java读取本地文件
JAVA读取WORD文档解决方案

JAVA读取WORD文档解决方案在Java中读取Word文档需要使用特定的Java库或API来解析和处理Word文档格式(.doc或.docx)。
在下面的解决方案中,我们将介绍两种流行的Java库,即Apache POI和JavaFX的XSSF。
1. Apache POI:Apache POI是一个流行的开源Java库,用于处理Microsoft Office 格式的文件,包括Word文档。
下面是使用Apache POI库读取Word文档的步骤:1.1 添加Apache POI依赖库到项目中。
在Maven项目中,可以在pom.xml文件中添加以下依赖项:```xml<dependency><groupId>org.apache.poi</groupId><artifactId>poi</artifactId><version>4.1.2</version></dependency><dependency><groupId>org.apache.poi</groupId><artifactId>poi-ooxml</artifactId><version>4.1.2</version></dependency>```1.2 使用`XWPFDocument`类打开Word文档。
下面是一个示例代码:```javaFileInputStream fis = newFileInputStream("path/to/word/document.docx");XWPFDocument document = new XWPFDocument(fis);fis.close(;```1.3 使用`XWPFParagraph`类和`XWPFRun`类来遍历Word文档中的段落和文本。
Java读取TXT文件的多种方式

Java读取TXT文件的多种方式按行读取TXT文件package zc;import java.io.BufferedReader;import java.io.File;import java.io.FileNotFoundException;import java.io.FileReader;import java.io.IOException;public class readLine {public static void main(String[] args) {// TODO Auto-generated method stubFile file = new File("C:/zc.txt");BufferedReader reader = null;String tempString = null;int line =1;try {System.out.println("以行为单位读取文件内容,一次读一整行:");reader = new BufferedReader(new FileReader(file));while ((tempString = reader.readLine()) != null) {System.out.println("Line"+ line + ":" +tempString);line ++ ;}reader.close();} catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch block e.printStackTrace();}finally{if(reader != null){try {reader.close();} catch (IOException e) {// TODO Auto-generated catch block e.printStackTrace();}}}}}按字节读取TXT文件package zc;import java.io.File;import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException;import java.io.InputStream;public class readerFileByChars {public static void main(String[] args) {// TODO Auto-generated method stubFile file = new File("c:/zc.txt");InputStream in = null;byte[] tempByte = new byte[1024];int byteread = 0;try {System.out.println("以字节为单位读取文件内容,一次读多个字节:");in = new FileInputStream(file);while ((byteread = in.read(tempByte)) != -1 ) {System.out.write(tempByte, 0, byteread);}} catch (FileNotFoundException e) {// TODO Auto-generated catch blocke.printStackTrace();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}finally{if (in != null) {try {in.close();} catch (IOException e) {// TODO Auto-generated catch blocke.printStackTrace();}} }}。
Java如何读取文本文件
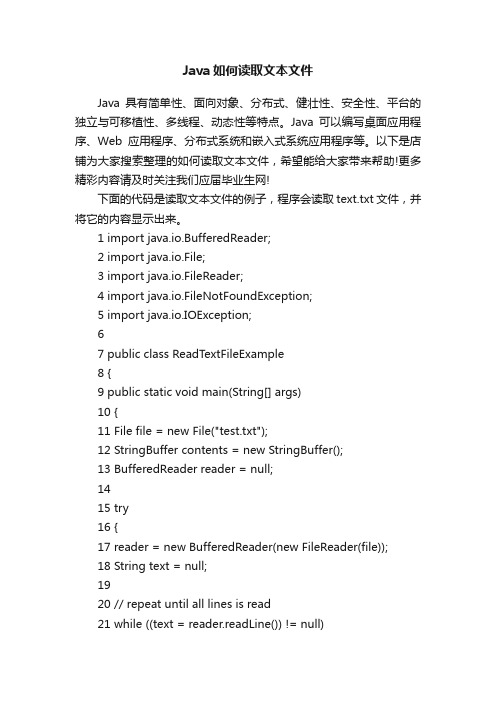
Java如何读取文本文件Java具有简单性、面向对象、分布式、健壮性、安全性、平台的独立与可移植性、多线程、动态性等特点。
Java可以编写桌面应用程序、Web应用程序、分布式系统和嵌入式系统应用程序等。
以下是店铺为大家搜索整理的如何读取文本文件,希望能给大家带来帮助!更多精彩内容请及时关注我们应届毕业生网!下面的代码是读取文本文件的例子,程序会读取text.txt文件,并将它的内容显示出来。
1 import java.io.BufferedReader;2 import java.io.File;3 import java.io.FileReader;4 import java.io.FileNotFoundException;5 import java.io.IOException;67 public class ReadTextFileExample8 {9 public static void main(String[] args)10 {11 File file = new File("test.txt");12 StringBuffer contents = new StringBuffer();13 BufferedReader reader = null;1415 try16 {17 reader = new BufferedReader(new FileReader(file));18 String text = null;1920 // repeat until all lines is read21 while ((text = reader.readLine()) != null)22 {23 contents.append(text)24 .append(System.getProperty(25 "line.separator"));26 }。
用Java读取Word文档
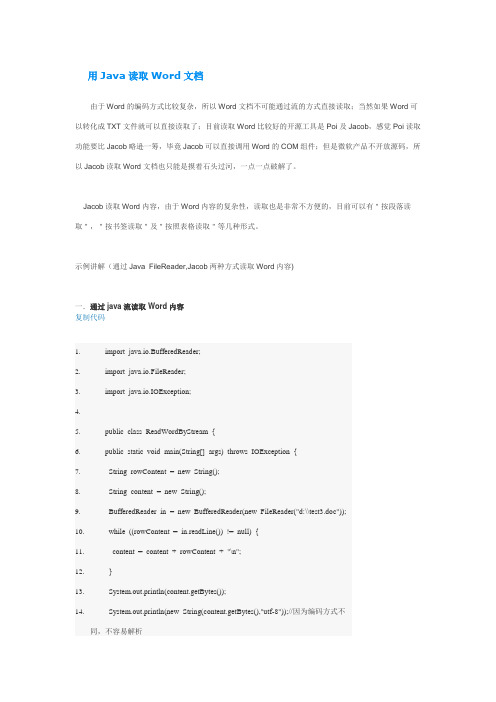
用Java读取Word文档由于Word的编码方式比较复杂,所以Word文档不可能通过流的方式直接读取;当然如果Word可以转化成TXT文件就可以直接读取了;目前读取Word比较好的开源工具是Poi及Jacob,感觉Poi读取功能要比Jacob略逊一筹,毕竟Jacob可以直接调用Word的COM组件;但是微软产品不开放源码,所以Jacob读取Word文档也只能是摸着石头过河,一点一点破解了。
Jacob读取Word内容,由于Word内容的复杂性,读取也是非常不方便的,目前可以有"按段落读取","按书签读取"及"按照表格读取"等几种形式。
示例讲解(通过Java FileReader,Jacob两种方式读取Word内容)一.通过java流读取Word内容复制代码1.import java.io.BufferedReader;2.import java.io.FileReader;3.import java.io.IOException;4.5.public class ReadWordByStream {6.public static void main(String[] args) throws IOException {7. String rowContent = new String();8. String content = new String();9. BufferedReader in = new BufferedReader(new FileReader("d:\\test3.doc"));10. while ((rowContent = in.readLine()) != null) {11.content = content + rowContent + "\n";12. }13. System.out.println(content.getBytes());14. System.out.println(new String(content.getBytes(),"utf-8"));//因为编码方式不同,不容易解析15. in.close();16.}17.18.}二.通过Jacob读取Word内容复制代码1.import com.jacob.activeX.ActiveXComponent;2.import Thread;3.import .Dispatch;4.import .Variant;5.6.public class WordReader {7.public static void main(String args[]) {8. ComThread.InitSTA();// 初始化com的线程9. ActiveXComponent wordApp = new ActiveXComponent("Word.Application"); // 启动word10. // Set the visible property as required.11. Dispatch.put(wordApp, "Visible", new Variant(true));// //设置word可见12. Dispatch docs = wordApp.getProperty("Documents").toDispatch();//所有文档窗口13.// String inFile = "d:\\test.doc";14.// Dispatch doc = Dispatch.invoke(docs,"Open",Dispatch.Method,15.// new Object[] { inFile, new Variant(false),new Variant(false) },//参数3,false:可写,true:只读16.// new int[1]).toDispatch();//打开文档17.18. Dispatch doc = Dispatch.call(docs, "Add").toDispatch(); //创建一个新文档19. Dispatch wordContent = Dispatch.get(doc, "Content").toDispatch(); //取得word文件的内容20. Dispatch font = Dispatch.get(wordContent, "Font").toDispatch();21. Dispatch.put(font, "Bold", new Variant(true)); // 设置为粗体22.Dispatch.put(font, "Italic", new Variant(true)); // 设置为斜体23.Dispatch.put(font, "Underline", new Variant(true));24.Dispatch.put(font, "Name", new Variant("宋体"));25.Dispatch.put(font, "Size", new Variant(14));26. for(int i=0;i<10;i++){//作为一个段落27.Dispatch.call(wordContent, "InsertAfter", "current paragraph"+i+" ");28. }29. for(int j=0;j<10;j++){//作为十个段落30. Dispatch.call(wordContent, "InsertAfter", "current paragraph"+j+"\r");31.}32. Dispatch paragraphs = Dispatch.get(wordContent, "Paragraphs")33. .toDispatch(); //所有段落34. int paragraphCount = Dispatch.get(paragraphs, "Count").getInt();35. System.out.println("paragraphCount:"+paragraphCount);36.37. for (int i = 1; i <= paragraphCount; i++) {38.Dispatch paragraph = Dispatch.call(paragraphs, "Item",39.new Variant(i)).toDispatch();40.Dispatch paragraphRange = Dispatch.get(paragraph, "Range")41..toDispatch();42.String paragraphContent = Dispatch.get(paragraphRange, "Text")43..toString();44.System.out.println(paragraphContent);45.//Dispatch.call(selection, "MoveDown");46. }47. // WordReader.class.getClass().getResource("/").getPath().substring+"test.doc";48. Dispatch.call(doc, "SaveAs","d:\\wordreader.doc");49. // Close the document without saving changes50. // 0 = wdDoNotSaveChanges51. // -1 = wdSaveChanges52. // -2 = wdPromptToSaveChanges53. ComThread.Release();//释放com线程54. Dispatch.call(docs, "Close", new Variant(0));55. docs = null;56. Dispatch.call(wordApp,"Quit");57. wordApp = null;58.}59.}用Java简单的读取word文档中的数据:第一步:下载tm-extractors-0.4.jar下载地址:/browser/elated-core/trunk/lib/tm-extractors-0.4.jar?rev =46并把它放到你的classpath路径下面。
java 读取本地文本文件的方法

java 读取本地文本文件的方法
在Java中,读取本地文本文件可以使用包中的Files类和Paths类。
以下是一个简单的示例代码,演示如何读取本地文本文件:
```java
import ;
import ;
import ;
import ;
public class ReadFileExample {
public static void main(String[] args) {
String filePath = "path/to/your/"; // 替换为你的文件路径
try {
List<String> lines = ((filePath));
for (String line : lines) {
(line);
}
} catch (IOException e) {
();
}
}
}
```
在上面的代码中,我们首先指定要读取的文件的路径。
然后,使用Files类的readAllLines()方法读取文件的全部内容,并将每行作为字符串存储在一个List中。
最后,我们遍历List并打印每行内容。
请注意,如果文件非常大,使用readAllLines()方法可能会导致内存不足的问题。
在这种情况下,可以考虑使用BufferedReader逐行读取文件,以减少内存的使用量。
java 根据路径获取文件方法

java 根据路径获取文件方法Java是一种广泛应用于软件开发的高级编程语言。
在Java中,我们经常需要根据路径获取文件。
本文将介绍如何使用Java来实现这一功能,并提供一步一步的指导。
第一步:导入相关的Java类库要使用Java来获取文件,我们需要导入相关的Java类库。
在这个场景下,我们需要导入java.io类库中的File类。
在Java中,File类提供了一些方法来操作文件和目录。
要导入File类,我们可以在Java源文件的开头添加以下代码:javaimport java.io.File;第二步:创建File对象在Java中,要获取文件,我们需要先创建一个File对象。
File对象代表文件系统中的一个文件或目录。
我们可以使用其构造函数来创建一个File 对象,构造函数可以接受文件路径作为参数。
以下是一个创建File对象的示例代码:javaString path = "C:\\myFolder\\myFile.txt";File file = new File(path);在上面的示例中,我们创建了一个名为file的File对象,该对象代表了路径为C:\myFolder\myFile.txt的文件。
请注意,在Java中,文件路径使用双反斜杠(\)来表示文件分隔符。
第三步:检查文件是否存在在创建File对象后,我们可以使用其exists()方法来检查文件是否存在。
exists()方法返回一个布尔值,如果文件存在,则返回true,否则返回false。
以下是一个检查文件是否存在的示例代码:javaif (file.exists()) {System.out.println("文件存在");} else {System.out.println("文件不存在");}第四步:获取文件的绝对路径要获取文件的绝对路径,我们可以使用File对象的getAbsolutePath()方法。
java读取xml文件内容
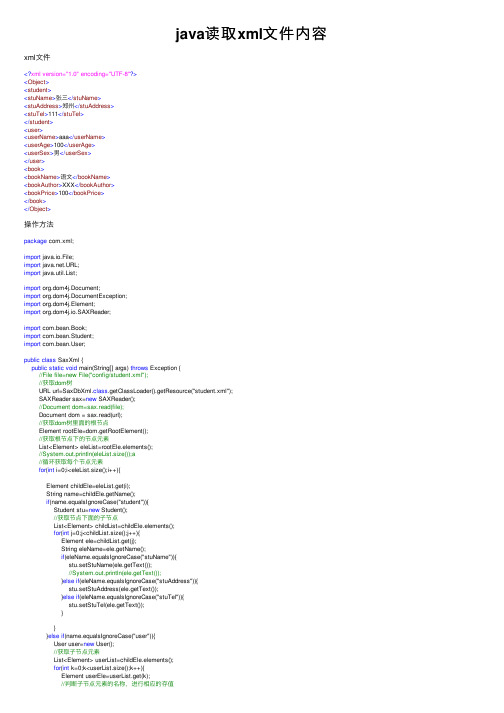
java读取xml⽂件内容xml⽂件<?xml version="1.0" encoding="UTF-8"?><Object><student><stuName>张三</stuName><stuAddress>郑州</stuAddress><stuTel>111</stuTel></student><user><userName>aaa</userName><userAge>100</userAge><userSex>男</userSex></user><book><bookName>语⽂</bookName><bookAuthor>XXX</bookAuthor><bookPrice>100</bookPrice></book></Object>操作⽅法package com.xml;import java.io.File;import .URL;import java.util.List;import org.dom4j.Document;import org.dom4j.DocumentException;import org.dom4j.Element;import org.dom4j.io.SAXReader;import com.bean.Book;import com.bean.Student;import er;public class SaxXml {public static void main(String[] args) throws Exception {//File file=new File("config/student.xml");//获取dom树URL url=SaxDbXml.class.getClassLoader().getResource("student.xml");SAXReader sax=new SAXReader();//Document dom=sax.read(file);Document dom = sax.read(url);//获取dom树⾥⾯的根节点Element rootEle=dom.getRootElement();//获取根节点下的节点元素List<Element> eleList=rootEle.elements();//System.out.println(eleList.size());a//循环获取每个节点元素for(int i=0;i<eleList.size();i++){Element childEle=eleList.get(i);String name=childEle.getName();if(name.equalsIgnoreCase("student")){Student stu=new Student();//获取节点下⾯的⼦节点List<Element> childList=childEle.elements();for(int j=0;j<childList.size();j++){Element ele=childList.get(j);String eleName=ele.getName();if(eleName.equalsIgnoreCase("stuName")){stu.setStuName(ele.getText());//System.out.println(ele.getText());}else if(eleName.equalsIgnoreCase("stuAddress")){stu.setStuAddress(ele.getText());}else if(eleName.equalsIgnoreCase("stuTel")){stu.setStuTel(ele.getText());}}}else if(name.equalsIgnoreCase("user")){User user=new User();//获取⼦节点元素List<Element> userList=childEle.elements();for(int k=0;k<userList.size();k++){Element userEle=userList.get(k);//判断⼦节点元素的名称,进⾏相应的存值if(userEle.getName().equalsIgnoreCase("userName")){user.setUserName(userEle.getText());}else if(userEle.getName().equalsIgnoreCase("userSex")){ user.setUserSex(userEle.getText());}else if(userEle.getName().equalsIgnoreCase("userAge")){ user.setUserAge(userEle.getText());}}}else if(name.equalsIgnoreCase("book")){Book book=new Book();List<Element> bookList=childEle.elements();for(int k=0;k<bookList.size();k++){Element bookEle=bookList.get(k);String eleName=bookEle.getName();if(eleName.equalsIgnoreCase("bookName")){book.setBookName(bookEle.getText());}else if(eleName.equalsIgnoreCase("bookAuthor")){book.setBookAuthor(bookEle.getText());}else if(eleName.equalsIgnoreCase("bookPrice")){book.setBookPrice(bookEle.getText());}}}}}}。
java读取txt文件

java读取txt⽂件⼀、读取txt⽂件。
1、步骤 :①、创建⽂件句柄File file = new File(filePath);②、将⽂件内容读取到内存中new FileInputStream(file)③、封装数据 InputStreamReaderInputStreamReader read = new InputStreamReader(new FileInputStream(file), encoding)④、读取数据BufferedReader bufferedReader = new BufferedReader(read); 每⾏数据bufferedReader.readLine()2、场景: txt⽂件内容:123456...读取每⾏内容, 并将每⾏内容⽤ , 拼接成 123,456public class ReadTxt {public static void main(String[] args) {String filePath = "/Users/xupengwei/Downloads/test.txt";readTxtFile(filePath );}public static void readTxtFile(String filePath) {try {String encoding = "GBK";File file = new File(filePath);if (file.isFile() && file.exists()) { // 判断⽂件是否存在InputStreamReader read = new InputStreamReader(new FileInputStream(file), encoding);// 考虑到编码格式 BufferedReader bufferedReader = new BufferedReader(read);String lineTxt = null;StringBuffer sb = new StringBuffer();while ((lineTxt = bufferedReader.readLine()) != null) {sb.append(lineTxt);sb.append(",");}System.out.println(sb.toString());read.close();} else {System.out.println("找不到指定的⽂件");}} catch (Exception e) {System.out.println("读取⽂件内容出错");e.printStackTrace();}}}⼆、拓展...场景 : 正则匹配。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
package tools;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
public class Dome {
public static void main(String[] args) throws FileNotFoundException { // 读取文件的位置
File file = new File("D:/java/answer/src/fiel.txt");
// 输出文件的位置
File brs = new File("C:/sss.txt");
BufferedWriter out = null;
BufferedReader br = null;
try {
br = new BufferedReader(new FileReader(file));
out = new BufferedWriter(new FileWriter(brs));
int i = 0;
Integer index = 0;
while (br.ready()) {
String str = br.readLine();
if (i % 3 == 0) {
StringBuffer bf = new StringBuffer(str);
String st = bf.substring(bf.indexOf(".") + 1, stIndexOf(")"));
String ok = st.substring(st.indexOf("(") + 1, st.length());
String sts = st.substring(0, st.indexOf("("));
System.out.print(sts + "\t");
out.write(sts.trim() + "\t");
if (ok.trim().equals("A")) {
index = 1;
} else if (ok.trim().equals("B")) {
index = 2;
} else if (ok.trim().equals("C")) {
index = 3;
} else {
index = 4;
}
} else if (i % 1 == 0) {
if (str.contains("A") & str.contains("B")) {
System.out.print(str.substring(str.indexOf("A.") + 2,str.indexOf("B")) + "\t");
out.write(str.substring(str.indexOf("A.") + 2,str.indexOf("B")).trim()+ "\t");
System.out.print(str.substring(str.indexOf("B.") + 2,str.length()) + "\t");
out.write(str.substring(str.indexOf("B.") + 2,str.length()).trim()+ "\t");
} else {
System.out.print(str.substring(str.indexOf("C.") + 2,str.indexOf("D")) + "\t");
out.write(str.substring(str.indexOf("C.") + 2,str.indexOf("D")).trim()+ "\t");
System.out.print(str.substring(str.indexOf("D.") + 2,str.length()) + "\t");
out.write(str.substring(str.indexOf("D.") + 2,str.length()).trim()+ "\t");
System.out.println(index);
out.write(index.toString());
out.newLine();
}
}
i++;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if(out !=null){
out.flush();
out.close();
}
if(br !=null)
br.close();
} catch (Exception e2) {
e2.printStackTrace();
}
}
}
}
用于提取考试题,只提取问题、答案、对应的正确答案
1.学习秘书实务的根本目的是(D )1-10
A. 掌握秘书专业知识
B. 提高秘书办事能力
C. 获得秘书职业资格
D. 培养学生专业素质
2.秘书学与应用写作学的关系是(D )1-10
A. 包容关系
B. 并列关系
C. 虚实关系
D. 交叉关系
3.秘书活动的随机性,决定了秘书工作( C )2-23
A. 必须规范性
B. 必须程序性
C. 不可预测性
D. 不可计划性
MYSQL
用LOAD DATA INFILE命令批量把数据添加到数据库中
语法:
LOAD DATA INFILE “文件本地地址例如:C:/xxxx.txt" INTO TABLE tableName;
OR
LOAD DATA INFILE “文件本地地址例如:C:/xxxx.txt" INTO TABLE tableName(column1,column2…………);。