使用boost中的filesystem类库遍历某个目录所有的文件
filesystem库用法

filesystem库用法文件系统库(filesystem library)是C++17新增的标准库之一,用于简化和统一文件和目录的操作。
使用filesystem库的一般步骤如下:1. 包含头文件:`#include <filesystem>`2. 命名空间:`using namespace std::filesystem;`3. 使用库中的功能,例如:- 创建目录:`create_directory(path);`- 删除目录或文件:`remove(path);`- 拷贝或移动目录或文件:`copy(path1, path2);` 或`rename(path1, path2);`- 获取文件大小:`file_size(path);`- 判断路径是否存在:`exists(path);`- 判断是否为目录:`is_directory(path);`- 遍历目录中的文件和子目录:使用迭代器或递归函数等。
以下是一个简单的示例代码,演示了filesystem库的基本用法:```cpp#include <iostream>#include <filesystem>using namespace std::filesystem;int main() {path filePath = "path/to/file.txt";if (exists(filePath)) {if (is_directory(filePath)) {std::cout << "Path is a directory." << std::endl;} else {std::cout << "Path is a file." << std::endl;std::cout << "Size: " << file_size(filePath) << " bytes." << std::endl;}} else {std::cout << "Path does not exist." << std::endl;}return 0;}```上述代码会判断给定的路径是否存在,并输出路径类型(文件还是目录)和文件大小(如果是文件的话)。
C++使用BOOST操作文件、目录
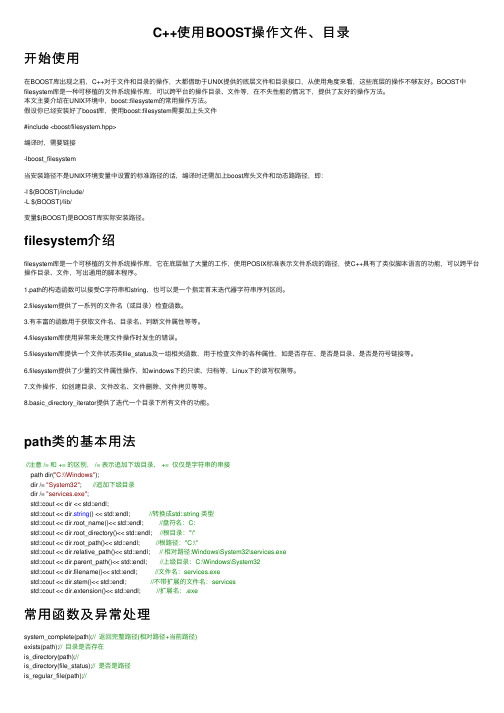
C++使⽤BOOST操作⽂件、⽬录开始使⽤在BOOST库出现之前,C++对于⽂件和⽬录的操作,⼤都借助于UNIX提供的底层⽂件和⽬录接⼝,从使⽤⾓度来看,这些底层的操作不够友好。
BOOST中filesystem库是⼀种可移植的⽂件系统操作库,可以跨平台的操作⽬录、⽂件等,在不失性能的情况下,提供了友好的操作⽅法。
本⽂主要介绍在UNIX环境中,boost::filesystem的常⽤操作⽅法。
假设你已经安装好了boost库,使⽤boost::filesystem需要加上头⽂件#include <boost/filesystem.hpp>编译时,需要链接-lboost_filesystem当安装路径不是UNIX环境变量中设置的标准路径的话,编译时还需加上boost库头⽂件和动态路路径,即:-I $(BOOST)/include/-L $(BOOST)/lib/变量$(BOOST)是BOOST库实际安装路径。
filesystem介绍filesystem库是⼀个可移植的⽂件系统操作库,它在底层做了⼤量的⼯作,使⽤POSIX标准表⽰⽂件系统的路径,使C++具有了类似脚本语⾔的功能,可以跨平台操作⽬录、⽂件,写出通⽤的脚本程序。
1.path的构造函数可以接受C字符串和string,也可以是⼀个指定⾸末迭代器字符串序列区间。
2.filesystem提供了⼀系列的⽂件名(或⽬录)检查函数。
3.有丰富的函数⽤于获取⽂件名、⽬录名、判断⽂件属性等等。
4.filesystem库使⽤异常来处理⽂件操作时发⽣的错误。
5.filesystem库提供⼀个⽂件状态类file_status及⼀组相关函数,⽤于检查⽂件的各种属性,如是否存在、是否是⽬录、是否是符号链接等。
6.filesystem提供了少量的⽂件属性操作,如windows下的只读、归档等,Linux下的读写权限等。
7.⽂件操作,如创建⽬录、⽂件改名、⽂件删除、⽂件拷贝等等。
boost::filesystem文件操作
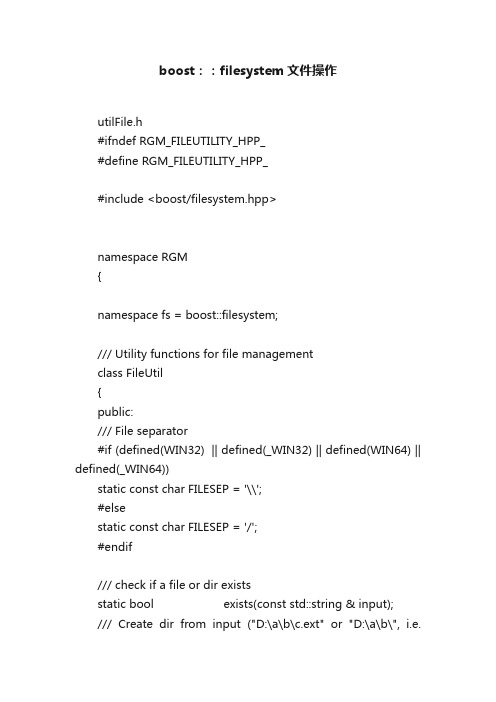
boost::filesystem文件操作utilFile.h#ifndef RGM_FILEUTILITY_HPP_#define RGM_FILEUTILITY_HPP_#include <boost/filesystem.hpp>namespace RGM{namespace fs = boost::filesystem;/// Utility functions for file managementclass FileUtil{public:/// File separator#if (defined(WIN32) || defined(_WIN32) || defined(WIN64) || defined(_WIN64))static const char FILESEP = '\\';#elsestatic const char FILESEP = '/';#endif/// check if a file or dir existsstatic bool exists(const std::string & input);/// Create dir from input ("D:\a\b\c.ext" or "D:\a\b\", i.e."D:\a\b")static void CreateDir( const std::string& input );/// Get (dir, filebasename, extname) from a full file namestatic std::vector<std::string> FileParts(const std::string& fileName);static std::string GetParentDir(const std::string& filePath);static std::string GetFileExtension(const std::string& filePath);static std::string GetFileBaseName(const std::string& filePath);/// Check if a file already existedstatic bool CheckFileExists(const std::string &fileName);/// Check if a dir existed, if not, create itstatic bool VerifyDirectoryExists(const std::string& directoryName, bool create=true);/// Delete all files under a dirstatic void ClearDirectory(const std::string& directoryName);/// Get all files under a dirstatic void GetFileList(std::vector<std::string>& files,const std::string& path,const std::string& ext, bool useRootDir=false);/// Get current work directorystatic std::string GetCurrentWorkDirectory();/// Add the file separator to filePath if necessarystatic void VerifyTheLastFileSep(std::string&filePath);}; // class FileUtil} // namespace RGM#endif // RGM_FILEUTILITY_HPP_utilFile.cpp#include <boost/foreach.hpp>#include "UtilFile.hpp"namespace RGM{bool FileUtil::exists(const std::string & input){return fs::exists(fs::path(input));}void FileUtil::CreateDir( const std::string& input ) {fs::path p(input.c_str());if (!fs::is_directory(p)) {fs::path pp = p.parent_path();fs::create_directories(pp);}}std::vector<std::string> FileUtil::FileParts(const std::string& fileName)//分离文件名{fs::path p( fileName.c_str() );std::vector<std::string> vstr(3);vstr[0] = p.parent_path().string();vstr[1] = p.stem().string();vstr[2] = p.extension().string();return vstr;}std::string FileUtil::GetParentDir(const std::string& filePath) {return fs::path(filePath.c_str()).parent_path().string();}std::string FileUtil::GetFileExtension(const std::string& filePath){return fs::path(filePath.c_str()).extension().string();}std::string FileUtil::GetFileBaseName(const std::string& filePath){return fs::path(filePath.c_str()).stem().string();}bool FileUtil::CheckFileExists(const std::string& fileName){return fs::exists(fs::path(fileName.c_str()));}bool FileUtil::VerifyDirectoryExists(const std::string& directoryName, bool create){fs::path p(directoryName.c_str());bool exist = fs::exists(p);if (!exist && create) {fs::create_directories(p);}return exist;}void FileUtil::ClearDirectory(const std::string& directoryName){fs::path p(directoryName.c_str());fs::remove_all( p );fs::create_directory( p );}//获取指定目录下所有文件void FileUtil::GetFileList(std::vector<std::string>& files, const std::string& path, const std::string& ext, bool useRootDir) {fs::path targetDir(path.c_str());fs::directory_iterator it(targetDir), eod;bool getAllFiles = (pare("*")==0);BOOST_FOREACH(fs::path const &p, std::make_pair(it, eod)) { if (fs::is_regular_file(p)) {if ( getAllFiles ) {useRootDir ? files.push_back( p.string() ) : files.push_back(p.filename().string());} else {if (pare(p.extension().string())==0) {useRootDir ? files.push_back( p.string() ) : files.push_back(p.filename().string());}}}}}//获取工作目录std::string FileUtil::GetCurrentWorkDirectory(){fs::path p = fs::initial_path();return p.string();}//在文件的结尾加上\\void FileUtil::VerifyTheLastFileSep(std::string &filePath) {if ( filePath.find_last_of("/\\") != (filePath.length() - 1) ) { filePath = filePath + FILESEP;}}} // namespace RGM。
boost::filesystem总结

boost::filesystem总结boost::filesystem是Boost C++ Libraries中的⼀个模块,主要作⽤是处理⽂件(Files)和⽬录(Directories)。
该模块提供的类boost::filesystem::path专门⽤来处理路径。
⽽且,该模块中还有很多独⽴的函数能够⽤来执⾏创建⽬录、检查⽂件是否存在等任务。
⼀、创建Paths定义路径时需要包含头⽂件boost/filesystem.hpp,并且使⽤命名空间boost::filesystem;路径的创建很简单,仅仅需要向类boost::filesystem::path()的构造器传递⼀个string;构造器的输⼊可以是⼀个没有意义的字符串,因为构造器不会去检测该路径是否是合法路径及是否存在;path对象是⼀个跨平台的路径对象。
path对象的属性有下列这些:1) path.string() 输出字符串形式的路径2) path.stem() ⽂件名,不带扩展名3) path.extension() 返回⽂件扩展名更详细的内容见。
⼆、⽂件和⽬录该部分包括下列函数:boost::filesystem::status(path) 查询⽂件或⽬录的状态,返回的是boost::filesystem::file_status类型的对象boost::filesystem::is_directory() 根据获取的状态判断是否是⽬录,返回boolboost::filesystem::is_empty() 判断是否为空boost::filesystem::is_regular_file() 根据获取的状态判断是否是普通⽂件,返回boolboost::filesystem::is_symlink() 判断符号连接(在windows系统中,后缀为lnk的⽂件为连接⽂件)boost::filesystem::exists() 判断是否存在boost::filesystem::file_size() 返回⽂件的size,按bytes计算boost::filesystem::last_write_time() 返回⽂件最后⼀次修改的时间boost::filesystem::space() 返回磁盘的总空间和剩余空间,boost::filesystem::create_directory() 创建⽬录boost::filesystem::create_directories() 递归创建整个⽬录结构boost::filesystem::remove() 删除⽬录boost::filesystem::remove_all() 递归删除整个⽬录结构boost::filesystem::rename() 重命名⽬录boost::filesystem::copy_file() 复制⽂件boost::filesystem::copy_directory() 复制⽬录boost::filesystem::absolute() 获取⽂件或⽬录的绝对路径boost::filesystem::current_path() 如果没有参数传⼊,则返回当前⼯作⽬录;否则,则将传⼊的⽬录设为当前⼯作⽬录三、⽬录迭代(Directory Iterators)boost::filesystem::directory_iterator() 迭代⽬录下的所有⽂件boost::filesystem::recursive_directory_iterator() 递归地遍历⼀个⽬录和⼦⽬录,也就是迭代整个⽬录结构下的所有⽂件四、⽂件流(File Streams)头⽂件<fstream>定义的⽂件流不能将boost::filesystem::path定义的⽬录作为参数。
c++读取整个文件内容的最简洁高效写法
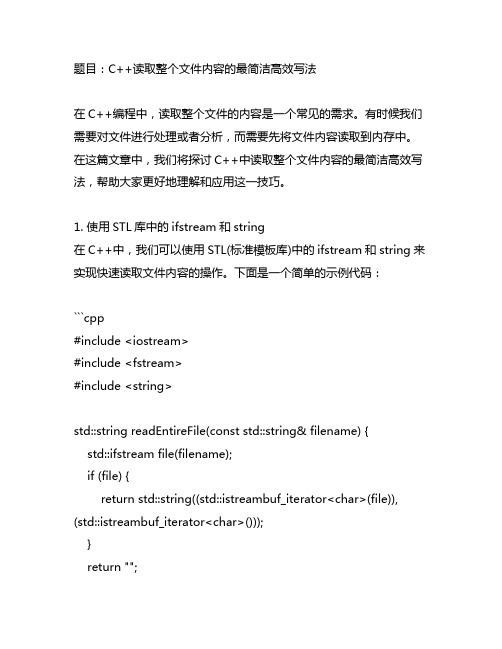
题目:C++读取整个文件内容的最简洁高效写法在C++编程中,读取整个文件的内容是一个常见的需求。
有时候我们需要对文件进行处理或者分析,而需要先将文件内容读取到内存中。
在这篇文章中,我们将探讨C++中读取整个文件内容的最简洁高效写法,帮助大家更好地理解和应用这一技巧。
1. 使用STL库中的ifstream和string在C++中,我们可以使用STL(标准模板库)中的ifstream和string来实现快速读取文件内容的操作。
下面是一个简单的示例代码:```cpp#include <iostream>#include <fstream>#include <string>std::string readEntireFile(const std::string& filename) {std::ifstream file(filename);if (file) {return std::string((std::istreambuf_iterator<char>(file)), (std::istreambuf_iterator<char>()));}return "";}int main() {std::string content = readEntireFile("example.txt");std::cout << content << std::endl;return 0;}```在这段代码中,我们首先包含了iostream、fstream和string这三个头文件。
然后定义了一个读取整个文件内容的函数readEntireFile,以及在main函数中调用该函数并输出文件内容的示例。
这段代码的关键点是使用了std::ifstream来打开文件,然后利用std::istreambuf_iterator<char>构造了一个string对象,从而将文件内容读取到了内存中。
boost::filesystem经常使用使用方法具体解释

boost::filesystem经常使⽤使⽤⽅法具体解释提⽰:filesystem库提供了两个头⽂件,⼀个是<boost/filesystem.hpp>,这个头⽂件包括基本的库内容。
它提供了对⽂件系统的重要操作。
同⼀时候它定义了⼀个类path。
正如⼤家所想的。
这个是⼀个可移植的路径表⽰⽅法,它是filesystem库的基础。
⼀个是<boost/filesystem/fstream.hpp>。
是对std::fstream的⼀个补充,使⽤能够使⽤类boost::path作为參数。
从⽽使得filesystem库与标准库的关系更亲热。
由于⽂件系统对于⼤多数系统来说都是共享的,所以不同的进程能够同⼀时候操作同⼀个对象,因此filesysetm不提供这⽅⾯的特性保证。
当然这样的保证也是不可能的。
或者⾄少昂贵的。
filesystem在不论什么时候,仅仅要不能完毕对应的任务。
它都可能抛出 basic_filesystem_error异常。
当然并不是总会抛出异常。
由于在库编译的时候能够关闭这个功能。
同⼀时候有两个函数提供了⽆异常版本号。
这是由于在任务不能完毕时并不是是异常。
filesystem库的全部内容定义在boost名字空间的⼀个下级名字空间⾥,它叫boost::filesytem。
在使⽤boost.filesytem之后,链接时须要加“-lboost_filesystem-mt”选项,由于这个须要额外的链接,并不是⼀个纯头⽂件的库。
本⽂中所⽤boost库为1_54#include<boost/filesystem.hpp>{boost::filesystem::path path("/test/test1"); //初始化boost::filesystem::path old_cpath = boost::filesystem::current_path(); //取得当前程序所在⽂件夹boost::filesystem::path parent_path = old_cpath.parent_path();//取old_cpath的上⼀层⽗⽂件夹路径boost::filesystem::path file_path = old_cpath / "file"; //path⽀持重载/运算符if(boost::filesystem::exists(file_path)) //推断⽂件存在性{std::string strPath = file_path.string();int x = 1;}else{//⽂件夹不存在;boost::filesystem::create_directory(file_path); //⽂件夹不存在。
boost常用库的使用介绍第一讲

boost常用库的使用介绍第一讲[object Object]Boost是一个C++库集合,包含了许多常用的工具和组件,用于增强C++的功能和性能。
Boost库广泛应用于各种领域,如网络编程、多线程、数据结构、算法等。
Boost库的使用可以大大简化C++开发过程,提高开发效率。
下面是一些常用的Boost库和它们的使用介绍:1. Boost.Filesystem:用于处理文件和目录的库。
它提供了一组易于使用和跨平台的API,可以进行文件和目录的创建、删除、移动、复制等操作。
2. Boost.Regex:正则表达式库,提供了强大的正则表达式功能,可以进行字符串匹配、替换等操作。
Boost.Regex支持多种正则表达式语法,包括Perl、ECMAScript等。
3. Boost.Thread:多线程库,提供了线程的创建、同步、互斥等功能。
Boost.Thread可以简化多线程编程,提高程序的并发性能。
4. Boost.Asio:网络编程库,提供了异步网络编程的功能。
它支持TCP、UDP、SSL等协议,可以用于开发高性能的网络应用程序。
5. Boost.SmartPtr:智能指针库,提供了shared_ptr、weak_ptr等智能指针类,用于管理动态分配的内存。
使用智能指针可以避免内存泄漏和悬挂指针等问题。
6. Boost.Algorithm:算法库,提供了一系列常用的算法,如排序、查找、字符串处理等。
Boost.Algorithm可以方便地进行各种数据处理操作。
7. Boost.Date_Time:日期和时间库,提供了日期和时间的表示、计算和格式化等功能。
它支持多种日期和时间表示方式,如Gregorian、Julian等。
8. Boost.Serialization:序列化库,用于将对象转换成字节流或从字节流中恢复对象。
Boost.Serialization可以方便地进行对象的序列化和反序列化操作。
Visual_C++_MFC文件操作大全

{
dwAttrs = GetFileAttributes(FileData.cFileName);
if (!(dwAttrs & FILE_ATTRIBUTE_READONLY))
fFinished = TRUE;
{
SetFileAttributes(szNewPath,
dwAttrs | FILE_ATTRIBUTE_READONLY);
}
return;
}
CString path;
path.Format("%s\\*.*",%%1);
BOOL bWorking = finder.FindFile(path);
while (bWorking)
{
bWorking = finder.FindNextFile();
c:\\usefile\\usefile.ini);
}
strTemp.Format("%d",nCount);
::WritePrivateProfileString("FileCount","Count",strTemp,"c:\\usefile\\usefile.ini");
//将文件总数写入,以便读出.
if (finder.IsDirectory())
{
RemoveDirectory(finder.GetFilePath());
}
}
6.清空文件夹
RemoveDirectory(%%1);
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
使用boost中的filesystem类库遍历某个目录所有的文件
#include <boost/filesystem/operations.hpp>
#include <boost/filesystem/path.hpp>
使用boost中的filesystem类库遍历某个目录所有的文件
int GetAllFileOfPath(const string strPath)
{
namespace fs = boost::filesystem;
// 得到配置文件夹.
if ( strPath.size() < 2 )
{
return 0;
}
fs::path full_path( fs::initial_path() );
full_path = fs::system_complete( fs::path( strPath, fs::native ) );
unsigned long file_count = 0;
unsigned long dir_count = 0;
unsigned long err_count = 0;
if ( !fs::exists( full_path ) )
{
std::cout << "找不到配置文件目录,请检查该目录是否存在:"
std::cout << full_path.native_file_string() << std::endl;
return -1;
}
// 遍历配置文件所在的文件夹,得到所有的配置文件名.
if ( fs::is_directory( full_path ) )
{
fs::directory_iterator end_iter;
for ( fs::directory_iterator dir_itr( full_path );
dir_itr != end_iter;
++dir_itr )
{
try
{
if ( fs::is_directory( *dir_itr ) )
{
string strSubDir(full_path.native_directory_string()) ;
strSubDir.append("\\");
strSubDir.append(dir_itr->leaf());
// 如果有子目录,则递归遍历.
GetAllFileOfPath(strSubDir);
}
else
{
// 先组成包括完整路径的文件名
string strFileName(full_path.native_directory_string());
strFileName.append("\\");
strFileName.append(dir_itr->leaf());
fs::path full_file( fs::initial_path() );
full_file = fs::system_complete(fs::path(strFileName, fs::native));
// 加载解析文件中的信息.
//do something;
}
}
catch ( const std::exception & ex )
{
++err_count;
string strMsg = dir_itr->leaf();
strMsg.append(",出现错误:");
strMsg.append(ex.what());
std::cout << strMsg.c_str() << std::endl;
}
}//<--for()
}
else // must be a file
{
string strMsg = full_path.native_file_string();
strMsg.append(",不是文件目录.");
std::cout << strMsg.c_str() << std::endl;
return -1;
}
return err_count;
}。