链表实现多项式相加实验报告
(2023)数据结构利用链表计算一元多项式课程设计实验报告(一)

(2023)数据结构利用链表计算一元多项式课程设计实验报告(一)2023数据结构课程设计实验报告——利用链表计算一元多项式实验背景和目的在本课程设计实验中,我们旨在通过使用链表数据结构,实现对一元多项式的计算功能。
通过本次实验,我们将深入学习和掌握链表的基础知识和应用技能,掌握实现链表操作的代码实现方式,提高编程实践能力和解决问题的能力。
思路和方法首先,我们需要定义链表节点数据结构,包含多项式中的系数和指数两个数据成员。
然后,我们需要实现一元多项式的相加、相减、求导、求值等基本操作。
其中,相加和相减操作需要利用链表遍历的方式,比较两个多项式中的指数,进行对应系数的加减,并将结果存储到新的链表中。
求导操作只需要遍历链表,将每一项的指数减一,系数乘以指数值,再将其插入到新的链表中即可。
求值操作仅需要遍历链表,根据指数和系数计算多项式值即可。
在具体实现过程中,我们需要注意边界条件的判断和处理,如何处理空链表、单项式情况等。
还需要精细设计代码逻辑,避免重复遍历链表,浪费时间和空间资源。
结果分析和展示经过数次测试和调试,我们最终实现了一元多项式的链表计算功能。
我们在终端输入多项式的系数和指数,再根据指令进行相应的操作。
结果能够准确输出,并且经过大量数据测试,程序运行稳定,没有出现崩溃和错误的情况。
总结和反思通过本次实验,我们进一步深入学习了链表数据结构的应用方法和相关算法。
我们学会了如何通过遍历链表实现复杂计算操作,如一元多项式的求导、求值等。
在实现过程中,我们对代码结构和逻辑设计进行反复思考和优化,提高了自己的编程能力和解决问题的能力。
同时,我们也需要进一步加强数据结构的学习,提升自己的算法水平,为后续的专业学习和职业发展打下坚实的基础。
可能的改进和优化方案虽然我们已经实现了一元多项式链表计算功能,但是我们也发现了以下几点可以改进和优化的方案:•异常处理:在用户输入有误或者数据不规范的情况下,程序可能会出现崩溃或者不符合预期的结果。
多项式加法实验报告
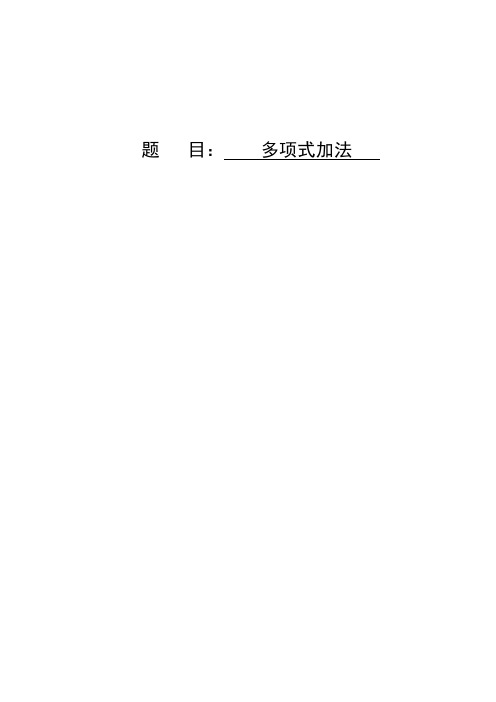
题目:多项式加法多项式加法一、课题概述线性表是一种最简单、最基本,也是最常用的数据结构,其用途十分广泛,例如,用带表头结点的单链表求解一元整系数多项式加法和乘法运算。
现给两个一元整系数多项式,请求解两者之和。
输入两组数据,每一组代表一个一元整系数多项式,有多行组成,其中每一行给出多项式每一项的系数和指数,这些行按指数递减次序排序,每一组结束行为0 -1。
输出三组数据,前两组为一元整系数多项式,最后一组为两个多项式的和。
一元整系数多项式输出形式如下:(1)多项式项4x输出为4X(2)多项式项4x2输出为4X^2(3)第一项系数为正数时,加号不要输出(4)除常系数项外,项系数为1不显式输出,-1输出为-例如,4x3- x2+x-1正确输出形式为4X^3-X^2+X-1,错误输出形式为+4X^3-1X^2+1X-1二、设计与实现1、类的层次关系及核心算法分析:项节点类Trem中定义了三个私有变量,系数coef、指数exp和指向下一个项节点的指针域link。
多项式类Polynominal被声明成项节点类Trem类的友元类。
公有函数InsertAfter构造一个新的项节点,其系数为c指数为e,并将新节点插入在调用该函数的项节点及后继节点之间。
多项式类Polynominal中包含了3个公有成员函数:AddTerms,Output和PolyAdd。
AddTerms函数通过输入流in,输入多项式的各项构造一个多项式的单循环链表;Output函数将多项式按降幂方式送输出流;PolyAdd函数实现将多项式r加到指针this指示的多项式上。
AddTerms函数从输入流in按降幂输入各项(c,e)来构造多项式的单循环链表,当输入(0,-1)是构造过程结束。
Output函数遍历单循环链表将多项式按降幂方式送输出流,它调用项类Trem 上重载的“<<”操作符实现按项输出。
多项式加法,设有多项式p(x)和q(x),分别用单循环链表表示。
实验三链表及其多项式相加 - 副本
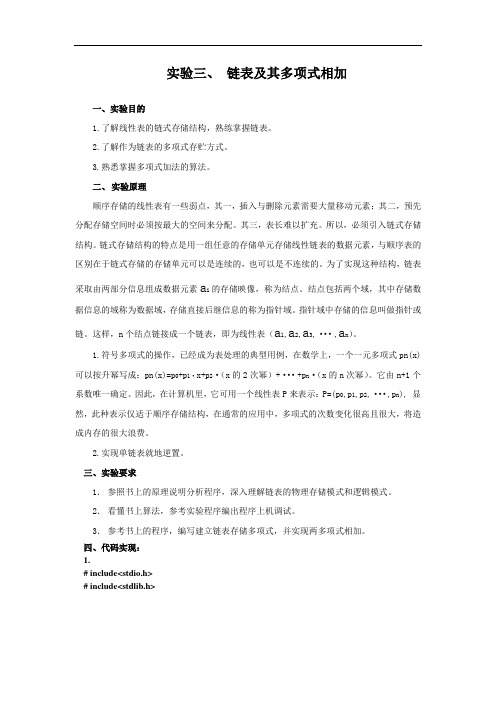
实验三、链表及其多项式相加一、实验目的1.了解线性表的链式存储结构,熟练掌握链表。
2.了解作为链表的多项式存贮方式。
3.熟悉掌握多项式加法的算法。
二、实验原理顺序存储的线性表有一些弱点,其一,插入与删除元素需要大量移动元素;其二,预先分配存储空间时必须按最大的空间来分配。
其三,表长难以扩充。
所以,必须引入链式存储结构。
链式存储结构的特点是用一组任意的存储单元存储线性链表的数据元素,与顺序表的区别在于链式存储的存储单元可以是连续的,也可以是不连续的。
为了实现这种结构,链表采取由两部分信息组成数据元素a i的存储映像,称为结点。
结点包括两个域,其中存储数据信息的域称为数据域,存储直接后继信息的称为指针域。
指针域中存储的信息叫做指针或链。
这样,n个结点链接成一个链表,即为线性表(a1,a2,a3,···,a n)。
1.符号多项式的操作,已经成为表处理的典型用例,在数学上,一个一元多项式pn(x)可以按升幂写成:pn(x)=p0+p1·x+p2·(x的2次幂)+···+p n·(x的n次幂)。
它由n+1个系数唯一确定。
因此,在计算机里,它可用一个线性表P来表示:P=(p0,p1,p2,···,p n), 显然,此种表示仅适于顺序存储结构,在通常的应用中,多项式的次数变化很高且很大,将造成内存的很大浪费。
2.实现单链表就地逆置。
三、实验要求1.参照书上的原理说明分析程序,深入理解链表的物理存储模式和逻辑模式。
2.看懂书上算法,参考实验程序编出程序上机调试。
3.参考书上的程序,编写建立链表存储多项式,并实现两多项式相加。
四、代码实现:1.# include<stdio.h># include<stdlib.h>typedef struct Polynode{int coef;int exp;Polynode *next;}Polynode,* Polylist;Polylist polycreate(){Polynode * head,*rear,*s;int c,e;head=(Polynode *)malloc(sizeof(Polynode)); rear=head;scanf("%d,%d",&c,&e);while(c!=0){s=(Polynode *)malloc(sizeof(Polynode));s->coef=c;s->exp=e;rear->next=s;rear=s;scanf("%d,%d",&c,&e);}rear->next=NULL;return (head);}void polyadd(Polylist polya,Polylist polyb) {Polynode *p,*q,*tail,*temp;int sum;p=polya->next;q=polyb->next;tail=polya;while(p!=NULL && q!=NULL){if (p->exp<q->exp){tail->next=p;tail=p;p=p->next;}else if (p->exp==q->exp){sum=p->coef+q->coef;if (sum!=0){p->coef=sum;tail->next=p;tail=p;p=p->next;temp=q;q=q->next;free(temp);}else{temp=p;p=p->next;free(temp);temp=q;q=q->next;free(temp);}}else{tail->next=q;tail=q;q=q->next;}}if(p==NULL)tail->next=p;elsetail->next=q;}void main(){Polynode *p;printf("请输入第一个多项式,次数从低到高:\n"); Polylist A=polycreate();printf("\n多项式创建完成!\n");printf("\n请输入第二个多项式,次数从低到高:\n"); Polylist B=polycreate();printf("\n多项式创建完成!\n\n");polyadd(A,B);p=A->next;while(p!=NULL){printf("[%d %d] ",p->coef,p->exp);p=p->next;}printf("\n\n");}2.# include<stdio.h># include<stdlib.h>typedef struct Node{char data;struct Node * next;}Node,* Linklist;void Initlist(Linklist *L){*L=(Linklist)malloc(sizeof(Node));(*L)->next=NULL;}void CreateFromHead(Linklist L){Node *s;char c;int flag=1;while(flag){c=getchar();if (c!='$'){s=(Node *)malloc(sizeof(Node));s->data=c;s->next=L->next;L->next=s;}else flag=0;}}void Reverselist(Linklist L){Linklist p,q;p=L->next;L->next=NULL;while(p!=NULL){q=p->next;p->next=L->next;L->next=p;p=q;}}void main (){Linklist p;Linklist L;Initlist(&L);printf("Please input datas:\n");CreateFromHead(L);p=L;while (p->next!=NULL){p=p->next;printf("%c ",p->data);}Reverselist(L);printf("\n\n");}五:结果验证:1.2。
多项式相加相乘链表实现模板
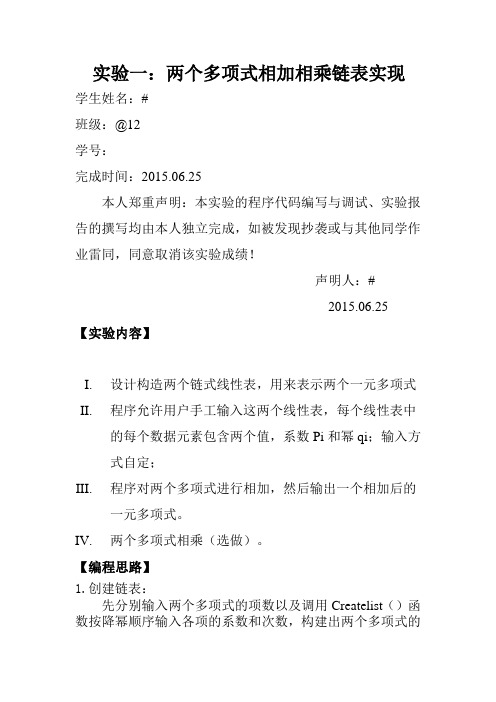
实验一:两个多项式相加相乘链表实现学生姓名:#班级:@12学号:完成时间:2015.06.25本人郑重声明:本实验的程序代码编写与调试、实验报告的撰写均由本人独立完成,如被发现抄袭或与其他同学作业雷同,同意取消该实验成绩!声明人:#2015.06.25【实验内容】I.设计构造两个链式线性表,用来表示两个一元多项式II.程序允许用户手工输入这两个线性表,每个线性表中的每个数据元素包含两个值,系数Pi和幂qi;输入方式自定;III.程序对两个多项式进行相加,然后输出一个相加后的一元多项式。
IV.两个多项式相乘(选做)。
【编程思路】1.创建链表:先分别输入两个多项式的项数以及调用Createlist()函数按降幂顺序输入各项的系数和次数,构建出两个多项式的链表。
两个多项式的系数和次数同样按降幂顺序存入两个线性表a、b中,头结点分别为head_a,head_b。
2.多项式相加:创建一个新链表,作为相加后所得结果的多项式的链表寄存处。
定义两个临时链表节点指针pa和pb,分别指向两个链表头结点的下一位(注:链表的头结点不存项)。
通过比较两个多项式的首项确定最高次幂,用max_qi记录。
然后用一个for循环从两个链表中寻找,判断pa和pb当前位置有无次数为i(i为循环变量)的项,有则合并同类项。
如果pa、pb次数等于i,两对应系数相加,和其系数一起存入新节点中,再将新节点插入新链表中,pa、pb随后后移一个单位;如果pa的次数小于i,pb次数等于i,则只加pb项,也存入新节点并插入新链表中,随后pb向后移动一个单位;如果pb的次数小于i,pa次数等于i,处理方法与上一种情况类似。
如果pa和pb次数均小于i,则i减小1,进入下一次循环,再作比较。
若pa或pb为空,则将不为空的多项式链表的剩余项逐一赋给新链表即可。
3.多项式相乘:创建一个新链表,作为相乘后所得结果的多项式的链表寄存处。
定义两个临时链表节点指针pa和pb,分别指向两个链表头结点的下一位。
多项式加法的链表设计与实现
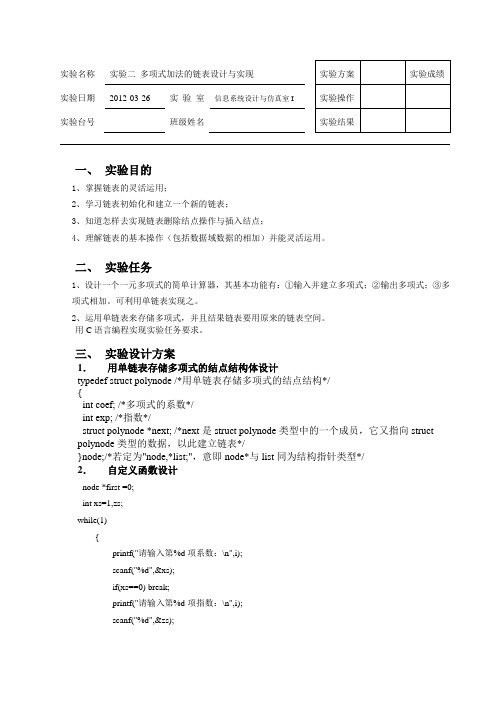
printf("coef:");
scanf("%d",&c); /*输入系数*/
printf("exp: ");
scanf("%d",&e); /*输入指针*/
while(c!=0) /*输入系数为0时,表示多项式的输入结束*/
//////补充代码......
pre->next=p;
}
else if(p->exp==q->exp) /*若指数相等,则相应的系数相加*/
{
sum=p->coef+q->coef;
if(sum!=0)
{/*将p结点加入到和多项式中,删除结点q*/
p->coef=sum;
pre->next=p;pre=pre->next;p=p->next;
polyb=create(); /*调用建立链表函数,创建多项式B*/
print(polyb);
printf("Sum of the poly is:\n");
polyadd(polya,polyb); /*调用一元多项式相加函数*/
print(polya); /*调用输出函数,打印结果*/
printf("\n");
存放在多项式polya中,并将多项式ployb删除*/
{
node *p,*q,*pre,*temp;
int sum;
p=polya->next;/*令p和q分别指向polya和polyb多项式链表中的第一个结点*/
(链表实现)写出两个一元多项式相加的算法

(链表实现)写出两个⼀元多项式相加的算法int c, e;head=new node; //⽣成头结点head->next=NULL;cout<<"请分别输⼊新的⼀项的系数、指数(以输⼊9999作为结束):"<<endl;cin>>c>>e;while(c!=9999 && e!=9999) //输⼊并检测结束{s=new node; //⽣成新结点s->co = c; //装⼊数据s->exp = e;p=head;while(p->next!=NULL){p=p->next;}p->next = s; //插到最后⼀个结点后s->next = NULL;cout<<"请分别输⼊新的⼀项的系数、指数(以输⼊9999作为结束):"<<endl;cin>>c>>e;}return head;}void Display(node * head){if(head==NULL){cout<<"多项式不存在!"<<endl;return ;}node* p;p=head->next;while(p!=NULL){if(p->co>0)cout<<p->co<<"x^"<<p->exp;elsecout<<"("<<p->co<<")"<<"x^"<<p->exp;if(p->next!=NULL)cout<<"+";p=p->next;}cout<<endl<<endl;}node* Add(node * A, node * B){node * C=new node;C->next=NULL;node * p1=A->next;node * p2=B->next;node * p3;node * rearC=C;while(p1!=NULL && p2!=NULL){。
数据结构多项式相加实验报告doc
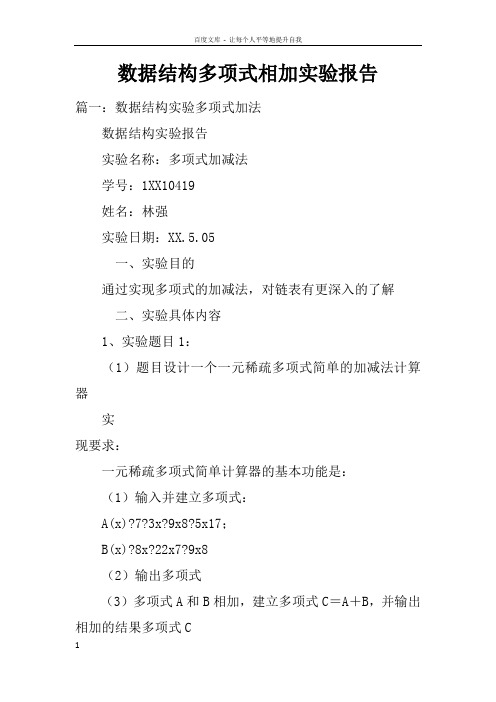
数据结构多项式相加实验报告篇一:数据结构实验多项式加法数据结构实验报告实验名称:多项式加减法学号:1XX10419姓名:林强实验日期:XX.5.05一、实验目的通过实现多项式的加减法,对链表有更深入的了解二、实验具体内容1、实验题目1:(1)题目设计一个一元稀疏多项式简单的加减法计算器实现要求:一元稀疏多项式简单计算器的基本功能是:(1)输入并建立多项式:A(x)?7?3x?9x8?5x17;B(x)?8x?22x7?9x8(2)输出多项式(3)多项式A和B相加,建立多项式C=A+B,并输出相加的结果多项式C(4)选作:多项式A和B相减,建立多项式C=A-B,并输出相加的结果多项式D(2)分析1:本程序的任务是实现两个多项式的加法其中多项式的系数为浮点型,指数为整数,输出的结果也为系数和指数。
(1)输入的形式和输入值的范围:输入多项式的系数a和未知数X的指数b,当a和b都为零时,输入结束。
输入值的范围:a为实数,b为整数。
(2)输出形式:输出多项式的系数和多项式未知数X 的指数即(a,b)形式。
(3)程序所能达到的功能,实现两个多项式的加法,并输出最后的结果2:整个程序运行期间实行动态创建节点,一边输入数据,一边创建节点当将全部数据输入到单链表中后再调用多项式加法这个函数,并一边实现多项式的相加,一边释放节点,有效防止了在程序反复运行过程中可能出现系统空间不够分配的现象(3)实验代码typedef int Status;#define OVERFLOW -1#define null 0typedef struct Lnode{float coef; //存储项系数int expn;//存储项指数struct Lnode *next;}Lnode,*LinkList;typedef LinkList polynomial;Status InitList_L(LinkList &L) {//初始化头节点L=(LinkList)malloc(sizeof(Lnode));if(!L)return(-1);L->next=null;return 1;}void AddPolyn(polynomial pa, polynomial pb){ //实现两个多项式相加的算法float x;polynomial qa;polynomial qb;polynomial s;polynomial u;qa=pa->next; qb=pb->next; s=pa;while(qa&&qb){if(qa->expnexpn){s=qa;qa=qa->next;}else if(qa->expn==qb->expn){x=qa->coef+qb->coef;if(x!=0){qa->coef=x;s=qa;qa=qa->next;u=qb;qb=qb->next;free(u);}else{s->next=qa->next;free(qa);qa=s->next;u=qb;qb=qb->next;free(u);}}else if(qa->expn>qb->expn){ u=qb->next;s->next=qb;s=qb;qb->next=qa;qb=u;}}if(qb)qa->next=qb;free(pb);}void main(){float a;int b;polynomial L1;polynomial L2; LinkList q;LinkList p;LinkList m;LinkList n;InitList_L(L1);q=L1;InitList_L(L2);p=L2;cout 请输入数据:" for(;;){ cin>>a;cin>>b;if(a==0&&b==0) break;m=new Lnode;m->coef=a;m->expn=b;q->next=m;q=m;q->next=null;}//循环输入第一个多项式的系数与指数for(;;){cin>>a;cin>>b;if(a==0&&b==0)break;n=new Lnode;n->coef=a;n->expn=b;p->next=n;p=n;p->next=null;}//循环输入第二个多项式的系数与指数AddPolyn(L1,L2);//调用多项式相加的算法while((L1->next)!=null){coutnext->coefnext->expn L1=L1->next;}//输出计算结果}三、实验小结通过编写多项加法这个程序,我将自己所学到的创建链表,初始化链表和多项式加法算法都应用了一次,这使我们不仅仅只是理论化的学习书本上的知识,而是将学习到的理论知识应用到实际的操作中来增强我们的实际操作能力,这使我增加了实际操作经验,也使我通过实际操作来认识到自己在程序编写上的不足从而增强了我的实际编写程序的能力。
数据结构实验报告完成多项式的运算
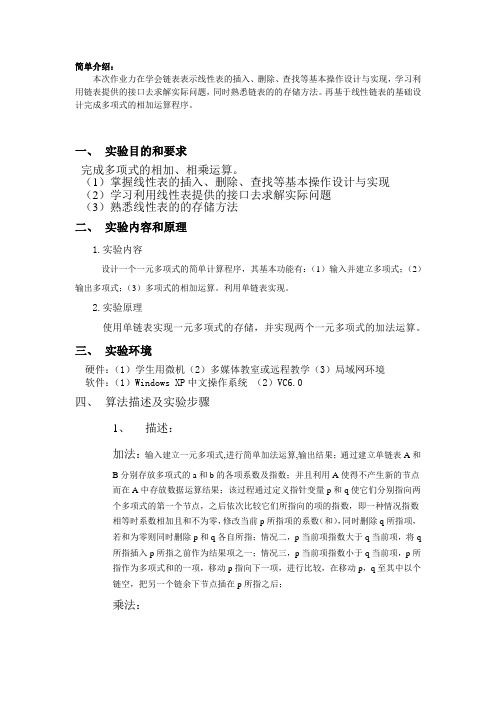
简单介绍:本次作业力在学会链表表示线性表的插入、删除、查找等基本操作设计与实现,学习利用链表提供的接口去求解实际问题,同时熟悉链表的的存储方法。
再基于线性链表的基础设计完成多项式的相加运算程序。
一、实验目的和要求完成多项式的相加、相乘运算。
(1)掌握线性表的插入、删除、查找等基本操作设计与实现(2)学习利用线性表提供的接口去求解实际问题(3)熟悉线性表的的存储方法二、实验内容和原理1.实验内容设计一个一元多项式的简单计算程序,其基本功能有:(1)输入并建立多项式;(2)输出多项式;(3)多项式的相加运算。
利用单链表实现。
2.实验原理使用单链表实现一元多项式的存储,并实现两个一元多项式的加法运算。
三、实验环境硬件:(1)学生用微机(2)多媒体教室或远程教学(3)局域网环境软件:(1)Windows XP中文操作系统(2)VC6.0四、算法描述及实验步骤1、描述:加法:输入建立一元多项式,进行简单加法运算,输出结果;通过建立单链表A和B分别存放多项式的a和b的各项系数及指数;并且利用A使得不产生新的节点而在A中存放数据运算结果;该过程通过定义指针变量p和q使它们分别指向两个多项式的第一个节点,之后依次比较它们所指向的项的指数,即一种情况指数相等时系数相加且和不为零,修改当前p所指项的系数(和),同时删除q所指项,若和为零则同时删除p和q各自所指;情况二,p当前项指数大于q当前项,将q所指插入p所指之前作为结果项之一;情况三,p当前项指数小于q当前项,p所指作为多项式和的一项,移动p指向下一项,进行比较,在移动p,q至其中以个链空,把另一个链余下节点插在p所指之后;乘法:定义指针p,q指向所操作节点,通过A链表的每一项与B链表各项相乘,指数相加,系数相乘,将值赋给新节点各自域,构成一新的链表,最后返回头结点。
可这样有一个问题,即新生成的链表,即最终结果混乱,没有对数据进行过滤,相同指数项应在执行加法运算,所以可以这样实现,通过A链表的每一项与B链表各项相乘的新生成节点单独构成一链表,并将第一个链表加入另一新链表,循环此操作将后生成的链表加之先前的链表,即可实现排序问题。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
实验报告
课程名称:数据结构
题目:链表实现多项式相加
班级:
学号:
姓名:
完成时间:2012年10月17日
1、实验目的和要求
1)掌握链表的运用方法;
2)学习链表的初始化并建立一个新的链表;
3)知道如何实现链表的插入结点与删除结点操作;
4)了解链表的基本操作并灵活运用
2、实验内容
1)建立两个链表存储一元多项式;
2)实现两个一元多项式的相加;
3)输出两个多项式相加后得到的一元多项式。
3、算法基本思想
数降序存入两个链表中,将大小较大的链表作为相加后的链表寄存处。
定义两个临时链表节点指针p,q,分别指向两个链表头结点。
然后将另一个链表中从头结点开始依次与第一个链表比较,如果其指数比第一个小,则p向后移动一个单位,如相等,则将两节点的系数相加作为第一个链表当前节点的系数,如果为0,则将此节点栓掉。
若果较大,则在p前插入q,q向后移动一个,直到两个链表做完为止。
4、算法描述
用链表实现多项式相加的程序如下:
#include<stdio.h>
#include<stdlib.h>
#include<math.h>
struct node{
int exp;
float coef;
struct node*next;
};
void add_node(struct node*h1,struct node*h2);
void print_node(struct node*h);
struct node*init_node()
{
struct node*h=(struct node*)malloc(sizeof(struct node)),*p,*q;
int exp;
float coef=1.0;
h->next=NULL;
printf("请依次输入多项式的系数和指数(如:\"2 3\";输入\"0 0\"时结束):\n");
p=(struct node*)malloc(sizeof(struct node));
q=(struct node*)malloc(sizeof(struct node));
for(;fabs(coef-0.0)>1.0e-6;)
{
scanf("%f %d",&coef,&exp);
if(fabs(coef-0.0)>1.0e-6)
{
q->next=p;
p->coef=coef;
p->exp=exp;
p->next=NULL;
add_node(h,q);
}
}
free(p);
free(q);
return(h);
}
void add_node(struct node*h1,struct node*h2)
{
struct node*y1=h1,*y2=h2;
struct node*p,*q;
y1=y1->next;
y2=y2->next;
for(;y1||y2;)
if(y1)
{
if(y2)
{
if(y1->exp<y2->exp)
y1=y1->next;
else if(y1->exp==y2->exp)
{
y1->coef+=y2->coef;
if(y1->coef==0)
{
for(p=h1;p->next!=y1;p=p->next);
p->next=y1->next;
free(y1);
y1=p->next;
}
else
y1=y1->next;
y2=y2->next;
}
else if(y1->exp>y2->exp)
{
for(p=h1;p->next!=y1;p=p->next);
q=(struct node*)malloc(sizeof(struct node));
q->exp=y2->exp;
q->coef=y2->coef;
q->next=y1;
p->next=q;
y2=y2->next;
}
}
else
return;
}
else if(y2)
do{
q=(struct node*)malloc(sizeof(struct node));
q->exp=y2->exp;
q->coef=y2->coef;
q->next=NULL;
for(p=h1;p->next!=y1;p=p->next);
p->next=q;
y1=q;
y2=y2->next;
}while(y2);
else
return;
}
void print_node(struct node*h)
{
if(h->next==NULL)
printf("y=0\n");
else
{
printf("y=");
for(;h->next;)
{
h=h->next;
if(h->exp==0)
{
printf("%f",h->coef);
if(h->next&&h->next->coef>0.0)
printf("+");
}
else
{
printf("%fx%d",h->coef,h->exp);
if(h->next&&h->next->coef>0.0)
printf("+");
}
}
printf("\n");
}
}
main()
{
struct node*y1=(struct node*)malloc(sizeof(struct node));
struct node*y2=(struct node*)malloc(sizeof(struct node));
y1=init_node();
printf("第一个多项式为:\n");
print_node(y1);
y2=init_node();
printf("第二个多项式为:\n");
print_node(y2);
printf("两个多项式的和为:\n");
add_node(y1,y2);
print_node(y1);
}
5、测试数据与运行结果
输入多项式系数和指数后计算得到结果的截图为:
6、实验总结
此次上机实验应用了链表实现了一次实际操作,完成了一个一元多项式的简单相加,不仅对此次编译程序的算法思想有了新的认识,还让我深刻的体会到了链表的重要性以及其应用的方便,并且对指针加深了印象,应用了书本中的算法思想,对我以后的编译以及完成新的程序有很大的帮助。