JAVA继承和多态实验报告
实验三 继承和多态
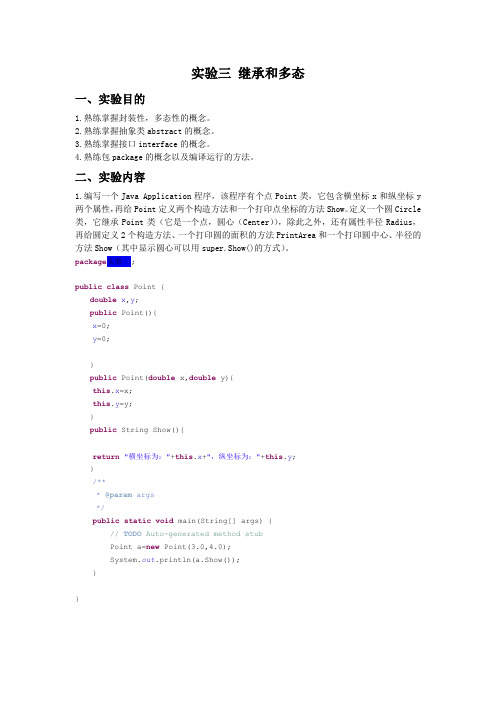
实验三继承和多态一、实验目的1.熟练掌握封装性,多态性的概念。
2.熟练掌握抽象类abstract的概念。
3.熟练掌握接口interface的概念。
4.熟练包package的概念以及编译运行的方法。
二、实验内容1.编写一个Java Application程序,该程序有个点Point类,它包含横坐标x和纵坐标y 两个属性,再给Point定义两个构造方法和一个打印点坐标的方法Show。
定义一个圆Circle 类,它继承Point类(它是一个点,圆心(Center)),除此之外,还有属性半径Radius,再给圆定义2个构造方法、一个打印圆的面积的方法PrintArea和一个打印圆中心、半径的方法Show(其中显示圆心可以用super.Show()的方式)。
package实验三;public class Point {double x,y;public Point(){x=0;y=0;}public Point(double x,double y){this.x=x;this.y=y;}public String Show(){return"横坐标为:"+this.x+",纵坐标为:"+this.y;}/*** @param args*/public static void main(String[] args) {// TODO Auto-generated method stubPoint a=new Point(3.0,4.0);System.out.println(a.Show());}}package实验三;public class Circle extends Point {double Radius;public Circle(){super(0,0);Radius=0;}public Circle(double x,double y,double z){super(x,y);Radius=z;}public String Show(){System.out.println("圆心的"+super.Show()+"半径为:"+Radius);return"圆心的"+super.Show()+"半径为:"+Radius;}public String PrintArea(){System.out.println("圆的面积为:"+Math.PI*Radius*Radius);return"圆的面积为:"+Math.PI*Radius*Radius;}/*** @param args*/public static void main(String[] args) {// TODO Auto-generated method stubCircle a=new Circle(3,4,5);a.Show();a.PrintArea();}}2.编写一测试类,对其进行编译、运行。
实验报告5 继承与多态
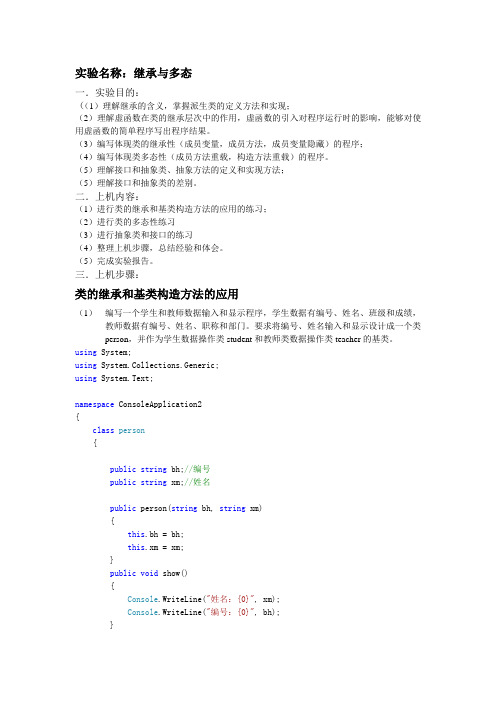
实验名称:继承与多态一.实验目的:((1)理解继承的含义,掌握派生类的定义方法和实现;(2)理解虚函数在类的继承层次中的作用,虚函数的引入对程序运行时的影响,能够对使用虚函数的简单程序写出程序结果。
(3)编写体现类的继承性(成员变量,成员方法,成员变量隐藏)的程序;(4)编写体现类多态性(成员方法重载,构造方法重载)的程序。
(5)理解接口和抽象类、抽象方法的定义和实现方法;(5)理解接口和抽象类的差别。
二.上机内容:(1)进行类的继承和基类构造方法的应用的练习;(2)进行类的多态性练习(3)进行抽象类和接口的练习(4)整理上机步骤,总结经验和体会。
(5)完成实验报告。
三.上机步骤:类的继承和基类构造方法的应用(1)编写一个学生和教师数据输入和显示程序,学生数据有编号、姓名、班级和成绩,教师数据有编号、姓名、职称和部门。
要求将编号、姓名输入和显示设计成一个类person,并作为学生数据操作类student和教师类数据操作类teacher的基类。
using System;using System.Collections.Generic;using System.Text;namespace ConsoleApplication2{class person{public string bh;//编号public string xm;//姓名public person(string bh, string xm){this.bh = bh;this.xm = xm;}public void show(){Console.WriteLine("姓名:{0}", xm);Console.WriteLine("编号:{0}", bh);}}class student : person{public string bj;//班级public int cj;//成绩public student(string sbh, string sxm, string sbj, int scj): base(sbh, sxm){bh = sbh;xm = sxm;bj = sbj;cj = scj;}public new void show(){Console.WriteLine("***student***");Console.WriteLine("姓名:{0}", xm);Console.WriteLine("编号:{0}", bh);Console.WriteLine("班级:{0}", bj);Console.WriteLine("成绩:{0}", cj);}}class teacher : person{public string zc;//职称public string bm;//部门public teacher(string tbh, string txm, string tzc, string tbm) : base(tbh, txm){bh = tbh;xm = txm;zc = tzc;bm = tbm;}public new void show(){Console.WriteLine("***teacher***");Console.WriteLine("姓名:{0}", xm);Console.WriteLine("编号:{0}", bh);Console.WriteLine("职称:{0}", zc);Console.WriteLine("部门:{0}", bm);}}class program{static void Main(string[] args){student st = new student("050013", "张三", "信管091", 91);st.show();teacher te = new teacher("046950", "范仲淹", "特级教师", "教务处"); te.show();Console.ReadLine();}}}运行结果:(2)将以上程序尝试改成通过调用基类构造方法的方式来初始化编号和姓名,并总结调用基类构造方法的应用要点。
Java实验报告继承、多态、接口和异常处理
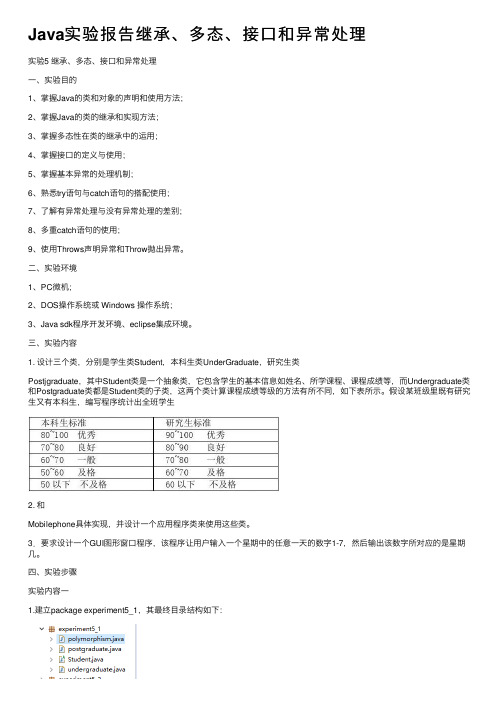
Java实验报告继承、多态、接⼝和异常处理实验5 继承、多态、接⼝和异常处理⼀、实验⽬的1、掌握Java的类和对象的声明和使⽤⽅法;2、掌握Java的类的继承和实现⽅法;3、掌握多态性在类的继承中的运⽤;4、掌握接⼝的定义与使⽤;5、掌握基本异常的处理机制;6、熟悉try语句与catch语句的搭配使⽤;7、了解有异常处理与没有异常处理的差别;8、多重catch语句的使⽤;9、使⽤Throws声明异常和Throw抛出异常。
⼆、实验环境1、PC微机;2、DOS操作系统或 Windows 操作系统;3、Java sdk程序开发环境、eclipse集成环境。
三、实验内容1. 设计三个类,分别是学⽣类Student,本科⽣类UnderGraduate,研究⽣类Postjgraduate,其中Student类是⼀个抽象类,它包含学⽣的基本信息如姓名、所学课程、课程成绩等,⽽Undergraduate类和Postgraduate类都是Student类的⼦类,这两个类计算课程成绩等级的⽅法有所不同,如下表所⽰。
假设某班级⾥既有研究⽣⼜有本科⽣,编写程序统计出全班学⽣2. 和Mobilephone具体实现,并设计⼀个应⽤程序类来使⽤这些类。
3.要求设计⼀个GUI图形窗⼝程序,该程序让⽤户输⼊⼀个星期中的任意⼀天的数字1-7,然后输出该数字所对应的是星期⼏。
四、实验步骤实验内容⼀1.建⽴package experiment5_1,其最终⽬录结构如下:2.建⽴Student类:package experiment5_1;public abstract class Student {final static int CourseNo = 3;String name;String type;int[] courses;String courseGrade;public Student(String name) {/doc/1bd4a299a66e58fafab069dc5022aaea998f41e2.html = name; courses = new int[CourseNo];courseGrade = "" ;}public abstract void calculateGrade();public String getName( ) {return name;}public String getType( ) {return type ;}public String getCourseGrade( ) {return courseGrade;}public int getCourseScore(int courseNumber) {return courses[courseNumber];}public void setName(String name) {/doc/1bd4a299a66e58fafab069dc5022aaea998f41e2.html = name;}public void setType(String type) {this.type = type;}public void setCourseScore(int courseNumber, int courseScore) { //按课程索引号设置课程成绩this.courses[courseNumber] = courseScore ;}}3.建⽴外部类(1)研究⽣类Postjgraduatepackage experiment5_1;public class postgraduate extends Student {public postgraduate(String name) {super(name);type = "研究⽣";}public void calculateGrade() {// TODO Auto-generated method stubint total = 0;double average = 0;for (int i = 0; i < CourseNo; i++) {total += courses[i];};average = total / CourseNo;if (average>=90&&average<100) courseGrade = "优秀"; else if (average>=80&&average<90) courseGrade = "良好"; else if (average>=70&&average<80) courseGrade = "⼀般"; else if (average>=60&&average<70) courseGrade = "及格"; else courseGrade = "不及格";}}(2)本科⽣类UnderGraduatepackage experiment5_1;public class undergraduate extends Student {public undergraduate(String name ) {super(name);type = "本科⽣";}public void calculateGrade() {int total = 0;double average = 0;for (int i = 0; i < CourseNo; i++) {total += getCourseScore(i) ;};average = total / CourseNo;if (average>=80&&average<100) courseGrade = "优秀"; else if (average>=70&&average<80) courseGrade = "良好"; else if (average>=60&&average<70) courseGrade = "⼀般"; else if (average>=50&&average<60) courseGrade = "及格"; else courseGrade = "不及格";}}4.编写代码测试函数package experiment5_1;public class polymorphism {public static void main(String[] args) {Student[] students = new Student[5];students[0] = new undergraduate("陈建平");students[1] = new undergraduate("鲁向东");students[2] = new postgraduate("匡晓华");students[3] = new undergraduate("周丽娜");students[4] = new postgraduate("梁欣欣");for (int i=0; i<5 ;i++) {students[i].setCourseScore(0,87);students[i].setCourseScore(1,90);students[i].setCourseScore(2,78);}for (int i=0; i<5 ;i++) {students[i].calculateGrade();}System.out.println("姓名" + " 类型" +" 成绩");System.out.println("-----------------------");for (int i=0; i<5 ;i++) {System.out.println(students[i].getName( )+" "+students[i].getType( )+" "+students[i].getCourseGrade( ));}}}实验内容⼆1.建⽴package experiment5_2,其最终⽬录结构如下:2.写接⼝Soundable代码:package experiment5_2;public interface Soundable {public void increaseV olume( );public void decreaseV olume( );public void stopSound( );public void playSound( );}3.创建三个类Radio、Walkman和Mobilephone具体实现,分别添加代码:// Mobilephone类package experiment5_2;class Mobilephone implements Soundable{public void increaseV olume( ) {System.out.println("增⼤⼿机⾳量");}public void decreaseV olume( ) {System.out.println("减⼩⼿机⾳量");}public void stopSound( ) {System.out.println("关闭⼿机");}public void playSound( ) {System.out.println("⼿机发出来电铃声");}}// Walkman类package experiment5_2;class Walkman implements Soundable { public void increaseV olume( ) { System.out.println("增⼤随声听⾳量"); }public void decreaseV olume( ) { System.out.println("减⼩随声听⾳量"); }public void stopSound( ) {System.out.println("关闭随声听");}public void playSound( ) {System.out.println("随声听发出⾳乐"); }}// Radio类package experiment5_2;class Radio implements Soundable{ public void increaseV olume( ) { System.out.println("增⼤收⾳机⾳量"); }public void decreaseV olume( ) { System.out.println("减⼩收⾳机⾳量"); }public void stopSound( ) {System.out.println("关闭收⾳机");}public void playSound( ) {System.out.println("收⾳机播放⼴播"); }}4.创建people类及编写测试代码://People类package experiment5_2;class People {public void listen(Soundable s) {s.playSound( );}}//测试代码package experiment5_2;import java.util.Scanner;public class InterfaceTest {public static void main(String[] args) {int i;People sportsman = new People( );Scanner scanner = new Scanner(System.in);Soundable[] soundDevice = new Soundable[3];//往声⾳设备数组中放⼊能发声的设备soundDevice[0] = new Radio( );soundDevice[1] = new Walkman( );soundDevice[2] = new Mobilephone();System.out.println("你想听什么? 请输⼊选择:0-收⾳机1-随声听2-⼿机");i = scanner.nextInt( );//开始听声⾳sportsman.listen(soundDevice[i]);soundDevice[i].increaseV olume( );soundDevice[i].stopSound();scanner.close();}}实验内容三1.建⽴package experiment5_3,其最终⽬录结构如下package experiment5_3;import java.awt.*;import java.awt.event.*;import javax.swing.*;public class DateTransf extends JFrame implements KeyListener{/****/private static final long serialVersionUID = 1L;private static DateTransf frm;private static JTextField txt;private static JTextField data;DateTransf() {setTitle("数字与星期转换");setLocation(700, 300);setSize(400,130);}public static void main(String[] args) {frm = new DateTransf();frm.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);frm.setLayout(new GridLayout(2,2));txt = new JTextField(12);data = new JTextField(12);JLabel lable1 = new JLabel("输⼊数字:");JLabel lable2 = new JLabel("星期⼏:");frm.add(lable1);txt.addKeyListener(frm);frm.add(txt);frm.add(lable2);frm.add(data);frm.setVisible(true);}public void keyPressed(KeyEvent e) {if (e.getSource() == txt) {if (e.getKeyCode() == KeyEvent.VK_ENTER) // 判断按下的键是否是回车键{try {int number = Integer.parseInt(txt.getText());switch (number) {case 1:data.setText("Mon");break;case 2:data.setText("Tue");break;case 3:data.setText("Wen");break;case 4:data.setText("Thu");break;case 5:data.setText("Fri");break;case 6:data.setText("Sat");break;case 7:data.setText("Sun");break;default:JOptionPane.showMessageDialog(null, "您输⼊的数字不是1~7","⽆效⽇期",/doc/1bd4a299a66e58fafab069dc5022aaea998f41e2.html RMATION_MESSAGE); break;}}catch (Exception e1) {// TODO: handle exceptionJOptionPane.showMessageDialog(null, "您输⼊的不是整数","⽆效⽇期",/doc/1bd4a299a66e58fafab069dc5022aaea998f41e2.html RMA TION_MESSAGE); }}}}public void keyReleased(KeyEvent e) {}public void keyTyped(KeyEvent e) {}}2.编译运⾏五、实验结果●实验内容⼀结果:●实验内容⼆结果:●实验内容三结果:六、实验⼩结1.这次实验的内容⽐较多,需要掌握Java的类和对象的声明和使⽤⽅法、Java的类的继承和实现⽅法、多态性在类的继承中的运⽤、接⼝的定义与使⽤、基本异常的处理机制、try语句与catch语句的搭配使⽤等等;2. 在实验过程中,重点是要区分好实例和类,灵活掌握类的调⽤。
java类的继承实验报告

java类的继承实验报告Java类的继承实验报告引言:在面向对象的编程语言中,继承是一种重要的概念。
Java作为一门面向对象的编程语言,也支持类的继承。
本篇实验报告将介绍Java类的继承的基本概念、语法以及实际应用。
一、继承的基本概念继承是指一个类可以派生出另一个类,被派生出的类称为子类,派生出子类的类称为父类。
子类可以继承父类的属性和方法,并且可以在此基础上进行扩展或修改。
继承的关系可以形成类的层次结构,使得代码的复用性和可维护性得到提高。
二、继承的语法在Java中,使用关键字"extends"来实现类的继承关系。
子类通过继承父类来获得父类的属性和方法。
语法格式如下:```javaclass 子类名 extends 父类名 {// 子类的成员变量和方法}```在子类中可以重写父类的方法,以实现自己的逻辑。
使用关键字"super"可以调用父类的构造方法和成员变量。
三、继承的实际应用1. 代码复用继承的一个主要优势是可以实现代码的复用。
子类可以继承父类的属性和方法,避免了重复编写相同的代码。
例如,假设有一个父类"Animal",子类"Dog"和"Cat"可以继承父类的一些通用属性和方法,如"eat()"和"sleep()"。
2. 多态性继承也为多态性的实现提供了基础。
多态性是指一个对象可以根据不同的类型表现出不同的行为。
通过继承,可以将不同的子类对象赋给父类引用,实现对不同子类对象的统一操作。
例如,有一个父类"Shape",子类"Circle"和"Rectangle"可以继承父类的方法"draw()",并在自己的类中实现具体的绘制逻辑。
通过将子类对象赋给父类引用,可以统一调用"draw()"方法,实现对不同形状的绘制。
Java实验指导4继承与多态
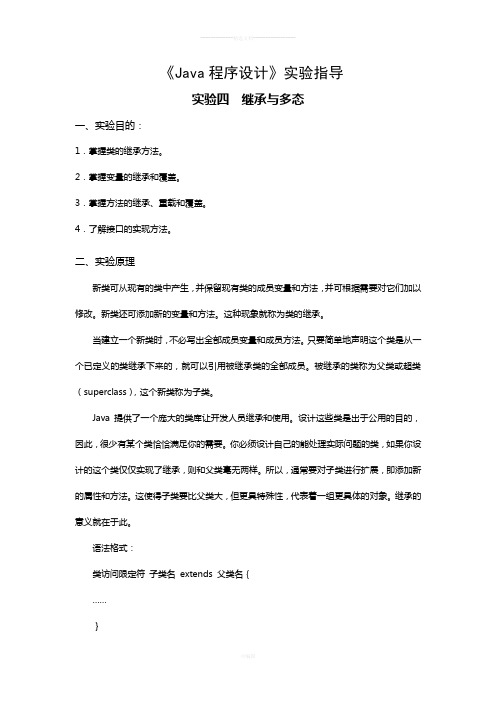
《Java程序设计》实验指导实验四继承与多态一、实验目的:1.掌握类的继承方法。
2.掌握变量的继承和覆盖。
3.掌握方法的继承、重载和覆盖。
4.了解接口的实现方法。
二、实验原理新类可从现有的类中产生,并保留现有类的成员变量和方法,并可根据需要对它们加以修改。
新类还可添加新的变量和方法。
这种现象就称为类的继承。
当建立一个新类时,不必写出全部成员变量和成员方法。
只要简单地声明这个类是从一个已定义的类继承下来的,就可以引用被继承类的全部成员。
被继承的类称为父类或超类(superclass),这个新类称为子类。
Java 提供了一个庞大的类库让开发人员继承和使用。
设计这些类是出于公用的目的,因此,很少有某个类恰恰满足你的需要。
你必须设计自己的能处理实际问题的类,如果你设计的这个类仅仅实现了继承,则和父类毫无两样。
所以,通常要对子类进行扩展,即添加新的属性和方法。
这使得子类要比父类大,但更具特殊性,代表着一组更具体的对象。
继承的意义就在于此。
语法格式:类访问限定符子类名extends 父类名{……}三、实验内容及要求:1. 定义一个接口(ShapeArea),其中包含返回面积的方法(getArea)。
定义一个矩形类(Rectangle),派生出一个正方形类(Square),再定义一个圆类(Circle),三者都要求实现接口ShapeArea,自行扩充成员变量和方法。
在主方法中建一数组,数组中放入一些上述类型的对象,并计算它们的面积之和。
2. 运行下面的程序,理解成员变量的继承与隐藏,方法的覆盖与重载。
class A{int sum,num1,num2;public A(){num1=10;num2=20;sum=0;}void sum1(){sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}void sum2(int n){num1=n;sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}}class B extends A{int num2;public B(){num2=200;}void sum2(){sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}void sum2(int n){num1=n;sum=num1+num2;System.out.println("sum="+num1+"+"+num2+"="+sum);}void sum3(int n){super.sum2(n);System.out.println("sum="+num1+"+"+num2+"="+sum);}}public class test{public static void main (String arg[]){B m=new B();m.sum1();m.sum2();m.sum2(50);m.sum3(50);}}。
实验三 继承和多态
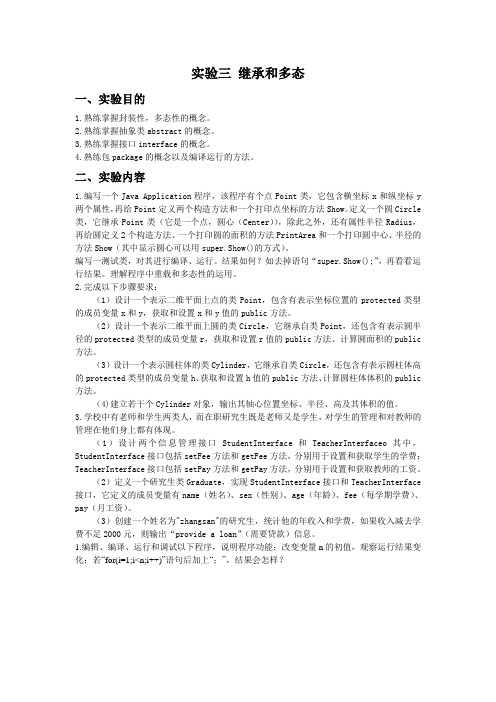
实验三继承和多态一、实验目的1.熟练掌握封装性,多态性的概念。
2.熟练掌握抽象类abstract的概念。
3.熟练掌握接口interface的概念。
4.熟练包package的概念以及编译运行的方法。
二、实验内容1.编写一个Java Application程序,该程序有个点Point类,它包含横坐标x和纵坐标y 两个属性,再给Point定义两个构造方法和一个打印点坐标的方法Show。
定义一个圆Circle 类,它继承Point类(它是一个点,圆心(Center)),除此之外,还有属性半径Radius,再给圆定义2个构造方法、一个打印圆的面积的方法PrintArea和一个打印圆中心、半径的方法Show(其中显示圆心可以用super.Show()的方式)。
编写一测试类,对其进行编译、运行。
结果如何?如去掉语句“super.Show();”,再看看运行结果。
理解程序中重载和多态性的运用。
2.完成以下步骤要求:(1)设计一个表示二维平面上点的类Point,包含有表示坐标位置的protected类型的成员变量x和y,获取和设置x和y值的public方法。
(2)设计一个表示二维平面上圆的类Circle,它继承自类Point,还包含有表示圆半径的protected类型的成员变量r,获取和设置r值的public方法、计算圆面积的public 方法。
(3)设计一个表示圆柱体的类Cylinder,它继承自类Circle,还包含有表示圆柱体高的protected类型的成员变量h、获取和设置h值的public方法、计算圆柱体体积的public 方法。
(4)建立若干个Cylinder对象,输出其轴心位置坐标、半径、高及其体积的值。
3.学校中有老师和学生两类人,而在职研究生既是老师又是学生,对学生的管理和对教师的管理在他们身上都有体现。
(1)设计两个信息管理接口StudentInterface和TeacherInterfaceo其中,StudentInterface接口包括setFee方法和getFee方法,分别用于设置和获取学生的学费;TeacherInterface接口包括setPay方法和getPay方法,分别用于设置和获取教师的工资。
继承与多态java
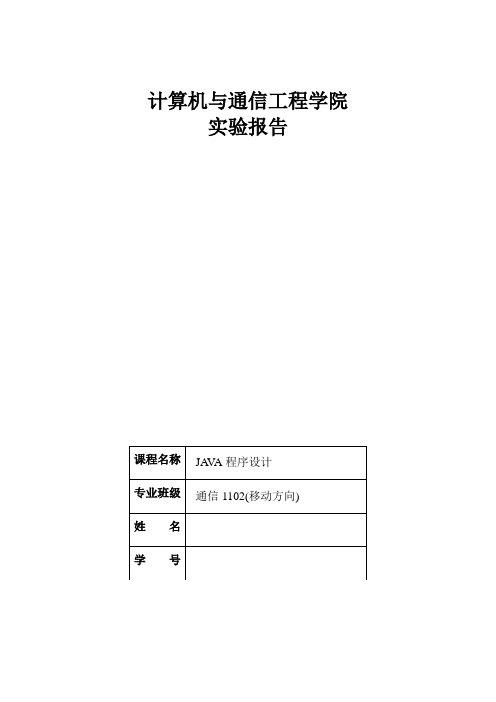
super(newsurname,newname,newssn);
hourly=newhourly;
hours=newhours;
}
public void weeksalary()
{ week=hourly*hours;
}
public String toString()
{ return surname+name+"\t"+ssn+"\t"+week;
专业班级
通信1102(移动方向)
姓名
学号
时间:2013.11.11
地点:计通学院106机房
实验名称:继承与多态
实验前任务
实验目的:
1.理解继承是如何促进软件重用的
2.理解父类和子类的概念
3.能够通过继承已有的类来创建新类,并能吸收其属性和行为
4.理解访问修饰符protected
5.更够用关键字super访问父类成员
AmericanPeople americanPeople=new AmericanPeople();
BeijingPeople beijingPeople=new BeijingPeople();
chinaPeople.speakHello();
americanPeople.speakHello();
注:用相应的输出语句表示出语义即可。
3.抽象类和抽象方法。定义一个抽象类Shape,类里有一个抽象方法display();定义一个Cricle类,继承了Shape类,并实现抽象方法display();定义一个Rectangle类,继承了Shape类,并实现抽象方法display();定义一个Triangle类,继承了Shape类,并实现抽象方法display();定义一个类,实例化上述几个类的对角,并使用上述几个类的display方法。注:用相应的输出语句表示出语义即可
类的继承与多态性实验报告
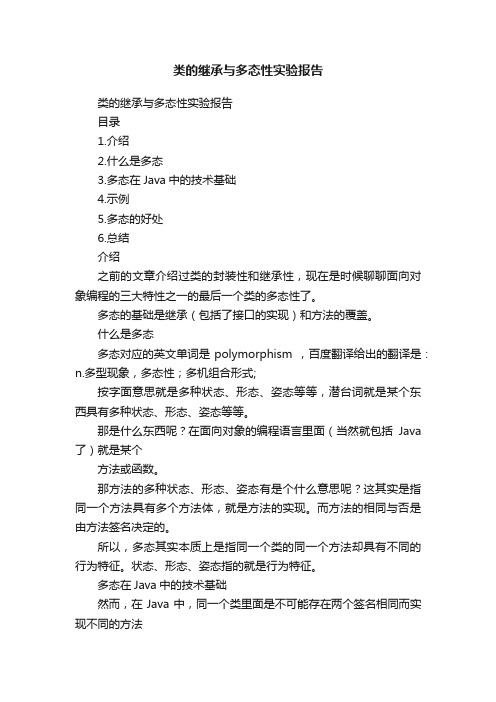
类的继承与多态性实验报告类的继承与多态性实验报告目录1.介绍2.什么是多态3.多态在Java中的技术基础4.示例5.多态的好处6.总结介绍之前的文章介绍过类的封装性和继承性,现在是时候聊聊面向对象编程的三大特性之一的最后一个类的多态性了。
多态的基础是继承(包括了接口的实现)和方法的覆盖。
什么是多态多态对应的英文单词是polymorphism ,百度翻译给出的翻译是:n.多型现象,多态性;多机组合形式;按字面意思就是多种状态、形态、姿态等等,潜台词就是某个东西具有多种状态、形态、姿态等等。
那是什么东西呢?在面向对象的编程语言里面(当然就包括Java 了)就是某个方法或函数。
那方法的多种状态、形态、姿态有是个什么意思呢?这其实是指同一个方法具有多个方法体,就是方法的实现。
而方法的相同与否是由方法签名决定的。
所以,多态其实本质上是指同一个类的同一个方法却具有不同的行为特征。
状态、形态、姿态指的就是行为特征。
多态在Java中的技术基础然而,在Java中,同一个类里面是不可能存在两个签名相同而实现不同的方法的,否则的话会导致无法判断该执行哪个方法,因此在编译时就会报错。
所以,肯定是在两个类中才有可能存在两个签名相同而实现不同的方法,一个实现在这个类,另一个实现在另一个类。
而如果这两个类毫无瓜葛,那么肯定就与多态的本质(同一个类的同一个方法却具有不同的行为特征)自相矛盾了。
所以,这两个类肯定是有某种联系的。
我们再想想,什么概念是能够让两个不同的类却又能称为同一个类的? 答案就是类的继承/扩展,就是现实中的“某东西是某类东西”的概念,就是“具体和抽象”的思想。
比如,男人是人,女人也是人,男人类和女人类就借助于人类建立了某种联系,而人类具有的某个行为在男人类和女人类中是有着不同体现的,比如人类的吃饭这个行为,男人类的体现是狼吞虎咽,女人类的体现是细嚼慢咽。
例子不是很恰当,但意思就是这么个意思。
所以说,Java里面多态的技术基础就是方法的覆盖,当然,在Java中覆盖不仅仅发生在类的继承/扩展上,还可能发生在接口的实现上。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验项目名称:继承和多态
(所属课程:Java语言程序设计)
院系:专业班级:姓名:
学号:实验地点:指导老师:
本实验项目成绩:教师签字:日期:
1.实验目的
(1)掌握类的继承机制。
(2)熟悉类中成员变量和方法的访问控制。
(3)熟悉方法或构造方法多态性。
2.实验内容
(1)模拟编写程序,理解类的继承、多态、继承和多态规则。
(2)独立编程,实现类的继承和多态。
3.实验作业
设计一个类Shape(图形)包含求面积和周长的area()方法和perimeter()方法以及设置颜色的方法SetColor(),并利用Java多态技术设计其子类Circle(圆形)类、Rectangle (矩形)类和Triangle(三角形)类,并分别实现相应的求面积和求周长的方法。
每个类都要覆盖toString方法。
海伦公式:三角形的面积等于s(s-a)(s-b)(s-c)的开方,其中s=(a+b+c)/2
程序代码为:
Class包
package Class;
public class Shape {
private String color = "while";
public Shape(String color){
this.color = color;
}
public void setColor(String color){
this.color = color;
}
public String getColor(){
return color;
}
public double getArea(){
return 0;
}
public double getPerimeter(){
return 0;
}
public String toString(){
return"color:" + color;
}
}
package Class;
public class Circle extends Shape {
private double radius;
public Circle(String color,double radius) { super(color);
this.radius = radius;
}
public void setRadius(double radius){
this.radius = radius;
}
public double getRadius(){
return radius;
}
public double getCircleArea(){
return 3.14*radius*radius;
}
public double getCirclePerimeter(){
return 3.14*2*radius;
}
public String toString(){
return"The Area is:" + getCircleArea()
+ "\nThe Perimeter is:" + getCirclePerimeter();
}
}
package Class;
public class Rectangle extends Shape{
private double width;
private double height;
public Rectangle(String color,double width,double height) { super(color);
this.width = width;
this.height = height;
}
public void setWidth(double width){
this.width = width;
}
public double getWidth(){
return width;
}
public void setHeight(double height){
this.height = height;
}
public double getHeight(){
return height;
}
public double getRectangleArea(){
return width*height;
}
public double getRectanglePerimeter(){
return 2*(width + height);
}
public String toString(){
return"The Area is:" + getRectangleArea()
+ "\nThe Perimeter is:" + getRectanglePerimeter();
}
}
package Class;
public class Triangle extends Shape{
private double a;
private double b;
private double c;
private double s;
public Triangle(String color,double a,double b,double c, double s){ super(color);
this.a = a;
this.b = b;
this.c = c;
this.s = s;
}
public void setA(double a){
this.a = a;
}
public double getA(){
return a;
}
public void setB(double b){
this.b = b;
}
public double getB(){
return b;
}
public void setC(double c){
this.c = c;
}
public double getC(){
return c;
}
public double getTriangleArea(){
return Math.sqrt(s*(s-a)*(s-b)*(s-c));
}
public double getTrianglePerimeter(){
return a + b + c;
}
public String toString(){
return"The Area is:" + getTriangleArea()
+ "\nThe Perimeter is:" + getTrianglePerimeter();
}
}
Main包
package Main;
import Class.Shape;
import Class.Circle;
import Class.Rectangle;
import Class.Triangle;
import java.util.Scanner;
public class test {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入圆的半径: ");
double radius = input.nextDouble();
Circle circle = new Circle(null, radius);
System.out.println(circle.toString());
System.out.print("\n请输入矩形的宽: ");
double width = input.nextDouble();
System.out.print("请输入矩形的高: ");
double height = input.nextDouble();
Rectangle rectangle = new Rectangle(null, width, height);
System.out.println(rectangle.toString());
System.out.print("\n请输入三角形的第一条边 a: ");
double a = input.nextDouble();
System.out.print("请输入三角形的第二条边 b: ");
double b = input.nextDouble();
System.out.print("请输入三角形的第三条边 c: ");
double c = input.nextDouble();
double s = (a + b + c)/2;
Triangle triangle = new Triangle(null, a, b, c, s);
System.out.println(triangle.toString());
}
}
运行结果为:
4.实验总结
(1)通过实验掌握了类的继承机制。
(2)熟悉了类中成员变量和方法的访问控制。
(3)了解了方法或构造方法多态性。