暴力破解密码C语言
rsa加密解密算法c语言程序
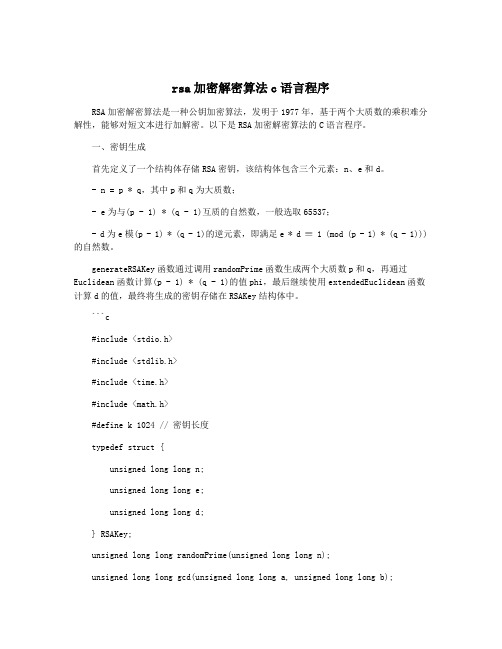
rsa加密解密算法c语言程序RSA加密解密算法是一种公钥加密算法,发明于1977年,基于两个大质数的乘积难分解性,能够对短文本进行加解密。
以下是RSA加密解密算法的C语言程序。
一、密钥生成首先定义了一个结构体存储RSA密钥,该结构体包含三个元素:n、e和d。
- n = p * q,其中p和q为大质数;- e为与(p - 1) * (q - 1)互质的自然数,一般选取65537;- d为e模(p - 1) * (q - 1)的逆元素,即满足e * d ≡ 1 (mod (p - 1) * (q - 1)))的自然数。
generateRSAKey函数通过调用randomPrime函数生成两个大质数p和q,再通过Euclidean函数计算(p - 1) * (q - 1)的值phi,最后继续使用extendedEuclidean函数计算d的值,最终将生成的密钥存储在RSAKey结构体中。
```c#include <stdio.h>#include <stdlib.h>#include <time.h>#include <math.h>#define k 1024 // 密钥长度typedef struct {unsigned long long n;unsigned long long e;unsigned long long d;} RSAKey;unsigned long long randomPrime(unsigned long long n);unsigned long long gcd(unsigned long long a, unsigned long long b);unsigned long long Euclidean(unsigned long long a, unsigned long long b);RSAKey generateRSAKey();// 生成一个小于n的随机质数unsigned long long randomPrime(unsigned long long n) {unsigned long long p;do {p = rand() % n;while (!(p & 1)) // 确保p为奇数p = rand() % n;} while (gcd(p, n) != 1); // 确保p和n互质return p;}二、加密和解密下面定义了两个函数:encrypt和decrypt,其中encrypt函数用于将明文转换为密文,decrypt函数用于将密文转换为明文。
RSA加密解密算法C语言代码

#include<stdio.h>#include<string.h>#include <stdlib.h>#include <time.h>#include <math.h>#include <malloc.h>#define MAX 100#define LEN sizeof(struct slink)void sub(int a[MAX],int b[MAX] ,int c[MAX] );struct slink{int bignum[MAX];/*bignum[98]用来标记正负号,1正,0负bignum[99]来标记实际长度*/struct slink *next;};/*/--------------------------------------自己建立的大数运算库-------------------------------------*/void print( int a[MAX] ){int i;for(i=0;i<a[99];i++)printf("%d",a[a[99]-i-1]);printf("\n\n");return;}int cmp(int a1[MAX],int a2[MAX]){ int l1, l2;int i;l1=a1[99];l2=a2[99];if (l1>l2)return 1;if (l1<l2)return -1;for(i=(l1-1);i>=0;i--){if (a1[i]>a2[i])return 1 ;if (a1[i]<a2[i])return -1;}}void mov(int a[MAX],int *b){int j;for(j=0;j<MAX;j++)b[j]=a[j];return ;}void mul(int a1[MAX],int a2[MAX],int *c) {int i,j;int y;int x;int z;int w;int l1, l2;l1=a1[MAX-1];l2=a2[MAX-1];if (a1[MAX-2]=='-'&& a2[MAX-2]=='-') c[MAX-2]=0;else if (a1[MAX-2]=='-')c[MAX-2]='-';else if (a2[MAX-2]=='-')c[MAX-2]='-';for(i=0;i<l1;i++){for(j=0;j<l2;j++){x=a1[i]*a2[j];y=x/10;z=x%10;w=i+j;c[w]=c[w]+z;c[w+1]=c[w+1]+y+c[w]/10;c[w]=c[w]%10;}}w=l1+l2;if(c[w-1]==0)w=w-1;c[MAX-1]=w;return;}void add(int a1[MAX],int a2[MAX],int *c) {int i,l1,l2;int len,temp[MAX];int k=0;l1=a1[MAX-1];l2=a2[MAX-1];if((a1[MAX-2]=='-')&&(a2[MAX-2]=='-')) {c[MAX-2]='-';}else if (a1[MAX-2]=='-'){mov(a1,temp);temp[MAX-2]=0;sub(a2,temp,c);return;}else if (a2[MAX-2]=='-'){mov(a2,temp);temp[98]=0;sub(a1,temp,c);return;}if(l1<l2)len=l1;else len=l2;for(i=0;i<len;i++){c[i]=(a1[i]+a2[i]+k)%10;k=(a1[i]+a2[i]+k)/10;}if(l1>len){for(i=len;i<l1;i++){c[i]=(a1[i]+k)%10;k=(a1[i]+k)/10;}if(k!=0){c[l1]=k;len=l1+1;}}else{for(i=len;i<l2;i++){c[i]=(a2[i]+k)%10;k=(a2[i]+k)/10;}if(k!=0){c[l2]=k;len=l2+1;}else len=l2;}c[99]=len;return;}void sub(int a1[MAX],int a2[MAX],int *c) {int i,l1,l2;int len,t1[MAX],t2[MAX];int k=0;l1=a1[MAX-1];l2=a2[MAX-1];if ((a1[MAX-2]=='-') && (a2[MAX-2]=='-')) {mov(a1,t1);mov(a2,t2);t1[MAX-2]=0;t2[MAX-2]=0;sub(t2,t1,c);return;}else if( a2[MAX-2]=='-'){mov(a2,t2);t2[MAX-2]=0;add(a1,t2,c);return;}else if (a1[MAX-2]=='-'){mov(a2,t2);add(a1,t2,c);return;}if(cmp(a1,a2)==1){len=l2;for(i=0;i<len;i++){if ((a1[i]-k-a2[i])<0){c[i]=(a1[i]-a2[i]-k+10)%10;k=1;}else{c[i]=(a1[i]-a2[i]-k)%10;k=0;}}for(i=len;i<l1;i++){if ((a1[i]-k)<0){c[i]=(a1[i]-k+10)%10;k=1;}else{c[i]=(a1[i]-k)%10;k=0;}}if(c[l1-1]==0)/*使得数组C中的前面所以0字符不显示了,如1000-20=0980--->显示为980了*/ {len=l1-1;i=2;while (c[l1-i]==0)/*111456-111450=00006,消除0后变成了6;*/{len=l1-i;i++;}else{len=l1;}}elseif(cmp(a1,a2)==(-1)){c[MAX-2]='-';len=l1;for(i=0;i<len;i++){if ((a2[i]-k-a1[i])<0){c[i]=(a2[i]-a1[i]-k+10)%10;k=1;}else{c[i]=(a2[i]-a1[i]-k)%10;k=0;}}for(i=len;i<l2;i++){if ((a2[i]-k)<0){c[i]=(a2[i]-k+10)%10;k=1;}else{c[i]=(a2[i]-k)%10;k=0;}}if(c[l2-1]==0){len=l2-1;i=2;while (c[l1-i]==0){len=l1-i;i++;}}else len=l2;}else if(cmp(a1,a2)==0){len=1;c[len-1]=0;}c[MAX-1]=len;return;}void mod(int a[MAX],int b[MAX],int *c)/*/c=a mod b//注意:经检验知道此处A和C的数组都改变了。
C语言加密与解密算法

C语言加密与解密算法在计算机科学与信息安全领域,加密与解密算法起着至关重要的作用。
加密算法用于将原始数据转换为不可读的密文,而解密算法则用于将密文还原为可读的原始数据。
C语言是一种常用的编程语言,具备高效性和灵活性,适用于加密与解密算法的开发。
本文将介绍几种常用的C语言加密与解密算法。
一、凯撒密码算法凯撒密码算法是一种最简单的替换加密算法,通过将字母按照固定的偏移量进行替换来实现加密与解密。
以下是一个简单的C语言凯撒密码实现例子:```c#include <stdio.h>void caesarEncrypt(char* message, int key) {int i = 0;while (message[i] != '\0') {if (message[i] >= 'a' && message[i] <= 'z') {message[i] = (message[i] - 'a' + key) % 26 + 'a';} else if (message[i] >= 'A' && message[i] <= 'Z') {message[i] = (message[i] - 'A' + key) % 26 + 'A';}i++;}}void caesarDecrypt(char* message, int key) {int i = 0;while (message[i] != '\0') {if (message[i] >= 'a' && message[i] <= 'z') {message[i] = (message[i] - 'a' - key + 26) % 26 + 'a'; } else if (message[i] >= 'A' && message[i] <= 'Z') {message[i] = (message[i] - 'A' - key + 26) % 26 + 'A'; }i++;}}int main() {char message[] = "Hello, World!";int key = 3;printf("Original message: %s\n", message);caesarEncrypt(message, key);printf("Encrypted message: %s\n", message);caesarDecrypt(message, key);printf("Decrypted message: %s\n", message);return 0;}```以上程序演示了凯撒密码的加密与解密过程,通过指定偏移量实现对消息的加密与解密。
C语言中的数据加密与解密技术

C语言中的数据加密与解密技术数据加密与解密技术在现代信息安全领域中起着至关重要的作用,能够保护数据的隐私性和完整性,防止数据被非法窃取和篡改。
在C语言中,我们可以利用各种加密算法实现数据加密和解密操作,确保数据在传输和存储过程中的安全性。
在C语言中,常用的数据加密算法包括对称加密算法和非对称加密算法。
对称加密算法使用相同的密钥进行加密和解密操作,简单高效,适用于小规模数据加密。
常见的对称加密算法有DES、AES等。
非对称加密算法需要一对公钥和私钥,公钥用于加密数据,私钥用于解密数据,安全性更高,适用于加密大规模数据。
常见的非对称加密算法有RSA、ECC等。
在C语言中,我们可以使用openssl库或者自行实现上述加密算法来实现数据加密和解密。
下面以AES对称加密算法为例进行介绍。
首先,我们需要在C语言中引入openssl库,通过以下方式进行安装和引入:```sudo apt-get install libssl-dev``````c#include <openssl/aes.h>#include <openssl/rand.h>```接下来,我们可以定义一个函数来实现数据的加密和解密操作:```cvoid encrypt_decrypt_data(unsigned char *data, int data_len, unsigned char *key, unsigned char *iv, int encrypt) {AES_KEY aes_key;AES_set_encrypt_key(key, 128, &aes_key);if (encrypt) {AES_cbc_encrypt(data, data, data_len, &aes_key, iv, AES_ENCRYPT);} else {AES_cbc_encrypt(data, data, data_len, &aes_key, iv, AES_DECRYPT);}}```在上面的函数中,我们使用AES算法对数据进行加密或解密操作,其中data 为待加密或解密的数据,data_len为数据长度,key为密钥,iv为初始化向量,encrypt为加密标志。
C语言加密解密源程序代码
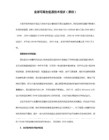
#include<stdio.h>
#include<stdlib.h>
#define M 1000 //预设一个指定文件大小的数字M,方便以后改变
void yiwei() ;
int main()
{
printf("\n$****本程序只能对英文文本进行加密解密操作,如有不便尽请谅解****$");
printf("\n");
printf("\n$******************退出程序输入:1*********************$"); // 欢迎界面
printf("\n");
printf("\n$***************移位加密与解密输入:2******************$"); //
fclose(outfile);
break;
}
printf("\n");
printf("\n*-·-·-感谢您的使用,欢迎再次使用-·-·-*\n\n"); // 使用完感谢语!
exit(0);
}
/*****************调用二进制加密解密函数*********************/
printf(" --> ");
rewind(outfile);
while((data=fgetc(outfile))!=EOF)
printf("%c",data);
printf("\n\n");
ncrack使用方法
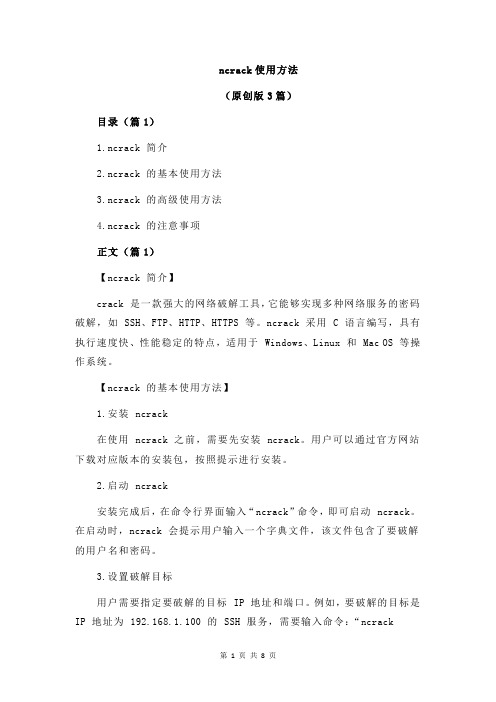
ncrack使用方法(原创版3篇)目录(篇1)1.ncrack 简介2.ncrack 的基本使用方法3.ncrack 的高级使用方法4.ncrack 的注意事项正文(篇1)【ncrack 简介】crack 是一款强大的网络破解工具,它能够实现多种网络服务的密码破解,如 SSH、FTP、HTTP、HTTPS 等。
ncrack 采用 C 语言编写,具有执行速度快、性能稳定的特点,适用于 Windows、Linux 和 Mac OS 等操作系统。
【ncrack 的基本使用方法】1.安装 ncrack在使用 ncrack 之前,需要先安装 ncrack。
用户可以通过官方网站下载对应版本的安装包,按照提示进行安装。
2.启动 ncrack安装完成后,在命令行界面输入“ncrack”命令,即可启动 ncrack。
在启动时,ncrack 会提示用户输入一个字典文件,该文件包含了要破解的用户名和密码。
3.设置破解目标用户需要指定要破解的目标 IP 地址和端口。
例如,要破解的目标是IP 地址为 192.168.1.100 的 SSH 服务,需要输入命令:“ncrack192.168.1.100 22”。
4.开始破解设置好目标后,输入“y”开始破解。
ncrack 会根据字典文件中的信息,尝试对目标进行密码破解。
破解过程中,用户可以通过查看输出信息,了解破解进度。
【ncrack 的高级使用方法】1.使用多个字典文件用户可以指定多个字典文件,以提高破解成功率。
在启动 ncrack 时,输入“L”命令,然后输入字典文件的路径。
2.暴力破解用户可以通过设置字典文件中密码的长度和复杂度,实现暴力破解。
例如,可以设置密码长度为 8 位,包含大小写字母、数字和特殊字符。
3.缓存破解结果为了提高破解速度,用户可以将破解结果进行缓存。
在破解过程中,输入“C”命令,即可将当前破解结果进行缓存。
【ncrack 的注意事项】1.遵守法律法规在使用 ncrack 时,请遵守我国相关法律法规,切勿用于非法目的。
C加密解密算法

1、方法一 (不可逆)public string EncryptPassword(string PasswordString,string PasswordFormat ){string encryptPassword = null;if (PasswordFormat="SHA1"){encryptPassword=FormsAuthortication.HashPasswordForStoringInConfigFile(PasswordS tring,"SHA1");}elseif (PasswordFormat="MD5"){ encryptPassword=FormsAuthortication.HashPasswordForStoringInConfigFile(Passwor dString,"MD5");}return encryptPassword ;}2、方法二 (可逆)public interface IBindesh{string encode(string str);string decode(string str);}public class EncryptionDecryption : IBindesh{public string encode(string str){string htext = "";for ( int i = 0; i < str.Length; i++){htext = htext + (char) (str[i] + 10 - 1 * 2);}return htext;public string decode(string str){string dtext = "";for ( int i=0; i < str.Length; i++){dtext = dtext + (char) (str[i] - 10 + 1*2);}return dtext;}3、方法三 (可逆)const string KEY_64 = "VavicApp";//注意了,是8个字符,64位const string IV_64 = "VavicApp";public string Encode(string data){byte[] byKey = System.Text.ASCIIEncoding.ASCII.GetBytes(KEY_64);byte[] byIV = System.Text.ASCIIEncoding.ASCII.GetBytes(IV_64);DESCryptoServiceProvider cryptoProvider = new DESCryptoServiceProvider();int i = cryptoProvider.KeySize;MemoryStream ms = new MemoryStream();CryptoStream cst = new CryptoStream(ms, cryptoProvider.CreateEncryptor(byKey,byIV), CryptoStreamMode.Write);StreamWriter sw = new StreamWriter(cst);sw.Write(data);sw.Flush();cst.FlushFinalBlock();sw.Flush();return Convert.ToBase64String(ms.GetBuffer(), 0, (int)ms.Length);public string Decode(string data){byte[] byKey = System.Text.ASCIIEncoding.ASCII.GetBytes(KEY_64);byte[] byIV = System.Text.ASCIIEncoding.ASCII.GetBytes(IV_64);byte[] byEnc;try{byEnc = Convert.FromBase64String(data);}catch{return null;}DESCryptoServiceProvider cryptoProvider = new DESCryptoServiceProvider();MemoryStream ms = new MemoryStream(byEnc);CryptoStream cst = new CryptoStream(ms, cryptoProvider.CreateDecryptor(byKey,byIV), CryptoStreamMode.Read);StreamReader sr = new StreamReader(cst);return sr.ReadToEnd();}4、MD5不可逆加密(32位加密)public string GetMD5(string s, string _input_charset){/**//**//**//// <summary>/// 与ASP兼容的MD5加密算法/// </summary>MD5 md5 = new MD5CryptoServiceProvider();byte[] t = puteHash(Encoding.GetEncoding(_input_charset).GetBytes(s));StringBuilder sb = new StringBuilder(32);for (int i = 0; i < t.Length; i++){sb.Append(t[i].ToString("x").PadLeft(2, '0'));}return sb.ToString();}(16位加密)public static string GetMd5Str(string ConvertString){MD5CryptoServiceProvider md5 = new MD5CryptoServiceProvider();string t2 =BitConverter.ToString(puteHash(UTF8Encoding.Default.GetBytes(ConvertStrin g)), 4, 8);t2 = t2.Replace("-", "");return t2;}5、加解文本文件//加密文件private static void EncryptData(String inName, String outName, byte[] desKey, byte[]desIV){//Create the file streams to handle the input and output files.FileStream fin = new FileStream(inName, FileMode.Open, FileAccess.Read); FileStream fout = new FileStream(outName, FileMode.OpenOrCreate, FileAccess.Write);fout.SetLength(0);//Create variables to help with read and write.byte[] bin = new byte[100]; //This is intermediate storage for the encryption.long rdlen = 0; //This is the total number of bytes written. long totlen = fin.Length; //This is the total length of the input file. int len; //This is the number of bytes to be written ata time.DES des = new DESCryptoServiceProvider();CryptoStream encStream = new CryptoStream(fout, des.CreateEncryptor(desKey, desIV),CryptoStreamMode.Write);//Read from the input file, then encrypt and write to the output file.while (rdlen < totlen){len = fin.Read(bin, 0, 100);encStream.Write(bin, 0, len);rdlen = rdlen + len;}encStream.Close();fout.Close();fin.Close();}//解密文件private static void DecryptData(String inName, String outName, byte[] desKey, byte[]desIV){//Create the file streams to handle the input and output files.FileStream fin = new FileStream(inName, FileMode.Open, FileAccess.Read); FileStream fout = new FileStream(outName, FileMode.OpenOrCreate, FileAccess.Write);fout.SetLength(0);//Create variables to help with read and write.byte[] bin = new byte[100]; //This is intermediate storage for the encryption.long rdlen = 0; //This is the total number of bytes written. long totlen = fin.Length; //This is the total length of the input file. int len; //This is the number of bytes to be written at a time.DES des = new DESCryptoServiceProvider();CryptoStream encStream = new CryptoStream(fout, des.CreateDecryptor(desKey, desIV),CryptoStreamMode.Write);//Read from the input file, then encrypt and write to the output file. while (rdlen < totlen){len = fin.Read(bin, 0, 100);encStream.Write(bin, 0, len);rdlen = rdlen + len;}encStream.Close();fout.Close();fin.Close();}6、using System;using System.Collections.Generic;using System.Text;using System.Security.Cryptography;using System.IO;namespace Component{public class Security{public Security(){}//默认密钥向量private static byte[] Keys = { 0x12, 0x34, 0x56, 0x78, 0x90, 0xAB, 0xCD, 0xEF };/**//**//**//**//**//**//**//// <summary>/// DES加密字符串/// </summary>/// <param name="encryptString">待加密的字符串</param>/// <param name="encryptKey">加密密钥,要求为8位</param>/// <returns>加密成功返回加密后的字符串,失败返回源串</returns>public static string EncryptDES(string encryptString, string encryptKey){try{byte[] rgbKey = Encoding.UTF8.GetBytes(encryptKey.Substring(0, 8));byte[] rgbIV = Keys;byte[] inputByteArray = Encoding.UTF8.GetBytes(encryptString); DESCryptoServiceProvider dCSP = new DESCryptoServiceProvider(); MemoryStream mStream = new MemoryStream();CryptoStream cStream = new CryptoStream(mStream, dCSP.CreateEncryptor(rgbKey,rgbIV), CryptoStreamMode.Write);cStream.Write(inputByteArray, 0, inputByteArray.Length);cStream.FlushFinalBlock();return Convert.ToBase64String(mStream.ToArray());}catch{return encryptString;}}/**//**//**//**//**//**//**//// <summary>/// DES解密字符串/// </summary>/// <param name="decryptString">待解密的字符串</param>/// <param name="decryptKey">解密密钥,要求为8位,和加密密钥相同</param> /// <returns>解密成功返回解密后的字符串,失败返源串</returns>public static string DecryptDES(string decryptString, string decryptKey) {try{byte[] rgbKey = Encoding.UTF8.GetBytes(decryptKey);byte[] rgbIV = Keys;byte[] inputByteArray = Convert.FromBase64String(decryptString); DESCryptoServiceProvider DCSP = new DESCryptoServiceProvider(); MemoryStream mStream = new MemoryStream();CryptoStream cStream = new CryptoStream(mStream, DCSP.CreateDecryptor(rgbKey,rgbIV), CryptoStreamMode.Write);cStream.Write(inputByteArray, 0, inputByteArray.Length);cStream.FlushFinalBlock();return Encoding.UTF8.GetString(mStream.ToArray()); }catch{return decryptString;}}}}。
c语言解密代码
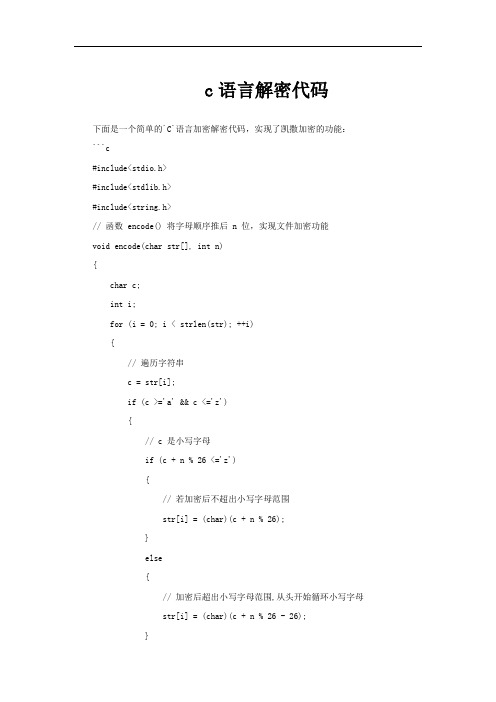
c语言解密代码下面是一个简单的`C`语言加密解密代码,实现了凯撒加密的功能:```c#include<stdio.h>#include<stdlib.h>#include<string.h>// 函数 encode() 将字母顺序推后 n 位,实现文件加密功能void encode(char str[], int n){char c;int i;for (i = 0; i < strlen(str); ++i){// 遍历字符串c = str[i];if (c >='a' && c <='z'){// c 是小写字母if (c + n % 26 <='z'){// 若加密后不超出小写字母范围str[i] = (char)(c + n % 26);}else{// 加密后超出小写字母范围,从头开始循环小写字母 str[i] = (char)(c + n % 26 - 26);}elseif (c >='A' && c <='Z'){// c 为大写字母if (c + n % 26 <= 'Z'){// 加密后不超出大写字母范围str[i] = (char)(c + n % 26);}else{// 加密后超出大写字母范围,从头开始循环大写字母 str[i] = (char)(c + n % 26 - 26);}}else{// 不是字母,不加密str[i] = c;printf("\nAfter encode: \n");puts(str);}}// 输出加密后的字符串printf("\nAfter encode: \n");puts(str);}// 实现解密功能,将字母顺序前移 n 位void decode(char str[], int n){int i;for (i = 0; i < strlen(str); ++i){c = str[i];if (c >='a' && c <='z'){// 解密后还为小写字母,直接解密if (c - n % 26 >='a'){str[i] = (char)(c - n % 26);}else{// 解密后不为小写字母了,通过循环小写字母处理为小写字母 str[i] = (char)(c - n % 26 + 26);}}elseif (c >= 'A' && c <='Z'){// c 为大写字母if (c - n % 26 >='A'){// 解密后还为大写字母str[i] = (char)(c - n % 26);}else{// 解密后不为大写字母了,循环大写字母,处理为大写字母str[i] = (char)(c - n % 26 + 26);}}else{// 不是字母,不加密str[i] = c;}}// 输出解密后的字符串printf("\nAfter decode: \n");puts(str);}int main(){char str[20];int n;printf("请输入字符串(以空格结束输入):\n");gets(str);printf("请输入密钥(1-25的整数):\n");scanf("%d", &n);printf("加密前的字符串为:%s\n", str);encode(str, n);printf("加密后的字符串为:%s\n", str);decode(str, n);printf("解密后的字符串为:%s\n", str);return 0;}```在上述代码中,加密函数`encode()`通过将字符串中的每个字符循环向后移动`n`位实现加密,解密函数`decode()`通过将字符串中的每个字符循环向前移动`n`位实现解密。