自动化外文翻译
自动化专业外文翻译---模糊逻辑控制机器人走迷宫
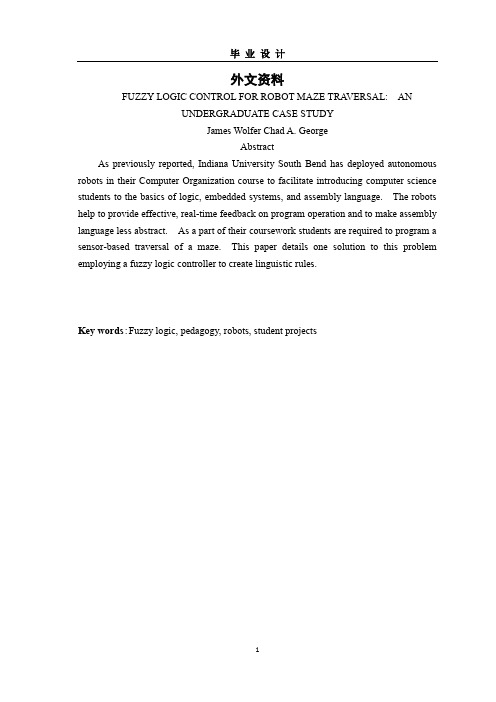
外文资料FUZZY LOGIC CONTROL FOR ROBOT MAZE TRA VERSAL: ANUNDERGRADUATE CASE STUDYJames Wolfer Chad A. GeorgeAbstractAs previously reported, Indiana University South Bend has deployed autonomous robots in their Computer Organization course to facilitate introducing computer science students to the basics of logic, embedded systems, and assembly language. The robots help to provide effective, real-time feedback on program operation and to make assembly language less abstract. As a part of their coursework students are required to program a sensor-based traversal of a maze. This paper details one solution to this problem employing a fuzzy logic controller to create linguistic rules.Key words:Fuzzy logic, pedagogy, robots, student projectsINTRODUCTIONAssembly language programming in a computer science environment is often taught using abstract exercises to illustrate concepts and encourage student proficiency.To augment this approach we have elected to provide hands-on, real-world experience to our students by introducing robots into our assembly language class.Observing the physical action of robots can generate valuable feedback and have real-world consequences – robots hitting walls make students instantly aware of program errors, for example.It also provides insight into the realities of physical machines such as motor control, sensor calibration, and noise. To help provide a meaningful experience for our computer organization students, we reviewed the course with the following objectives in mind:• Expand the experience of our students in a manner that enhances the student's insight, provides a hands-on, visual, environment for them to learn, and forms an integrated component for future classes.•Remove some of the abstraction inherent in the assembly language class. Specifically, to help enhance the error detection environment.• Provide a kinesthetic aspect to our pedagogy.• Build student expertise early in their program that could lead to research projects and advanced classroom activities later in their program. Specifically, in this case, to build expertise to support later coursework in intelligent systems and robotics.As one component in meeting these objectives we, in cooperation with the Computer Science department, the Intelligent Systems Laboratory, and the University Center for Excellence in Teaching, designed a robotics laboratory to support the assembly language portion of the computer organization class as described in [1].The balance of this report describes one example project resulting from this environment. Specifically, we describe the results of a student project developing an assembly language fuzzy engine, membership function creation, fuzzy controller, and resulting robot behavior in a Linux-based environment.We also describe subsequent software devlopment in C# under Windows, including graphical membership tuning, real-time display of sensor activation, and fuzzy controller system response. Collectively these tools allow for robust controller development, assemblylanguage support, and an environment suitable for effective classroom and publicdisplay.BACKGROUNDRobots have long been recognized for their potential educational utility, with examples ranging from abstract, simulated, robots, such as Karel[2] and Turtle[3] for teaching programming and geometry respectively, to competitive events such as robotic soccer tournaments[4].As the cost of robotics hardware has decreased their migration into the classroom has accelerated [5, 6]. Driven by the combined goals for this class and the future research objectives, as well as software availability, we chose to use off-the-shelf, Khepera II, robots from K-Team[7].SIMULATED ROBOT DIAGRAMThe K-Team Kephera II is a small, two-motor robot which uses differential wheel speed for steering. Figure 1 shows a functional diagram of the robot. In addition to thetwo motors it includes a series of eight infrared sensors, six along the “front” and two in the “back”of the robot. This robot also comes with an embedded system-call library, a variety of development tools, and the availability of several simulators. The embedded code in the Khepera robots includes a relatively simple, but adequate, command level interface which communicates with the host via a standard serial port. This allows students to write their programs using the host instruction set (Intel Pentium in this case), send commands, and receive responses such as sensor values, motor speed and relative wheel position.We also chose to provide a Linux-based programming environment to our students by adapting and remastering the Knoppix Linux distribution [9]. Our custom distribution supplemented Knoppix with modified simulators for the Khepera, the interface library (including source code),manuals, and assembler documentation. Collectively, this provides a complete development platform.The SIM Kheperasimulator[8] includes source code in C, and provides a workable subset of the native robot command language. It also has the ability to redirect input and output to the physical robot from the graphics display. Figure 2 shows the simulated Khepera robot in a maze environment and Figure 3 shows an actual Khepera in a physical maze. To provide a seamless interface to the simulator and robots we modified the original simulator to more effectively communicate through a pair of Linuxpipes, and we developed a small custom subroutine library callable from the student's assembly language programs.Assignments for the class range from initial C assignments to call the robot routines to assembly language assignments culminating in the robot traversing the maze. FUZZY CONTROLLEROne approach to robot control, fuzzy logic, attempts to encapsulate important aspects of human decision making. By forming a representation tolerant of vague, imprecise, ambiguous, and perhaps missing information fuzzy logic enhances the ability to deal with real-world problems. Furthermore, by empirically modeling a system engineering experience and intuition can be incorporated into a final design.Typical fuzzy controller design [10] consists of:• Defining the control objectives and criteria• Determining the input and output relationships• Creating fuzzy membership functions, along withsubsequent rules, to encapsulate a solution fromintput to output.• Apply necessary input/output conditioning• Test, evaluate, and tune the resulting system.Figure 4 illustrates the conversion from sensor input to a fuzzy-linguistic value. Given three fuzzy possibilities, …too close‟, …too far‟, and …just right‟, along with a sensor reading we can ascertain the degree to which the sensor reading belongs to each of these fuzzy terms. Note that while Figure 4 illustrates a triangular membership set, trapezoids and other shapes are also common.Once the inputs are mapped to their corresponding fuzzy sets the fuzzy attributes are used, expert system style, to trigger rules governing the consequent actions, in this case, of the robot.For example, a series of rules for a robot may include:• If left-sensor is too close and right sensor is too far then turn right.• If left sensor is just right and forward sensor is too far then drive straight.• If left sensor is too far and forward sensor is too far then turn left.• If forward sensor is close then turn right sharply.The logical operators …and‟, …or‟, and …not‟ are calculated as follows: …and‟ represents set intersection and is calculated as the minimum value, …or‟ is calculated as the maximum value or the union of the sets, and …not‟ finds the inverse of the set, calculated as 1.0-fitness.Once inputs have been processed and rules applied, the resulting fuzzy actions must be mapped to real-world control outputs. Figure 5 illustrates this process. Here output is computed as the coordinate of the centroid of the aggregate area of the individual membership sets along the horizontal axis.ASSEMBLY LANGUAGE IMPLEMENTATIONTwo implementations of the fuzzy robot controller were produced. The first was written in assembly language for the Intel cpu architecture under the Linux operating system, the second in C# under Windows to provide a visually intuitive interface for membership set design and public demonstration.Figure 6 shows an excerpt of pseudo-assembly language program. The actual program consists of approximately eight hundred lines of hand-coded assembly language. In the assembly language program subroutine calls are structured with parameters pushed onto the stack. Note that the code for pushing parameters has been edited from this example to conserve space and to illustrate the overall role of the controller. In this code-fragment the …open_pipes‟ routine establishes contact with the simulator or robot. Once communication is established, a continous loop obtains sensor values, encodes them as fuzzy inputs, interprets them through the rule base to linguistic output members which are then converted to control outputs which are sent to the robot. The bulk of the remaining code implements the fuzzy engine itself.FUZZY CONTROLLER MAIN LOOPMembership sets were manually defined to allow the robot to detect and track walls, avoid barriers, and negotiate void spaces in it field of operation. Using this controller, both the simulated robot and the actual Khepera successfully traversed a variety of maze configurations.ASSEMBLY LANGUAGE OBSERV ATIONSWhile implementing the input fuzzification and output defuzzification in assembly language was tedious compared with the same task in a high level language, the logic engine proved to be well suited to description in assembly language.The logic rules were defined in a type of psuedo-code using …and‟, …or‟, …not‟ as operators and using the fuzzy input and output membership sets as parameters. With the addition of input, output and flow control operators, the assembly language logic engine simply had to evaluate these psuedo-code expressions in order to map fuzzy inputs memberships to fuzzy output memberships.Other than storing the current membership fitness values from the inputfuzzyfication, the only data structure needed for the logic engine is a stack to hold intermediate calculations. This is convenient under assembly language since the CPUs stack is immediately available as well as the nescesary stack operators.There were seven commands implemented by the logic rule interpreter: IN, OUT, AND, OR, NOT, DONE, and EXIT.•IN – reads the current fitness from an input membership set and places the value on the stack.•OUT – assigns the value on the top of the stack as the fitness value of an output membership set if it is greater than the existing fitness value for that set.•AND – performs the intersection operation by replacing the top two elements on the stack with the minimum element.•OR – performs the union operation by replace the top two elements on the stack with their maximum.•NOT – replaces the top value on the stack with its compliment.•DONE – pops the top value off the stack to prepare for the next rule•EXIT – signals the end of the logic rule definition and exits the interpreter.As an example the logic rule “If left-sensor is too close and right sensor is too far then turn right”, might be define d by the following fuzzy logic psuedo-code: IN, left_sensor[ TOO_CLOSE ]IN, right_sensor[ TOO_FAR ] ANDOUT, left_wheel[ FWD ]OUT, right_wheel[ STOP ]DONEEXITBy utilizing the existing CPU stack and implementing the logic engine as anpsuedo-code interpreter, the assembly language version is capable of handling arbitrarily complicated fuzzy rules composed of the simple logical operators provided. IMPLEMENTATIONWhile the assembly language programming was the original focus of the project, ultimately we felt that a more polished user interface was desirable for membership set design, fuzzy rule definition, and controller response monitoring. To provide these facilities the fuzzy controller was reimplemented in C# under Windows. through 10 illustrate the capabilities of the resulting software. Specifically, Figure 7 illustrates user interface for membership defination, in this case …near‟. Figure 8 illustrates theinterface for defining the actual fuzzy rules. Figure 9 profiles the output response with respect to a series of simulated inputs. Finally, real-time monitoring of the system is also implemented as illustrated in 10 which shows the robot sensor input values.Since the Khepera simulator was operating system specific, the C# program controls the robot directly. Again, the robot was successful at navigating the maze using a controller specified with this interface.SUMMARYTo summarize, we have developed a student-centric development environment for teaching assembly language programming. As one illustration of its potential we profiled a project implementing a fuzzy-logic engine and controller, along with a subsequent implementation in the C# programming language. Together these projects help to illustrate the viability of a robot-enhanced environment for assembly language programming.REFERENCES[1] Wolfer, J &Rababaah, H. R. A., “Creating a Hands-On Robot Environment for Teaching Assembly Language Programming”, Global Conference on Engineering and Technology Education, 2005[2] Pattic R.E., Karel the Robot: a gentle introduction to the art of programming, 2nd edition. Wiley, 1994[3] Abelson H. and diSessa A., Turtle geometry: the computer as a medium for exploring mathematics. MIT Press, 1996[4] Amirijoo M., Tesanovic A., and Nadjm-Tehrani S., “Raising motivation in real-time laboratories: the soccer scenario” in SIGCSE Technical Symposium on Computer Sciences Education, pp. 265-269, 2004.[5] Epp E.C., “Robot control and embedded systems on inexpensive linux platforms workshop,” in SIGCSE Technical Symposium on Computer Science Education, p. 505, 2004[6] Fagin B. and Merkle L., “Measuring the effectiveness of robots in teaching computer science,” in SIGCSE Technical Symposium on Computer Science Education, PP. 307-311, 2003.[7] K-Team Khepera Robots, , accessed 09/06/05.[8] Michel O., “Khepera Simulator package version 2.0: Freeware mobile robot simulator written at the university of nice Sophia-Antipolis by Olivier Michel. Downloadable from the world wide web. http://diwww.epfl.ch/lami/team/michel/khep-sim, accessed 09/06/05.[9] Knoppix Official Site, , accessed 09/06/05.[10] Earl Cox., The Fuzzy Systems Handbook, Academic Press, New York, 1999.模糊逻辑控制机器人走迷宫James Wolfer Chad A. George摘要美国印第安纳大学南本德已部署在他们的计算机组织课程自主机器人,以方便学生介绍计算机科学逻辑的基础知识,嵌入式系统和汇编语言。
自动化专业英语翻译

自动化专业英语翻译A: Electrical NetworksAn electrical circuit or network is composed of elements such as resistors, inductors,and capacitors connected together in some manner. If the network contains no energy sources, such as batteries or electrical generators, it is known as a passive network.On the other hand, if one or more energy sources are present, the resultant combination is an active network. In studying the behavior of an electrical network, we are interested in determining the voltages and currents that exist within the circuit. Since a network is composed of passive circuit elements, we must first define theelectrical characteristics of these elements.一个电路由一些元件,如电阻、电感和电容,以某种方式连接组成。
如果电路中不包括电源,如电池或者发电机,则称为无源网络。
另一方面,如果电路中有一个或多个电源,称为有源网络。
在研究电路的行为中,确定存在于电路中的电压和电流是我们感兴趣的。
由于一个电路网络由无源元件组成,我们必须首先定义这些元件的电的特性。
自动化专业常用英语词汇
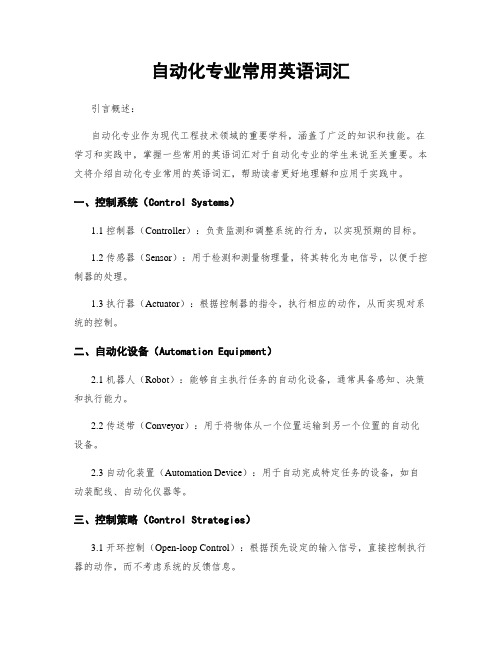
自动化专业常用英语词汇引言概述:自动化专业作为现代工程技术领域的重要学科,涵盖了广泛的知识和技能。
在学习和实践中,掌握一些常用的英语词汇对于自动化专业的学生来说至关重要。
本文将介绍自动化专业常用的英语词汇,帮助读者更好地理解和应用于实践中。
一、控制系统(Control Systems)1.1 控制器(Controller):负责监测和调整系统的行为,以实现预期的目标。
1.2 传感器(Sensor):用于检测和测量物理量,将其转化为电信号,以便于控制器的处理。
1.3 执行器(Actuator):根据控制器的指令,执行相应的动作,从而实现对系统的控制。
二、自动化设备(Automation Equipment)2.1 机器人(Robot):能够自主执行任务的自动化设备,通常具备感知、决策和执行能力。
2.2 传送带(Conveyor):用于将物体从一个位置运输到另一个位置的自动化设备。
2.3 自动化装置(Automation Device):用于自动完成特定任务的设备,如自动装配线、自动化仪器等。
三、控制策略(Control Strategies)3.1 开环控制(Open-loop Control):根据预先设定的输入信号,直接控制执行器的动作,而不考虑系统的反馈信息。
3.2 闭环控制(Closed-loop Control):根据系统的反馈信息,调整控制器的输出信号,以实现对系统的精确控制。
3.3 模糊控制(Fuzzy Control):基于模糊逻辑理论,将模糊的输入转化为模糊的输出,用于处理复杂的非线性系统。
四、通信协议(Communication Protocols)4.1 以太网(Ethernet):一种常用的局域网通信协议,用于实现设备之间的数据传输和通信。
4.2 控制网(ControlNet):一种用于工业自动化领域的网络通信协议,支持实时数据传输和设备控制。
4.3 无线通信(Wireless Communication):通过无线信号进行数据传输和通信的技术,如Wi-Fi、蓝牙等。
自动化专业英语全文翻译

《自动化专业英语教程》-王宏文主编-全文翻译PART 1Electrical and Electronic Engineering BasicsUNIT 1A Electrical Networks ————————————3B Three-phase CircuitsUNIT 2A The Operational Amplifier ———————————5B TransistorsUNIT 3A Logical Variables and Flip-flop ——————————8B Binary Number SystemUNIT 4A Power Semiconductor Devices ——————————11B Power Electronic ConvertersUNIT 5A Types of DC Motors —————————————15B Closed-loop Control of DC DriversUNIT 6A AC Machines ———————————————19B Induction Motor DriveUNIT 7A Electric Power System ————————————22B Power System AutomationPART 2Control TheoryUNIT 1A The World of Control ————————————27B The Transfer Function and the Laplace Transformation —————29 UNIT 2A Stability and the Time Response —————————30B Steady State—————————————————31 UNIT 3A The Root Locus —————————————32B The Frequency Response Methods: Nyquist Diagrams —————33 UNIT 4A The Frequency Response Methods: Bode Piots —————34B Nonlinear Control System 37UNIT 5 A Introduction to Modern Control Theory 38B State Equations 40UNIT 6 A Controllability, Observability, and StabilityB Optimum Control SystemsUNIT 7 A Conventional and Intelligent ControlB Artificial Neural NetworkPART 3 Computer Control TechnologyUNIT 1 A Computer Structure and Function 42B Fundamentals of Computer and Networks 43UNIT 2 A Interfaces to External Signals and Devices 44B The Applications of Computers 46UNIT 3 A PLC OverviewB PACs for Industrial Control, the Future of ControlUNIT 4 A Fundamentals of Single-chip Microcomputer 49B Understanding DSP and Its UsesUNIT 5 A A First Look at Embedded SystemsB Embedded Systems DesignPART 4 Process ControlUNIT 1 A A Process Control System 50B Fundamentals of Process Control 52UNIT 2 A Sensors and Transmitters 53B Final Control Elements and ControllersUNIT 3 A P Controllers and PI ControllersB PID Controllers and Other ControllersUNIT 4 A Indicating InstrumentsB Control PanelsPART 5 Control Based on Network and InformationUNIT 1 A Automation Networking Application AreasB Evolution of Control System ArchitectureUNIT 2 A Fundamental Issues in Networked Control SystemsB Stability of NCSs with Network-induced DelayUNIT 3 A Fundamentals of the Database SystemB Virtual Manufacturing—A Growing Trend in AutomationUNIT 4 A Concepts of Computer Integrated ManufacturingB Enterprise Resources Planning and BeyondPART 6 Synthetic Applications of Automatic TechnologyUNIT 1 A Recent Advances and Future Trends in Electrical Machine DriversB System Evolution in Intelligent BuildingsUNIT 2 A Industrial RobotB A General Introduction to Pattern RecognitionUNIT 3 A Renewable EnergyB Electric VehiclesUNIT 1A 电路电路或电网络由以某种方式连接的电阻器、电感器和电容器等元件组成。
自动化中英文对照表
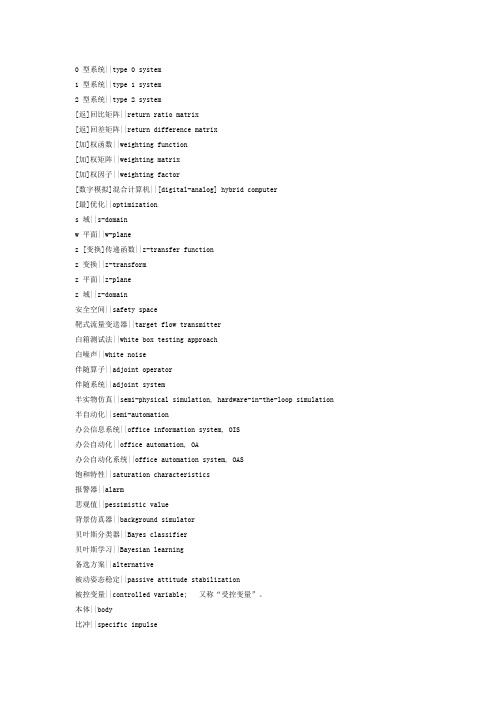
0 型系统||type 0 system1 型系统||type 1 system2 型系统||type 2 system[返]回比矩阵||return ratio matrix[返]回差矩阵||return difference matrix[加]权函数||weighting function[加]权矩阵||weighting matrix[加]权因子||weighting factor[数字模拟]混合计算机||[digital-analog] hybrid computer[最]优化||optimizations 域||s-domainw 平面||w-planez [变换]传递函数||z-transfer functionz 变换||z-transformz 平面||z-planez 域||z-domain安全空间||safety space靶式流量变送器||target flow transmitter白箱测试法||white box testing approach白噪声||white noise伴随算子||adjoint operator伴随系统||adjoint system半实物仿真||semi-physical simulation, hardware-in-the-loop simulation 半自动化||semi-automation办公信息系统||office information system, OIS办公自动化||office automation, OA办公自动化系统||office automation system, OAS饱和特性||saturation characteristics报警器||alarm悲观值||pessimistic value背景仿真器||background simulator贝叶斯分类器||Bayes classifier贝叶斯学习||Bayesian learning备选方案||alternative被动姿态稳定||passive attitude stabilization被控变量||controlled variable; 又称“受控变量”。
自动化专业-外文文献-英文文献-外文翻译-plc方面

1、外文原文(复印件)A: Fundamentals of Single-chip MicrocomputerTh e si ng le-ch i p mi cr oc om pu ter is t he c ul mi nat i on o f bo th t h e d ev el op me nt o f th e d ig it al com p ut er an d t he int e gr at ed ci rc ui ta r gu ab ly th e t ow m os t s i gn if ic ant i nv en ti on s o f t h e 20t h c en tu ry[1].Th es e to w t ype s o f a rc hi te ct ur e a re fo un d i n s i ng le—ch ip m i cr oc om pu te r。
S o me em pl oy th e s p li t p ro gr am/d at a me mo ry of t he H a rv ar d ar ch it ect u re, sh ow n in Fi g.3-5A—1,ot he r s fo ll ow t hep h il os op hy, wi del y a da pt ed f or ge n er al—pu rp os e c o mp ut er s an dm i cr op ro ce ss or s, of ma ki ng no lo gi c al di st in ct io n be tw ee n p ro gr am a n d da ta m em or y a s i n th e Pr in cet o n ar ch it ec tu re,sh ow n in F ig。
3-5A-2.In g en er al te r ms a s in gl e—ch i p mi cr oc om pu ter isc h ar ac te ri zed b y the i nc or po ra tio n of al l t he uni t s o f a co mp ut er i n to a s in gl e de v i ce,as s ho wn i n F ig3—5A—3。
自动化英语
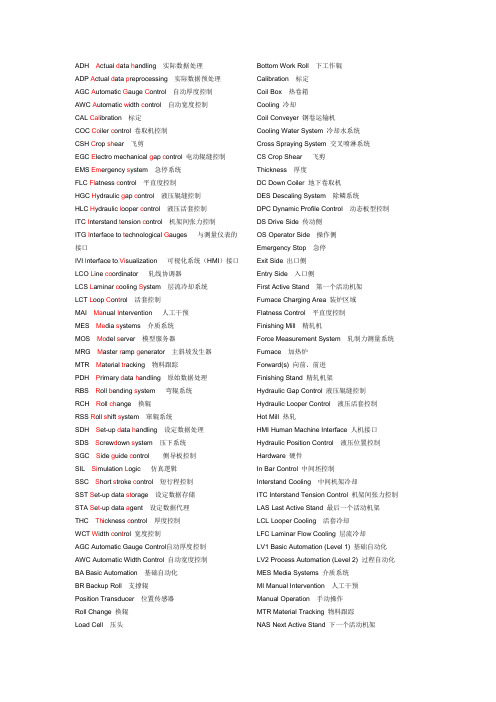
ADH A ctual d ata h andling 实际数据处理ADP A ctual d ata p reprocessing 实际数据预处理AGC A utomatic G auge C ontrol 自动厚度控制AWC A utomatic w idth c ontrol 自动宽度控制CAL Cal ibration 标定COC Co iler c ontrol 卷取机控制CSH C rop sh ear 飞剪EGC E lectro mechanical g ap c ontrol 电动辊缝控制EMS Em ergency s ystem 急停系统FLC Fl atness c ontrol 平直度控制HGC H ydraulic g ap c ontrol 液压辊缝控制HLC H ydraulic l ooper c ontrol 液压活套控制ITC I nterstand t ension c ontrol 机架间张力控制ITG I nterface to t echnological G auges 与测量仪表的接口IVI I nterface to Vi sualization 可视化系统(HMI)接口LCO L ine co ordinator 轧线协调器LCS L aminar c ooling S ystem 层流冷却系统LCT L oop C on t rol 活套控制MAI Ma nual I ntervention 人工干预MES Me dia s ystems 介质系统MOS Mo del s erver 模型服务器MRG M aster r amp g enerator 主斜坡发生器MTR M aterial tr acking 物料跟踪PDH P rimary d ata h andling 原始数据处理RBS R oll b ending s ystem 弯辊系统RCH R oll ch ange 换辊RSS R oll s hift s ystem 窜辊系统SDH S et-up d ata h andling 设定数据处理SDS S crew d own s ystem 压下系统SGC S ide g uide c ontrol 侧导板控制SIL Si mulation L ogic 仿真逻辑SSC S hort s troke c ontrol 短行程控制SST S et-up data st orage 设定数据存储STA S e t-up data a gent 设定数据代理THC Th ickness c ontrol 厚度控制WCT W idth c on t rol 宽度控制AGC Automatic Gauge Control自动厚度控制AWC Automatic Width Control 自动宽度控制BA Basic Automation 基础自动化BR Backup Roll 支撑辊Position Transducer 位置传感器Roll Change 换辊Load Cell 压头Bottom Work Roll 下工作辊Calibration 标定Coil Box 热卷箱Cooling 冷却Coil Conveyer 钢卷运输机Cooling Water System 冷却水系统Cross Spraying System 交叉喷淋系统CS Crop Shear 飞剪Thickness 厚度DC Down Coiler 地下卷取机DES Descaling System 除鳞系统DPC Dynamic Profile Control 动态板型控制DS Drive Side 传动侧OS Operator Side 操作侧Emergency Stop 急停Exit Side 出口侧Entry Side 入口侧First Active Stand 第一个活动机架Furnace Charging Area 装炉区域Flatness Control 平直度控制Finishing Mill 精轧机Force Measurement System 轧制力测量系统Furnace 加热炉Forward(s) 向前、前进Finishing Stand 精轧机架Hydraulic Gap Control 液压辊缝控制Hydraulic Looper Control 液压活套控制Hot Mill 热轧HMI Human Machine Interface 人机接口Hydraulic Position Control 液压位置控制Hardware 硬件In Bar Control 中间坯控制Interstand Cooling 中间机架冷却ITC Interstand Tension Control 机架间张力控制LAS Last Active Stand 最后一个活动机架LCL Looper Cooling 活套冷却LFC Laminar Flow Cooling 层流冷却LV1 Basic Automation (Level 1) 基础自动化LV2 Process Automation (Level 2) 过程自动化MES Media Systems 介质系统MI Manual Intervention 人工干预Manual Operation 手动操作MTR Material Tracking 物料跟踪NAS Next Active Stand 下一个活动机架OLS Oil Lubrication System 润滑系统Operation Mode 操作模式Profile and Flatness Control 板型和平直度控制Pressure Measurement System 压力测量系统Profile Control 板型控制Pinch Roll Unit 夹送辊单元Pulpit 操作台Quick Stop 快停Reset 复位Roll Change 换辊Runout Table 输出辊道Request 请求Roughing Mill 粗轧机Roll Shifting Systems 窜辊系统Roller Table 辊道Roughing Stand (Rougher) 粗轧机架SDS Screwdown System 压下系统SG Side Guides 侧导板Position Measurement System 位置测量系统SSC Short Stroke Control 短行程控制Strip Tracking 带钢跟踪Servo-Valve 伺服阀Swivel 旋转Software 软件Slab Weighing System 板坯称重系统Top Backup Roll 上支撑辊Tension System 张力系统THC Thickness Control 厚度控制TWR Top Work Roll 上工作辊Work Roll Balancing 工作辊平衡Work Roll Cooling 工作辊冷却Weighing Machine 称重机Work Roll 工作辊WRB Work Roll Balancing/Bending System 工作辊平衡/弯辊系统Work Roll Change 工作辊换辊Work Roll Positive Bending 工作辊正弯辊WRS Work Roll Shifting System 工作辊窜辊系统Roll Cooling and Interstand Cooling 轧辊冷却和中间机架冷却Tension Measurement System 张力测量系统Compensation 补偿Setpoint 设定值Manual intervention 人工干预Lead factor 超前因数Lag factor 滞后因数Increase 增加decrease 减小pass through operation mode 直通操作模式Furnace加热炉descaler 除鳞箱enter table入口辊道bar reject device 中间坯剔除装置rollertable辊道work roll 工作辊backup roll 支撑辊mill stand 轧机机架sensor 传感器position transducer 位置传感器pressure transducer 压力传感器valve 阀operator panel(OP) 操作面板main control desk 主控台rougher 粗轧机edger 立辊轧机strip带钢entry side guide入口侧导板entry chute with deflector roll入口导槽及偏转辊bending device弯曲设备coiling station卷取站forming roll成型辊pusher推卷器stabilizer稳定器peeler开卷器uncoiling station开卷站heat preservation side guide保湿侧导板holdback pin开尾销pinch roll unit夹送辊单元hold back roll缓冲辊local desk就地操作台limit switch极限开关coil box热卷箱pass schedules轧制表push button按钮cradle roll 托卷辊Oscillate 摆动scrap废料spray nozzle 喷嘴stand zeroing机架调零mechanical 机械的synchronize同步hydraulic cylinder 液压缸Proportional valve 比例阀cylinder 液压缸remote 远程conveyor 运输机Coil discharge 卸卷pulse 脉冲telegram 报文Ethernet-bus 以太网总线mandrel outboard bearing 芯轴外支撑even passes 偶道次odd passes 奇道次。
自动化外文参考文献(精选120个最新)
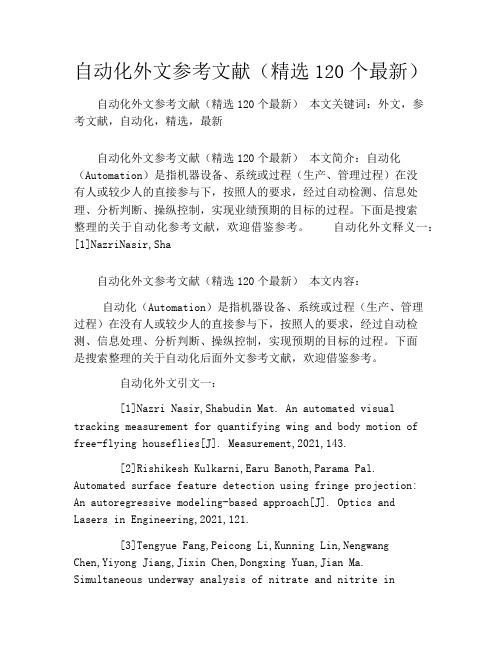
自动化外文参考文献(精选120个最新)自动化外文参考文献(精选120个最新)本文关键词:外文,参考文献,自动化,精选,最新自动化外文参考文献(精选120个最新)本文简介:自动化(Automation)是指机器设备、系统或过程(生产、管理过程)在没有人或较少人的直接参与下,按照人的要求,经过自动检测、信息处理、分析判断、操纵控制,实现业绩预期的目标的过程。
下面是搜索整理的关于自动化参考文献,欢迎借鉴参考。
自动化外文释义一:[1]NazriNasir,Sha自动化外文参考文献(精选120个最新)本文内容:自动化(Automation)是指机器设备、系统或过程(生产、管理过程)在没有人或较少人的直接参与下,按照人的要求,经过自动检测、信息处理、分析判断、操纵控制,实现预期的目标的过程。
下面是搜索整理的关于自动化后面外文参考文献,欢迎借鉴参考。
自动化外文引文一:[1]Nazri Nasir,Shabudin Mat. An automated visual tracking measurement for quantifying wing and body motion of free-flying houseflies[J]. Measurement,2021,143.[2]Rishikesh Kulkarni,Earu Banoth,Parama Pal. Automated surface feature detection using fringe projection: An autoregressive modeling-based approach[J]. Optics and Lasers in Engineering,2021,121.[3]Tengyue Fang,Peicong Li,Kunning Lin,NengwangChen,Yiyong Jiang,Jixin Chen,Dongxing Yuan,Jian Ma. Simultaneous underway analysis of nitrate and nitrite inestuarine and coastal waters using an automated integrated syringe-pump-based environmental-water analyzer[J]. Analytica Chimica Acta,2021,1076.[4]Shengfeng Chen,Jian Liu,Xiaosong Zhang,XinyuSuo,Enhui Lu,Jilong Guo,Jianxun Xi. Development ofpositioning system for Nuclear-fuel rod automated assembly[J]. Robotics and Computer Integrated Manufacturing,2021,61.[5]Cheng-Ta Lee,Yu-Ching Lee,Albert Y. Chen. In-building automated external defibrillator location planning and assessment through building information models[J]. Automation in Construction,2021,106.[6]Torgeir Aleti,Jason I. Pallant,Annamaria Tuan,Tom van Laer. Tweeting with the Stars: Automated Text Analysis of the Effect of Celebrity Social Media ications on ConsumerWord of Mouth[J]. Journal of Interactive Marketing,2021,48.[7]Daniel Bacioiu,Geoff Melton,MayorkinosPapaelias,Rob Shaw. Automated defect classification of SS304 TIG welding process using visible spectrum camera and machine learning[J]. NDT and E International,2021,107.[8]Marcus von der Au,Max Schwinn,KatharinaKuhlmeier,Claudia Büchel,Bj?rn Meermann. Development of an automated on-line purification HPLC single cell-ICP-MS approach for fast diatom analysis[J]. Analytica ChimicaActa,2021,1077.[9]Jitendra Mehar,Ajam Shekh,Nethravathy M. U.,R. Sarada,Vikas Singh Chauhan,Sandeep Mudliar. Automation ofpilot-scale open raceway pond: A case study of CO 2 -fed pHcontrol on Spirulina biomass, protein and phycocyanin production[J]. Journal of CO2 Utilization,2021,33.[10]John T. Sloop,Henry J.B. Bonilla,TinaHarville,Bradley T. Jones,George L. Donati. Automated matrix-matching calibration using standard dilution analysis withtwo internal standards and a simple three-port mixing chamber[J]. Talanta,2021,205.[11]Daniel J. Spade,Cathy Yue Bai,ChristyLambright,Justin M. Conley,Kim Boekelheide,L. Earl Gray. Corrigendum to “Validation of an automated counting procedure for phthalate-induced testicular multinucleated germ cells” [Toxicol. Lett. 290 (2021) 55–61][J]. Toxicology Letters,2021,313.[12]Christian P. Janssen,Shamsi T. Iqbal,Andrew L. Kun,Stella F. Donker. Interrupted by my car? Implications of interruption and interleaving research for automatedvehicles[J]. International Journal of Human - Computer Studies,2021,130.[13]Seunguk Lee,Si Kuan Thio,Sung-Yong Park,Sungwoo Bae. An automated 3D-printed smartphone platform integrated with optoelectrowetting (OEW) microfluidic chip for on-site monitoring of viable algae in water[J]. Harmful Algae,2021,88.[14]Yuxia Duan,Shicai Liu,Caiqi Hu,Junqi Hu,Hai Zhang,Yiqian Yan,Ning Tao,Cunlin Zhang,Xavier Maldague,Qiang Fang,Clemente Ibarra-Castanedo,Dapeng Chen,Xiaoli Li,Jianqiao Meng. Automated defect classification in infrared thermography based on a neural network[J]. NDT and E International,2021,107.[15]Alex M. Pagnozzi,Jurgen Fripp,Stephen E. Rose. Quantifying deep grey matter atrophy using automated segmentation approaches: A systematic review of structural MRI studies[J]. NeuroImage,2021,201.[16]Jin Ye,Zhihong Xuan,Bing Zhang,Yu Wu,LiLi,Songshan Wang,Gang Xie,Songxue Wang. Automated analysis of ochratoxin A in cereals and oil by iaffinity magnetic beads coupled to UPLC-FLD[J]. Food Control,2021,104.[17]Anne Bech Risum,Rasmus Bro. Using deep learning to evaluate peaks in chromatographic data[J].Talanta,2021,204.[18]Faris Elghaish,Sepehr Abrishami,M. Reza Hosseini,Soliman Abu-Samra,Mark Gaterell. Integrated project delivery with BIM: An automated EVM-based approach[J]. Automation in Construction,2021,106.[19]Carl J. Pearson,Michael Geden,Christopher B. Mayhorn. Who's the real expert here? Pedigree's unique bias on trust between human and automated advisers[J]. Applied Ergonomics,2021,81.[20]Vibhas Mishra,Dani?l M.J. Peeters,Mostafa M. Abdalla. Stiffness and buckling analysis of variablestiffness laminates including the effect of automated fibre placement defects[J]. Composite Structures,2021,226.[21]Jenny S. Wesche,Andreas Sonderegger. When computers take the lead: The automation of leadership[J]. Computers in Human Behavior,2021,101.[22]Murat Ayaz,Hüseyin Yüksel. Design of a new cost-efficient automation system for gas leak detection in industrial buildings[J]. Energy & Buildings,2021,200.[23]Stefan A. Mann,Juliane Heide,Thomas Knott,Razvan Airini,Florin Bogdan Epureanu,Alexandru-FlorianDeftu,Antonia-Teona Deftu,Beatrice Mihaela Radu,Bogdan Amuzescu. Recording of multiple ion current components and action potentials in human induced pluripotent stem cell-derived cardiomyocytes via automated patch-clamp[J]. Journal of Pharmacological and Toxicological Methods,2021,100.[24]Rhar? de Almeida Cardoso,Alexandre Cury,Flavio Barbosa. Automated real-time damage detection strategy using raw dynamic measurements[J]. Engineering Structures,2021,196.[25]Mengmeng Zhong,Tielong Wang,Chengdu Qi,Guilong Peng,Meiling Lu,Jun Huang,Lee Blaney,Gang Yu. Automated online solid-phase extraction liquid chromatography tandem mass spectrometry investigation for simultaneous quantification of per- and polyfluoroalkyl substances, pharmaceuticals and personal care products, and organophosphorus flame retardants in environmental waters[J]. Journal of Chromatography A,2021,1602.[26]Pau Climent-Pér ez,Susanna Spinsante,Alex Mihailidis,Francisco Florez-Revuelta. A review on video-based active and assisted living technologies for automated lifelogging[J]. Expert Systems With Applications,2021,139.[27]William Snyder,Marisa Patti,Vanessa Troiani. An evaluation of automated tracing for orbitofrontal cortexsulcogyral pattern typing[J]. Journal of Neuroscience Methods,2021,326.[28]Juan Manuel Davila Delgado,LukumonOyedele,Anuoluwapo Ajayi,Lukman Akanbi,OlugbengaAkinade,Muhammad Bilal,Hakeem Owolabi. Robotics and automated systems in construction: Understanding industry-specific challenges for adoption[J]. Journal of Building Engineering,2021,26.[29]Mohamed Taher Alrefaie,Stever Summerskill,Thomas W Jackon. In a heart beat: Using driver’s physiological changes to determine the quality of a takeover in highly automated vehicles[J]. Accident Analysis andPrevention,2021,131.[30]Tawseef Ayoub Shaikh,Rashid Ali. Automated atrophy assessment for Alzheimer's disease diagnosis from brain MRI images[J]. Magnetic Resonance Imaging,2021,62.自动化外文参考文献二:[31]Vaanathi Sundaresan,Giovanna Zamboni,Campbell Le Heron,Peter M. Rothwell,Masud Husain,Marco Battaglini,Nicola De Stefano,Mark Jenkinson,Ludovica Griffanti. Automatedlesion segmentation with BIANCA: Impact of population-level features, classification algorithm and locally adaptive thresholding[J]. NeuroImage,2021,202.[32]Ho-Jun Suk,Edward S. Boyden,Ingrid van Welie. Advances in the automation of whole-cell patch clamp technology[J]. Journal of Neuroscience Methods,2021,326.[33]Ivana Duznovic,Mathias Diefenbach,Mubarak Ali,Tom Stein,Markus Biesalski,Wolfgang Ensinger. Automated measuring of mass transport through synthetic nanochannels functionalized with polyelectrolyte porous networks[J]. Journal of Membrane Science,2021,591.[34]James A.D. Cameron,Patrick Savoie,Mary E.Kaye,Erik J. Scheme. Design considerations for the processing system of a CNN-based automated surveillance system[J]. Expert Systems With Applications,2021,136.[35]Ebrahim Azadniya,Gertrud E. Morlock. Automated piezoelectric spraying of biological and enzymatic assays for effect-directed analysis of planar chromatograms[J]. Journal of Chromatography A,2021,1602.[36]Lilla Z?llei,Camilo Jaimes,Elie Saliba,P. Ellen Grant,Anastasia Yendiki. TRActs constrained by UnderLying INfant anatomy (TRACULInA): An automated probabilistic tractography tool with anatomical priors for use in the newborn brain[J]. NeuroImage,2021,199.[37]Kate?ina Fikarová,David J. Cocovi-Solberg,María Rosende,Burkhard Horstkotte,Hana Sklená?ová,Manuel Miró. A flow-based platform hyphenated to on-line liquid chromatography for automatic leaching tests of chemical additives from microplastics into seawater[J]. Journal of Chromatography A,2021,1602.[38]Darko ?tern,Christian Payer,Martin Urschler. Automated age estimation from MRI volumes of the hand[J]. Medical Image Analysis,2021,58.[39]Jacques Blum,Holger Heumann,Eric Nardon,Xiao Song. Automating the design of tokamak experiment scenarios[J]. Journal of Computational Physics,2021,394.[40]Elton F. de S. Soares,Carlos Alberto V.Campos,Sidney C. de Lucena. Online travel mode detection method using automated machine learning and feature engineering[J]. Future Generation Computer Systems,2021,101.[41]M. Marouli,S. Pommé. Autom ated optical distance measurements for counting at a defined solid angle[J].Applied Radiation and Isotopes,2021,153.[42]Yi Dai,Zhen-Hua Yu,Jian-Bo Zhan,Bao-Shan Yue,Jiao Xie,Hao Wang,Xin-Sheng Chai. Determination of starch gelatinization temperatures by an automated headspace gas chromatography[J]. Journal of Chromatography A,2021,1602.[43]Marius Tarp?,Tobias Friis,Peter Olsen,MartinJuul,Christos Georgakis,Rune Brincker. Automated reduction of statistical errors in the estimated correlation functionmatrix for operational modal analysis[J]. Mechanical Systems and Signal Processing,2021,132.[44]Wenxia Dai,Bisheng Yang,Xinlian Liang,ZhenDong,Ronggang Huang,Yunsheng Wang,Wuyan Li. Automated fusionof forest airborne and terrestrial point clouds throughcanopy density analysis[J]. ISPRS Journal of Photogrammetry and Remote Sensing,2021,156.[45]Jyh-Haur Woo,Marcus Ang,Hla Myint Htoon,Donald Tan. Descemet Membrane Endothelial Keratoplasty Versus Descemet Stripping Automated Endothelial Keratoplasty andPenetrating Keratoplasty[J]. American Journal of Ophthalmology,2021,207.[46]F. Wilde,S. Marsen,T. Stange,D. Moseev,J.W. Oosterbeek,H.P. Laqua,R.C. Wolf,K. Avramidis,G.Gantenbein,I.Gr. Pagonakis,S. Illy,J. Jelonnek,M.K. Thumm,W7-X team. Automated mode recovery for gyrotrons demonstrated at Wendelstein 7-X[J]. Fusion Engineering and Design,2021,148.[47]Andrew Kozbial,Lekhana Bhandary,Shashi K. Murthy. Effect of yte seeding density on dendritic cell generation in an automated perfusion-based culture system[J]. Biochemical Engineering Journal,2021,150.[48]Wen-Hao Su,Steven A. Fennimore,David C. Slaughter. Fluorescence imaging for rapid monitoring of translocation behaviour of systemic markers in snap beans for automatedcrop/weed discrimination[J]. Biosystems Engineering,2021,186.[49]Ki-Taek Lim,Dinesh K. Patel,Hoon Se,JanghoKim,Jong Hoon Chung. A fully automated bioreactor system for precise control of stem cell proliferation anddifferentiation[J]. Biochemical Engineering Journal,2021,150.[50]Mitchell L. Cunningham,Michael A. Regan,Timothy Horberry,Kamal Weeratunga,Vinayak Dixit. Public opinion about automated vehicles in Australia: Results from a large-scale national survey[J]. Transportation Research Part A,2021,129.[51]Yi Xie,Qiaobei You,Pingyang Dai,Shuyi Wang,Peiyi Hong,Guokun Liu,Jun Yu,Xilong Sun,Yongming Zeng. How to achieve auto-identification in Raman analysis by spectral feature extraction & Adaptive Hypergraph[J].Spectrochimica Acta Part A: Molecular and Biomolecular Spectroscopy,2021,222.[52]Ozal Yildirim,Muhammed Talo,Betul Ay,Ulas Baran Baloglu,Galip Aydin,U. Rajendra Acharya. Automated detection of diabetic subject using pre-trained 2D-CNN models with frequency spectrum images extracted from heart ratesignals[J]. Computers in Biology and Medicine,2021,113.[53]Marius Kern,Laura Tusa,Thomas Lei?ner,Karl Gerald van den Boogaart,Jens Gutzmer. Optimal sensor selection for sensor-based sorting based on automated mineralogy data[J]. Journal of Cleaner Production,2021,234.[54]Karim Keddadouche,Régis Braucher,Didier L.Bourlès,Mélanie Baroni,Valéry Guillou,La?titia Léanni,Georges Auma?tre. Design and performance of an automated chemical extraction bench for the preparation of 10 Be and 26 Al targets to be analyzed by accelerator mass spectrometry[J]. Nuclear Inst. and Methods in Physics Research, B,2021,456.[55]Christian P. Janssen,Stella F. Donker,Duncan P. Brumby,Andrew L. Kun. History and future of human-automation interaction[J]. International Journal of Human - Computer Studies,2021,131.[56]Victoriya Orlovskaya,Olga Fedorova,Michail Nadporojskii,Raisa Krasikova. A fully automated azeotropic drying free synthesis of O -(2-[ 18 F]fluoroethyl)- l -tyrosine ([ 18 F]FET) using tetrabutylammonium tosylate[J]. Applied Radiation and Isotopes,2021,152.[57]Dinesh Krishnamoorthy,Kjetil Fjalestad,Sigurd Skogestad. Optimal operation of oil and gas production usingsimple feedback control structures[J]. Control Engineering Practice,2021,91.[58]Nick Oliver,Thomas Calvard,Kristina Poto?nik. Safe limits, mindful organizing and loss of control in commercial aviation[J]. Safety Science,2021,120.[59]Bo Sui,Nils Lubbe,Jonas B?rgman. A clustering approach to developing car-to-two-wheeler test scenarios for the assessment of Automated Emergency Braking in China using in-depth Chinese crash data[J]. Accident Analysis and Prevention,2021,132.[60]Ji-Seok Yoon,Eun Young Choi,Maliazurina Saad,Tae-Sun Choi. Automated integrated system for stained neuron detection: An end-to-end framework with a high negative predictive rate[J]. Computer Methods and Programs in Biomedicine,2021,180.自动化外文参考文献八:[61]Min Wang,Barbara E. Glick-Wilson,Qi-Huang Zheng. Facile fully automated radiosynthesis and quality control of O -(2-[ 18 F]fluoroethyl)- l -tyrosine ([ 18 F]FET) for human brain tumor imaging[J]. Applied Radiation andIsotopes,2021,154.[62]Fabian Pütz,Finbarr Murphy,Martin Mullins,LisaO'Malley. Connected automated vehicles and insurance: Analysing future market-structure from a business ecosystem perspective[J]. Technology in Society,2021,59.[63]Victoria A. Banks,Neville A. Stanton,Katherine L. Plant. Who is responsible for automated driving? A macro-level insight into automated driving in the United Kingdom using the Risk Management Framework and Social NetworkAnalysis[J]. Applied Ergonomics,2021,81.[64]Yingjun Ye,Xiaohui Zhang,Jian Sun. Automated vehicle’s behavior decision making using deep reinforcement learning and high-fidelity simulation environment[J]. Transportation Research Part C,2021,107.[65]Hasan Alkaf,Jameleddine Hassine,TahaBinalialhag,Daniel Amyot. An automated change impact analysis approach for User Requirements Notation models[J]. TheJournal of Systems & Software,2021,157.[66]Zonghua Luo,Jiwei Gu,Robert C. Dennett,Gregory G. Gaehle,Joel S. Perlmutter,Delphine L. Chen,Tammie L.S. Benzinger,Zhude Tu. Automated production of a sphingosine-1 phosphate receptor 1 (S1P1) PET radiopharmaceutical [ 11C]CS1P1 for human use[J]. Applied Radiation andIsotopes,2021,152.[67]Sarfraz Qureshi,Wu Jiacheng,Jeroen Anton van Kan. Automated alignment and focusing system for nuclear microprobes[J]. Nuclear Inst. and Methods in Physics Research, B,2021,456.[68]Srikanth Sagar Bangaru,Chao Wang,MarwaHassan,Hyun Woo Jeon,Tarun Ayiluri. Estimation of the degreeof hydration of concrete through automated machine learning based microstructure analysis – A study on effect of image magnification[J]. Advanced Engineering Informatics,2021,42.[69]Fang Tengyue,Li Peicong,Lin Kunning,Chen Nengwang,Jiang Yiyong,Chen Jixin,Yuan Dongxing,Ma Jian. Simultaneous underway analysis of nitrate and nitrite in estuarine and coastal waters using an automated integrated syringe-pump-based environmental-water analyzer.[J]. Analytica chimica acta,2021,1076.[70]Ramos Inês I,Carl Peter,Schneider RudolfJ,Segundo Marcela A. Automated lab-on-valve sequential injection ELISA for determination of carbamazepine.[J]. Analytica chimica acta,2021,1076.[71]Au Marcus von der,Schwinn Max,Kuhlmeier Katharina,Büchel Claudia,Meermann Bj?rn. Development of an automated on-line purification HPLC single cell-ICP-MS approach for fast diatom analysis.[J]. Analytica chimica acta,2021,1077.[72]Risum Anne Bech,Bro Rasmus. Using deep learning to evaluate peaks in chromatographic data.[J].Talanta,2021,204.[73]Spade Daniel J,Bai Cathy Yue,LambrightChristy,Conley Justin M,Boekelheide Kim,Gray L Earl. Corrigendum to "Validation of an automated counting procedure for phthalate-induced testicular multinucleated germ cells" [Toxicol. Lett. 290 (2021) 55-61].[J]. Toxicologyletters,2021,313.[74]Zhong Mengmeng,Wang Tielong,Qi Chengdu,Peng Guilong,Lu Meiling,Huang Jun,Blaney Lee,Yu Gang. Automated online solid-phase extraction liquid chromatography tandem mass spectrometry investigation for simultaneousquantification of per- and polyfluoroalkyl substances, pharmaceuticals and personal care products, and organophosphorus flame retardants in environmental waters.[J]. Journal of chromatography. A,2021,1602.[75]Stein Christopher J,Reiher Markus. autoCAS: A Program for Fully Automated MulticonfigurationalCalculations.[J]. Journal of computationalchemistry,2021,40(25).[76]Alrefaie Mohamed Taher,Summerskill Stever,Jackon Thomas W. In a heart beat: Using driver's physiological changes to determine the quality of a takeover in highly automated vehicles.[J]. Accident; analysis andprevention,2021,131.[77]Shaikh Tawseef Ayoub,Ali Rashid. Automatedatrophy assessment for Alzheimer's disease diagnosis frombrain MRI images.[J]. Magnetic resonance imaging,2021,62.[78]Xie Yi,You Qiaobei,Dai Pingyang,Wang Shuyi,Hong Peiyi,Liu Guokun,Yu Jun,Sun Xilong,Zeng Yongming. How to achieve auto-identification in Raman analysis by spectral feature extraction & Adaptive Hypergraph.[J]. Spectrochimica acta. Part A, Molecular and biomolecular spectroscopy,2021,222.[79]Azadniya Ebrahim,Morlock Gertrud E. Automated piezoelectric spraying of biological and enzymatic assays for effect-directed analysis of planar chromatograms.[J]. Journal of chromatography. A,2021,1602.[80]Fikarová Kate?ina,Cocovi-Solberg David J,Rosende María,Horstkotte Burkhard,Sklená?ová Hana,Miró Manuel. Aflow-based platform hyphenated to on-line liquid chromatography for automatic leaching tests of chemical additives from microplastics into seawater.[J]. Journal of chromatography. A,2021,1602.[81]Moitra Dipanjan,Mandal Rakesh Kr. Automated AJCC (7th edition) staging of non-small cell lung cancer (NSCLC) using deep convolutional neural network (CNN) and recurrent neural network (RNN).[J]. Health information science and systems,2021,7(1).[82]Ramos-Payán María. Liquid - Phase microextraction and electromembrane extraction in millifluidic devices:A tutorial.[J]. Analytica chimica acta,2021,1080.[83]Z?llei Lilla,Jaimes Camilo,Saliba Elie,Grant P Ellen,Yendiki Anastasia. TRActs constrained by UnderLying INfant anatomy (TRACULInA): An automated probabilistic tractography tool with anatomical priors for use in the newborn brain.[J]. NeuroImage,2021,199.[84]Sedghi Gamechi Zahra,Bons Lidia R,Giordano Marco,Bos Daniel,Budde Ricardo P J,Kofoed Klaus F,Pedersen Jesper Holst,Roos-Hesselink Jolien W,de Bruijne Marleen. Automated 3D segmentation and diameter measurement of the thoracic aorta on non-contrast enhanced CT.[J]. European radiology,2021,29(9).[85]Smith Claire,Galland Barbara C,de Bruin Willemijn E,Taylor Rachael W. Feasibility of Automated Cameras to Measure Screen Use in Adolescents.[J]. American journal of preventive medicine,2021,57(3).[86]Lambert Marie-?ve,Arsenault Julie,AudetPascal,Delisle Benjamin,D'Allaire Sylvie. Evaluating an automated clustering approach in a perspective of ongoing surveillance of porcine reproductive and respiratory syndrome virus (PRRSV) field strains.[J]. Infection, genetics and evolution : journal of molecular epidemiology and evolutionary genetics in infectious diseases,2021,73.[87]Slanetz Priscilla J. Does Computer-aided Detection Help in Interpretation of Automated Breast US?[J]. Radiology,2021,292(3).[88]Sander Laura,Pezold Simon,Andermatt Simon,Amann Michael,Meier Dominik,Wendebourg Maria J,Sinnecker Tim,Radue Ernst-Wilhelm,Naegelin Yvonne,Granziera Cristina,Kappos Ludwig,Wuerfel Jens,Cattin Philippe,Schlaeger Regina. Accurate, rapid and reliable, fully automated MRI brainstem segmentation for application in multiple sclerosis and neurodegenerative diseases.[J]. Human brainmapping,2021,40(14).[89]Pajkossy Péter,Sz?ll?si ?gnes,Racsmány Mihály. Retrieval practice decreases processing load of recall: Evidence revealed by pupillometry.[J]. International journal of psychophysiology : official journal of the International Organization of Psychophysiology,2021,143.[90]Kaiser Eric A,Igdalova Aleksandra,Aguirre Geoffrey K,Cucchiara Brett. A web-based, branching logic questionnaire for the automated classification ofmigraine.[J]. Cephalalgia : an international journal of headache,2021,39(10).自动化外文参考文献四:[91]Kim Jin Ju,Park Younhee,Choi Dasom,Kim Hyon Suk. Performance Evaluation of a New Automated Chemiluminescent Ianalyzer-Based Interferon-Gamma Releasing Assay AdvanSure I3 in Comparison With the QuantiFERON-TB Gold In-Tube Assay.[J]. Annals of laboratory medicine,2021,40(1).[92]Yang Shanling,Gao Xican,Liu Liwen,Shu Rui,Yan Jingru,Zhang Ge,Xiao Yao,Ju Yan,Zhao Ni,Song Hongping. Performance and Reading Time of Automated Breast US with or without Computer-aided Detection.[J]. Radiology,2021,292(3).[93]Hung Andrew J,Chen Jian,Ghodoussipour Saum,OhPaul J,Liu Zequn,Nguyen Jessica,Purushotham Sanjay,Gill Inderbir S,Liu Yan. A deep-learning model using automated performance metrics and clinical features to predict urinary continence recovery after robot-assisted radical prostatectomy.[J]. BJU international,2021,124(3).[94]Kim Ryan S,Kim Gene. Double Descemet Stripping Automated Endothelial Keratoplasty (DSAEK): Secondary DSAEK Without Removal of the Failed Primary DSAEK Graft.[J]. Ophthalmology,2021,126(9).[95]Sargent Alexandra,Theofanous Ioannis,Ferris Sarah. Improving laboratory workflow through automated pre-processing of SurePath specimens for human papillomavirus testing with the Abbott RealTime assay.[J]. Cytopathology : official journal of the British Society for Clinical Cytology,2021,30(5).[96]Saba Tanzila. Automated lung nodule detection and classification based on multiple classifiers voting.[J]. Microscopy research and technique,2021,82(9).[97]Ivan D. Welsh,Jane R. Allison. Automated simultaneous assignment of bond orders and formal charges[J]. Journal of Cheminformatics,2021,11(1).[98]Willem Jespers,MauricioEsguerra,Johan ?qvist,Hugo Gutiérrez-de-Terán. QligFEP: an automated workflow for small molecule free energycalculations in Q[J]. Journal of Cheminformatics,2021,11(1).[99]Manav Raj,Robert Seamans. Primer on artificial intelligence and robotics[J]. Journal of OrganizationDesign,2021,8(1).[100]Yvette Pronk,Peter Pilot,Justus M.Brinkman,Ronald J. Heerwaarden,Walter Weegen. Response rate and costs for automated patient-reported outcomes collection alone compared to combined automated and manual collection[J]. Journal of Patient-Reported Outcomes,2021,3(1).[101]Tristan Martin,Ana?s Moyon,Cyril Fersing,Evan Terrier,Aude Gouillet,Fabienne Giraud,BenjaminGuillet,Philippe Garrigue. Have you looked for “stranger things” in your automated PET dose dispensing system? A process and operators qualification scheme[J]. EJNMMI Radiopharmacy and Chemistry,2021,4(1).[102]Manuel Peuster,Michael Marchetti,Ger ardo García de Blas,Holger Karl. Automated testing of NFV orchestrators against carrier-grade multi-PoP scenarios using emulation-based smoke testing[J]. EURASIP Journal on Wireless ications and Networking,2021,2021(1).[103]R. Ferrús,O. Sallent,J. Pérez-Romero,R. Agustí. On the automation of RAN slicing provisioning: solution framework and applicability examples[J]. EURASIP Journal on Wireless ications and Networking,2021,2021(1).[104]Duo Li,Peter Wagner. Impacts of gradual automated vehicle penetration on motorway operation: a comprehensive evaluation[J]. European Transport Research Review,2021,11(1).[105]Abel Gómez,Ricardo J. Rodríguez,María-Emilia Cambronero,Valentín Valero. Profiling the publish/subscribe paradigm for automated analysis using colored Petri nets[J]. Software & Systems Modeling,2021,18(5).[106]Dipanjan Moitra,Rakesh Kr. Mandal. Automated AJCC (7th edition) staging of non-small cell lung cancer (NSCLC) using deep convolutional neural network (CNN) and recurrent neural network (RNN)[J]. Health Information Science and Systems,2021,7(1).[107]Marta D’Alonzo,Laura Martincich,Agnese Fenoglio,Valentina Giannini,Lisa Cellini,ViolaLiberale,Nicoletta Biglia. Nipple-sparing mastectomy: external validation of a three-dimensional automated method to predict nipple occult tumour involvement on preoperative breast MRI[J]. European Radiology Experimental,2021,3(1).[108]N. V. Dozmorov,A. S. Bogomolov,A. V. Baklanov. An Automated Apparatus for Measuring Spectral Dependences ofthe Mass Spectra and Velocity Map Images of Photofragments[J]. Instruments and Experimental Techniques,2021,62(4).[109]Zhiqiang Sun,Bingzhao Gao,Jiaqi Jin,Kazushi Sanada. Modelling, Analysis and Simulation of a Novel Automated Manual Transmission with Gearshift Assistant Mechanism[J]. International Journal of Automotive Technology,2021,20(5).[110]Andrés Vega,Mariano Córdoba,Mauricio Castro-Franco,Mónica Balzarini. Protocol for automating errorremoval from yield maps[J]. Precision Agriculture,2021,20(5).[111]Bethany L. Lussier,DaiWai M. Olson,Venkatesh Aiyagari. Automated Pupillometry in Neurocritical Care: Research and Practice[J]. Current Neurology and Neuroscience Reports,2021,19(10).[112] B. Haskali,Peter D. Roselt,David Binns,Amit Hetsron,Stan Poniger,Craig A. Hutton,Rodney J. Hicks. Automated preparation of clinical grade [ 68 Ga]Ga-DOTA-CP04, a cholecystokinin-2 receptor agonist, using iPHASE MultiSyn synthesis platform[J]. EJNMMI Radiopharmacy andChemistry,2021,4(1).[113]Ju Hyun Ahn,Minho Na,Sungkwan Koo,HyunsooChun,Inhwan Kim,Jong Won Hur,Jae Hyuk Lee,Jong G. Ok. Development of a fully automated desktop chemical vapor deposition system for programmable and controlled carbon nanotube growth[J]. Micro and Nano Systems Letters,2021,7(1).[114]Kamellia Shahi,Brenda Y. McCabe,Arash Shahi. Framework for Automated Model-Based e-Permitting System forMunicipal Jurisdictions[J]. Journal of Management in Engineering,2021,35(6).[115]Ahmed Khalafallah,Yasmin Shalaby. Change Orders: Automating Comparative Data Analysis and Controlling Impacts in Public Projects[J]. Journal of Construction Engineering and Management,2021,145(11).[116]José ?. Martínez-Huertas,OlgaJastrzebska,Ricardo Olmos,José A. León. Automated summary evaluation with inbuilt rubric method: An alternative to constructed responses and multiple-choice testsassessments[J]. Assessment & Evaluation in Higher Education,2021,44(7).[117]Samsonov,Koshel,Walther,Jenny. Automated placement of supplementary contour lines[J]. International Journal of Geographical Information Science,2021,33(10).[118]Veronika V. Odintsova,Peter J. Roetman,Hill F. Ip,René Pool,Camiel M. Van der Laan,Klodiana-DaphneTona,Robert R.J.M. Vermeiren,Dorret I. Boomsma. Genomics of human aggression: current state of genome-wide studies and an automated systematic review tool[J]. PsychiatricGenetics,2021,29(5).[119]Sebastian Eggert,Dietmar W Hutmacher. In vitro disease models 4.0 via automation and high-throughput processing[J]. Biofabrication,2021,11(4).[120]Asad Mahmood,Faizan Ahmad,Zubair Shafiq,Padmini Srinivasan,Fareed Zaffar. A Girl Has No Name: Automated Authorship Obfuscation using Mutant-X[J]. Proceedings on Privacy Enhancing Technologies,2021,2021(4).。
- 1、下载文档前请自行甄别文档内容的完整性,平台不提供额外的编辑、内容补充、找答案等附加服务。
- 2、"仅部分预览"的文档,不可在线预览部分如存在完整性等问题,可反馈申请退款(可完整预览的文档不适用该条件!)。
- 3、如文档侵犯您的权益,请联系客服反馈,我们会尽快为您处理(人工客服工作时间:9:00-18:30)。
景德镇陶瓷学院毕业设计(论文)有关外文翻译院系:机械电子工程学院专业:自动化姓名:肖骞学号: 201010320116指导教师:万军完成时间: 2014.5.8说明1、将与课题有关的专业外文翻译成中文是毕业设计(论文)中的一个不可缺少的环节。
此环节是培养学生阅读专业外文和检验学生专业外文阅读能力的一个重要环节。
通过此环节进一步提高学生阅读专业外文的能力以及使用外文资料为毕业设计服务,并为今后科研工作打下扎实的基础。
2、要求学生查阅与课题相关的外文文献3篇以上作为课题参考文献,并将其中1篇(不少于3000字)的外文翻译成中文。
中文的排版按后面格式进行填写。
外文内容是否与课题有关由指导教师把关,外文原文附在后面。
3、指导教师应将此外文翻译格式文件电子版拷给所指导的学生,统一按照此排版格式进行填写,完成后打印出来。
4、请将封面、译文与外文原文装订成册。
5、此环节在开题后毕业设计完成前完成。
6、指导教师应从查阅的外文文献与课题紧密相关性、翻译的准确性、是否通顺以及格式是否规范等方面去进行评价。
指导教师评语:签名:年月日TMS320LF2407, TMS320LF2406, TMS320LF2402TMS320LC2406, TMS320LC2404, MS320LC2402DSP CONTROLLERSThe TMS320LF240x and TMS320LC240x devices, new members of the ‘24x family of digital signal processor (DSP) controllers, are part of the C2000 platform of fixed-point DSPs. The ‘240x devices offer the enhanced TMS320 architectural design of the ‘C2xx core CPU for low-cost, low-power, high-performance processing capabilities. Several advanced peripherals, optimized for digital motor and motion control applications, have been integrated to provide a true single chip DSP controller. While code-compatible with the existing ‘24x DSP controller devices, the ‘240x offers increased processing performance (30 MIPS) and a higher level of peripheral integration. See the TMS320x240x device summary section for device-specific features.The ‘240x family offers an array of memory sizes and different peripherals tailored to meet the specific price/performance points required by various applications. Flash-based devices of up to 32K words offer a reprogrammable solution useful for:◆Applications requiring field programmability upgrades.◆Development and initial prototyping of applications that migrate toROM-based devices.Flash devices and corresponding ROM devices are fully pin-to-pin compatible. Note that flash-based devices contain a 256-word boot ROM to facilitate in-circuit programming.All ‘240x devices offer at least one event manager module which has been optimized for digital motor control and power conversion applications. Capabilities of this module include centered- and/or edge-aligned PWM generation, programmable deadband to prevent shoot-through faults, and synchronized analog-to-digital conversion. Devices with dual event managers enable multiple motor and/or convertercontrol with a single ‗240x DSP controller.The high performance, 10-bit analog-to-digital converter (ADC) has a minimum conversion time of 500 ns and offers up to 16 channels of analog input. The auto sequencing capability of the ADC allows a maximum of 16 conversions to take place in a single conversion session without any CPU overhead.A serial communications interface (SCI) is integrated on all devices to provide asynchronous communication to other devices in the system. For systems requiring additional communication interfaces; the ‘2407, ‘2406, and ‘2404 offer a 16-bit synchronous serial peripheral interface (SPI). The ‘2407 and ‘2406 offer a controller area network (CAN) communications module that meets 2.0B specifications. To maximize device flexibility, functional pins are also configurable as general purpose inputs/outputs (GPIO).To streamline development time, JTAG-compliant scan-based emulation has been integrated into all devices. This provides non-intrusive real-time capabilities required to debug digital control systems. A complete suite of code generation tools from C compilers to the industry-standard Code Composerdebugger supports this family. Numerous third party developers not only offer device-level development tools, but also system-level design and development support.PERIPHERALSThe integrated peripherals of the TMS320x240x are described in the following subsections:●Two event-manager modules (EV A, EVB)●Enhanced analog-to-digital converter (ADC) module●Controller area network (CAN) module●Serial communications interface (SCI) module●Serial peripheral interface (SPI) module●PLL-based clock module●Digital I/O and shared pin functions●External memory interfaces (‘LF2407 only)Watchdog (WD) timer moduleEvent manager modules (EV A, EVB)The event-manager modules include general-purpose (GP) timers, full-compare/PWM units, capture units, and quadrature-encoder pulse (QEP) circuits. EV A‘s and EVB‘s timers, compare units, and capture units function identically. However, timer/unit names differ for EV A and EVB. Table 1 shows the module and signal names used. Table 1 shows the features and functionality available for the event-manager modules and highlights EV A nomenclature.Event managers A and B have identical peripheral register sets with EV A starting at 7400h and EVB starting at 7500h. The paragraphs in this section describe the function of GP timers, compare units, capture units, and QEPs using EV A nomenclature. These paragraphs are applicable to EVB with regard to function—however, module/signal names would differ.Table 1. Module and Signal Names for EV A and EVBEVENT MANAGER MODULESEV AMODULESIGNALEVBMODULESIGNALGP Timers Timer 1Timer 2T1PWM/T1CMPT2PWM/T2CMPTimer 3Timer 4T3PWM/T3CMPT4PWM/T4CMPCompare Units Compare 1Compare 2Compare 3PWM1/2PWM3/4PWM5/6Compare 4Compare 5Compare 6PWM7/8PWM9/10PWM11/12Capture Units Capture 1Capture 2Capture 3CAP1CAP2CAP3Capture 4Capture 5Capture 6CAP4CAP5CAP6QEP QEP1QEP2QEP1QEP2QEP3QEP4QEP3QEP4External Inputs DirectionExternalClockTDIRATCLKINADirectionExternal ClockTDIRBTCLKINBGeneral-purpose (GP) timersThere are two GP timers: The GP timer x (x = 1 or 2 for EV A; x = 3 or 4 for EVB) includes:● A 16-bit timer, up-/down-counter, TxCNT, for reads or writes● A 16-bit timer-compare register, TxCMPR (double-buffered with shadowregister), for reads or writes● A 16-bit timer-period register, TxPR (double-buffered with shadowregister), for reads or writes● A 16-bit timer-control register,TxCON, for reads or writes●Selectable internal or external input clocks● A programmable prescaler for internal or external clock inputs●Control and interrupt logic, for four maskable interrupts: underflow,overflow, timer compare, and period interrupts● A selectable direction input pin (TDIR) (to count up or down whendirectional up-/down-count mode is selected)The GP timers can be operated independently or synchronized with each other. The compare register associated with each GP timer can be used for compare function and PWM-waveform generation. There are three continuous modes of operations for each GP timer in up- or up/down-counting operations. Internal or external input clocks with programmable prescaler are used for each GP timer. GP timers also provide the time base for the other event-manager submodules: GP timer 1 for all the compares and PWM circuits, GP timer 2/1 for the capture units and the quadrature-pulse counting operations. Double-buffering of the period and compare registers allows programmable change of the timer (PWM) period and the compare/PWM pulse width as needed.Full-compare unitsThere are three full-compare units on each event manager. These compare units use GP timer1 as the time base and generate six outputs for compare and PWM-waveform generation using programmable deadband circuit. The state of each of the six outputs is configured independently. The compare registers of the compare units are double-buffered, allowing programmable change of the compare/PWM pulse widths as needed.Programmable deadband generatorThe deadband generator circuit includes three 8-bit counters and an 8-bit compare register. Desired deadband values (from 0 to 24 µs) can be programmed into the compare register for the outputs of the three compare units. The deadband generation can be enabled/disabled for each compare unit output individually. The deadband-generator circuit produces two outputs (with or without deadband zone) for each compare unit output signal. The output states of the deadband generator are configurable and changeable as needed by way of the double-buffered ACTR register.PWM waveform generationUp to eight PWM waveforms (outputs) can be generated simultaneously by each event manager: three independent pairs (six outputs) by the three full-compare units with programmable deadbands, and two independent PWMs by the GP-timer compares.PWM characteristicsCharacteristics of the PWMs are as follows:●16-bit registers●Programmable deadband for the PWM output pairs, from 0 to 24 µs●Minimum deadband width of 50 ns●Change of the PWM carrier frequency for PWM frequency wobbling asneeded●Change of the PWM pulse widths within and after each PWM period asneeded●External-maskable power and drive-protection interrupts●Pulse-pattern-generator circuit, for programmable generation of asymmetric,symmetric, and four-space vector PWM waveforms●Minimized CPU overhead using auto-reload of the compare and periodregistersCapture unitThe capture unit provides a logging function for different events or transitions. The values of the GP timer 2 counter are captured and stored in the two-level-deep FIFO stacks when selected transitions are detected on capture input pins, CAPx (x = 1, 2, or 3 for EV A; and x = 4, 5, or 6 for EVB). The capture unit consists of three capture circuits.Capture units include the following features:●One 16-bit capture control register, CAPCON (R/W)●One 16-bit capture FIFO status register, CAPFIFO (eight MSBs areread-only, eight LSBs are write-only)●Selection of GP timer 2 as the time base●Three 16-bit 2-level-deep FIFO stacks, one for each capture unit●Three Schmitt-triggered capture input pins (CAP1, CAP2, and CAP3)—oneinput pin per capture unit. [All inputs are synchronized with the device (CPU)clock. In order for a transition to be captured, the input must hold at itscurrent level to meet two rising edges of the device clock. The input pinsCAP1 and CAP2 can also be used as QEP inputs to the QEP circuit.]●User-specified transition (rising edge, falling edge, or both edges) detection●Three maskable interrupt flags, one for each capture unitEnhanced analog-to-digital converter (ADC) moduleA simplified functional block diagram of the ADC module is shown in Figure 1. The ADC module consists of a 10-bit ADC with a built-in sample-and-hold (S/H) circuit. Functions of the ADC module include:●10-bit ADC core with built-in S/H●Fast conversion time (S/H + Conversion) of 500 ns●16-channel, muxed inputs●Autosequencing capability provides up to 16 ―autoconversions‖ in a singlesession. Each conversion can be programmed to select any 1 of 16 inputchannels●Sequencer can be operated as two independent 8-state sequencers or as onelarge 16-state sequencer (i.e., two cascaded 8-state sequencers)●Sixteen result registers (individually addressable) to store conversion values●Multiple triggers as sources for the start-of-conversion (SOC) sequence✧S/W – software immediate start✧EV A – Event manager A (multiple event sources within EV A)✧EVB – Event manager B (multiple event sources within EVB)✧Ext – External pin (ADCSOC)●Flexible interrupt control allows interrupt request on every end of sequence(EOS) or every other EOS●Sequencer can operate in ―start/stop‖ mode, allowing multiple―time-sequenced triggers‖ to synchronize conversions●EV A and EVB triggers can operate independently in dual-sequencer mode●Sample-and-hold (S/H) acquisition time window has separate prescalecontrol●Built-in calibration mode●Built-in self-test modeThe ADC module in the ‘240x has been enhanced to provide flexible interface to event managers A and B. The ADC interface is built around a fast, 10-bit ADC module with total conversion time of 500 ns (S/H + conversion). The ADC module has 16 channels, configurable as two independent 8-channel modules to service event managers A and B. The two independent 8-channel modules can be cascaded to form a 16-channel module. Figure 2 shows the block diagram of the ‘240x ADC module.The two 8-channel modules have the capability to autosequence a series of conversions, each module has the choice of selecting any one of the respective eight channels available through an analog mux. In the cascaded mode, the autosequencer functions as a single 16-channel sequencer. On each sequencer, once the conversion is complete, the selected channel value is stored in its respective RESULT register. Autosequencing allows the system to convert the same channel multiple times, allowing the user to perform oversampling algorithms. This gives increased resolution over traditional single-sampled conversion results.From TMS320LF2407, TMS320LF2406, TMS320LF2402TMS320LC2406, TMS320LC2404, MS320LC2402数字信号处理控制器TMS320LF240x和TMS320LC240x系列芯片作为’24x系列DSP控制器的新成员,是C2000平台下的一种定点DSP芯片。