操作系统实验--进程调度和内存管理---java语言版本
进程调度程序实验报告

一、实验目的通过本次实验,加深对进程调度原理和算法的理解,掌握进程调度程序的设计与实现方法。
实验要求我们使用高级编程语言编写一个简单的进程调度程序,实现不同调度算法的模拟,并通过实验验证算法的性能。
二、实验环境1. 操作系统:Windows 102. 编程语言:Java3. 开发工具:IntelliJ IDEA三、实验内容本次实验主要实现以下调度算法:1. 先来先服务(FCFS)2. 最短作业优先(SJF)3. 时间片轮转(RR)四、实验步骤1. 定义进程类(Process):```javapublic class Process {private String processName; // 进程名称private int arrivalTime; // 到达时间private int burstTime; // 运行时间private int waitingTime; // 等待时间private int turnaroundTime; // 周转时间// 构造函数public Process(String processName, int arrivalTime, int burstTime) {this.processName = processName;this.arrivalTime = arrivalTime;this.burstTime = burstTime;}// 省略getter和setter方法}```2. 定义调度器类(Scheduler):```javapublic class Scheduler {private List<Process> processes; // 进程列表private int currentTime; // 当前时间// 构造函数public Scheduler(List<Process> processes) {this.processes = processes;this.currentTime = 0;}// FCFS调度算法public void fcfs() {for (Process process : processes) {process.setWaitingTime(currentTime -process.getArrivalTime());currentTime += process.getBurstTime();process.setTurnaroundTime(currentTime -process.getArrivalTime());}}// SJF调度算法public void sjf() {processes.sort((p1, p2) -> p1.getBurstTime() -p2.getBurstTime());for (Process process : processes) {process.setWaitingTime(currentTime -process.getArrivalTime());currentTime += process.getBurstTime();process.setTurnaroundTime(currentTime -process.getArrivalTime());}}// RR调度算法public void rr(int quantum) {List<Process> sortedProcesses = new ArrayList<>(processes);sortedProcesses.sort((p1, p2) -> p1.getArrivalTime() -p2.getArrivalTime());int timeSlice = quantum;for (Process process : sortedProcesses) {if (process.getBurstTime() > timeSlice) {process.setWaitingTime(currentTime - process.getArrivalTime());currentTime += timeSlice;process.setTurnaroundTime(currentTime - process.getArrivalTime());process.setBurstTime(process.getBurstTime() - timeSlice);} else {process.setWaitingTime(currentTime - process.getArrivalTime());currentTime += process.getBurstTime();process.setTurnaroundTime(currentTime - process.getArrivalTime());process.setBurstTime(0);}}}}```3. 测试调度程序:```javapublic class Main {public static void main(String[] args) {List<Process> processes = new ArrayList<>();processes.add(new Process("P1", 0, 5));processes.add(new Process("P3", 4, 2));processes.add(new Process("P4", 6, 4));Scheduler scheduler = new Scheduler(processes); System.out.println("FCFS调度结果:");scheduler.fcfs();for (Process process : processes) {System.out.println(process);}processes = new ArrayList<>();processes.add(new Process("P1", 0, 5));processes.add(new Process("P2", 1, 3));processes.add(new Process("P3", 4, 2));processes.add(new Process("P4", 6, 4));System.out.println("SJF调度结果:");scheduler.sjf();for (Process process : processes) {System.out.println(process);}processes = new ArrayList<>();processes.add(new Process("P1", 0, 5));processes.add(new Process("P2", 1, 3));processes.add(new Process("P3", 4, 2));System.out.println("RR调度结果(时间片为2):");scheduler.rr(2);for (Process process : processes) {System.out.println(process);}}}```五、实验结果与分析通过实验,我们可以观察到以下结果:1. FCFS调度算法简单,但可能导致长作业等待时间过长。
操作系统实验报告进程调度
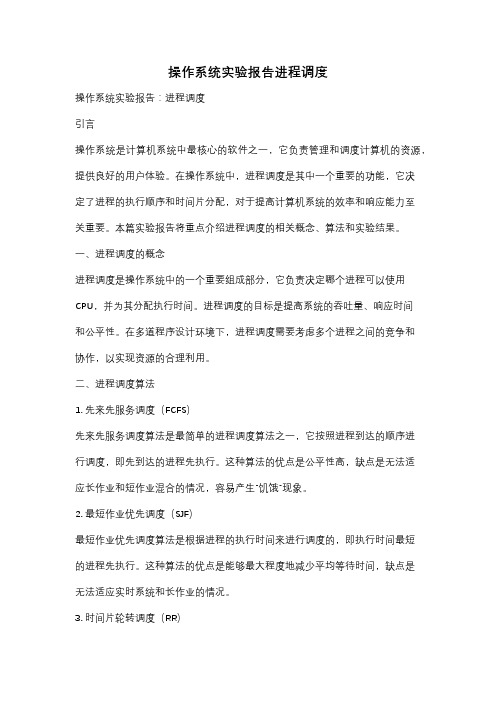
操作系统实验报告进程调度操作系统实验报告:进程调度引言操作系统是计算机系统中最核心的软件之一,它负责管理和调度计算机的资源,提供良好的用户体验。
在操作系统中,进程调度是其中一个重要的功能,它决定了进程的执行顺序和时间片分配,对于提高计算机系统的效率和响应能力至关重要。
本篇实验报告将重点介绍进程调度的相关概念、算法和实验结果。
一、进程调度的概念进程调度是操作系统中的一个重要组成部分,它负责决定哪个进程可以使用CPU,并为其分配执行时间。
进程调度的目标是提高系统的吞吐量、响应时间和公平性。
在多道程序设计环境下,进程调度需要考虑多个进程之间的竞争和协作,以实现资源的合理利用。
二、进程调度算法1. 先来先服务调度(FCFS)先来先服务调度算法是最简单的进程调度算法之一,它按照进程到达的顺序进行调度,即先到达的进程先执行。
这种算法的优点是公平性高,缺点是无法适应长作业和短作业混合的情况,容易产生"饥饿"现象。
2. 最短作业优先调度(SJF)最短作业优先调度算法是根据进程的执行时间来进行调度的,即执行时间最短的进程先执行。
这种算法的优点是能够最大程度地减少平均等待时间,缺点是无法适应实时系统和长作业的情况。
3. 时间片轮转调度(RR)时间片轮转调度算法是一种抢占式调度算法,它将CPU的执行时间划分为固定大小的时间片,并按照轮转的方式分配给各个进程。
当一个进程的时间片用完后,它将被挂起,等待下一次调度。
这种算法的优点是能够保证每个进程都能够获得一定的执行时间,缺点是无法适应长作业和短作业混合的情况。
4. 优先级调度(Priority Scheduling)优先级调度算法是根据进程的优先级来进行调度的,优先级高的进程先执行。
这种算法的优点是能够根据进程的重要性和紧急程度进行灵活调度,缺点是可能会导致低优先级的进程长时间等待。
三、实验结果与分析在实验中,我们使用了不同的进程调度算法,并对其进行了性能测试。
操作系统进程调度模拟课程设计(java)
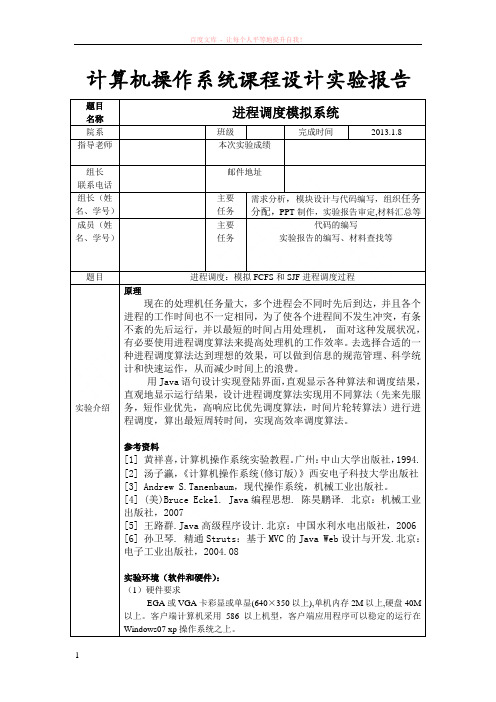
计算机操作系统课程设计实验报告题目名称进程调度模拟系统院系班级完成时间2013.1.8 指导老师本次实验成绩组长联系电话邮件地址组长(姓名、学号)主要任务需求分析,模块设计与代码编写,组织任务分配,PPT制作,实验报告审定,材料汇总等成员(姓名、学号)主要任务代码的编写实验报告的编写、材料查找等题目进程调度:模拟FCFS和SJF进程调度过程实验介绍原理现在的处理机任务量大,多个进程会不同时先后到达,并且各个进程的工作时间也不一定相同,为了使各个进程间不发生冲突,有条不紊的先后运行,并以最短的时间占用处理机,面对这种发展状况,有必要使用进程调度算法来提高处理机的工作效率。
去选择合适的一种进程调度算法达到理想的效果,可以做到信息的规范管理、科学统计和快速运作,从而减少时间上的浪费。
用Java语句设计实现登陆界面,直观显示各种算法和调度结果,直观地显示运行结果,设计进程调度算法实现用不同算法(先来先服务,短作业优先,高响应比优先调度算法,时间片轮转算法)进行进程调度,算出最短周转时间,实现高效率调度算法。
参考资料[1] 黄祥喜,计算机操作系统实验教程。
广州:中山大学出版社,1994.[2] 汤子瀛,《计算机操作系统(修订版)》西安电子科技大学出版社[3] Andrew S.Tanenbaum,现代操作系统,机械工业出版社。
[4] (美)Bruce Eckel. Java编程思想. 陈昊鹏译. 北京:机械工业出版社,2007[5] 王路群.Java高级程序设计.北京:中国水利水电出版社,2006[6] 孙卫琴. 精通Struts:基于MVC的Java Web设计与开发.北京:电子工业出版社,2004.08实验环境(软件和硬件):(1)硬件要求EGA或VGA卡彩显或单显(640×350以上),单机内存2M以上,硬盘40M 以上。
客户端计算机采用586以上机型,客户端应用程序可以稳定的运行在Windows07 xp操作系统之上。
《操作系统》上机实验报告—进程调度
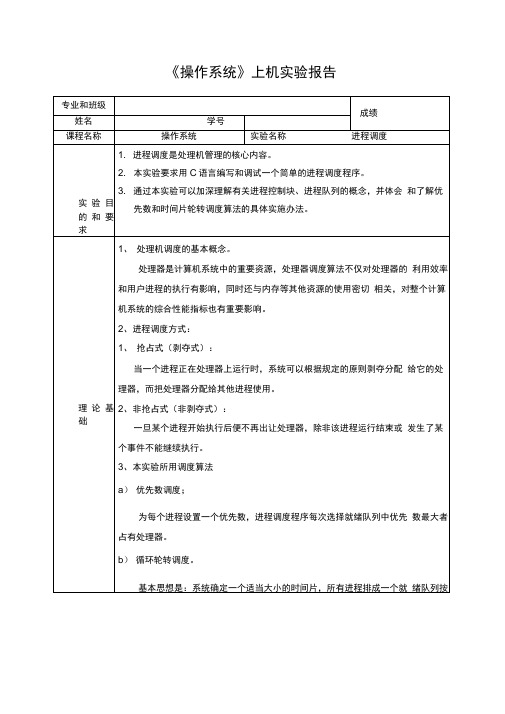
《操作系统》上机实验报告实验算法主体内容及#include<stdio.h>#include<dos.h>#include<stdlib.h>#include<conio.h>#include<iostream.h>#define P_NUM 5 // 共有5 个进程#define P_TIME 50 //作为优先数计算时所用的值enum state{ready,execute,block, finish};//进程的状态,使用枚举struct pcb{char name[4]; // 进程名称int priority; //进程优先级int cputime; //已经占有cpu运行的时间int needtime; //还需要运行的时间int count; //在时间片轮转法中使用的int round; //在时间片轮转法中使用的state process; //进程的状态pcb *next; //指向下一个进程的pcb};pcb *get_process() //通过输入各进程的值来建立pcb队列,并返回其首元素的指针{pcb *q;pcb *t;pcb *p;int i=0;coutvv"请输入进程名与时间"<<endl;while(i<P_NUM){q=(struct pcb *)malloc(sizeof(pcb));cin>>q->name; cin>>q->needtime; q->cputime=O;q->priority=P_TIME-q->needtime;q->round=0; q->count=0;q->process=ready;q->next=NULL;if (i==0){p=q;t=q;}else{ t->next=q; t=q; } i++;}return p;}void display(pcb *p) //显示本轮运行后的进程各状态情况{coutvv" 进程各状态情况"vvendl;coutvv"名称"vv" "vv"进入时间"vv" "vv"还需时间"vv" "vv"优先级"vv" "vv"状态"vvendl;while(p){coutvvp->name;coutvv" ";coutvvp->cputime;coutvv" ";coutvvp->needtime;coutvv" ";cout<vp_>priority;coutvv"";switch(p->process) //对枚举类型的输出方法{case ready:cout< <"就绪"<<endl;break;case execute:cout< <"执行"<<endl;break;case block:cout<<"阻塞"<<endl;break;case finish:cout<<"完成"<<endl;break;} p=p->next;}}int process_finish(pcb *q) //判断所有的进程是否运行结束{ -int b=1;while(q&&b){b=b&&q->needtime==O; q=q_>next;} return b;}void cpuexe(pcb *q) //优先级调度算法的一次执行{pcb *t;t=q;int i=0;while(q){if (q->process!=finish){q_>process=ready;if(q->needtime==0) q->process=finish;} if(i<q->priority) if(q->process!=finish){ t=q;i=q->priority;} q=q->next;}t->needtime-=1;t_>priority_=3; if(t->needtime==0)t->process=finish; t->cputime+=1;}void priority_cal() //优先级调度算法{pcb *p;P=get_process();〃取得进程队列int cpu=0;while(!process_finish(p)) //若进程并未全部结束,则还需要执行{cpu++;coutvv"运行次数:"vvcpuwendl; cpuexe(p);//一次cpu的执行display(p);//显示本次执行结果}}pcb * get_process_round()Jpcb *q;pcb *t;pcb *p;int i=0;coutvv"请输入进程名与时间"《endl;while (i<P_NUM){q=(struct pcb *)malloc(sizeof(pcb));cin>>q->name;cin>>q->needtime; q->cputime=O;q->round=0;q->count=0;q_>process=ready; q->next=NULL; if(i==0){p=q;t=q;}else{} i++; } return p;} t->next=q; t=q;void cpu_round(pcb *q){-q->count++;q->cputime+=2;q->needtime-=2;if(q->needtime<0)q->needtime=0;q->round++;q->process=execute;}pcb *get_next(pcb *k,pcb *head){ -pcb *t;t=k; do{t=t->next;}while (t && t->process==finish);if(t==NULL){} return t; t=head;while (t->next!=k && t->process==finish) {t=t->next;}void set_state(pcb *p){-while(p){if (p->needtime==O){p->process=finish;}if (p->process==execute){p_>process=ready;} p=p_>next;}}void display_round(pcb *p){ -cout«" 进程各状态情况"vvendl;coutvv"名称"vv" "vv"进入时间"vv" "vv"还需时间"vv" "vv"时间片"vv"" vv"次数"vv""vv"状态"vvendl;while(p){coutvvp->name;coutvv" ";coutvvp->cputime;coutvv" ";coutvvp->needtime;coutvv" ";coutvvp->round;coutvv"";coutvvp->count;coutvv"";switch(p->process){case ready:coutv v"就绪"vvendl;break;case execute:coutvv'执行"vvendl;break;case finish:coutvv"完成"vvendl;break;}p=p->next;}}void round_cal(){pcb *p;pcb *r;p=get_process();int cpu=0;r=p;while(!process_finish(p)){cpu+=2;cpu_round(r);r=get_next(r,p);coutvv"运行次数"vvcpuvvendl;display_round(p);set_state(p);} }-void display_menu(){ -coutvv"进程调度算法操作:"vvendl;coutvv"1 优先数"vvendl;coutvv"2 时间片轮转"vvendl;coutvv"3 退出"vvendl;}void main(){display_menu();int k;printf("请选择:");scanf("%d",&k);switch(k){case 1:priority_cal();break;case 2:round_cal();break;case 3:break;}} ----------------------------------------------------------------------------------------------------------测试数据:¥间出择1A.时退选r 5642 3込簷运行结果:1优先数S却曰石石<奪--a S 亠 亡疋出尢尤扫 亡、 ^a ^T B a 抄各时 各时 进还进还称进入时|可0 3 0I! IS 运行次数 “称进入时间II态成養成成忧完就完完完&0 94 2R p f c 32 3 4 3 % 扰冋运行次数心 泊称进入吋冋R5 R 5 C4 卜2佳行次数陰态成成成成成状§_f c s ^H Z B6 4 28尸尤32 3 4结果截图与分析2、时间片轮转10 0名称进入时问64 42 运行次数t k 称进入吋间A称进入时间竇鶴躺翻聶s _^->4p 者者者奁廿者_J-^□者者HiH8 数 謝还轎時 0 00 0 0次数0 口2 1 21 2 3 3216 6 42 2 1 20 Q 0D F次数3 E34 4 1 1 e s 02 0 0态成成态成衣成成些兀执完lla兀。
操作系统进程调度实验报告

《计算机操作系统》课程实验报告题目实验一进程调度学院: 计算机学院专业: 计算机科学与技术姓名班级学号2015年10月21日实验一进程调度1.实验目的:通过对进程调度算法的模拟,进一步理解进程的基本概念,加深对进程运行状态和进程调度过程、调度算法的理解。
2.实验内容:用C语言实现对N个进程采用某种进程调度算法先来先服务调度、短作业优先调度的调度。
3.设计实现:要求给出设计源码,设计源码要有详细注释,#include <stdio.h>#include<iostream>using namespace std;struct program{char name; /*进程名*/int atime; /*到达时间*/int stime; /*服务时间*/int ftime; /*完成时间*/int rtime; /*周转时间*/float qrtime; /*带权周转时间*/};void xianshi(struct program a[],int n){int i,j;struct program t;/*将进程按时间排序*/printf("根据到达时间重新排序:\n");printf("*****进程*************到达时间***************服务时间*****\n");for(j=0;j<n-1;j++)for(i=0;i<n-1-j;i++)if(a[i].atime>a[i+1].atime){t.atime=a[i].atime;a[i].atime=a[i+1].atime;a[i+1].atime=t.atime;=a[i].name;a[i].name=a[i+1].name;a[i+1].name=;t.stime=a[i].stime;a[i].stime=a[i+1].stime;a[i+1].stime=t.stime;}for(i=0;i<n;i++)printf(" %c %d %d |\n",a[i].name,a[i].atime,a[i].stime);printf("----------------------------------------------------\n"); }void fcfs(struct program a[],int n){int i;int time=0;for(i=0;i<n;i++){time=time+a[i].stime;a[i].ftime=a[0].atime+time;a[i].rtime=a[i].ftime-a[i].atime;a[i].qrtime=(float)a[i].rtime/a[i].stime;}printf("\nFCFS算法:\n");printf("*****进程****到达时间****完成时间******周转时间*******带权周转时间*****\n");for(i=0;i<n;i++){printf(" %c %d %.2d %.2d %.2f |\n",a[i].name,a[i].atime,a[i].ftime,a[i].rtime,a[i].qrtime);}printf("-----------------------------------------------------------------------\n");}void main(){int i,m;struct program pro[4];/*创建进程 */printf(" ******先来先服务算法****** \n");printf("请输入进程的数目:\n");scanf("%d",&m);i=m;for(i=0;i<m;i++){printf("请输入进程%d的进程名,到达时间,服务时间\n",i+1);cin>>pro[i].name>>pro[i].atime>>pro[i].stime;}xianshi(pro,m);fcfs(pro,m);getchar();}#include <stdio.h>#include<iostream>using namespace std;struct program{char name; /*进程名*/float atime; /*到达时间*/float stime; /*服务时间*/float ftime; /*完成时间*/float rtime; /*周转时间*/float qrtime; /*带权周转时间*/};void xianshi(struct program a[],int n){int i,j;struct program t;/*将进程按时间排序*/printf("重新排序:\n");printf("*****进程*************到达时间***************服务时间*****\n");for(j=0;j<n-1;j++)for(i=1;i<n-1-j;i++)if(a[i].stime>a[i+1].stime){t.atime=a[i].atime;a[i].atime=a[i+1].atime;a[i+1].atime=t.atime;=a[i].name;a[i].name=a[i+1].name;a[i+1].name=;t.stime=a[i].stime;a[i].stime=a[i+1].stime;a[i+1].stime=t.stime;}for(i=0;i<n;i++)printf(" %c %f %f |\n",a[i].name,a[i].atime,a[i].stime);printf("----------------------------------------------------\n"); }void SJF(struct program a[],int n){int i;a[0].ftime=a[0].atime+a[0].stime;a[0].rtime=a[0].ftime-a[0].atime;a[0].qrtime=a[0].rtime/a[0].stime;for(i=1;i<n;i++){a[i].ftime=a[i-1].ftime+a[i].stime;a[i].rtime=a[i].ftime-a[i].atime;a[i].qrtime=a[i].rtime/a[i].stime;}printf("\nSJF算法:\n");printf("*****进程****到达时间****完成时间******周转时间*******带权周转时间*****\n");for(i=0;i<n;i++){printf(" %c %.2f %.2f %.2f %.2f |\n",a[i].name,a[i].atime,a[i].ftime,a[i].rtime,a[i].qrtime);}printf("-----------------------------------------------------------------------\n");}void main(){int i,m;struct program pro[4];/*创建进程 */printf(" ******短作业优先算法****** \n");printf("请输入进程的数目:\n");scanf("%d",&m);i=m;for(i=0;i<m;i++){printf("请输入进程%d的进程名,到达时间,服务时间\n",i+1);cin>>pro[i].name>>pro[i].atime>>pro[i].stime;}xianshi(pro,m);SJF(pro,m); getchar(); }4.实验结果5.实验过程中出现的问题及解决办法先来先服务调度算法就是根据进程达到的时间为依据,哪一个进程先来那么该进程就会先执行;最短进程优先调度算法则是以每个进程执行所需时间长短为依据,某一个进程执行所需花的时间要短些那么该进程就先执行。
操作系统综合性实验报告-进程调度(含代码)

int count;
// 记录进程执行的次数
struct node *next;
// 队列指针
}PCB;
PCB *ready=NULL,*run=NULL,*finish=NULL; // 和完成队列
定义三个队列, 就绪队列, 执 行队列
int num; void GetFirst(); void Output(); void InsertTime(PCB *in); void InsertFinish(PCB *in); TimeCreate(); void RoundRun(); void main() {
三、实验内容
1. 用 C 语言(或其它语言,如 Java )编程实现对 N 个进程采用某种进程调度算
法(如动态优先权调度算法、先来先服务算法、短进程优先算法、时间片轮转调度
算法)调度执行的模拟。
2. 每个用来标识进程的进程控制块 typedef struct node {
PCB 可用结构来描述,包括以下字段:
一个时间片,当执行完时,有一个计时器发出时钟中断请求,该进程停止,并被送
到就绪队列的末尾,然后再把处理机分配就绪队列的队列进程,同时也让它执行一
个时间片。 ( 3 )、通过亲手实验, 对上述写的时间片的工作流程和原理有了更贴切的认识。
另外本次实验遇到了很大的麻烦,其实大部分代码是借鉴网上的,但自己通过修改, 来获取自己想要的,在自己的努力和同学的帮助下终于调试正确,很是高兴。
void InsertTime(PCB *in)
// 将进程插入到就绪队列尾部
{ PCB *fst; fst = ready;
if(ready == NULL) {
in->next = ready; ready = in; } else { while(fst->next != NULL) {
操作系统实验报告 进程调度
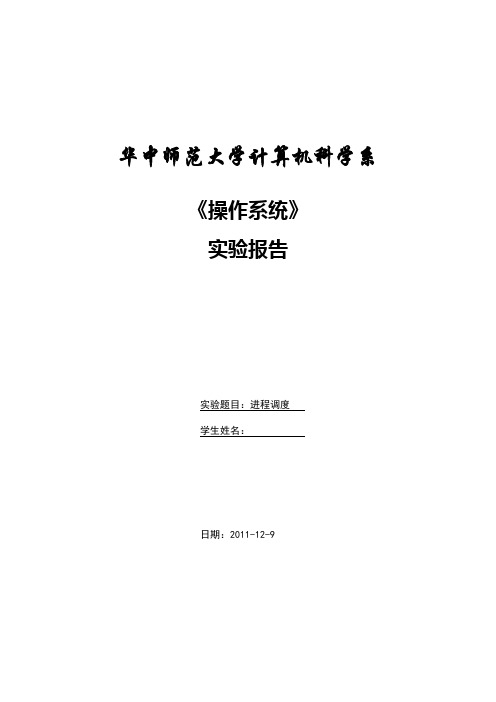
华中师范大学计算机科学系《操作系统》实验报告实验题目:进程调度学生姓名:日期:2011-12-9实验2进程调度【实验目的】(1)通过编写程序实现进程或作业先来先服务、高优先权、按时间片轮转调度算法,使学生进一步掌握进程调度的概念和算法,加深对处理机分配的理解。
(2)了解Windows2000/XP中进程(线程)的调度机制。
(3)学习使用Windows2000/XP中进程(线程)调度算法,掌握相应的与调度有关的Win32 API函数。
【实验内容】在Windows XP、Windows 2000等操作系统下,使用的VC、VB、java或C等编程语言,利用相应的WIN32 API函数,编写程序实现进程或作业先来先服务、高优先权、按时间片轮转调度算法。
【实验步骤、过程】(含原理图、流程图、关键代码,或实验过程中的记录、数据等)1、进程调度算法:采用多级反馈队列调度算法。
其基本思想是:当一个新进程进入内在后,首先将它放入第一个队列的末尾,按FCFS原则排队等待高度。
当轮到该进程执行时,如能在该时间片内完成,便可准备撤离系统;如果它在一个时间片结束时尚为完成,调度程序便将该进程转入第二队列的末尾,再同样地按FCFS原则等待调度执行,以此类推。
2、实验步骤:(1)按先来先服务算法将进程排成就绪队列。
(2)检查所有队列是否为空,若空则退出,否则将队首进程调入执行。
3、流程图:是4、代码:#include<iostream>#include<string>using namespace std;class Process{public:string ProcessName; // 进程名字int Time; // 进程需要时间int leval; // 进程优先级int LeftTime; // 进程运行一段时间后还需要的时间};//////////////////////////////////////////////////////////////////////////////////////////////////void Copy ( Process proc1, Process proc2); // 把proc2赋值给proc1void Sort( Process pr[], int size) ; // 此排序后按优先级从大到小排列void sort1(Process pr[], int size) ; // 此排序后按需要的cpu时间从小到大排列void Fcfs( Process pr[], int num, int Timepice); // 先来先服务算法void TimeTurn( Process process[], int num, int Timepice); // 时间片轮转算法void Priority( Process process[], int num, int Timepice); // 优先级算法//////////////////////////////////////////////////////////////////////////////////////////////////void main(){int TimePice;int num;cout<<" *********创建进程*********\n"<<endl;cout<<" 输入进程个数:";cin>>num;const int Size =30;Process process[Size] ;for( int i=0; i< num; i++){string name;int CpuTime;int Leval;cout<<"\n 输入第"<< i+1<<" 个进程的名字、运行时间和优先级:"<<endl;cin>> name;cin>> CpuTime>> Leval;process[i].ProcessName =name;process[i].Time =CpuTime;process[i].leval =Leval;cout<<endl;}int a;cout<<endl;cout<<" *******输入此进程时间片大小:******: ";cin>>TimePice;cout<<"\n *******选择调度算法:*************\n"<<endl;cout<<"**************************************************************"<<endl; cout<<"* 1: FCFS 2: 时间片轮换3: 优先级调度4: 最短作业优先*"<<endl; cout<<"**************************************************************"<<endl; cin>> a;for ( int k=0;k<num;k++)process[k].LeftTime=process[k].Time ;//对进程剩余时间初始化cout<<" // 说明: 在本程序所列进程信息中,优先级一项是指进程运行后的优先级!! "; cout<<endl; cout<<endl;if(a==1)Fcfs(process,num,TimePice);else if(a==2)TimeTurn( process, num, TimePice);else if(a==3){Sort( process, num);Priority( process , num, TimePice);}else // 最短作业算法,先按时间从小到到排序,再调用Fcfs算法即可{sort1(process,num);Fcfs(process,num,TimePice);}}void Copy ( Process proc1, Process proc2){proc1.leval =proc2.leval ;proc1.ProcessName =proc2.ProcessName ;proc1.Time =proc2.Time ;}void Sort( Process pr[], int size) //以进程优先级高低排序{for( int i=1;i<size;i++){Process temp;temp = pr[i];int j=i;while(j>0 && temp.leval<pr[j-1].leval){pr[j] = pr[j-1];j--;}pr[j] = temp;}for( int d=size-1;d>size/2;d--){Process temp;temp=pr [d];pr [d] = pr [size-d-1];pr [size-d-1]=temp;}}void sort1 ( Process pr[], int size) // 以进程时间从低到高排序{// 直接插入排序for( int i=1;i<size;i++){Process temp;temp = pr[i];int j=i;while(j>0 && temp.Time < pr[j-1].Time ){pr[j] = pr[j-1];j--;}pr[j] = temp;}}//// 先来先服务算法的实现void Fcfs( Process process[], int num, int Timepice){while(true){if(num==0){cout<<" 所有进程都已经执行完毕!\n"<<endl;exit(1);}if(process[0].LeftTime<=0){process[0].LeftTime=0;cout<<" 进程"<<process[0].ProcessName<< " 已经执行完毕!\n"<<endl;for (int i=0;i<num;i++)process[i]=process[i+1];num--;}else if(process[num-1].LeftTime<=0){process[num-1].LeftTime=0;cout<<" 进程"<<process[num-1].ProcessName<< " 已经执行完毕!\n"<<endl;num--;}else{cout<<endl;process[0].LeftTime=process[0].LeftTime- Timepice;if(process[0].LeftTime<=0)process[0].LeftTime=0;process[0].leval =process[0].leval-1;cout<<"进程名字"<<"共需占用CPU时间"<<" 还需要占用时间"<<" 优先级"<<" 状态"<<endl;cout<<" "<<process[0].ProcessName <<" "<<process[0].Time <<" ";cout<<process[0].LeftTime <<" "<<process[0].leval<<" 运行"<<endl;for(int s=1;s<num;s++){if(process[s].LeftTime<=0)process[s].LeftTime=0;cout<<"进程名字"<<"共需占用CPU时间"<<" 还需要占用时间"<<" 优先级"<<" 状态"<<endl;cout<<" "<<process[s].ProcessName <<" "<<process[s].Time <<" ";cout<<process[s].LeftTime <<" "<<process[s].leval<<" 等待"<<endl; ;}}cout<<endl;system(" pause");cout<<endl;}}/// 时间片轮转调度算法实现void TimeTurn( Process process[], int num, int Timepice){while(true){if(num==0){cout<<" 所有进程都已经执行完毕!\n"<<endl;exit(1);}if(process[0].LeftTime<=0){process[0].LeftTime=0;cout<<" 进程"<<process[0].ProcessName<< " 已经执行完毕!\n"<<endl;for (int i=0;i<num;i++)process[i]=process[i+1];num--;}if( process[num-1].LeftTime <=0 ){process[num-1].LeftTime=0;cout<<" 进程" << process[num-1].ProcessName <<" 已经执行完毕! \n"<<endl;num--;}else if(process[0].LeftTime > 0){cout<<endl; //输出正在运行的进程process[0].LeftTime=process[0].LeftTime- Timepice;if(process[0].LeftTime<0)process[0].LeftTime=0;process[0].leval =process[0].leval-1;cout<<"进程名字"<<"共需占用CPU时间"<<" 还需要占用时间"<<" 优先级"<<" 状态"<<endl;cout<<" "<<process[0].ProcessName <<" "<<process[0].Time <<" ";cout<<process[0].LeftTime <<" "<<process[0].leval<<" 运行"<<endl;for(int s=1;s<num;s++){if(process[s].LeftTime<0)process[s].LeftTime=0;cout<<"进程名字"<<"共需占用CPU时间"<<" 还需要占用时间"<<" 优先级"<<" 状态"<<endl;cout<<" "<<process[s].ProcessName <<" "<<process[s].Time <<" ";cout<<process[s].LeftTime <<" "<<process[s].leval;if(s==1)cout<<" 就绪"<<endl;elsecout<<" 等待"<<endl;}Process temp;temp = process[0];for( int j=0;j<num;j++)process[j] = process[j+1];process[num-1] = temp;}elsesystem(" pause");cout<<endl;system(" pause");cout<<endl;}}/// 优先级调度算法的实现void Priority( Process process[], int num, int Timepice){while( true){if(num==0){cout<< "所有进程都已经执行完毕!\n"<<endl;exit(1);}if(process[0].LeftTime<=0){process[0].LeftTime=0;cout<<" 进程" << process[0].ProcessName <<" 已经执行完毕! \n"<<endl;for( int m=0;m<num;m++)process[m] = process[m+1]; //一个进程执行完毕后从数组中删除num--; // 此时进程数目减少一个}if( num!=1 && process[num-1].LeftTime <=0 ){process[num-1].LeftTime=0;cout<<" 进程" << process[num-1].ProcessName <<" 已经执行完毕! \n"<<endl;num--;}if(process[0].LeftTime > 0){cout<<endl; //输出正在运行的进程process[0].LeftTime=process[0].LeftTime- Timepice;if(process[0].LeftTime<0)process[0].LeftTime=0;process[0].leval =process[0].leval-1;cout<<"进程名字"<<"共需占用CPU时间"<<" 还需要占用时间"<<" 优先级"<<" 状态"<<endl;cout<<" "<<process[0].ProcessName <<" "<<process[0].Time <<" ";cout<<process[0].LeftTime <<" "<<process[0].leval<<" 运行"<<endl;// 输出其他进程for(int s=1;s<num;s++){if(process[s].LeftTime<0)process[s].LeftTime=0;cout<<"进程名字"<<"共需占用CPU时间"<<" 还需要占用时间"<<" 优先级"<<" 状态"<<endl;cout<<" "<<process[s].ProcessName <<" "<<process[s].Time <<" ";cout<<process[s].LeftTime <<" "<<process[s].leval ;if(s==1)cout<<" 就绪"<<endl;elsecout<<" 等待"<<endl;}} // elseSort(process, num);cout<<endl;system(" pause");cout<<endl;} // while}【实验小结】本次试验的题目——进程管理。
操作系统实验报告java

操作系统实验报告java实验报告:操作系统实验一、实验目的本次实验旨在通过使用Java语言编写一个简单的操作系统,加深对操作系统原理的理解,掌握操作系统底层的相关知识。
二、实验环境1.Java编程环境:JDK 82.开发工具:Eclipse IDE3.操作系统:Windows 10三、实验内容1.实验背景介绍在本章节中,我们将介绍操作系统的基本概念、功能以及其在计算机系统中的作用。
2.实验设计与实现本章节将详细描述操作系统实现的设计思路和具体的实现过程,包括创建进程、调度算法、内存管理、文件系统等。
2.1 创建进程在本节中,我们将介绍如何使用Java语言创建一个进程,并实现进程的并发执行。
2.2 调度算法本节将讨论操作系统中的调度算法,例如先来先服务、短作业优先、时间片轮转等,并给出具体的实现代码。
2.3 内存管理在本节中,我们将介绍操作系统的内存管理机制,包括分区分配、动态存储分配等,并给出相关的实现方法。
2.4 文件系统本节将详细介绍操作系统中的文件系统的设计和实现,包括文件的存储、目录管理、文件访问控制等。
四、实验结果与分析1.实验结果展示在本章节中,我们将展示实验的运行结果,并对实验结果进行详细的分析和解释。
2.实验评估与讨论本节将对实验结果进行评估和讨论,包括对实验系统性能的评估以及可能存在的问题和改进方向的讨论。
五、实验总结本次实验通过使用Java语言编写一个简单的操作系统,使我们更深入地了解了操作系统的原理和实现,掌握了操作系统底层的相关知识。
六、附件本文档涉及的附件包括实验代码和相关实验结果的截图。
附件一:源代码文件(OS.java)附件二:实验结果截图(result.png)七、法律名词及注释1.操作系统:操作系统是一种控制和管理计算机硬件与软件资源的程序。
2.进程:进程是计算机中正在运行的一个程序实例,它具有独立的内存空间和系统资源。
3.调度算法:调度算法是操作系统中用于决定进程执行顺序的一种算法,目的是合理利用系统资源,提高系统性能。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
源代码:第一个类:divDTOpublic class divDTO{private int divBase;private int length;private int divFlag;public divDTO(int divBase,int length,int divFlag) {this.divBase = divBase;this.divFlag = divFlag;this.length = length;}public divDTO(){}public void setDivBase(int base){this.divBase = base;}public int getDivBase(){return this.divBase;}public void setLength(int length){this.length = length;}public int getLength(){return this.length;}public void setDivFlag(int flag){this.divFlag = flag;}public int getDivFalg(){return this.divFlag;}}2.第二个类:PcbDTOpublic class PcbDTO{static final int Running = 1;static final int Ready = 2;static final int Waiting = 3;private String processName;private int runTime;private int prority;private int processState;private int base;private int limit;private int pcbFlag;public PcbDTO(String name, int time,int pro,int base,int limit) {this.processName = name;this.runTime = time;this.prority = pro;this.processState = 0;this.limit = limit;this.base = base;}public PcbDTO(){this.pcbFlag = 0;}public void setProcessName(String name){this.processName = name;}public String getProcessName(){return processName;}public void setRunTime(int time){this.runTime = time;}public int getRunTime(){return this.runTime;}public void setPrority(int prority) {this.prority = prority;}public int getPrority(){return this.prority;}public void setProcessState(int state) {this.processState = state;}public String getProcessState(){String s = new String();if(this.processState == 1){s = "running";}else if(this.processState == 2){s = "ready";}else if(this.processState == 3){s = "waiting";}return s;}public int getBase(){return this.base;}public void setBase(int base){this.base = base;}public void setLimit(int limit){this.limit = limit;}public int getLimit(){return this.limit;}}第三个类:主类import javax.swing.*;import java.util.*;import java.awt.*;import java.awt.event.*; import javax.swing.event.*; public class MainFrame{private JList readyList;private JList waitingList;private JList jobList;private JButton susButton;private JButton relaxButton;private JButton startButton;private JButton newButton;private JLabel nameLabel;private JLabel prorityLabel;private JLabel timeLabel;private JLabel jobLabel;private JLabel readyLabel;private JLabel waitingLabel;private JLabel runningLabel;private JLabel spaceLabel;private JLabel divLabel;private JLabel allocLabel;private JTable readyTable;private JTable runningTable;private JTable divTable;private JTable allocTable;private JTextField nameText;private JTextField timeText;private JTextField spaceText;private JComboBox prorityCom;private JPanel newPanel;private JPanel waitingPanel;private JPanel readyPanel;Vector jobVectorName;Vector jobDtoVector;Vector waitingVectorName;Vector waitingDtoVector;PcbDTO []readyDtoArray;PcbDTO []newDtoArray;divDTO []divDtoArray;PcbDTO []newSort;Object[][] readydata;Object[][] runningdata;Object[][] divdata;Object[][] allocdata;int first;int end;int point;PcbDTO a ;public MainFrame(){a = new PcbDTO();first = 0;end = 0;point = 0;JFrame jf = new JFrame("进程调度-ws");Container c = jf.getContentPane();c.setLayout(null);//c.setBackground(Color.pink);newPanel = new JPanel();newPanel.setLayout(null);waitingPanel = new JPanel();waitingPanel.setLayout(null);//waitingPanel.setBackground(Color.pink);readyPanel = new JPanel();readyPanel.setLayout(null);susButton = new JButton("挂起");relaxButton = new JButton("释放");startButton = new JButton("开始"); newButton = new JButton("新建进程");nameLabel = new JLabel("进程名"); prorityLabel = new JLabel("优先级"); timeLabel = new JLabel("运行时间"); jobLabel = new JLabel("后备队列"); readyLabel = new JLabel("就绪队列"); waitingLabel = new JLabel("等待队列"); runningLabel = new JLabel("运行进程"); spaceLabel = new JLabel("需要空间"); divLabel = new JLabel("未分分区表"); allocLabel = new JLabel("内存分配表");nameText = new JTextField(); timeText = new JTextField(); spaceText = new JTextField(); prorityCom = new JComboBox(); prorityCom.setToolTipText("优先级");readyDtoArray = new PcbDTO[6]; newSort = new PcbDTO[6];for(int i = 0; i< 6;i++){newSort[i] = new PcbDTO();}newDtoArray = new PcbDTO[100]; jobDtoVector = new Vector(); jobVectorName = new Vector(); waitingDtoVector = new Vector(); waitingVectorName = new Vector(); divDtoArray = new divDTO[20];for(int i = 0; i < 20; i++){divDtoArray[i] = new divDTO();divDtoArray[i].setDivFlag(0);}divDtoArray[0].setDivFlag(1); divDtoArray[0].setDivBase(20); divDtoArray[0].setLength(180); readydata = new Object[6][4]; runningdata = new Object[2][3]; divdata = new Object[20][3]; allocdata = new Object[20][3];String []col1 = {"进程","时间","优先级","状态"}; String []col2 = {"进程","时间","优先级"};String []col3 = {"起址","长度","状态"};String []col4 = {"起址","长度","占用进程"}; readyTable = new JTable(readydata,col1);//readyTable.setEnabled(false);runningTable = new JTable(runningdata,col2); runningTable.setRowHeight(22); runningTable.setEnabled(false);allocTable = new JTable(allocdata,col4); allocTable.setEnabled(false);divTable = new JTable(divdata,col3);divTable.setEnabled(false);divTable.setValueAt(String.valueOf(20),0,0); divTable.setValueAt(String.valueOf(180),0,1); divTable.setValueAt(String.valueOf(1),0,2); JScrollPane runningSP = new JScrollPane(); JScrollPane readySP2 = new JScrollPane(); JScrollPane divSP = new JScrollPane(); JScrollPane allocSP = new JScrollPane(); runningSP.getViewport().add(runningTable); readySP2.getViewport().add(readyTable);divSP.getViewport().add(divTable);allocSP.getViewport().add(allocTable);//int []prorityArray = new int[10];for(int i = 0;i < 10;i++){prorityCom.addItem(i);//prorityArray[i] = i;}jobList = new JList();waitingList = new JList();JScrollPane readySP = new JScrollPane(readyList); JScrollPane jobSP = new JScrollPane(jobList); JScrollPane waitingSP = new JScrollPane(waitingList);newPanel.setSize(450,100);newPanel.setLocation(0,0);nameLabel.setSize(80,20);nameLabel.setLocation(10,5);nameText.setLocation(10,30); prorityLabel.setSize(80,20); prorityLabel.setLocation(120,5); prorityCom.setSize(100,25); prorityCom.setLocation(120,30); timeLabel.setSize(80,20); timeLabel.setLocation(230,5); timeText.setSize(100,25); timeText.setLocation(230,30); spaceLabel.setSize(80,20); spaceLabel.setLocation(340,5); spaceText.setSize(100,25); spaceText.setLocation(340,30); newButton.setSize(100,20); newButton.setLocation(320,70);waitingPanel.setSize(190,410); waitingPanel.setLocation(0,100);jobLabel.setSize(100,20); jobLabel.setLocation(10,2); jobSP.setSize(180,105); jobSP.setLocation(10,25); waitingLabel.setSize(100,20); waitingLabel.setLocation(10,129); waitingSP.setSize(180,105); waitingSP.setLocation(10,150); divLabel.setSize(100,20); divLabel.setLocation(10,253); divSP.setSize(180,113);divSP.setLocation(10,273); relaxButton.setSize(80,20); relaxButton.setLocation(110,388);readyPanel.setSize(260,410); readyPanel.setLocation(190,100);readyLabel.setSize(100,22); readyLabel.setLocation(10,2); allocLabel.setSize(100,20); allocLabel.setLocation(10,232); startButton.setSize(80,20); startButton.setLocation(177,388);susButton.setLocation(95,388);readySP2.setSize(250,117);readySP2.setLocation(10,25);runningLabel.setLocation(10,142);runningLabel.setSize(100,20);runningSP.setSize(250,65);runningSP.setLocation(10,167);allocSP.setSize(250,130);allocSP.setLocation(10,255);c.add(newPanel);newPanel.add(nameLabel);newPanel.add(nameText);newPanel.add(prorityLabel);newPanel.add(prorityCom);newPanel.add(timeText);newPanel.add(timeLabel);newPanel.add(newButton);newPanel.add(spaceLabel);newPanel.add(spaceText);c.add(waitingPanel);waitingPanel.add(jobLabel);waitingPanel.add(jobSP);waitingPanel.add(waitingLabel);waitingPanel.add(waitingSP);waitingPanel.add(divLabel);waitingPanel.add(divSP);waitingPanel.add(relaxButton);c.add(readyPanel);readyPanel.add(readyLabel);readyPanel.add(allocLabel);readyPanel.add(runningLabel);readyPanel.add(startButton);readyPanel.add(susButton);readyPanel.add(allocSP);readyPanel.add(runningSP);readyPanel.add(readySP2);jf.setSize(470,550);jf.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);jf.setLocationRelativeTo(null);jf.setVisible(true);startButton.addActionListener(new MyActionListener());newButton.addActionListener(new MyActionListener());susButton.addActionListener(new MyActionListener());relaxButton.addActionListener(new MyActionListener());}public void sus(){try{Thread.sleep(1000);}catch(Exception ex){}}class MyActionListener implements ActionListener{public void actionPerformed(ActionEvent e){int count = 0;PcbDTO test = new PcbDTO();JButton jb = (JButton)e.getSource();int max = -1;if(jb == startButton){//while(true)//{int runAllocFlag = -1;if((String)runningTable.getValueAt(0,0)==null||(String)runningTable.getValueAt(0,0)=="") {try{Thread.sleep(0);}catch(Exception ex){}//System.out.println("到3");for(int j = first;j != end; ){if(!readyDtoArray[j].getProcessState().equals("waiting")){max = j;break;}j = (j+1)%6;}for(int j = first;j%6 != end; ){if(!readyDtoArray[j].getProcessState().equals("waiting")){if(readyDtoArray[j].getPrority() > readyDtoArray[max].getPrority()){max = j;}}j = (j+1)%6;}if(max>=0){a = readyDtoArray[max];readyDtoArray[max] = readyDtoArray[first];readyDtoArray[first] = a;readyTable.setValueAt(readyDtoArray[max].getProcessName(),max,0);readyTable.setValueAt(readyDtoArray[max].getRunTime(),max,1);readyTable.setValueAt(readyDtoArray[max].getPrority(),max,2);readyTable.setValueAt(readyDtoArray[max].getProcessState(),max,3);readyTable.setValueAt("",first,0);readyTable.setValueAt("",first,1);readyTable.setValueAt("",first,2);readyTable.setValueAt("",first,3);runningTable.setValueAt(a.getProcessName(),0,0);runningTable.setValueAt(a.getRunTime(),0,1);runningTable.setValueAt(a.getPrority(),0,2);readyDtoArray[first].setRunTime(readyDtoArray[first].getRunTime()-1);if(0 != readyDtoArray[first].getPrority()){readyDtoArray[first].setPrority(readyDtoArray[first].getPrority()-1);}first = (first+1)%6;}else{System.out.println("cpu等待中……");}}else{/*try{Thread.sleep(2000);}catch(InterruptedException e1){System.out.println(e1);}*///System.out.println("到1");runningTable.setValueAt("",0,0);runningTable.setValueAt("",0,1);runningTable.setValueAt("",0,2);//如果运行时间为0则撤销进程,否则将进程重新添加到就绪队列中if( a.getRunTime()<=0){//收回内存空间for(int i = 0;i < point; i++){if(newSort[i].getBase()>=a.getBase()){newSort[i] = newSort[i+1];}}point--;//设置内存分配表的内容for(int i = 0; i < point;i++){allocTable.setValueAt(String.valueOf(newSort[i].getBase()),i,0);allocTable.setValueAt(String.valueOf(newSort[i].getLimit()),i,1);allocTable.setValueAt(newSort[i].getProcessName(),i,2);}allocTable.setValueAt("",point,0);allocTable.setValueAt("",point,1);allocTable.setValueAt("",point,2);//把收回的内存加入到记录未分分区的数组int memoryEnd = 0;int location = 0;int up = -1;//int down = -1;for(int i = 0;i<20;i++){if(divDtoArray[i].getDivFalg() == 1){memoryEnd = divDtoArray[i].getDivBase()+divDtoArray[i].getLength();if(memoryEnd == a.getBase()){up = i;}if(divDtoArray[i].getDivBase() == (a.getBase()+a.getLimit())){down = i;}}}if(up >= 0&&down >= 0){divDtoArray[up].setLength((divDtoArray[up].getLength()+a.getLimit()+divDtoArray[down].ge tLength()));divDtoArray[down].setDivFlag(0);for(int i = (down+1); i < 20;i++){if(divDtoArray[i].getDivFalg() == 1){divDtoArray[i-1].setDivBase(divDtoArray[i].getDivBase());divDtoArray[i-1].setDivFlag(1);divDtoArray[i-1].setLength(divDtoArray[i].getLength());divDtoArray[i].setDivFlag(0);}else{divTable.setValueAt("",i-1,0);divTable.setValueAt("",i-1,1);divTable.setValueAt("",i-1,2);break;}}}else if(up >= 0&&down < 0){divDtoArray[up].setLength((divDtoArray[up].getLength()+a.getLimit()));}else if(up < 0&&down >= 0){divDtoArray[down].setLength((divDtoArray[down].getLength()+a.getLimit()));divDtoArray[down].setDivBase(a.getBase());}else if(up < 0&&down < 0){for(int i = 0; i < 20; i++){if(divDtoArray[i].getDivBase()>a.getBase()||divDtoArray[i].getDivFalg()==0){location = i;break;}}for(int i = 20; i >location;i--)if(divDtoArray[i-1].getDivFalg()==1){divDtoArray[i].setDivBase(divDtoArray[i-1].getDivBase());divDtoArray[i].setDivFlag(1);divDtoArray[i].setLength(divDtoArray[i-1].getLength());}}divDtoArray[location].setDivBase(a.getBase());divDtoArray[location].setDivFlag(1);divDtoArray[location].setLength(a.getLimit());}//设置未分分区表的内容for(int i = 0; i < 20;i++){if(divDtoArray[i].getDivFalg()==1){divTable.setValueAt(String.valueOf(divDtoArray[i].getDivBase()),i,0);divTable.setValueAt(String.valueOf(divDtoArray[i].getLength()),i,1);divTable.setValueAt(String.valueOf(divDtoArray[i].getDivFalg()),i,2);}}if(!jobDtoVector.isEmpty()){int runLength = 0;PcbDTO jobToReady = (PcbDTO)jobDtoVector.elementAt(0);for(int i = 0; i < 20; i++){if(divDtoArray[i].getDivFalg() == 1){if(divDtoArray[i].getLength() >= jobToReady.getLimit()){runAllocFlag = i;break;}}if(runAllocFlag >= 0){jobDtoVector.removeElementAt(0);jobVectorName.remove(jobVectorName.indexOf(jobToReady.getProcessName()));jobList.setListData(jobVectorName);jobToReady.setProcessState(PcbDTO.Ready);jobToReady.setBase(divDtoArray[runAllocFlag].getDivBase());runLength = divDtoArray[runAllocFlag].getLength()- jobToReady.getLimit();if(runLength == 0){int i = runAllocFlag;divDtoArray[i].setDivFlag(0);for(; i < 19;i++){if(divDtoArray[i+1].getDivFalg() == 1){divDtoArray[i] = divDtoArray[i+1];divDtoArray[i+1].setDivFlag(0);}divTable.setValueAt(String.valueOf(divDtoArray[i].getDivBase()),i,0);divTable.setValueAt(String.valueOf(divDtoArray[i].getLength()),i,1);divTable.setValueAt(String.valueOf(divDtoArray[i].getDivFalg()),i,2);}divTable.setValueAt(String.valueOf(divDtoArray[i].getDivFalg()),i,2);}else if(runLength > 0){int c2 = divDtoArray[runAllocFlag].getDivBase()+ jobToReady.getLimit();divDtoArray[runAllocFlag].setDivBase(c2);divDtoArray[runAllocFlag].setLength(runLength);divTable.setValueAt(String.valueOf(c2),runAllocFlag,0);divTable.setValueAt(String.valueOf(runLength),runAllocFlag,1);divTable.setValueAt(String.valueOf(divDtoArray[runAllocFlag].getDivFalg()),runAllocFlag,2);}readyDtoArray[end] = jobToReady;readyTable.setValueAt(jobToReady.getProcessName(),end,0);readyTable.setValueAt(jobToReady.getRunTime(),end,1);readyTable.setValueAt(jobToReady.getPrority(),end,2);readyTable.setValueAt(jobToReady.getProcessState(),end,3);end = (end+1)%6;int runi = 0;//用于记录当前新生成的PcbDTO对象应该插入到newSort中的位置for(; runi < point; runi++){if(jobToReady.getBase() < newSort[runi].getBase()){break;}}//如果不是插入到数组末尾,则把比它大的都向后挪一位并设置JTable中的显示for(int i = point; i > runi; i--){newSort[i] = newSort[i-1];allocTable.setValueAt(String.valueOf(newSort[i].getBase()),i,0);allocTable.setValueAt(String.valueOf(newSort[i].getLimit()),i,1);allocTable.setValueAt(newSort[i].getProcessName(),i,2);}//插入新生成的对象newSort[runi] = jobToReady;allocTable.setValueAt(String.valueOf(jobToReady.getBase()),runi,0);allocTable.setValueAt(String.valueOf(jobToReady.getLimit()),runi,1);allocTable.setValueAt(jobToReady.getProcessName(),runi,2);point++;}}}else{readyDtoArray[end] = a;readyTable.setValueAt(a.getProcessName(),end,0);readyTable.setValueAt(a.getRunTime(),end,1);readyTable.setValueAt(a.getPrority(),end,2);readyTable.setValueAt(a.getProcessState(),end,3);end = (end+1)%6;}}//}}else if(jb == newButton){int newAllocFlag = -1;int newLength = 0;if(nameText.getText().trim().length() == 0){JOptionPane.showMessageDialog(null,"进程名不能为空!");}else if(timeText.getText().trim().length() == 0){JOptionPane.showMessageDialog(null,"运行时间不能为空");}else if(spaceText.getText().trim().length() == 0){JOptionPane.showMessageDialog(null,"空间不能为空");}else{test.setRunTime(Integer.parseInt(timeText.getText()));test.setLimit(Integer.parseInt(spaceText.getText()));String s = prorityCom.getSelectedItem().toString();test.setPrority(Integer.parseInt(s));test.setProcessName(nameText.getText().trim());newDtoArray[count] = test;jobDtoVector.add(newDtoArray[count]);jobVectorName.add(newDtoArray[count].getProcessName());jobList.setListData(jobVectorName);count++;nameText.setText("");timeText.setText("");spaceText.setText("");PcbDTO b = (PcbDTO)jobDtoVector.elementAt(0);for(int i = 0; i < 20; i++){if(divDtoArray[i].getDivFalg() == 1){if(divDtoArray[i].getLength() >= b.getLimit()){newAllocFlag = i;break;}}}//在就绪队列未满且内存有足够空间时将后备队列jobDtoVetor中的对象添加到就绪队列中if((end + 2)%6 != first&&newAllocFlag >= 0){jobDtoVector.removeElementAt(0);b.setProcessState(PcbDTO.Ready);b.setBase(divDtoArray[newAllocFlag].getDivBase());newLength = divDtoArray[newAllocFlag].getLength()- b.getLimit();if(newLength == 0){int i = newAllocFlag;divDtoArray[i].setDivFlag(0);for(; i < 19;i++){if(divDtoArray[i+1].getDivFalg() == 1){divDtoArray[i] = divDtoArray[i+1];divDtoArray[i+1].setDivFlag(0);}divTable.setValueAt(String.valueOf(divDtoArray[i].getDivBase()),i,0);divTable.setValueAt(String.valueOf(divDtoArray[i].getLength()),i,1);divTable.setValueAt(String.valueOf(divDtoArray[i].getDivFalg()),i,2);}divTable.setValueAt(String.valueOf(divDtoArray[i].getDivFalg()),i,2);}else if(newLength > 0){int c1 = divDtoArray[newAllocFlag].getDivBase()+ b.getLimit();divDtoArray[newAllocFlag].setDivBase(c1);divDtoArray[newAllocFlag].setLength(newLength);divTable.setValueAt(String.valueOf(c1),newAllocFlag,0);divTable.setValueAt(String.valueOf(newLength),newAllocFlag,1);divTable.setValueAt(String.valueOf(divDtoArray[newAllocFlag].getDivFalg()),newAllocFlag,2);}readyDtoArray[end] = b;jobVectorName.remove(jobVectorName.indexOf(b.getProcessName()));readyTable.setValueAt(b.getProcessName(),end,0);readyTable.setValueAt(b.getRunTime(),end,1);readyTable.setValueAt(b.getPrority(),end,2);readyTable.setValueAt("ready",end,3);end =(end+1)%6;int newi = 0;//用于记录当前新生成的PcbDTO对象应该插入到newSort中的位置for(; newi < point; newi++){if(b.getBase() < newSort[newi].getBase()){break;}}//如果不是插入到数组末尾,则把比它大的都向后挪一位并设置JTable中的显示for(int i = point; i > newi; i--){newSort[i] = newSort[i-1];allocTable.setValueAt(String.valueOf(newSort[i].getBase()),i,0);allocTable.setValueAt(String.valueOf(newSort[i].getLimit()),i,1);allocTable.setValueAt(newSort[i].getProcessName(),i,2);}//插入新生成的对象newSort[newi] = b;allocTable.setValueAt(String.valueOf(b.getBase()),newi,0);allocTable.setValueAt(String.valueOf(b.getLimit()),newi,1);allocTable.setValueAt(b.getProcessName(),newi,2);point++;}}}else if(jb == susButton){if(readyDtoArray[readyTable.getSelectedRow()] != null){if(!readyDtoArray[readyTable.getSelectedRow()].getProcessState().equals("waiting")){readyDtoArray[readyTable.getSelectedRow()].setProcessState(PcbDTO.Waiting);readyTable.setValueAt("waiting",readyTable.getSelectedRow(),3); waitingDtoVector.add(readyDtoArray[readyTable.getSelectedRow()]);waitingVectorName.add(readyDtoArray[readyTable.getSelectedRow()].getProcessName());waitingList.setListData(waitingVectorName);}else{System.out.println("已挂起");}}else{JOptionPane.showMessageDialog(null,"请选择要挂起的进程");//System.out.println("请选择要挂起的进程");}}else if(jb == relaxButton){String s = (String)waitingList.getSelectedValue();if(s != null){waitingVectorName.remove(s);PcbDTO p = new PcbDTO();for(int i = 0; i < waitingDtoVector.size(); i++){p = (PcbDTO)waitingDtoVector.elementAt(i);if(s.equals(p.getProcessName())){p.setProcessState(PcbDTO.Ready);waitingDtoVector.remove(p);break;}}for(int i = 0;i < 6;i++){if(s.equals(readyDtoArray[i].getProcessName())){readyTable.setValueAt("ready",i,3);break;}}waitingList.setListData(waitingVectorName);}else{JOptionPane.showMessageDialog(null,"请选择要解挂的进程");//System.out.println("没有选择的进程");}}}}public static void main(String args[]){new MainFrame();}}相关截图如下:运行后开始界面输入进程后界面建立后挂起挂起后各内存分配解挂操作异常时的提示。