(完整版)数据结构实验答案及解析
数据结构实验报告答案

数据结构实验报告答案数据结构实验报告答案引言:数据结构是计算机科学中的重要概念,它涉及组织和管理数据的方法和技术。
在本次实验中,我们将研究和实践几种常见的数据结构,包括数组、链表、栈和队列。
通过这些实验,我们将深入理解数据结构的原理和应用。
一、数组数组是一种线性数据结构,它由一系列相同类型的元素组成。
数组的特点是可以通过索引来访问和修改元素,具有随机访问的能力。
在本次实验中,我们将实现一个简单的数组类,并进行一些基本操作,如插入、删除和查找。
首先,我们定义一个数组类,包含以下成员变量和方法:- size:数组的大小- elements:存储元素的数组- insert(index, element):在指定位置插入元素- remove(index):删除指定位置的元素- get(index):获取指定位置的元素- search(element):查找元素在数组中的位置通过实现上述方法,我们可以对数组进行各种操作。
例如,我们可以在数组的末尾插入一个元素,然后在指定位置删除一个元素。
我们还可以通过元素的值来查找其在数组中的位置。
二、链表链表是另一种常见的线性数据结构,它由一系列节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。
链表的特点是插入和删除操作的效率较高,但随机访问的效率较低。
在本次实验中,我们将实现一个简单的单向链表,并进行一些基本操作。
首先,我们定义一个节点类,包含以下成员变量和方法:- data:节点的数据元素- next:指向下一个节点的指针然后,我们定义一个链表类,包含以下成员变量和方法:- head:链表的头节点- insert(element):在链表的末尾插入一个节点- remove(element):删除链表中指定的节点- search(element):查找链表中指定元素的节点通过实现上述方法,我们可以对链表进行各种操作。
例如,我们可以在链表的末尾插入一个节点,然后删除链表中指定的节点。
数据结构实验报告-答案.doc

数据结构实验报告-答案数据结构(C语言版)实验报告专业班级学号姓名实验1实验题目:单链表的插入和删除实验目的:了解和掌握线性表的逻辑结构和链式存储结构,掌握单链表的基本算法及相关的时间性能分析。
实验要求:建立一个数据域定义为字符串的单链表,在链表中不允许有重复的字符串;根据输入的字符串,先找到相应的结点,后删除之。
实验主要步骤:1、分析、理解给出的示例程序。
2、调试程序,并设计输入数据(如:bat,cat,eat,fat,hat,jat,lat,mat,#),测试程序的如下功能:不允许重复字符串的插入;根据输入的字符串,找到相应的结点并删除。
3、修改程序:(1)增加插入结点的功能。
(2)将建立链表的方法改为头插入法。
程序代码:#include“stdio.h“#include“string.h“#include“stdlib.h“#include“ctype. h“typedefstructnode//定义结点{chardata[10];//结点的数据域为字符串structnode*next;//结点的指针域}ListNode;typedefListNode*LinkList;//自定义LinkList单链表类型LinkListCreatListR1();//函数,用尾插入法建立带头结点的单链表LinkListCreatList(void);//函数,用头插入法建立带头结点的单链表ListNode*LocateNode();//函数,按值查找结点voidDeleteList();//函数,删除指定值的结点voidprintlist();//函数,打印链表中的所有值voidDeleteAll();//函数,删除所有结点,释放内存ListNode*AddNode();//修改程序:增加节点。
用头插法,返回头指针//==========主函数==============voidmain(){charch[10],num[5];LinkListhead;head=C reatList();//用头插入法建立单链表,返回头指针printlist(head);//遍历链表输出其值printf(“Deletenode(y/n):“);//输入“y“或“n“去选择是否删除结点scanf(“%s“,num);if(strcmp(num,“y“)==0||strcmp(num,“Y“)==0){printf(“PleaseinputDelete_data:“);scanf(“%s“,ch);//输入要删除的字符串DeleteList(head,ch);printlist(head);}printf(“Addnode?(y/n):“);//输入“y“或“n“去选择是否增加结点scanf(“%s“,num);if(strcmp(num,“y“)==0||strcmp(num,“Y“)==0){head=A ddNode(head);}printlist(head);DeleteAll(head);//删除所有结点,释放内存}//==========用尾插入法建立带头结点的单链表===========LinkListCreatListR1(void){charch[10];LinkListhead=(Li nkList)malloc(sizeof(ListNode));//生成头结点ListNode*s,*r,*pp;r=head;r->next=NULL;printf(“Input#toend“);//输入“#“代表输入结束printf(“\nPleaseinputN ode_data:“);scanf(“%s“,ch);//输入各结点的字符串while(strcmp(ch,“#“)!=0){pp=LocateNode(head,ch);//按值查找结点,返回结点指针if(pp==NULL){//没有重复的字符串,插入到链表中s=(ListNode*)malloc(sizeof(ListNode));strcpy(s->data,ch);r->next=s;r=s; r->next=NULL;}printf(“Input#toend“);printf(“PleaseinputNode_data:“);scanf(“%s“,ch);}returnhead;//返回头指针}//==========用头插入法建立带头结点的单链表===========LinkListCreatList(void){charch[100];LinkListhead,p;head =(LinkList)malloc(sizeof(ListNode));head->next=NULL;while(1){printf(“Input#toend“);printf(“PleaseinputNode_data:“);scanf(“%s“,ch);if(strcmp (ch,“#“)){if(LocateNode(head,ch)==NULL){strcpy(head->data,ch);p=(Li nkList)malloc(sizeof(ListNode));p->next=head;head=p;}}elsebreak;}retu rnhead;}//==========按值查找结点,找到则返回该结点的位置,否则返回NULL==========ListNode*LocateNode(LinkListhead,char*key){List Node*p=head->next;//从开始结点比较while(p!=NULL//扫描下一个结点returnp;//若p=NULL则查找失败,否则p指向找到的值为key的结点}//==========修改程序:增加节点=======ListNode*AddNode(LinkListhead){charch[10];ListNode*s,*pp ;printf(“\nPleaseinputaNewNode_data:“);scanf(“%s“,ch);//输入各结点的字符串pp=LocateNode(head,ch);//按值查找结点,返回结点指针printf(“ok2\n“);if(pp==NULL){//没有重复的字符串,插入到链表中s=(ListNode*)malloc(sizeof(ListNode));strcpy(s->data,ch);printf(“ok3\n“);s->next=head->next;head->next=s;}returnhead;}//==========删除带头结点的单链表中的指定结点=======voidDeleteList(LinkListhead,char*key){ListNode*p,*r,*q=hea d;p=LocateNode(head,key);//按key值查找结点的if(p==NULL){//若没有找到结点,退出printf(“positionerror”);exit(0);}while(q->next!=p)//p 为要删除的结点,q为p的前结点q=q->next;r=q->next;q->next=r->next;free(r);//释放结点}//===========打印链表=======voidprintlist(LinkListhead){ListNode*p=head->next;//从开始结点打印while(p){printf(“%s,“,p->data);p=p->next;}printf(“\n“);}//==========删除所有结点,释放空间===========voidDeleteAll(LinkListhead){ListNode*p=head,*r;while( p->next){r=p->next;free(p);p=r;}free(p);}实验结果:Input#toendPleaseinputNode_data:batInput#toendPleaseinputNode_data: catInput#toendPleaseinputNode_data:eatInput#toendPleaseinputNode_da ta:fatInput#toendPleaseinputNode_data:hatInput#toendPleaseinputNode_ data:jatInput#toendPleaseinputNode_data:latInput#toendPleaseinputNode _data:matInput#toendPleaseinputNode_data:#mat,lat,jat,hat,fat,eat,cat,bat ,Deletenode(y/n):yPleaseinputDelete_data:hatmat,lat,jat,fat,eat,cat,bat,Ins ertnode(y/n):yPleaseinputInsert_data:putposition:5mat,lat,jat,fat,eat,put,c at,bat,请按任意键继续...示意图:latjathatfateatcatbatmatNULLheadlatjathatfateatcatbatmatheadlatjatfateat putcatbatmatheadNULLNULL心得体会:本次实验使我们对链表的实质了解更加明确了,对链表的一些基本操作也更加熟练了。
国家开放大学《数据结构》课程实验报告(实验3 ——栈、队列、递归设计)参考答案

x=Pop(s); /*出栈*/
printf("%d ",x);
InQueue(sq,x); /*入队*/
}
printf("\n");
printf("(10)栈为%s,",(StackEmpty(s)?"空":"非空"));
printf("队列为%s\n",(QueueEmpty(sq)?"空":"非空"));
ElemType Pop(SeqStack *s); /*出栈*/
ElemType GetTop(SeqStack *s); /*取栈顶元素*/
void DispStack(SeqStack *s); /*依次输出从栈顶到栈底的元素*/
void DispBottom(SeqStack *s); /*输出栈底元素*/
} SeqQueue; /*定义顺序队列*/
void InitStack(SeqStack *s); /*初始化栈*/
int StackEmpty(SeqStack *s); /*判栈空*/
int StackFull(SeqStack *s); /*判栈满*/
void Push(SeqStack *s,ElemType x); /*进栈*/
sq=(SeqQueue *)malloc(sizeof(SeqQueue));
InitQueue(sq);
printf("(8)队列为%s\n",(QueueEmpty(sq)?"空":"非空"));
printf("(9)出栈/入队的元素依次为:");
数据结构(第4版)习题及实验参考答案数据结构复习资料完整版(c语言版)
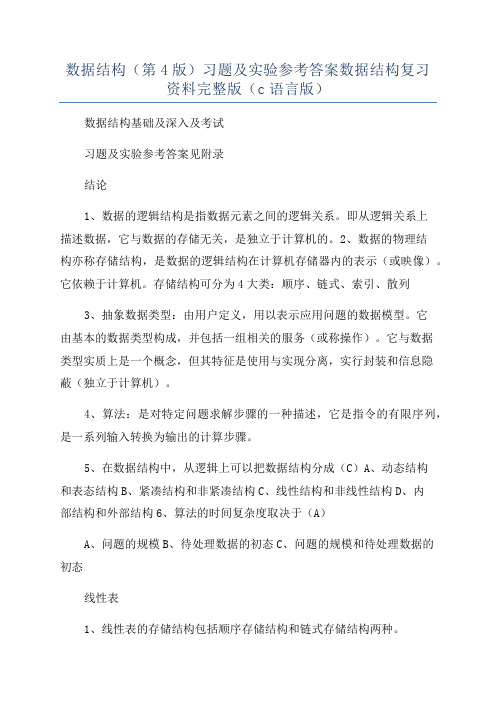
数据结构(第4版)习题及实验参考答案数据结构复习资料完整版(c语言版)数据结构基础及深入及考试习题及实验参考答案见附录结论1、数据的逻辑结构是指数据元素之间的逻辑关系。
即从逻辑关系上描述数据,它与数据的存储无关,是独立于计算机的。
2、数据的物理结构亦称存储结构,是数据的逻辑结构在计算机存储器内的表示(或映像)。
它依赖于计算机。
存储结构可分为4大类:顺序、链式、索引、散列3、抽象数据类型:由用户定义,用以表示应用问题的数据模型。
它由基本的数据类型构成,并包括一组相关的服务(或称操作)。
它与数据类型实质上是一个概念,但其特征是使用与实现分离,实行封装和信息隐蔽(独立于计算机)。
4、算法:是对特定问题求解步骤的一种描述,它是指令的有限序列,是一系列输入转换为输出的计算步骤。
5、在数据结构中,从逻辑上可以把数据结构分成(C)A、动态结构和表态结构B、紧凑结构和非紧凑结构C、线性结构和非线性结构D、内部结构和外部结构6、算法的时间复杂度取决于(A)A、问题的规模B、待处理数据的初态C、问题的规模和待处理数据的初态线性表1、线性表的存储结构包括顺序存储结构和链式存储结构两种。
2、表长为n的顺序存储的线性表,当在任何位置上插入或删除一个元素的概率相等时,插入一个元素所需移动元素的平均次数为(E),删除一个元素需要移动的元素的个数为(A)。
A、(n-1)/2B、nC、n+1D、n-1E、n/2F、(n+1)/2G、(n-2)/23、“线性表的逻辑顺序与存储顺序总是一致的。
”这个结论是(B)A、正确的B、错误的C、不一定,与具体的结构有关4、线性表采用链式存储结构时,要求内存中可用存储单元的地址(D)A、必须是连续的B、部分地址必须是连续的C一定是不连续的D连续或不连续都可以5、带头结点的单链表为空的判定条件是(B)A、head==NULLB、head->ne某t==NULLC、head->ne某t=headD、head!=NULL6、不带头结点的单链表head为空的判定条件是(A)A、head==NULLB、head->ne某t==NULLC、head->ne某t=headD、head!=NULL7、非空的循环单链表head的尾结点P满足(C)A、p->ne某t==NULLB、p==NULLC、p->ne某t==headD、p==head8、在一个具有n个结点的有序单链表中插入一个新结点并仍然有序的时间复杂度是(B)A、O(1)B、O(n)C、O(n2)D、O(nlog2n)数据结构(第4版)习题及实验参考答案9、在一个单链表中,若删除p所指结点的后继结点,则执行(A)A、p->ne某t=p->ne某t->ne某t;B、p=p->ne某t;p->ne某t=p->ne某t->ne某t;C、p->ne某t=p->ne某t;D、p=p->ne某t->ne某t;10、在一个单链表中,若在p所指结点之后插入所指结点,则执行(B)A、->ne某t=p;p->ne某t=;B、->ne某t=p->ne某t;p->ne某t=;C、->ne某t=p->ne某t;p=;D、p->ne某t=;->ne某t=p;11、在一个单链表中,已知q是p的前趋结点,若在q和p之间插入结点,则执行(C)A、->ne某t=p->ne某t;p->ne某t=;B、p->ne某t=->ne某t;->ne某t=p;C、q->ne某t=;->ne某t=p;D、p->ne某t=;->ne某t=q;12、在线性结构中,第一个结点没有前趋结点,其余每个结点有且只有1个前趋结点。
数据结构实验题参考答案
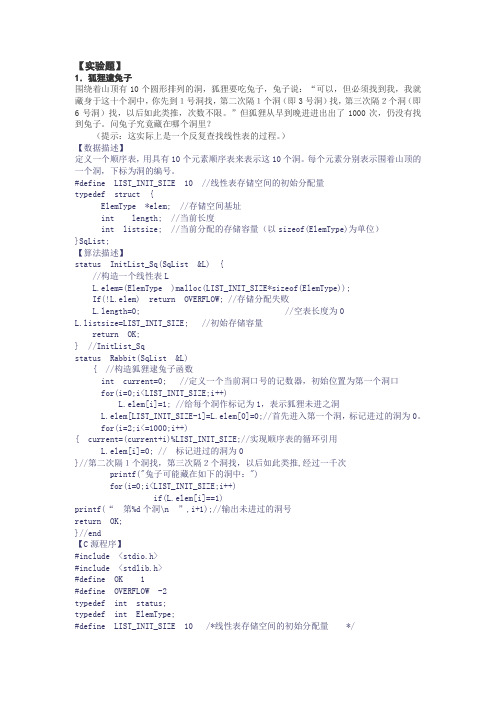
【实验题】1.狐狸逮兔子围绕着山顶有10个圆形排列的洞,狐狸要吃兔子,兔子说:“可以,但必须找到我,我就藏身于这十个洞中,你先到1号洞找,第二次隔1个洞(即3号洞)找,第三次隔2个洞(即6号洞)找,以后如此类推,次数不限。
”但狐狸从早到晚进进出出了1000次,仍没有找到兔子。
问兔子究竟藏在哪个洞里?(提示:这实际上是一个反复查找线性表的过程。
)【数据描述】定义一个顺序表,用具有10个元素顺序表来表示这10个洞。
每个元素分别表示围着山顶的一个洞,下标为洞的编号。
#define LIST_INIT_SIZE 10 //线性表存储空间的初始分配量typedef struct {ElemType *elem; //存储空间基址int length; //当前长度int listsize; //当前分配的存储容量(以sizeof(ElemType)为单位)}SqList;【算法描述】status InitList_Sq(SqList &L) {//构造一个线性表LL.elem=(ElemType )malloc(LIST_INIT_SIZE*sizeof(ElemType));If(!L.elem) return OVERFLOW; //存储分配失败L.length=0; //空表长度为0L.listsize=LIST_INIT_SIZE; //初始存储容量return OK;} //InitList_Sqstatus Rabbit(SqList &L){ //构造狐狸逮兔子函数int current=0; //定义一个当前洞口号的记数器,初始位置为第一个洞口for(i=0;i<LIST_INIT_SIZE;i++)L.elem[i]=1; //给每个洞作标记为1,表示狐狸未进之洞L.elem[LIST_INIT_SIZE-1]=L.elem[0]=0;//首先进入第一个洞,标记进过的洞为0。
数据结构(c语言版)课后习题答案完整版
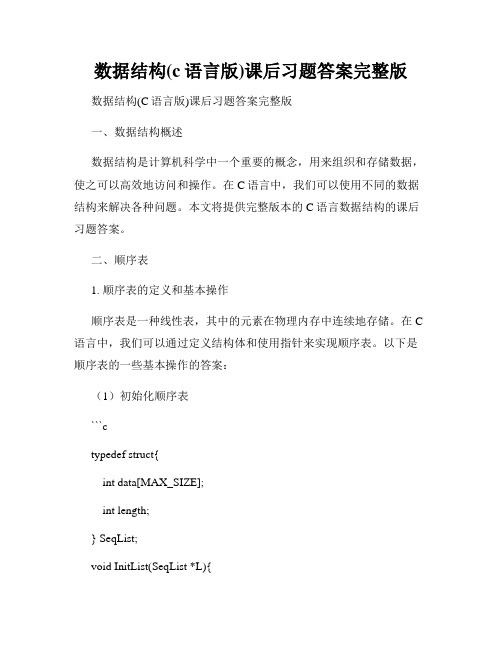
数据结构(c语言版)课后习题答案完整版数据结构(C语言版)课后习题答案完整版一、数据结构概述数据结构是计算机科学中一个重要的概念,用来组织和存储数据,使之可以高效地访问和操作。
在C语言中,我们可以使用不同的数据结构来解决各种问题。
本文将提供完整版本的C语言数据结构的课后习题答案。
二、顺序表1. 顺序表的定义和基本操作顺序表是一种线性表,其中的元素在物理内存中连续地存储。
在C 语言中,我们可以通过定义结构体和使用指针来实现顺序表。
以下是顺序表的一些基本操作的答案:(1)初始化顺序表```ctypedef struct{int data[MAX_SIZE];int length;} SeqList;void InitList(SeqList *L){L->length = 0;}```(2)插入元素到顺序表中```cbool Insert(SeqList *L, int pos, int elem){if(L->length == MAX_SIZE){return false; // 顺序表已满}if(pos < 1 || pos > L->length + 1){return false; // 位置不合法}for(int i = L->length; i >= pos; i--){L->data[i] = L->data[i-1]; // 向后移动元素 }L->data[pos-1] = elem;L->length++;return true;}```(3)删除顺序表中的元素```cbool Delete(SeqList *L, int pos){if(pos < 1 || pos > L->length){return false; // 位置不合法}for(int i = pos; i < L->length; i++){L->data[i-1] = L->data[i]; // 向前移动元素 }L->length--;return true;}```(4)查找顺序表中的元素```cint Search(SeqList L, int elem){for(int i = 0; i < L.length; i++){if(L.data[i] == elem){return i + 1; // 找到元素,返回位置 }}return -1; // 未找到元素}```2. 顺序表习题解答(1)逆置顺序表```cvoid Reverse(SeqList *L){for(int i = 0; i < L->length / 2; i++){int temp = L->data[i];L->data[i] = L->data[L->length - 1 - i]; L->data[L->length - 1 - i] = temp;}}```(2)顺序表元素去重```cvoid RemoveDuplicates(SeqList *L){for(int i = 0; i < L->length; i++){for(int j = i + 1; j < L->length; j++){if(L->data[i] == L->data[j]){Delete(L, j + 1);j--;}}}}```三、链表1. 单链表单链表是一种常见的链式存储结构,每个节点包含数据和指向下一个节点的指针。
数据结构(含答案)
数据结构(含答案)数据结构数据结构是计算机科学的基础知识之一,它在计算机领域中有着重要的地位。
本文将介绍数据结构的概念、常见的数据结构类型以及其应用。
同时,还会对一些常见的数据结构问题进行解答。
一、概念简介在计算机科学中,数据结构是指存储和组织数据的方式。
它关注数据元素之间的关系,以及如何对数据进行插入、删除和查询等操作。
数据结构可以分为线性结构和非线性结构两大类。
1.1 线性结构线性结构是最简单的一种数据结构,它的特点是数据元素之间存在一对一的关系。
常见的线性结构包括数组、链表、栈和队列。
- 数组是一种连续存储数据元素的结构,可以通过下标快速访问元素。
但是数组的大小固定,插入和删除操作比较耗时。
- 链表是一种通过指针连接数据元素的结构,可以动态地进行插入和删除操作。
但是链表的随机访问效率较低。
- 栈是一种先进后出(LIFO)的数据结构,只能在栈顶进行插入和删除操作。
常见的应用场景包括函数调用、表达式求值等。
- 队列是一种先进先出(FIFO)的数据结构,插入操作在队尾进行,删除操作在队头进行。
常见的应用场景包括任务调度、消息传递等。
1.2 非线性结构非线性结构中,数据元素之间的关系不是一对一的,包括树和图等结构。
- 树是一种层次结构,由节点和边组成。
树的常见应用包括文件系统、数据库索引等。
- 图是由节点和边组成的网络结构,节点之间的关系可以是任意的。
图的应用非常广泛,包括社交网络、路由算法等。
二、数据结构问题解答2.1 如何判断一个链表中是否存在环?使用快慢指针可以判断一个链表中是否存在环。
假设有两个指针,一个每次移动一步,另一个每次移动两步。
如果链表中存在环,那么快指针迟早会追上慢指针。
如果快指针到达链表尾部时都没有追上慢指针,那么链表中不存在环。
2.2 如何判断一个二叉树是否是平衡二叉树?平衡二叉树是一种左子树和右子树高度差不超过1的二叉树。
判断一个二叉树是否是平衡二叉树可以使用递归的方法。
数据结构实验报告 答案
数据结构实验报告答案一、实验目的本次数据结构实验的主要目的是通过实际编程和操作,深入理解和掌握常见的数据结构,如数组、链表、栈、队列、树和图等,并能够运用这些数据结构解决实际问题,提高编程能力和算法设计能力。
二、实验环境操作系统:Windows 10编程语言:C++开发工具:Visual Studio 2019三、实验内容1、数组操作定义一个整数数组,实现数组元素的输入、输出和查找功能。
对数组进行排序(选择排序、冒泡排序等),并输出排序后的数组。
2、链表操作构建一个单向链表,实现链表节点的插入、删除和遍历操作。
反转链表,并输出反转后的链表。
3、栈和队列操作用数组实现栈和队列的数据结构,实现入栈、出栈、入队、出队等基本操作。
利用栈实现表达式求值(中缀表达式转后缀表达式,然后计算后缀表达式的值)。
4、树的操作构建二叉树(可以采用顺序存储或链式存储),实现二叉树的前序、中序和后序遍历。
实现二叉树的查找、插入和删除节点操作。
5、图的操作用邻接矩阵或邻接表表示图,实现图的深度优先遍历和广度优先遍历。
求解图的最短路径(Dijkstra 算法或 Floyd 算法)。
四、实验步骤及代码实现1、数组操作```cppinclude <iostream>using namespace std;//数组输入函数void inputArray(int arr, int size) {cout <<"请输入"<< size <<"个整数:"<< endl; for (int i = 0; i < size; i++){cin >> arri;}}//数组输出函数void outputArray(int arr, int size) {cout <<"数组元素为:"<< endl;for (int i = 0; i < size; i++){cout << arri <<"";}cout << endl;}//数组查找函数int searchArray(int arr, int size, int target) {for (int i = 0; i < size; i++){if (arri == target) {return i;}}return -1;}//选择排序函数void selectionSort(int arr, int size) {for (int i = 0; i < size 1; i++){int minIndex = i;for (int j = i + 1; j < size; j++){if (arrj < arrminIndex) {minIndex = j;}}if (minIndex!= i) {int temp = arri;arri = arrminIndex;arrminIndex = temp;}}}//冒泡排序函数void bubbleSort(int arr, int size) {for (int i = 0; i < size 1; i++){for (int j = 0; j < size i 1; j++){if (arrj > arrj + 1) {int temp = arrj;arrj = arrj + 1;arrj + 1 = temp;}}}}int main(){int size = 10;inputArray(arr, size);outputArray(arr, size);int target = 5;int result = searchArray(arr, size, target);if (result!=-1) {cout <<"找到目标元素"<< target <<",在数组中的索引为"<< result << endl;} else {cout <<"未找到目标元素"<< target << endl;}selectionSort(arr, size);outputArray(arr, size);bubbleSort(arr, size);outputArray(arr, size);return 0;}2、链表操作```cppinclude <iostream>using namespace std;//链表节点结构体struct ListNode {int data;ListNode next;ListNode(int x) : data(x), next(NULL) {}};//链表插入函数void insertNode(ListNode& head, int val) {ListNode newNode = new ListNode(val);if (head == NULL) {head = newNode;return;}ListNode curr = head;while (curr>next!= NULL) {curr = curr>next;}curr>next = newNode;}//链表删除函数void deleteNode(ListNode& head, int val) {if (head == NULL) {return;}if (head>data == val) {ListNode temp = head;head = head>next;delete temp;return;}ListNode curr = head;while (curr>next!= NULL && curr>next>data!= val) {curr = curr>next;}if (curr>next!= NULL) {ListNode temp = curr>next;curr>next = curr>next>next;delete temp;}}//链表遍历函数void traverseList(ListNode head) {ListNode curr = head;while (curr!= NULL) {cout << curr>data <<"";curr = curr>next;}cout << endl;}//链表反转函数ListNode reverseList(ListNode head) {ListNode prev = NULL;ListNode curr = head;while (curr!= NULL) {ListNode nextTemp = curr>next; curr>next = prev;prev = curr;curr = nextTemp;}return prev;}int main(){ListNode head = NULL;insertNode(head, 1);insertNode(head, 2);insertNode(head, 3);insertNode(head, 4);insertNode(head, 5);traverseList(head);deleteNode(head, 3);traverseList(head);ListNode reversedHead = reverseList(head);traverseList(reversedHead);return 0;}```3、栈和队列操作```cppinclude <iostream>using namespace std;//用数组实现栈const int MAX_SIZE = 100;class Stack {private:int arrMAX_SIZE;int top;public:Stack(){top =-1;}//入栈void push(int val) {if (top == MAX_SIZE 1) {cout <<"栈已满,无法入栈" << endl; return;}arr++top = val;}//出栈int pop(){if (top ==-1) {cout <<"栈为空,无法出栈" << endl; return -1;}int val = arrtop;top;return val;}//查看栈顶元素int peek(){if (top ==-1) {cout <<"栈为空" << endl;return -1;}return arrtop;}//判断栈是否为空bool isEmpty(){return top ==-1;}};//用数组实现队列class Queue {private:int arrMAX_SIZE;int front, rear;public:Queue(){front = rear =-1;}//入队void enqueue(int val) {if ((rear + 1) % MAX_SIZE == front) {cout <<"队列已满,无法入队" << endl; return;}if (front ==-1) {front = 0;}rear =(rear + 1) % MAX_SIZE;arrrear = val;}//出队int dequeue(){if (front ==-1) {cout <<"队列为空,无法出队" << endl; return -1;}int val = arrfront;if (front == rear) {front = rear =-1;} else {front =(front + 1) % MAX_SIZE;}return val;}//查看队头元素int peek(){if (front ==-1) {cout <<"队列为空" << endl;return -1;}return arrfront;}//判断队列是否为空bool isEmpty(){return front ==-1;}};//表达式求值函数int evaluateExpression(string expression) {Stack operandStack;Stack operatorStack;for (int i = 0; i < expressionlength(); i++){char c = expressioni;if (isdigit(c)){int operand = 0;while (i < expressionlength()&& isdigit(expressioni)){operand = operand 10 +(expressioni++'0');}i;operandStackpush(operand);} else if (c =='+'|| c ==''|| c ==''|| c =='/'){while (!operatorStackisEmpty()&&precedence(operatorStackpeek())>= precedence(c)){int operand2 = operandStackpop();int operand1 = operandStackpop();char op = operatorStackpop();int result = performOperation(operand1, operand2, op);operandStackpush(result);}operatorStackpush(c);} else if (c =='('){operatorStackpush(c);} else if (c ==')'){while (operatorStackpeek()!='('){int operand2 = operandStackpop();int operand1 = operandStackpop();char op = operatorStackpop();int result = performOperation(operand1, operand2, op);operandStackpush(result);}operatorStackpop();}}while (!operatorStackisEmpty()){int operand2 = operandStackpop();int operand1 = operandStackpop();char op = operatorStackpop();int result = performOperation(operand1, operand2, op);operandStackpush(result);}return operandStackpop();}//运算符优先级函数int precedence(char op) {if (op =='+'|| op ==''){return 1;} else if (op ==''|| op =='/'){return 2;}return 0;}//运算函数int performOperation(int operand1, int operand2, char op) {switch (op) {case '+':return operand1 + operand2;case '':return operand1 operand2;case '':return operand1 operand2;case '/':if (operand2!= 0) {return operand1 / operand2;} else {cout <<"除数不能为 0" << endl;return -1;}}return -1;}int main(){Stack stack;stackpush(1);stackpush(2);stackpush(3);cout <<"栈顶元素:"<< stackpeek()<< endl;cout <<"出栈元素:"<< stackpop()<< endl;cout <<"栈是否为空:"<<(stackisEmpty()?"是" :"否")<< endl;Queue queue;queueenqueue(1);queueenqueue(2);queueenqueue(3);cout <<"队头元素:"<< queuepeek()<< endl;cout <<"出队元素:"<< queuedequeue()<< endl;cout <<"队列是否为空:"<<(queueisEmpty()?"是" :"否")<< endl;string expression ="2+34";int result = evaluateExpression(expression);cout << expression <<"="<< result << endl; return 0;}```4、树的操作```cppinclude <iostream>using namespace std;//二叉树节点结构体struct TreeNode {int val;TreeNode left;TreeNode right;TreeNode(int x) : val(x), left(NULL), right(NULL) {}};//前序遍历函数void preOrderTraversal(TreeNode root) {return;}cout << root>val <<"";preOrderTraversal(root>left);preOrderTraversal(root>right);}//中序遍历函数void inOrderTraversal(TreeNode root) {if (root == NULL) {return;}inOrderTraversal(root>left);cout << root>val <<"";inOrderTraversal(root>right);}//后序遍历函数void postOrderTraversal(TreeNode root) {return;}postOrderTraversal(root>left);postOrderTraversal(root>right);cout << root>val <<"";}//查找函数TreeNode searchBST(TreeNode root, int val) {if (root == NULL || root>val == val) {return root;}if (val < root>val) {return searchBST(root>left, val);} else {return searchBST(root>right, val);}}//插入函数TreeNode insertBST(TreeNode root, int val) {if (root == NULL) {return new TreeNode(val);}if (val < root>val) {root>left = insertBST(root>left, val);} else if (val > root>val) {root>right = insertBST(root>right, val);}return root;}//删除函数TreeNode deleteNodeBST(TreeNode root, int key) {if (root == NULL) {return root;}if (key < root>val) {root>left = deleteNodeBST(root>left, key);} else if (key > root>val) {root>right = deleteNodeBST(root>right, key);} else {if (root>left == NULL) {TreeNode temp = root>right;delete root;return temp;} else if (root>right == NULL) {TreeNode temp = root>left;delete root;return temp;}TreeNode minNode = root>right;while (minNode>left!= NULL) {minNode = minNode>left;}root>val = minNode>val;root>right = deleteNodeBST(root>right, minNode>val);}return root;}int main(){TreeNode root = new TreeNode(4);root>left = new TreeNode(2);root>right = new TreeNode(6);root>left>left = new TreeNode(1);root>left>right = new TreeNode(3);root>right>left = new TreeNode(5);root>right>right = new TreeNode(7);cout <<"前序遍历:"<< endl; preOrderTraversal(root);cout << endl;cout <<"中序遍历:"<< endl; inOrderTraversal(root);cout << endl;cout <<"后序遍历:"<< endl; postOrderTraversal(root);cout << endl;int target = 3;TreeNode foundNode = searchBST(root, target);if (foundNode!= NULL) {cout <<"找到目标节点"<< target << endl;} else {cout <<"未找到目标节点"<< target << endl;}root = insertBST(root, 8);cout <<"插入节点 8 后的中序遍历:"<< endl; inOrderTraversal(root);cout << endl;root = deleteNodeBST(root, 2);cout <<"删除节点 2 后的中序遍历:。
(完整版)数据结构实验答案及解析
《数据结构》实验指导2013 / 2014 学年第 2学期姓名:______________学号:_________班级:______________指导教师:______________潍坊学院计算机工程学院2014预备实验 C语言的函数数组指针结构体知识一、实验目的1、复习C语言中函数、数组、指针和结构体的概念。
2、熟悉利用C语言进行程序设计的一般方法。
二、实验内容和要求1、调试程序:输出100以内所有的素数(用函数实现)。
#include<stdio.h>/*判断一个数是否为素数*/int isprime(int n){for(int m=2;m*m<=n;m++){if(n%m= =0) return 0;return 1;}/*输出100以内所有素数*/int main(){int i;for(i=2;i<100;i++)if(isprime(i)= =1) printf(“%4d”,i);return 0;}运行结果:2、调试程序:对一维数组中的元素进行逆序排列。
#include<stdio.h>#define N 10int main(){int a[N]={0,1,2,3,4,5,6,7,8,9},i,temp;printf(“the original Array is:\n ”);for(i=0;i<N;i++)printf(“%4d”,a[i]);for(i=0;i<N/2;i++){ /*交换数组元素使之逆序*/temp=a[i];a[i]=a[N-i-1];a[N-i-1]=temp;}printf(“\nthe changed Array is:\n”);for(i=0;i<N;i++)printf(“%4d”,a[i]);return 0;}运行结果:3、调试程序:在二维数组中,若某一位置上的元素在该行中最大,而在该列中最小,则该元素即为该二维数组的一个鞍点。
数据结构实验答案1
数据结构实验答案1重庆文理学院软件工程学院实验报告册专业:_____软件工程__ _班级:_____软件工程2班__ _学号:_____201258014054 ___姓名:_____周贵宇___________课程名称:___ 数据结构 _指导教师:_____胡章平__________2013年 06 月 25 日实验序号 1 实验名称实验一线性表基本操作实验地点S-C1303 实验日期2013年04月22日实验内容1.编程实现在顺序存储的有序表中插入一个元素(数据类型为整型)。
2.编程实现把顺序表中从i个元素开始的k个元素删除(数据类型为整型)。
3.编程序实现将单链表的数据逆置,即将原表的数据(a1,a2….an)变成(an,…..a2,a1)。
(单链表的数据域数据类型为一结构体,包括学生的部分信息:学号,姓名,年龄)实验过程及步骤1.#include#include#include#define OK 1#define ERROR 0#define TRUE 1#define FALSE 0#define ElemType int#define MAXSIZE 100 /*此处的宏定义常量表示线性表可能达到的最大长度*/typedef struct{ ElemType elem[MAXSIZE]; /*线性表占用的数组空间*/ int last; /*记录线性表中最后一个元素在数组elem[ ]中的位置(下标值),空表置为-1*/}SeqList;#include "common.h"#include "seqlist.h"void px(SeqList *A,int j);void main(){ SeqList *l;int p,q,r;int i;l=(SeqList*)malloc(sizeof(SeqList));printf("请输入线性表的长度:");scanf("%d",&r);l->last = r-1;printf("请输入线性表的各元素值:\n");for(i=0; i<=l->last; i++){scanf("%d",&l->elem[i]);}px(l,i);printf("请输入要插入的值:\n");scanf("%d",&l->elem[i]);i++;px(l,i);l->last++;for(i=0; i<=l->last; i++){ printf("%d ",l->elem[i]);}printf("\n");}void px(SeqList *A,int j){ int i,temp,k;for(i=0;i<j;i++)< p="">{ for(k=0;k<j-1;k++)< p="">{if(A->elem[i]elem[k]){temp=A->elem[i];A->elem[i]=A->elem[k];A->elem[k]=temp;}}}}2.#include#include#include#define OK 1#define ERROR 0#define TRUE 1#define FALSE 0#define ElemType int#define MAXSIZE 100 /*此处的宏定义常量表示线性表可能达到的最大长度*/typedef struct{ ElemType elem[MAXSIZE]; /*线性表占用的数组空间*/ int last; /*记录线性表中最后一个元素在数组elem[ ]中的位置(下标值),空表置为-1*/}SeqList;#include "common.h"#include "seqlist.h"void px(SeqList *A,int j);int DelList(SeqList *L,int i,SeqList *e,int j)/*在顺序表L中删除第i个数据元素,并用指针参数e返回其值。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
《数据结构》实验指导2013 / 2014 学年第 2学期姓名:______________学号:_________班级:______________指导教师:______________潍坊学院计算机工程学院2014预备实验 C语言的函数数组指针结构体知识一、实验目的1、复习C语言中函数、数组、指针和结构体的概念。
2、熟悉利用C语言进行程序设计的一般方法。
二、实验内容和要求1、调试程序:输出100以内所有的素数(用函数实现)。
#include<stdio.h>/*判断一个数是否为素数*/int isprime(int n){for(int m=2;m*m<=n;m++){if(n%m= =0) return 0;return 1;}/*输出100以内所有素数*/int main(){int i;for(i=2;i<100;i++)if(isprime(i)= =1) printf(“%4d”,i);return 0;}运行结果:2、调试程序:对一维数组中的元素进行逆序排列。
#include<stdio.h>#define N 10int main(){int a[N]={0,1,2,3,4,5,6,7,8,9},i,temp;printf(“the original Array is:\n ”);for(i=0;i<N;i++)printf(“%4d”,a[i]);for(i=0;i<N/2;i++){ /*交换数组元素使之逆序*/temp=a[i];a[i]=a[N-i-1];a[N-i-1]=temp;}printf(“\nthe changed Array is:\n”);for(i=0;i<N;i++)printf(“%4d”,a[i]);return 0;}运行结果:3、调试程序:在二维数组中,若某一位置上的元素在该行中最大,而在该列中最小,则该元素即为该二维数组的一个鞍点。
要求从键盘上输入一个二维数组,当鞍点存在时,把鞍点找出来。
#include<stdio.h>#define M 3#define N 4int main(){int a[M][N],i,j,k;printf(“请输入二维数组的数据:\n”);for(i=0;i<M;i++)for(j=0;j<N;j++)scanf(“%d”,&a[i][j]);for(i=0;i<M;i++){ /*输出矩阵*/for(j=0;j<N;j++)printf(“%4d”,a[i][j]);printf(“\n”);}for(i=0;i<M;i++){k=0;for(j=1;j<N;j++) /*找出第i行的最大值*/if(a[i][j]>a[i][k])k=j;for(j=0;j<M;j++) /*判断第i行的最大值是否为该列的最小值*/if(a[j][k]<a[i][k])break;if(j==M) /*在第i行找到鞍点*/printf(“%d,%d,%d\n”),a[i][k],i,k);}return 0;}运行结果:4、调试程序:利用指针输出二维数组的元素。
#include<stdio.h>int main(){int a[3][4]={1,3,5,7,9,11,13,15,17,19,21,23};int *p;for(p=a[0];p<a[0]+12;p++){if((p-a[0])%4= =0) printf(“\n”);printf(%4d”,*p);}return 0;}运行结果:5、调试程序:输入10个学生的成绩,每个学生成绩包括学号、姓名和三门课的成绩。
要求打印出三门课的平均成绩及成绩最高者的姓名和成绩。
#include<stdio.h>#define N 10;struct student{char num[6]; /*学号*/char name[8]; /*姓名*/int score[3]; /*成绩*/float avr; /*平均成绩*/}stu[N];int main(){int i,j,max,maxi,sum;float average;for(i=0;i<N;i++){ /*输入10个学生的成绩信息*/ printf(“\n请输入第%d学生的成绩:\n”,i+1);printf(“学号:”);scanf(“%s”,stu[i].num);printf(“姓名”);scanf(“%s”,stu[i].name);for(j=0;j<3;j++){printf(“成绩%d”,j+1);scanf(“%d”,&stu[i].score[j]);}}average=0;max=0;maxi=0;for(i=0;i<N;i++){ /*计算平均成绩,找出成绩最高的学生*/ sum=0;for(j=0;j<3;j++)sum+=stu[i].score[j];stu[i].avr=sum/3.0;average+=stu[i].avr;if(sum>max){max=sum;maxi=i;}}average/=10;printf(“学号姓名成绩1 成绩2 成绩3 平均分\n);for(i=0;i<10;i++){printf(“%8s%10s”,stu[i].num,stu[i].name);for(j=0;j<3;j++)printf(“%7d”,stu[i].score[j]);printf(“%6.2f\n”,stu[i].avr);}printf(“平均成绩是:%5.2f\n”,average);printf(“最好成绩的学生是:%s,总分是%d”,stu[maxi].name,max);return 0;}运行结果三、实验小结对C语言中函数、数组、指针和结构体的概念,有了进一步的加深。
并且可以利用C语言进行初步程序设计。
四、教师评语实验一顺序表与链表一、实验目的1、掌握线性表中元素的前驱、后续的概念。
2、掌握顺序表与链表的建立、插入元素、删除表中某元素的算法。
3、对线性表相应算法的时间复杂度进行分析。
4、理解顺序表、链表数据结构的特点(优缺点)。
二、实验内容和要求1、阅读下面程序,在横线处填写函数的基本功能。
并运行程序,写出结果。
#include<stdio.h>#include<malloc.h>#define ERROR 0#define OK 1#define INIT_SIZE 5 /*初始分配的顺序表长度*/#define INCREM 5 /*溢出时,顺序表长度的增量*/typedef int ElemType; /*定义表元素的类型*/typedef struct Sqlist{ElemType *slist; /*存储空间的基地址*/int length; /*顺序表的当前长度*/int listsize; /*当前分配的存储空间*/}Sqlist;int InitList_sq(Sqlist *L); /*初始化顺序表L,并将其长度设为0 */int CreateList_sq(Sqlist *L,int n); /*构造顺序表的长度为n */ int ListInsert_sq(Sqlist *L,int i,ElemType e);/*在顺序线性表L中第i个元素之前插入新的元素e */int PrintList_sq(Sqlist *L); /*输出顺序表的元素*/int ListDelete_sq(Sqlist *L,int i); /*删除第i个元素*/int ListLocate(Sqlist *L,ElemType e); /*查找值为e的元素*/int InitList_sq(Sqlist *L){L->slist=(ElemType*)malloc(INIT_SIZE*sizeof(ElemType));if(!L->slist) return ERROR;L->length=0;L->listsize=INIT_SIZE;return OK;}/*InitList*/int CreateList_sq(Sqlist *L,int n){ElemType e;int i;for(i=0;i<n;i++){printf("input data %d",i+1);scanf("%d",&e);if(!ListInsert_sq(L,i+1,e))return ERROR;}return OK;}/*CreateList*//*输出顺序表中的元素*/int PrintList_sq(Sqlist *L){int i;for(i=1;i<=L->length;i++)printf("%5d",L->slist[i-1]);return OK;}/*PrintList*/int ListInsert_sq(Sqlist *L,int i,ElemType e){int k;if(i<1||i>L->length+1)return ERROR;if(L->length>=L->listsize){L->slist=(ElemType*)realloc(L->slist,(INIT_SIZE+INCREM)*sizeof(ElemType)); if(!L->slist)return ERROR;L->listsize+=INCREM;}for(k=L->length-1;k>=i-1;k--){L->slist[k+1]=k;}L->slist[i-1]=e;L->length++;return OK;}/*ListInsert*//*在顺序表中删除第i个元素*/int ListDelete_sq(Sqlist *L,int i){if((i<1)||(i>L->length)) return ERROR;for(p=i-1;p<->length-1;p++)L->slist[p]=L->slist[p+1];L->length--;return OK;}/*在顺序表中查找指定值元素,返回其序号*/int ListLocate(Sqlist *L,ElemType e){}int main(){Sqlist sl;int n;printf("please input n:"); /*输入顺序表的元素个数*/ scanf("%d",&n);if(n>0){printf("\n1-Create Sqlist:\n");InitList_sq(&sl);CreateList_sq(&sl,n);printf("\n2-Print Sqlist:\n");PrintList_sq(&sl);}elseprintf("ERROR");return 0;}算法分析与运行结果please input n:51-Create Sqlist:input data 10input data 25input data 38input data 43input data 562-Print Sqlist:0 5 8 3 6Press any key to continue2、为第1题补充删除和查找功能函数,并在主函数中补充代码验证算法的正确性。