程序设计综合训练
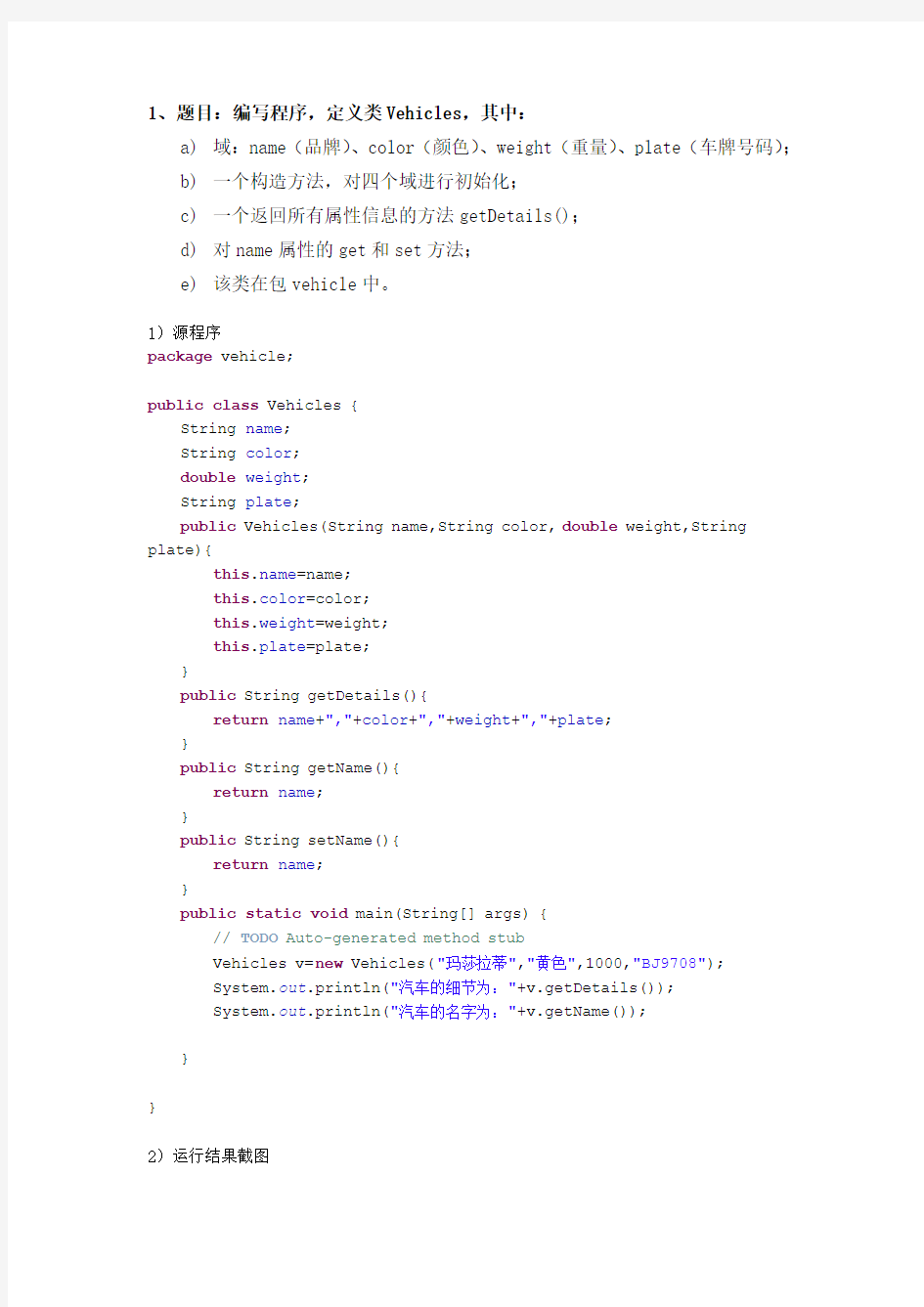
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
1、题目:编写程序,定义类Vehicles,其中:
a)域:name(品牌)、color(颜色)、weight(重量)、plate(车牌号码);
b)一个构造方法,对四个域进行初始化;
c)一个返回所有属性信息的方法getDetails();
d)对name属性的get和set方法;
e)该类在包vehicle中。
1)源程序
package vehicle;
public class Vehicles {
String name;
String color;
double weight;
String plate;
public Vehicles(String name,String color,double weight,String plate){
=name;
this.color=color;
this.weight=weight;
this.plate=plate;
}
public String getDetails(){
return name+","+color+","+weight+","+plate;
}
public String getName(){
return name;
}
public String setName(){
return name;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Vehicles v=new Vehicles("玛莎拉蒂","黄色",1000,"BJ9708");
System.out.println("汽车的细节为:"+v.getDetails());
System.out.println("汽车的名字为:"+v.getName());
}
}
2)运行结果截图
汽车的细节为:玛莎拉蒂,黄色,1000.0,BJ9708
汽车的名字为:玛莎拉蒂
2.题目:编写程序,定义类Car,它是类Vehicles的子类。其中:
a)该类在包vehicle中;
b)域:seats(座位数),speed(速度);
c)对seats的get和set方法;
d)构造方法,对6个域进行初始化,对继承自父类的域,调用父类的构造
方法进行初始化;
e)覆盖父类中的getDetails()方法,返回所有域的信息;
f)对speed的speedUp()和slowDown()方法,通过方法的参数修改speed
的值。其中,最高时速不超过200,最低时速不低于0,在方法中实现对
速度的合法性检查;
g)main方法:定义两个Car类型的对象,分别调用getDetails()方法返回
这两个对象的信息,调用speedUp()和slowDown()方法改变Car对象的
速度。
1)源程序
package vehicle;
public class Car extends Vehicles{
int seats;
double speed;
public int get_seats(){
return seats;
}
public int set_seats(){
return seats;
}
public Car(String name, String color, double weight, String plate,int seats,double speed) {
super(name, color, weight, plate);
this.seats=seats;
this.speed=speed;
}
public String getDetails(){
return
name+","+color+","+weight+","+plate+","+seats+","+speed;
}
public String speedUp(){
if (speed>200)
return"您超速了!";
else
return"您的速度合法,小于最高速度";
}
public String slowDown(){
if (speed<0)
return"您应该提速!";
else
return"您的速度合法,大于最低速度";
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Car ca=new Car("宾利","黑色",1500,"BJ9999",4,236);
Car c=new Car("福特","白色",1000,"BJ7766",1,185);
System.out.println("汽车的细节为:"+ca.getDetails());
System.out.println("该汽车的速度为:"+ca.speedUp());
System.out.println("该汽车的速度为:"+ca.slowDown());
System.out.println("汽车的细节为:"+c.getDetails());
System.out.println("该汽车的速度为:"+c.speedUp());
System.out.println("该汽车的速度为:"+c.slowDown());
}
}
2)运行结果截图
汽车的细节为:宾利,黑色,1500.0,BJ9999,4,236.0
该汽车的速度为:您超速了!
该汽车的速度为:您的速度合法,大于最低速度
汽车的细节为:福特,白色,1000.0,BJ7766,1,185.0
该汽车的速度为:您的速度合法,小于最高速度
该汽车的速度为:您的速度合法,大于最低速度