面向对象技术 第七章继承和多态.jsp
第七章 面向对象的Java实现-继承和多态

Java教员
.NET教员
10
为什么需要继承 4-4
public class JavaTeacher2 extends Teacher { public JavaTeacher2(String myName, String mySchool) { super(myName, mySchool); 子类自动继承父类的属 } 性和方法,子类中不再 public void giveLesson(){ 存在重复代码 System.out.println("启动 Eclipse"); super.giveLesson(); } } public class DotNetTeacher2 extends Teacher { public DotNetTeacher2(String myName, String mySchool) { super(myName, mySchool); } public void giveLesson(){ System.out.println("启动 Visual Studio .NET"); super.giveLesson(); } } 使用继承,可以有效实现代码复用
4
本章目标
掌握继承 掌握super关键字 掌握多态
5
生活中的继承 2-1
生活中,继承的例子随处可见
动物 谁是父类? 谁是子类? 能说出继承的特点吗?
食草动物
食肉动物
继承需要符合的关系:is-a,父类更通用、子类更具体
6
生活中的继承 2-2
子类具有父类的一般特性(包括属性和行为), 以及自身特殊的特性
可以接收子类类型 public class HQ3 { public void judge(Teacher t){ t.introduction(); t.giveLesson(); 根据实际创建的对象 } 类型调用相应方法 大家好!我是北京中心的李明. 启动 Eclipse 知识点讲解 总结提问
面向对象程序设计——员工继承关系程序设计
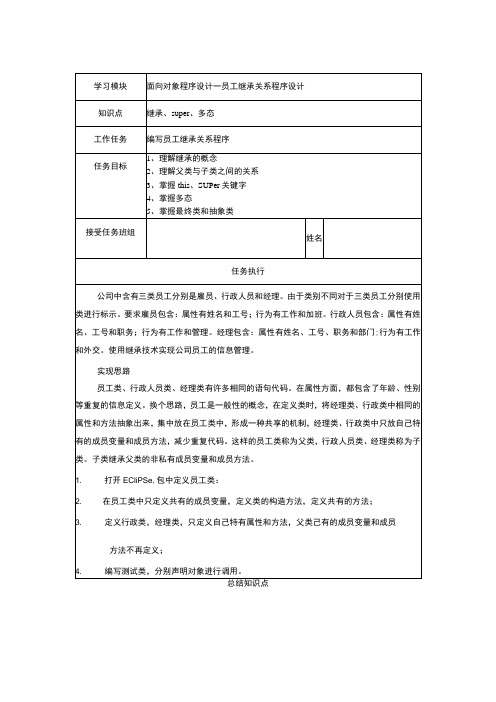
学生根据对执行任务过程进行自评,给出相应的成绩,占10%
学生互评
学生间根据课堂口语表达及表现,互相评价,占10%
教师评价
教师根据对学生的学习态度、出勤、课堂表现、沟通与表达能力,并结合执行任务过程的各个环节进行评价,占20驰
合计
1.打开ECliPSe,包中定义员工类:
2.在员工类中只定义共有的成员变量,定义类的构造方法,定义共有的方法;
3.定义行政类,经理类,只定义自己特有属性和方法,父类己有的成员变量和成员
方法不再定义;
4.编写测试类,分别声明对象进行调用。
总结知识点
评价
类别
比例
成绩
签名
成果评定
根据学习成果评定成绩,占60%
实现思路
员工类、行政人员类、经理类有许多相同的语句代码。在属性方面,都包含了年龄、性别等重复的信息定义。换个思路,员工是一般性的概念,在定义类时,将经理类、行政类中相同的属性和方法抽象出来,集中放在员工类中,形成一种共享的机制,经理类、行政类中只放自己特有的成员变量和成员方法,减少重复代码。这样的员工类称为父类,行政人员类、经理类称为子类。子类继承父类的非私有成员变量和成员方法。
学习模块
面向对象程序设计一员工继承关系程序设计
知识点
继承、super、多态
工作任务
编写员工继承关系程序
任务目标
1、理解继承的概念
2、理解父类与子类之间的关系
3、掌握this、SUPer关键字
4、掌握多态
5、掌握最终类和抽象类
接受任务班组
姓名
任务行
公司中含有三类员工分别是雇员、行政人员和经理。由于类别不同对于三类员工分别使用类进行标示。要求雇员包含:属性有姓名和工号;行为有工作和加班。行政人员包含:属性有姓名、工号和职务;行为有工作和管理。经理包含:属性有姓名、工号、职务和部门;行为有工作和外交。使用继承技术实现公司员工的信息管理。
面向对象知识点总结
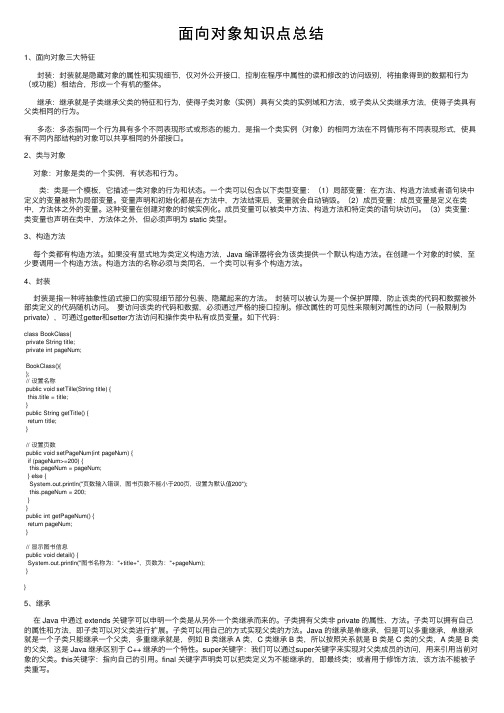
⾯向对象知识点总结1、⾯向对象三⼤特征封装:封装就是隐藏对象的属性和实现细节,仅对外公开接⼝,控制在程序中属性的读和修改的访问级别,将抽象得到的数据和⾏为(或功能)相结合,形成⼀个有机的整体。
继承:继承就是⼦类继承⽗类的特征和⾏为,使得⼦类对象(实例)具有⽗类的实例域和⽅法,或⼦类从⽗类继承⽅法,使得⼦类具有⽗类相同的⾏为。
多态:多态指同⼀个⾏为具有多个不同表现形式或形态的能⼒,是指⼀个类实例(对象)的相同⽅法在不同情形有不同表现形式,使具有不同内部结构的对象可以共享相同的外部接⼝。
2、类与对象对象:对象是类的⼀个实例,有状态和⾏为。
类:类是⼀个模板,它描述⼀类对象的⾏为和状态。
⼀个类可以包含以下类型变量:(1)局部变量:在⽅法、构造⽅法或者语句块中定义的变量被称为局部变量。
变量声明和初始化都是在⽅法中,⽅法结束后,变量就会⾃动销毁。
(2)成员变量:成员变量是定义在类中,⽅法体之外的变量。
这种变量在创建对象的时候实例化。
成员变量可以被类中⽅法、构造⽅法和特定类的语句块访问。
(3)类变量:类变量也声明在类中,⽅法体之外,但必须声明为 static 类型。
3、构造⽅法每个类都有构造⽅法。
如果没有显式地为类定义构造⽅法,Java 编译器将会为该类提供⼀个默认构造⽅法。
在创建⼀个对象的时候,⾄少要调⽤⼀个构造⽅法。
构造⽅法的名称必须与类同名,⼀个类可以有多个构造⽅法。
4、封装封装是指⼀种将抽象性函式接⼝的实现细节部分包装、隐藏起来的⽅法。
封装可以被认为是⼀个保护屏障,防⽌该类的代码和数据被外部类定义的代码随机访问。
要访问该类的代码和数据,必须通过严格的接⼝控制。
修改属性的可见性来限制对属性的访问(⼀般限制为private),可通过getter和setter⽅法访问和操作类中私有成员变量。
如下代码:class BookClass{private String title;private int pageNum;BookClass(){};// 设置名称public void setTille(String title) {this.title = title;}public String getTitle() {return title;}// 设置页数public void setPageNum(int pageNum) {if (pageNum>=200) {this.pageNum = pageNum;} else {System.out.println("页数输⼊错误,图书页数不能⼩于200页,设置为默认值200");this.pageNum = 200;}}public int getPageNum() {return pageNum;}// 显⽰图书信息public void detail() {System.out.println("图书名称为:"+title+",页数为:"+pageNum);}}5、继承在 Java 中通过 extends 关键字可以申明⼀个类是从另外⼀个类继承⽽来的。
面向对象的三大特性(封装-继承-多态)
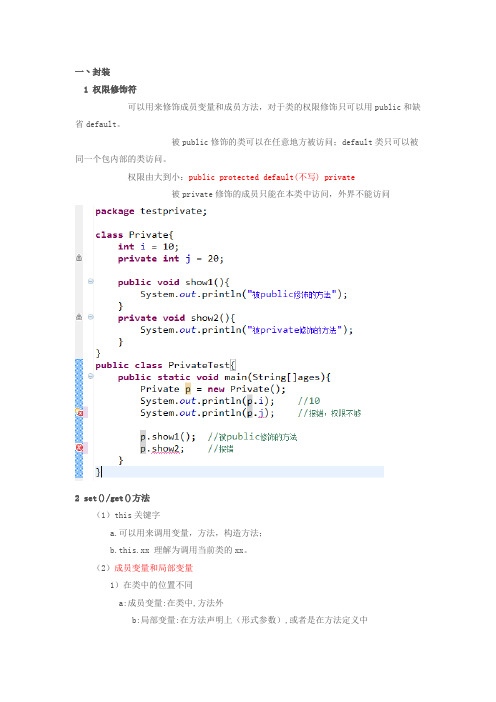
一丶封装1 权限修饰符可以用来修饰成员变量和成员方法,对于类的权限修饰只可以用public和缺省default。
被public修饰的类可以在任意地方被访问;default类只可以被同一个包内部的类访问。
权限由大到小:public protected default(不写) private被private修饰的成员只能在本类中访问,外界不能访问2 set()/get()方法(1)this关键字a.可以用来调用变量,方法,构造方法;b.this.xx 理解为调用当前类的xx。
(2)成员变量和局部变量1)在类中的位置不同a:成员变量:在类中,方法外b:局部变量:在方法声明上(形式参数),或者是在方法定义中2)在内存中的位置不同a:成员变量:在堆内存b:局部变量:在栈内存3)生命周期不同a:成员变量:随着对象的创建而存在,随着对象的消失而消失b:局部变量:随着方法调用而存在,随着方法的调用结束而消失4)初始化值不同a:成员变量:有默认值b:局部变量:必须初始化值,否则报错!(在使用它之前,没有初始化) (3)set()/get()方法当成员变量被private修饰时,不在本类中无法直接访问,便需要set()/get()方法来解决这个问题3 封装性封装:是面向对象的第一大特性,所谓封装,就是值对外部不可见(一般而言被private修饰),外部只能通过对象提供的接口(如set()/get()方法)来访问。
封装的好处:a.良好的封装能够减少耦合;b.类内部的结构可以自己修改,对外部影响不大;c.可以对成员进行更精准的控制(防止出现与事实不符的情况);d.影藏实现细节。
注意:在开发中,类的成员变量全部要进行封装,封装之后通过set()/get()方法访问。
二丶继承extends1 实现:通过 class Zi extends Fu{} 实现类的继承(1)子类继承父类,父类中声明的属性,方法,子类都可以获取到;当父类中有私有的属性方法时,子类同样可以获取到,由于封装性的设计,使得子类不能直接调用访问。
封装、继承和多态的概念

封装、继承和多态的概念
封装、继承和多态是面向对象编程中的三个重要概念,下面分别进行详细解释:
一、封装
封装是指将对象的属性和方法封装在一起,形成一个独立的单元,对外部隐藏对象的实现细节,只暴露必要的接口供外部使用。
封装可以有效地保护对象的数据和行为,避免外部的误操作和非法访问,提高了代码的安全性和可维护性。
在面向对象编程中,封装是实现信息隐藏和数据保护的重要手段。
二、继承
继承是指一个类可以从另一个类中继承属性和方法,从而可以重用已有的代码和功能。
继承是面向对象编程中实现代码复用的重要手段,可以减少代码的重复性,提高代码的可读性和可维护性。
继承可以分为单继承和多继承两种方式,单继承是指一个类只能从一个父类中继承,而多继承是指一个类可以从多个父类中继承属性和方法。
三、多态
多态是指同一个方法在不同的对象上可以有不同的行为,即同一个方法可以有多
种不同的实现方式。
多态是面向对象编程中的重要概念,可以提高代码的灵活性和可扩展性。
多态可以分为编译时多态和运行时多态两种方式,编译时多态是指方法的重载,即同一个类中可以有多个同名但参数不同的方法;而运行时多态是指方法的重写,即子类可以重写父类的方法,从而实现不同的行为。
通过多态,可以实现面向对象编程中的“开闭原则”,即对扩展开放,对修改关闭。
简述面向对象中的多态

简述面向对象中的多态
面向对象中的多态是指一个对象可以以多种形态存在。
简单来说,多态就是同一个方法可以有不同的实现方式。
在面向对象的程序设计中,多态是一种非常重要的概念。
它能够提高代码的灵活性和可扩展性,使得代码更易于维护和扩展。
多态的实现方式主要有两种:静态多态和动态多态。
静态多态是通过函数的重载和运算符的重载来实现的。
函数的重载是指在同一个类中定义多个同名函数,但这些函数具有不同的参数列表。
运算符的重载是指对于某个运算符,可以定义多个不同的操作方式。
在编译时,编译器会根据调用时的参数类型来确定具体调用的函数或运算符。
动态多态是通过继承和虚函数来实现的。
继承是指子类可以继承父类的属性和方法,通过定义一个指向父类对象的指针或引用,可以调用子类对象中重写的方法。
虚函数是在父类中声明为虚函数的函数,子类可以对其进行重写。
在运行时,根据实际对象的类型来调用相应的方法。
多态具有很多优点。
首先,它可以提高代码的重用性,一个类的方法可以被多个类继承并重写,这样可以减少代码的重复编写。
其次,多态可以使代码更加灵活,可以根据需要动态地根据对象的类型来调用相应的方法。
再次,多态可以提高代
码的可扩展性,当需要添加新的功能时,只需要在子类中重写相应的方法即可,而不需要修改已有的代码。
总之,多态是面向对象编程中非常重要的概念,通过使用多态可以使代码更灵活、可扩展和易于维护。
它是面向对象编程中的重要特性之一,值得我们深入理解和应用。
面向对象的继承原理是什么
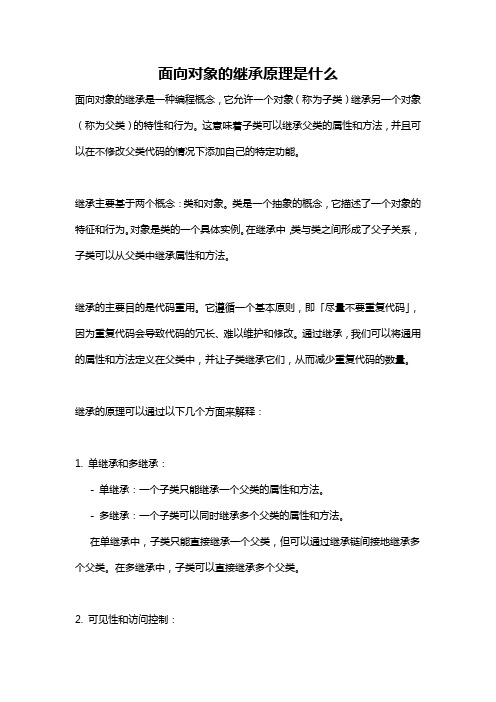
面向对象的继承原理是什么面向对象的继承是一种编程概念,它允许一个对象(称为子类)继承另一个对象(称为父类)的特性和行为。
这意味着子类可以继承父类的属性和方法,并且可以在不修改父类代码的情况下添加自己的特定功能。
继承主要基于两个概念:类和对象。
类是一个抽象的概念,它描述了一个对象的特征和行为。
对象是类的一个具体实例。
在继承中,类与类之间形成了父子关系,子类可以从父类中继承属性和方法。
继承的主要目的是代码重用。
它遵循一个基本原则,即「尽量不要重复代码」,因为重复代码会导致代码的冗长、难以维护和修改。
通过继承,我们可以将通用的属性和方法定义在父类中,并让子类继承它们,从而减少重复代码的数量。
继承的原理可以通过以下几个方面来解释:1. 单继承和多继承:- 单继承:一个子类只能继承一个父类的属性和方法。
- 多继承:一个子类可以同时继承多个父类的属性和方法。
在单继承中,子类只能直接继承一个父类,但可以通过继承链间接地继承多个父类。
在多继承中,子类可以直接继承多个父类。
2. 可见性和访问控制:继承关系中,父类的属性和方法可以被子类继承和访问。
然而,有时我们希望将某些属性或方法隐藏起来,只允许在父类内部访问。
这就引出了可见性和访问控制的概念。
- 公共可见性:表示属性和方法允许在类的内部和外部访问。
- 受保护的可见性:表示属性和方法只允许在类的内部和子类中访问。
- 私有可见性:表示属性和方法只允许在类的内部访问。
可见性和访问控制允许我们灵活地控制子类对父类的继承内容的访问权限。
3. 方法重写和多态:子类有权重写继承自父类的方法,以满足自身的需求。
这被称为方法重写。
在方法重写中,子类可以实现与父类相同的方法名称、参数列表和返回类型,从而覆盖父类的方法。
多态是指同一个方法调用可以具有不同的实现方式。
通过方法重写,子类可以以不同的方式实现继承自父类的方法。
当我们通过一个父类类型的变量引用一个子类对象时,可以根据实际运行时的子类类型来决定调用哪个方法。
java的封装,继承和多态(思维导图)
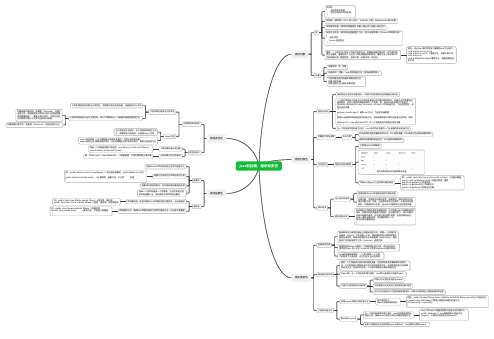
java的封装,继承和多态类和对象类类声明 { 成员变量的声明; 成员方法的声明及实现; }声明类:[修饰符] class 类<泛型> [extends 父类] [implements 接口列表]声明成员变量:[修饰符]数据类型 变量[=表达式]{,变量[=表达式]}成员方法声明:[修饰符]返回值类型 方法([形式参数列表])[throws 异常类列表]{语句序列;[return[返回值]]; }重载:一个类中可以有多个同名的成员方法,前提是参数列表不同,称为类的成员方法重载,重载的多个方法为一种功能提供多种实现。
重载方法之间必须以不同的参数列表(数据类型、参数个数、参数次序)来区别。
例如,MyDate 类可声明多个重载的set()方法如下: void set(int y,int m, int d)void set(int m, int d) //重载方法,参数个数不同void set(int d) void set(MyDate date)//重载方法,参数的数据类型不同对象对象声明:类 对象构造实例:对象 = new 类的构造方法([实际参数列表])引用对象的成员变量和调用成员方法:对象.成员变量 对象.成员方法([实际参数列表])类的封装性构造与析构类的构造方法用于创建类的一个实例并对实例的成员变量进行初始化一个类可声明多个构造方法对成员变量进行不同需求的初始化,构造方法不需要写返回值类型,因为它返回的就是该类的一个实例。
例:MyDate类声明以下构造方法:public MyDate(int year, int month, int day)// 声明构造方法,方法名同类名,初始化成员变量 {set(year, month day);// 调用 set()方法,为成员变量赋值}使用new运算符调用指定类的构造方法,实际参数列表必须符合构造方法声明。
例如:MyDate d1 = new MyDate(2017,10,1);//创建实例并初始化成员变量当一个类没有声明构造方法时,Java 自动为该类提供一个无参数的默认构造方法对象的引用与运算this引用访问本类的成员变量和成员方法:this.成员变量,this.成员方法([实际参数列表])调用本类重载的构造方法:this([实际参数列表])访问控制类的访问控制权限公有和(public)和缺省类中成员4级访问控制权限及范围声明set()和get()方法存取对象的属性例:public void set(int year, int month, int day) //设置日期值 public void set(MyDate date)//设置日期值,重载 public int getYear()//获得年份 public int getMonth()// 获得月份 public int getDay()//获得当月日期静态成员定义及访问格式使用关键字static声明的成员称为静态成员在类内部,可直接访问静态成员,省略类名。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
// ax7_5{ private int x,y; protected int play(int s,int t) { 使用 int m; protected定 x=s; y=t; 义的成员变量 m=(x>y)?x/y:y/x; 或方法具有二 return m; 重性,类本身、 } } 子类或包中的 public class GetNum7_5 方法可以访问 { 它们,而其它 public static void main(String args[]) { 类没有访问权 int result; 限 Max7_5 ss=new Max7_5(); result=ss.play(5,45); System.out.println(" result= "+result); } }
//使用import引入已经定义的,属性为public的 Account7_3类 ,引用其方法 import bag.Account7_3 ; //引用公共类Account7_3 public class Useraccount7_3 { public static void main(String args[]) { Account7_3 myaccount=new Account7_3(); //创建类对象 ="张三 "; 通过import引用在本类 myaccount.address="银川市北京路100号 "; 中可以直接建立相应的 myaccount.balance=123.40; 类对象,并应用其中的 myaccount.display(); 具有public权限的变量 } } 和方法
编译此程序,要给Account7_3.class文件定位:附件一 D:\Javakj\Javalt>Javac –classpath d:\Javakj\Javalt Useraccount7_3.Java 程序运行结果 name:张三 address:西安市兴庆路19号 balance:123.4
多态机制
多态机制是面向对象程序设计的一个重要特征。Java 的 多态机制主要表现在方法的重载、构造方法的重载以及方法 的覆盖几个方面。 多态的特点是采用同名的方式,根据调用方法时传送的参 数的多少以及传送参数类型的不同,调用不同的方法,这样对 于类的编制而言,可以采用同样的方法获得不同的行为特征。 所谓方法的重载,就是在类中创建了多个方法,它们具有相 同的名称,但有不同的参数和不同的定义。在调用方法时依据 其参数个数及类型自动选择相匹配的方法去执行。达到各种对 象处理类似问题时具有统一的接口之目的。
//定义构造方法的重载
Public class Point{
protected int x,y; public Point( ){setPoint(0,0);} public Point(int a,int b){setPoint(a,b);} piblic void setPoint(int a,int b){ x=a; Y=b; } public int getx( ){return x;} public int gety( ){return y;}
不 想 让 其 它 类 访 问 就 设 置 为 私 有 权 限
// 创建私有方法,在构造方法中引用方法,私有变量的引用权限 class Scope7_4{ private int x=10; Scope7_4( ) { int x=1000; a( ); b( ); } private void a( ) { int x=200; x*=4; System.out.println("A of a method is "+x); } private void b( ) { x*=2; System.out.println("B of a method is "+x); } } public class Samp7_4 { public static void main(String args[]) { int x=100; System.out.println("x of main method is "+x); Scope7_4 b1=new Scope7_4( ); // b1.a( );//方法a()已被构造函数初始化 // b1.b( ); System.out.println("x of main method is "+x); } }
// 定义多个同名方法, 实现的重载方法 // 这些方法具有相同的名称,但有不同的参数和不同的定义 public class Calculate7_7 { public static int abs1(int x) {//定义整数运算的方法 int y; y=x; if(y<0) y=-y+5; return y; } public static double abs1(double x) {//定义双精数运算的方法 double y; y=x; if(y<0) y=-y*2; return y; } public static void main(String args[] ) { int m=-25; double n=-8.27; System.out.println("m="+m+" "+abs1(m)); System.out.println("n="+n+" "+abs1(n)); } }
public 修饰的类是公共类,修饰的变量是公共变量, 修饰的方法是公共方法。这些类、变量、方法可以 供其它类访问。 一个Java程序文件只能定义一个public类,而且程 序文件必须与它同名。为其它程序共享的类须经过 编译进行打包,形成一个包文件,然后用import语 句加以引用。
打包是在Java程序编译时进行的,注意参数-d 被编译程序所在路径 Javac –d 被编译程序所在路径 被编译程序 名.Java 被编译程序的第一行用package 包名 ;给出它被打 入的包,该包与系统创建的子目录同名。
// 将x17_6类独立建成X17_6.Java类文件,放入bag包中以便其他程序直接调用 //编译命令为 D:\Java程序设计例题> Javac -d D:\Java程序设计例题 X17_6.Java package bag; public class X17_6{ public X17_6(){ System.out.println("this is a construct"); } public void doing( ) { System.out.println("could you tell me"); } // 引用包bag中的X17_6.Java类文件 } import bag.X17_6; public class X27_6{ 未设定public或 static void store(){ private等访问 System.out.println("this is a store processing"); 权限的均为 } friendly,包内 public static void main(String args[]) 的所有类可以 { 访问它们,包 X17_6 y=new X17_6(); 外的不行 y.doing(); store(); } }
// 定义 属性为public的 Account7_3类 ,及其引用其方法,使用编译命令 // D:\Java程序设计例题> Javac -d D:\Java程序设计例题 Account7_3.Java package bag ; // 创建包bag ,将类放入bag包中 public class Account7_3 { public String name; public String address; 这里创建了一个包其 public double balance; 名字叫bag,并且将编 public void display() 译后的 { Account7_3.class 文 System.out.print(" name:"); 件装入包中 System.out.println(name); System.out.print(" Address:"); System.out.println( address); System.out.print(" balance:"); System.out.println( balance); } }
对象变量的四种访问权限 public, protected, private , friendly.
//引用私有方法和修改私有成员变量 class def{ private int x,y,z=0; private void method1(){ int x=3,y=4,z; z=x*y; System.out.println("z="+z); } public void show() { System.out.println("z="+z); } } public class Change7_1{ public static void main(String args[]) { def ss=new def(); ss.method1();//调用私有方法出错 ss.z=12;//修改私有变量出错 ss.show(); } }
//引用私有方法和修改私有成员变量 class def{ private int x,y,z=0; public void method1(){ int x=3,y=4,z; z=x*y; System.out.println("z="+z); } public void show() { System.out.println("z="+z); } } public class Change7_2{ public static void main(String args[]) { int z=20; def ss=new def(); ss.method1(); ss.show(); } }