0;
for(k=1;k<=(n-i)*2;k++)
cout<<" ";
for(j=1;j<=i-1;j++)
{
q.get_front(s2);
q.serve();
cout<<s1+s2<<" ";
q.append(s1+s2);
s1=s2;
{return head;}
//输出链表中的所有元素
error_code print()
}
}
error_code stack::push(const elementtype x)
{
if(full())return overflow;
data[count]=x;
count++;
return success;
}
error_code stack::pop()
{
if(empty())return underflow;
数据结构上机实验源代码
栈的应用
十进制数转换为八进制数,
逆序输出所输入的数
实验代码:
//stack.h,头文件
class stack{
public:stack();
bool empty()const;
bool full()const;
error_code gettop(elementtype &x)const;
{ห้องสมุดไป่ตู้
node *p=head;
int j=0;
while(j!=i-1&&p!=NULL)
{p=p->next;
j++;
}
if(i<1||i>length()){cout<<"此元素不存在"<<endl;
return arrange_error;}
node *u=p->next;
p->next=u->next;
x分别为25,85,110和8
<5>将单链表L中的奇数项和偶数项结点分解开,并分别连成一个带头结点的单链表,然后再将这两个新链表同时输出在屏幕上,并保留原链表的显示结果,以便对照求解结果。
实验测试数据基本要求:
第一组数据:链表元素为(1,2,3,4,5,6,7,8,9,10,20,30,40,50,60)
while(p!=NULL)
{if(j==i)return p;
p=p->next;
j++;}
return NULL;
}
/***************在第i节点之前插入数为x的节点****************/
error_code insert(const int i,const elementtype x)
第二组数据:链表长度n=0,x=100,i=5
<3>删除链表中第i个元素结点。
实验测试数据基本要求:
第一组数据:链表长度n≥10,i分别为5,n,1,n+1,0
第二组数据:链表长度n=0,i=5
<4>在一个递增有序的链表L中插入一个值为x的元素,并保持其递增有序特性。
实验测试数据基本要求:
链表元素为(10,20,30,40,50,60,70,80,90,100),
count=0;
}
int length() //求链表的长度
{
return count;
}
/***********取链表中第i个节点的指针**********************/
node * locate(const int i)
{
node *p=head;
int j=0;
if(i<1||i>length())return NULL;
}
private:int count;
int front;
int rear;
int data[maxlen];
};
//主程序
#include<iostream.h>
enum error_code{overflow,underflow,success};
typedef int elementtype;
}
error_code get_front(elementtype &x){
if(empty())return underflow;
x=data[(front+1)%maxlen];
return success;
}
error_code append(const elementtype x)
{
if(full())return overflow;
const int maxlen=20;
#include"queue.h"
void out_number(int n) //打印前n行的杨辉三角
{
int s1,s2;
int i;
int j;
int k;
queue q;
for(i=1;i<=(n-1)*2;i++)
cout<<" ";
cout<<"1 "<<endl;
cin>>n;
Dec_to_Ocx(n);
}
队列的应用
打印n行杨辉三角
实验代码:
//queue.h
class queue{
public:queue(){
count=0;
front=rear=0;}
bool empty(){
return count==0;
}
bool full(){
return count==maxlen-1;
error_code push(const elementtype x);
error_code pop();
private:
int count;
elementtype data[maxlen];
};
stack::stack(){
count=0;
}
bool stack::empty()const
{
return count==0;
if(head->next->data>x){node *u=new node;
u->data=x;
u->next=head->next;
head->next=u;
return success;
}
while(p->next!=NULL){
if(p->next->data>x){node *u=new node;
}
bool stack::full()const
{
return count==maxlen;