第8章 ASP组件
visual basic 2012 大学教程(第8章)

8. FilesConsciousness ... does not appear to itself chopped up in bits. ... A “river”or a “stream” are the metaphors by which it is most naturally described.—William JamesI can only assume that a “Do Not File” document is filed in a “Do NotFile” file.—Senator Frank Church, Senate Intelligence Subcommittee Hearing, 1975ObjectivesIn this chapter you’ll learn:• To use file processing to implement a business app.• To create, write to and read from files.• To become familia r with sequential-access file processing.• To use classes StreamWriter and StreamReader to write text to and read textfrom files.• To organize GUI commands in menus.• To manage resources with Using statements and the Finally block of a Trystatement.Outline8.1 Introduction8.2 Data Hierarchy8.3 Files and Streams8.4 Test-Driving the Credit Inquiry App8.5 Writing Data Sequentially to a Text File8.5.1 Class CreateAccounts8.5.2 Opening the File8.5.3 Managing Resources with the Using Statement8.5.4 Adding an Account to the File8.5.5 Closing the File and Terminating the App8.6 Building Menus with the Windows Forms Designer8.7Credit Inquiry App: Reading Data Sequentially from a Text File8.7.1 Implementing the Credit Inquiry App8.7.2 Selecting the File to Process8.7.3 Specifying the Type of Records to Display8.7.4 Displaying the Records8.8 Wrap-UpSummary | Terminology | Self-Review Exercises | Answers to Self-Review Exercises | Exercises8.1. IntroductionVariables and arrays offer only temporary storage of data in memory—the data is lost, for example, when a local variable ―goes out of scope‖ or when the app terminates. By contrast, files (and databases, which we cover in Chapter 12) are used for long-term retention of large (and often vast) amounts of data, even after the app that created the data terminates, so data maintained in files is often called persistent data. Computers store files on secondary storage devices, such as magnetic disks, optical disks (like CDs, DVDs and Bluray Discs™), USB flash drives and magnet ic tapes. In this chapter, we explain how to create, write to and read from data files. We continue our treatment of GUIs, explaining how to organize commands in menus and showing how to use the Windows Forms Designer to rapidly create menus. We also discuss resource management—as apps execute, they often acquire resources, such as memory and files, that need to be returned to the system so they can be reused at a later point. We show how to ensure that resources are properly returned to the system when the y’re no longer needed.8.2. Data HierarchyUltimately, all data items that computers process are reduced to combinations of 0s and 1s. This occurs because it’s simple and economical to build electronic devices that can assume two stable states—one represents 0 and the other represents 1. It’s remarkable that the impressive functions performed by computers involve only the most fundamental manipulations of 0s and 1s!BitsThe smallest data item that computers support is called a bit, short for ―binary digit‖—a digit that can assume either the value 0 or the value 1. Computer circuitry performs various simple bit manipulations, such as examining the value of a bit, setting the value of a bit and reversing a bit (from 1 to 0 or from 0 to 1). For more information on the binary number system, see Appendix C, Number Systems.CharactersProgramming with data in the low-level form of bits is cumbersome. It’s preferable to program with data in forms such as decimal digits (that is, 0, 1, 2, 3, 4, 5, 6, 7, 8 and 9), letters (that is, the uppercase letters A–Z and the lowercase letters a–z) and special symbols (that is, $, @, %, &, *, (, ), -, +, ", :, ?, / and many others). Digits, letters and special symbols are referred to as characters. The set of all characters used to write programs and represent data items on a particular computer is called that computer’s character set. Because computers process only 0s and 1s, every character in a computer’s character set is represented as a pattern of 0s and 1s. Bytes are composed of eight bits. Visual Basic uses the Unicode character set, in which each character is typically composed of two bytes (and hence 16 bits). You create programs and data items with characters; computers manipulate and process these characters as patterns of bits. FieldsJust as characters are composed of bits, fields are composed of characters. A field is a group of characters that conveys meaning. For example, a field consisting of uppercase and lowercase letters can represent a person’s name.Data HierarchyData items processed by computers form a data hierarchy (Fig. 8.1), in which data items become larger and more complex in structure as we progress up the hierarchy from bits to characters to fields to larger data aggregates.Fig. 8.1. Data hierarchy (assuming that files are organized into records). RecordsTypically, a record is composed of several related fields. In a payroll system, for example, a record for a particular employee might include the following fields:1. Employee identification number2. Name3. Address4. Hourly pay rate5. Number of exemptions claimed6. Year-to-date earnings7. Amount of taxes withheldIn the preceding example, each field is associated with the same employee. A data file can be implemented as a group of related records.1A company’s payroll file normally contains one record for each employee. Companies typically have many files, some containing millions, billions or even trillions of characters of information.1. In some operating systems, a file is viewed as nothing more than a collection ofbytes, and any organization of the bytes in a file (such as organizing the data into records) is a view created by the programmer.To facilitate the retrieval of specific records from a file, at least one field in each record can be chosen as a record key, which identifies a record as belonging to a particular person or entity and distinguishes that record from all others. For example, in a payroll record, the employee identification number normally would be the record key. Sequential FilesThere are many ways to organize records in a file. A common organization is called a sequential file in which records typically are stored in order by a record-key field. In a payroll file, records usually are placed in order by employee identification number. DatabasesMost businesses use many different files to store data. For example, a company might have payroll files, accounts receivable files (listing money due from clients), accounts payable files (listing money due to suppliers), inventory files (listing facts about all the items handled by the business) and many other files. Related files often are stored in a database. A collection of programs designed to create and manage databases is called a database management system (DBMS). You’ll learn about databases in Chapter 12 and you’ll do additional work with databases in Chapter 13, Web App Development with , and online Chapters 24–25.8.3. Files and StreamsVisual Basic views a file simply as a sequential stream of bytes (Fig. 8.2). Depending on the operating system, each file ends either with an end-of-file marker or at a specific byte number that’s recorded in a system-maintained administrative data structure for the file. You open a file from a Visual Basic app by creating an object that enables communication between an app and a particular file, such as an object of class StreamWriter to write text to a file or an object of class StreamReader to read text from a file.Fig. 8.2. Visual Basic’s view of an n-byte file.8.4. Test-Driving the Credit Inquiry AppA credit manager would like you to implement a Credit Inquiry app that enables the credit manager to separately search for and display account information for customers with• debit balances—customers who owe the company money for previously received goods and services• credit balances—customers to whom the company owes money• zero balances—customers who do not owe the company moneyThe app reads records from a text file then displays the contents of each record that matches the type selected by the credit manager, whom we shall refer to from this point forward simply as ―the user.‖Opening the FileWhen the user initially executes the Credit Inquiry app, the Button s at the bottom of the window are disabled (Fig. 8.3(a))—the user cannot interact with them until a file has been selected. The company could have several files containing account data, so to begin processing a file of accounts, the user selects Open...from the app’s custom File menu (Fig. 8.3(b)), which you’ll create in Section 8.6. This displays an Open dialog (Fig.8.3(c)) that allows the user to specify the name and location of the file from which the records will be read. In our case, we stored the file in the folder C:\DataFiles and named the file Accounts.txt. The left side of the dialog allows the user to locate the file on disk. The user can then select the file in the right side of the dialog and click the Open Button to submit the file name to the app. The File menu also provides an Exit menu item that allows the user to terminate the app.a) Initial GUI with Buttons disabled until the user selects a file from which to readrecordsb) Selecting the Open... menu item from the File menu displays the Open dialog inpart (c)c) The Open dialog allows the user to specify the location and name of the fileFig. 8.3. GUI for the Credit Inquiry app.Displaying Accounts with Debit, Credit and Zero BalancesAfter selecting a file name, the user can click one of the Button s at the bottom of the window to display the records that match the specified account type. Figure 8.4(a) shows the accounts with debit balances. Figure 8.4(b) shows the accounts with credit balances. Figure 8.4(c) shows the accounts with zero balances.a) Clicking the Debit Balances Button displays the accounts with positive balances(that is, the people who owe the company money)b) Clicking the Credit Balances Button displays the accounts with negative balances(that is, the people to whom the company owes money)c) Clicking the Zero Balances Button displays the accounts with zero balances (that is, the people who do not have a balance because they’ve already paid or have nothad any recent transactions)Fig.8.4. GUI for Credit Inquiry app.8.5. Writing Data Sequentially to a Text FileBefore we can implement the Credit Inquiry app, we must create the file from which that app will read records. Our first app builds the sequential file containing the account information for the company’s clients. For each client, the app obtains through its GUI the c lient’s account number, first name, last name and balance—the amount of money that the client owes to the company for previously purchased goods and services. The data obtained for each client constitutes a ―record‖ for that client. In this app, the accoun t number is used as the record key—files are often maintained in order by their record keys. For simplicity, this app assumes that the user enters records in account number order.GUI for the Create Accounts AppThe GUI for the Create Accounts app is shown in Fig. 8.5. This app introduces the Menu-Strip control which enables you to place a menu bar in your window. It alsointroduces ToolStripMenuItem controls which are used to create menus and menu items. We show how use the IDE to build the menu and menu items in Section 8.6. There you’ll see that the menu and menu item variable names are generated by the IDE and begin with capital letters. Like other controls, you can change the variable names in theProperties window by modifying the (Name) property.Fig. 8.5. GUI for the Create Accounts app.Interacting with the Create Accounts AppWhen the user initially executes this app, the Close menu item, the TextBox es and the Add Account Button are disabled (Fig. 8.6(a))—the user can interact with these controls only after specifying the file into which the records will be saved. To begin creating a fileof accounts, the user selects File > New... (Fig. 8.6(b)), which displays a Save As dialog (Fig. 8.6(c)) that allows the user to specify the name and location of the file into which the records will be placed. The File menu provides two other menu items—Close to close the file so the user can create another file and Exit to terminate the app. After the user specifies a file name, the app opens the file and enables the controls, so the user can begin entering account information. Figure 8.6(d)–(h) shows the sample data being entered for five accounts. The app does not depict how the records are stored in the file. This is a text file, so after you close the app, you can open the file in any text editor to see its contents. Figure 8.6(j)shows the file’s contents in Notepad.a) Initial GUI before user selects a fileb) Selecting New... to create a filec) Save As dialog displayed when user selects New... from the File menu. In this case,the user is naming the file Accounts.txt and placing the file in the C:\DataFilesfolder.d) Creating account 100e) Creating account 200f) Creating account 300g) Creating account 400h) Creating account 500i) Closing the filej) The Accounts.txt file open in Notepad to show how the records were written to the file. Note the comma separators between the data itemsFig. 8.6. User creating a text file of account information.8.5.1. Class CreateAccountsLet’s now study the declaration of class CreateAccounts, which begins in Fig. 8.7. Framework Class Library classes are grouped by functionality into namespaces, which make it easier for you to find the classes needed to perform particular tasks. Line 3 is an Imports statement, which indicates that we’re using classes from the System.IO namespace. This namespace contains stream classes such as StreamWriter (for text output) and Stream-Reader (for text input). Line 6 declares fileWriter as an instance variable of type Stream-Writer. We’ll use this variable to interact with the file that the user selects.Click here to view code image1' Fig. 8.7: CreateAccounts.vb2' App that creates a text file of account information.3Imports System.IO ' using classes from this namespace45Public Class CreateAccounts6Dim fileWriter As StreamWriter' writes data to text file7Fig. 8.7. App that creates a text file of account information.You must import System.IO before you can use the namespace’s classes. In fact, all namespaces except System must be imported into a program to use the classes in those namespaces. Namespace System is imported by default into every program. Classes like String, Convert and Math that we’ve used frequently in earlier examples are declared in the System namespace. So far, we have not used Imports statements in any of our programs, but we have used many classes from namespaces that must be imported. For example, all of the GUI controls you’ve used so far are classes in theSystem.Windows.Forms namespace.So why were we able to compile those programs? When you create a project, each Visual Basic project type automatically imports several namespaces that are commonlyused with that project type. You can see the namespaces (Fig. 8.8) that were automatically imported into your project by right clicking the project’s name in the Solution Explorer window, selecting Properties from the menu and clicking the References tab. The list appears under Imported namespaces:—each namespace with a checkmark is automatically imported into the project. This app is a Windows Forms app. The System.IO namespace is not imported by default. To import a namespace, you can either use an Imports statement (as in line 3 of Fig. 8.7) or you can scroll through the list in Fig. 8.8 and check the checkbox for the namespace you wish to import.Fig. 8.8. Viewing the namespaces that are pre-Imported into a Windows Forms app.8.5.2. Opening the FileWhen the user selects File > New..., method NewToolStripMenuItem_Click (Fig. 8.9) is called to handle the New... m enu item’s Click event. This method opens the file. First, line 12 calls method CloseFile (Fig. 8.11, lines 102–111) in case the user previously opened another file during the current execution of the app. CloseFile closes the file associated with this app’s StreamWriter.Click here to view code image8' create a new file in which accounts can be stored9Private Sub NewToolStripMenuItem_Click(sender As Object,10 e As EventArgs) Handles NewToolStripMenuItem.Click1112 CloseFile() ' ensure that any prior file is closed13Dim result As DialogResult' stores result of Save dialog14Dim fileName As String' name of file to save data1516' display dialog so user can choose the name of the file to save17Using fileChooser As New SaveFileDialog()18 result = fileChooser.ShowDialog()19 fileName = fileChooser.FileName ' get specified file name20End Using' automatic call to fileChooser.Dispose() occurs here2122' if user did not click Cancel23If result <> Windows.Forms.DialogResult.Cancel Then24Try25' open or create file for writing26 fileWriter = New StreamWriter(fileName, True)2728' enable controls29 CloseToolStripMenuItem.Enabled = True30 addAccountButton.Enabled = True31 accountNumberTextBox.Enabled = True32 firstNameTextBox.Enabled = True33 lastNameTextBox.Enabled = True34 balanceTextBox.Enabled = True35Catch ex As IOException36 MessageBox.Show("Error Opening File", "Error",37MessageBoxButtons.OK, MessageBoxIcon.Error)38End Try39End If40End Sub' NewToolStripMenuItem_Click41Fig. 8.9. Using the SaveFileDialog to allow the user to select the file into whichrecords will be written.Next, lines 17–20 of Fig. 8.9 display the Save As dialog and get the file name specified by the user. First, line 17 creates the SaveFileDialog object (namespace System.Windows.Forms) named fileChooser. Line 18 calls its ShowDialog method to display the SaveFileDialog (Fig. 8.6(c)). This dialog prevents the user from interacting with any other window in the app until the user closes it by clicking either Save or Cancel, so it’s a modal dialog. The user selects the location where the file should be stored and specifies the file name, then clicks Save. Method ShowDialog returns a DialogResult enumeration constant specifying which button (Save or Cancel) the user clicked to close the dialog. This is assigned to the DialogResult variable result (line 18). Line 19 uses SaveFileDialog property FileName to obtain the location and name of the file.8.5.3. Managing Resources with the Using StatementLines 17–20 introduce the Using statement, which simplifies writing code in which you obtain, use and release a resource. In this case, the resource is a SaveFileDialog. Windows and dialogs are limited system resources that occupy memory and should be returned to the system (to free up that memory) as soon as they’re no longer needed. Inall our previous apps, this happens when the app terminates. In a long-running app, if resources are not returned to the system when they’re no longer needed, a resource leak occurs and the resources are not available for use in this or other apps. Objects that represent such resources typically provide a Dispose method that must be called to return the resources to the system. The Using statement in lines 17–20 creates a SaveFileDialog object, uses it in lines 18–19, then automatically calls its Dispose method to release the object’s resources as soon as End Using is reached, thusguaranteeing that the resources are returned to the system and the memory they occupy is freed up (even if an exception occurs).Line 23 tests whether the user clicked Cancel by comparing result to the constant Windows.Forms.DialogResult.Cancel. If not, line 26 creates a StreamWriter object that we’ll use to write data to the file. The two arguments are a String representing the location and name of the file, and a Boolean indicating what to do if the file already exists. If the file doesn’t exist, this statement creates the file. If the file does exist, the second argument (True) indicates that new data written to the file should be appended at the end of the file’s current contents. If the second argument is False and the file already exists, the file’s contents will be discarded and new data will be written starting at the beginning of the file. Lines 29–34 enable the Close menu item and the TextBox es and Button that are used to enter records into the app. Lines 35–37 catch an IOException if there’s a problem opening the file. If so, the app displays an error message. If no exception occurs, the file is opened for writing. Most file-processing operations have the potential to throw exceptions, so such operations are typically placed in Try statements.8.5.4. Adding an Account to the FileAfter typing information in each TextBox, the user clicks the Add Account Button, which calls method addAccountButton_Click (Fig. 8.10) to save the data into the file. If the user entered a valid account number (that is, an integer greater than zero), lines 56–59 write the record to the file by invoking the StreamWriter’s WriteLine method, which writes a sequence of characters to the file and positions the output cursor to the beginning of the next line in the file. We separate each field in the record with a comma in this example (this is known as a comma-delimited text file), and we place each record on its own line in the file. If an IOException occurs when attempting to write the record to the file, lines 64–66 Catch the exception and display an appropriate message to the user. Similarly, if the user entered invalid data in the accountNumberTextBox or balanceTextBox lines 67–69 catch the FormatExceptions thrown by class Convert’s methods and display an appropriate error message. Lines 73–77 clear the TextBox es and return the focus to the accountNumberTextBox so the user can enter the next record. Click here to view code image42' add an account to the file43Private Sub addAccountButton_Click(sender As Object,44 e As EventArgs) Handles addAccountButton.Click4546' determine whether TextBox account field is empty47If accountNumberTextBox.Text <> String.Empty Then48' try to store record to file49Try50' get account number51Dim accountNumber As Integer =52 Convert.ToInt32(accountNumberTextBox.Text)5354If accountNumber > 0Then' valid account number?55' write record data to file separating fields by commas56 fileWriter.WriteLine(accountNumber & "," &57 firstNameTextBox.Text & "," &58 lastNameTextBox.Text & "," &59 Convert.ToDecimal(balanceTextBox.Text))60Else61 MessageBox.Show("Invalid Account Number", "Error",62MessageBoxButtons.OK, MessageBoxIcon.Error)63End If64Catch ex As IOException65 MessageBox.Show("Error Writing to File", "Error",66MessageBoxButtons.OK, MessageBoxIcon.Error)67Catch ex As FormatException68 MessageBox.Show("Invalid account number or balance",69"Format Error", MessageBoxButtons.OK,MessageBoxIcon.Error)70End Try71End If7273 accountNumberTextBox.Clear()74 firstNameTextBox.Clear()75 lastNameTextBox.Clear()76 balanceTextBox.Clear()77 accountNumberTextBox.Focus()78End Sub' addAccountButton_Click79Fig. 8.10. Writing an account record to the file.8.5.5. Closing the File and Terminating the AppWhen the user selects File > Close, method CloseToolStripMenuItem_Click (Fig. 8.11, lines 81–91) calls method CloseFile (lines 102–111) to close the file. Then lines 85–90 disable the controls that should not be available when a file is not open.Click here to view code image80' close the currently open file and disable controls81Private Sub CloseToolStripMenuItem_Click(sender As Object,82 e As EventArgs) Handles CloseToolStripMenuItem.Click8384 CloseFile() ' close currently open file85 CloseToolStripMenuItem.Enabled = False86 addAccountButton.Enabled = False87 accountNumberTextBox.Enabled = False88 firstNameTextBox.Enabled = False89 lastNameTextBox.Enabled = False90 balanceTextBox.Enabled = False91End Sub' CloseToolStripMenuItem_Click9293' exit the app94Private Sub ExitToolStripMenuItem_Click(sender As Object,95 e As EventArgs) Handles ExitToolStripMenuItem.Click9697 CloseFile() ' close the file before terminating app98 Application.Exit() ' terminate the app99End Sub' ExitToolStripMenuItem_Click100101' close the file102Sub CloseFile()103If fileWriter IsNot Nothing Then104Try105 fileWriter.Close() ' close StreamWriter106Catch ex As IOException107 MessageBox.Show("Error closing file", "Error",108MessageBoxButtons.OK, MessageBoxIcon.Error)109End Try110End If111End Sub' CloseFile112End Class' CreateAccountsFig. 8.11. Closing the file and terminating the app.When the user clicks the Exit menu item, method ExitToolStripMenuItem_Click (lines 94–99) respo nds to the menu item’s Click event by exiting the app. Line 97 closes the StreamWriter and the associated file, then line 98 terminates the app. The call to method Close (line 105) is located in a Try block. Method Close throws an IOExceptionif the file cannot be closed properly. In this case, it’s important to notify the user that the information in the file or stream might be corrupted.8.6. Building Menus with the Windows Forms DesignerIn the test-drive of the Credit Inquiry app (Section 8.4) and in the overview of the Create Accounts app (Section 8.5), we demonstrated how menus provide a convenient way to organize the commands that you use to interact with an app without ―cluttering‖ its user interface. Menus contain groups of related commands. When a command is selected, the app performs a specific action (for example, select a file to open, exit the app, etc.).Menus make it simple and straightforward to locate an app’s commands. They can also make it easier for users to use apps. For example, many apps provide a File menu that contains an Exit menu item to terminate the app. If this menu item is always placed in the File menu, then users become accustomed to going to the File menu to terminate an app. When they use a new app and it has a File menu, they’ll already be familiar with the location of the Exit command.The menu that contains a menu ite m is that menu item’s parent menu. In the Create Accounts app, File is the parent menu that contains three menu items—Ne w..., Close and Exit.Adding a MenuStrip to the FormBefore you can place a menu on your app, you must provide a MenuStrip to organize and manage the app’s menus. Double click the MenuStrip control in the Toolbox. This creates a menu bar (the MenuStrip) across the top of the Form (below the title bar; Fig.8.12) and places a MenuStrip icon in the component tray (the gray area) at the bottom of the designer. You can access the MenuStrip’s properties in the Properties window by clicking the MenuStrip icon in the component tray. We set the MenuStrip’s (Name) property to applicationMenuStrip.。
ASP完整教程(完全ASP编程)
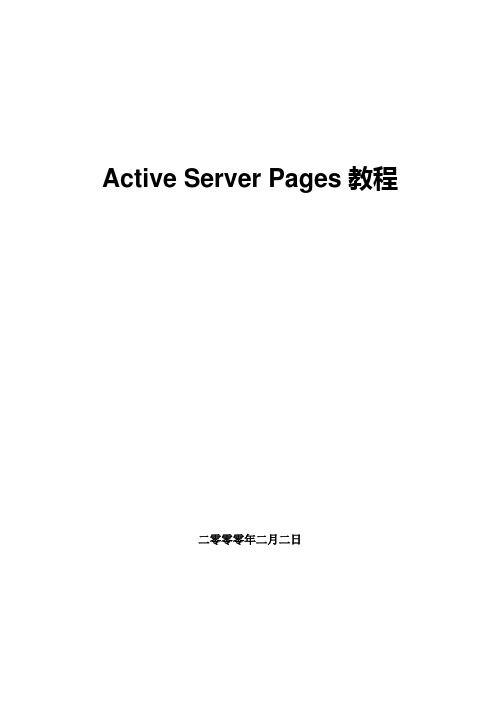
Active Server Pages教程二零零零年二月二日目录第一章引言 (3)第二章简介 (8)第三章ASP基础 (13)第四章脚本基础 (19)第五章脚本变量、函数、过程和条件语句 (22)第六章脚本循环语句 (27)第七章内建Request对象 (30)第八章内建Reponse对象 (36)第九章内建对象Application 和Session (42)第十章Global.asa文件的使用及Chat程序 (48)第十一章内建对象Server (53)第十二章数据库查询语言(1) (57)第十三章数据库查询语言(2) (62)第十四章ActiveX组件 (66)第十五章常用的ASP ActiveX组件 (70)第十六章其它的ASP常用组件 (75)第十七章ADO存取数据库时如何分页显示 (81)第十八章堵住ASP漏洞 (87)第十九章最新的ASP、IIS安全漏洞 (91)第一章引言您的网站是否仍一如既往地保持“静态”?目前呈几何增长的互联网网站中,有相当一部分仍固守“静态”,无形中已大大落后于时代的步伐。
所谓“静态”指的就是网站的网页内容“固定不变”,当用户浏览器通过互联网的HTTP(Hypertext Transport Pr otocol)协议向WEB服务器请求提供网页内容时,服务器仅仅是将原已设计好的静态HTML文档传送给用户浏览器。
其页面的内容使用的仅仅是标准的HTML代码,最多再加上流行的GIF89A格式的动态图片,比如产生几只小狗小猫跑来跑去的动画效果。
若网站维护者要更新网页的内容,就必须手动地来更新其所有的HTML文档。
“静态”网站的致命弱点就是不易维护,为了不断更新网页内容,你必须不断地重复制作HTML文档,随着网站内容和信息量的日益扩增,你就会感到工作量大得出乎想象。
你不禁要问:那么什么是动态网站呢?这就是本文将重点讲述的核心,所谓“动态”,并不是指那几个放在网页上的GIF动态图片,在这里笔者为动态页面的概念制定了以下几条规则:1、“交互性”即网页会根据用户的要求和选择而动态改变和响应,将浏览器作为客户端界面,这将是今后WEB发展的大事所趋。
ASP动态网页设计教程.ppt

1.2.2 ASP的运行环境及安装
如果只是用HTML设计网页,并不需要特殊的环境,
直接在浏览器上浏览即可。而用ASP设计的网页是在服
务器端运行的,因此必须配置相应的运行环境。 ASP的运行环境可以选择如下安装:
如果是在Windows 95/98平台上运行ASP文件,需要安装个
人Web服务器PWS。 如果是在Windows NT 4.0 Server平台上运行ASP文件,需
1
第1章 ASP设计基础
1.1 Web基础
1.1.1 Web概述 1.1.2 Web常用术语 1、1、3WEB服务器技术 1.2.1 什么是ASP 1.2.2 ASP的运行环境及安装 1.2.3 创建虚拟目录 1.3.1 ASP的一个简单实例 1.3.2 ASP文件的基本构成和约定 1.4.1 ASP的开发工具 1.4.2 ASP的调试技巧
16
1.2.3 创建虚拟目录(续上)
③ 选中“默认Web站点”,单击右键,选择“新建虚拟目录”,出现
如图1-9所示安装向导对话框。
17
1.2.3 创建虚拟目录(续上)
④ 在向导过程中,依次输入相应内容:别名“ASPWEB”、目录
“D:\ASPWEB”以及权限“至少包含读取和运行脚本”后,单击
“完成”按钮即可,此时如图1-10所示。
22
1.3.2 ASP文件的基本构成和约定
1、基本构成
从上述这个简单的ASP程序中,经分析发现,通常由以下3个部分构成:
普通的HTML文件,即一般的Web页面内容 放在<% … %>内的Script脚本语言代码,由服务器端运行。 放在<Script> … </Script>内的Script脚本语言代码,由客户端直接运行。
BS架构基础编程之

▪ 全书从体系上提成四大部分,共十章。
▪ 第一部分 网站编程基础 ▪ 第二部分 ASP数据库编程基础 ▪ 第三部分 ASP组件编程基础 ▪ 第四部分 课程设计与项目开发
第一部分 网站编程基础
▪ 第1章 网站编程体系概述与环境配置,简介配置
运营环境IIS5.0,测试ASP运营环境和了解BS架 构。
Hale Waihona Puke ▪ ASP 和 是 微 软 企 业 旳 产 品 , JSP 最 初 是 SUN
(Stanford University Network)企业推出旳,PHP是由 一 种 网 络 小 组 开 发 和 维 护 旳 。 目 前 最 常 用 旳 是 ASP 、 和JSP。
本书构造
第三部分 ASP组件编程基础
▪ 第8章ASP内置组件,简介文件组件、页面链接组件,浏览
器组件与广告组件旳使用措施。
▪ 第9章 使用第三方组件,简介怎样使用第三方组件来扩充
ASP旳功能。并简介文件上传组件与Email组件旳使用措施。
▪ 第10章ASP操作XML文件,简介XML旳基本概念,以及怎
样利用ASP程序来操作XML文件。
▪ 本书在编写时,主要采用案例教学法,列举
了大量旳例子。
▪ 程序要反复看,看完后要到达这种程度:不
但要精通每一句,而且对程序旳总体思想和 总体构造牢固掌握,还要清楚本例学习到旳 新知识。
▪ 假如能一边看,一边亲自输入练习,就更加
好了。看明白后来,就能够动手修改以符合 您旳要求。
全书体系
▪ 本书全方面简介客户端和服务器端旳编程
▪ 第2章HTML编程基础,简介HTML编程基础以及
怎样利用CSS语言进行页面美化。
▪ 第3章VBScript编程基础,简介脚本语言
VB Web应用程序开发

8.2 控件
• 8.2.1 控件概述 • 控件基本上有两个系列,分别是HTML服务器控件和Web (标准)服务器控件(如图8-1所示)。System.Web.UI.HtmlControls 是HTML服务器控件的命名空间,System.Web.UI.WebControls是 Web服务器控件的命名空间。
8.1.4 常用内置对象
• 在中,有5个常用内臵对象,它们是Request对象、Response对 象、Server对象、Application对象和Session对象。这些对象用来维护有 关当前应用程序、每个用户会话、当前 HTTP 请求、请求的 Web 窗体 页等信息,主要用于与用户的交互。因此,它们在应用程序扮 演非常重要的角色。 1.Response对象 Response对象用于向客户端发送信息,该对象提供了很多有用的属性和方 法。 (1)利用Response对象发送信息 Response对象最常用的功能就是向浏览器发送信息,而实现这一功能最常 用的是它的Write方法,其使用形式为: Response.Write(value) value代表发送的信息,可以是任何类型的变量或者表达式。
•
8.2.4 Button、ImageButton和 LinkButton控件
• 这三个控件使用户可以指示已完成表单或要执行特定的命令,功 能类似,但在网页上显示的方式都不同。 • 【例8-2】使用Button控件触发客户端事件。
8.2.5 Image控件和ImageMap控件
• Image控件在Web页上显示一幅图像,该图像的路径通过ImageUrl 属性设臵。图像URL既可以是相对的,也可以是绝对的,并且大 多数程序员明显喜欢相对URL,因为它们使一个Web站点更容易 迁移。由于某种原因不能找到图像或者浏览器不能生成图像时, 还可以指定要显示的替代文本。这种情况下使用的属性是 AlternateText。在页上该图像与其他元素的对齐方式使用 ImageAlign属性设臵。
《网页设计与制作》习题参考答案

《网页设计与制作》习题参考答案第一章网页设计与制作概述1、填空题(1)静态网页、动态网页(2)HyperText Mark-up Language、超文本标记语言或超文本链接标示语言(3)文字、图形图像、动画、视频(4)Microsoft Frontpage(或SharePoint Designer)、Adobe Dreamweaver2、问答题(1)www的特点有哪些?答:1)万维网是无数个网络站点和网页的集合,它们在一起构成了因特网最主要的部分。
2)WWW是建立在客户机/服务器模型之上的。
3)WWW是以超文本标注语言HTML(Hyper Markup Language)与超文本传输协议HTTP(Hyper Text Transfer Protocol)为基础。
4)WWW能够提供面向Internet服务的、一致的用户界面的信息浏览系统。
5)WWW浏览提供界面友好的信息查询接口。
(2)网页的基本元素有哪些?答:组成网页的元素主要有文字、图像、声音、动画、视频、超链接以及交互式处理等。
它们的特点如下:文字:网页中的大多数信息主要以文本方式显示,文字是网页的主体,负责传达信息的功能。
图像:图片给网页添加了色彩,使网页做到了图文并茂,形象生动。
动画:动画是动态的图形,添加动画可以使网页更加生动。
常用的动画格式包括动态GIF图片和Flash动画声音和视频:声音是多媒体网页中的重要组成部分,支持网络的声音文件格式很多,主要有MIDI、WA V、MP3和AIF等。
网页中支持的视频文件格式主要有Realplay、Mpeg、A VI和DivX等。
表格:在网页中使用表格可以控制网页中信息的结构布局。
超链接:超链接是网页与其他网络资源联系的纽带,是网页区别于传统媒体的重要特点导航栏:导航栏的作用是引导浏览者游历所有站点。
表单:表单类似于Windows程序的窗体,用来将浏览者提供的信息,提交给服务器端程序进行处理。
其他常见元素:包括悬停按钮、Java特效和ActiveX等各种特效。
ASPNET动态网页设计习题答案-作者-张德芬-教材习题和实训解答

动态网页设计-—习题和实训参考答案第1章ASP。
NET概述 (1)第2章HTML语言基础 (2)第3章语法基础 (3)第4章服务器控件 (6)第5章验证控件 (7)第6章常用内置对象 (9)第7章访问数据库 (10)第8章ASP。
NET开发实训 (12)第1章概述实训:本章的实训内容主要是建立的运行和编辑环境。
具体包括以下几项任务:1、建立以各自名字命名的文件夹,作为本课程学习目录2、安装IIS,安装完后在IE中输入http://localhost,看能否打开欢迎页面,检测是否安装成功。
localhost也可用“127。
0。
0。
1"代替。
答:安装IIS的步骤详见教材P5。
3、建立一个虚拟目录,以第1步建立的文件夹为物理目录,虚拟目录名用姓名的拼音答:建立虚拟目录的步骤详见教材P8。
4、建立.NET运行环境(1)下载和安装MDAC答:MDAC的安装详见教材P10。
(2)下载和安装。
NET Framework 1。
1版可发行组件包答:.NET Framework 1.1版可发行组件包的安装详见教材P11。
5、安装Dreamweaver 8答:Dreamweaver 8的安装详见教材P14。
6、在Dreamweaver 8中建立站点答:Dreamweaver 8中建立站点的步骤详见教材P18。
习题:1、解释动态网页和静态网页的含义。
在网页中插入动画或影视媒体是否就称其为动态网页? 答:根据网页的内容是固定的还是程序生成的,网页分为两种:静态网页和动态网页。
这两种网页的制作语言不同.静态网页使用语言是HTML(超文本标记语言),网页的内容是事先编制好的。
动态网页的网页内容不是固定不变的,而是由程序动态创建的。
根据实现技术,动态网页又可以细分为客户端动态网页和服务器端动态网页.在网页中插入动画或影视媒体并不是动态网页。
2、ASP与有什么不同?答:ASP。
NET与ASP有很大的不同。
ASP程序是解释执行,未经优化;而ASP。
网络程序ASP课后题答案

目录第1章ASP程序设计概述 (1)习题1 (1)第2章HTML基础知识 (5)习题2 (5)第3章VBScript基础知识 (11)习题3 (11)第4章Request和Response对象 (21)习题4 (21)第5章Session和Application对象 (34)习题5 (34)第6章Server对象 (40)习题6 (40)第7章数据库基础知识 (47)习题7 (47)第8章ASP存取数据库 (50)习题8 (50)第9章深入进行数据库编程 (57)习题9 (57)第10章文件存取组件及其他组件 (62)习题10 (62)第11章使用第三方组件 (68)习题11 (68)第12章网络程序开发实例 (73)习题12 (73)第1章ASP程序设计概述习题11. 选择题(可多选)(1)静态网页的扩展名一般是:A.htm B .php C.asp D.jsp答案:A(2)ASP文件的扩展名是:A.htmB. txtC. docD. asp答案:D(3)当前的Web程序开发中通常采用什么模式?A. C/SB. B/SC. B/BD. C/C答案:B解释:C/S是客户机(client)/服务器(server),B/S是浏览器(browser)/服务器。
C/S一般要求用户有特定的客户端。
比如QQ就是C/S模式,你的桌面上的QQ就是腾讯公司的特定的客户端,而服务器就是腾讯的服务器。
而B/S模式则不需要特定的客户端,只要有普通浏览器,就可以访问到服务器了,Web页就是B/S 模式。
(4)小王正在家里通过拨号上网访问搜狐主页,此时,他自己的计算机是:A. 客户端B. 既是服务器端又是客户端C. 服务器端D. 既不是服务器端也不是客户端答案:A(5)小王正在访问自己计算机上的网页,此时,他自己的计算机是:A. 客户端B. 既是服务器端又是客户端C. 服务器端D. 既不是服务器端也不是客户端答案:B(6)ASP脚本代码是在哪里执行的?A. 客户端B. 第一次在客户端,以后在服务器端C. 服务器端D. 第一次在服务器端,以后在客户端答案:C解释:ASP脚本代码是在服务器端运行的,服务器将其解释执行为标准的HTML 代码,然后发送到客户端。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
8.6 CDONTS组件
在ASP中实现电子邮件的功能。 CDONTS组件是IIS 4.0或IIS 5.0中提供的一种发送Email 的 组 件 , 其 实 例 的 创 建 也 要 使 用 Server 对 象 的 CreateObject方法,语法如下: Set myMail= Server.CreateObject("CDONTS.NewMail")
2.Ad Rotator组件的方法 该组件只有GetAdvertisement一个方法,用 于重新取得一个将要显示的图片和超文本链接。 当用户第一次启动网页或重新刷新时,系统会 重新取得下一个图片。该方法的语法格式如下 所示: GetAdvertisement(rotationSchedulePath) 其 中 rotationSchedulePath 指 定 Rotator Schedule文件相对于虚拟目录的位置,例如: MyAd.GetAdvertisement("myadrot.txt")
第8章 ASP组件
8.1 8.2 8.3 8.4 8.5 8.6 8.7 ASP组件概述 Ad Rotator组件 Browser Capabilities组件 Content Linking组件 计数器组件 CDONTS组件 其他ASP组件
8.1 ASP组件概述
ASP组件均遵循Microsoft的ActiveX标准。 ActiveX组件是一个文件,该文件包含执行一项或 一组任务的代码,组件可以执行某些特定的功能。 安装好ASP平台后,所有的ASP内置组件都被安装 注册到服务器上,调用内置组件步骤: 首先,必须创建组件的一个实例,并将该实例赋予 一个变量。 然后,调用组件的方法和属性,完成相应的功能。 ASP的内置组件有13种,而且还可以安装其他第三 方的组件。
8.2 Ad Rotator组件
可以很容易地实现在Web页面上插 入一个循环播放广告横幅的播放器。语 法如下:
Set AdRotator变量 =
Server.CreateObject("MSWC.AdRotator") AdRotator变量.属性 = 属性值 或 AdRotator变量.方法(循环定时文件路径)
2.重定向文件 重定向文件是Ad Rotator组件中的可选项, 如果在循环定时文件中指定使用重定向文件, 每当用户单击广告时,其发出的请求对象会 重定向到该文件。重定向文件是使用 Request.querystring("URL")来取得与被单击 图像相关的“URL”的。
8.3 Browser Capabilities组件
说明
8.6.2 CDONTS组件的使用
在使用前,保证在计算机中有CDONTS.DLL文件 (该文件一般存放在Winnt\System32目录下)。 首先使用DOS下的“regsvr32”命令注册该组件。 注册该组件语法为: regsvr32 [physical path]cdonts.dll 注意:应该指定cdonts.dll文件所在的物理路径。 注销该组件的语法为: regsvr32/u [physical path]cdonts.dll 就可以创建该组件的实例,然后利用该组件提供 的属性和方法进行邮件的发送。
8.6.1
属性 To
CDONTS组件的属性和方法
说明 设置收件人的邮件地址
Ac,Bcc
From Subject Body
设置一个或多个参阅的邮件地址,是用于设置抄送人与密件 抄送人的邮件地址
设置发件人的邮件地址 设置邮件主题 设置邮件内容
方法 Send AttachFile 发送邮件 设置附加文件
8.5 计数器组件
在一个计数器组件中可以有多个不同名称的计数 器,利用这些不同的计数器可以统计网站中多个元 素的数据。 计数器组件的创建语法如下: Set MyCount= Server.CreateObject("MSWC.Counters")
8.5.1 计数器组件的方法
方法名 语法 描述
Get
此组件用来获取客户端浏览器的类型。 工作原理如下:当客户的浏览器连接到服务器时,会 自 动 向 服 务 器 发 送 一 个 用 户 代 理 ( User Agent ) 的 HTTP标头(header),该标头是一个声明浏览器及其 版 本 的 ASCII 字 符 串 。 可 以 使 用 如 下 代 码 获 得 这 段 header。 <%Request.ServerVariables("HTTP_USER_AGENT ")%> 并将取得的用户代理HTTP头字符串与Browscap.ini文 件内容进行比较,若找到匹配项,根据Browscap.ini文 件中的定义就可以确定该浏览器的特性。
指定该浏览器是否支持用于Web预测的Channel Definition Format
指定该浏览器是否支持Cookies 指定该浏览器是否支持框架 指定该浏览器是否支持Java程序 指定该浏览器是否支持Jscript 指定该浏览器的运行平台 指定该浏览器是否支持表格 指定该浏览器是否支持VBScript 指定该浏览器的版本号
8.2.2 Ad Rotator组件相关文件 Ad Rotator组件除了必要的Adrot.dll文件外, 还要有循环定时文件,另外也可以有重定向 文件。
1.循环定时文件 包含Ad Rotator组件用于管理和显示各种广告图像 的信息。文件格式如下: [REDIRECT URL] [WIDTH numWidth] [HEIGHT numHeight] [BORDER numBorder] * adURL adHomePageURL Text impressions
Ad Rotator广告轮显组件的需要3个设置文件,分 别如下: 1、计划文件:记录所有广告信息的文本文件 Ad Rotator组件的工作是通过读取Ad Rotator计 划文件来完成的,包括与要显示的图象文件的路径 有关的信息以及每个图象的不同属性。 格式如下:Redirect URL Width Height Border * adURL adHomeURL Text impressions
Remove
Set
设置指定计数器的数值
8.5.2 计数器组件的使用
ASP中的计数器组件通常在Global.asa文件 中声明,如下所示: <OBJECT RUNAT=Server SCOPE=Application ID=MyCount PROGID="MSWC.Counters"> </OBJECT> 在Global.asa文件中利用HTML的 <OBJECT>标记创建一个名为MyCount的计 数器实例。
8.3.1 Browscap.ini文件
该文件中主要定义了各种浏览器所具有的属性。 [; comments] [HTTPUserAgentHeader] [parent = browserDefinition] [property1 = value1] ... [propertyN = valueN] [Default Browser Capability Settings] [defaultProperty1 = defaultValue1] ... [defaultPropertyN = defaultValueN]
允许用户指定显示广告时四周是否带边框。size指定显示的广 告四周的边框宽度,其默认值在 Rotator Schedule文件的文件 头中设置 允许用户指定是否将广告作为超链接显示。value指定广告是否 为超链接。参数值为布尔值:为TRUE时,将广告作为超链接显 示;为FALSE时,将广告不作为超链接显示。默认值是TRUE
Set NextLink=
Server.CreateObject("MSWC.NextLink")
8.4.1 线性排列顺序文件 包含Web页的相关信息列表,该文件必须在 Web服务器的虚拟路径上可用。 语法如下:
Web-page-URL [ text-description [ comment]] Web-page-URL 以 filename 或 directory\filename 表 示的Web页的虚拟或相对URL。 text-description是Web-page-URL的描述文字。 comment为组件不处理时的说明性文字。
2、超链接处理文件:引导用户到相应的广告网页 3、显示广告图片文件:放置广告图片的文件
8.2.1 Ad Rotator组件的属性和方法 1.Ad Rotator组件的属性
属性名 语法 描述
Border
Border = size Clickable = value TargetFra me=frame
组件并不能真正检测浏览器的功能,其检测的依 据是User Agent标题和Browscap.ini文件中的定义。如 果 Browscap.ini 文 件 的 内 容 发 生 变 化 , Browser Capabilities组件报告的浏览器能力也会相应发生变化。 Browser Capabilities组件除包含一系列属性、方法 外,还有一个外部驱动文件Browscap.ini文件。语法如 下: Set Browser类型变量 =Server.CreateObject("MSWC.BrowserType")
8.3.2 Browser Capabilities组件的使用 首先要创建一个Browser Capabilities的组件 实例。 然后再通过该组件实例判断浏览器的类型及 相关特性。
8.4 Content Linking组件
Content Linking组件的主要目的在于管理网 页或网址间的超文本链接,可以通过一个网页 或网址的线性排列顺序列表来管理多个网页或 网址间的超文本链接顺序。 Content Linking组可创建一个管理URL列 表的Nextlink对象,其语法如下: