java+JDBC小项目《学生管理系统》源码带注解
(完整版)JAVA学生管理系统源代码

JA V A学生管理系统源代码一、程序import java.util.*;public class Test {//主程序public static void main(String[] args){Scanner in = new Scanner(System.in);System.out.println("------请定义学生的人数:------");Student[] stuArr = new Student[in.nextInt()];Admin adminStu = new Admin();while(true){System.out.println("-----请选择你要执行的功能-----");System.out.println("10:添加一个学生");System.out.println("11:查找一个学生");System.out.println("12:根据学生编号更新学生基本信息");System.out.println("13:根据学生编号删除学生");System.out.println("14:根据编号输入学生各门成绩");System.out.println("15:根据某门成绩进行排序");System.out.println("16:根据总分进行排序");System.out.println("99:退出系统");String number = in.next();if(number.equals("10")){System.out.println("请输入学生的编号:");int num = in.nextInt();System.out.println("请输入学生的姓名:");String name = in.next();System.out.println("请输入学生的年龄:");int age = in.nextInt();adminStu.Create(num,name,age,stuArr);//添加学生}else if(number.equals("11")){System.out.println("执行查找学生基本信息的操作");System.out.println("请输入学生的编号进行查找:");int num = in.nextInt();adminStu.find(num,stuArr);//查找学生}else if(number.equals("12")){System.out.println("执行更新学员的基本信息操作");System.out.println("请输入学生的编号:");int num = in.nextInt();System.out.println("请输入学生的姓名:");String name = in.next();System.out.println("请输入学生的年龄:");int age = in.nextInt();adminStu.update(num,name,age,stuArr);//更新学生基本信息}else if(number.equals("13")){System.out.println("执行删除学生操作");System.out.println("请输入学生编号:");int num = in.nextInt();adminStu.delete(num,stuArr);//删除学生}else if(number.equals("14")){System.out.println("执行输入成绩操作");System.out.println("请输入学生编号:");int num = in.nextInt();adminStu.input(num, stuArr);//输入成绩}else if(number.equals("15")){System.out.println("执行根据某科目成绩排序操作");System.out.println("请选择需要排序的科目名(1.java 2.C# 3.html 4.sql):");int num = in.nextInt();adminStu.courseSort(num,stuArr);//按科目排序}else if(number.equals("16")){System.out.println("执行根据总分排序操作");adminStu.sumSort(stuArr);//按总分排序}else if(number.equals("99")){System.out.println("--------程序已退出--------");//break;System.exit(0);}}}}二、学生类public class Student {//学生类private int num;private String name;private int age;private int java;private int C;private int html;private int sql;private int sum;private int avg;public int getNum() {return num;}public void setNum(int num) { this.num = num;}public String getName() {return name;}public void setName(String name) { = name;}public int getAge() {return age;}public void setAge(int age) { this.age = age;}public int getJava() {return java;}public void setJava(int java) { this.java = java;}public int getC() {return C;}public void setC(int c) {C = c;}public int getHtml() {return html;}public void setHtml(int html) { this.html = html;}public int getSql() {return sql;}public void setSql(int sql) { this.sql = sql;}public int getSum() {return sum;}public void setSum() {this.sum = this.java+this.C+this.html+this.sql;}public int getAvg() {return avg;}public void setAvg() {this.avg = this.sum/4;}public String toString(){String str= "\t"+this.num+"\t"++"\t"+this.age+"\t"+this.java+"\t"+this.C+"\t"+this.html+"\t"+this.sql+"\t"+this.sum+"\t"+this.avg;return str;}}三、管理学生类import java.util.*;public class Admin {//用来管理学生的一个类String msg = "\t编号\t姓名\t年龄\tjava\tC#\thtml\tsql\t总分\t 平均分";public void print(Student[] arr){//刷新数据的方法System.out.println(msg);for(int i=0;i<arr.length;i++){if(arr[i]!=null){arr[i].setSum();arr[i].setAvg();System.out.println(arr[i]);}}}public boolean exist(int num,Student stu){//判断学生是否存在的方法if(stu!=null){if(stu.getNum()==num){return true;}else{return false;}}return false;}public void Create(int num,String name,int age,Student[] arr){//添加学生的方法Student stu = new Student();stu.setNum(num);stu.setName(name);stu.setAge(age);int i = this.setIndex(arr);if(i==99999){System.out.println("学生人数已添满,不能再添加了");}else{arr[i]=stu;}this.print(arr);}public int setIndex(Student[] arr){//返回数组为空的下标for(int i=0;i<arr.length;i++){if(arr[i]==null){return i;}}return 99999;}public void find(int num,Student[] arr){//查询学生的方法for(int i=0;i<arr.length;i++){//判断学生是否存在if(this.exist(num,arr[i])==true){System.out.println(msg);System.out.println(arr[i]);return;}}System.out.println("-----没有这个学生的存在-----");}public void update(int num,String name,int age,Student[] arr){//更新学生基本信息的方法for(int i=0;i<arr.length;i++){if(this.exist(num, arr[i])==true){arr[i].setName(name);arr[i].setAge(age);System.out.println("--------更新学生信息成功!-------");this.print(arr);return;}}System.out.println("------没找到这个学生更新信息失败------");}public void delete(int num,Student[] arr){//删除学生的方法for(int i=0;i<arr.length;i++){if(this.exist(num,arr[i])){arr[i] = null;this.print(arr);return;}}System.out.println("您所指定编号的学生不存在");}public void input(int num,Student[] arr){//输入学生成绩的方法for(int i=0;i<arr.length;i++){if(this.exist(num, arr[i])){Scanner in = new Scanner(System.in);System.out.println("请输入"+arr[i].getName()+"java 的成绩:");if(in.hasNextInt()){//输入非整形数则不执行arr[i].setJava(in.nextInt());}else{return;}System.out.println("请输入"+arr[i].getName()+"C#的成绩:");if(in.hasNextInt()){arr[i].setC(in.nextInt());}else{return;}System.out.println("请输入"+arr[i].getName()+"html 的成绩:");if(in.hasNextInt()){arr[i].setHtml(in.nextInt());}else{return;}System.out.println("请输入"+arr[i].getName()+"sql 的成绩:");if(in.hasNextInt()){arr[i].setSql(in.nextInt());}else{return;}this.print(arr);return;}}}public void courseSort(int num,Student[] arr){//根据指定科目排序的方法if(num==1){//这里不能用冒泡排序(因为冒泡排序是相邻的比较,而相邻的可能不存在,那么相隔的两个就不能交换)for(int i=0;i<arr.length;i++){for(int j=i+1;j<arr.length;j++){if(arr[i]!=null&&arr[j]!=null){if(arr[i].getJava()<arr[j].getJava()){Student t = arr[i];arr[i] = arr[j];arr[j] = t;}}}}}else if(num==2){for(int i=0;i<arr.length;i++){for(int j=i+1;j<arr.length;j++){if(arr[i]!=null&&arr[j]!=null){if(arr[i].getC()<arr[j].getC()){Student t = arr[i];arr[i] = arr[j];arr[j] = t;}}}}}else if(num==3){for(int i=0;i<arr.length;i++){for(int j=i+1;j<arr.length;j++){if(arr[i]!=null&&arr[j]!=null){if(arr[i].getHtml()<arr[j].getHtml()){Student t = arr[i];arr[i] = arr[j];arr[j] = t;}}}}}else if(num==4){for(int i=0;i<arr.length;i++){for(int j=i+1;j<arr.length;j++){if(arr[i]!=null&&arr[j]!=null){if(arr[i].getSql()<arr[j].getSql()){Student t = arr[i];arr[i] = arr[j];arr[j] = t;}}}}}this.print(arr);}public void sumSort(Student[] arr){//根据总分排序的方法for(int i=0;i<arr.length;i++){for(int j=i+1;j<arr.length;j++){if(arr[i]!=null&&arr[j]!=null){if(arr[i].getSum()<arr[j].getSum()){Student t = arr[i];arr[i] = arr[j];arr[j] = t;}}}}this.print(arr);}}。
java 编写简单的学生信息管理系统--附上源码

学生信息管理系统(Java版)废话不多说,源码如下:源码如下:/*学生信息管理系统,实现学生信息:*增加int[] a=new int[9]*删除*查找*更改*/import java.util.Scanner;//导入java输入流import ng.*;import java.io.*;class Student{private static Student[] s=new Student[2];int n=0;private String name;private int num;private String classAge;public void judge()throws IOException{int i;char ch;String str;Scanner In=new Scanner(System.in);if(n==0){System.out.print("你还没有录入任何学生,是否录入(Y/N):");str=In.next();ch=str.charAt(0);while(ch!='Y'&&ch!='y'&&ch!='N'&&ch!='n'){System.out.print("输入有误,请重新输入:");str=In.next();ch=str.charAt(0);}if(ch=='Y'||ch=='y'){this.add();}if(ch=='N'||ch=='n'){this.menu();}}}public void menu()throws IOException//定义菜单函数{int a;//定义switch语句变量Scanner in=new Scanner(System.in);//实例化输入流对象System.out.println("*********学生信息管理系统功能表*********"); System.out.println("***** 1.增加*****");System.out.println("***** 2.显示*****");System.out.println("***** 3.修改*****");System.out.println("***** 4.删除*****");System.out.println("***** 5.查看*****");System.out.println("***** 0.退出*****");System.out.println("****************************************"); System.out.print("请选择(0~5):");a=in.nextInt();while(a<0||a>5){System.out.print("输入超出范围,请重新输入:");a=in.nextInt();}switch(a){case 1:this.add();break;case 2:this.show();break;case 3:this.modif();break;case 4:this.delete();break;case 5:this.look();break;case 0:System.exit(0);break;}}public void add()throws IOException//定义增加函数{String str,str1,str2;int i,num1,t=1;char ch,ch1;FileWriter fw=new FileWriter("F://javaFile//student.txt",true);fw.write(" 录入的学生信息列表\r\n\r\n学号姓名班级\r\n"); Scanner In=new Scanner(System.in);while(t==1){System.out.print("请输入学生学号:");num1=In.nextInt();for(i=0;i<n;i++){while(s[i].num==num1){System.out.println("已存在此学号,请重新输入");System.out.print("请输入学号:");num1=In.nextInt();}}s[n].num=num1;str2=String.valueOf(num1);fw.write(str2+" ");System.out.println();System.out.print("请输入学生姓名:");s[n].name=In.next();fw.write(s[n].name+" ");System.out.println();System.out.print("请输入学生班级:");s[n].classAge=In.next();fw.write(s[n].classAge+"\r\n");++n;fw.close();System.out.println();System.out.print("是否继续添加(Y/N)");str=In.next();ch=str.charAt(0);while(ch!='N'&&ch!='n'&&ch!='Y'&&ch!='y'){System.out.print("输入有误,请重新输入:");str=In.next();ch=str.charAt(0);}if(ch=='N'||ch=='n'){break;}}System.out.println();System.out.print("是否返回主菜单(Y/N)");str1=In.next();ch1=str1.charAt(0);while(ch1!='Y'&&ch1!='y'&&ch1!='N'&&ch1!='n'){System.out.print("输入有误,请重新输入:");str1=In.next();ch1=str1.charAt(0);}if(ch1=='Y'||ch1=='y'){this.menu();}if(ch1=='N'||ch1=='n'){System.out.println("正在退出...谢谢使用!");System.exit(0);}}public void show()throws IOException{int i;this.judge();System.out.println("本次操作共录入"+n+"位学生!");System.out.println("你录入的学生信息如下:");System.out.println();System.out.println("学号\t\t姓名\t班级");for(i=0;i<n;i++){System.out.println(s[i].num+" "+s[i].name+" "+s[i].classAge);}System.out.println("系统返回主菜单!");this.menu();}public void delete()throws IOException//删除信息功能实现注:本功能暂时不具备可扩展性{this.judge();int j=0,t=0,k=0,num1;char ch;String str;Scanner pin=new Scanner(System.in);num1=pin.nextInt();for(j=0;j<n;j++){if(s[j].num==num1){k=1;t=j;}}if(k==0){System.out.println("对不起!你要删除的学号不存在!");System.out.println("系统将返回主菜单!");this.menu();}if(k==1){System.out.println("你要删除的学生信息如下:");//打印管理员要删除的学生信息System.out.println("学号\t姓名\t班级");//本功能暂时不备扩展性System.out.println(s[t].num+" "+s[t].name+" "+s[t].classAge);System.out.println();System.out.print("你确定要删除(Y/N):");str=pin.next();ch=str.charAt(0);while(ch!='Y'&&ch!='y'&&ch!='N'&&ch!='n'){System.out.print("输入有误,请重新输入:");str=pin.next();ch=str.charAt(0);}if(ch=='N'||ch=='n'){System.out.println();System.out.println("系统返回主菜单!");this.menu();}if(ch=='Y'||ch=='y'){for(j=t;j<n-1;j++){s[j]=s[j+1];}n--;System.out.println("系统返回主菜单!");this.menu();}}}public void look()throws IOException{FileReader fr=new FileReader("F://javaFile//student.txt"); int a;while((a=fr.read())!=-1){System.out.print((char)a);}fr.close();System.out.println("系统返回主菜单!");System.out.println();this.menu();}public void modif()throws IOException{this.judge();int j=0,t=0,k=0,num2,num3,moi,c=1;char ch;String str,str1,str2;Scanner pin=new Scanner(System.in);System.out.print("请输入要修改的学号:");num2=pin.nextInt();for(j=0;j<n;j++){if(s[j].num==num2){k=1;t=j;}}if(k==0){System.out.println("对不起!你要修改的学号不存在!"); System.out.println("系统将返回主菜单!");this.menu();}if(k==1){System.out.println("你要修改的学生信息如下:");//打印管理员要删除的学生信息System.out.println("学号\t姓名\t班级");//本功能暂时不备扩展性System.out.println(s[t].num+" "+s[t].name+" "+s[t].classAge);System.out.println();System.out.print("你确定要修改(Y/N):");str=pin.next();ch=str.charAt(0);while(ch!='Y'&&ch!='y'&&ch!='N'&&ch!='n'){System.out.print("输入有误,请重新输入:");str=pin.next();ch=str.charAt(0);}if(ch=='N'||ch=='n'){System.out.println();System.out.println("系统返回主菜单!");this.menu();}while(c==1){if(ch=='Y'||ch=='y'){System.out.println("****************************************");System.out.println("***** 1.修改学号*****");System.out.println("***** 2.修改班级*****");System.out.println("***** 3.修改姓名*****");System.out.println("****************************************");System.out.print("请选择:");moi=pin.nextInt();switch(moi){case 1:System.out.print("请输入新的学号:");num3=pin.nextInt();s[t].num=num3;break;case 2:System.out.print("请输入新的班级:");str1=pin.next();s[t].classAge=str1;break;case 3:System.out.print("请输入新的姓名:");str2=pin.next();s[t].name=str2;break;}System.out.println("数据已成功修改!");}System.out.print("是否继续修改(Y/N)");str=pin.next();ch=str.charAt(0);System.out.println();while(ch!='Y'&&ch!='y'&&ch!='N'&&ch!='n'){System.out.print("输入有误,请重新输入:");str=pin.next();ch=str.charAt(0);}if(ch=='N'||ch=='n'){break;}}}System.out.println();System.out.println("系统返回主菜单!");this.menu();}public static void main(String[] args)throws IOException {Student stu=new Student();for(int i=0;i<2;i++){s[i]=new Student();}stu.menu();}}。
学生信息管理系统源代码(java)

目录1.Client.java (2)2.LoginGUI.java (2)3.AdminGUI.java (6)4.StudentGUI.java (14)5.Select.java (17)6.UpdateAndInsert.java (24)7.Delete.java (27)8.Student.java (29)9.Administrator.java (31)1.Client.java/*程序入口*/package ms;public class Client{public static void main(String[] args){LoginGUI lg=new LoginGUI();lg.login();}}2.LoginGUI.java/*登陆主窗口*/package ms;import java.awt.BorderLayout;import java.awt.Choice;import java.awt.GridLayout;import java.awt.TextField;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.awt.event.WindowAdapter;import java.awt.event.WindowEvent;import javax.swing.ImageIcon;import javax.swing.JButton;import javax.swing.JFrame;import javax.swing.JLabel;import javax.swing.JOptionPane;import javax.swing.JPanel;public class LoginGUI{JFrame log=null; //登陆主窗口JPanel jPanel1=null; //用于存放用户名、密码、登录方式标签JPanel jPanel2=null; //用于存放用户名、密码输入框和登录方式选择框JPanel jPanel3=null; //用于存放登陆、退出按钮JLabel jLabel1=null; //用于存放用户名标签JLabel jLabel2=null; //用于存放密码标签JLabel jLabel3=null; //用于存放登录方式标签JLabel jLabel4=null; //学生管理系统登陆标签TextField t1=null; //用于存放用户名输入TextField t2=null; //用于存放密码输入Choice c=null; //用于存放登录方式选择JButton jB1=null; //登陆按钮JButton jB2=null; //退出按钮String UserName=null; //存放用户输入的用户名String UserPassword=null; //存放用户输入的密码int UserLimit=-1; //存放用户选择登陆权限public LoginGUI(){super();this.log = new JFrame("学生学籍管理系统");this.jPanel1=new JPanel();this.jPanel2=new JPanel();this.jPanel3=new JPanel();jLabel1=new JLabel("用户名");jLabel2=new JLabel("密码");jLabel3=new JLabel("登录方式");jLabel4=new JLabel(new ImageIcon("image/login.jpg"));t1=new TextField();t2=new TextField();c=new Choice(); //创建一个登录方式下拉选择框c.add("学生登陆");c.add("管理员登陆");jB1=new JButton("登陆"); //创建登陆按钮jB2=new JButton("退出"); //创建退出按钮}public void login(){BorderLayout border=new BorderLayout();border.setVgap(15);border.setHgap(10);log.setLayout(border); //设置登陆主窗口布局管理器为BorderLayoutlog.add(jPanel1, BorderLayout.WEST);log.add(jPanel2, BorderLayout.CENTER);log.add(jPanel3, BorderLayout.SOUTH);log.add(jLabel4,BorderLayout.NORTH);jPanel1.setLayout(new GridLayout(3,1,30,20)); //3行1列jPanel2.setLayout(new GridLayout(3,1,30,20));jPanel3.setLayout(new GridLayout(1,2,10,30));jPanel1.add(jLabel1); //添加三个标签用户名、密码、登陆方式jPanel1.add(jLabel2);jPanel1.add(jLabel3);jPanel2.add(t1); //添加用户名、密码输入文本框登陆方式选择框jPanel2.add(t2);jPanel2.add(c);jPanel3.add(jB1); //添加两个按钮jPanel3.add(jB2);jB1.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){erName=LoginGUI.this.t1.getText();erPassword=LoginGUI.this.t2.getText();erLimit=LoginGUI.this.c.getSelectedIndex();if(erLimit==0){StudentGUI ll=new StudentGUI(LoginGUI.this.log,erName);Select sel=new Select();sel.setStudentList();for(int i=0;i<sel.getStudentAllId().size();i++){if(sel.getStudentAllId().get(i).toString().equals(erNa me)&&sel.getStudentAllPassword().get(i).equals(erPass word)){log.setVisible(false);ll.studentGo();}else if(i==sel.getStudentAllId().size()-1){JOptionPane.showMessageDialog(LoginGUI.this.log,"用户名密码错误");}}}else if(erLimit==1){AdminGUI admin=new AdminGUI(LoginGUI.this.log);Select sel=new Select();sel.setAdminList();for(int i=0;i<sel.getAdminAllId().size();i++){if(sel.getAdminAllId().get(i).toString().equals(erNam e)&&sel.getAdminAllPassword().get(i).equals(erPasswor d)){log.setVisible(false);admin.adminGo();}else if(i==sel.getAdminAllId().size()-1){JOptionPane.showMessageDialog(LoginGUI.this.log,"用户名密码错误");}}}}});jB2.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){System.exit(0);}});log.setSize(240,260);log.setLocationRelativeTo(null);log.setResizable(false);log.addWindowListener(new WindowAdapter(){@Overridepublic void windowClosing(WindowEvent e){System.exit(0);}});log.setVisible(true);}}3.AdminGUI.java/*管理员登陆图形界面*/package ms;import java.awt.BorderLayout;import java.awt.GridLayout;import java.awt.TextArea;import java.awt.TextField;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.awt.event.WindowAdapter;import java.awt.event.WindowEvent;import javax.swing.ImageIcon;import javax.swing.JButton;import javax.swing.JDialog;import javax.swing.JFrame;import javax.swing.JLabel;import javax.swing.JOptionPane;import javax.swing.JPanel;public class AdminGUI{JDialog log=null;//管理员登陆主窗口JPanel jp1=null;//用于存放管理员登陆标签和jp5JPanel jp2=null;//用于存放查询修改增加删除按钮JPanel jp3=null;//用于存放信息块和jp5JPanel jp4=null;//用于存放修改插入删除按钮JPanel jp5=null;//用于存放请输入学号文本框JLabel jadminLog=null; //管理员登陆标签JLabel jLabel=null; //请输入学号标签TextField tf=null; //请输入学号文本框JButton jb1=null;//查询按钮JButton jb2=null;//修改按钮JButton jb3=null;//增加按钮JButton jb4=null;//删除按钮JButton jb5=null; //列出全部学生信息TextArea ta=null;//信息文本框JLabel jl=null; //用于站位String id=null; //用于保存用户输入查询学号TextField[] jtf = new T extField[9] ;JDialog jdialog=null;public AdminGUI(JFrame log){super();this.log = new JDialog(log,"管理员登陆",true);jp1=new JPanel();jp2=new JPanel();jp3=new JPanel();jp4=new JPanel();jp5=new JPanel();jadminLog=new JLabel(new ImageIcon("image/admin.jpg"));jLabel=new JLabel("请输入学号");tf=new TextField();jb1=new JButton("查询");jb2=new JButton("修改");jb3=new JButton("增加");jb4=new JButton("删除");jb5=new JButton("列出全部学生信息");ta=new TextArea("点击查询按钮将在此显示查询信息");jl=new JLabel();}public void adminGo(){BorderLayout bl=new BorderLayout();bl.setVgap(30);bl.setHgap(30);log.setLayout(bl);log.add(jp1,BorderLayout.NORTH);BorderLayout bl1=new BorderLayout();bl1.setVgap(20);jp1.setLayout(bl1);jp1.add(jadminLog,BorderLayout.CENTER);jp5.setLayout(new GridLayout(1,2,200,200));jp5.add(jLabel);jp5.add(tf);log.add(jp2,BorderLayout.WEST);jp2.setLayout(new GridLayout(2,1,200,300));jp2.add(jb1);jp2.add(jb5);log.add(jp3,BorderLayout.CENTER);jp3.setLayout(new BorderLayout(10, 10));jp3.add(jp5,BorderLayout.NORTH);jp3.add(ta);jp5.setLayout(new GridLayout(1,5,10,10));jp5.add(jLabel);jp5.add(tf);log.add(jp4,BorderLayout.SOUTH);jp4.setLayout(new GridLayout(1,3,200,30));jp4.add(jb2);jp4.add(jb3);jp4.add(jb4);log.addWindowListener(new WindowAdapter(){@Overridepublic void windowClosing(WindowEvent e){System.exit(0);}});jb1.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){AdminGUI.this.id=AdminGUI.this.tf.getText();Select sel=new Select();sel.selectStudent(AdminGUI.this.id);if(sel.getStudent()!=null){Student student=sel.getStudent();AdminGUI.this.ta.setText("学号\t\t"+"姓名\t\t"+"性别\t\t"+"出生日期\t\t"+"民族\t\t"+"籍贯\t\t"+"专业\t\t"+"班级\t\t"+"联系电话\t\t"+"密码\t\t");AdminGUI.this.ta.append("\n"+student.getId()+"\t\t"+student.getNa me()+"\t\t"+student.getSex()+"\t\t"+student.getBornDate()+"\t\t"+student.getNationality()+"\t\t"+student.getNationality()+"\t\t"+stu dent.getMaj()+"\t"+student.getCla()+"\t\t"+student.getTelNum()+"\t\t"+student.getPassword()+"\n");}else{AdminGUI.this.ta.setText("查无此人");}}});jb5.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){Select sel=new Select();sel.selectAllStudent();AdminGUI.this.ta.setText("学号\t\t"+"姓名\t\t"+"性别\t\t"+"出生日期\t\t"+"民族\t\t"+"籍贯\t\t"+"专业\t\t"+"班级\t\t"+"联系电话\t\t"+"密码\t\t\n");for(int i=0;i<sel.getAllStudent().size();i++){Student student=sel.getAllStudent().get(i);AdminGUI.this.ta.append(student.getId()+"\t\t"+student.getName() +"\t\t"+student.getSex()+"\t\t"+student.getBornDate()+"\t\t"+student.getNationality()+"\t\t"+student.getHometown()+"\t\t"+st udent.getMaj()+"\t"+student.getCla()+"\t\t"+student.getTelNum()+"\t\t"+student.getPa ssword()+"\n");}}});log.setSize(850,580);log.setLocationRelativeTo(null);log.setResizable(false);jb3.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){jdialog=new JDialog(AdminGUI.this.log);String[] str=new String[]{"姓名","性别","出生日期","民族","籍贯","专业","班级","联系电话","密码"};JLabel[] jlabel=new JLabel[9];for(int i=0;i<9;i++){jlabel[i]=new JLabel(str[i]);jtf[i]=new TextField();}JPanel jpanel1=new JPanel();JPanel jpanel2=new JPanel();JButton jbutton1=new JButton("确定");JButton jbutton2=new JButton("取消");jdialog.setLayout(new BorderLayout());jdialog.add(jpanel1,BorderLayout.CENTER);jdialog.add(jpanel2,BorderLayout.SOUTH);jpanel1.setLayout(new GridLayout(9,2,10,10));for(int i=0;i<9;i++){jpanel1.add(jlabel[i]);jpanel1.add(jtf[i]);}jpanel2.add(jbutton1);jpanel2.add(jbutton2);jbutton1.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){Student student=new Student();student.setName(AdminGUI.this.jtf[0].getText());student.setSex(AdminGUI.this.jtf[1].getText()); student.setBornDate(AdminGUI.this.jtf[2].getText());student.setNationality(AdminGUI.this.jtf[3].getText());student.setHometown(AdminGUI.this.jtf[4].getText());student.setMaj(AdminGUI.this.jtf[5].getText());student.setCla(AdminGUI.this.jtf[6].getText());student.setTelNum(AdminGUI.this.jtf[7].getText()); student.setPassword(AdminGUI.this.jtf[8].getText());UpdateAndInsert up=new UpdateAndInsert();if(up.insertStudent(student)==true){JOptionPane.showMessageDialog(AdminGUI.this.log,"添加成功");}jdialog.dispose();}});jbutton2.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){jdialog.dispose();}});jdialog.setSize(500,400);jdialog.setLocationRelativeTo(null);jdialog.setResizable(false);jdialog.setVisible(true);}});jb4.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){if(new Delete().delete(AdminGUI.this.tf.getText())==true){JOptionPane.showMessageDialog(AdminGUI.this.log,"刪除成功");}elseJOptionPane.showMessageDialog(AdminGUI.this.log,"刪除失敗");}});jb2.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){Select sel=new Select();AdminGUI.this.id=AdminGUI.this.tf.getText();sel.selectStudent(AdminGUI.this.tf.getText());Student student=sel.getStudent();if(student==null){JOptionPane.showMessageDialog(AdminGUI.this.log,"请检查输入的学号");}else{jdialog=new JDialog(AdminGUI.this.log);String[] str=new String[]{"姓名","性别","出生日期","民族","籍贯","专业","班级","联系电话","密码"};JLabel[] jlabel=new JLabel[9];for(int i=0;i<9;i++){jlabel[i]=new JLabel(str[i]);}jtf[0]=new TextField(student.getName());jtf[1]=new TextField(student.getSex());jtf[2]=new TextField(student.getBornDate());jtf[3]=new TextField(student.getNationality());jtf[4]=new TextField(student.getHometown());jtf[5]=new TextField(student.getMaj());jtf[6]=new TextField(student.getCla());jtf[7]=new TextField(student.getTelNum());jtf[8]=new TextField(student.getPassword());JPanel jpanel1=new JPanel();JPanel jpanel2=new JPanel();JButton jbutton1=new JButton("确定");JButton jbutton2=new JButton("取消");jdialog.setLayout(new BorderLayout());jdialog.add(jpanel1,BorderLayout.CENTER);jdialog.add(jpanel2,BorderLayout.SOUTH);jpanel1.setLayout(new GridLayout(9,2,10,10));for(int i=0;i<9;i++){jpanel1.add(jlabel[i]);jpanel1.add(jtf[i]);}jpanel2.add(jbutton1);jpanel2.add(jbutton2);jbutton1.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){Student student=new Student(Integer.parseInt(AdminGUI.this.id), jtf[0].getText(), jtf[1].getText(), jtf[2].getText(), jtf[3].getText(), jtf[4].getText(), jtf[5].getText(), jtf[6].getText(), jtf[7].getText(), jtf[8].getText());UpdateAndInsert up=new UpdateAndInsert();if(up.updateStudent(student)==true){JOptionPane.showMessageDialog(AdminGUI.this.log,"更改成功");;}jdialog.dispose();}});jbutton2.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){jdialog.dispose();}});jdialog.setSize(500,400);jdialog.setLocationRelativeTo(null);jdialog.setResizable(false);jdialog.setVisible(true);}}});log.setVisible(true);}}4.StudentGUI.java/*学生操作图形界面*/package ms;import java.awt.BorderLayout;import java.awt.GridLayout;import java.awt.TextField;import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent; import javax.swing.ImageIcon;import javax.swing.JButton;import javax.swing.JDialog;import javax.swing.JFrame;import javax.swing.JLabel;import javax.swing.JOptionPane;import javax.swing.JPanel;public class StudentGUI{JDialog log=null;//学生登入类主窗口JPanel jp1=null;//用于存放登入块JPanel jp2=null;//用于存放信息块JPanel jp3=null;//用于密码存放修改块JPanel jp4=null;//用于存放我的信息按钮JLabel jl1=null;//学生登入标签JLabel jl2=null;//学号标签JLabel jl3=null;//学生姓名标签JLabel jl4=null;//性别标签JLabel jl5=null;//出生日期标签JLabel jl6=null;//民族标签JLabel jl7=null;//籍贯标签JLabel jl8=null;//专业标签JLabel jl9=null;//班级标签JLabel jl10=null;//联系电话标签JLabel jl11=null;//请输入密码标签JButton jb1=null;//我的信息按钮JButton jb2=null;//密码修改按钮TextField t1=null;//学号文本框TextField t2=null;//学生姓名文本框TextField t3=null;//性别文本框TextField t4=null;//出生日期文本框TextField t5=null;//民族文本框TextField t6=null;//籍贯文本框TextField t7=null;//专业文本框TextField t8=null;//班级文本框TextField t9=null;//联系电话文本框TextField t10=null;//密码文本框JPanel jp5=new JPanel();//存放密码输入框String id=null;public StudentGUI(JFrame log,String str){super();this.log = new JDialog(log,"学生登入",true);jp1=new JPanel();jp2=new JPanel();jp3=new JPanel();jp4=new JPanel();jl1=new JLabel(new ImageIcon("image/student.jpg"));jl2=new JLabel("学号:");jl3=new JLabel("学生姓名:");jl4=new JLabel("性别:");jl5=new JLabel("出生日期:");jl6=new JLabel("民族:");jl7=new JLabel("籍贯:");jl8=new JLabel("专业:");jl9=new JLabel("班级:");jl10=new JLabel("联系电话:");jl11=new JLabel("请输入新密码:");jb1=new JButton("我的信息");jb2=new JButton("密码修改");t1=new TextField();t2=new TextField();t3=new TextField();t4=new TextField();t5=new TextField();t6=new TextField();t7=new TextField();t8=new TextField();t9=new TextField();t10=new TextField();this.id=str;}public void studentGo(){BorderLayout bl=new BorderLayout();bl.setHgap(30);bl.setVgap(30);log.setLayout(bl);log.add(jp1,BorderLayout.NORTH);//将登入块信息块密码修改块放入主窗口log.add(jp2,BorderLayout.CENTER);log.add(jp3,BorderLayout.SOUTH);log.add(jp4,BorderLayout.WEST);jp1.add(jl1,BorderLayout.CENTER);jp2.setLayout(new GridLayout(5,2,20,10));jp2.add(jl2);jp2.add(t1);jp2.add(jl3);jp2.add(t2);jp2.add(jl4);jp2.add(t3);jp2.add(jl5);jp2.add(t4);jp2.add(jl6);jp2.add(t5);jp2.add(jl7);jp2.add(t6);jp2.add(jl8);jp2.add(t7);jp2.add(jl9);jp2.add(t8);jp2.add(jl10);jp2.add(t9);jp4.add(jb1);BorderLayout bl1=new BorderLayout();bl1.setHgap(38);jp3.setLayout(bl1);jp3.add(jb2,BorderLayout.WEST);jp3.add(jp5,BorderLayout.CENTER);jp5.setLayout(new GridLayout());jp5.add(jl11);jp5.add(t10);jb1.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){Select sel=new Select();sel.selectStudent(id);Student student=sel.getStudent();t1.setText(new Integer(student.getId()).toString());t2.setText(student.getName());t3.setText(student.getSex());t4.setText(student.getBornDate());t5.setText(student.getNationality());t6.setText(student.getHometown());t7.setText(student.getMaj());t8.setText(student.getCla());t9.setText(student.getTelNum());}});jb2.addActionListener(new ActionListener(){@Overridepublic void actionPerformed(ActionEvent e){String password=StudentGUI.this.t10.getText();UpdateAndInsert up=new UpdateAndInsert();if(up.updatePassword(id, password)==true){JOptionPane.showMessageDialog(StudentGUI.this.log,"修改成功");}}});log.addWindowListener(new WindowAdapter(){@Overridepublic void windowClosing(WindowEvent e){System.exit(0);}});log.setSize(550,420);log.setLocationRelativeTo(null);log.setResizable(false);log.setVisible(true);}}5.Select.java/* 查询类包括查询的各种方法*/package ms;import java.sql.*;import java.util.LinkedList;public class Select{private LinkedList<Integer> studentAllId=null;private LinkedList<String> studentAllPassword=null;private LinkedList<Integer> adminAllId=null;private LinkedList<String> adminAllPassword=null;private Student student=null;private LinkedList<Student> allStudent=null;// 定义MySQL的数据库驱动程序public static final String DBDRIVER = "org.gjt.mm.mysql.Driver" ;// 定义MySQL数据库的连接地址public static final String DBURL = "jdbc:mysql://localhost:3306/studentMS" ;// MySQL数据库的连接用户名public static final String DBUSER = "root" ;// MySQL数据库的连接密码public static final String DBPASS = "systemout" ;public Select(){super();this.studentAllId = new LinkedList<Integer>();this.studentAllPassword = new LinkedList<String>();this.adminAllId = new LinkedList<Integer>();this.adminAllPassword = new LinkedList<String>();this.allStudent = new LinkedList<Student>();}public LinkedList<Integer> getStudentAllId(){return studentAllId;}public LinkedList<String> getStudentAllPassword(){return studentAllPassword;}public LinkedList<Integer> getAdminAllId(){return adminAllId;}public LinkedList<String> getAdminAllPassword(){return adminAllPassword;}public Student getStudent(){return student;}public LinkedList<Student> getAllStudent(){return allStudent;}public void setStudentList() //设置studentAllId 和studentAllPassword{Connection conn = null ; // 数据库连接Statement stmt = null ; // 数据库的操作对象ResultSet rs = null ; // 保存查询结果String sql = "select id,password from student";try{Class.forName(DBDRIVER) ; // 加载驱动程序}catch(ClassNotFoundException e){e.printStackTrace() ;}try{conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS) ;}catch(SQLException e){e.printStackTrace() ;}try{stmt = conn.createStatement() ; //得到stmt对象用于执行sql语句} catch (SQLException e1){e1.printStackTrace();}try{rs = stmt.executeQuery(sql) ;//保存sql查询结果} catch (SQLException e1){e1.printStackTrace();}try{while(rs.next()){studentAllId.add(rs.getInt(1));studentAllPassword.add(rs.getString(2));}} catch (SQLException e1){e1.printStackTrace();}try{rs.close() ;stmt.close() ;conn.close() ; // 数据库关闭}catch(SQLException e){e.printStackTrace() ;}}public void setAdminList(){Connection conn = null ; // 数据库连接Statement stmt = null ; // 数据库的操作对象ResultSet rs = null ; // 保存查询结果String sql = "select id,password from admin";try{Class.forName(DBDRIVER) ; // 加载驱动程序}catch(ClassNotFoundException e){e.printStackTrace() ;}try{conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS) ;}catch(SQLException e){e.printStackTrace() ;}try{stmt = conn.createStatement() ; //得到stmt对象用于执行sql语句} catch (SQLException e1){e1.printStackTrace();}try{rs = stmt.executeQuery(sql) ;//保存sql查询结果} catch (SQLException e1){e1.printStackTrace();}try{while(rs.next()){adminAllId.add(rs.getInt(1));adminAllPassword.add(rs.getString(2));}} catch (SQLException e1){e1.printStackTrace();}try{rs.close() ;stmt.close() ;conn.close() ; // 数据库关闭}catch(SQLException e){e.printStackTrace() ;}}public boolean selectStudent(String str){Connection conn = null ; // 数据库连接PreparedStatement pstmt = null ; // 数据库的操作对象ResultSet rs = null ; // 保存查询结果String sql = "select name,sex,bornDate,nationality,hometown,maj,cla,telNum,password "+ "from student where id=?";try{Class.forName(DBDRIVER) ; // 加载驱动程序}catch(ClassNotFoundException e){e.printStackTrace() ;}try{conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS) ;}catch(SQLException e){e.printStackTrace() ;}try{pstmt = conn.prepareStatement(sql) ; //得到stmt对象用于执行sql语句} catch (SQLException e1){e1.printStackTrace();}try{pstmt.setString(1, str);} catch (SQLException e2){e2.printStackTrace();}try{rs = pstmt.executeQuery() ;//保存sql查询结果} catch (SQLException e1){e1.printStackTrace();}try{while(rs.next()){student=new Student(Integer.parseInt(str),rs.getString(1),rs.getString(2),rs.getString(3),rs.getString(4),rs.getString(5),rs.getString(6),rs.getString(7),rs.getString(8),rs.getString(9));}} catch (SQLException e1){e1.printStackTrace();}try{rs.close() ;pstmt.close() ;conn.close() ; // 数据库关闭}catch(SQLException e){e.printStackTrace() ;}if(student !=null){return true;}else{return false;}}public void selectAllStudent(){Connection conn = null ; // 数据库连接PreparedStatement pstmt = null ; // 数据库的操作对象ResultSet rs = null ; // 保存查询结果String sql = "select * "+"from student";try{Class.forName(DBDRIVER) ; // 加载驱动程序}catch(ClassNotFoundException e){e.printStackTrace() ;}try{conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS) ;}catch(SQLException e){e.printStackTrace() ;}try{pstmt = conn.prepareStatement(sql) ; //得到stmt对象用于执行sql语句} catch (SQLException e1){e1.printStackTrace();}try{rs = pstmt.executeQuery() ;//保存sql查询结果} catch (SQLException e1){e1.printStackTrace();}try{while(rs.next()){student=new Student(rs.getInt(1),rs.getString(2),rs.getString(3),rs.getString(4),rs.getString(5),rs.getString(6),rs.getString(7),rs.getString(8),rs.getString(9),rs.getString(10));allStudent.add(student);}} catch (SQLException e1){e1.printStackTrace();}try{rs.close() ;pstmt.close() ;conn.close() ; // 数据库关闭}catch(SQLException e){e.printStackTrace() ;}}}6.UpdateAndInsert.java/*修改&&插入类定义了修改和插入的所有方法*/package ms;import java.sql.Connection;import java.sql.DriverManager;import java.sql.SQLException;import java.sql.Statement;public class UpdateAndInsert{// 定义MySQL的数据库驱动程序public static final String DBDRIVER = "org.gjt.mm.mysql.Driver" ;// 定义MySQL数据库的连接地址public static final String DBURL = "jdbc:mysql://localhost:3306/studentMS" ;// MySQL数据库的连接用户名public static final String DBUSER = "root" ;// MySQL数据库的连接密码public static final String DBPASS = "systemout" ;public boolean insertStudent (Student student){String name=student.getName();String sex=student.getSex();String bornDate=student.getBornDate();String nationality=student.getNationality();String hometown=student.getHometown();String maj=student.getMaj();String cla=student.getCla();String telNum=student.getTelNum();String password=student.getPassword();Connection conn = null ; // 数据库连接Statement stmt = null ; // 数据库操作try{Class.forName(DBDRIVER) ;} catch (ClassNotFoundException e){// TODO Auto-generated catch blocke.printStackTrace();} // 加载驱动程序String sql = "INSERT INTO student(name,sex,bornDate,nationality,hometown,maj,cla,telNum,passw ord) "+" VALUES('"+name+"','"+sex+"','"+bornDate+"','"+nationality+"','"+hometown+"' ,'"+maj+"','"+cla+"','"+telNum+"','"+password+"')";try{conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS) ;} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();}try{stmt = conn.createStatement() ;} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();} // 实例化Statement对象try{stmt.executeUpdate(sql) ;} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();} // 执行数据库更新操作try{stmt.close() ; // 关闭操作conn.close() ;} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();} // 数据库关闭return true;}public boolean updateStudent(Student student){Select sel=new Select();if(sel.selectStudent(newInteger(student.getId()).toString())==false){return false;}Connection conn = null ; // 数据库连接Statement stmt = null ; // 数据库操作String name=student.getName();String sex=student.getSex();String bornDate=student.getBornDate();String nationality=student.getNationality();String hometown =student.getHometown();String maj=student.getMaj();String cla=student.getCla();String telNum=student.getTelNum();String password=student.getPassword();String sql="UPDATE student SET name='"+name+"',sex='"+sex+"',bornDate='"+bornDate+"',nationality ='"+nationality+"',hometown='"+hometown+"',maj='"+maj+"',cla='"+c la+"',telNum='"+telNum+"',password='"+password+"' WHERE id="+student.getId() ;try{conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS) ;} catch (SQLException e1){// TODO Auto-generated catch blocke1.printStackTrace();}try{stmt = conn.createStatement() ;} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();} // 实例化Statement对象try{stmt.executeUpdate(sql) ;} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();} // 执行数据库更新操作try{stmt.close() ; // 关闭操作conn.close() ; // 数据库关闭} catch (SQLException e){// TODO Auto-generated catch blocke.printStackTrace();}return true;}。
[工学]学生信息管理系统完整源码
![[工学]学生信息管理系统完整源码](https://img.taocdn.com/s3/m/3483296b26284b73f242336c1eb91a37f1113291.png)
学生信息管理系统完整源代码注:本系统采用C/S结构,运用Java GUI知识编写,数据库为SQL SERVER 2005,没有采用典型的三级框架结构,所以代码有冗余,仅供参考。
一、数据表及数据源首先创建数据库,包含数据表如下:数据库创建完成后,新建一个名为SIMS的数据源,不会建数据源的同学可以在去搜索创建数据源的详细步骤,这里的数据名称一定要为SIMS,否则在以后程序连接数据库的语句中会出现错误。
二、操作演示三、代码部分创建Java工程,创建名称为SIMS的包,一下Java类均包含在一个包内。
1.登录界面package SIMS;import javax.swing.*;import java.awt.*;import java.awt.event.*;import java.sql.*;import java.text.SimpleDateFormat;import java.util.*;import java.util.Date;public class login extends JFrame implements ActionListener{String userID; //保留用户输入IDString password; //保留用户输入passwordJLabel jlID=new JLabel("用户ID:"); //使用文本创建标签对象 JLabel jlPwd=new JLabel("密码:");JTextField jtID=new JTextField(); //创建ID输入框JPasswordField jpPwd=new JPasswordField(); //创建密码输入框ButtonGroup bg=new ButtonGroup(); //创建ButtonGroup组件对象JPanel jp=new JPanel(); //创建Panel容器JLabel jl=new JLabel();JRadioButton jrb1=new JRadioButton("管理员");JRadioButton jrb2=new JRadioButton("教师");JRadioButton jrb3=new JRadioButton("学生",true);JButton jb1=new JButton("登录");JButton jb2=new JButton("重置");public login(){this.setLayout(null); //设置窗口布局管理器this.setTitle("学生信息管理系统"); //设置窗口标题this.setBounds(200,150,500,300); //设置主窗体位置大小和可见性this.setVisible(true); //设置窗口的可见性this.setResizable(false);jlID.setBounds(150,60,100,20); //设置ID框属性jtID.setBounds(220,60,100,20); //设置ID输入框属性jlPwd.setBounds(150,90,100,20); //设置密码框属性jpPwd.setBounds(220,90,100,20); //设置密码输入框属性jp.setBounds(35,120,400,250); //设置JPanel容器属性jb1.setBounds(160,170,60,20); //设置登录按钮属性jb2.setBounds(250,170,60,20); //设置取消按钮属性jb1.addActionListener(this); //设置登录按钮监听器jb2.addActionListener(this); //设置取消按钮监听器jl.setBounds(340,75,130,20); //设置提示框属性bg.add(jrb1); //将所有空间加入窗体bg.add(jrb2);bg.add(jrb3);this.add(jlID);this.add(jlPwd);this.add(jtID);this.add(jpPwd);this.add(jb1);this.add(jb2);this.add(jl);jp.add(jrb1);jp.add(jrb2);jp.add(jrb3);this.add(jp);centerShell(this);this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);}private void centerShell(JFrame shell) //窗口在屏幕中间显示{//得到屏幕的宽度和高度int screenHeight = Toolkit.getDefaultToolkit().getScreenSize().height;int screenWidth = Toolkit.getDefaultToolkit().getScreenSize().width;//得到Shell窗口的宽度和高度int shellHeight = shell.getBounds().height;int shellWidth = shell.getBounds().width;//如果窗口大小超过屏幕大小,让窗口与屏幕等大if(shellHeight > screenHeight)shellHeight = screenHeight;if(shellWidth > screenWidth)shellWidth = screenWidth;//让窗口在屏幕中间显示shell.setLocation(( (screenWidth - shellWidth) / 2),((screenHeight - shellHeight) / 2) );}public boolean equals(Object obj){ //重写equals方法判断字符串相等if(obj==null)return false;if(this == obj){return true;}if(obj instanceof String) {String str = (String)obj;return str.equals(userID);}return false;}public void actionPerformed(ActionEvent e){userID=jtID.getText(); //获取用户输入IDpassword=jpPwd.getText(); //获取用户输入密码if(e.getSource()==jb1){ //处理登录事件if(userID.equals("") || password.equals("")){jl.setFont(new Font("red",Font.BOLD,12)); //设置提示字体jl.setForeground(Color.red);jl.setText("请输入用户ID和密码");}else{Connection con=null;try{String url="jdbc:odbc:SIMS"; //连接数据库con=DriverManager.getConnection(url,"","");//获取连接字符串Statement stat=con.createStatement();if(jrb1.isSelected())//如果登录选中的管理员{ResultSet rs=stat.executeQuery("select * from Admin"); //判断输入用户名是否存在int flag=0;while(rs.next()){if(rs.getString(1).equals(userID)){flag=1;break;}}if(flag==0){jl.setFont(new Font("red",Font.BOLD,12));//设置提示字体jl.setForeground(Color.red);jl.setText("用户ID不存在");}if(flag==1){ResultSet rss=stat.executeQuery("selectAdmin_Pwd,Admin_Name from Admin where Admin_ID='"+userID+"'");//从表Admin获取信息while(rss.next()){String str=rss.getString(1);if(str.equals(password)){new admin(rss.getString(2));//创建admin窗口this.dispose(); //释放窗体}else{jl.setFont(new Font("red",Font.BOLD,12)); //设置提示字体jl.setForeground(Color.red);jl.setText("密码错误");}}}}else if(jrb2.isSelected()){ResultSet rs=stat.executeQuery("select * from Teacher_Info"); //判断输入用户名是否存在int flag=0;while(rs.next()){if(rs.getString(1).equals(userID)){flag=1;break;}}if(flag==0){jl.setFont(new Font("red",Font.BOLD,12));//设置提示字体jl.setForeground(Color.red);jl.setText("用户ID不存在");}if(flag==1){ResultSet rss=stat.executeQuery("selectTea_Pwd,Tea_Names from Teacher_Info where Tea_ID='"+userID+"'");//从表Teacher_Info获取信息while(rss.next()){String str=rss.getString(1);if(str.equals(password)){new teacher(rss.getString(2),userID);//创建admin窗口this.dispose(); //释放窗体}else{jl.setFont(new Font("red",Font.BOLD,12));//设置提示字体jl.setForeground(Color.red);jl.setText("密码错误");}}}}else if(jrb3.isSelected()){ResultSet rs=stat.executeQuery("select * from Student_Info"); //判断输入用户名是否存在int flag=0;while(rs.next()){if(rs.getString(1).equals(userID)){flag=1;break;}}if(flag==0){jl.setFont(new Font("red",Font.BOLD,12));//设置提示字体jl.setForeground(Color.red);jl.setText("用户ID不存在");}if(flag==1){ResultSet rsss=stat.executeQuery("selectStu_Pwd,Stu_Name from Student_Info where Stu_ID='"+userID+"'");//从表Student_Info获取信息while(rsss.next()){String str=rsss.getString(1);if(str.equals(password)){new student(rsss.getString(2),userID);//创建admin窗口this.dispose(); //释放窗体}else{jl.setFont(new Font("red",Font.BOLD,12));//设置提示字体jl.setForeground(Color.red);jl.setText("密码错误");}}}}}catch(Exception ex){ex.getStackTrace();}finally{try{con.close();}catch(Exception exc){exc.printStackTrace();}}}}else if(e.getSource()==jb2){ //处理登录事件jtID.setText("");jpPwd.setText("");jrb3.setSelected(true);jl.setText("");}}public static void main(String[] args){new login();}}2.添加课程package SIMS;import javax.swing.*;import java.sql.*;import java.awt.*;import java.awt.event.*;public class add_course extends JFrame implements ActionListener{ static add_course ss;String courseID=""; //课程名String coursename=""; //课程名String count=""; //课时JLabel warning=new JLabel(); //输入信息提示框JLabel title=new JLabel();JLabel note1=new JLabel("*");JLabel note2=new JLabel("*");JLabel jlcourseID=new JLabel("课程号:"); //使用文本框创建标签对象JLabel jlcoursename=new JLabel("课程名:");JLabel jlcount=new JLabel("课时:");JTextField jtcourseID=new JTextField(); //创建文本框对象JTextField jtcoursename=new JTextField();JTextField jtcount=new JTextField();JButton submit=new JButton("添加"); //创建按钮对象JButton reset=new JButton("重置");public add_course(){ //添加教师账号信息this.setTitle("添加课程信息"); //设置窗口标题this.setLayout(null); //设置窗口布局管理器this.add(jlcourseID); //将控件添加到窗体this.add(title);this.add(jlcoursename);this.add(jlcount);this.add(jtcourseID);this.add(jtcoursename);this.add(jtcount);this.add(note1);this.add(note2);this.add(submit);this.add(reset);this.add(warning);title.setFont(new Font("red",Font.BOLD,15)); //设置提示字体title.setForeground(Color.red);note1.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note1.setForeground(Color.red);note2.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note2.setForeground(Color.red);warning.setFont(new Font("red",Font.BOLD,12)); //设置提示字体warning.setForeground(Color.red);title.setText("添加课程信息"); //设置控件及窗体位置大小title.setBounds(222,20,150,20);jlcourseID.setBounds(180,80,100,20);jlcoursename.setBounds(180,140,100,20);jlcount.setBounds(180,200,100,20);jtcourseID.setBounds(250,80,140,20);jtcoursename.setBounds(250,140,140,20);jtcount.setBounds(250,200,140,20);note1.setBounds(400,80,140,20);note2.setBounds(400,140,140,20);submit.setBounds(200,270,60,20);reset.setBounds(300,270,60,20);warning.setBounds(420,140,150,20); //设置提示框位置大小submit.addActionListener(this); //添加监听器reset.addActionListener(this);this.setSize(600,400); //设置窗体大小centerShell(this); //设置窗口位置在屏幕中央this.setResizable(false); //设置窗体不可变大小this.setVisible(true); //设置窗口可见性this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);}public boolean equals(Object obj){ //重写equals方法判断字符串相等if(obj==null)return false;if(this == obj){return true;}if(obj instanceof String) {String str = (String)obj;return str.equals(courseID);}return false;}public void actionPerformed(ActionEvent e){courseID=jtcourseID.getText(); //获取用户输入内容coursename=jtcoursename.getText();count=jtcount.getText();int temp=0,flag=0;Connection con=null;if(e.getSource()==submit){ //判断是否已输入必填信息if(courseID.equals("") || coursename.equals("")){warning.setText("请输入必填信息");}else{try{String url="jdbc:odbc:SIMS"; //连接数据库con=DriverManager.getConnection(url,"",""); //获取连接字符串Statement stat=con.createStatement();ResultSet rs=stat.executeQuery("select Course_ID from Course");while(rs.next()){if(rs.getString(1).equals(courseID)){warning.setText("课程ID已存在");flag=1; //判断用户名唯一break;}}if(flag!=1){if(!count.equals("")){temp=stat.executeUpdate("insert intoCourse(Course_ID,Course_Name,Course_Count)values('"+courseID+"','"+coursename+"','"+count+"')");}else{temp=stat.executeUpdate("insert intoCourse(Course_ID,Course_Name) values('"+courseID+"','"+coursename+"')");}}if(temp==1){JOptionPane.showMessageDialog(ss,"添加成功");warning.setText("");}else{JOptionPane.showMessageDialog(ss,"添加失败");}}catch(Exception ex){ex.getStackTrace();}}}else if(e.getSource()==reset){warning.setText("");jtcourseID.setT ext("");jtcoursename.setText("");jtcount.setText("");}}private void centerShell(JFrame shell) //窗口在屏幕中间显示{//得到屏幕的宽度和高度int screenHeight = Toolkit.getDefaultToolkit().getScreenSize().height;int screenWidth = Toolkit.getDefaultToolkit().getScreenSize().width;//得到Shell窗口的宽度和高度int shellHeight = shell.getBounds().height;int shellWidth = shell.getBounds().width;//如果窗口大小超过屏幕大小,让窗口与屏幕等大if(shellHeight > screenHeight)shellHeight = screenHeight;if(shellWidth > screenWidth)shellWidth = screenWidth;//让窗口在屏幕中间显示shell.setLocation(((screenWidth - shellWidth)/ 2),((screenHeight - shellHeight)/2));}}3.添加学生package SIMS;import javax.swing.*;import java.sql.*;import java.awt.*;import java.awt.event.*;public class add_student extends JFrame implements ActionListener{static add_teacher ss;String userID=""; //用户名String pwd1=""; //密码String pwd2=""; //确认密码String getsdept=""; //院系String name=""; //姓名JLabel warning=new JLabel(); //输入信息提示框JLabel title=new JLabel();JLabel note1=new JLabel("*");JLabel note2=new JLabel("*");JLabel note3=new JLabel("*");JLabel jlID=new JLabel("学号:"); //创建文本框对象 JLabel jlName=new JLabel("姓名:");JLabel jlPwd=new JLabel("密码:");JLabel jlPwd2=new JLabel("确认密码:");JLabel sdept=new JLabel("学院:");JTextField jtID=new JTextField();JTextField jtName=new JTextField();JPasswordField jtPwd=new JPasswordField ();JPasswordField jtPwd2=new JPasswordField ();JTextField jtsdept=new JTextField();JButton submit=new JButton("添加"); //创建按钮对象JButton reset=new JButton("重置");public add_student(){this.setTitle("添加学生账号信息"); //设置窗口标题this.setLayout(null); //设置窗口布局管理器this.add(jlID); //将控件添加到窗体this.add(title);this.add(jlName);this.add(jlPwd);this.add(jlPwd2);this.add(sdept);this.add(jtID);this.add(jtName);this.add(jtPwd);this.add(jtPwd2);this.add(jtsdept);this.add(note1);this.add(note2);this.add(note3);this.add(submit);this.add(reset);this.add(warning);title.setFont(new Font("red",Font.BOLD,15)); //设置提示字体title.setForeground(Color.red);note1.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note1.setForeground(Color.red);note2.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note2.setForeground(Color.red);note3.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note3.setForeground(Color.red);warning.setFont(new Font("red",Font.BOLD,12)); //设置提示字体warning.setForeground(Color.red);title.setText("添加学生账号信息");title.setBounds(222,20,150,20);jlID.setBounds(180,60,100,20);jlName.setBounds(180,100,100,20);jlPwd.setBounds(180,140,100,20);jlPwd2.setBounds(180,180,100,20);sdept.setBounds(180,220,100,20);jtID.setBounds(250,60,140,20);jtName.setBounds(250,100,140,20);jtPwd.setBounds(250,140,140,20);jtPwd2.setBounds(250,180,140,20);jtsdept.setBounds(250,220,140,20);note1.setBounds(400,60,140,20);note2.setBounds(400,140,140,20);note3.setBounds(400,180,140,20);submit.setBounds(200,270,60,20);reset.setBounds(300,270,60,20);warning.setBounds(420,100,150,20);submit.addActionListener(this);reset.addActionListener(this);this.setSize(600,400);centerShell(this);this.setVisible(true);this.setResizable(false); //设置窗体不可变大小this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);}public boolean equals(Object obj){ //重写equals方法判断字符串相等if(obj==null)return false;if(this == obj){return true;}if(obj instanceof String) {String str = (String)obj;return str.equals(pwd1);}return false;}public void actionPerformed(ActionEvent e){userID=jtID.getText(); //获取用户输入内容pwd1=jtPwd.getText();pwd2=jtPwd2.getText();getsdept=jtsdept.getText();name=jtName.getText();int temp=0,flag=0;Connection con=null;if(e.getSource()==submit){if(userID.equals("") || pwd1.equals("") || pwd2.equals("")){ //判断是否已输入必填信息warning.setText("请输入必填信息");}else if(!pwd1.equals(pwd2)){ //判断两次输入密码是否相同warning.setText("两次输入密码不相同");}else{try{String url="jdbc:odbc:SIMS"; //连接数据库con=DriverManager.getConnection(url,"",""); //获取连接字符串Statement stat=con.createStatement();ResultSet rs=stat.executeQuery("select Stu_ID from Student_Info");while(rs.next()){if(rs.getString(1).equals(userID)){warning.setText("用户ID已存在");flag=1; //判断用户名唯一break;}}if(flag!=1){if(!name.equals("") && !getsdept.equals("")){temp=stat.executeUpdate("insert intoStudent_Info(Stu_ID,Stu_Name,Stu_Pwd,Depart)values('"+userID+"','"+name+"','"+pwd1+"','"+getsdept+"')");}else if(!name.equals("") && getsdept.equals("")){temp=stat.executeUpdate("insert intoStudent_Info(Stu_ID,Stu_Name,Stu_Pwd) values('"+userID+"','"+name+"','"+pwd1+"')");}else if(name.equals("") && !getsdept.equals("")){temp=stat.executeUpdate("insert intoStudent_Info(Stu_ID,Stu_Pwd,Depart) values('"+userID+"','"+pwd1+"','"+getsdept+"')");}else{temp=stat.executeUpdate("insert intoStudent_Info(Stu_ID,Stu_Pwd) values('"+userID+"','"+pwd1+"')");}}if(temp==1){JOptionPane.showMessageDialog(ss,"添加成功");}else{JOptionPane.showMessageDialog(ss,"添加失败");}}catch(Exception ex){ex.getStackTrace();}}}else if(e.getSource()==reset){ //重置所有控件warning.setText("");jtID.setText("");jtName.setText("");jtPwd.setText("");jtPwd2.setText("");jtsdept.setText("");}}private void centerShell(JFrame shell) //窗口在屏幕中间显示{//得到屏幕的宽度和高度int screenHeight = Toolkit.getDefaultToolkit().getScreenSize().height;int screenWidth = Toolkit.getDefaultToolkit().getScreenSize().width;//得到Shell窗口的宽度和高度int shellHeight = shell.getBounds().height;int shellWidth = shell.getBounds().width;//如果窗口大小超过屏幕大小,让窗口与屏幕等大if(shellHeight > screenHeight)shellHeight = screenHeight;if(shellWidth > screenWidth)shellWidth = screenWidth;//让窗口在屏幕中间显示shell.setLocation(((screenWidth - shellWidth)/ 2),((screenHeight - shellHeight)/2));}//public static void main(String args[]){// new add_student();//}}4.添加教师package SIMS;import javax.swing.*;import java.sql.*;import java.awt.*;import java.awt.event.*;public class add_teacher extends JFrame implements ActionListener{static add_teacher ss;String userID=""; //用户名String pwd1=""; //密码String pwd2=""; //确认密码String getsdept=""; //院系String name=""; //姓名JLabel warning=new JLabel(); //输入信息提示框JLabel title=new JLabel();JLabel note1=new JLabel("*");JLabel note2=new JLabel("*");JLabel note3=new JLabel("*");JLabel jlID=new JLabel("教工号:"); //使用文本框创建标签对象 JLabel jlName=new JLabel("姓名:");JLabel jlPwd=new JLabel("密码:");JLabel jlPwd2=new JLabel("确认密码:");JLabel sdept=new JLabel("学院:");JTextField jtID=new JTextField(); //创建文本框对象JTextField jtName=new JTextField();JPasswordField jtPwd=new JPasswordField ();JPasswordField jtPwd2=new JPasswordField ();JTextField jtsdept=new JTextField();JButton submit=new JButton("添加"); //创建按钮对象JButton reset=new JButton("重置");public add_teacher(){ //添加教师账号信息this.setTitle("添加教师账号信息"); //设置窗口标题this.setLayout(null); //设置窗口布局管理器this.add(jlID); //将控件添加到窗体this.add(title);this.add(jlName);this.add(jlPwd);this.add(jlPwd2);this.add(sdept);this.add(jtID);this.add(jtName);this.add(jtPwd);this.add(jtPwd2);this.add(jtsdept);this.add(note1);this.add(note2);this.add(note3);this.add(submit);this.add(reset);this.add(warning);title.setFont(new Font("red",Font.BOLD,15)); //设置提示字体title.setForeground(Color.red);note1.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note1.setForeground(Color.red);note2.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note2.setForeground(Color.red);note3.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note3.setForeground(Color.red);warning.setFont(new Font("red",Font.BOLD,12)); //设置提示字体warning.setForeground(Color.red);title.setText("添加教师账号信息"); //设置控件及窗体位置大小title.setBounds(222,20,150,20);jlID.setBounds(180,60,100,20);jlName.setBounds(180,100,100,20);jlPwd.setBounds(180,140,100,20);jlPwd2.setBounds(180,180,100,20);sdept.setBounds(180,220,100,20);jtID.setBounds(250,60,140,20);jtName.setBounds(250,100,140,20);jtPwd.setBounds(250,140,140,20);jtPwd2.setBounds(250,180,140,20);jtsdept.setBounds(250,220,140,20);note1.setBounds(400,60,140,20);note2.setBounds(400,140,140,20);note3.setBounds(400,180,140,20);submit.setBounds(200,270,60,20);reset.setBounds(300,270,60,20);warning.setBounds(420,100,150,20); //设置提示框位置大小submit.addActionListener(this); //添加监听器reset.addActionListener(this);this.setSize(600,400); //设置窗体大小centerShell(this); //设置窗口位置在屏幕中央this.setResizable(false); //设置窗体不可变大小this.setVisible(true); //设置窗口可见性this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);}public boolean equals(Object obj){ //重写equals方法判断字符串相等if(obj==null)return false;if(this == obj){return true;}if(obj instanceof String) {String str = (String)obj;return str.equals(pwd1);}return false;}public void actionPerformed(ActionEvent e){userID=jtID.getText(); //获取用户输入内容pwd1=jtPwd.getText();pwd2=jtPwd2.getText();getsdept=jtsdept.getText();name=jtName.getText();int temp=0,flag=0;Connection con=null;if(e.getSource()==submit){ //判断是否已输入必填信息if(userID.equals("") || pwd1.equals("") || pwd2.equals("")){warning.setText("请输入必填信息");}else if(!pwd1.equals(pwd2)){ //判断两次输入密码是否一致warning.setText("两次输入密码不相同");}else{try{String url="jdbc:odbc:SIMS"; //连接数据库con=DriverManager.getConnection(url,"",""); //获取连接字符串Statement stat=con.createStatement();ResultSet rs=stat.executeQuery("select Tea_ID from Teacher_Info");while(rs.next()){if(rs.getString(1).equals(userID)){warning.setText("用户ID已存在");flag=1; //判断用户名唯一break;}}if(flag!=1){if(!name.equals("") && !getsdept.equals("")){temp=stat.executeUpdate("insert intoTeacher_Info(Tea_ID,Tea_Names,T ea_Pwd,Depart)values('"+userID+"','"+name+"','"+pwd1+"','"+getsdept+"')");}else if(!name.equals("") && getsdept.equals("")){temp=stat.executeUpdate("insert intoTeacher_Info(Tea_ID,Tea_Names,T ea_Pwd)values('"+userID+"','"+name+"','"+pwd1+"')");}else if(name.equals("") && !getsdept.equals("")){temp=stat.executeUpdate("insert intoTeacher_Info(Tea_ID,Tea_Pwd,Depart) values('"+userID+"','"+pwd1+"','"+getsdept+"')");}else{temp=stat.executeUpdate("insert intoTeacher_Info(Tea_ID,Tea_Pwd) values('"+userID+"','"+pwd1+"')");}}if(temp==1){JOptionPane.showMessageDialog(ss,"添加成功");}else{JOptionPane.showMessageDialog(ss,"添加失败");}}catch(Exception ex){ex.getStackTrace();}}}else if(e.getSource()==reset){warning.setText("");jtID.setText("");jtName.setText("");jtPwd.setText("");jtPwd2.setText("");jtsdept.setText("");}}private void centerShell(JFrame shell) //窗口在屏幕中间显示{//得到屏幕的宽度和高度int screenHeight = Toolkit.getDefaultToolkit().getScreenSize().height;int screenWidth = Toolkit.getDefaultToolkit().getScreenSize().width;//得到Shell窗口的宽度和高度int shellHeight = shell.getBounds().height;int shellWidth = shell.getBounds().width;//如果窗口大小超过屏幕大小,让窗口与屏幕等大if(shellHeight > screenHeight)shellHeight = screenHeight;if(shellWidth > screenWidth)shellWidth = screenWidth;//让窗口在屏幕中间显示shell.setLocation(((screenWidth - shellWidth)/ 2),((screenHeight - shellHeight)/2));}// public static void main(String[] args){// new add_teacher();// }}5.添加授课信息package SIMS;import javax.swing.*;import java.sql.*;import java.awt.*;import java.awt.event.*;public class add_tc extends JFrame implements ActionListener{static add_tc ss;String courseID=""; //课程名String teachername=""; //课程名JLabel warning=new JLabel(); //输入信息提示框JLabel title=new JLabel();JLabel note1=new JLabel("*");JLabel note2=new JLabel("*");JLabel jlcourseID=new JLabel("课程号:"); //使用文本框创建标签对象JLabel jlteachername=new JLabel("教师号:");JTextField jtcourseID=new JTextField(); //创建文本框对象JTextField jtteachername=new JTextField();JButton submit=new JButton("添加"); //创建按钮对象JButton reset=new JButton("重置");public add_tc(){ //添加授课信息this.setTitle("添加授课信息"); //设置窗口标题this.setLayout(null); //设置窗口布局管理器this.add(jlcourseID); //将控件添加到窗体this.add(jlteachername);this.add(title);this.add(jtcourseID);this.add(jtteachername);this.add(note1);this.add(note2);this.add(submit);this.add(reset);this.add(warning);title.setFont(new Font("red",Font.BOLD,15)); //设置提示字体title.setForeground(Color.red);note1.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note1.setForeground(Color.red);note2.setFont(new Font("red",Font.BOLD,20)); //设置提示字体note2.setForeground(Color.red);warning.setFont(new Font("red",Font.BOLD,12)); //设置提示字体warning.setForeground(Color.red);title.setText("添加授课信息"); //设置控件及窗体位置大小title.setBounds(222,20,150,20);jlcourseID.setBounds(180,80,100,20);jlteachername.setBounds(180,140,100,20);jtcourseID.setBounds(250,80,140,20);jtteachername.setBounds(250,140,140,20);note1.setBounds(400,80,140,20);note2.setBounds(400,140,140,20);submit.setBounds(200,250,60,20);reset.setBounds(300,250,60,20);warning.setBounds(420,140,150,20); //设置提示框位置大小submit.addActionListener(this); //添加监听器reset.addActionListener(this);this.setSize(600,400); //设置窗体大小centerShell(this); //设置窗口位置在屏幕中央this.setResizable(false); //设置窗体不可变大小this.setVisible(true); //设置窗口可见性this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);}public boolean equals(Object obj){ //重写equals方法判断字符串相等if(obj==null)return false;if(this == obj){return true;}if(obj instanceof String) {String str = (String)obj;return str.equals(courseID);}return false;}public void actionPerformed(ActionEvent e){courseID=jtcourseID.getText(); //获取用户输入内容teachername=jtteachername.getText();int temp=0,flag1=0,flag2=0,flag3=0;Connection con=null;if(e.getSource()==submit){ //判断是否已输入必填信息if(courseID.equals("") || teachername.equals("")){warning.setText("请输入必填信息");}else{try{String url="jdbc:odbc:SIMS"; //连接数据库con=DriverManager.getConnection(url,"",""); //获取连接字符串Statement stat=con.createStatement();ResultSet rs=stat.executeQuery("select Course_ID from Course");while(rs.next()){if(rs.getString(1).equals(courseID)){flag1=1; //判断课程ID存在break;}}ResultSet rss=stat.executeQuery("select Tea_ID fromTeacher_Info");while(rss.next()){if(rss.getString(1).equals(teachername)){flag2=1; //判断教师ID存在break;}}if(flag1!=1){warning.setText("课程ID不存在");}else if(flag2!=1){warning.setText("教师ID不存在");}ResultSet rsss=stat.executeQuery("select Course_ID,T ea_ID from tc");while(rsss.next()){if(rsss.getString(1).equals(courseID) &&rsss.getString(2).equals(teachername)){flag3=1;warning.setText("授课信息重复");。
简单学生管理信息系统源代码
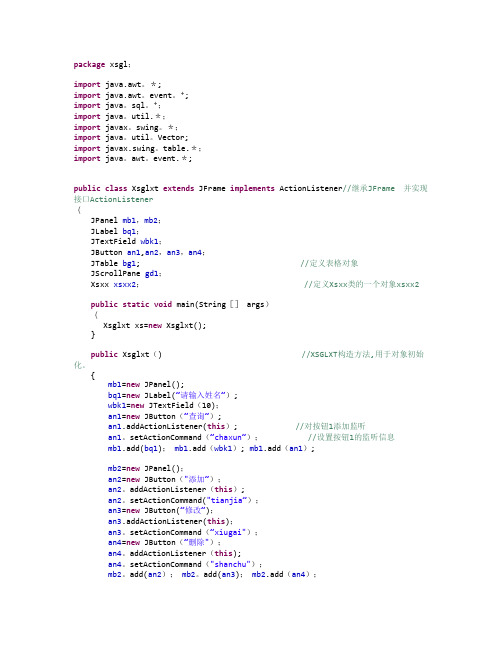
package xsgl;import java.awt。
*;import java.awt。
event。
*;import java。
sql。
*;import java。
util.*;import javax。
swing。
*;import java。
util。
Vector;import javax.swing。
table.*;import java。
awt。
event.*;public class Xsglxt extends JFrame implements ActionListener//继承JFrame 并实现接口ActionListener{JPanel mb1,mb2;JLabel bq1;JTextField wbk1;JButton an1,an2,an3,an4;JTable bg1; //定义表格对象JScrollPane gd1;Xsxx xsxx2;//定义Xsxx类的一个对象xsxx2 public static void main(String[] args){Xsglxt xs=new Xsglxt();}public Xsglxt() //XSGLXT构造方法,用于对象初始化。
{mb1=new JPanel();bq1=new JLabel(”请输入姓名”);wbk1=new JTextField(10);an1=new JButton(”查询”);an1.addActionListener(this); //对按钮1添加监听an1。
setActionCommand(”chaxun”);//设置按钮1的监听信息mb1.add(bq1);mb1.add(wbk1); mb1.add(an1);mb2=new JPanel();an2=new JButton("添加”);an2。
addActionListener(this);an2。
setActionCommand("tianjia”);an3=new JButton(”修改”);an3.addActionListener(this);an3。
用java做的学籍管理系统源码(有注释)

p11=new Panel();
p12=new Panel();
p13=new Panel();
p14=new Panel();
p15=new Panel();
p11.add(lblName);
p11.add(txtName);
JLabel label=null;
JLabel label2;
public ManagerWindow()
{
p=new JPanel();
lblName=new Label("管理员帐号:");
txtName=new TextField(10);
fileMenu=new JMenu("菜单选项");
fileMenu.add(录入);
fileMenu.add(修改);
fileMenu.add(查询);
fileMenu.add(删除);
fileMenu.add(欢迎);
fileMenu.add(关于);
Button btnSubmit,btnReset,guanbi;
Panel p1,p11,p12,p2,p13,p14,p15;
JMenuBar bar;
ImageIcon icon;
JMenu fileMenu;
JMenuItem 录入,修改,查询,删除,欢迎,退出,关于;
基本信息修改=new ModifySituation(file);
基本信息查询=new Inquest(this,file);
基本信息删除=new Delete(file);
学生管理系统数据库设计代码java

学生管理系统数据库设计代码java以下是一个简单的学生管理系统的数据库设计代码示例,使用Java语言实现:```javaimport java.sql.*;public class StudentManagementSystem {private Connection connection;public StudentManagementSystem() {try {// 连接数据库connection =DriverManager.getConnection('jdbc:mysql://localhost:3306/st udent_management_system?useUnicode=true&characterEncoding=u tf8','root', 'password');} catch (SQLException e) {e.printStackTrace();}}public void createTables() {try {Statement statement =connection.createStatement();// 创建学生表String createStudentTableSQL = 'CREATE TABLE students (' +'id INT PRIMARY KEY AUTO_INCREMENT,' + 'name VARCHAR(50) NOT NULL,' +'age INT NOT NULL,' +'gender VARCHAR(10) NOT NULL)';statement.executeUpdate(createStudentTableSQL);// 创建课程表String createCourseTableSQL = 'CREATE TABLE courses (' +'id INT PRIMARY KEY AUTO_INCREMENT,' + 'name VARCHAR(50) NOT NULL)';statement.executeUpdate(createCourseTableSQL);// 创建学生-课程关联表String createStudentCourseTableSQL = 'CREATE TABLE student_course (' +'student_id INT NOT NULL,' +'course_id INT NOT NULL,' +'PRIMARY KEY (student_id, course_id),' + 'FOREIGN KEY (student_id) REFERENCES students(id) ON DELETE CASCADE,' +'FOREIGN KEY (course_id) REFERENCES courses(id) ON DELETE CASCADE)';statement.executeUpdate(createStudentCourseTableSQL);statement.close();} catch (SQLException e) {e.printStackTrace();}}public void closeConnection() {try {if (connection != null) {connection.close();}} catch (SQLException e) {e.printStackTrace();}}public static void main(String[] args) {StudentManagementSystem system = new StudentManagementSystem();system.createTables();system.closeConnection();}}```这段代码创建了三个表: 'students', 'courses'和'student_course'。
java学生管理系统源代码

显示=new JButton("显示");
录入.addActionListener(new InputAct());
查询.addActionListener(new InquestAct());
修改.addActionListener(new ModifyAct());
}
public static void main(String[] args) {StudentManager ff=new StudentManager();}
public class InputAct implements ActionListener{
//Java Group Project_StudentManagement源码
//NetBeans IDE 6.5 环境
package studentmanager;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
Statement stmt;
ResultSet rs;
String url = "jdbc:mysql://localhost/test?user=root&password=0&useUnicode=true&&characterEncoding=8859_1";
int ok=JOptionPane.showConfirmDialog(null,m,"确认",
JOptionPane.YES_NO_OPTION,RMATION_MESSAGE);
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
Java+javabean+JDBC学生管理系统一、项目结构本项目是使用javabean和jdbc做的,这个包是实体包这个是菜单包,源码不会再发这些,自己写就好了。
二、项目运行结果三、源码废话不多说,直接上源码:这两个是关键源码,是负责登录和学生信息操作的逻辑类:public class AdminDaoImpl extends DBHelper implements AdminDao { Admin admin = null;/*** 登录*/@SuppressWarnings("resource")@Overridepublic Admin login(String name) {String sql = "select * from admin where username=?";Object[] param = {name};Object obj = this.excute(sql, param);ResultSet rs = (ResultSet) obj;try {while (rs.next()) {admin = new Admin();String username = rs.getString("username");String password = rs.getString("password");admin.setUsername(username);admin.setPassword(password);}} catch (SQLException e) {System.out.println("未找到此name");}return admin;}}public class StudentDaoImpl extends DBHelper implements StudentDao {Student stu = null;List<Student> list = null;@SuppressWarnings("resource")@Overridepublic Student getInfoByid(int id) {String sql = "select * from student where id=?";Object[] param = { id };Object obj = this.excute(sql, param);ResultSet rs = (ResultSet) obj;stu = new Student();try {while (rs.next()) {stu.setId(rs.getInt("id"));stu.setName(rs.getString("name"));stu.setAge(rs.getInt("age"));stu.setGender(rs.getString("gender"));stu.setGrade(rs.getString("grade"));stu.setPhone(rs.getLong("phone"));stu.setEmail(rs.getString("email"));stu.setAddress(rs.getString("address"));}} catch (SQLException e) {e.printStackTrace();} finally {this.closeAll();}return stu;}@SuppressWarnings("resource")@Overridepublic List<Student> getAllStu() {String sql = "select * from student";Object obj = this.excute(sql, null);ResultSet rs = (ResultSet) obj;list = new ArrayList<Student>();try {while (rs.next()) {stu = new Student();stu.setId(rs.getInt("id"));stu.setName(rs.getString("name"));stu.setAge(rs.getInt("age"));stu.setGender(rs.getString("gender"));stu.setGrade(rs.getString("grade"));stu.setPhone(rs.getLong("phone"));stu.setEmail(rs.getString("email"));stu.setAddress(rs.getString("address"));list.add(stu);}} catch (SQLException e) {e.printStackTrace();} finally {this.closeAll();}return list;}@SuppressWarnings("resource")@Overridepublic String getNameById(int id) {String name = null;String sql = "select name from student where id=?";Object[] param = { id };Object obj = this.excute(sql, param);ResultSet rs = (ResultSet) obj;try {while (rs.next()) {name = rs.getString("name");}} catch (SQLException e) {e.printStackTrace();} finally {this.closeAll();}return name;}@SuppressWarnings("resource")@Overridepublic int getidByIntput(int id) {int num = 0;String sql = "select id from student where id=?";Object[] param = { id };Object obj = this.excute(sql, param);ResultSet rs = (ResultSet) obj;try {while (rs.next()) {num = rs.getInt("id");}} catch (SQLException e) {e.printStackTrace();} finally {this.closeAll();}return num;}@Overridepublic boolean addStudent(Object[] param) {boolean b = false;String sql = "insert into student values(?,?,?,?,?,?,?,?)";Object obj = this.excute(sql, param);b = (boolean) obj;return b;}@Overridepublic boolean removeStuById(int id) {boolean b = false;String sql = "delete from student where id=?";Object[] param = { id };Object obj = this.excute(sql, param);b = (boolean) obj;return b;}@Overridepublic boolean modifyAllStuById(Student stu) {boolean b = false;String sql = "update student set age = ?,grade=?,address=?,phone=?,email=? where id = ?";Object[] param = { stu.getAge(), stu.getGrade(), stu.getAddress(),stu.getPhone(), stu.getEmail(), stu.getId() };Object obj = this.excute(sql, param);b = (boolean) obj;return b;}@Overridepublic boolean modifyPartStuById(Student stu, String attr) { boolean b = false;if (attr.equals("age")) {String sql = "update student set age =? where id=?";Object[] param = { stu.getAge(), stu.getId() };Object obj = this.excute(sql, param);b = (boolean) obj;} else if (attr.equals("grade")) {String sql = "update student set grade =? where id=?";Object[] param = { stu.getGrade(), stu.getId() };Object obj = this.excute(sql, param);b = (boolean) obj;} else if (attr.equals("address")) {String sql = "update student set address =? where id=?";Object[] param = { stu.getAddress(), stu.getId() };Object obj = this.excute(sql, param);b = (boolean) obj;} else if (attr.equals("phone")) {String sql = "update student set phone =? where id=?";Object[] param = { stu.getPhone(), stu.getId() };Object obj = this.excute(sql, param);b = (boolean) obj;} else if (attr.equals("email")) {String sql = "update student set email =? where id=?";Object[] param = { stu.getEmail(), stu.getId() };Object obj = this.excute(sql, param);b = (boolean) obj;}return b;}}好吧,到此为止,逻辑算是完成了,接下来就是工具包,也就是JDBC通式public class DBHelper {private static final String url= "jdbc:mysql://localhost:3306/sms?characterEncoding=utf-8";private static final String Driver = "com.mysql.jdbc.Driver";private static final String name = "root";private static final String pwd = "sa123456";private Connection conn = null;private PreparedStatement pstmt = null;private ResultSet rs = null;/*** 创建数据库连接** @returnpublic Connection Getconn() {try {Class.forName(Driver);conn = DriverManager.getConnection(url, name, pwd);} catch (ClassNotFoundException e) {System.out.println("注册驱动失败");} catch (SQLException e) {System.out.println("驱动包路径错误");}return conn;}public Object excute(String sql, Object[] param) { int a = 0;Object o = null;this.Getconn();try {pstmt = conn.prepareStatement(sql);if (param != null) {for (int i = 0; i < param.length; i++) {pstmt.setObject(i + 1, param[i]);}}boolean b = pstmt.execute();if (b) {rs = pstmt.getResultSet();o = rs;} else {a = pstmt.getUpdateCount();if (a > 0) {o = true;} else {o = false;}closeAll();}} catch (SQLException e) {e.printStackTrace();}return o;}* 关闭数据库*/public void closeAll() {try {if (rs != null) {rs.close();}if (pstmt != null) {pstmt.close();}if (conn != null) {conn.close();}} catch (SQLException e) {System.out.println("错误关闭");}}}至于这个类,是一些控制台输入信息判断,当然可以贴出来供大家参考~/*** 匹配信息** @author Administrator**/public class Matches {Scanner input = new Scanner(System.in);static String id = null;static String gender = null;static String age = null;static String grade = null;static String phone = null;static String email = null;/*** 匹配id** @return*/public String matchesId() {id = input.next();if (Pattern.matches("^[0-9]{1,}$", id)) {} else {System.out.println("输入错误,只能输入数字:");this.matchesId();}return id;}/*** 匹配性别** @return*/public String matchesGender() {gender = input.next();if (!(gender.equals("男") || gender.equals("女"))) { System.out.println("性别只能是男或者女:");this.matchesGender();}return gender;}/*** 匹配年龄** @return*/public int matchesAge() {age = input.next();if (!Pattern.matches("^[0-9]{1,}$", age)) {System.out.println("以上输入不合法,只能输入1-120之内的数字:");this.matchesAge();} else if(Integer.valueOf(age) < 1 || Integer.valueOf(age) > 120) {System.out.println("以上输入不合法,只能输入1-120之内的数字:");this.matchesAge();}return Integer.parseInt(age);}* 匹配年级** @return*/public String matchesGrade() {grade = input.next();if(!(grade.equals("初级") || grade.equals("中级") || grade.equals("高级"))) {System.out.println("无此年级设置,年级只能输入初级、中级或高级,请重新输入:");this.matchesGrade();}return grade;}/*** 匹配手机号** @return*/public long matchesPhone() {phone = input.next();if (!Pattern.matches("^[0-9]{11}$", phone)) {System.out.println("输入有误,电话号码只能是11位数字,请重新输入:");this.matchesPhone();}return Long.parseLong(phone);}/*** 匹配email** @return*/public String matchesEmail() {email = input.next();if(!Pattern.matches("^[0-9a-zA-Z]+@[0-9a-zA-Z]+.[0-9a-zA-Z]+$", email)) {System.out.println("邮箱格式有误,请输入正确的电子邮箱(包含@和.com)");this.matchesEmail();return email;}}好了,别的我就不说了,怎么调用,我更就不用说了吧?本文为原创作品,转载需注明出处。