6数据结构实验
数据结构 实验

数据结构实验数据结构实验1. 实验目的通过本次实验,掌握数据结构的基本概念,了解常见的数据结构和其应用。
2. 实验内容本次实验主要包括以下内容:1. 数组:了解数组的概念、特点和基本操作;掌握数组的存储方式和访问方法;基于数组实现常见数据结构(如栈、队列)。
2. 链表:理解链表的概念及其不同类型(如单向链表、双向链表);学习链表的插入、删除和查找操作;比较链表和数组的优缺点。
3. 树:了解树的基本概念及其特点;学习树的存储方式和遍历方法;掌握二叉树的特点和基本操作(如插入、删除、查找)。
4. 图:理解图的基本概念和相关术语;学习图的存储方式和遍历方法;掌握图的常见算法(如深度优先搜索、广度优先搜索)。
3. 实验步骤根据实验内容,按以下步骤进行实验:3.1 数组1. 了解数组的概念和特点,例如数组是一种线性表数据结构,用于存储连续的元素。
2. 学习数组的基本操作,如创建数组、访问数组元素、修改数组元素的值。
3. 理解数组的存储方式,例如元素在内存中是连续存储的。
4. 实现栈和队列的基本功能,并使用数组作为底层数据结构。
3.2 链表1. 理解链表的概念及其不同类型,如单向链表和双向链表。
2. 学习链表的插入、删除和查找操作,例如在链表中插入一个节点、删除一个节点、查找指定值的节点。
3. 比较链表和数组的优缺点,例如链表的插入和删除操作效率更高,但访问元素的效率较低。
4. 实现链表的基本功能,如创建链表、插入节点、删除节点、查找节点。
3.3 树1. 了解树的基本概念和特点,如树由节点和边组成,每个节点最多有一个父节点和多个子节点。
2. 学习树的存储方式,如链式存储和数组存储。
3. 掌握二叉树的特点和基本操作,如二叉树是每个节点最多有两个子节点的树结构。
4. 实现二叉树的基本功能,如插入节点、删除节点、查找节点。
3.4 图1. 理解图的基本概念和相关术语,如图由顶点和边组成。
2. 学习图的存储方式,如邻接矩阵和邻接表。
数据结构实验报告

数据结构实验报告数据结构实验报告1-引言本实验旨在深入理解数据结构的基本知识,并通过实践掌握相关算法和数据结构的应用。
本报告详细描述了实验的背景、目的、实验环境、实验内容和实验结果分析等内容。
2-实验背景介绍数据结构的概念和作用,解释为什么数据结构在计算机科学中至关重要。
同时,介绍本次实验所涉及的具体数据结构和算法,如数组、链表、栈、队列、二叉树等。
3-实验目的明确本次实验的目标,如掌握数据结构的基本操作,理解不同数据结构的适用场景,评估不同算法的时间和空间复杂度等。
4-实验环境描述实验所使用的软硬件环境,包括计算机配置、操作系统、编程语言和相关的开发工具等。
5-实验内容详细描述实验的具体步骤和要求,包括以下几个部分:5-1 数据结构的创建和初始化:例如,创建一个数组或链表,并初始化数据。
5-2 数据结构的插入和删除操作:例如,在数组中插入一个元素或删除一个元素。
5-3 数据结构的遍历和搜索:例如,遍历树的节点或搜索链表中指定的元素。
5-4 数据结构的排序和查找:例如,对数组进行排序或在有序链表中查找指定元素。
5-5 实验的额外要求:例如,优化算法的实现、分析不同数据结构的性能等。
6-实验结果分析对实验的结果进行详细的分析和解释,包括各个数据结构和算法的性能比较、时间复杂度和空间复杂度的评估等。
7-结论总结本次实验的主要内容和收获,归纳实验结果,并对实验过程中遇到的问题和不足进行反思和改进。
附件:随报告一同提交的附件包括:源代码、实验数据集等相关文件。
法律名词及注释:1-版权:指作品的创作权、发表权和署名权等综合权利。
2-侵权:指未经权利人允许,在未向权利人支付报酬的情况下,使用受版权保护的作品的行为。
3-知识产权:包括著作权、商标权、专利权等,是指人们在创造性劳动中创造出的精神财富所享有的权利。
数据结构实验报告简版

数据结构实验报告数据结构实验报告实验目的本实验旨在通过实践,加深对数据结构的理解,掌握数据结构的基本操作,并学会运用数据结构解决实际问题。
实验背景数据结构是计算机科学中非常重要的基础知识,它是研究各种数据结构及其相应算法的学科。
数据结构可以提供对数据的组织、存储和管理方式,从而有效地支持计算机程序的设计和运行。
实验内容本实验主要包括以下几个方面的内容:1. 线性表的操作- 插入操作:向线性表的指定位置插入元素。
- 删除操作:从线性表中删除指定位置的元素。
- 查找操作:在线性表中查找指定元素。
- 遍历操作:依次访问线性表中的所有元素。
2. 栈的应用- 中缀表达式转后缀表达式:将带有括号的中缀表达式转换为无括号的后缀表达式。
- 后缀表达式求值:根据后缀表达式计算其值。
3. 队列的应用- 模拟打印任务:根据打印任务的到达时间和执行时间,模拟打印机的工作过程。
4. 递归的应用- 计算斐波那契数列:通过递归函数计算斐波那契数列的第n 项值。
实验步骤根据实验内容,进行以下步骤:1. 线性表的操作1. 初始化线性表。
2. 实现插入操作,并在指定位置插入元素。
3. 实现删除操作,并从指定位置删除元素。
4. 实现查找操作,并根据指定元素在线性表中查找。
5. 实现遍历操作,并依次访问线性表中的所有元素。
2. 栈的应用1. 实现中缀表达式转后缀表达式的函数,并进行测试。
2. 实现后缀表达式求值的函数,并进行测试。
3. 队列的应用1. 实现模拟打印任务的函数,并根据指定的打印任务进行测试。
4. 递归的应用1. 实现计算斐波那契数列的递归函数,并计算第n项的值。
实验结果经过上述步骤的实现和测试,得到以下实验结果:- 线性表的操作:插入、删除、查找和遍历操作均得到正确的结果。
- 栈的应用:中缀表达式转后缀表达式和后缀表达式求值的函数均能正确运行。
- 队列的应用:模拟打印任务的函数能够按照指定的顺序执行打印任务。
- 递归的应用:计算斐波那契数列的递归函数能够正确计算任意一项的值。
数据结构实验

数据结构实验数据结构实验是计算机科学与技术专业的重要课程之一。
通过对这门课程的学习和实验,可以让学生深入了解数据结构在计算机科学中的重要性和应用。
一、实验的目的与意义数据结构实验的主要目的是帮助学生更深入地理解数据结构在计算机科学中的应用。
在实验中,学生可以通过编写代码和执行各种数据结构算法来更好地理解数据结构的实现原理。
通过实验,学生可以更清楚地了解算法的效率、时间复杂度和空间复杂度等概念。
此外,数据结构实验也有助于提高学生的编程能力。
在实验中,学生需要编写具有规范的代码,确保算法的正确性,同时还需要处理大量的数据,这可以提高学生的编程能力和耐心。
二、实验内容简介数据结构实验通常包括以下几个方面的内容:1.线性结构:顺序存储和链式存储线性表、栈、队列等。
2.非线性结构:数组、链表、二叉树等。
3.查找算法:顺序查找、二分查找、哈希查找等。
4.排序算法:插入排序、选择排序、归并排序、堆排序等。
5.图论算法:图的遍历、最短路径、最小生成树等。
6.字符串算法:KMP算法、BM算法等。
三、实验中的具体操作实验中的具体操作是根据具体的算法和数据结构来进行的。
以下是一个简单的例子:线性表的实验假设学生已经学习了顺序存储结构和链式存储结构的操作,以下是在实验中需要进行的具体操作:1.顺序存储结构创建一个空的顺序表插入一个元素到指定位置删除一个元素查找指定元素的位置输出顺序表的所有元素2.链式存储结构创建一个空的链表插入一个元素到指定位置删除一个元素查找指定元素的位置输出链表的所有元素在实验中,学生需要将这些操作封装成具体的函数,并且通过调用这些函数来实现对线性表的操作。
同时,学生还需要进行大量的测试和调试,以保证代码的正确性和实验的效果。
四、实验中的注意事项在进行数据结构实验时,学生需要注意以下几个方面:1.理论和实验相结合:不仅要理解理论知识,还要进行实验操作,才能更好地掌握数据结构。
2.代码规范:要写出规范、可读性强的代码,让他人容易理解。
数据结构实验总结

数据结构实验总结数据结构实验是计算机科学与技术专业的一门重要实践课程,通过实际操作和实验验证,帮助学生理解和掌握各种常见的数据结构及其应用。
本文将对数据结构实验进行总结,包括实验目的、实验内容、实验过程和实验收获等方面。
一、实验目的数据结构实验的主要目的是帮助学生:1. 理解数据结构的基本概念和原理;2. 掌握各种数据结构的特点、操作和应用场景;3. 学会使用编程语言实现各种数据结构;4. 分析和解决实际问题时,选择合适的数据结构和算法。
二、实验内容数据结构实验通常包括以下几个方面的内容:1. 线性表:实现顺序表和链表,并比较它们在插入、删除、查找等操作上的性能差异;2. 栈和队列:实现顺序栈、链栈、顺序队列和链队列,并应用于实际问题中;3. 树:实现二叉树、二叉搜索树、平衡二叉树等,并进行遍历、插入、删除等操作;4. 图:实现有向图和无向图,并进行深度优先搜索和广度优先搜索;5. 排序和查找:实现各种排序算法(如冒泡排序、插入排序、快速排序等)和查找算法(如顺序查找、二分查找等);6. 哈希表:实现哈希表,并解决冲突问题;7. 字符串:实现字符串的匹配算法(如KMP算法);8. 综合实验:综合应用各种数据结构解决实际问题。
三、实验过程数据结构实验的进行通常包括以下几个步骤:1. 理解实验要求和目标,阅读实验指导书和相关资料;2. 设计实验方案,包括选择适当的数据结构和算法,并合理安排实验的步骤和操作;3. 编写程序代码,实现所选数据结构及其相关操作;4. 运行程序,测试和调试,确保程序的正确性和稳定性;5. 进行实验数据的收集和分析,比较不同数据结构和算法的性能差异;6. 总结实验结果,得出结论,分析实验中遇到的问题及解决方法;7. 撰写实验报告,包括实验目的、内容、过程、结果和分析等内容。
四、实验收获通过数据结构实验的学习和实践,我获得了以下几方面的收获:1. 对各种常见的数据结构有了更深入的理解,包括它们的特点、操作和应用场景;2. 学会使用编程语言实现各种数据结构,并掌握了相应的算法;3. 锻炼了分析和解决实际问题的能力,能够选择合适的数据结构和算法;4. 培养了团队合作和沟通能力,在与同学们一起完成实验任务的过程中,学会了相互配合和交流;5. 培养了耐心和细致的工作态度,实验过程中需要不断调试和优化,要求我保持耐心和细致地分析问题。
数据结构实训实验报告

一、实验背景数据结构是计算机科学中一个重要的基础学科,它研究如何有效地组织和存储数据,并实现对数据的检索、插入、删除等操作。
为了更好地理解数据结构的概念和原理,我们进行了一次数据结构实训实验,通过实际操作来加深对数据结构的认识。
二、实验目的1. 掌握常见数据结构(如线性表、栈、队列、树、图等)的定义、特点及操作方法。
2. 熟练运用数据结构解决实际问题,提高算法设计能力。
3. 培养团队合作精神,提高实验报告撰写能力。
三、实验内容本次实验主要包括以下内容:1. 线性表(1)实现线性表的顺序存储和链式存储。
(2)实现线性表的插入、删除、查找等操作。
2. 栈与队列(1)实现栈的顺序存储和链式存储。
(2)实现栈的入栈、出栈、判断栈空等操作。
(3)实现队列的顺序存储和链式存储。
(4)实现队列的入队、出队、判断队空等操作。
3. 树与图(1)实现二叉树的顺序存储和链式存储。
(2)实现二叉树的遍历、查找、插入、删除等操作。
(3)实现图的邻接矩阵和邻接表存储。
(4)实现图的深度优先遍历和广度优先遍历。
4. 算法设计与应用(1)实现冒泡排序、选择排序、插入排序等基本排序算法。
(2)实现二分查找算法。
(3)设计并实现一个简单的学生成绩管理系统。
四、实验步骤1. 熟悉实验要求,明确实验目的和内容。
2. 编写代码实现实验内容,对每个数据结构进行测试。
3. 对实验结果进行分析,总结实验过程中的问题和经验。
4. 撰写实验报告,包括实验目的、内容、步骤、结果分析等。
五、实验结果与分析1. 线性表(1)顺序存储的线性表实现简单,但插入和删除操作效率较低。
(2)链式存储的线性表插入和删除操作效率较高,但存储空间占用较大。
2. 栈与队列(1)栈和队列的顺序存储和链式存储实现简单,但顺序存储空间利用率较低。
(2)栈和队列的入栈、出队、判断空等操作实现简单,但需要考虑数据结构的边界条件。
3. 树与图(1)二叉树和图的存储结构实现复杂,但能够有效地表示和处理数据。
数据结构图实验报告
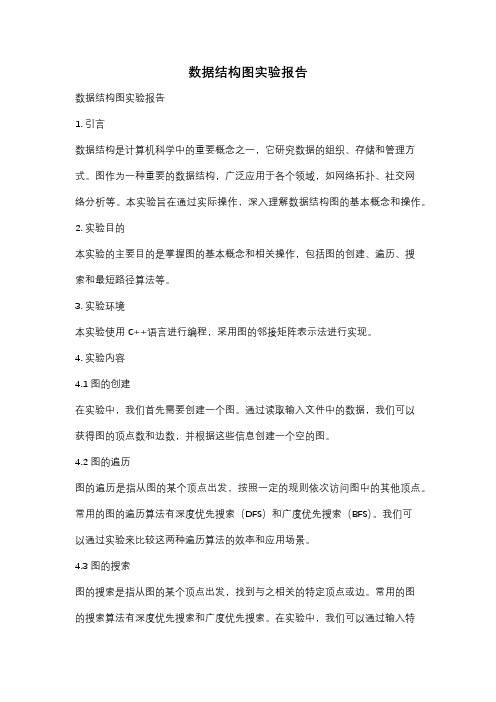
数据结构图实验报告数据结构图实验报告1. 引言数据结构是计算机科学中的重要概念之一,它研究数据的组织、存储和管理方式。
图作为一种重要的数据结构,广泛应用于各个领域,如网络拓扑、社交网络分析等。
本实验旨在通过实际操作,深入理解数据结构图的基本概念和操作。
2. 实验目的本实验的主要目的是掌握图的基本概念和相关操作,包括图的创建、遍历、搜索和最短路径算法等。
3. 实验环境本实验使用C++语言进行编程,采用图的邻接矩阵表示法进行实现。
4. 实验内容4.1 图的创建在实验中,我们首先需要创建一个图。
通过读取输入文件中的数据,我们可以获得图的顶点数和边数,并根据这些信息创建一个空的图。
4.2 图的遍历图的遍历是指从图的某个顶点出发,按照一定的规则依次访问图中的其他顶点。
常用的图的遍历算法有深度优先搜索(DFS)和广度优先搜索(BFS)。
我们可以通过实验来比较这两种遍历算法的效率和应用场景。
4.3 图的搜索图的搜索是指从图的某个顶点出发,找到与之相关的特定顶点或边。
常用的图的搜索算法有深度优先搜索和广度优先搜索。
在实验中,我们可以通过输入特定的顶点或边,来观察图的搜索算法的执行过程和结果。
4.4 图的最短路径算法图的最短路径算法是指在图中找到两个顶点之间的最短路径。
常用的最短路径算法有迪杰斯特拉算法和弗洛伊德算法。
通过实验,我们可以比较这两种算法的执行效率和应用场景。
5. 实验结果与分析通过实验,我们可以得到以下结论:- 图的邻接矩阵表示法在创建和操作图的过程中具有较高的效率。
- 深度优先搜索算法适用于查找图中的连通分量和回路等问题。
- 广度优先搜索算法适用于查找图中的最短路径和最小生成树等问题。
- 迪杰斯特拉算法适用于求解单源最短路径问题,而弗洛伊德算法适用于求解多源最短路径问题。
6. 实验总结通过本次实验,我们深入学习了数据结构图的基本概念和相关操作。
图作为一种重要的数据结构,具有广泛的应用价值。
在今后的学习和工作中,我们可以运用所学的知识,解决实际问题,提高工作效率。
数据结构实验报告

数据结构实验报告一、实验目的数据结构是计算机科学中重要的基础课程,通过本次实验,旨在深入理解和掌握常见数据结构的基本概念、操作方法以及在实际问题中的应用。
具体目的包括:1、熟练掌握线性表(如顺序表、链表)的基本操作,如插入、删除、查找等。
2、理解栈和队列的特性,并能够实现其基本操作。
3、掌握树(二叉树、二叉搜索树)的遍历算法和基本操作。
4、学会使用图的数据结构,并实现图的遍历和相关算法。
二、实验环境本次实验使用的编程环境为具体编程环境名称,编程语言为具体编程语言名称。
三、实验内容及步骤(一)线性表的实现与操作1、顺序表的实现定义顺序表的数据结构,包括数组和表的长度等。
实现顺序表的初始化、插入、删除和查找操作。
2、链表的实现定义链表的节点结构,包含数据域和指针域。
实现链表的创建、插入、删除和查找操作。
(二)栈和队列的实现1、栈的实现使用数组或链表实现栈的数据结构。
实现栈的入栈、出栈和栈顶元素获取操作。
2、队列的实现采用循环队列的方式实现队列的数据结构。
完成队列的入队、出队和队头队尾元素获取操作。
(三)树的实现与遍历1、二叉树的创建以递归或迭代的方式创建二叉树。
2、二叉树的遍历实现前序遍历、中序遍历和后序遍历算法。
3、二叉搜索树的操作实现二叉搜索树的插入、删除和查找操作。
(四)图的实现与遍历1、图的表示使用邻接矩阵或邻接表来表示图的数据结构。
2、图的遍历实现深度优先遍历和广度优先遍历算法。
四、实验结果与分析(一)线性表1、顺序表插入操作在表尾进行时效率较高,在表头或中间位置插入时需要移动大量元素,时间复杂度较高。
删除操作同理,在表尾删除效率高,在表头或中间删除需要移动元素。
2、链表插入和删除操作只需修改指针,时间复杂度较低,但查找操作需要遍历链表,效率相对较低。
(二)栈和队列1、栈栈的特点是先进后出,适用于函数调用、表达式求值等场景。
入栈和出栈操作的时间复杂度均为 O(1)。
2、队列队列的特点是先进先出,常用于排队、任务调度等场景。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
if(x == '#' ) {
bt = NULL; } else {
bt = new BiTNode; bt->data = x; bt->lchild = this->CreateBiTree(); bt->rchild = this->CreateBiTree();
} this->T = bt; return bt; };
void BITREE::InOrderTraverse(BiTree bt) {
if(bt != NULL) {
if(bt->lchild != NULL) {
this->InOrderTraverse(bt->lchild); } cout<<bt->data<<" "; if(bt->rchild != NULL) {
//用循环链表实现
{
int i,j;
peo *p,*q,*head;
head=p=q=(peo *)malloc(sizeof(peo));
p->num=0;p->next=head;
for(i=1;i<n;i++)
{ቤተ መጻሕፍቲ ባይዱ
p=(peo *)malloc(sizeof(peo));
p->num=i;q->next=p;p->next=head;
this->Destory(bt->lchild); }
if(bt->rchild != NULL) {
this->Destory(bt->rchild); } cout<<bt->data<<" "; delete bt; } else { return;
} } 关于图: #include "MGraph.h"
preOrder(*newBTree); deleteNode(newBTree,5); printf("\n---------midOrder-----"); midOrder(*newBTree); printf("\n---------preOrder-----"); preOrder(*newBTree); printf("\n");
void preOrder(PBinTreeNode ptree) {
PBinTreeNode p;
p=ptree; if(p==NULL)return; printf(" %d ",p->key); preOrder(p->llink); preOrder(p->rlink); }
void postOrder(PBinTreeNode ptree) {
free(position); } } int main() { PBinTree newBTree; insertNode(newBTree,5); insertNode(newBTree,3); insertNode(newBTree,7); insertNode(newBTree,2); insertNode(newBTree,4); insertNode(newBTree,6); insertNode(newBTree,8); insertNode(newBTree,1); printf("---------midOrder-----"); midOrder(*newBTree); printf("\n---------preOrder-----");
printf("\n 是根节点!"); tag=0; } else
printf("\n 它的父节点是%d",parent->key); if(position->llink==NULL){
if(tag==-1)parent->llink=position->rlink; if(tag==1)parent->rlink=position->rlink; free(position); }else{ PBinTreeNode trchild=position->llink; while(trchild->rlink!=NULL){ trchild=trchild->rlink; } trchild->rlink=position->rlink; if(tag==-1)parent->llink=position->llink; if(tag==1)parent->rlink=position->llink; if(tag==0)*pbtree=position->llink;
}
void insertNode(PBinTree ptree,KeyType key) {
PBinTreeNode p,position; if(searchNode(ptree,key,&position)==TRUE) return; p=(PBinTreeNode)malloc(sizeof(struct BinTreeNode)); if(p==NULL){printf("Error!\n");exit(1);} p->key=key; p->llink=p->rlink=NULL; if(position==NULL) *ptree=p; else if(key<position->key)
this->InOrderTraverse(bt->rchild); } } else { return; } //-+a##*b##-c##d##/e##f## }; void BITREE::Destory(BiTree bt) { if(bt != NULL) { if(bt->lchild != NULL) {
void deleteNode(PBinTree pbtree,KeyType key) {
PBinTreeNode position,p,q,parent; p=*pbtree,parent=p; int tag=0; position=NULL; while(p!=NULL){ // printf("%d",p->key); if(p->key==key){
【实验内容】(问题描述,数据描述,算法描述,程序清单,测试数据) 二叉树采用二叉链表作存储结构,用编程实现二叉树的如下基本操作: 1. 按先序序列构造一棵二叉链表表示的二叉树 T; 2. 对这棵二叉树进行中序遍历,输出结点的遍历序列; 3. 给定的顶点和边的信息构造图的邻接矩阵存储; 4. 对改图进行深度优先搜索,输出搜索得到的结点序列。 【实验要求】 上交实验报告,要求同上。 关于树: #include <iostream> #include "BiTree.h" using namespace std; BiTree BITREE::CreateBiTree() {
①实验名称 ②实验目的 ③实验内容:问题描述:数据描述:算法描述:程序清单:测试数据 算法: #include <stdio.h> #include <stdlib.h> typedef struct LPeople { int num; struct LPeople *next; }peo;
void Joseph(int n,int m)
KeyType key; DataType other; /*元素的属性*/ PBinTreeNode llink,rlink; }; typedef struct BinTreeNode * BinTree; typedef BinTree * PBinTree;
int searchNode(PBinTree ptree,KeyType key,PBinTreeNode *position)
【实验内容】 约瑟夫问题的实现:n 只猴子要选猴王,所有的猴子按 1,2,…,n 编号围坐一圈,从第 一号开始按 1,2…,m 报数,凡报到 m 号的猴子退出圈外,如此次循环报数,知道圈内 剩下一只猴子时,这个猴子就是猴王。编写一个程序实现上述过程,n 和 m 由键盘输入。 【实验要求】 1、 要求用顺序表和链表分别实现约瑟夫问题。 2、 独立完成,严禁抄袭。 3、 上的实验报告有如下部分组成:
【实验内容】 1、 要求将二叉排序树的建立、插入、删除、显示等算法合并在一个综合程序中,用户
可通过菜单选择方式运行各种操作算法。 2、 一直哈希表的表长为 m,哈希函数为 H(key)=key MOD p,用开放定址法(增量序
列采用线性探测再散列)解决冲突,试编写构造哈希表的程序。 二叉排排序树:
#include<stdio.h> #include<malloc.h> #define TRUE 1 #define FALSE 0 typedef int DataType; typedef int KeyType; struct BinTreeNode; typedef struct BinTreeNode * PBinTreeNode; struct BinTreeNode {
哈希表:
#include<malloc.h> #define NULL 0 typedef char * InfoType; typedef int KeyType; typedef struct node {
position=p; break; }else if(p->key>key){ parent=p; p=p->llink; tag=-1; }else { parent=p; p=p->rlink;